-
Notifications
You must be signed in to change notification settings - Fork 49
v4 create command
Inhere edited this page May 29, 2022
·
9 revisions
新版本命令更加的灵活和易用
- 支持通过注释编写选项、参数绑定
- 编写规则简单,功能更强
- 支持设置选项、参数的数据类型
- 选项支持设置多个别名 eg:
-n, --nm, --no-merges
- 命令也支持添加子命令,且不限制层级
先看一份命令代码示例,来自我的工具应用项目 inhere/kite
<?php declare(strict_types=1);
/**
* This file is part of Kite.
*
* @link https://github.com/inhere/kite
* @author https://github.com/inhere
* @license MIT
*/
namespace Inhere\Kite\Console\Command;
use Inhere\Console\Command;
use Inhere\Console\IO\Input;
use Inhere\Console\IO\Output;
use Toolkit\Stdlib\OS;
use function is_scalar;
/**
* Class DemoCommand
*/
class EnvCommand extends Command
{
protected static string $name = 'env';
protected static string $desc = 'print system ENV information';
/**
* print system ENV information
*
* @options
* --format Format the env value
* --match-value bool;Match ENV value by keywords. default is match key.
* --split Split the env value by given char. eg ':' ','
* -s, --search The keywords for search ENV information
*
* @arguments
* name The name in the ENV or keywords for search ENV keys
*
* @param Input $input
* @param Output $output
*/
protected function execute(Input $input, Output $output)
{
$keywords = $this->flags->getOpt('search');
$name = $this->flags->getFirstArg();
if (!$name && !$keywords) {
// env | grep XXX
$output->aList($_SERVER, 'ENV Information', ['ucFirst' => false]);
return;
}
$output->info("do something ...");
}
}
TIP: 创建好命令后需要注册到 Application, 请看 注册命令 章节
- 必须继承基础类
Inhere\Console\Command
- 必须实现父类的抽象方法
execute(Input $input, Output $output)
- 命令的主体逻辑就在这个方法编写
-
$name
命令名称,必须且不能为空 -
$desc
命令描述说明
命令渲染效果 kite env -h
:
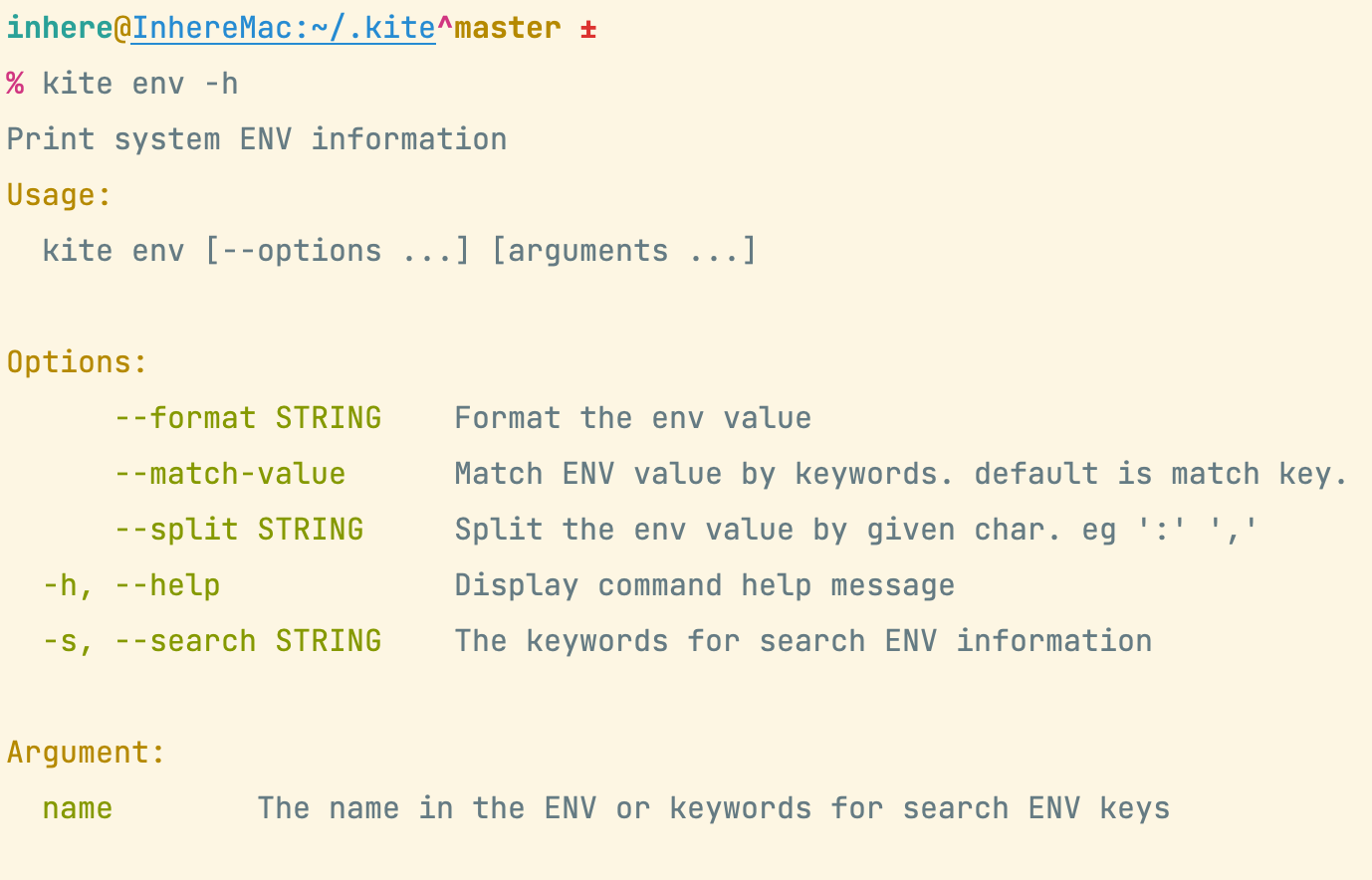
注意运行命令应当遵循通用的Linux命令调用格式:
ENTRY CMD --OPTIONS ARGUMENTS
可以观察到上面示例的 execute
方法注释是有一定格式的。
命令可以通过方法注释快速绑定命令选项和参数,运行时console会自动解析并绑定到当前命令
-
@arguments
后面的即是命令参数 -
@options
后面的即是命令选项- 注意选项名和后面的设置描述需间隔一定距离
- 规则以分号
;
分割每个部分 (完整规则:type;desc;required;default;shorts
) - 默认是 string 类型,可以忽略
选项参数解析使用的 php-toolkit/pflag 更多说明可以点击查看
除了使用注释快速的绑定选项、参数,也可以使用 FlagsParser
来绑定命令的选项、参数
/**
* @param FlagsParser $fs
*
* @return void
*/
protected function configFlags(FlagsParser $fs): void
{
// 绑定选项
$fs->addOptByRule('update, up', 'bool;update linux command docs to latest');
$fs->addOptByRule('init, i', 'bool;update linux command docs to latest');
