-
Notifications
You must be signed in to change notification settings - Fork 1
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
[4주차 기본/심화/생각 과제] #18
base: main
Are you sure you want to change the base?
Conversation
const location = useLocation(); | ||
return ( | ||
<> | ||
<Finder /> |
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
Finder
컴포넌트가 Search
컴포넌트에서도 중복해서 나타나는데, App.jsx
에 넣어줘서 공통 컴포넌트로 쓸 수는 없으려나??
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
오 좋은 생각인 것 같다.
아직 완성은 아니구 나도 이 쪽에 고민이 좀 있는 것 같아.
의견 땡큐땡큐!!
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
오 좋은 생각인 것 같다. 아직 완성은 아니구 나도 이 쪽에 고민이 좀 있는 것 같아. 의견 땡큐땡큐!!
나도 이제 과제 시작해서 컴포넌트 구조 고민중인데 과제 엄청 빨리 했길래 의견 하나 남기고 갑니다.. 빠른과제 굿..
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
나도 과제 다 하면 놀러갈게 ~~~ 굿!
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
이전에 봤던 코드보다 훨씬 깔쌈해졌네 !!! 보기 편해요🤩
코멘트할 부분들 남겨놨어용
<QueryClientProvider client={queryClient}> | ||
<RecoilRoot> | ||
<Router /> | ||
</RecoilRoot> | ||
</QueryClientProvider> |
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
간지 ㅋ ㅋ
} | ||
|
||
export default function App() { | ||
const queryClient = new QueryClient(); |
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
요거 컴포넌트 밖에 빼줘도 되지 않을까욥
{ | ||
refetchOnWindowFocus: false, | ||
retry: 0, | ||
enabled: false, | ||
} |
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
중복되는 옵션은 최상단에서도 default option으로 처리해줄 수 있어요!
🔗 공식문서
export default function useGetTeamInfo() { | ||
const { isLoading, isError, data, refetch } = useQuery( | ||
["teaminfo"], | ||
getMyTeamAPI, |
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
같은 get api 함순데 여기는 함수호출이고, 아래서는 화살표 함수를 사용하네요 !
통일성 있게 호출하는 것이 좋아보입니다 !
export default function useGetTeamInfo() { | ||
const { isLoading, isError, data, refetch } = useQuery( |
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
해당 훅스는 get 요청의 유틸함수가 담긴 userAPI 과는 다른 폴더에 있는 게 좋을 것 같아요
return res( | ||
ctx.status(200), | ||
ctx.json({ | ||
name: ["YOOJS1205", "henization", "iamphj3", "seobbang"], |
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
name: ["YOOJS1205", "henization", "iamphj3", "seobbang"], | |
name: ["YOOJS1205", "henization", "iamphj3", "seobbang", "joohaem"], |
const historyState = atom({ | ||
key: `historyState${v1()}`, | ||
default: JSON.parse(localStorage.getItem("history") || "[]"), | ||
}); |
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
atom 을 관리하는 폴더도 분리하면 좋을 것 같네요!
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
uuid
는 머지요??!
setHistory((prev) => { | ||
if (prev.indexOf(e.target.value) === -1) { | ||
return [...prev, e.target.value]; | ||
} else { | ||
return prev; | ||
} | ||
}); |
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
히스토리를 어떻게 바꾸는지 방법을 onSearchUser
함수가 알 필요는 없어보입니다.
함수 분리를 해도 좋을 것 같아요
const onClickMemberButton = (e) => { | ||
if (e.target.nodeName.toLowerCase() === "button") { |
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
위임 멋지네ㄷ ㄷ
✨ 구현 기능 명세
기본 과제
react-router-dom
을 이용해url
에 따른 컴포넌트를 나타낸다.검색 history를 구현한다.
Loading을 나타내는 state혹은 suspense를 추가하여 로딩 중을 나타내는 UI를 구성한다.
유저 정보 박스 내에 닫기 버튼을 구현하여, 검색 전 상태로 되돌리도록 구현한다.
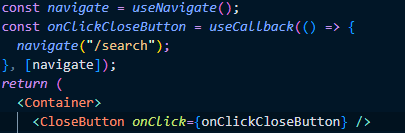

심화 과제
msw
를 이용하여 팀원의 명단을 불러오는 기능을 구현하였습니다.생각 과제
https://github.com/IN-SOPT-WEB/JunSangYOO/tree/week4/week4
🎁 PR Point
1. 사용자가 입력한 인풋값을
useNavigate
를 통하여 다른 컴포넌트로 전달하였습니다.useGetUserInfo
커스텀 훅을 만들어서 쿼리로 관리하였습니다.react-query
를 활용하였습니다.2. 최근 검색어 기능 구현
recoil
을 활용하였습니다.localStorage
와useRecoilState
를 이용하여 구현하였습니다.3. 로딩중 상태 처리
react-query
와suspense
,lazy
의 조합으로 선언적으로 처리하였습니다.4. 팀원 명단 불러오기
msw
와react-query
를 활용하여 팀원 명단을 불러오는 코드를 작성하였습니다.😭 소요 시간, 어려웠던 점
6h
suspense
로 로딩 상태 처리하는 방법을 공부했습니다.😎 구현 결과물
기본과제
심화과제