-
Notifications
You must be signed in to change notification settings - Fork 0
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
梁怀刚
committed
Jul 12, 2024
1 parent
05d699c
commit df0a650
Showing
23 changed files
with
3,709 additions
and
0 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,46 @@ | ||
--- | ||
authors: Harry | ||
tags: [daily] | ||
--- | ||
|
||
## 前端基础算法——二分查找 | ||
|
||
|
||
> 二分查找,其查找的列表必须有序。时间复杂度为:O(log n) | ||
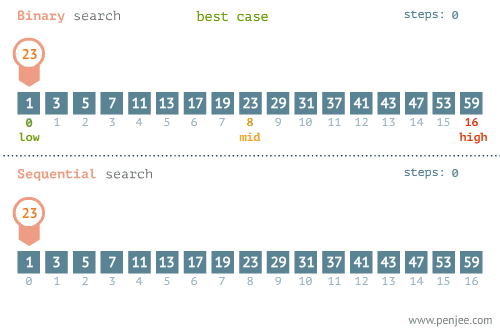 | ||
|
||
二分查询/简单查询对比(查找数23) | ||
|
||
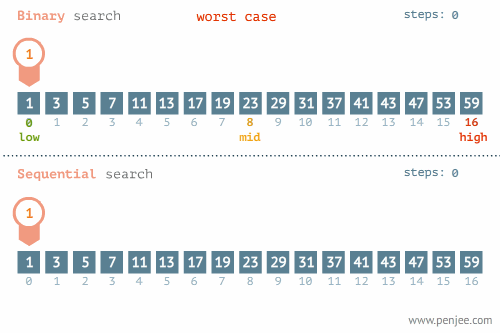 | ||
|
||
二分查询/简单查询对比(查找数1) | ||
|
||
<!--kg-card-begin: markdown--> | ||
|
||
``` | ||
function binarySearch (list, item) { | ||
// 保存最小与最大位置 | ||
let min = 0 | ||
let max = list.length - 1 | ||
// 最小位置是否小与等于最大位置 | ||
while (min <= max) { | ||
let index = Math.floor((min + max) / 2) | ||
let val = list[index] | ||
if (val === item) { | ||
return index | ||
} else if (val > item) { | ||
max = index - 1 | ||
} else { | ||
min = index + 1 | ||
} | ||
} | ||
return null | ||
} | ||
``` | ||
|
||
<!--kg-card-end: markdown--> | ||
|
||
[binarySearch](https://codepen.io/ZhelinCheng/embed/preview/dQjjZK?height=300\&slug-hash=dQjjZK\&default-tabs=js,result\&host=https://codepen.io) |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,40 @@ | ||
## 前端基础算法——选择排序 | ||
|
||
|
||
<!--kg-card-begin: markdown--> | ||
|
||
> 选择排序,运行时间:O(n^2) | ||
``` | ||
// 找出数组中最小的数字 | ||
function findSmallest (arr) { | ||
let minValue = arr[0] | ||
let minIndex = 0 | ||
for (let i in arr) { | ||
let item = arr[i] | ||
if (item >= minValue) { continue } | ||
minIndex = i | ||
minValue = item | ||
} | ||
return minIndex | ||
} | ||
// 对数字进行排序 | ||
function selectionSort (arr) { | ||
let len = arr.length | ||
let newArr = [] | ||
for (let i = 0; i < len; i++) { | ||
let minIndex = findSmallest(arr) | ||
newArr.push(arr[minIndex]) | ||
arr.splice(minIndex, 1) | ||
} | ||
return newArr | ||
} | ||
``` | ||
|
||
<!--kg-card-end: markdown--> | ||
|
||
[Selection Sort](https://codepen.io/ZhelinCheng/embed/preview/WYaZOX?height=300\&slug-hash=WYaZOX\&default-tabs=js,result\&host=https://codepen.io) |
Oops, something went wrong.