diff --git a/.gitignore b/.gitignore
new file mode 100644
index 0000000..97008e5
--- /dev/null
+++ b/.gitignore
@@ -0,0 +1,2 @@
+node_modules
+yarn.lock
\ No newline at end of file
diff --git a/README.md b/README.md
index 79e2e93..aaecbd5 100644
--- a/README.md
+++ b/README.md
@@ -2,127 +2,238 @@
[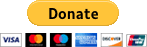](https://www.paypal.com/cgi-bin/webscr?cmd=_donations&business=7LKYWG9LXNQ9C&lc=ES&item_name=Tito%20Hinostroza&item_number=2153&no_note=0&cn=Dar%20instrucciones%20especiales%20al%20vendedor%3a&no_shipping=2¤cy_code=USD&bn=PP%2dDonationsBF%3abtn_donateCC_LG%2egif%3aNonHosted)
+#### Note from maintainer, I have not changed the above donation link. Please donate to the original author.
+
+# Release Status
+
+This is the first "stable" version. Previous versions had an API that was in flux. We're now in the feature release phase of development.
+
# Bootstable
-Javascript library to make HMTL tables editable.
-
+Tiny class to make bootstrap tables editable.
-"Bootstable" is a javascript library (plug-in), that lets convert a HTML static table to a editable table.
-A table is made editable, including several buttons to perform the edition actions.
+
+
+"Bootstable" is a javascript class, that converts HTML static tables into an editable table.
No database connection is included. The library was designed to work offline, when editing.
-Edition options includes:
+In order to save to a database, use the onEditSave hooks to call an API to save the data.
+
+Editing options includes:
-* Edit fields.
-* Remove rows.
-* Add rows.
+- Edit Row
+- Remove Row
+- Add Row
## Dependencies:
-* Jquery
-* Bootstrap
+- Bootstrap
+- Bootstrap Icons
Bootstrap is necessary to format correctly the controls used, and to draw icons.
-It's possible to work without Bootstrap too. In this case style is missing.
+
+This will work without Bootsrap, however the structures are heavily reliant on CSS classes provided by Bootstrap 5+ and display issues will result. To adjust, modify your CSS/SASS to handle the classes presented.
+
+Bootstrap Icons is used for glyphs in the buttons.
## Requirements
1. For a table to be editable, it needs to have the following structure:
-```
+```html
-
-
-
...
-
-
-
-
-
...
-
-
+
+
+
+
+
+ ...
+
+
+
+
+
+
+
+ ...
+
+
```
-2. Bootstable needs the ID of the table to edit, and can only work on a single table.
-
- $('.mytable').SetEditable(); //BAD! No class reference allowed.
- $('table').SetEditable(); //BAD! No several tables allowed.
+You can also hide columns and set a "role" for that column. In this case you can maintain an "ID" column for interacting with a database.
+
+```html
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+```
-If several tables need to be editable in a same Web page, it's needed to set each table:
+2. Bootstable needs the ID of the table to edit, and can only work on a single table.
- $('#mytable1').SetEditable(); //GOOD!
- $('#mytable2').SetEditable(); //GOOD!
+ const bstable = new bootstable("mytable", options)
-LIMITATION: When using several editable tables, events won't work properly.
+3. To edit multiple tables on a single page, instantiate the class for each table.
## Examples
Sets all the columns of #mytable editable:
- $('#mytable').SetEditable();
+```javascript
+const bstable = new bootstable("mytable");
+```
Sets the columns 0 and 1 of #mytable editable:
- $('#mytable').SetEditable({
- columnsEd: "0,1" //editable columns
- });
-
-Includes a "New row" button (Obsolete):
-
- $('#mytable').SetEditable({
- columnsEd: "0,1",
- $addButton: $('#but_add')
- });
-
-Includes a "New row" button (Prefered):
+```javascript
+const bstable = new bootstable("mytable", {
+ columnsEd: [0, 1], //editable columns
+});
+```
- $('#mytable').SetEditable();
+Includes a "New row" button, this will add as a new button to the table headers:
- $('#but_add').click(function() {
- rowAddNew('mytable');
- });
+```javascript
+const bstable = new bootstable("mytable", {
+ columnsEd: [0, 1],
+ $addButton: "buttonId",
+});
+```
Set a "New row" button to add a row and set initial values:
- $('#mytable').SetEditable();
-
- $('#but_add').click(function() {
- rowAddNew('mytable', [1,2,3]);
- });
+```javascript
+const bstable = new bootstable("mytable", {
+ $addButton: "buttonId",
+ defaultValues: [1, 2, 3],
+});
+```
Set a "New row" button to add a row, set initial values and turn to edit mode:
- $('#mytable').SetEditable();
-
- $('#but_add').click(function() {
- rowAddNewAndEdit('mytable', [1,2,3]);
- });
+```javascript
+const bstable = new bootstable("mytable", {
+ $addButton: "buttonId",
+ defaultValues: [1, 2, 3],
+ addButtonEdit: true, // Forces bootstable to edit the new row immediately.
+});
+```
Parameters:
- columnsEd: null, //Index to editable columns. If null, all columns will be editables. Ex.: "1,2,3,4,5"
- $addButton: null, //Jquery object of "Add" button. OBSOLETE.
- bootstrap: true, //Indicates if library is going to worl with Bootstrap library.
- onEdit: function() {}, //Called after edition
- onBeforeDelete: function() {}, //Called before deletion
- onDelete: function() {}, //Called after deletion
- onAdd: function() {} //Called when added a new row
+```javascript
+const options = {
+ // Properties
+ columnsEd: Array(), // Default: null -- Index to editable columns. If null, all columns will be editable. Ex.: [ 0,1,2,3,4,5 ]
+ $addButton: string, // Default: null -- ID of "Add" button.
+ defaultValues: Array(), // Default: null -- Set default values, must match the number of editable columns
+ addButtonEdit: boolean, // Default: false -- Should bootstable edit the rows after adding?
+ buttons: Object(), // Overide default buttons
+ exportCsvButton: boolean, Default: false -- add an export to CSV button
+ exportJsonButton: boolean, Default: false -- add an export to JSON button
+
+ // Callbacks
+ onEdit: (rowElement) => {}, // Called after clicking edit button
+ onBeforeDelete: (rowElement) => {}, // Called just before deletion must return a boolean, true means row will be deleted.
+ onDelete: (rowElement) => {}, // Called after deletion button, but after onBeforeDelete. If onBeforeDelete returns false, bypass.
+ onAdd: (rowElement) => {} // Called when new row is added to table
+};
+```
# Utilities
There are two functions, included in the library, to facilitate the export of the table:
-* function TableToCSV(tabId, separator)
-* function TableToJson(tabId)
+```javascript
+// Get a CSV string and/or trigger a browser download
+const csvString = bstable.TableToCSV(
+ tableId,
+ separator,
+ downloadBool,
+ filenameStr
+);
+
+// Get a JSON string and/or trigger a browser download
+const jsonString = bstable.TableToJson(tableId, downloadBool, filenameStr);
+```
-These functions return a string in the appropriate format (CSV or JSON) from any HTML table.
+These functions return a string of the data, but can be set to create a file download by setting downloadBool to true and supplying a filename for download.
+
+# Default Buttons
+
+In order to self-stylize buttons, pass a replacement object for the button(s) you wish to modify:
+
+```javascript
+const buttons = {
+ bEdit: {
+ className: "btn btn-sm btn-primary",
+ icon: "fa fa-pencil",
+ display: "block",
+ onclick: (but) => {
+ var target = but.target;
+ if (target.tagName == "I") {
+ target = but.target.parentNode;
+ }
+ this.butRowEdit(target);
+ },
+ },
+ bElim: {
+ className: "btn btn-sm btn-danger",
+ icon: "fa fa-trash",
+ display: "block",
+ onclick: (but) => {
+ var target = but.target;
+ if (target.tagName == "I") {
+ target = but.target.parentNode;
+ }
+ this.butRowDelete(target);
+ },
+ },
+ bAcep: {
+ className: "btn btn-sm btn-success",
+ icon: "fa fa-check",
+ display: "none",
+ onclick: (but) => {
+ var target = but.target;
+ if (target.tagName == "I") {
+ target = but.target.parentNode;
+ }
+ this.butRowAcep(target);
+ },
+ },
+ bCanc: {
+ className: "btn btn-sm btn-warning",
+ icon: "fa fa-remove",
+ display: "none",
+ onclick: (but) => {
+ var target = but.target;
+ if (target.tagName == "I") {
+ target = but.target.parentNode;
+ }
+ this.butRowCancel(target);
+ },
+ },
+};
+```
-# References
+# References (Obsolete, needs updating)
Some page explaining the use of bootstable:
-* https://medium.com/@diyifang/bootstable-js-editable-table-with-bootstrap-6694f016f1b8
-* https://codepen.io/diyifang/pen/mXdQmB
-* http://ivanovdmitry.com/blog/post/create-editable-tables-with-jquery-and-bootstrap-bootstable
+- https://medium.com/@diyifang/bootstable-js-editable-table-with-bootstrap-6694f016f1b8
+- https://codepen.io/diyifang/pen/mXdQmB
+- http://ivanovdmitry.com/blog/post/create-editable-tables-with-jquery-and-bootstrap-bootstable
diff --git a/bootstable.js b/bootstable.js
index 35165c7..05c59d9 100644
--- a/bootstable.js
+++ b/bootstable.js
@@ -1,262 +1,504 @@
/*
Bootstable
@description Javascript library to make HMTL tables editable, using Bootstrap
- @version 1.1
- @autor Tito Hinostroza
+ @version 1.5.32
+ @author Tito Hinostroza
+ @contributors Tyler Hardison
*/
"use strict";
-//Global variables
-var params = null; //Parameters
-var colsEdi =null;
-var newColHtml = '
'+
-''+
-''+
-''+
-''+
- '
';
- //Case NOT Bootstrap
- var newColHtml2 = '
'+
- ''+
- ''+
- ''+
- ''+
- '
';
-var colEdicHtml = '
'+newColHtml+'
';
-$.fn.SetEditable = function (options) {
- var defaults = {
- columnsEd: null, //Index to editable columns. If null all td editables. Ex.: "1,2,3,4,5"
- $addButton: null, //Jquery object of "Add" button
- bootstrap: true, //Indicates bootstrap is present.
- onEdit: function() {}, //Called after edition
- onBeforeDelete: function() {}, //Called before deletion
- onDelete: function() {}, //Called after deletion
- onAdd: function() {} //Called when added a new row
- };
- params = $.extend(defaults, options);
- var $tabedi = this; //Read reference to the current table.
- $tabedi.find('thead tr').append('