-
Notifications
You must be signed in to change notification settings - Fork 0
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Basic flow editor and connect dots tool #179
Draft
MichaelTamaki
wants to merge
1
commit into
main
Choose a base branch
from
issue-96-flow-editor
base: main
Could not load branches
Branch not found: {{ refName }}
Loading
Could not load tags
Nothing to show
Loading
Are you sure you want to change the base?
Some commits from the old base branch may be removed from the timeline,
and old review comments may become outdated.
Draft
Changes from all commits
Commits
File filter
Filter by extension
Conversations
Failed to load comments.
Loading
Jump to
Jump to file
Failed to load files.
Loading
Diff view
Diff view
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,47 @@ | ||
<template> | ||
<g v-if="isViewModeFlow"> | ||
<g v-for="cachedFlow in selectedDotsFlows" :key="cachedFlow"> | ||
<polyline | ||
class="grapher--flow-line" | ||
v-if="cachedFlow && cachedFlow.length > 1" | ||
:points=" | ||
cachedFlow.map((flowBeat) => `${flowBeat.x},${flowBeat.y}`).join(` `) | ||
" | ||
/> | ||
</g> | ||
</g> | ||
</template> | ||
|
||
<script lang="ts"> | ||
import { FlowBeat } from "@/models/util/FlowBeat"; | ||
import { CalChartState } from "@/store"; | ||
import { VIEW_MODES } from "@/store/constants"; | ||
import Vue from "vue"; | ||
|
||
/** | ||
* Renders each selected dots' cachedFlow. | ||
*/ | ||
export default Vue.extend({ | ||
name: "GrapherFlow", | ||
computed: { | ||
isViewModeFlow(): boolean { | ||
return this.$store.state.viewMode === VIEW_MODES.FLOW; | ||
}, | ||
selectedDotsFlows(): (FlowBeat[] | null)[] { | ||
const { selectedDotIds, show, selectedSS } = this.$store | ||
.state as CalChartState; | ||
return show.stuntSheets[selectedSS].stuntSheetDots | ||
.filter((dot) => selectedDotIds.includes(dot.id)) | ||
.map((dot) => dot.cachedFlow); | ||
}, | ||
}, | ||
}); | ||
</script> | ||
|
||
<style scoped lang="scss"> | ||
.grapher--flow-line { | ||
fill: none; | ||
stroke: $founders-rock; | ||
There was a problem hiding this comment. Choose a reason for hiding this commentThe reason will be displayed to describe this comment to others. Learn more. 🐻 |
||
stroke-width: 0.75; | ||
} | ||
</style> |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,39 @@ | ||
<template> | ||
<div> | ||
<b-field label="View Mode"> | ||
There was a problem hiding this comment. Choose a reason for hiding this commentThe reason will be displayed to describe this comment to others. Learn more. What if we put "View Mode" under the view menu bar? |
||
<b-select v-model="viewMode"> | ||
<option | ||
v-for="viewModeOption in viewModeOptions" | ||
:key="viewModeOption" | ||
:value="viewModeOption" | ||
> | ||
{{ viewModeOption }} | ||
</option> | ||
</b-select> | ||
</b-field> | ||
</div> | ||
</template> | ||
|
||
<script lang="ts"> | ||
import { CalChartState } from "@/store"; | ||
import { VIEW_MODES } from "@/store/constants"; | ||
import { Mutations } from "@/store/mutations"; | ||
import Vue from "vue"; | ||
|
||
export default Vue.extend({ | ||
name: "ViewModeForm", | ||
computed: { | ||
viewMode: { | ||
get(): VIEW_MODES { | ||
return (this.$store.state as CalChartState).viewMode; | ||
}, | ||
set(viewModeOption: VIEW_MODES): void { | ||
this.$store.commit(Mutations.SET_VIEW_MODE, viewModeOption); | ||
}, | ||
}, | ||
viewModeOptions(): string[] { | ||
return Object.values(VIEW_MODES); | ||
}, | ||
}, | ||
}); | ||
</script> |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,11 @@ | ||
/** | ||
* Defines the different ways that the data can be viewed and interacted with in the UI | ||
*/ | ||
export enum VIEW_MODES { | ||
// "Stuntsheet" mode is for viewing and updating a specific stuntsheet. | ||
// Used for drawing and editing formations. | ||
STUNTSHEET = "Stuntsheet", | ||
// "Flow" mode is for viewing and updating the transition between two stuntsheets. | ||
// Used for creating and editing continuities. | ||
FLOW = "Flow", | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Oops, something went wrong.
