diff --git a/README.md b/README.md
index 942717f..556fdf1 100644
--- a/README.md
+++ b/README.md
@@ -5,7 +5,7 @@
[](https://pypi.org/project/lightweight-charts/)
[](https://python.org "Go to Python homepage")
[](https://github.com/louisnw01/lightweight-charts-python/blob/main/LICENSE)
-[](https://lightweight-charts-python.readthedocs.io/en/latest/docs.html)
+[](https://lightweight-charts-python.readthedocs.io/en/latest/common_methods.html)
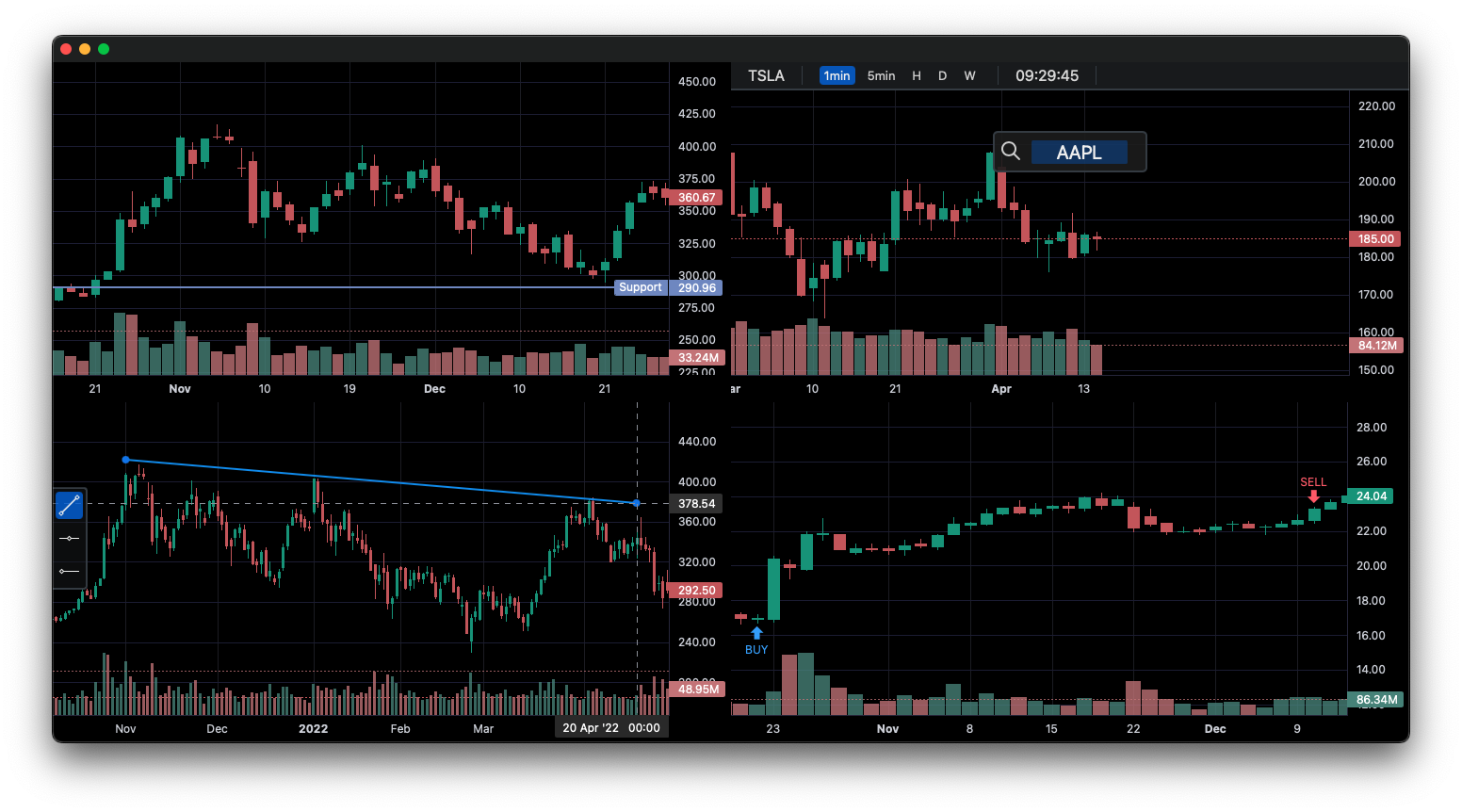
@@ -24,9 +24,10 @@ ___
1. Simple and easy to use.
2. Blocking or non-blocking GUI.
3. Streamlined for live data, with methods for updating directly from tick data.
-4. Multi-Pane Charts using the [`SubChart`](https://lightweight-charts-python.readthedocs.io/en/latest/docs.html#subchart).
+4. Multi-Pane Charts using the [`SubChart`](https://lightweight-charts-python.readthedocs.io/en/latest/common_methods.html#create-subchart-subchart).
5. The Toolbox, allowing for trendlines, rays and horizontal lines to be drawn directly onto charts.
-6. [Callbacks](https://lightweight-charts-python.readthedocs.io/en/latest/docs.html#callbacks) allowing for timeframe (1min, 5min, 30min etc.) selectors, searching, and more.
+6. [Callbacks](https://lightweight-charts-python.readthedocs.io/en/latest/callbacks.html) allowing for timeframe (1min, 5min, 30min etc.) selectors, searching, hotkeys, and more.
+7. Tables for watchlists, order entry, and trade management.
7. Direct integration of market data through [Polygon.io's](https://polygon.io/?utm_source=affiliate&utm_campaign=pythonlwcharts) market data API.
__Supports:__ Jupyter Notebooks, PyQt, wxPython, Streamlit, and asyncio.
@@ -194,9 +195,7 @@ ___
### 6. Callbacks:
```python
-import asyncio
import pandas as pd
-
from lightweight_charts import Chart
@@ -204,49 +203,45 @@ def get_bar_data(symbol, timeframe):
if symbol not in ('AAPL', 'GOOGL', 'TSLA'):
print(f'No data for "{symbol}"')
return pd.DataFrame()
- return pd.read_csv(f'bar_data/{symbol}_{timeframe}.csv')
+ return pd.read_csv(f'../examples/6_callbacks/bar_data/{symbol}_{timeframe}.csv')
class API:
def __init__(self):
self.chart = None # Changes after each callback.
- async def on_search(self, searched_string): # Called when the user searches.
+ def on_search(self, searched_string): # Called when the user searches.
new_data = get_bar_data(searched_string, self.chart.topbar['timeframe'].value)
if new_data.empty:
return
- self.chart.topbar['corner'].set(searched_string)
+ self.chart.topbar['symbol'].set(searched_string)
self.chart.set(new_data)
- async def on_timeframe_selection(self): # Called when the user changes the timeframe.
- new_data = get_bar_data(self.chart.topbar['corner'].value, self.chart.topbar['timeframe'].value)
+ def on_timeframe_selection(self): # Called when the user changes the timeframe.
+ new_data = get_bar_data(self.chart.topbar['symbol'].value, self.chart.topbar['timeframe'].value)
if new_data.empty:
return
- self.chart.set(new_data)
-
- async def on_horizontal_line_move(self, line_id, price):
+ self.chart.set(new_data, True)
+
+ def on_horizontal_line_move(self, line_id, price):
print(f'Horizontal line moved to: {price}')
-async def main():
+if __name__ == '__main__':
api = API()
chart = Chart(api=api, topbar=True, searchbox=True, toolbox=True)
chart.legend(True)
- chart.topbar.textbox('corner', 'TSLA')
+ chart.topbar.textbox('symbol', 'TSLA')
chart.topbar.switcher('timeframe', api.on_timeframe_selection, '1min', '5min', '30min', default='5min')
df = get_bar_data('TSLA', '5min')
chart.set(df)
-
- chart.horizontal_line(200, interactive=True)
-
- await chart.show_async(block=True)
+ chart.horizontal_line(200, interactive=True)
-if __name__ == '__main__':
- asyncio.run(main())
+ chart.show(block=True)
```
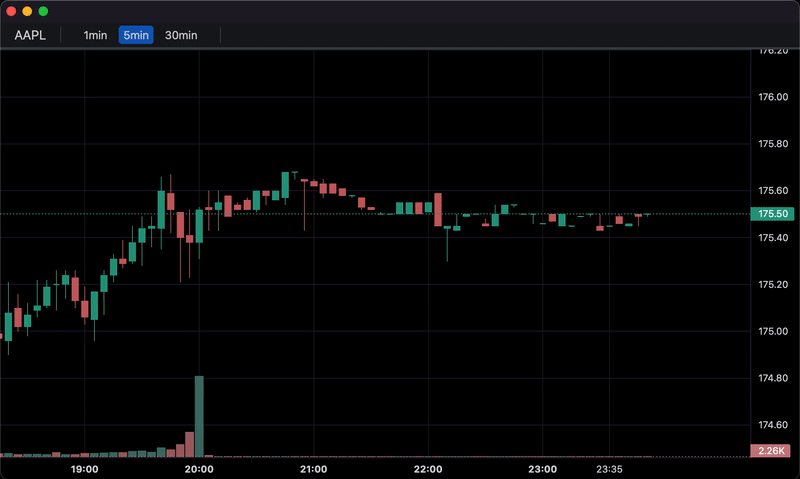
@@ -254,7 +249,7 @@ ___
-[](https://lightweight-charts-python.readthedocs.io/en/latest/docs.html)
+[](https://lightweight-charts-python.readthedocs.io/en/latest/common_methods.html)
Inquiries: [shaders_worker_0e@icloud.com](mailto:shaders_worker_0e@icloud.com)
___
diff --git a/cover.png b/cover.png
index 291ef1b..890b8c9 100644
Binary files a/cover.png and b/cover.png differ
diff --git a/docs/source/callbacks.md b/docs/source/callbacks.md
new file mode 100644
index 0000000..48ee7fd
--- /dev/null
+++ b/docs/source/callbacks.md
@@ -0,0 +1,132 @@
+# Callbacks
+
+The `Chart` object allows for asynchronous and synchronous callbacks to be passed back to python, allowing for more sophisticated chart layouts including searching, timeframe selectors text boxes, and hotkeys using the `add_hotkey` method.
