-
-
Notifications
You must be signed in to change notification settings - Fork 5.6k
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Enhancing Gitea OAuth2 Provider with Granular Scopes for Resource Access #31609
Comments
Hi @marcellmars, thanks for this issue. Sorry you didn't get a response sooner. This is most definitely planned, and so I'll reopen this issue so it can be tracked. |
Thanks for coming back. If anyone gets into it, maybe they can cherry-pick it back from Forgejo, where I expanded this initial idea, with great support from the community, into a PR. |
Any updates? I cherry-picked PR from Forgejo and it worked so it will be nice to have this in regular Gitea. |
The previously linked PR should be ready for the new v9 Forgejo release, scheduled for 16 October 2024. During testing, I realized that—following my initial goal of creating a minimal OIDC Single-Sign-On—using only the However, when preparing a good example for the documentation, I discovered that the This led to another PR that attempts to address that issue. I hope both PRs make it into the next release. Once it lands in Forgejo, I’ll see how to move it forward for Gitea. |
I don't know whether we can easily cherry-pick code from that project because of the LICENSE compatibility between GPLv3 and MIT(Gitea). And I sent #32148 maybe break the patch but I think it's necessary to make the code clear. |
I'm not certain about the LICENSE compatibility, but I've been informed that Forgejo contributors are definitely allowed to contribute to Gitea as well. I applied both pull requests from Forgejo against the #32148 and I managed to get it all in, together with tests. I did it in two big commits. I hope that will be comprehensible enough. The pull request is here. I don't know when @lunny is that ok? |
Thank you very much. I will review those pull requests ASAP. And if you can send docs pull request to https://gitea.com/gitea/docs, that would be better. |
i deleted the old PR and made a new cleaned up one here |
…ess (#32573) Resolve #31609 This PR was initiated following my personal research to find the lightest possible Single Sign-On solution for self-hosted setups. The existing solutions often seemed too enterprise-oriented, involving many moving parts and services, demanding significant resources while promising planetary-scale capabilities. Others were adequate in supporting basic OAuth2 flows but lacked proper user management features, such as a change password UI. Gitea hits the sweet spot for me, provided it supports more granular access permissions for resources under users who accept the OAuth2 application. This PR aims to introduce granularity in handling user resources as nonintrusively and simply as possible. It allows third parties to inform users about their intent to not ask for the full access and instead request a specific, reduced scope. If the provided scopes are **only** the typical ones for OIDC/OAuth2—`openid`, `profile`, `email`, and `groups`—everything remains unchanged (currently full access to user's resources). Additionally, this PR supports processing scopes already introduced with [personal tokens](https://docs.gitea.com/development/oauth2-provider#scopes) (e.g. `read:user`, `write:issue`, `read:group`, `write:repository`...) Personal tokens define scopes around specific resources: user info, repositories, issues, packages, organizations, notifications, miscellaneous, admin, and activitypub, with access delineated by read and/or write permissions. The initial case I wanted to address was to have Gitea act as an OAuth2 Identity Provider. To achieve that, with this PR, I would only add `openid public-only` to provide access token to the third party to authenticate the Gitea's user but no further access to the API and users resources. Another example: if a third party wanted to interact solely with Issues, it would need to add `read:user` (for authorization) and `read:issue`/`write:issue` to manage Issues. My approach is based on my understanding of how scopes can be utilized, supported by examples like [Sample Use Cases: Scopes and Claims](https://auth0.com/docs/get-started/apis/scopes/sample-use-cases-scopes-and-claims) on auth0.com. I renamed `CheckOAuthAccessToken` to `GetOAuthAccessTokenScopeAndUserID` so now it returns AccessTokenScope and user's ID. In the case of additional scopes in `userIDFromToken` the default `all` would be reduced to whatever was asked via those scopes. The main difference is the opportunity to reduce the permissions from `all`, as is currently the case, to what is provided by the additional scopes described above. Screenshots:  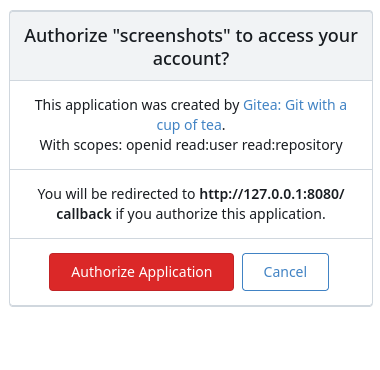 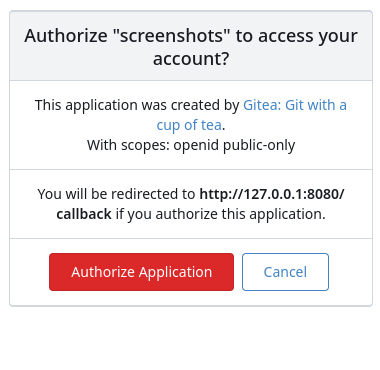  --------- Co-authored-by: wxiaoguang <[email protected]>
Feature Description
Title
"Enhancing OAuth2 Scopes in Gitea for More Granular Access Control"
Hi everyone,
After some research among OAuth2 provider solutions, I ended up with a desire for Gitea to handle that job! ;)
Gitea is useful for more than just coder communities. It doesn't require too many resources and does a great job managing users and allowing them to maintain their accounts.
Other solutions I found either ask for enterprise requirements or don't do a good enough job managing users.
For my use case, Gitea does exactly what I expect from an OAuth2 provider, except for the granular settings of what resources can be accessed through OAuth2 clients using Gitea as the OAuth2 provider.
From my understanding, Gitea serves the usual suspects such as
openid
,profile
,email
, andgroups
, but it also implicitly addsread/write all
so every token gets access to everything under the user who accepts the OAuth2 client/service. That has obviously served all the users well so far.I think it would be great if OAuth2 clients could ask for what they need by requesting additional scopes such as
read:user
,read:repository
,read:issue
,write:issue
,public-only
, etc.In my case, I would be happy to ask Gitea to only allow
read:user
. This would make Gitea the best OAuth2 provider for me.This itch pushed me into my first attempt to hack on Gitea.
I found that I could add a check for additional scopes in CheckOAuthAccessToken and pass it further so it could be used in userIDFromToken. Instead of
store.GetData()["ApiTokenScope"] = auth_model.AccessTokenScopeAll
(which allows access to all resources under the user), the scopes requested by the OAuth2 client are used.The new function
grantAdditionalScopes
adds only the scopes found asAccessTokenScope
(all
,public-only
,read:activitypub
,write:activitypub
,read:admin
,write:admin
,read:misc
,write:misc
etc..This means one could ask for read-only access to
user
,issue
, andactivitypub
withread:user
,read:issue
, andread:activitypub
. This would come after the usual OAuth2 suspects:openid
,profile
,email
, andgroups
.My approach here is based on reading and understanding how scopes might be used, and one of the examples I found that confirm my understanding is Sample Use Cases: Scopes and Claims at auth0.com.
In my internal tests, this worked fine. I'm not sure if this is the best direction.
While working on this, I felt it would be nice to list requested scopes in the consent snippet for client authorization.
It shouldn't be a big deal to even add the possibility for the user to change/reduce the requested scopes.
Here are a few snippets that made it work for me. I'm interested to hear your feedback on this.
The text was updated successfully, but these errors were encountered: