-
Notifications
You must be signed in to change notification settings - Fork 0
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
add new function , change composer, fix testcase
- Loading branch information
Showing
5 changed files
with
427 additions
and
95 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1,10 +1,17 @@ | ||
# Laravel API Response Formatter | ||
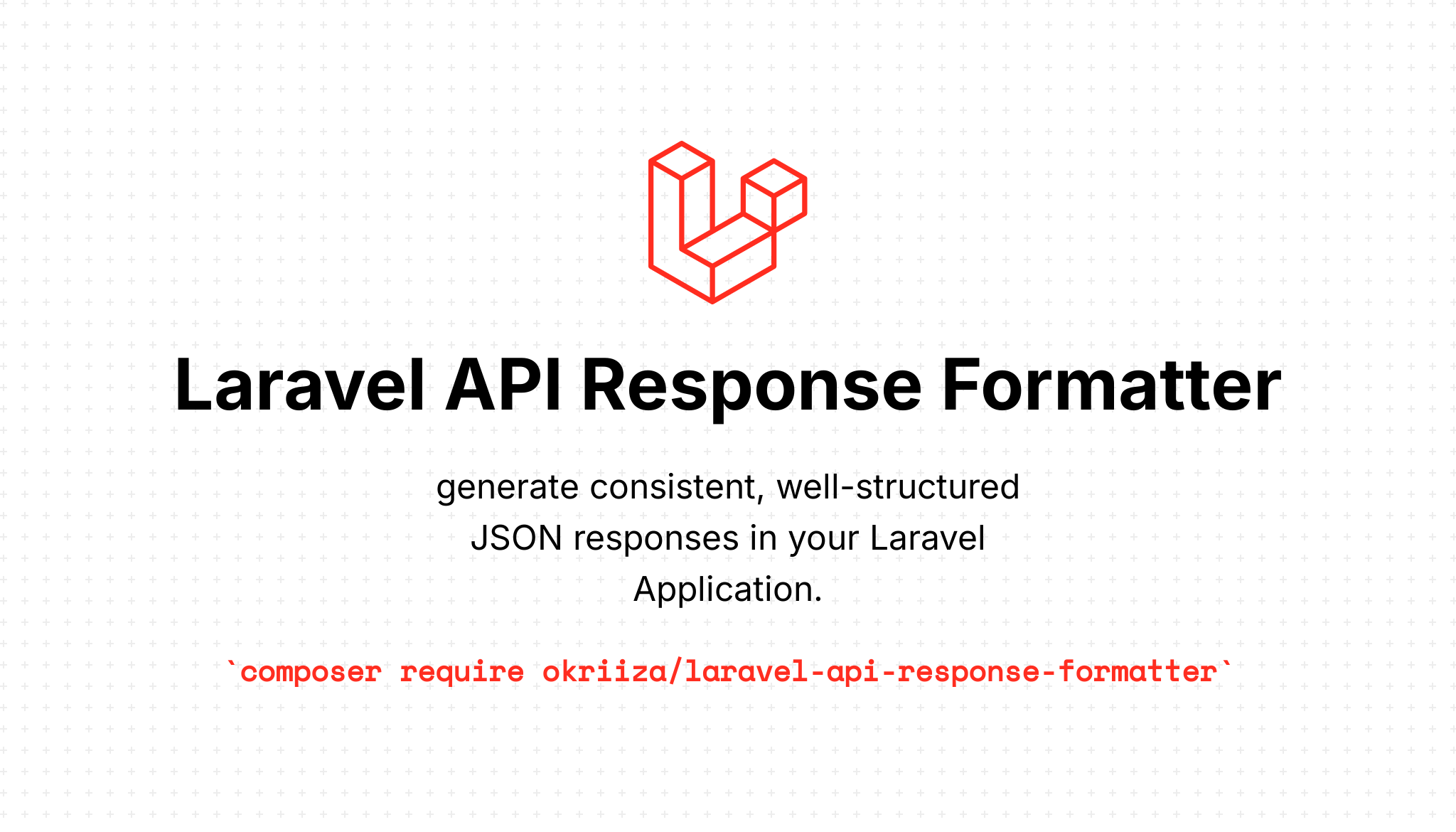 | ||
|
||
[](https://packagist.org/packages/okriiza/laravel-api-response-formatter) | ||
[](https://packagist.org/packages/okriiza/laravel-api-response-formatter) | ||
[](https://opensource.org/licenses/MIT) | ||
|
||
a simple package Format API responses throughout your Laravel application | ||
# Laravel API Response Formatter | ||
|
||
`Laravel API Response Formatter` is a class that provides methods for formatting API responses in a standardized format. It simplifies the process of creating consistent and well-structured JSON responses in your API. | ||
|
||
## Requirements | ||
|
||
- PHP `^7.4 | ^8.0` | ||
- Laravel 6, 7, 8, 9 or 10 | ||
|
||
## Installation | ||
|
||
|
@@ -16,49 +23,101 @@ composer require okriiza/laravel-api-response-formatter | |
|
||
The package will automatically register itself. | ||
|
||
## Usage | ||
## Function List | ||
|
||
To use the package, simply call the success or error method from the ApiResponseFormatter class. Here's an example of how to use it: | ||
The `Laravel API Response Formatter` class provides the following functions: | ||
|
||
```php | ||
use Okriiza\ApiResponseFormatter\ApiResponseFormatter; | ||
``` | ||
| Function | Description | | ||
| -------------------- | ----------------------------------------------------------------------------------------- | | ||
| `success()` | Formats a success response with optional data, message, status, and HTTP code. | | ||
| `created()` | Formats a created response with optional data, message, status, and HTTP code. | | ||
| `noContent()` | Formats a no content response with optional data, message, status, and HTTP code. | | ||
| `error()` | Formats an error response with optional data, message, status, and HTTP code. | | ||
| `unAuthenticated()` | Formats an unauthenticated response with optional data, message, status, and HTTP code. | | ||
| `forbidden()` | Formats a forbidden response with optional data, message, status, and HTTP code. | | ||
| `notFound()` | Formats a not found response with optional data, message, status, and HTTP code. | | ||
| `methodNotAllowed()` | Formats a method not allowed response with optional data, message, status, and HTTP code. | | ||
| `failedValidation()` | Formats a failed validation response with optional data, message, status, and HTTP code. | | ||
|
||
And use it like this: | ||
## Parameters | ||
|
||
The functions in the `Laravel API Response Formatter` class accept the following parameters: | ||
|
||
- `$data` (optional): The data to be included in the response. It can be of any type. | ||
- `$message` (optional): The message to be included in the response. If not provided, a default message will be used. | ||
- `$status` (optional): The success status of the response. Defaults to `true` for success responses and `false` for error responses. | ||
- `$httpCode` (optional): The HTTP response code to be returned. It defaults to the corresponding HTTP status code for each response type. | ||
|
||
## Example Usage | ||
|
||
Here's an example of how you can use the `Laravel API Response Formatter` class in a user controller: | ||
|
||
```php | ||
<?php | ||
|
||
use Okriiza\ApiResponseFormatter\ApiResponseFormatter; | ||
|
||
// Example success response | ||
$user = User::find(1); | ||