+
+`QtChart`and `WxChart` can also use callbacks.
+
+A variety of the parameters below should be passed to the Chart upon decaration.
+* `api`: The class object that the fixed callbacks will always be emitted to.
+* `topbar`: Adds a [TopBar](#topbar) to the `Chart` or `SubChart` and allows use of the `create_switcher` method.
+* `searchbox`: Adds a search box onto the `Chart` or `SubChart` that is activated by typing.
+
+___
+## How to use Callbacks
+
+Fixed Callbacks are emitted to the class given as the `api` parameter shown above.
+
+Take a look at this minimal example:
+
+```python
+class API:
+ def __init__(self):
+ self.chart = None
+
+ def on_search(self, string):
+ print(f'Search Text: "{string}" | Chart/SubChart ID: "{self.chart.id}"')
+```
+Upon searching in a pane, the expected output would be akin to:
+```
+Search Text: "AAPL" | Chart/SubChart ID: "window.blyjagcr"
+```
+The ID shown above will change depending upon which pane was used to search, due to the instance of `self.chart` dynamically updating to the latest pane which triggered the callback.
+`self.chart` will update upon each callback, allowing for access to the specific pane in question.
+
+```{important}
+* When using `show` rather than `show_async`, block should be set to `True` (`chart.show(block=True)`).
+* `API` class methods can be either coroutines or normal methods.
+* Non fixed callbacks (switchers, hotkeys) can be methods, coroutines, or regular functions.
+```
+
+There are certain callbacks which are always emitted to a specifically named method of API:
+* Search callbacks: `on_search`
+* Interactive Horizontal Line callbacks: `on_horizontal_line_move`
+
+___
+
+## `TopBar`
+The `TopBar` class represents the top bar shown on the chart when using callbacks:
+
+
+
+This class is accessed from the `topbar` attribute of the chart object (`chart.topbar.
`), after setting the topbar parameter to `True` upon declaration of the chart.
+
+Switchers and text boxes can be created within the top bar, and their instances can be accessed through the `topbar` dictionary. For example:
+
+```python
+chart = Chart(api=api, topbar=True)
+
+chart.topbar.textbox('symbol', 'AAPL') # Declares a textbox displaying 'AAPL'.
+print(chart.topbar['symbol'].value) # Prints the value within ('AAPL')
+
+chart.topbar['symbol'].set('MSFT') # Sets the 'symbol' textbox to 'MSFT'
+print(chart.topbar['symbol'].value) # Prints the value again ('MSFT')
+```
+___
+
+### `switcher`
+`name: str` | `method: function` | `*options: str` | `default: str`
+
+* `name`: the name of the switcher which can be used to access it from the `topbar` dictionary.
+* `method`: The function from the `api` class given to the constructor that will receive the callback.
+* `options`: The strings to be displayed within the switcher. This may be a variety of timeframes, security types, or whatever needs to be updated directly from the chart.
+* `default`: The initial switcher option set.
+___
+
+### `textbox`
+`name: str` | `initial_text: str`
+
+* `name`: the name of the text box which can be used to access it from the `topbar` dictionary.
+* `initial_text`: The text to show within the text box.
+___
+
+## Callbacks Example:
+
+```python
+import pandas as pd
+from lightweight_charts import Chart
+
+
+def get_bar_data(symbol, timeframe):
+ if symbol not in ('AAPL', 'GOOGL', 'TSLA'):
+ print(f'No data for "{symbol}"')
+ return pd.DataFrame()
+ return pd.read_csv(f'../examples/6_callbacks/bar_data/{symbol}_{timeframe}.csv')
+
+
+class API:
+ def __init__(self):
+ self.chart = None # Changes after each callback.
+
+ def on_search(self, searched_string): # Called when the user searches.
+ new_data = get_bar_data(searched_string, self.chart.topbar['timeframe'].value)
+ if new_data.empty:
+ return
+ self.chart.topbar['symbol'].set(searched_string)
+ self.chart.set(new_data)
+
+ def on_timeframe_selection(self): # Called when the user changes the timeframe.
+ new_data = get_bar_data(self.chart.topbar['symbol'].value, self.chart.topbar['timeframe'].value)
+ if new_data.empty:
+ return
+ self.chart.set(new_data, True)
+
+ def on_horizontal_line_move(self, line_id, price):
+ print(f'Horizontal line moved to: {price}')
+
+
+if __name__ == '__main__':
+ api = API()
+
+ chart = Chart(api=api, topbar=True, searchbox=True, toolbox=True)
+ chart.legend(True)
+
+ chart.topbar.textbox('symbol', 'TSLA')
+ chart.topbar.switcher('timeframe', api.on_timeframe_selection, '1min', '5min', '30min', default='5min')
+
+ df = get_bar_data('TSLA', '5min')
+ chart.set(df)
+
+ chart.horizontal_line(200, interactive=True)
+
+ chart.show(block=True)
+```
diff --git a/docs/source/charts.md b/docs/source/charts.md
new file mode 100644
index 0000000..322bd5c
--- /dev/null
+++ b/docs/source/charts.md
@@ -0,0 +1,209 @@
+# Charts
+
+This page contains a reference to all chart objects that can be used within the library. They all have access to the common methods.
+
+___
+
+## Chart
+`volume_enabled: bool` | `width: int` | `height: int` | `x: int` | `y: int` | `on_top: bool` | `maximize: bool` | `debug: bool` |
+`api: object` | `topbar: bool` | `searchbox: bool` | `toolbox: bool`
+
+The main object used for the normal functionality of lightweight-charts-python, built on the pywebview library.
+
+```{important}
+The `Chart` object should be defined within an `if __name__ == '__main__'` block.
+```
+___
+
+### `show`
+`block: bool`
+
+Shows the chart window, blocking until the chart has loaded. If `block` is enabled, the method will block code execution until the window is closed.
+___
+
+### `hide`
+
+Hides the chart window, which can be later shown by calling `chart.show()`.
+___
+
+### `exit`
+
+Exits and destroys the chart window.
+
+___
+
+### `show_async`
+`block: bool`
+
+Show the chart asynchronously.
+___
+
+### `screenshot`
+`-> bytes`
+
+Takes a screenshot of the chart, and returns a bytes object containing the image. For example:
+
+```python
+if __name__ == '__main__':
+ chart = Chart()
+ df = pd.read_csv('ohlcv.csv')
+ chart.set(df)
+ chart.show()
+
+ img = chart.screenshot()
+ with open('screenshot.png', 'wb') as f:
+ f.write(img)
+```
+
+```{important}
+This method should be called after the chart window has loaded.
+```
+___
+
+## QtChart
+`widget: QWidget` | `volume_enabled: bool`
+
+The `QtChart` object allows the use of charts within a `QMainWindow` object, and has similar functionality to the `Chart` and `ChartAsync` objects for manipulating data, configuring and styling.
+
+Callbacks can be recieved through the Qt event loop.
+___
+
+### `get_webview`
+
+`-> QWebEngineView`
+
+Returns the `QWebEngineView` object.
+
+___
+### Example:
+
+```python
+import pandas as pd
+from PyQt5.QtWidgets import QApplication, QMainWindow, QVBoxLayout, QWidget
+
+from lightweight_charts.widgets import QtChart
+
+app = QApplication([])
+window = QMainWindow()
+layout = QVBoxLayout()
+widget = QWidget()
+widget.setLayout(layout)
+
+window.resize(800, 500)
+layout.setContentsMargins(0, 0, 0, 0)
+
+chart = QtChart(widget)
+
+df = pd.read_csv('ohlcv.csv')
+chart.set(df)
+
+layout.addWidget(chart.get_webview())
+
+window.setCentralWidget(widget)
+window.show()
+
+app.exec_()
+```
+___
+
+## WxChart
+`parent: wx.Panel` | `volume_enabled: bool`
+
+The WxChart object allows the use of charts within a `wx.Frame` object, and has similar functionality to the `Chart` and `ChartAsync` objects for manipulating data, configuring and styling.
+
+Callbacks can be recieved through the Wx event loop.
+___
+
+### `get_webview`
+`-> wx.html2.WebView`
+
+Returns a `wx.html2.WebView` object which can be used to for positioning and styling within wxPython.
+___
+
+### Example:
+
+```python
+import wx
+import pandas as pd
+
+from lightweight_charts.widgets import WxChart
+
+
+class MyFrame(wx.Frame):
+ def __init__(self):
+ super().__init__(None)
+ self.SetSize(1000, 500)
+
+ panel = wx.Panel(self)
+ sizer = wx.BoxSizer(wx.VERTICAL)
+ panel.SetSizer(sizer)
+
+ chart = WxChart(panel)
+
+ df = pd.read_csv('ohlcv.csv')
+ chart.set(df)
+
+ sizer.Add(chart.get_webview(), 1, wx.EXPAND | wx.ALL)
+ sizer.Layout()
+ self.Show()
+
+
+if __name__ == '__main__':
+ app = wx.App()
+ frame = MyFrame()
+ app.MainLoop()
+
+```
+___
+
+## StreamlitChart
+`parent: wx.Panel` | `volume_enabled: bool`
+
+The `StreamlitChart` object allows the use of charts within a Streamlit app, and has similar functionality to the `Chart` object for manipulating data, configuring and styling.
+
+This object only supports the displaying of **static** data, and should not be used with the `update_from_tick` or `update` methods. Every call to the chart object must occur **before** calling `load`.
+___
+
+### `load`
+
+Loads the chart into the Streamlit app. This should be called after setting, styling, and configuring the chart, as no further calls to the `StreamlitChart` will be acknowledged.
+___
+
+### Example:
+```python
+import pandas as pd
+from lightweight_charts.widgets import StreamlitChart
+
+chart = StreamlitChart(width=900, height=600)
+
+df = pd.read_csv('ohlcv.csv')
+chart.set(df)
+
+chart.load()
+```
+___
+
+## JupyterChart
+
+The `JupyterChart` object allows the use of charts within a notebook, and has similar functionality to the `Chart` object for manipulating data, configuring and styling.
+
+This object only supports the displaying of **static** data, and should not be used with the `update_from_tick` or `update` methods. Every call to the chart object must occur **before** calling `load`.
+___
+
+### `load`
+
+Renders the chart. This should be called after setting, styling, and configuring the chart, as no further calls to the `JupyterChart` will be acknowledged.
+___
+
+### Example:
+```python
+import pandas as pd
+from lightweight_charts import JupyterChart
+
+chart = JupyterChart()
+
+df = pd.read_csv('ohlcv.csv')
+chart.set(df)
+
+chart.load()
+```
diff --git a/docs/source/common_methods.md b/docs/source/common_methods.md
new file mode 100644
index 0000000..70965bf
--- /dev/null
+++ b/docs/source/common_methods.md
@@ -0,0 +1,362 @@
+# Common Methods
+The methods below can be used within all chart objects.
+
+___
+## `set`
+`data: pd.DataFrame` `render_drawings: bool`
+
+Sets the initial data for the chart. The data must be given as a DataFrame, with the columns:
+
+`time | open | high | low | close | volume`
+
+The `time` column can also be named `date` or be the index, and the `volume` column can be omitted if volume is not enabled. Column names are not case sensitive.
+
+If `render_drawings` is `True`, any drawings made using the `toolbox` will be redrawn with the new data. This is designed to be used when switching to a different timeframe of the same symbol.
+
+```{important}
+the `time` column must have rows all of the same timezone and locale. This is particularly noticeable for data which crosses over daylight saving hours on data with intervals of less than 1 day. Errors are likely to be raised if they are not converted beforehand.
+```
+
+An empty `DataFrame` object or `None` can also be given to this method, which will erase all candle and volume data displayed on the chart.
+___
+
+## `update`
+`series: pd.Series`
+
+Updates the chart data from a `pd.Series` object. The bar should contain values with labels akin to `set`.
+___
+
+## `update_from_tick`
+`series: pd.Series` | `cumulative_volume: bool`
+
+Updates the chart from a tick. The series should use the labels:
+
+`time | price | volume`
+
+As before, the `time` can also be named `date`, and the `volume` can be omitted if volume is not enabled. The `time` column can also be the name of the Series object.
+
+```{information}
+The provided ticks do not need to be rounded to an interval (1 min, 5 min etc.), as the library handles this automatically.
+```
+
+If `cumulative_volume` is used, the volume data given will be added onto the latest bar of volume data.
+___
+
+## `create_line` (Line)
+`color: str` | `width: int` | `price_line: bool` | `price_label: bool` | `-> Line`
+
+Creates and returns a `Line` object, representing a `LineSeries` object in Lightweight Charts and can be used to create indicators. As well as the methods described below, the `Line` object also has access to:
+[`title`](#title), [`marker`](#marker), [`horizontal_line`](#horizontal-line) [`hide_data`](#hide-data), [`show_data`](#show-data) and[`price_line`](#price-line).
+
+Its instance should only be accessed from this method.
+
+
+### `set`
+`data: pd.DataFrame` `name: str`
+
+Sets the data for the line.
+
+When not using the `name` parameter, the columns should be named: `time | value` (Not case sensitive).
+
+Otherwise, the method will use the column named after the string given in `name`. This name will also be used within the legend of the chart. For example:
+```python
+line = chart.create_line()
+
+# DataFrame with columns: date | SMA 50
+df = pd.read_csv('sma50.csv')
+
+line.set(df, name='SMA 50')
+```
+
+
+### `update`
+`series: pd.Series`
+
+Updates the data for the line.
+
+This should be given as a Series object, with labels akin to the `line.set()` function.
+
+
+### `delete`
+Irreversibly deletes the line.
+
+___
+
+## `lines`
+`-> List[Line]`
+
+Returns a list of all lines for the chart or subchart.
+___
+
+## `trend_line`
+`start_time: str/datetime` | `start_value: float/int` | `end_time: str/datetime` | `end_value: float/int` | `color: str` | `width: int` | `-> Line`
+
+Creates a trend line, drawn from the first point (`start_time`, `start_value`) to the last point (`end_time`, `end_value`).
+___
+
+## `ray_line`
+`start_time: str/datetime` | `value: float/int` | `color: str` | `width: int` | `-> Line`
+
+Creates a ray line, drawn from the first point (`start_time`, `value`) and onwards.
+___
+
+## `marker`
+`time: datetime` | `position: 'above'/'below'/'inside'` | `shape: 'arrow_up'/'arrow_down'/'circle'/'square'` | `color: str` | `text: str` | `-> str`
+
+Adds a marker to the chart, and returns its id.
+
+If the `time` parameter is not given, the marker will be placed at the latest bar.
+
+When using multiple markers, they should be placed in chronological order or display bugs may be present.
+___
+
+## `remove_marker`
+`marker_id: str`
+
+Removes the marker with the given id.
+
+Usage:
+```python
+marker = chart.marker(text='hello_world')
+chart.remove_marker(marker)
+```
+___
+
+## `horizontal_line` (HorizontalLine)
+`price: float/int` | `color: str` | `width: int` | `style: 'solid'/'dotted'/'dashed'/'large_dashed'/'sparse_dotted'` | `text: str` | `axis_label_visible: bool` | `interactive: bool` | `-> HorizontalLine`
+
+Places a horizontal line at the given price, and returns a `HorizontalLine` object, representing a `PriceLine` in Lightweight Charts.
+
+If `interactive` is set to `True`, this horizontal line can be edited on the chart. Upon its movement a callback will also be emitted to an `on_horizontal_line_move` method, containing its ID and price. The toolbox should be enabled during its usage. It is designed to be used to update an order (limit, stop, etc.) directly on the chart.
+
+
+### `update`
+`price: float/int`
+
+Updates the price of the horizontal line.
+
+### `label`
+`text: str`
+
+Updates the label of the horizontal line.
+
+### `delete`
+
+Irreversibly deletes the horizontal line.
+___
+
+## `remove_horizontal_line`
+`price: float/int`
+
+Removes a horizontal line at the given price.
+___
+
+## `clear_markers`
+
+Clears the markers displayed on the data.
+___
+
+## `clear_horizontal_lines`
+
+Clears the horizontal lines displayed on the data.