$fs->addOptByRule('search, s', 'string;input keywords for search');
// 绑定参数
// - 这里没有设置必须 可以不传,获取到就是空string
$fs->addArg('keywords', 'the keywords for search or show docs', 'string');
}
通过 aliases()
方法可以设置当前命令的别名
/**
* @return string[]
*/
public static function aliases(): array
{
return ['md', 'mkdown'];
}
设置别名后,通过别名也可以访问执行命令。如下 kite md
:
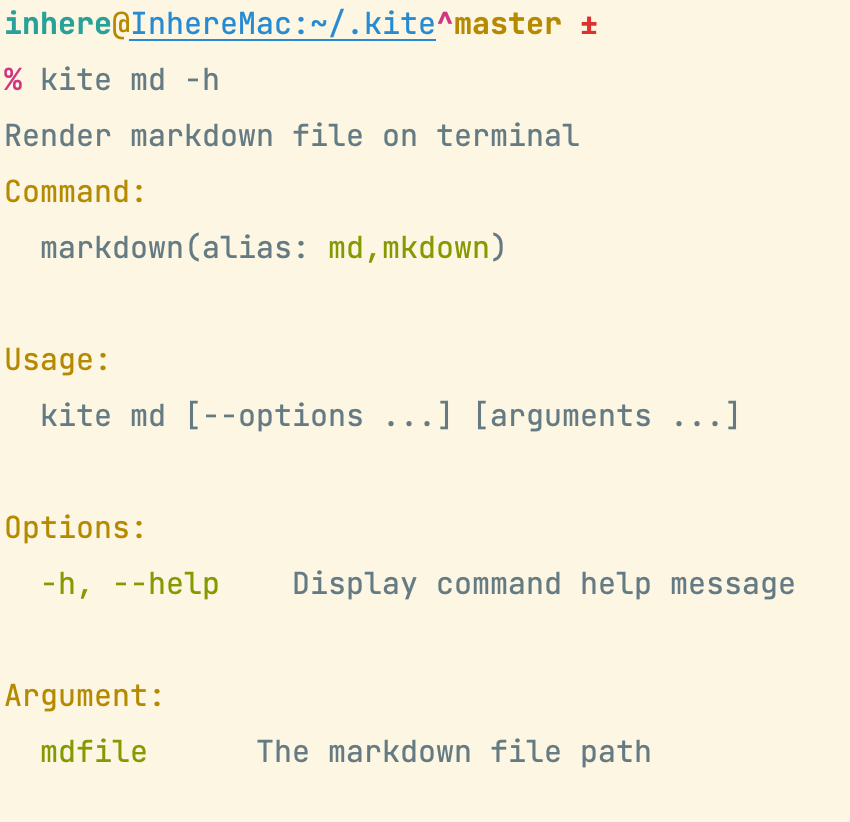
v4 版本之后,独立命令也可以通过 subCommands
绑定子级命令,并且支持多个层级。可以实现类似于 git remote add
这样的多层级命令
protected function subCommands(): array
{
return [
OpenCmd::class,
LnCommand::class,
];
}
查看效果:
来自 inhere/kite:
kite tool -h
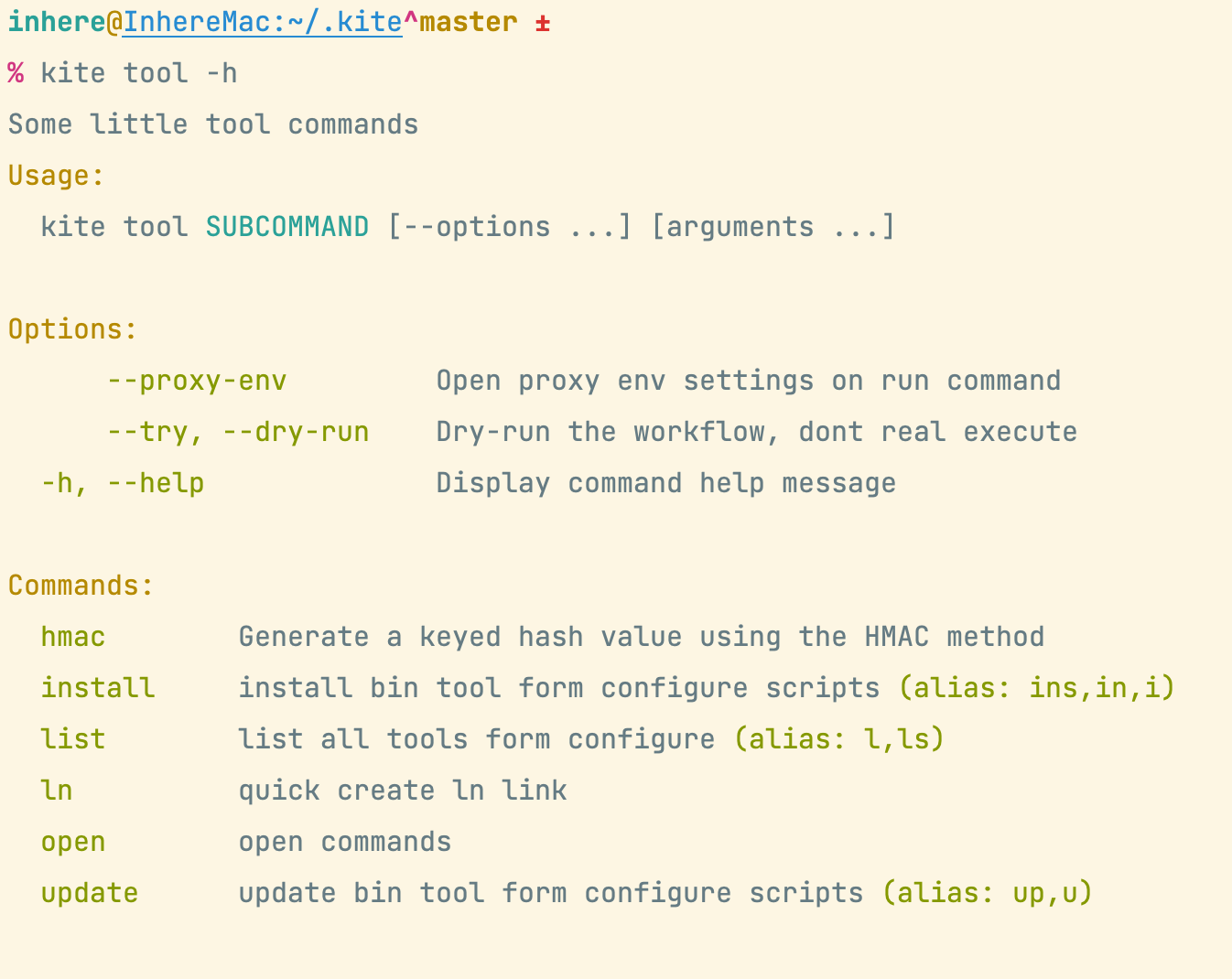
-
public static function isEnabled(): bool
使用此方法,可以禁用当前命令 -
protected function configFlags(FlagsParser $fs): void
使用此方法,通过FlagsParser
对象来配置命令的选项和参数 -
protected function annotationVars(): array
继承此方法可以添加自定义的帮助信息变量,可以在命令注释里使用 -
protected function beforeExecute(): bool
将会在命令执行前调用 - 更多请查看命令的父类
Inhere\Console\Handler\AbstractHandler
拥有的方法
我的其他PHP项目
- inhere/kite 方便本地开发和使用的个人CLI工具应用
- php-toolkit/pflag PHP编写的,通用的命令行标志(选项和参数)解析库
- phppkg/easytpl 使用简单且快速的 PHP 模板引擎
- inhere/php-validate 一个简洁小巧且功能完善的php验证库
- inhere/sroute 轻量且快速的HTTP请求路由库