diff --git a/docs/.vitepress/config.ts b/docs/.vitepress/config.ts
new file mode 100644
index 0000000..fac6149
--- /dev/null
+++ b/docs/.vitepress/config.ts
@@ -0,0 +1,64 @@
+import { defineConfig } from 'vitepress';
+import { name, description, repository, license, author } from '../../package.json';
+import typedocSidebar from '../api/typedoc-sidebar.json';
+
+const cleanName = name.replace('@sgratzl/', '');
+
+// https://vitepress.dev/reference/site-config
+export default defineConfig({
+ title: cleanName,
+ description,
+ base: `/${cleanName}/`,
+ useWebFonts: false,
+ themeConfig: {
+ // https://vitepress.dev/reference/default-theme-config
+ nav: [
+ { text: 'Home', link: '/' },
+ { text: 'Getting Started', link: '/getting-started' },
+ { text: 'Examples', link: '/examples/' },
+ { text: 'API', link: '/api/' },
+ { text: 'Related Plugins', link: '/related' },
+ ],
+
+ sidebar: [
+ {
+ text: 'Examples',
+ items: [
+ { text: 'Basic', link: '/examples/' },
+ { text: 'Choropleth US Map', link: '/examples/choropleth' },
+ { text: 'Bubble Map', link: '/examples/bubbleMap' },
+ { text: 'Custom Color Scale', link: '/examples/custom' },
+ { text: 'Legend Customization', link: '/examples/legend' },
+ { text: 'Tooltip Center', link: '/examples/center' },
+ { text: 'Projection Offset', link: '/examples/offset' },
+ { text: 'Equal Earth Projection', link: '/examples/projection' },
+ { text: 'World Atlas', link: '/examples/earth' },
+ { text: 'Bubble Map Area Mode', link: '/examples/area' },
+ ],
+ },
+ {
+ text: 'API',
+ collapsed: true,
+ items: typedocSidebar,
+ },
+ ],
+
+ socialLinks: [{ icon: 'github', link: repository.url.replace('.git', '') }],
+
+ footer: {
+ message: `Released under the ${license} license.`,
+ copyright: `Copyright © 2019-present ${author.name}`,
+ },
+
+ editLink: {
+ pattern: `${repository.url.replace('.git', '')}/edit/main/docs/:path`,
+ },
+
+ search: {
+ provider: 'local',
+ },
+ },
+});
diff --git a/docs/.vitepress/theme/index.ts b/docs/.vitepress/theme/index.ts
new file mode 100644
index 0000000..02d8508
--- /dev/null
+++ b/docs/.vitepress/theme/index.ts
@@ -0,0 +1,42 @@
+import Theme from 'vitepress/theme';
+import { createTypedChart } from 'vue-chartjs';
+import { Tooltip, PointElement } from 'chart.js';
+import {
+ BubbleMapController,
+ ChoroplethController,
+ ColorScale,
+ ColorLogarithmicScale,
+ SizeLogarithmicScale,
+ SizeScale,
+ GeoFeature,
+ ProjectionScale,
+} from '../../../src';
+
+export default {
+ ...Theme,
+ enhanceApp({ app }) {
+ app.component(
+ 'BubbleMapChart',
+ createTypedChart('bubbleMap', [
+ ProjectionScale,
+ BubbleMapController,
+ SizeScale,
+ SizeLogarithmicScale,
+ PointElement,
+ GeoFeature,
+ Tooltip,
+ ])
+ );
+ app.component(
+ 'ChoroplethChart',
+ createTypedChart('choropleth', [
+ ProjectionScale,
+ ChoroplethController,
+ ColorScale,
+ ColorLogarithmicScale,
+ GeoFeature,
+ Tooltip,
+ ])
+ );
+ },
+};
diff --git a/docs/examples/albers.ts b/docs/examples/albers.ts
new file mode 100644
index 0000000..34193cd
--- /dev/null
+++ b/docs/examples/albers.ts
@@ -0,0 +1,45 @@
+import type { ChartConfiguration } from 'chart.js';
+import { Feature, topojson } from '../../src';
+
+// #region data
+import states10m from 'us-atlas/states-10m.json';
+
+const nation: Feature = topojson.feature(states10m as any, states10m.objects.nation as any).features[0];
+const states: Feature = topojson.feature(states10m as any, states10m.objects.states as any).features;
+
+export const data: ChartConfiguration<'choropleth'>['data'] = {
+ labels: states.map((d) => d.properties.name),
+ datasets: [
+ {
+ label: 'States',
+ outline: nation,
+ data: states.map((d) => ({
+ feature: d,
+ value: Math.random() * 11,
+ })),
+ },
+ ],
+};
+// #endregion data
+// #region config
+export const config: ChartConfiguration<'choropleth'> = {
+ type: 'choropleth',
+ data,
+ options: {
+ scales: {
+ projection: {
+ axis: 'x',
+ projection: 'albersUsa',
+ },
+ color: {
+ axis: 'x',
+ quantize: 5,
+ legend: {
+ position: 'bottom-right',
+ align: 'right',
+ },
+ },
+ },
+ },
+};
+// #endregion config
diff --git a/docs/examples/area.md b/docs/examples/area.md
new file mode 100644
index 0000000..eaa1362
--- /dev/null
+++ b/docs/examples/area.md
@@ -0,0 +1,24 @@
+---
+title: Bubble Map Area Mode
+---
+
+# Bubble Map Area Mode
+
+
+
+
+
+### Code
+
+:::code-group
+
+<<< ./area.ts#config [config]
+
+<<< ./bubbleMap.ts#data [data]
+
+:::
diff --git a/docs/examples/area.ts b/docs/examples/area.ts
new file mode 100644
index 0000000..22bfbb0
--- /dev/null
+++ b/docs/examples/area.ts
@@ -0,0 +1,26 @@
+import type { ChartConfiguration } from 'chart.js';
+import { Feature, topojson } from '../../src';
+import { data } from './bubbleMap';
+
+// #region config
+export const config: ChartConfiguration<'bubbleMap'> = {
+ type: 'bubbleMap',
+ data,
+ options: {
+ scales: {
+ projection: {
+ axis: 'x',
+ projection: 'albersUsa',
+ },
+ size: {
+ axis: 'x',
+ size: [1, 20],
+ mode: 'area',
+ },
+ },
+ layout: {
+ // padding: 20
+ },
+ },
+};
+// #endregion config
diff --git a/docs/examples/bubbleMap.md b/docs/examples/bubbleMap.md
new file mode 100644
index 0000000..56b96d1
--- /dev/null
+++ b/docs/examples/bubbleMap.md
@@ -0,0 +1,24 @@
+---
+title: Bubble Map
+---
+
+# Bubble Map
+
+
+
+
+
+### Code
+
+:::code-group
+
+<<< ./bubbleMap.ts#config [config]
+
+<<< ./bubbleMap.ts#data [data]
+
+:::
diff --git a/docs/examples/bubbleMap.ts b/docs/examples/bubbleMap.ts
new file mode 100644
index 0000000..8c2ff1b
--- /dev/null
+++ b/docs/examples/bubbleMap.ts
@@ -0,0 +1,51 @@
+import type { ChartConfiguration } from 'chart.js';
+import { Feature, topojson } from '../../src';
+// #region data
+import states10m from 'us-atlas/states-10m.json';
+import capitals from './data/us-capitals.json';
+import ChartDataLabels from 'chartjs-plugin-datalabels';
+
+const states: Feature = topojson.feature(states10m as any, states10m.objects.states as any).features;
+
+export const data: ChartConfiguration<'bubbleMap'>['data'] = {
+ labels: capitals.map((d) => d.description),
+ datasets: [
+ {
+ outline: states,
+ showOutline: true,
+ backgroundColor: 'steelblue',
+ data: capitals.map((d) => Object.assign(d, { value: Math.round(Math.random() * 10) })),
+ },
+ ],
+};
+// #endregion data
+// #region config
+export const config: ChartConfiguration<'bubbleMap'> = {
+ type: 'bubbleMap',
+ data,
+ options: {
+ plugins: {
+ datalabels: {
+ align: 'top',
+ formatter: (v) => {
+ return v.description;
+ },
+ },
+ },
+ scales: {
+ projection: {
+ axis: 'x',
+ projection: 'albersUsa',
+ },
+ size: {
+ axis: 'x',
+ size: [1, 20],
+ },
+ },
+ layout: {
+ // padding: 20
+ },
+ },
+ plugins: [ChartDataLabels],
+};
+// #endregion config
diff --git a/docs/examples/center.md b/docs/examples/center.md
new file mode 100644
index 0000000..7f96ce7
--- /dev/null
+++ b/docs/examples/center.md
@@ -0,0 +1,24 @@
+---
+title: Custom Tooltip Center
+---
+
+# Custom Tooltip Center
+
+
+
+
+
+### Code
+
+:::code-group
+
+<<< ./center.ts#config [config]
+
+<<< ./center.ts#data [data]
+
+:::
diff --git a/docs/examples/center.ts b/docs/examples/center.ts
new file mode 100644
index 0000000..7714fdc
--- /dev/null
+++ b/docs/examples/center.ts
@@ -0,0 +1,49 @@
+import type { ChartConfiguration } from 'chart.js';
+import { Feature, topojson } from '../../src';
+
+// #region data
+import states10m from 'us-atlas/states-10m.json';
+import capitals from './data/us-capitals.json';
+
+const nation: Feature = topojson.feature(states10m as any, states10m.objects.nation as any).features[0];
+const states: Feature = topojson.feature(states10m as any, states10m.objects.states as any).features;
+
+const lookup = new Map(capitals.map(({ name, latitude, longitude }) => [name, { latitude, longitude }]));
+
+export const data: ChartConfiguration<'choropleth'>['data'] = {
+ labels: states.map((d) => d.properties.name),
+ datasets: [
+ {
+ label: 'States',
+ outline: nation,
+ data: states.map((d) => ({
+ feature: d,
+ center: lookup.get(d.properties.name),
+ value: Math.random() * 11,
+ })),
+ },
+ ],
+};
+// #endregion data
+// #region config
+export const config: ChartConfiguration<'choropleth'> = {
+ type: 'choropleth',
+ data,
+ options: {
+ scales: {
+ projection: {
+ axis: 'x',
+ projection: 'albersUsa',
+ },
+ color: {
+ axis: 'x',
+ quantize: 5,
+ legend: {
+ position: 'bottom-right',
+ align: 'right',
+ },
+ },
+ },
+ },
+};
+// #endregion config
diff --git a/docs/examples/choropleth.md b/docs/examples/choropleth.md
new file mode 100644
