diff --git a/Assets/logo.png b/Assets/logo.png
new file mode 100644
index 0000000..a3048c1
Binary files /dev/null and b/Assets/logo.png differ
diff --git a/README.md b/README.md
index a211f52..9330563 100644
--- a/README.md
+++ b/README.md
@@ -3,9 +3,12 @@
[](https://www.nuget.org/packages/calebs.extensions)
[](https://www.nuget.org/packages/calebs.extensions)
[](https://github.com/calebjenkins/Calebs.Extensions/actions/workflows/ci.yml)
+
+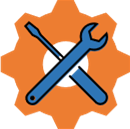
# Calebs.Extensions
Useful extension methods and attributes for working with enums, strings and lists. The majority of these extensions were born out of working with various models while building micro services.
+
### Installing Calebs.Extensions
You should install [Extensions with NuGet](https://www.nuget.org/packages/Calebs.Extensions):
@@ -63,6 +66,9 @@ To use this helper - register `IFileIO` is your `DI` with `FileIO` as the implme
- Compare
- string?.ValueOrEmpty()
+## ObjectExtensions
+- ToSafeString()
+
## ListExtensions
- ToDelimitedList
- ToUpper
@@ -87,3 +93,4 @@ Merges to `main` publish to nuget as a major release.
- 1.3.0 - added `IFileIO` - an interface + implementation for making common filesystem opperations easier to test
- 1.3.1 - suppressed some test warnings and updated the GH workflows
- 1.4.0 - added `CreatedDiretory` and `DeleteDirectory` to `IFileIO`
+- 1.5.0 - Added package logo and `ToSafeString` for `ObjectExtensions`
diff --git a/src/ExtensionTests/ObjectExtensionTests.cs b/src/ExtensionTests/ObjectExtensionTests.cs
new file mode 100644
index 0000000..84aa7d9
--- /dev/null
+++ b/src/ExtensionTests/ObjectExtensionTests.cs
@@ -0,0 +1,24 @@
+
+namespace ExtensionTests;
+
+using Calebs.Extensions;
+
+public class ObjectExtensionTests
+{
+ [Fact]
+ public void Nullable_Objects_Should_Return_Empty_String()
+ {
+ ExampleModel m = null;
+ var result = m.ToSafeString();
+ result.IsNullOrEmpty().Should().BeTrue();
+ }
+
+ [Fact]
+ public void Int_Should_Return_String_Value()
+ {
+ int five = 5;
+ var result = five.ToSafeString();
+ result.Should().Be("5");
+ }
+}
+
diff --git a/src/ExtensionTests/StringExtensionTests.cs b/src/ExtensionTests/StringExtensionTests.cs
index 3a63b21..383bc43 100644
--- a/src/ExtensionTests/StringExtensionTests.cs
+++ b/src/ExtensionTests/StringExtensionTests.cs
@@ -26,5 +26,4 @@ public void ShouldReturnValueIfNotNull()
v2.Should().Be(value);
}
-}
-
+}
\ No newline at end of file
diff --git a/src/Extensions/Console/ConsoleExtensions.cs b/src/Extensions/Console/ConsoleExtensions.cs
new file mode 100644
index 0000000..8f2f5e8
--- /dev/null
+++ b/src/Extensions/Console/ConsoleExtensions.cs
@@ -0,0 +1,20 @@
+
+namespace Calebs.Extensions.Console
+{
+ public static class ConsoleExtensions
+ {
+ public static void WriteLine (this System.ConsoleColor color, string text)
+ {
+ color.Write(text);
+ System.Console.WriteLine();
+ }
+
+ public static void Write (this System.ConsoleColor color, string text)
+ {
+ var currentColor = System.Console.ForegroundColor;
+ System.Console.ForegroundColor = color;
+ System.Console.Write(text);
+ System.Console.ForegroundColor = currentColor;
+ }
+ }
+}
diff --git a/src/Extensions/Extensions.csproj b/src/Extensions/Extensions.csproj
index 5b6c251..89ba40e 100644
--- a/src/Extensions/Extensions.csproj
+++ b/src/Extensions/Extensions.csproj
@@ -10,7 +10,7 @@
Debug;Release;NET7
Calebs.Extensions
Calebs.Extensions
- 1.4.0
+ 1.5.0
true
Caleb Jenkins
Caleb Jenkins
@@ -25,12 +25,16 @@
true
LICENSE
Calebs.Extensions
-
+ logo.png
Calebs.Extensions
+
+ True
+
+
True
diff --git a/src/Extensions/ObjectExtensions.cs b/src/Extensions/ObjectExtensions.cs
new file mode 100644
index 0000000..cdbc33b
--- /dev/null
+++ b/src/Extensions/ObjectExtensions.cs
@@ -0,0 +1,10 @@
+namespace Calebs.Extensions;
+
+public static class ObjectExtensions
+{
+ public static string ToSafeString(this object o)
+ {
+ return o?.ToString() ?? string.Empty;
+ }
+}
+
diff --git a/src/Extensions/StringExtensions.cs b/src/Extensions/StringExtensions.cs
index 1872ad7..1981f59 100644
--- a/src/Extensions/StringExtensions.cs
+++ b/src/Extensions/StringExtensions.cs
@@ -36,3 +36,4 @@ public static string ValueOrEmpty(this string? value)
return value;
}
}
+