return ApiResponseFormatter::success($user, 'User found'); | ||
class UserController extends Controller | ||
{ | ||
public function show($id): JsonResponse | ||
{ | ||
$user = User::find($id); | ||
|
||
if ($user) { | ||
return ApiResponseFormatter::success($user); | ||
} else { | ||
return ApiResponseFormatter::notFound(null, 'User not found'); | ||
} | ||
} | ||
|
||
public function create(Request $request): JsonResponse | ||
{ | ||
// Validation logic | ||
|
||
// Example error response | ||
return ApiResponseFormatter::error('User not found', 404); | ||
if ($validationFails) { | ||
return ApiResponseFormatter::failedValidation($validationErrors); | ||
} | ||
|
||
$user = User::create($request->all()); | ||
|
||
return ApiResponseFormatter::created($user); | ||
} | ||
} | ||
``` | ||
|
||
By default, the package returns a JSON response with the following format: | ||
In the above example, the `show()` method fetches a user by ID and returns a success response if the user exists. If the user is not found, it returns a not found response. The `create()` method performs validation and creates a new user. If the validation fails, it returns a failed validation response. Otherwise, it returns a created response with the created user. | ||
|
||
```php | ||
```json | ||
{ | ||
"meta": { | ||
"code": 200, | ||
"status": true, | ||
"message": null | ||
"success": true, | ||
"message": "OK" | ||
}, | ||
"result": null | ||
"result": { | ||
"id": 1, | ||
"name": "John Doe", | ||
"email": "[email protected]" | ||
} | ||
} | ||
``` | ||
|
||
You can customize the response message, status, and code by passing the appropriate arguments to the success or error method. | ||
And for an error case: | ||
|
||
### Testing | ||
```json | ||
{ | ||
"meta": { | ||
"code": 404, | ||
"success": false, | ||
"message": "User not found" | ||
}, | ||
"result": null | ||
} | ||
``` | ||
|
||
You can run the tests using PHPUnit. Simply run the following command in the package directory: | ||
The `meta` object contains information about the response, such as the response code, status, and message. The `result` object holds the actual response data. | ||
|
||
```bash | ||
vendor/bin/phpunit | ||
``` | ||
Note: The examples provided are simplified and may require modifications to fit your specific use case | ||
|
||
## Contributing | ||
|
||
|
@@ -72,40 +131,8 @@ If you discover any security related issues, please email [email protected] ins | |
|
||
This package was created by [Rendi Okriza](https://github.com/okriiza) | ||
|
||
- [All Contributors](../../contributors) | ||
- [All Contributors](../../contributors) | ||
|
||
## License | ||
|
||
The MIT License (MIT). Please see [License File](LICENSE.md) for more information. | ||
|
||
And here's an example code: | ||
|
||
```php | ||
<?php | ||
|
||
namespace App\Http\Controllers; | ||
|
||
use App\Http\Controllers\Controller; | ||
use App\Models\User; | ||
use Okriiza\ApiResponseFormatter\ApiResponseFormatter; | ||
|
||
class UserController extends Controller | ||
{ | ||
public function index() | ||
{ | ||
$users = User::all(); | ||
return ApiResponseFormatter::success($users, 'All users fetched successfully'); | ||
} | ||
|
||
public function show($id) | ||
{ | ||
$user = User::find($id); | ||
|
||
if (!$user) { | ||
return ApiResponseFormatter::error('User not found', 404); | ||
} | ||
|
||
return ApiResponseFormatter::success($user, 'User fetched successfully'); | ||
} | ||
} | ||
``` | ||
The Laravel API Response Formatter package is open-sourced software licensed under the [MIT license](https://opensource.org/licenses/MIT). |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Oops, something went wrong.