-
-
Notifications
You must be signed in to change notification settings - Fork 232
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
Tables Feature - Added the `create_table` method, which returns a `Table` object. This can be used to display watchlists, order windows, position windows and more. - See the new page on the docs for more information! Bugs - Fixed a bug preventing the named column of a line to not work as a label of the series. - Fixed a bug causing drawings loaded from the minute timeframe to not show on a daily timeframe. - Fixed a bug causing `chart.exit` to not work. - Fixed a bug preventing the chart from being moved after placing a ray. - Fixed the ‘price in hoveringOver’ web console error. Enhancements - The date/time column can also be the `name` of the passed series object. - Added the `label` method to `HorizontalLine`, allowing for the price line label of horizontal lines to be updated. - `None` or an empty DataFrame can now be passed to `line.set` as a means to clear it. - Seperate Chart objects will now run on the same pywebview instance. This means that any Chart objects created after the first will inherit the first Chart’s API. - Reorganized the documentation for clarity.
- Loading branch information
Showing
20 changed files
with
1,290 additions
and
872 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
|
@@ -5,7 +5,7 @@ | |
[](https://pypi.org/project/lightweight-charts/) | ||
[](https://python.org "Go to Python homepage") | ||
[](https://github.com/louisnw01/lightweight-charts-python/blob/main/LICENSE) | ||
[](https://lightweight-charts-python.readthedocs.io/en/latest/docs.html) | ||
[](https://lightweight-charts-python.readthedocs.io/en/latest/common_methods.html) | ||
|
||
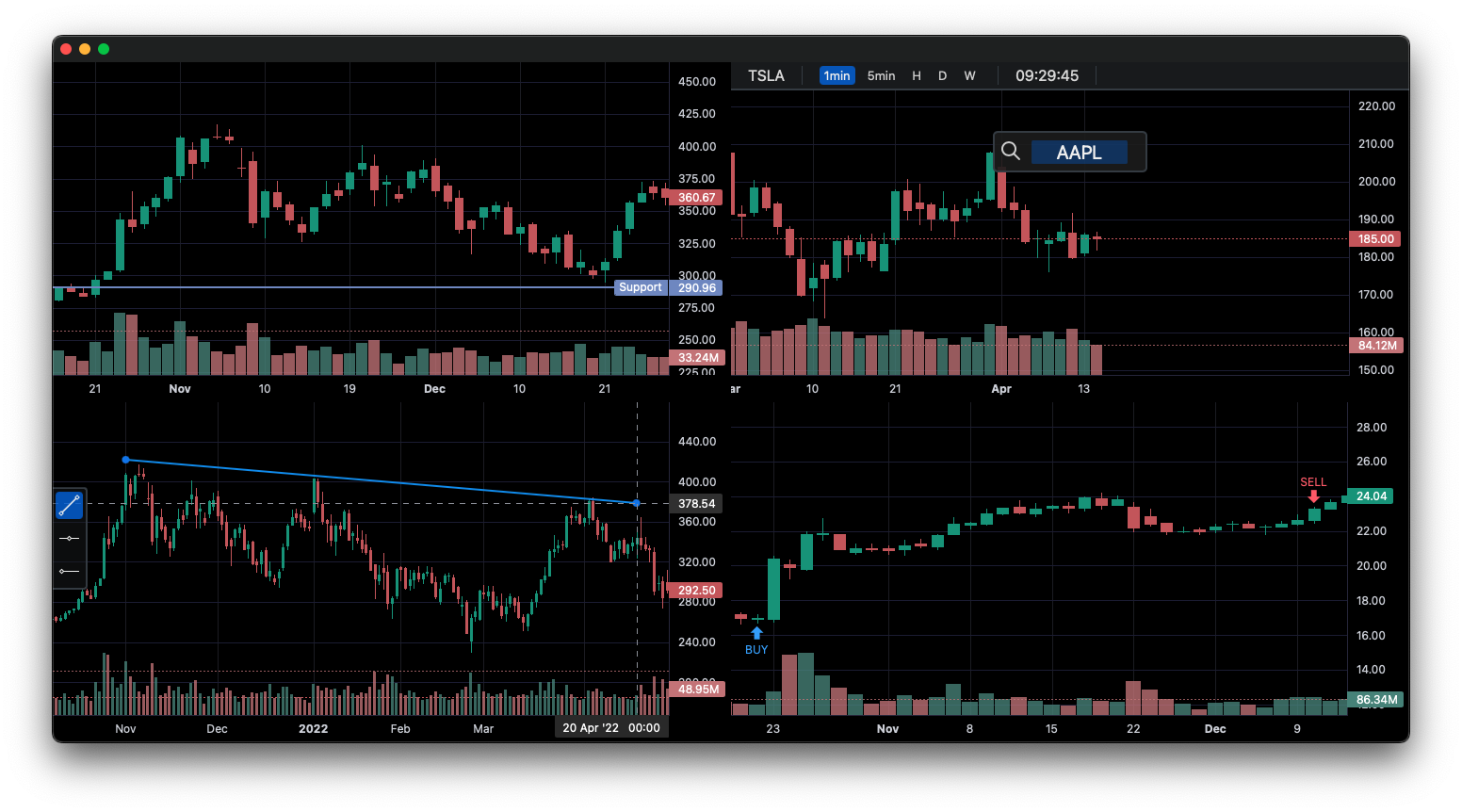 | ||
|
||
|
@@ -24,9 +24,10 @@ ___ | |
1. Simple and easy to use. | ||
2. Blocking or non-blocking GUI. | ||
3. Streamlined for live data, with methods for updating directly from tick data. | ||
4. Multi-Pane Charts using the [`SubChart`](https://lightweight-charts-python.readthedocs.io/en/latest/docs.html#subchart). | ||
4. Multi-Pane Charts using the [`SubChart`](https://lightweight-charts-python.readthedocs.io/en/latest/common_methods.html#create-subchart-subchart). | ||
5. The Toolbox, allowing for trendlines, rays and horizontal lines to be drawn directly onto charts. | ||
6. [Callbacks](https://lightweight-charts-python.readthedocs.io/en/latest/docs.html#callbacks) allowing for timeframe (1min, 5min, 30min etc.) selectors, searching, and more. | ||
6. [Callbacks](https://lightweight-charts-python.readthedocs.io/en/latest/callbacks.html) allowing for timeframe (1min, 5min, 30min etc.) selectors, searching, hotkeys, and more. | ||
7. Tables for watchlists, order entry, and trade management. | ||