index 0000000..bb057a7
--- /dev/null
+++ b/docs/examples/choropleth.md
@@ -0,0 +1,24 @@
+---
+title: Choropleth US Map
+---
+
+# Choropleth US Map
+
+
+
+
+
+### Code
+
+:::code-group
+
+<<< ./albers.ts#config [config]
+
+<<< ./albers.ts#data [data]
+
+:::
diff --git a/docs/examples/custom.md b/docs/examples/custom.md
new file mode 100644
index 0000000..17d575d
--- /dev/null
+++ b/docs/examples/custom.md
@@ -0,0 +1,24 @@
+---
+title: Custom Color Scale
+---
+
+# Custom Color Scale
+
+
+
+
+
+### Code
+
+:::code-group
+
+<<< ./custom.ts#config [config]
+
+<<< ./albers.ts#data [data]
+
+:::
diff --git a/docs/examples/custom.ts b/docs/examples/custom.ts
new file mode 100644
index 0000000..bd06ef9
--- /dev/null
+++ b/docs/examples/custom.ts
@@ -0,0 +1,25 @@
+import type { ChartConfiguration } from 'chart.js';
+import { data } from './albers';
+
+// #region config
+export const config: ChartConfiguration<'choropleth'> = {
+ type: 'choropleth',
+ data,
+ options: {
+ scales: {
+ projection: {
+ axis: 'x',
+ projection: 'albersUsa',
+ },
+ color: {
+ axis: 'x',
+ interpolate: (v) => (v < 0.5 ? 'green' : 'red'),
+ legend: {
+ position: 'bottom-right',
+ align: 'right',
+ },
+ },
+ },
+ },
+};
+// #endregion config
diff --git a/docs/examples/data/README.md b/docs/examples/data/README.md
new file mode 100644
index 0000000..4cbfb55
--- /dev/null
+++ b/docs/examples/data/README.md
@@ -0,0 +1 @@
+from https://gist.githubusercontent.com/mbostock/9535021/raw/928a5f81f170b767162f8f52dbad05985eae9cac/us-state-capitals.csv
diff --git a/docs/examples/data/us-capitals.json b/docs/examples/data/us-capitals.json
new file mode 100644
index 0000000..b782af4
--- /dev/null
+++ b/docs/examples/data/us-capitals.json
@@ -0,0 +1,302 @@
+[
+ {
+ "name": "Alabama",
+ "description": "Montgomery",
+ "latitude": 32.377716,
+ "longitude": -86.300568
+ },
+ {
+ "name": "Alaska",
+ "description": "Juneau",
+ "latitude": 58.301598,
+ "longitude": -134.420212
+ },
+ {
+ "name": "Arizona",
+ "description": "Phoenix",
+ "latitude": 33.448143,
+ "longitude": -112.096962
+ },
+ {
+ "name": "Arkansas",
+ "description": "Little Rock",
+ "latitude": 34.746613,
+ "longitude": -92.288986
+ },
+ {
+ "name": "California",
+ "description": "Sacramento",
+ "latitude": 38.576668,
+ "longitude": -121.493629
+ },
+ {
+ "name": "Colorado",
+ "description": "Denver",
+ "latitude": 39.739227,
+ "longitude": -104.984856
+ },
+ {
+ "name": "Connecticut",
+ "description": "Hartford",
+ "latitude": 41.764046,
+ "longitude": -72.682198
+ },
+ {
+ "name": "Delaware",
+ "description": "Dover",
+ "latitude": 39.157307,
+ "longitude": -75.519722
+ },
+ {
+ "name": "Hawaii",
+ "description": "Honolulu",
+ "latitude": 21.307442,
+ "longitude": -157.857376
+ },
+ {
+ "name": "Florida",
+ "description": "Tallahassee",
+ "latitude": 30.438118,
+ "longitude": -84.281296
+ },
+ {
+ "name": "Georgia",
+ "description": "Atlanta",
+ "latitude": 33.749027,
+ "longitude": -84.388229
+ },
+ {
+ "name": "Idaho",
+ "description": "Boise",
+ "latitude": 43.617775,
+ "longitude": -116.199722
+ },
+ {
+ "name": "Illinois",
+ "description": "Springfield",
+ "latitude": 39.798363,
+ "longitude": -89.654961
+ },
+ {
+ "name": "Indiana",
+ "description": "Indianapolis",
+ "latitude": 39.768623,
+ "longitude": -86.162643
+ },
+ {
+ "name": "Iowa",
+ "description": "Des Moines",
+ "latitude": 41.591087,
+ "longitude": -93.603729
+ },
+ {
+ "name": "Kansas",
+ "description": "Topeka",
+ "latitude": 39.048191,
+ "longitude": -95.677956
+ },
+ {
+ "name": "Kentucky",
+ "description": "Frankfort",
+ "latitude": 38.186722,
+ "longitude": -84.875374
+ },
+ {
+ "name": "Louisiana",
+ "description": "Baton Rouge",
+ "latitude": 30.457069,
+ "longitude": -91.187393
+ },
+ {
+ "name": "Maine",
+ "description": "Augusta",
+ "latitude": 44.307167,
+ "longitude": -69.781693
+ },
+ {
+ "name": "Maryland",
+ "description": "Annapolis",
+ "latitude": 38.978764,
+ "longitude": -76.490936
+ },
+ {
+ "name": "Massachusetts",
+ "description": "Boston",
+ "latitude": 42.358162,
+ "longitude": -71.063698
+ },
+ {
+ "name": "Michigan",
+ "description": "Lansing",
+ "latitude": 42.733635,
+ "longitude": -84.555328
+ },
+ {
+ "name": "Minnesota",
+ "description": "St. Paul",
+ "latitude": 44.955097,