+___
+
+## `precision`
+`precision: int`
+
+Sets the precision of the chart based on the given number of decimal places.
+___
+
+## `price_scale`
+`mode: 'normal'/'logarithmic'/'percentage'/'index100'` | `align_labels: bool` | `border_visible: bool` | `border_color: str` | `text_color: str` | `entire_text_only: bool` | `ticks_visible: bool` | `scale_margin_top: float` | `scale_margin_bottom: float`
+
+Price scale options for the chart.
+___
+
+## `time_scale`
+`right_offset: int` | `min_bar_spacing: float` | `visible: bool` | `time_visible: bool` | `seconds_visible: bool` | `border_visible: bool` | `border_color: str`
+
+Timescale options for the chart.
+___
+
+## `layout`
+`background_color: str` | `text_color: str` | `font_size: int` | `font_family: str`
+
+Global layout options for the chart.
+___
+
+## `grid`
+`vert_enabled: bool` | `horz_enabled: bool` | `color: str` | `style: 'solid'/'dotted'/'dashed'/'large_dashed'/'sparse_dotted'`
+
+Grid options for the chart.
+___
+
+## `candle_style`
+`up_color: str` | `down_color: str` | `wick_enabled: bool` | `border_enabled: bool` | `border_up_color: str` | `border_down_color: str` | `wick_up_color: str` | `wick_down_color: str`
+
+ Candle styling for each of the candle's parts (border, wick).
+
+```{admonition} Color Formats
+:class: note
+
+Throughout the library, colors should be given as either:
+* rgb: `rgb(100, 100, 100)`
+* rgba: `rgba(100, 100, 100, 0.7)`
+* hex: `#32a852`
+```
+___
+
+## `volume_config`
+`scale_margin_top: float` | `scale_margin_bottom: float` | `up_color: str` | `down_color: str`
+
+Volume config options.
+
+```{important}
+The float values given to scale the margins must be greater than 0 and less than 1.
+```
+___
+
+## `crosshair`
+`mode` | `vert_visible: bool` | `vert_width: int` | `vert_color: str` | `vert_style: str` | `vert_label_background_color: str` | `horz_visible: bool` | `horz_width: int` | `horz_color: str` | `horz_style: str` | `horz_label_background_color: str`
+
+Crosshair formatting for its vertical and horizontal axes.
+
+`vert_style` and `horz_style` should be given as one of: `'solid'/'dotted'/'dashed'/'large_dashed'/'sparse_dotted'`
+___
+
+## `watermark`
+`text: str` | `font_size: int` | `color: str`
+
+Overlays a watermark on top of the chart.
+___
+
+## `legend`
+`visible: bool` | `ohlc: bool` | `percent: bool` | `lines: bool` | `color: str` | `font_size: int` | `font_family: str`
+
+Configures the legend of the chart.
+___
+
+## `spinner`
+`visible: bool`
+
+Shows a loading spinner on the chart, which can be used to visualise the loading of large datasets, API calls, etc.
+___
+
+## `price_line`
+`label_visible: bool` | `line_visible: bool` | `title: str`
+
+Configures the visibility of the last value price line and its label.
+___
+
+## `fit`
+
+Attempts to fit all data displayed on the chart within the viewport (`fitContent()`).
+___
+
+## `hide_data`
+
+Hides the candles on the chart.
+___
+
+## `show_data`
+
+Shows the hidden candles on the chart.
+___
+
+## `add_hotkey`
+`modifier: 'ctrl'/'shift'/'alt'/'meta'` | `key: str/int/tuple` | `method: object`
+
+Adds a global hotkey to the chart window, which will execute the method or function given.
+
+When using a number in `key`, it should be given as an integer. If multiple key commands are needed for the same function, you can pass a tuple to `key`. For example:
+
+```python
+def place_buy_order(key):
+ print(f'Buy {key} shares.')
+
+
+def place_sell_order(key):
+ print(f'Sell all shares, because I pressed {key}.')
+
+
+chart.add_hotkey('shift', (1, 2, 3), place_buy_order)
+chart.add_hotkey('shift', 'X', place_sell_order)
+```
+
+___
+
+## `create_table`
+`width: int/float` | `height: int/float` | `headings: tuple[str]` | `widths: tuple[float]` | `alignments: tuple[str]` | `position: 'left'/'right'/'top'/'bottom'` | `draggable: bool` | `method: object`
+
+Creates and returns a [`Table`](https://lightweight-charts-python.readthedocs.io/en/latest/tables.html) object.
+___
+
+## `create_subchart` (SubChart)
+`volume_enabled: bool` | `position: 'left'/'right'/'top'/'bottom'`, `width: float` | `height: float` | `sync: bool/str` | `-> SubChart`
+
+Creates and returns a `SubChart` object, placing it adjacent to the previous `Chart` or `SubChart`. This allows for the use of multiple chart panels within the same `Chart` window. Its instance should only be accessed by using this method.
+
+`position`: specifies how the Subchart will float.
+
+`height` | `width`: Specifies the size of the Subchart, where `1` is the width/height of the window (100%)
+
+`sync`: If given as `True`, the Subchart's timescale and crosshair will follow that of the declaring `Chart` or `SubChart`. If a `str` is passed, the `SubChart` will follow the panel with the given id. Chart ids can be accessed from the`chart.id` and `subchart.id` attributes.
+
+```{important}
+`width` and `height` should be given as a number between 0 and 1.
+```
+
+`SubCharts` are arranged horizontally from left to right. When the available space is no longer sufficient, the subsequent `SubChart` will be positioned on a new row, starting from the left side.
+
+### Grid of 4 Example:
+
+```python
+import pandas as pd
+from lightweight_charts import Chart
+
+if __name__ == '__main__':
+ chart = Chart(inner_width=0.5, inner_height=0.5)
+ chart2 = chart.create_subchart(position='right', width=0.5, height=0.5)
+ chart3 = chart.create_subchart(position='left', width=0.5, height=0.5)
+ chart4 = chart.create_subchart(position='right', width=0.5, height=0.5)
+
+ chart.watermark('1')
+ chart2.watermark('2')
+ chart3.watermark('3')
+ chart4.watermark('4')
+
+ df = pd.read_csv('ohlcv.csv')
+ chart.set(df)
+ chart2.set(df)
+ chart3.set(df)
+ chart4.set(df)
+
+ chart.show(block=True)
+
+```
+
+
+### Synced Line Chart Example:
+
+```python
+import pandas as pd
+from lightweight_charts import Chart
+
+if __name__ == '__main__':
+ chart = Chart(inner_width=1, inner_height=0.8)
+ chart.time_scale(visible=False)
+
+ chart2 = chart.create_subchart(width=1, height=0.2, sync=True, volume_enabled=False)
+
+ df = pd.read_csv('ohlcv.csv')
+ df2 = pd.read_csv('rsi.csv')
+
+ chart.set(df)
+ line = chart2.create_line()
+ line.set(df2)
+
+ chart.show(block=True)
+
+```
+
+
diff --git a/docs/source/conf.py b/docs/source/conf.py
index b78e2d2..57feba5 100644
--- a/docs/source/conf.py
+++ b/docs/source/conf.py
@@ -1,7 +1,7 @@
project = 'lightweight-charts-python'
copyright = '2023, louisnw'
author = 'louisnw'
-release = '1.0.14.4'
+release = '1.0.15'
extensions = ["myst_parser"]
diff --git a/docs/source/docs.md b/docs/source/docs.md
deleted file mode 100644
index 9f1dbdf..0000000
--- a/docs/source/docs.md
+++ /dev/null
@@ -1,773 +0,0 @@
-# Docs
-
-[](https://github.com/louisnw01/lightweight-charts-python "Go to GitHub repo")
-[](https://pypi.org/project/lightweight-charts/)
-[](https://python.org "Go to Python homepage")
-[](https://github.com/louisnw01/lightweight-charts-python/blob/main/LICENSE)
-[](https://github.com/louisnw01/lightweight-charts-python)
-[](https://github.com/louisnw01/lightweight-charts-python)
-___
-
-## Common Methods
-The methods below can be used within all chart objects.
-
-___
-### `set`
-`data: pd.DataFrame` `render_drawings: bool`
-
-Sets the initial data for the chart.
-
-The data must be given as a DataFrame, with the columns:
-
-`time | open | high | low | close | volume`
-
-The `time` column can also be named `date` or be the index, and the `volume` column can be omitted if volume is not enabled.
-
-Column names are not case sensitive.
-
-If `render_drawings` is `True`, any drawings made using the `toolbox` will be redrawn with the new data. This is designed to be used when switching to a different timeframe of the same symbol.
-
-```{important}
-the `time` column must have rows all of the same timezone and locale. This is particularly noticeable for data which crosses over daylight saving hours on data with intervals of less than 1 day. Errors are likely to be raised if they are not converted beforehand.
-```
-
-An empty `DataFrame` object can also be given to this method, which will erase all candle and volume data displayed on the chart.
-___
-
-### `update`
-`series: pd.Series`
-
-Updates the chart data from a given bar.
-
-The bar should contain values with labels of the same name as the columns required for using `chart.set()`.
-___
-
-### `update_from_tick`
-`series: pd.Series` | `cumulative_volume: bool`
-
-Updates the chart from a tick.
-
-The series should use the labels:
-
-`time | price | volume`
-
-As before, the `time` can also be named `date`, and the `volume` can be omitted if volume is not enabled.
-
-```{information}
-The provided ticks do not need to be rounded to an interval (1 min, 5 min etc.), as the library handles this automatically.```````
-```
-
-If `cumulative_volume` is used, the volume data given will be added onto the latest bar of volume data.
-___
-
-### `create_line`
-`color: str` | `width: int` | `price_line: bool` | `price_label: bool` | `-> Line`
-
-Creates and returns a [Line](#line) object.
-___
-
-### `lines`
-`-> List[Line]`
-
-Returns a list of all lines for the chart or subchart.
-___
-
-### `trend_line`
-`start_time: str/datetime` | `start_value: float/int` | `end_time: str/datetime` | `end_value: float/int` | `color: str` | `width: int` | `-> Line`
-
-Creates a trend line, drawn from the first point (`start_time`, `start_value`) to the last point (`end_time`, `end_value`).
-___
-
-### `ray_line`
-`start_time: str/datetime` | `value: float/int` | `color: str` | `width: int` | `-> Line`
-
-Creates a ray line, drawn from the first point (`start_time`, `value`) and onwards.
-___
-
-### `marker`
-`time: datetime` | `position: 'above'/'below'/'inside'` | `shape: 'arrow_up'/'arrow_down'/'circle'/'square'` | `color: str` | `text: str` | `-> str`
-
-Adds a marker to the chart, and returns its id.
-
-If the `time` parameter is not given, the marker will be placed at the latest bar.
-
-When using multiple markers, they should be placed in chronological order or display bugs may be present.
-___
-
-### `remove_marker`
-`marker_id: str`
-
-Removes the marker with the given id.
-
-Usage:
-```python
-marker = chart.marker(text='hello_world')
-chart.remove_marker(marker)
-```
-___
-
-### `horizontal_line`
-`price: float/int` | `color: str` | `width: int` | `style: 'solid'/'dotted'/'dashed'/'large_dashed'/'sparse_dotted'` | `text: str` | `axis_label_visible: bool` | `interactive: bool` | `-> HorizontalLine`
-
-Places a horizontal line at the given price, and returns a HorizontalLine object.
-
-If `interactive` is set to `True`, this horizontal line can be edited on the chart. Upon its movement a callback will also be emitted to an `on_horizontal_line_move` method, containing its ID and price. The toolbox should be enabled during its usage. It is designed to be used to update an order (limit, stop, etc.) directly on the chart.
-___
-
-### `remove_horizontal_line`
-`price: float/int`
-
-Removes a horizontal line at the given price.
-___
-
-### `clear_markers`
-
-Clears the markers displayed on the data.
-___
-
-### `clear_horizontal_lines`
-
-Clears the horizontal lines displayed on the data.
-___
-
-### `precision`
-`precision: int`
-
-Sets the precision of the chart based on the given number of decimal places.
-___
-
-### `price_scale`
-`mode: 'normal'/'logarithmic'/'percentage'/'index100'` | `align_labels: bool` | `border_visible: bool` | `border_color: str` | `text_color: str` | `entire_text_only: bool` | `ticks_visible: bool` | `scale_margin_top: float` | `scale_margin_bottom: float`
-
-Price scale options for the chart.
-___
-
-### `time_scale`
-`right_offset: int` | `min_bar_spacing: float` | `visible: bool` | `time_visible: bool` | `seconds_visible: bool` | `border_visible: bool` | `border_color: str`
-
-Timescale options for the chart.
-___
-
-### `layout`
-`background_color: str` | `text_color: str` | `font_size: int` | `font_family: str`
-
-Global layout options for the chart.
-___
-
-### `grid`
-`vert_enabled: bool` | `horz_enabled: bool` | `color: str` | `style: 'solid'/'dotted'/'dashed'/'large_dashed'/'sparse_dotted'`
-
-Grid options for the chart.
-___
-
-### `candle_style`
-`up_color: str` | `down_color: str` | `wick_enabled: bool` | `border_enabled: bool` | `border_up_color: str` | `border_down_color: str` | `wick_up_color: str` | `wick_down_color: str`
-
- Candle styling for each of the candle's parts (border, wick).
-
-```{admonition} Color Formats
-:class: note
-
-Throughout the library, colors should be given as either:
-* rgb: `rgb(100, 100, 100)`
-* rgba: `rgba(100, 100, 100, 0.7)`
-* hex: `#32a852`
-```
-___
-
-### `volume_config`
-`scale_margin_top: float` | `scale_margin_bottom: float` | `up_color: str` | `down_color: str`
-
-Volume config options.
-
-```{important}
-The float values given to scale the margins must be greater than 0 and less than 1.
-```
-___
-
-### `crosshair`
-`mode` | `vert_visible: bool` | `vert_width: int` | `vert_color: str` | `vert_style: str` | `vert_label_background_color: str` | `horz_visible: bool` | `horz_width: int` | `horz_color: str` | `horz_style: str` | `horz_label_background_color: str`
-
-Crosshair formatting for its vertical and horizontal axes.
-
-`vert_style` and `horz_style` should be given as one of: `'solid'/'dotted'/'dashed'/'large_dashed'/'sparse_dotted'`
-___
-
-### `watermark`
-`text: str` | `font_size: int` | `color: str`
-
-Overlays a watermark on top of the chart.
-___
-
-### `legend`
-`visible: bool` | `ohlc: bool` | `percent: bool` | `lines: bool` | `color: str` | `font_size: int` | `font_family: str`
-
-Configures the legend of the chart.
-___
-
-### `spinner`
-`visible: bool`
-
-Shows a loading spinner on the chart, which can be used to visualise the loading of large datasets, API calls, etc.
-___
-
-### `price_line`
-`label_visible: bool` | `line_visible: bool` | `title: str`
-
-Configures the visibility of the last value price line and its label.
-___
-
-### `fit`
-
-Attempts to fit all data displayed on the chart within the viewport (`fitContent()`).
-___
-
-### `hide_data`
-
-Hides the candles on the chart.
-___
-
-### `show_data`
-
-Shows the hidden candles on the chart.
-___
-
-### `polygon`
-Used to access Polygon.io's API (see [here](https://lightweight-charts-python.readthedocs.io/en/latest/polygon.html)).
-___
-
-### `create_subchart`
-`volume_enabled: bool` | `position: 'left'/'right'/'top'/'bottom'`, `width: float` | `height: float` | `sync: bool/str` | `-> SubChart`
-
-Creates and returns a [SubChart](#subchart) object, placing it adjacent to the declaring `Chart` or `SubChart`.
-
-`position`: specifies how the `SubChart` will float within the `Chart` window.
-
-`height` | `width`: Specifies the size of the `SubChart`, where `1` is the width/height of the window (100%)
-
-`sync`: If given as `True`, the `SubChart`'s timescale and crosshair will follow that of the declaring `Chart` or `SubChart`. If a `str` is passed, the `SubChart` will follow the panel with the given id. Chart ids can be accessed from the`chart.id` and `subchart.id` attributes.
-
-```{important}
-`width` and `height` should be given as a number between 0 and 1.
-```
-
-___
-
-
-## Chart
-`volume_enabled: bool` | `width: int` | `height: int` | `x: int` | `y: int` | `on_top: bool` | `maximize: bool` | `debug: bool` |
-`api: object` | `topbar: bool` | `searchbox: bool` | `toolbox: bool`
-
-The main object used for the normal functionality of lightweight-charts-python, built on the pywebview library.
-
-```{important}
-The `Chart` object should be defined within an `if __name__ == '__main__'` block.
-```
-___
-
-### `show`
-`block: bool`
-
-Shows the chart window, blocking until the chart has loaded. If `block` is enabled, the method will block code execution until the window is closed.
-___
-
-### `hide`
-
-Hides the chart window, which can be later shown by calling `chart.show()`.
-___
-
-### `exit`
-
-Exits and destroys the chart window.
-
-___
-
-### `show_async`
-`block: bool`
-
-Show the chart asynchronously. This should be utilised when using [Callbacks](#callbacks).
-
-### `screenshot`
-`-> bytes`
-
-Takes a screenshot of the chart, and returns a bytes object containing the image. For example:
-
-```python
-if __name__ == '__main__':
- chart = Chart()
- df = pd.read_csv('ohlcv.csv')
- chart.set(df)
- chart.show()
-
- img = chart.screenshot()
- with open('screenshot.png', 'wb') as f:
- f.write(img)
-```
-
-```{important}
-This method should be called after the chart window has loaded.
-```
-___
-
-### `add_hotkey`
-`modifier: 'ctrl'/'shift'/'alt'/'meta'` | `key: str/int/tuple` | `method: object`
-
-Adds a global hotkey to the chart window, which will execute the method or function given.
-
-When using a number in `key`, it should be given as an integer. If multiple key commands are needed for the same function, you can pass a tuple to `key`. For example:
-
-```python
-def place_buy_order(key):
- print(f'Buy {key} shares.')
-
-
-def place_sell_order(key):
- print(f'Sell all shares, because I pressed {key}.')
-
-
-chart.add_hotkey('shift', (1, 2, 3), place_buy_order)
-chart.add_hotkey('shift', 'X', place_sell_order)
-```
-
-
-
-___
-
-## Line
-
-The `Line` object represents a `LineSeries` object in Lightweight Charts and can be used to create indicators. As well as the methods described below, the `Line` object also has access to:
-[`title`](#title), [`marker`](#marker), [`horizontal_line`](#horizontal-line) [`hide_data`](#hide-data), [`show_data`](#show-data) and[`price_line`](#price-line).
-
-```{important}
-The `Line` object should only be accessed from the [`create_line`](#create-line) method of `Chart`.
-```
-___
-
-### `set`
-`data: pd.DataFrame` `name: str`
-
-Sets the data for the line.
-
-When not using the `name` parameter, the columns should be named: `time | value` (Not case sensitive).
-
-Otherwise, the method will use the column named after the string given in `name`. This name will also be used within the legend of the chart. For example:
-```python
-line = chart.create_line()
-
-# DataFrame with columns: date | SMA 50
-df = pd.read_csv('sma50.csv')
-
-line.set(df, name='SMA 50')
-```
-___
-
-### `update`
-`series: pd.Series`
-
-Updates the data for the line.
-
-This should be given as a Series object, with labels akin to the `line.set()` function.
-___
-
-### `delete`
-
-Irreversibly deletes the line.
-___
-
-## HorizontalLine
-
-The `HorizontalLine` object represents a `PriceLine` in Lightweight Charts.
-
-```{important}
-The `HorizontalLine` object should only be accessed from the [`horizontal_line`](#horizontal-line) Common Method.
-```
-___
-
-### `update`
-`price: float/int`
-
-Updates the price of the horizontal line.
-
-___
-
-### `delete`
-
-Irreversibly deletes the horizontal line.
-___
-
-## SubChart
-
-The `SubChart` object allows for the use of multiple chart panels within the same `Chart` window. All of the [Common Methods](#common-methods) can be used within a `SubChart`. Its instance should be accessed using the [create_subchart](#create-subchart) method.
-
-`SubCharts` are arranged horizontally from left to right. When the available space is no longer sufficient, the subsequent `SubChart` will be positioned on a new row, starting from the left side.
-___
-
-### Grid of 4 Example:
-
-```python
-import pandas as pd
-from lightweight_charts import Chart
-
-if __name__ == '__main__':
- chart = Chart(inner_width=0.5, inner_height=0.5)
-
- chart2 = chart.create_subchart(position='right', width=0.5, height=0.5)
-
- chart3 = chart2.create_subchart(position='left', width=0.5, height=0.5)
-
- chart4 = chart3.create_subchart(position='right', width=0.5, height=0.5)
-
- chart.watermark('1')
- chart2.watermark('2')
- chart3.watermark('3')
- chart4.watermark('4')
-
- df = pd.read_csv('ohlcv.csv')
- chart.set(df)
- chart2.set(df)
- chart3.set(df)
- chart4.set(df)
-
- chart.show(block=True)
-
-```
-___
-
-### Synced Line Chart Example:
-
-```python
-import pandas as pd
-from lightweight_charts import Chart
-
-if __name__ == '__main__':
- chart = Chart(inner_width=1, inner_height=0.8)
- chart.time_scale(visible=False)
-
- chart2 = chart.create_subchart(width=1, height=0.2, sync=True, volume_enabled=False)
-
- df = pd.read_csv('ohlcv.csv')
- df2 = pd.read_csv('rsi.csv')
-
- chart.set(df)
- line = chart2.create_line()
- line.set(df2)
-
- chart.show(block=True)
-
-```
-___
-
-## Callbacks
-
-The `Chart` object allows for asynchronous and synchronous callbacks to be passed back to python when using the `show_async` method, allowing for more sophisticated chart layouts including searching, timeframe selectors, and text boxes.
-
-[`QtChart`](#qtchart) and [`WxChart`](#wxchart) can also use callbacks, however they use their respective event loops to emit callbacks rather than asyncio.
-
-A variety of the parameters below should be passed to the Chart upon decaration.
-* `api`: The class object that the fixed callbacks will always be emitted to (see [How to use Callbacks](#how-to-use-callbacks)).
-* `topbar`: Adds a [TopBar](#topbar) to the `Chart` or `SubChart` and allows use of the `create_switcher` method.
-* `searchbox`: Adds a search box onto the `Chart` or `SubChart` that is activated by typing.
-
-___
-### How to use Callbacks
-
-Fixed Callbacks are emitted to the class given as the `api` parameter shown above.
-
-Take a look at this minimal example:
-
-```python
-class API:
- def __init__(self):
- self.chart = None
-
- async def on_search(self, string):
- print(f'Search Text: "{string}" | Chart/SubChart ID: "{self.chart.id}"')
-```
-Upon searching in a pane, the expected output would be akin to:
-```
-Search Text: "AAPL" | Chart/SubChart ID: "window.blyjagcr"
-```
-The ID shown above will change depending upon which pane was used to search, due to the instance of `self.chart` dynamically updating to the latest pane which triggered the callback.
-`self.chart` will update upon each callback, allowing for access to the specific [Common Methods](#common-methods) for the pane in question.
-
-```{important}
-* Search callbacks will always be emitted to a method named `on_search`
-* `API` class methods can be either coroutines or normal methods.
-* Non fixed callbacks (switchers, hotkeys) can be methods, coroutines, or regular functions.
-```
-___
-
-### `TopBar`
-The `TopBar` class represents the top bar shown on the chart when using callbacks:
-
-
-
-This class is accessed from the `topbar` attribute of the chart object (`chart.topbar.`), after setting the topbar parameter to `True` upon declaration of the chart.
-
-Switchers and text boxes can be created within the top bar, and their instances can be accessed through the `topbar` dictionary. For example:
-
-```python
-chart = Chart(api=api, topbar=True)
-
-chart.topbar.textbox('symbol', 'AAPL') # Declares a textbox displaying 'AAPL'.
-print(chart.topbar['symbol'].value) # Prints the value within ('AAPL')
-
-chart.topbar['symbol'].set('MSFT') # Sets the 'symbol' textbox to 'MSFT'
-print(chart.topbar['symbol'].value) # Prints the value again ('MSFT')
-```
-___
-
-### `switcher`
-`name: str` | `method: function` | `*options: str` | `default: str`
-
-* `name`: the name of the switcher which can be used to access it from the `topbar` dictionary.
-* `method`: The function from the `api` class given to the constructor that will receive the callback.
-* `options`: The strings to be displayed within the switcher. This may be a variety of timeframes, security types, or whatever needs to be updated directly from the chart.
-* `default`: The initial switcher option set.
-___
-
-### `textbox`
-`name: str` | `initial_text: str`
-
-* `name`: the name of the text box which can be used to access it from the `topbar` dictionary.
-* `initial_text`: The text to show within the text box.
-___
-
-### Callbacks Example:
-
-```python
-import asyncio
-import pandas as pd
-from my_favorite_broker import get_bar_data
-
-from lightweight_charts import Chart
-
-
-class API:
- def __init__(self):
- self.chart = None
-
- async def on_search(self, searched_string): # Called when the user searches.
- timeframe = self.chart.topbar['timeframe'].value
- new_data = await get_bar_data(searched_string, timeframe)
- if not new_data:
- return
- self.chart.set(new_data) # sets data for the Chart or SubChart in question.
- self.chart.topbar['symbol'].set(searched_string)
-
- async def on_timeframe(self): # Called when the user changes the timeframe.
- timeframe = self.chart.topbar['timeframe'].value
- symbol = self.chart.topbar['symbol'].value
- new_data = await get_bar_data(symbol, timeframe)
- if not new_data:
- return
- self.chart.set(new_data)
-
-
-async def main():
- api = API()
-
- chart = Chart(api=api, topbar=True, searchbox=True)
-
- chart.topbar.textbox('symbol', 'TSLA')
- chart.topbar.switcher('timeframe', api.on_timeframe, '1min', '5min', '30min', 'H', 'D', 'W', default='5min')
-
- df = pd.read_csv('ohlcv.csv')
- chart.set(df)
-
- await chart.show_async(block=True)
-
-
-if __name__ == '__main__':
- asyncio.run(main())
-```
-___
-
-## Toolbox
-The Toolbox allows for trendlines, ray lines and horizontal lines to be drawn and edited directly on the chart.