7. Direct integration of market data through [Polygon.io's](https://polygon.io/?utm_source=affiliate&utm_campaign=pythonlwcharts) market data API. | ||
|
||
__Supports:__ Jupyter Notebooks, PyQt, wxPython, Streamlit, and asyncio. | ||
|
@@ -194,67 +195,61 @@ ___ | |
### 6. Callbacks: | ||
|
||
```python | ||
import asyncio | ||
import pandas as pd | ||
|
||
from lightweight_charts import Chart | ||
|
||
|
||
def get_bar_data(symbol, timeframe): | ||
if symbol not in ('AAPL', 'GOOGL', 'TSLA'): | ||
print(f'No data for "{symbol}"') | ||
return pd.DataFrame() | ||
return pd.read_csv(f'bar_data/{symbol}_{timeframe}.csv') | ||
return pd.read_csv(f'../examples/6_callbacks/bar_data/{symbol}_{timeframe}.csv') | ||
|
||
|
||
class API: | ||
def __init__(self): | ||
self.chart = None # Changes after each callback. | ||
|
||
async def on_search(self, searched_string): # Called when the user searches. | ||
def on_search(self, searched_string): # Called when the user searches. | ||
new_data = get_bar_data(searched_string, self.chart.topbar['timeframe'].value) | ||
if new_data.empty: | ||
return | ||
self.chart.topbar['corner'].set(searched_string) | ||
self.chart.topbar['symbol'].set(searched_string) | ||
self.chart.set(new_data) | ||
|
||
async def on_timeframe_selection(self): # Called when the user changes the timeframe. | ||
new_data = get_bar_data(self.chart.topbar['corner'].value, self.chart.topbar['timeframe'].value) | ||
def on_timeframe_selection(self): # Called when the user changes the timeframe. | ||
new_data = get_bar_data(self.chart.topbar['symbol'].value, self.chart.topbar['timeframe'].value) | ||
if new_data.empty: | ||
return | ||
self.chart.set(new_data) | ||
async def on_horizontal_line_move(self, line_id, price): | ||
self.chart.set(new_data, True) | ||
|
||
def on_horizontal_line_move(self, line_id, price): | ||
print(f'Horizontal line moved to: {price}') | ||
|
||
|
||
async def main(): | ||
if __name__ == '__main__': | ||
api = API() | ||
|
||
chart = Chart(api=api, topbar=True, searchbox=True, toolbox=True) | ||
chart.legend(True) | ||
|
||
chart.topbar.textbox('corner', 'TSLA') | ||
chart.topbar.textbox('symbol', 'TSLA') | ||
chart.topbar.switcher('timeframe', api.on_timeframe_selection, '1min', '5min', '30min', default='5min') | ||
|
||
df = get_bar_data('TSLA', '5min') | ||
chart.set(df) | ||
|
||
chart.horizontal_line(200, interactive=True) | ||
|
||
await chart.show_async(block=True) | ||
|
||
chart.horizontal_line(200, interactive=True) | ||
|
||
if __name__ == '__main__': | ||
asyncio.run(main()) | ||
chart.show(block=True) | ||
|
||
``` | ||
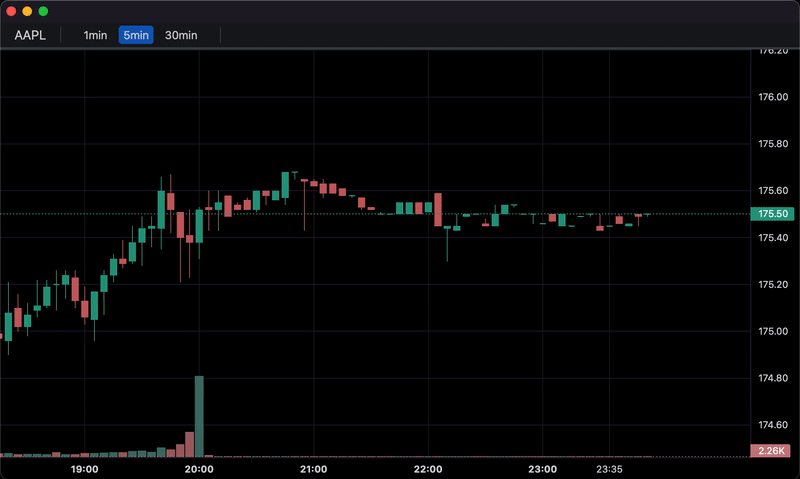 | ||
___ | ||
|
||
<div align="center"> | ||
|
||
[](https://lightweight-charts-python.readthedocs.io/en/latest/docs.html) | ||
[](https://lightweight-charts-python.readthedocs.io/en/latest/common_methods.html) | ||
|
||
Inquiries: [[email protected]](mailto:[email protected]) | ||
___ | ||
|
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,132 @@ | ||
# Callbacks | ||
|
||
The `Chart` object allows for asynchronous and synchronous callbacks to be passed back to python, allowing for more sophisticated chart layouts including searching, timeframe selectors text boxes, and hotkeys using the `add_hotkey` method. | ||
|
||
`QtChart`and `WxChart` can also use callbacks. | ||
|
||
A variety of the parameters below should be passed to the Chart upon decaration. | ||
* `api`: The class object that the fixed callbacks will always be emitted to. | ||
* `topbar`: Adds a [TopBar](#topbar) to the `Chart` or `SubChart` and allows use of the `create_switcher` method. | ||
* `searchbox`: Adds a search box onto the `Chart` or `SubChart` that is activated by typing. | ||
|
||
___ | ||
## How to use Callbacks | ||
|
||
Fixed Callbacks are emitted to the class given as the `api` parameter shown above. | ||
|
||
Take a look at this minimal example: | ||
|
||
```python | ||
class API: | ||
def __init__(self): | ||
self.chart = None | ||
|
||
def on_search(self, string): | ||
print(f'Search Text: "{string}" | Chart/SubChart ID: "{self.chart.id}"') | ||
``` | ||
Upon searching in a pane, the expected output would be akin to: | ||
``` | ||
Search Text: "AAPL" | Chart/SubChart ID: "window.blyjagcr" | ||
``` | ||
The ID shown above will change depending upon which pane was used to search, due to the instance of `self.chart` dynamically updating to the latest pane which triggered the callback. | ||
`self.chart` will update upon each callback, allowing for access to the specific pane in question. | ||
|
||
```{important} | ||
* When using `show` rather than `show_async`, block should be set to `True` (`chart.show(block=True)`). | ||
* `API` class methods can be either coroutines or normal methods. | ||
* Non fixed callbacks (switchers, hotkeys) can be methods, coroutines, or regular functions. | ||
``` | ||
|
||
There are certain callbacks which are always emitted to a specifically named method of API: | ||
* Search callbacks: `on_search` | ||
* Interactive Horizontal Line callbacks: `on_horizontal_line_move` | ||
|
||
___ | ||
|
||
## `TopBar` | ||
The `TopBar` class represents the top bar shown on the chart when using callbacks: | ||
|
||
 | ||
|
||
This class is accessed from the `topbar` attribute of the chart object (`chart.topbar.<method>`), after setting the topbar parameter to `True` upon declaration of the chart. | ||
|
||
Switchers and text boxes can be created within the top bar, and their instances can be accessed through the `topbar` dictionary. For example: | ||
|
||
```python | ||
chart = Chart(api=api, topbar=True) | ||
|
||
chart.topbar.textbox('symbol', 'AAPL') # Declares a textbox displaying 'AAPL'. | ||
print(chart.topbar['symbol'].value) # Prints the value within ('AAPL') | ||
|
||
chart.topbar['symbol'].set('MSFT') # Sets the 'symbol' textbox to 'MSFT' | ||
print(chart.topbar['symbol'].value) # Prints the value again ('MSFT') | ||
``` | ||
___ | ||
|
||
### `switcher` | ||
`name: str` | `method: function` | `*options: str` | `default: str` | ||
|
||
* `name`: the name of the switcher which can be used to access it from the `topbar` dictionary. | ||
* `method`: The function from the `api` class given to the constructor that will receive the callback. | ||
* `options`: The strings to be displayed within the switcher. This may be a variety of timeframes, security types, or whatever needs to be updated directly from the chart. | ||
* `default`: The initial switcher option set. | ||
___ | ||
|
||
### `textbox` | ||
`name: str` | `initial_text: str` | ||
|
||
* `name`: the name of the text box which can be used to access it from the `topbar` dictionary. | ||
* `initial_text`: The text to show within the text box. | ||
___ | ||
|
||
## Callbacks Example: | ||
|
||
```python | ||
import pandas as pd | ||
from lightweight_charts import Chart | ||
|
||
|
||
def get_bar_data(symbol, timeframe): | ||
if symbol not in ('AAPL', 'GOOGL', 'TSLA'): | ||
print(f'No data for "{symbol}"') | ||
return pd.DataFrame() | ||
return pd.read_csv(f'../examples/6_callbacks/bar_data/{symbol}_{timeframe}.csv') | ||
|
||
|
||
class API: | ||
def __init__(self): | ||
self.chart = None # Changes after each callback. | ||
|
||
def on_search(self, searched_string): # Called when the user searches. | ||
new_data = get_bar_data(searched_string, self.chart.topbar['timeframe'].value) | ||
if new_data.empty: | ||
return | ||
self.chart.topbar['symbol'].set(searched_string) | ||
self.chart.set(new_data) | ||
|
||
def on_timeframe_selection(self): # Called when the user changes the timeframe. | ||
new_data = get_bar_data(self.chart.topbar['symbol'].value, self.chart.topbar['timeframe'].value) | ||
if new_data.empty: | ||
return | ||
self.chart.set(new_data, True) | ||
|
||
def on_horizontal_line_move(self, line_id, price): | ||
print(f'Horizontal line moved to: {price}') | ||
|
||
|
||
if __name__ == '__main__': | ||
api = API() | ||
|
||
chart = Chart(api=api, topbar=True, searchbox=True, toolbox=True) | ||
chart.legend(True) | ||
|
||
chart.topbar.textbox('symbol', 'TSLA') | ||
chart.topbar.switcher('timeframe', api.on_timeframe_selection, '1min', '5min', '30min', default='5min') | ||
|
||
df = get_bar_data('TSLA', '5min') | ||
chart.set(df) | ||
|
||
chart.horizontal_line(200, interactive=True) | ||
|
||
chart.show(block=True) | ||
``` |
Oops, something went wrong.