+ "longitude": -93.102211
+ },
+ {
+ "name": "Mississippi",
+ "description": "Jackson",
+ "latitude": 32.303848,
+ "longitude": -90.182106
+ },
+ {
+ "name": "Missouri",
+ "description": "Jefferson City",
+ "latitude": 38.579201,
+ "longitude": -92.172935
+ },
+ {
+ "name": "Montana",
+ "description": "Helena",
+ "latitude": 46.585709,
+ "longitude": -112.018417
+ },
+ {
+ "name": "Nebraska",
+ "description": "Lincoln",
+ "latitude": 40.808075,
+ "longitude": -96.699654
+ },
+ {
+ "name": "Nevada",
+ "description": "Carson City",
+ "latitude": 39.163914,
+ "longitude": -119.766121
+ },
+ {
+ "name": "New Hampshire",
+ "description": "Concord",
+ "latitude": 43.206898,
+ "longitude": -71.537994
+ },
+ {
+ "name": "New Jersey",
+ "description": "Trenton",
+ "latitude": 40.220596,
+ "longitude": -74.769913
+ },
+ {
+ "name": "New Mexico",
+ "description": "Santa Fe",
+ "latitude": 35.68224,
+ "longitude": -105.939728
+ },
+ {
+ "name": "North Carolina",
+ "description": "Raleigh",
+ "latitude": 35.78043,
+ "longitude": -78.639099
+ },
+ {
+ "name": "North Dakota",
+ "description": "Bismarck",
+ "latitude": 46.82085,
+ "longitude": -100.783318
+ },
+ {
+ "name": "New York",
+ "description": "Albany",
+ "latitude": 42.652843,
+ "longitude": -73.757874
+ },
+ {
+ "name": "Ohio",
+ "description": "Columbus",
+ "latitude": 39.961346,
+ "longitude": -82.999069
+ },
+ {
+ "name": "Oklahoma",
+ "description": "Oklahoma City",
+ "latitude": 35.492207,
+ "longitude": -97.503342
+ },
+ {
+ "name": "Oregon",
+ "description": "Salem",
+ "latitude": 44.938461,
+ "longitude": -123.030403
+ },
+ {
+ "name": "Pennsylvania",
+ "description": "Harrisburg",
+ "latitude": 40.264378,
+ "longitude": -76.883598
+ },
+ {
+ "name": "Rhode Island",
+ "description": "Providence",
+ "latitude": 41.830914,
+ "longitude": -71.414963
+ },
+ {
+ "name": "South Carolina",
+ "description": "Columbia",
+ "latitude": 34.000343,
+ "longitude": -81.033211
+ },
+ {
+ "name": "South Dakota",
+ "description": "Pierre",
+ "latitude": 44.367031,
+ "longitude": -100.346405
+ },
+ {
+ "name": "Tennessee",
+ "description": "Nashville",
+ "latitude": 36.16581,
+ "longitude": -86.784241
+ },
+ {
+ "name": "Texas",
+ "description": "Austin",
+ "latitude": 30.27467,
+ "longitude": -97.740349
+ },
+ {
+ "name": "Utah",
+ "description": "Salt Lake City",
+ "latitude": 40.777477,
+ "longitude": -111.888237
+ },
+ {
+ "name": "Vermont",
+ "description": "Montpelier",
+ "latitude": 44.262436,
+ "longitude": -72.580536
+ },
+ {
+ "name": "Virginia",
+ "description": "Richmond",
+ "latitude": 37.538857,
+ "longitude": -77.43364
+ },
+ {
+ "name": "Washington",
+ "description": "Olympia",
+ "latitude": 47.035805,
+ "longitude": -122.905014
+ },
+ {
+ "name": "West Virginia",
+ "description": "Charleston",
+ "latitude": 38.336246,
+ "longitude": -81.612328
+ },
+ {
+ "name": "Wisconsin",
+ "description": "Madison",
+ "latitude": 43.074684,
+ "longitude": -89.384445
+ },
+ {
+ "name": "Wyoming",
+ "description": "Cheyenne",
+ "latitude": 41.140259,
+ "longitude": -104.820236
+ }
+]
diff --git a/docs/examples/earth.md b/docs/examples/earth.md
new file mode 100644
index 0000000..40608dd
--- /dev/null
+++ b/docs/examples/earth.md
@@ -0,0 +1,24 @@
+---
+title: World Atlas
+---
+
+# World Atlas
+
+
+
+
+
+### Code
+
+:::code-group
+
+<<< ./earth.ts#config [config]
+
+<<< ./earth.ts#data [data]
+
+:::
diff --git a/docs/examples/earth.ts b/docs/examples/earth.ts
new file mode 100644
index 0000000..7c86755
--- /dev/null
+++ b/docs/examples/earth.ts
@@ -0,0 +1,40 @@
+import type { ChartConfiguration } from 'chart.js';
+import { Feature, topojson } from '../../src';
+
+// #region data
+import countries50m from 'world-atlas/countries-50m.json';
+
+const countries: Feature = topojson.feature(countries50m as any, countries50m.objects.countries as any).features;
+
+export const data: ChartConfiguration<'choropleth'>['data'] = {
+ labels: countries.map((d) => d.properties.name),
+ datasets: [
+ {
+ label: 'Countries',
+ data: countries.map((d) => ({
+ feature: d,
+ value: Math.random(),
+ })),
+ },
+ ],
+};
+// #endregion data
+// #region config
+export const config: ChartConfiguration<'choropleth'> = {
+ type: 'choropleth',
+ data,
+ options: {
+ showOutline: true,
