You signed in with another tab or window. Reload to refresh your session.You signed out in another tab or window. Reload to refresh your session.You switched accounts on another tab or window. Reload to refresh your session.Dismiss alert
functioncreateStore(reducer,preloadedState,enhancer){// 删除了一些参数矫正的代码if(typeofenhancer!=='undefined'){if(typeofenhancer!=='function'){thrownewError('Expected the enhancer to be a function.')}// store enhancer的使用,也就是使用传入的中间件// enhancer就是后续会提到的applyMiddleware函数returnenhancer(createStore)(reducer,preloadedState)}letcurrentReducer=reducerletcurrentState=preloadedStateletcurrentListeners=[]letnextListeners=currentListenersletisDispatching=false// 这个方法用于保持nextListeners和currentListeners的同步// nextListeners 是从currentListeners浅拷贝来的。// 订阅的时候是操作nextListeners, 防止在dispatch的时候执行subscribe/unsubscribe带来的bug// 在dispatch 的时候会同步 currentListeners 和 nextListeners (currentListeners = nextListeners)functionensureCanMutateNextListeners(){if(nextListeners===currentListeners){nextListeners=currentListeners.slice()}}// 获取当前currentStatefunctiongetState(){if(isDispatching){thrownewError('You may not call store.getState() while the reducer is executing. '+'The reducer has already received the state as an argument. '+'Pass it down from the top reducer instead of reading it from the store.')}returncurrentState}// 订阅一个listener,是操作在nextListeners上的// 返回的是一个函数,用于删除这个订阅的listener,也是在nextListeners上操作的// 删除订阅不会在当前的dispatch中生效,而是会在下一次dispatch的时候生效functionsubscribe(listener){if(typeoflistener!=='function'){thrownewError('Expected the listener to be a function.')}if(isDispatching){thrownewError('You may not call store.subscribe() while the reducer is executing. '+'If you would like to be notified after the store has been updated, subscribe from a '+'component and invoke store.getState() in the callback to access the latest state. '+'See https://redux.js.org/api-reference/store#subscribelistener for more details.')}letisSubscribed=trueensureCanMutateNextListeners()nextListeners.push(listener)returnfunctionunsubscribe(){if(!isSubscribed){return}if(isDispatching){thrownewError('You may not unsubscribe from a store listener while the reducer is executing. '+'See https://redux.js.org/api-reference/store#subscribelistener for more details.')}isSubscribed=falseensureCanMutateNextListeners()constindex=nextListeners.indexOf(listener)nextListeners.splice(index,1)currentListeners=null}}// 触发action// 1. 执行reducer,更新state// 2. 执行订阅的listener// 返回的是传入的action, 用于调试记录functiondispatch(action){if(!isPlainObject(action)){thrownewError('Actions must be plain objects. '+'Use custom middleware for async actions.')}if(typeofaction.type==='undefined'){thrownewError('Actions may not have an undefined "type" property. '+'Have you misspelled a constant?')}if(isDispatching){thrownewError('Reducers may not dispatch actions.')}try{isDispatching=truecurrentState=currentReducer(currentState,action)}finally{isDispatching=false}constlisteners=(currentListeners=nextListeners)for(leti=0;i<listeners.length;i++){constlistener=listeners[i]listener()}returnaction}// 替换当前的reducerfunctionreplaceReducer(nextReducer){if(typeofnextReducer!=='function'){thrownewError('Expected the nextReducer to be a function.')}currentReducer=nextReducer// 触发一个内部的REPLACE actiondispatch({type: ActionTypes.REPLACE})}// 用于 observable/reactive librariesfunctionobservable(){constouterSubscribe=subscribereturn{subscribe(observer){if(typeofobserver!=='object'||observer===null){thrownewTypeError('Expected the observer to be an object.')}functionobserveState(){if(observer.next){observer.next(getState())}}observeState()constunsubscribe=outerSubscribe(observeState)return{ unsubscribe }},[$$observable](){returnthis}}}// 触发一个INIT action,用于创建初始state树dispatch({type: ActionTypes.INIT})return{
dispatch,
subscribe,
getState,
replaceReducer,[$$observable]: observable}}
applyMiddleware 详解
functionapplyMiddleware(...middlewares){// 返回的函数就是在createStore中调用的那个enhancerreturn(createStore)=>(...args)=>{// 创建store, 可以获取dispatch和getStateconststore=createStore(...args)letdispatch=()=>{thrownewError('Dispatching while constructing your middleware is not allowed. '+'Other middleware would not be applied to this dispatch.')}// 封装传入中间件的apiconstmiddlewareAPI={getState: store.getState,dispatch: (...args)=>dispatch(...args),}// 给中间件们绑定能dispatch和getState的apiconstchain=middlewares.map((middleware)=>middleware(middlewareAPI))// 从这里就可以看出middleware就是来加强dispatch的,在dispatch了一个action后搞点事情// compose的本质就是用于串联执行中间件// 这个dispatch也重写个middlewareAPI调用的dispatchdispatch=compose(...chain)(store.dispatch)return{
...store,
dispatch,}}}
Redux概念
Redux的核心本质就是一个发布订阅模式。
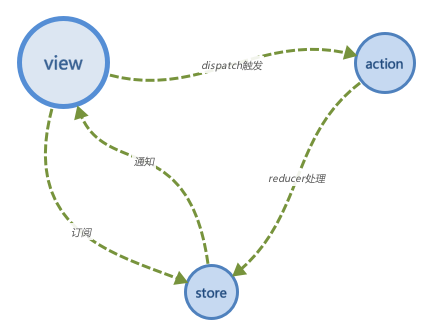
Redux的三个基本原则:
在Redux中一般只有一个store,用来存放数据。
更改状态的唯一方法是触发一个动作action,action 描述了这次修改行为的相关信息的对象。由于action只是简单的对象,因此可以将它们记录,序列化,存储并在以后进行调试。
为了描述 action 如何修改状态,需要使用reducer 函数。reducer 函数接收前一次的 state 和 action,返回新的 state, 而不是改变先前的state。只要传入相同 的state 和 action,无论reducer被调用多少次,那么就一定返回相同的结果。
Redux源码浅析
createStore
applyMiddleware 详解
详解
dispatch = compose(...chain)(store.dispatch)
compose的本质,组合多个函数, 用于串联执行中间件
先看看标准的middleware
去掉getState和dispatch的实现
dispatch = compose(...chain)(store.dispatch)
中的chain可以理解为:调用上面的chain
applyMiddleware的本质就是把中间件函数参数先一个一个的绑定好,来增强store 的dispatch的执行
combineReducers
The text was updated successfully, but these errors were encountered: