Help with the code for generic circuits #860
Replies: 2 comments 1 reply
-
I have put together a little documentation. Types// boolean
x := true;
x = false;
// int
x := 1; // decimal
x = 0xA; // hex
x = 01; // octal
// float
x := 1.0;
x = 1.0e2; // scientific (negative exponents NOT WORKING)
//x = 0x1.FpA; // hexfloat (NOT WORKING)
// string
x := "s";
// list
x := [1, 1.0, "1", [1]]; Conversion// explicit conversions
x := int(1.0); // float to int
//x := int("1"); // string to int (NOT WORKING)
x := float(1); // int to float
//x := float("1"); // string to float (NOT WORKING)
// implicit conversion
x := "" + 1; // int to string
//x := "" + 1.0 // float to string (NOT WORKING)
x := "" + [1] // list to string
x := 1; // x is int
y := 1.0 + x; // y is float Operators// assignment: := =
x := 1; // new defined, x is int
x = 2; // assignment
x = "1"; // ERROR x is int
x := "1"; // x is string
// math: ( ) + - * / % << >> ++ --
x := (1 + 2) * 3;
//x += 2; // inplace op (NOT WORKING)
x = x / 2; // x is int
x = x % 2; // modulo
x = x << 2; // arithmetic shift
x++; // post increment
//--x; // pre inc & dec (NOT WORKING)
// boolean logic: ! & | ^
x := true;
y := false;
z := !x & y ^ true;
// comparison: = != > < >= <=
x := 1 = 1; // equality Control flowif (x = 0) {
...
} else {
...
}
while (x != 0) {
...
}
for (i := 0; i < 10; i++) {
...
} Functions// creates a wire from (x0, y0) to (x0, y1)
void addWire(int x0, int y0, int x1, int y1);
// creates a component at (x, y)
Struct addComponent(String c, int x, int y);
// int(log2(x)) + 1
int bitsNeededFor(int x);
// rounded up value: floor(1.2) = 1
int ceil(float x);
// rounded down value: ceil(1.2) = 2
int floor(float x);
// rounded value: round(1.5) = 2
int round(float x);
// random number between 1 and x-1
int random(int x);
// minimum number of arguments
int|float min(int|float args...);
// maximum number of arguments
int|float max(int|float args...);
// absolute value
int|float abs(int|float x);
// prints arguments to console
void print(T args...);
// prints fomatted string with arguments to console
void printf(String s, T args...);
// prints newline seperated arguments to console
void println(T args...);
// logging function, returns same argument
T log(T x);
// formats string: format("%.2f", 1.5) = "1.50"
String format(String s, T args...);
// checks if argument is not null
boolean isPresent(T x);
// throws error and prints message
void panic(String s);
// returns context name?
String output(Context o);
// splits string " \r\t\n,:;"
List[String] splitString(String s);
// puts "n" at beginning if string is a number
String identifier(String s);
// loads hex file in little-endian
List[int] loadHex(String file, int dataBits);
// loads text file
String loadFile(String file);
// number of items in list
int sizeOf(List x); Attributes// build-in components
or := addComponent("Or", 0, 0); // creates or gate at (0, 0)
// atributes in Help
or.Bits = 2;
or.Inputs = 3;
or.inverterConfig = ["In_1", "In_3"];
or.rotation = 1; // 0: east, 1: north, 2: west, 3: south
or.wideShape = false;
split := addComponent("Splitter", 0, 0);
split.'Input Splitting' = "4"; // '' if attribite has whitespaces
split.'Output Splitting' = "0-2";
// custume components
circ := addComponent("circ.dig", 0, 0);
circ.Bits := 3; // attribute name in init, (:=) used
circ.this.rotation = 1; // (this) for attributes in Help Keywords// in init
x := 1; // generic variable of circuit
// in code
global.y := args.x * 2; // using generic variable
// in component 1
this.Bits = global.y; // args.x * 2 could be used
// in component 2
this.Bits = global.y; // variable y only calculated once |
Beta Was this translation helpful? Give feedback.
-
For the generation of the HDL code (VHDL and Verilog) a simple language was needed to generate executable HDL code from templates. |
Beta Was this translation helpful? Give feedback.
-
Can someone tell me “programming language” the generic code component uses?
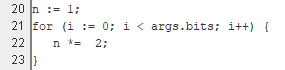
Overall, I understood how the parameterization of the generic circuit works. I searched the documentation and also the help menus of the different component for explanations.
But when it comes to more complex operation, I’m kind of ‘lost’ with the syntax of the code.
For example, why can I not use this statement:
I know that I can use
n = n*2;
in this example but I’m generally curious what kind of statements and (data-)structures are allowed in this code.Beta Was this translation helpful? Give feedback.
All reactions