Commit 78129b8
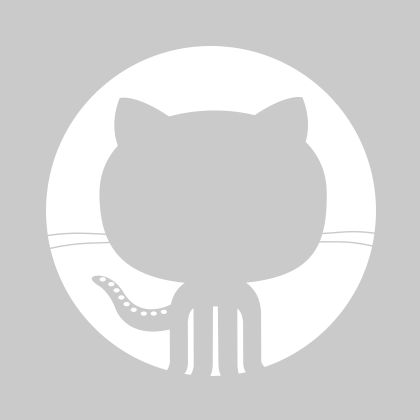
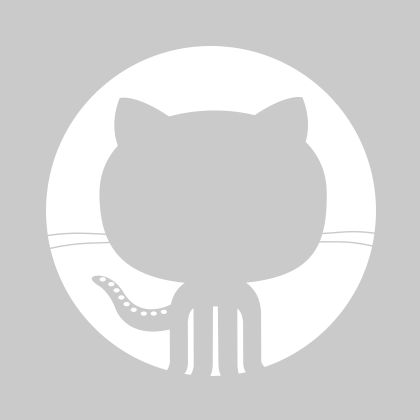
Sujay Jayakar
Convex, Inc.
1 parent 2839c16 commit 78129b8
File tree
27 files changed
+108
-98
lines changed- crates
- application/src
- common/src
- runtime
- testing
- sync
- convex
- examples
- src
- client
- sync
- database/src
- index_workers
- function_runner/src
- isolate/src
- isolate2
- local_backend/src/subs
- runtime/src
- value
- src
27 files changed
+108
-98
lines changedLines changed: 1 addition & 1 deletion
Some generated files are not rendered by default. Learn more about customizing how changed files appear on GitHub.
Lines changed: 8 additions & 0 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
193 | 193 |
| |
194 | 194 |
| |
195 | 195 |
| |
| 196 | + | |
| 197 | + | |
| 198 | + | |
| 199 | + | |
| 200 | + | |
| 201 | + | |
| 202 | + | |
| 203 | + |
Lines changed: 1 addition & 1 deletion
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
52 | 52 |
| |
53 | 53 |
| |
54 | 54 |
| |
55 |
| - | |
56 | 55 |
| |
57 | 56 |
| |
58 | 57 |
| |
| |||
74 | 73 |
| |
75 | 74 |
| |
76 | 75 |
| |
| 76 | + | |
77 | 77 |
| |
78 | 78 |
| |
79 | 79 |
| |
|
Lines changed: 2 additions & 4 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
135 | 135 |
| |
136 | 136 |
| |
137 | 137 |
| |
138 |
| - | |
139 |
| - | |
140 |
| - | |
141 |
| - | |
| 138 | + | |
142 | 139 |
| |
143 | 140 |
| |
144 | 141 |
| |
| |||
283 | 280 |
| |
284 | 281 |
| |
285 | 282 |
| |
| 283 | + | |
286 | 284 |
| |
287 | 285 |
| |
288 | 286 |
| |
|
Lines changed: 1 addition & 1 deletion
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
22 | 22 |
| |
23 | 23 |
| |
24 | 24 |
| |
25 |
| - | |
26 | 25 |
| |
27 | 26 |
| |
28 | 27 |
| |
29 | 28 |
| |
30 | 29 |
| |
| 30 | + | |
31 | 31 |
| |
32 | 32 |
| |
33 | 33 |
| |
|
Lines changed: 2 additions & 4 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
1 | 1 |
| |
2 | 2 |
| |
3 | 3 |
| |
4 |
| - | |
5 |
| - | |
6 |
| - | |
7 |
| - | |
| 4 | + | |
8 | 5 |
| |
9 | 6 |
| |
10 | 7 |
| |
| |||
17 | 14 |
| |
18 | 15 |
| |
19 | 16 |
| |
| 17 | + | |
20 | 18 |
| |
21 | 19 |
| |
22 | 20 |
| |
|
Lines changed: 2 additions & 1 deletion
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
20 | 20 |
| |
21 | 21 |
| |
22 | 22 |
| |
23 |
| - | |
24 | 23 |
| |
25 | 24 |
| |
26 | 25 |
| |
| |||
52 | 51 |
| |
53 | 52 |
| |
54 | 53 |
| |
| 54 | + | |
55 | 55 |
| |
56 | 56 |
| |
57 | 57 |
| |
| |||
203 | 203 |
| |
204 | 204 |
| |
205 | 205 |
| |
| 206 | + | |
206 | 207 |
| |
207 | 208 |
| |
208 | 209 |
| |
|
Lines changed: 7 additions & 5 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
18 | 18 |
| |
19 | 19 |
| |
20 | 20 |
| |
21 |
| - | |
22 | 21 |
| |
23 | 22 |
| |
24 | 23 |
| |
| |||
34 | 33 |
| |
35 | 34 |
| |
36 | 35 |
| |
37 |
| - | |
38 |
| - | |
39 |
| - | |
40 |
| - | |
| 36 | + | |
| 37 | + | |
| 38 | + | |
| 39 | + | |
| 40 | + | |
| 41 | + | |
| 42 | + | |
41 | 43 |
| |
42 | 44 |
| |
43 | 45 |
| |
|
Lines changed: 7 additions & 7 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
1 | 1 |
| |
2 | 2 |
| |
3 | 3 |
| |
4 |
| - | |
5 |
| - | |
6 |
| - | |
7 |
| - | |
| 4 | + | |
| 5 | + | |
8 | 6 |
| |
9 | 7 |
| |
10 |
| - | |
11 |
| - | |
12 |
| - | |
13 | 8 |
| |
14 | 9 |
| |
15 | 10 |
| |
16 | 11 |
| |
17 | 12 |
| |
18 | 13 |
| |
| 14 | + | |
| 15 | + | |
| 16 | + | |
| 17 | + | |
| 18 | + |
Lines changed: 1 addition & 1 deletion
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
21 | 21 |
| |
22 | 22 |
| |
23 | 23 |
| |
24 |
| - | |
25 | 24 |
| |
26 | 25 |
| |
27 | 26 |
| |
28 | 27 |
| |
29 | 28 |
| |
30 | 29 |
| |
| 30 | + | |
31 | 31 |
| |
32 | 32 |
| |
33 | 33 |
| |
|
Lines changed: 5 additions & 5 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
12 | 12 |
| |
13 | 13 |
| |
14 | 14 |
| |
15 |
| - | |
16 |
| - | |
17 |
| - | |
18 |
| - | |
| 15 | + | |
19 | 16 |
| |
20 | 17 |
| |
21 | 18 |
| |
22 | 19 |
| |
23 |
| - | |
| 20 | + | |
| 21 | + | |
| 22 | + | |
| 23 | + | |
24 | 24 |
| |
25 | 25 |
| |
26 | 26 |
| |
|
Lines changed: 5 additions & 5 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
10 | 10 |
| |
11 | 11 |
| |
12 | 12 |
| |
13 |
| - | |
14 |
| - | |
15 |
| - | |
16 |
| - | |
| 13 | + | |
17 | 14 |
| |
18 | 15 |
| |
19 | 16 |
| |
20 | 17 |
| |
21 |
| - | |
| 18 | + | |
| 19 | + | |
| 20 | + | |
| 21 | + | |
22 | 22 |
| |
23 | 23 |
| |
24 | 24 |
| |
|
Lines changed: 2 additions & 4 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
16 | 16 |
| |
17 | 17 |
| |
18 | 18 |
| |
19 |
| - | |
20 |
| - | |
21 |
| - | |
22 |
| - | |
| 19 | + | |
23 | 20 |
| |
24 | 21 |
| |
25 | 22 |
| |
26 | 23 |
| |
27 | 24 |
| |
28 | 25 |
| |
29 | 26 |
| |
| 27 | + | |
30 | 28 |
| |
31 | 29 |
| |
32 | 30 |
| |
|
Lines changed: 1 addition & 1 deletion
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
71 | 71 |
| |
72 | 72 |
| |
73 | 73 |
| |
74 |
| - | |
75 | 74 |
| |
76 | 75 |
| |
77 | 76 |
| |
| |||
86 | 85 |
| |
87 | 86 |
| |
88 | 87 |
| |
| 88 | + | |
89 | 89 |
| |
90 | 90 |
| |
91 | 91 |
| |
|
Lines changed: 5 additions & 3 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
455 | 455 |
| |
456 | 456 |
| |
457 | 457 |
| |
458 |
| - | |
| 458 | + | |
459 | 459 |
| |
460 | 460 |
| |
461 | 461 |
| |
| |||
469 | 469 |
| |
470 | 470 |
| |
471 | 471 |
| |
472 |
| - | |
| 472 | + | |
473 | 473 |
| |
| 474 | + | |
474 | 475 |
| |
475 | 476 |
| |
476 | 477 |
| |
| |||
612 | 613 |
| |
613 | 614 |
| |
614 | 615 |
| |
615 |
| - | |
| 616 | + | |
616 | 617 |
| |
617 | 618 |
| |
618 | 619 |
| |
| 620 | + | |
619 | 621 |
| |
620 | 622 |
| |
621 | 623 |
| |
|
Lines changed: 2 additions & 4 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
33 | 33 |
| |
34 | 34 |
| |
35 | 35 |
| |
36 |
| - | |
37 |
| - | |
38 |
| - | |
39 |
| - | |
| 36 | + | |
40 | 37 |
| |
41 | 38 |
| |
42 | 39 |
| |
| |||
47 | 44 |
| |
48 | 45 |
| |
49 | 46 |
| |
| 47 | + | |
50 | 48 |
| |
51 | 49 |
| |
52 | 50 |
| |
|
Lines changed: 3 additions & 5 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
28 | 28 |
| |
29 | 29 |
| |
30 | 30 |
| |
31 |
| - | |
32 |
| - | |
33 |
| - | |
34 |
| - | |
| 31 | + | |
35 | 32 |
| |
| 33 | + | |
36 | 34 |
| |
37 | 35 |
| |
38 | 36 |
| |
| |||
156 | 154 |
| |
157 | 155 |
| |
158 | 156 |
| |
159 |
| - | |
| 157 | + | |
160 | 158 |
| |
161 | 159 |
| |
162 | 160 |
| |
|
Lines changed: 4 additions & 3 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
7 | 7 |
| |
8 | 8 |
| |
9 | 9 |
| |
| 10 | + | |
10 | 11 |
| |
11 | 12 |
| |
12 | 13 |
| |
| |||
108 | 109 |
| |
109 | 110 |
| |
110 | 111 |
| |
111 |
| - | |
| 112 | + | |
112 | 113 |
| |
113 | 114 |
| |
114 | 115 |
| |
| |||
158 | 159 |
| |
159 | 160 |
| |
160 | 161 |
| |
161 |
| - | |
| 162 | + | |
162 | 163 |
| |
163 | 164 |
| |
164 | 165 |
| |
| |||
211 | 212 |
| |
212 | 213 |
| |
213 | 214 |
| |
214 |
| - | |
| 215 | + | |
215 | 216 |
| |
216 | 217 |
| |
217 | 218 |
| |
|
0 commit comments