+ showGraticule: true,
+ scales: {
+ projection: {
+ axis: 'x',
+ projection: 'equalEarth',
+ },
+ },
+ onClick: (evt, elems) => {
+ console.log(elems.map((elem) => elem.element.feature.properties.name));
+ },
+ },
+};
+// #endregion config
diff --git a/docs/examples/index.md b/docs/examples/index.md
new file mode 100644
index 0000000..aa300af
--- /dev/null
+++ b/docs/examples/index.md
@@ -0,0 +1,44 @@
+---
+title: Examples
+---
+
+# Examples
+
+
+
+## Choropleth Map
+
+
+
+### Code
+
+:::code-group
+
+<<< ./albers.ts#config [config]
+
+<<< ./albers.ts#data [data]
+
+:::
+
+## Bubble Map
+
+
+
+### Code
+
+:::code-group
+
+<<< ./bubbleMap.ts#config [config]
+
+<<< ./bubbleMap.ts#data [data]
+
+:::
diff --git a/docs/examples/legend.md b/docs/examples/legend.md
new file mode 100644
index 0000000..3848e5d
--- /dev/null
+++ b/docs/examples/legend.md
@@ -0,0 +1,24 @@
+---
+title: Legend Customization
+---
+
+# Legend Customization
+
+
+
+
+
+### Code
+
+:::code-group
+
+<<< ./legend.ts#config [config]
+
+<<< ./albers.ts#data [data]
+
+:::
diff --git a/docs/examples/legend.ts b/docs/examples/legend.ts
new file mode 100644
index 0000000..e5b0163
--- /dev/null
+++ b/docs/examples/legend.ts
@@ -0,0 +1,25 @@
+import type { ChartConfiguration } from 'chart.js';
+import { data } from './albers';
+
+// #region config
+export const config: ChartConfiguration<'choropleth'> = {
+ type: 'choropleth',
+ data,
+ options: {
+ scales: {
+ projection: {
+ axis: 'x',
+ projection: 'albersUsa',
+ },
+ color: {
+ axis: 'x',
+ quantize: 5,
+ legend: {
+ position: 'bottom-right',
+ align: 'right',
+ },
+ },
+ },
+ },
+};
+// #endregion config
diff --git a/docs/examples/offset.md b/docs/examples/offset.md
new file mode 100644
index 0000000..69ec687
--- /dev/null
+++ b/docs/examples/offset.md
@@ -0,0 +1,43 @@
+---
+title: Projection Offset
+---
+
+# Projection Offset and Scale
+
+
+
+## Projection Offset
+
+
+
+### Code
+
+:::code-group
+
+<<< ./offset.ts#config [config]
+
+<<< ./albers.ts#data [data]
+
+:::
+
+## Projection Scale
+
+
+
+### Code
+
+:::code-group
+
+<<< ./offset.ts#scale [config]
+
+<<< ./albers.ts#data [data]
+
+:::
diff --git a/docs/examples/offset.ts b/docs/examples/offset.ts
new file mode 100644
index 0000000..6ce255c
--- /dev/null
+++ b/docs/examples/offset.ts
@@ -0,0 +1,36 @@
+import type { ChartConfiguration } from 'chart.js';
+import { data } from './albers';
+
+// #region config
+export const offset: ChartConfiguration<'choropleth'> = {
+ type: 'choropleth',
+ data,
+ options: {
+ scales: {
+ projection: {
+ axis: 'x',
+ projection: 'albersUsa',
+ // offset in pixel
+ projectionOffset: [50, 0],
+ },
+ },
+ },
+};
+// #endregion config
+
+// #region scale
+export const scale: ChartConfiguration<'choropleth'> = {
+ type: 'choropleth',
+ data,
+ options: {
+ scales: {
+ projection: {
+ axis: 'x',
+ projection: 'albersUsa',
+ // custom scale factor,
+ projectionScale: 1.5,
+ },
+ },
+ },
+};
+// #endregion scale
diff --git a/docs/examples/projection.md b/docs/examples/projection.md
new file mode 100644
index 0000000..943db4e
--- /dev/null
+++ b/docs/examples/projection.md
@@ -0,0 +1,24 @@
+---
+title: Equal Earth Projection
+---
+
+# Equal Earth Projection
+
+
+
+
+
+### Code
+
+:::code-group
+
+<<< ./projection.ts#config [config]
+
+<<< ./albers.ts#data [data]
+
+:::
diff --git a/docs/examples/projection.ts b/docs/examples/projection.ts
new file mode 100644
index 0000000..d672598
--- /dev/null
+++ b/docs/examples/projection.ts
@@ -0,0 +1,25 @@
+import type { ChartConfiguration } from 'chart.js';
+import { data } from './albers';
+
+// #region config
+export const config: ChartConfiguration<'choropleth'> = {
+ type: 'choropleth',
+ data,
+ options: {
+ scales: {
+ projection: {
+ axis: 'x',
+ projection: 'equalEarth',
+ },
+ color: {
+ axis: 'x',
+ interpolate: (v) => (v < 0.5 ? 'green' : 'red'),
+ legend: {
+ position: 'bottom-right',
+ align: 'right',
+ },
+ },
+ },
+ },
+};
+// #endregion config
diff --git a/docs/getting-started.md b/docs/getting-started.md
new file mode 100644
index 0000000..8d94ef8
--- /dev/null
+++ b/docs/getting-started.md
@@ -0,0 +1,59 @@
+---
+title: Getting Started
+---
+
+Chart.js module for charting maps with legends. Adding new chart types: `choropleth` and `bubbleMap`.