-
-It can be used within any Chart object, and is enabled by setting the `toolbox` parameter to `True` upon Chart declaration.
-
-The following hotkeys can also be used when the Toolbox is enabled:
-* Alt+T: Trendline
-* Alt+H: Horizontal Line
-* Alt+R: Ray Line
-* Meta+Z or Ctrl+Z: Undo
-
-Drawings can also be deleted by right-clicking on them, which brings up a context menu.
-___
-
-### `save_drawings_under`
-`widget: Widget`
-
-Saves drawings under a specific `topbar` text widget. For example:
-
-```python
-chart.toolbox.save_drawings_under(chart.topbar['symbol'])
-```
-___
-
-### `load_drawings`
-`tag: str`
-
-Loads and displays drawings stored under the tag given.
-___
-### `import_drawings`
-`file_path: str`
-
-Imports the drawings stored at the JSON file given in `file_path`.
-
-___
-### `export_drawings`
-`file_path: str`
-
-Exports all currently saved drawings to the JSON file given in `file_path`.
-___
-
-## QtChart
-`widget: QWidget` | `volume_enabled: bool`
-
-The `QtChart` object allows the use of charts within a `QMainWindow` object, and has similar functionality to the `Chart` and `ChartAsync` objects for manipulating data, configuring and styling.
-
-Callbacks can be recieved through the Qt event loop, using an [API](#how-to-use-callbacks) class that uses **syncronous** methods instead of **asyncronous** methods.
-___
-
-### `get_webview`
-
-`-> QWebEngineView`
-
-Returns the `QWebEngineView` object.
-
-___
-### Example:
-
-```python
-import pandas as pd
-from PyQt5.QtWidgets import QApplication, QMainWindow, QVBoxLayout, QWidget
-
-from lightweight_charts.widgets import QtChart
-
-app = QApplication([])
-window = QMainWindow()
-layout = QVBoxLayout()
-widget = QWidget()
-widget.setLayout(layout)
-
-window.resize(800, 500)
-layout.setContentsMargins(0, 0, 0, 0)
-
-chart = QtChart(widget)
-
-df = pd.read_csv('ohlcv.csv')
-chart.set(df)
-
-layout.addWidget(chart.get_webview())
-
-window.setCentralWidget(widget)
-window.show()
-
-app.exec_()
-```
-___
-
-## WxChart
-`parent: wx.Panel` | `volume_enabled: bool`
-
-The WxChart object allows the use of charts within a `wx.Frame` object, and has similar functionality to the `Chart` and `ChartAsync` objects for manipulating data, configuring and styling.
-
-Callbacks can be recieved through the Wx event loop, using an [API](#how-to-use-callbacks) class that uses **syncronous** methods instead of **asyncronous** methods.
-___
-
-### `get_webview`
-`-> wx.html2.WebView`
-
-Returns a `wx.html2.WebView` object which can be used to for positioning and styling within wxPython.
-___
-
-### Example:
-
-```python
-import wx
-import pandas as pd
-
-from lightweight_charts.widgets import WxChart
-
-
-class MyFrame(wx.Frame):
- def __init__(self):
- super().__init__(None)
- self.SetSize(1000, 500)
-
- panel = wx.Panel(self)
- sizer = wx.BoxSizer(wx.VERTICAL)
- panel.SetSizer(sizer)
-
- chart = WxChart(panel)
-
- df = pd.read_csv('ohlcv.csv')
- chart.set(df)
-
- sizer.Add(chart.get_webview(), 1, wx.EXPAND | wx.ALL)
- sizer.Layout()
- self.Show()
-
-
-if __name__ == '__main__':
- app = wx.App()
- frame = MyFrame()
- app.MainLoop()
-
-```
-___
-
-## StreamlitChart
-`parent: wx.Panel` | `volume_enabled: bool`
-
-The `StreamlitChart` object allows the use of charts within a Streamlit app, and has similar functionality to the `Chart` object for manipulating data, configuring and styling.
-
-This object only supports the displaying of **static** data, and should not be used with the `update_from_tick` or `update` methods. Every call to the chart object must occur **before** calling `load`.
-___
-
-### `load`
-
-Loads the chart into the Streamlit app. This should be called after setting, styling, and configuring the chart, as no further calls to the `StreamlitChart` will be acknowledged.
-___
-
-### Example:
-```python
-import pandas as pd
-from lightweight_charts.widgets import StreamlitChart
-
-chart = StreamlitChart(width=900, height=600)
-
-df = pd.read_csv('ohlcv.csv')
-chart.set(df)
-
-chart.load()
-```
-___
-
-## JupyterChart
-
-The `JupyterChart` object allows the use of charts within a notebook, and has similar functionality to the `Chart` object for manipulating data, configuring and styling.
-
-This object only supports the displaying of **static** data, and should not be used with the `update_from_tick` or `update` methods. Every call to the chart object must occur **before** calling `load`.
-___
-
-### `load`
-
-Renders the chart. This should be called after setting, styling, and configuring the chart, as no further calls to the `JupyterChart` will be acknowledged.
-___
-
-### Example:
-```python
-import pandas as pd
-from lightweight_charts import JupyterChart
-
-chart = JupyterChart()
-
-df = pd.read_csv('ohlcv.csv')
-chart.set(df)
-
-chart.load()
-```
diff --git a/docs/source/index.md b/docs/source/index.md
index 05b6e69..d2b32fb 100644
--- a/docs/source/index.md
+++ b/docs/source/index.md
@@ -1,7 +1,11 @@
```{toctree}
:hidden:
-docs
+common_methods
+charts
+callbacks
+toolbox
+tables
polygon
Github Repository
```
diff --git a/docs/source/polygon.md b/docs/source/polygon.md
index ba3bbdd..9492aa6 100644
--- a/docs/source/polygon.md
+++ b/docs/source/polygon.md
@@ -8,7 +8,7 @@ To use data from Polygon, there are certain libraries (not listed as requirement
* Live data requires the `websockets` library.
___
## `polygon`
-`polygon` is a [Common Method](https://lightweight-charts-python.readthedocs.io/en/latest/docs.html#common-methods), and can be accessed from within any chart type.
+`polygon` is a [Common Method](https://lightweight-charts-python.readthedocs.io/en/latest/common_methods.html), and can be accessed from within any chart type.
`chart.polygon.`
@@ -107,7 +107,7 @@ This object requires the `requests` library for static data, and the `websockets
All data is requested within the chart window through searching and selectors.
-As well as the parameters from the [Chart](https://lightweight-charts-python.readthedocs.io/en/latest/docs.html#chart) object, PolygonChart also has the parameters:
+As well as the parameters from the [Chart](https://lightweight-charts-python.readthedocs.io/en/latest/charts.html#chart) object, PolygonChart also has the parameters:
* `api_key`: The user's Polygon.io API key.
* `num_bars`: The target number of bars to be displayed on the chart
diff --git a/docs/source/tables.md b/docs/source/tables.md
new file mode 100644
index 0000000..95015cd
--- /dev/null
+++ b/docs/source/tables.md
@@ -0,0 +1,115 @@
+# Table
+`width: int/float` | `height: int/float` | `headings: tuple[str]` | `widths: tuple[float]` | `alignments: tuple[str]` | `position: 'left'/'right'/'top'/'bottom'` | `draggable: bool` | `method: object`
+
+Tables are panes that can be used to gain further functionality from charts. They are intended to be used for watchlists, order management, or position management. It should be accessed from the `create_table` common method.
+
+The `Table` and `Row` objects inherit from dictionaries, and can be manipulated as such.
+
+
+
+`width`/`height`: Either given as a percentage (a `float` between 0 and 1) or as an integer representing pixel size.
+
+`widths`: Given as a `float` between 0 and 1.
+
+`position`: Used as you would when creating a `SubChart`, representing how the table will float within the window.
+
+`draggable`: If `True`, then the window can be dragged to any position within the window.
+
+`method`: If given this will be called when a row is clicked.
+___
+
+## `new_row` (Row)
+`*values` | `id: int` | `-> Row`
+
+Creates a new row within the table, and returns a `Row` object.
+
+if `id` is passed it should be unique to all other rows. Otherwise, the `id` will be randomly generated.
+
+
+### `background_color`
+`column: str` | `color: str`
+
+Sets the background color of the Row cell at the given column.
+
+### `delete`
+Deletes the row.
+___
+
+## `clear`
+Clears and deletes all table rows.