Add this suggestion to a batch that can be applied as a single commit.
This suggestion is invalid because no changes were made to the code.
Suggestions cannot be applied while the pull request is closed.
Suggestions cannot be applied while viewing a subset of changes.
Only one suggestion per line can be applied in a batch.
Add this suggestion to a batch that can be applied as a single commit.
Applying suggestions on deleted lines is not supported.
You must change the existing code in this line in order to create a valid suggestion.
Outdated suggestions cannot be applied.
This suggestion has been applied or marked resolved.
Suggestions cannot be applied from pending reviews.
Suggestions cannot be applied on multi-line comments.
Suggestions cannot be applied while the pull request is queued to merge.
Suggestion cannot be applied right now. Please check back later.
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
Maybe we should have the appearance of the connected dots mimic the starting dot's appearance? It might make situations like this where multiple different dot types have paths that overlap a bit clearer:
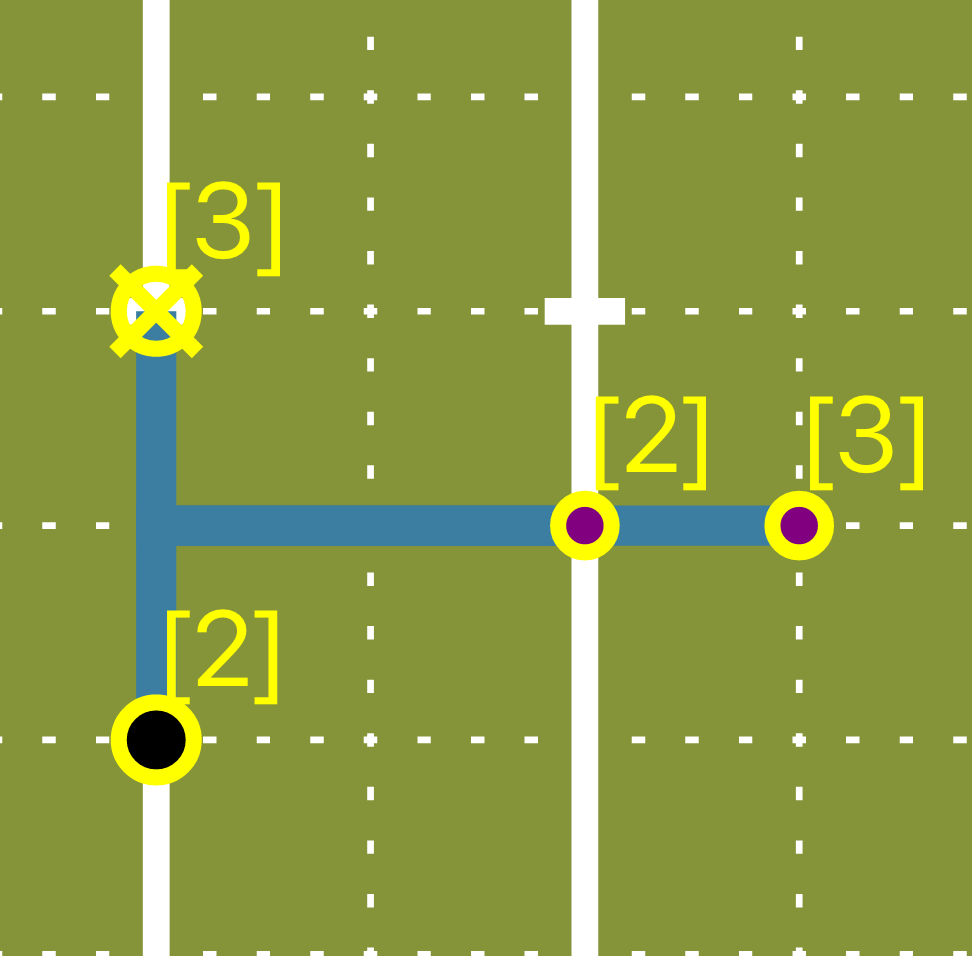
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
Good idea... Let's re-visit this once we get this in the hands of STUNT.