+
+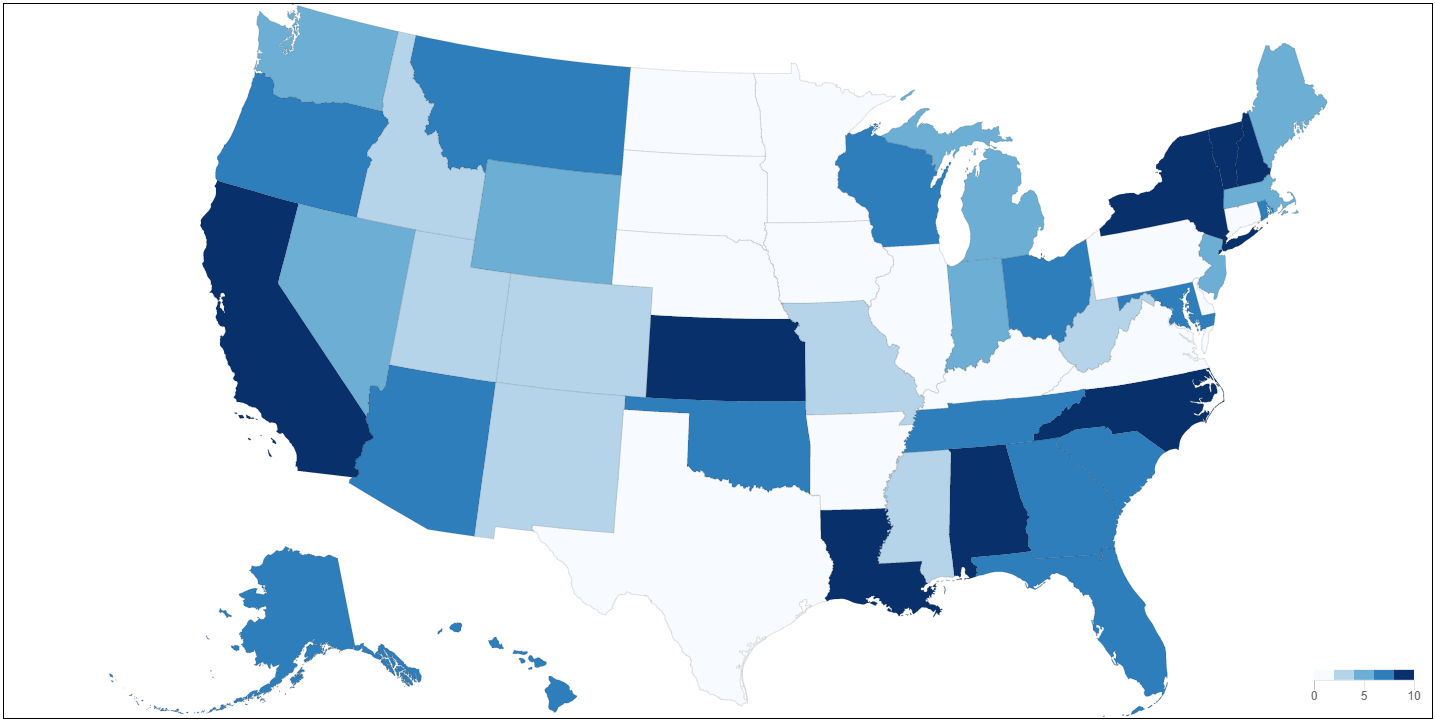
+
+[CodePen](https://codepen.io/sgratzl/pen/gOaBQep)
+
+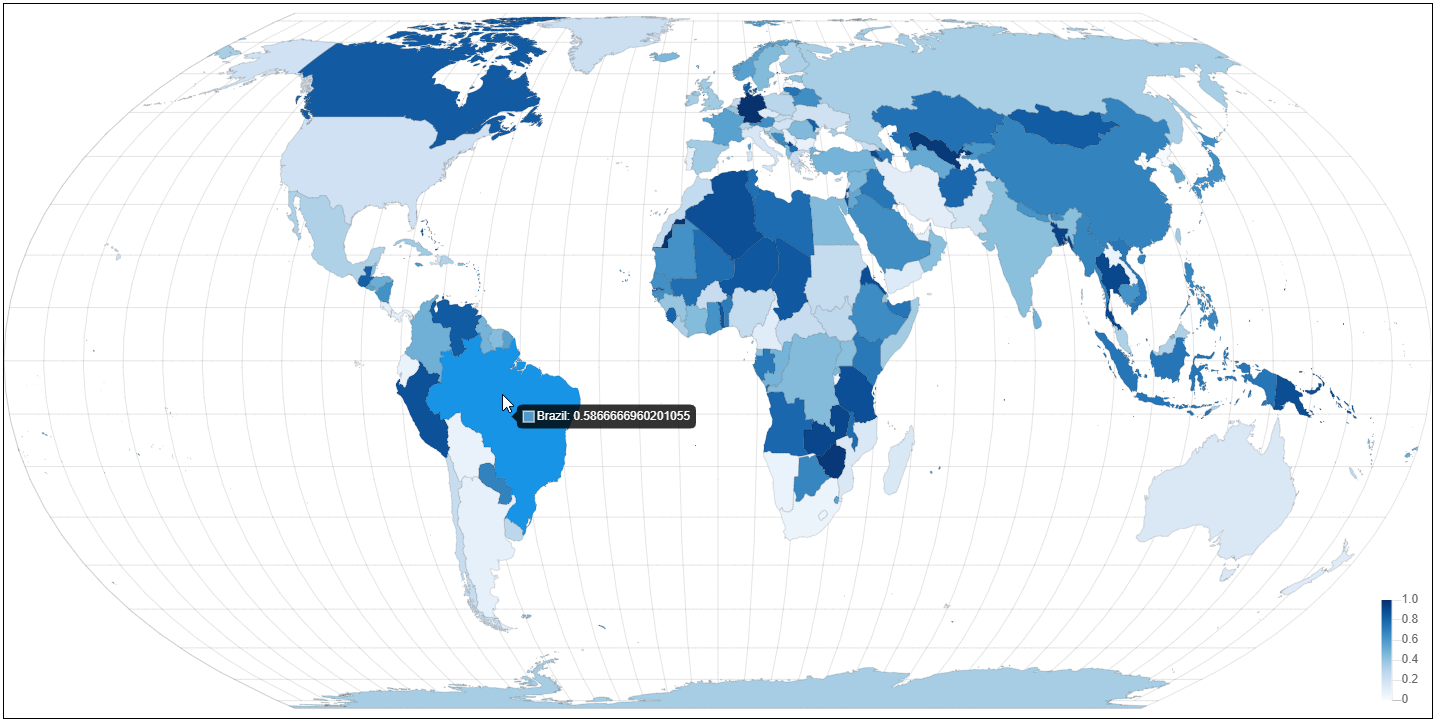
+
+[CodePen](https://codepen.io/sgratzl/pen/bGVmQKw)
+
+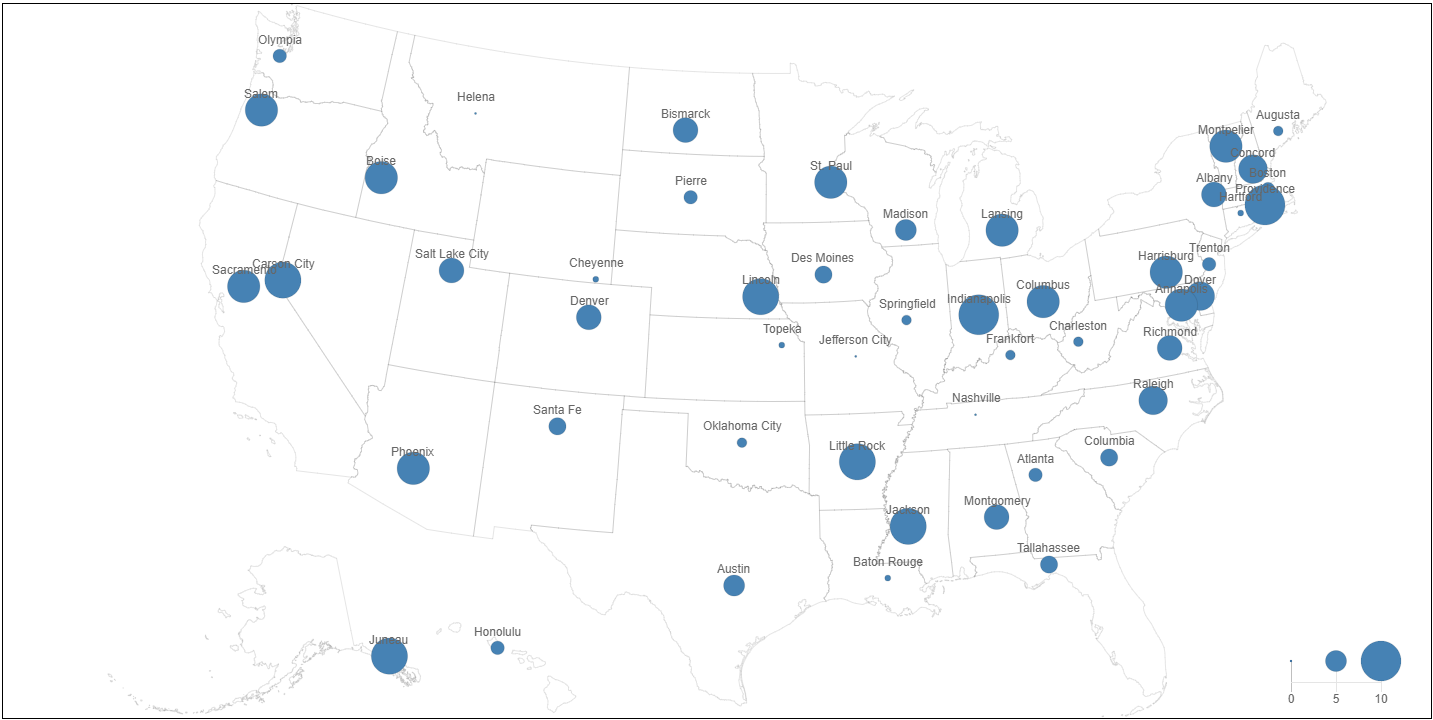
+
+[CodePen](https://codepen.io/sgratzl/pen/YzyJRvm)
+
+works great with https://github.com/chartjs/chartjs-plugin-datalabels
+
+## Install
+
+```sh
+npm install chart.js chartjs-chart-geo
+```
+
+## Usage
+
+see [Examples](./examples/)
+
+CodePens
+
+- [Choropleth](https://codepen.io/sgratzl/pen/gOaBQep)
+- [Earth Choropleth](https://codepen.io/sgratzl/pen/bGVmQKw)
+- [Bubble Map](https://codepen.io/sgratzl/pen/YzyJRvm)
+
+## Choropleth
+
+A choropleth map is a geo visualization where the area of a geometric feature encodes a value. For example [Choropleth](./examples/choropleth.md).