+___
+
+## `format`
+`column: str` | `format_str: str`
+
+Sets the format to be used for the given column. `table.VALUE` should be used as a placeholder for the cell value. For example:
+
+```python
+table.format('Daily %', f'{table.VALUE} %')
+table.format('PL', f'$ {table.VALUE}')
+```
+___
+
+## `visible`
+`visible: bool`
+
+Sets the visibility of the Table.
+___
+
+## Footer
+Tables can also have a footer containing a number of text boxes. To initialize this, call the `footer` attribute with the number of textboxes to be used:
+
+```python
+table.footer(3) # Footer will be displayed, with 3 text boxes.
+```
+To edit the textboxes, treat `footer` as a list:
+
+```python
+table.footer[0] = 'Text Box 1'
+table.footer[1] = 'Text Box 2'
+table.footer[2] = 'Text Box 3'
+```
+___
+
+## Example:
+
+```python
+import pandas as pd
+from lightweight_charts import Chart
+
+def on_row_click(row_id):
+ row = table.get(row_id)
+ print(row)
+
+ row['PL'] = round(row['PL']+1, 2)
+ row.background_color('PL', 'green' if row['PL'] > 0 else 'red')
+
+ table.footer[1] = row['Ticker']
+
+if __name__ == '__main__':
+ chart = Chart(width=1000, inner_width=0.7, inner_height=1)
+ subchart = chart.create_subchart(width=0.3, height=0.5)
+ df = pd.read_csv('ohlcv.csv')
+ chart.set(df)
+ subchart.set(df)
+
+ table = chart.create_table(width=0.3, height=0.2,
+ headings=('Ticker', 'Quantity', 'Status', '%', 'PL'),
+ widths=(0.2, 0.1, 0.2, 0.2, 0.3),
+ alignments=('center', 'center', 'right', 'right', 'right'),
+ position='left', method=on_row_click)
+
+ table.format('PL', f'£ {table.VALUE}')
+ table.format('%', f'{table.VALUE} %')
+
+ table.new_row('SPY', 3, 'Submitted', 0, 0)
+ table.new_row('AMD', 1, 'Filled', 25.5, 105.24)
+ table.new_row('NVDA', 2, 'Filled', -0.5, -8.24)
+
+ table.footer(2)
+ table.footer[0] = 'Selected:'
+
+ chart.show(block=True)
+
+```
+
diff --git a/docs/source/toolbox.md b/docs/source/toolbox.md
new file mode 100644
index 0000000..62adc41
--- /dev/null
+++ b/docs/source/toolbox.md
@@ -0,0 +1,102 @@
+# Toolbox
+The Toolbox allows for trendlines, ray lines and horizontal lines to be drawn and edited directly on the chart.
+
+It can be used within any Chart object, and is enabled by setting the `toolbox` parameter to `True` upon Chart declaration.
+
+The Toolbox should only be accessed from the `toolbox` attribute of the chart object. (`chart.toolbox.`)
+
+The following hotkeys can also be used when the Toolbox is enabled:
+* Alt+T: Trendline
+* Alt+H: Horizontal Line
+* Alt+R: Ray Line
+* Meta+Z or Ctrl+Z: Undo
+
+Drawings can also be deleted by right-clicking on them, which brings up a context menu.
+___
+
+## `save_drawings_under`
+`widget: Widget`
+
+Saves drawings under a specific `topbar` text widget. For example:
+
+```python
+chart.toolbox.save_drawings_under(chart.topbar['symbol'])
+```
+___
+
+## `load_drawings`
+`tag: str`
+
+Loads and displays drawings stored under the tag given.
+___
+
+## `import_drawings`
+`file_path: str`
+
+Imports the drawings stored at the JSON file given in `file_path`.
+___
+
+## `export_drawings`
+`file_path: str`
+
+Exports all currently saved drawings to the JSON file given in `file_path`.
+___
+
+## Example:
+
+To get started, create a file called `drawings.json`, which should only contain `{}`.
+
+```python
+import pandas as pd
+from lightweight_charts import Chart
+
+
+def get_bar_data(symbol, timeframe):
+ if symbol not in ('AAPL', 'GOOGL', 'TSLA'):
+ print(f'No data for "{symbol}"')
+ return pd.DataFrame()
+ return pd.read_csv(f'bar_data/{symbol}_{timeframe}.csv')
+
+
+class API:
+ def __init__(self):
+ self.chart: Chart = None
+
+ def on_search(self, searched_string):
+ new_data = get_bar_data(searched_string, self.chart.topbar['timeframe'].value)
+ if new_data.empty:
+ return
+ self.chart.topbar['symbol'].set(searched_string)
+ self.chart.set(new_data)
+ self.chart.toolbox.load_drawings(searched_string) # Loads the drawings saved under the symbol.
+
+ def on_timeframe_selection(self):
+ new_data = get_bar_data(self.chart.topbar['symbol'].value, self.chart.topbar['timeframe'].value)
+ if new_data.empty:
+ return
+ self.chart.set(new_data, render_drawings=True) # The symbol has not changed, so we want to re-render the drawings.
+
+
+if __name__ == '__main__':
+ api = API()
+
+ chart = Chart(api=api, topbar=True, searchbox=True, toolbox=True)
+ chart.legend(True)
+
+ chart.topbar.textbox('symbol', 'TSLA')
+ chart.topbar.switcher('timeframe', api.on_timeframe_selection, '1min', '5min', '30min', default='5min')
+
+ df = get_bar_data('TSLA', '5min')
+
+ chart.set(df)
+
+ chart.toolbox.import_drawings('drawings.json') # Imports the drawings saved in the JSON file.
+ chart.toolbox.load_drawings(chart.topbar['symbol'].value) # Loads the drawings under the default symbol.
+
+ chart.toolbox.save_drawings_under(chart.topbar['symbol']) # Saves drawings based on the symbol.
+
+ chart.show(block=True)
+
+ chart.toolbox.export_drawings('drawings.json') # Exports the drawings to the JSON file.
+
+```
diff --git a/examples/6_callbacks/callbacks.py b/examples/6_callbacks/callbacks.py
index 5c9b867..e7c4120 100644
--- a/examples/6_callbacks/callbacks.py
+++ b/examples/6_callbacks/callbacks.py
@@ -1,6 +1,4 @@
-import asyncio
import pandas as pd
-
from lightweight_charts import Chart
@@ -15,24 +13,24 @@ class API:
def __init__(self):
self.chart: Chart = None # Changes after each callback.
- async def on_search(self, searched_string): # Called when the user searches.
+ def on_search(self, searched_string): # Called when the user searches.
new_data = get_bar_data(searched_string, self.chart.topbar['timeframe'].value)
if new_data.empty:
return
self.chart.topbar['symbol'].set(searched_string)
self.chart.set(new_data)
- async def on_timeframe_selection(self): # Called when the user changes the timeframe.
+ def on_timeframe_selection(self): # Called when the user changes the timeframe.
new_data = get_bar_data(self.chart.topbar['symbol'].value, self.chart.topbar['timeframe'].value)
if new_data.empty:
return
- self.chart.set(new_data, render_drawings=True)
+ self.chart.set(new_data, True)
- async def on_horizontal_line_move(self, line_id, price):
+ def on_horizontal_line_move(self, line_id, price):
print(f'Horizontal line moved to: {price}')
-async def main():
+if __name__ == '__main__':
api = API()
chart = Chart(api=api, topbar=True, searchbox=True, toolbox=True)
@@ -46,8 +44,4 @@ async def main():
chart.horizontal_line(200, interactive=True)
- await chart.show_async(block=True)
-
-
-if __name__ == '__main__':
- asyncio.run(main())
+ chart.show(block=True)
\ No newline at end of file
diff --git a/lightweight_charts/abstract.py b/lightweight_charts/abstract.py
index 49d445d..81144eb 100644
--- a/lightweight_charts/abstract.py
+++ b/lightweight_charts/abstract.py
@@ -5,6 +5,7 @@
import pandas as pd
from typing import Union, Literal, Dict, List
+from lightweight_charts.table import Table
from lightweight_charts.util import LINE_STYLE, MARKER_POSITION, MARKER_SHAPE, CROSSHAIR_MODE, _crosshair_mode, \
_line_style, \
MissingColumn, _js_bool, _price_scale_mode, PRICE_SCALE_MODE, _marker_position, _marker_shape, IDGen
@@ -12,7 +13,7 @@
JS = {}
current_dir = os.path.dirname(os.path.abspath(__file__))
-for file in ('pkg', 'funcs', 'callback', 'toolbox'):
+for file in ('pkg', 'funcs', 'callback', 'toolbox', 'table'):
with open(os.path.join(current_dir, 'js', f'{file}.js'), 'r', encoding='utf-8') as f:
JS[file] = f.read()
@@ -41,6 +42,7 @@