+
+::: warning
+This plugin is _not_ providing the geometric data files (like GeoJson or TopoJson) but they need to manually imported and defined.
+:::
+
+Each data point is an object with a feature and a corresponding value. see also [IChoroplethDataPoint](/api/interfaces/interface.IChoroplethDataPoint.html)
+
+### Configuration
+
+The controller has the following options [IChoroplethControllerDatasetOptions](/api/interfaces/interface.IChoroplethControllerDatasetOptions.html).
+In addition, the projection of the geometric feature to the pixel space is defined in the `projection` scale. see [IProjectionScaleOptions](/api/interfaces/interface.IProjectionScaleOptions.html) for available options. The conversion from a value to a color is performed by the `color` scale. see [IColorScaleOptions](/api/interfaces/interface.IColorScaleOptions.html) for available options.
+
+## Bubble Map
+
+A bubble is using the area / radius of a circle to encode a value at a specific latitude / longitude. For example [BubbleMap](./examples/bubbleMap.md). Therefore, a data point has to look like this [IBubbleMapDataPoint](/api/interfaces/interface.IBubbleMapDataPoint.html).
+
+### Configuration
+
+The controller has the following options [IBubbleMapControllerDatasetOptions](/api/interfaces/interface.IBubbleMapControllerDatasetOptions.html).
+In addition, the projection of the geometric feature to the pixel space is defined in the `projection` scale. see [IProjectionScaleOptions](/api/interfaces/interface.IProjectionScaleOptions.html) for available options. The conversion from a value to a radius / area is performed by the `size` scale. see [ISizeScaleOptions](/api/interfaces/interface.ISizeScaleOptions.html) for available options.
diff --git a/docs/index.md b/docs/index.md
new file mode 100644
index 0000000..52714db
--- /dev/null
+++ b/docs/index.md
@@ -0,0 +1,22 @@
+---
+# https://vitepress.dev/reference/default-theme-home-page
+layout: home
+
+hero:
+ name: 'chartjs-chart-geo'
+ text: 'chart.js plugin'
+ tagline: Chart.js module for charting maps
+ actions:
+ - theme: brand
+ text: Getting Started
+ link: /getting-started
+ - theme: alt
+ text: Examples
+ link: /examples/
+ - theme: alt
+ text: API
+ link: /api/
+# features:
+# - title: Feature A
+# details: Lorem ipsum dolor sit amet, consectetur adipiscing elit
+---
diff --git a/docs/related.md b/docs/related.md
new file mode 100644
index 0000000..bf01c2d
--- /dev/null
+++ b/docs/related.md
@@ -0,0 +1,15 @@
+---
+title: Related Plugins
+---
+
+There are several related chart.js plugins providing additional functionality and chart types:
+
+- [chartjs-chart-boxplot](https://github.com/sgratzl/chartjs-chart-boxplot) for rendering boxplots and violin charts
+- [chartjs-chart-error-bars](https://github.com/sgratzl/chartjs-chart-error-bars) for rendering errors bars to bars and line charts
+- [chartjs-chart-funnel](https://github.com/sgratzl/chartjs-chart-funnel) for rendering funnel charts
+- [chartjs-chart-geo](https://github.com/sgratzl/chartjs-chart-geo) for rendering map, bubble maps, and choropleth charts
+- [chartjs-chart-graph](https://github.com/sgratzl/chartjs-chart-graph) for rendering graphs, trees, and networks
+- [chartjs-chart-pcp](https://github.com/sgratzl/chartjs-chart-pcp) for rendering parallel coordinate plots
+- [chartjs-chart-venn](https://github.com/sgratzl/chartjs-chart-venn) for rendering venn and euler diagrams
+- [chartjs-chart-wordcloud](https://github.com/sgratzl/chartjs-chart-wordcloud) for rendering word clouds
+- [chartjs-plugin-hierarchical](https://github.com/sgratzl/chartjs-plugin-hierarchical) for rendering hierarchical categorical axes which can be expanded and collapsed