You signed in with another tab or window. Reload to refresh your session.You signed out in another tab or window. Reload to refresh your session.You switched accounts on another tab or window. Reload to refresh your session.Dismiss alert
Our app is using a grid view with liquid pull to refresh. It works great if you have a lis that is long enough to overflow the view and cause it to scroll. However if you have a short list, the grid view is not scrolling and you cannot pull it to refresh.
To Reproduce
I attached your sample code edited to use a GridView with only 3 items in it. You can't pull down until you add more items into the list.
Describe the bug
Our app is using a grid view with liquid pull to refresh. It works great if you have a lis that is long enough to overflow the view and cause it to scroll. However if you have a short list, the grid view is not scrolling and you cannot pull it to refresh.
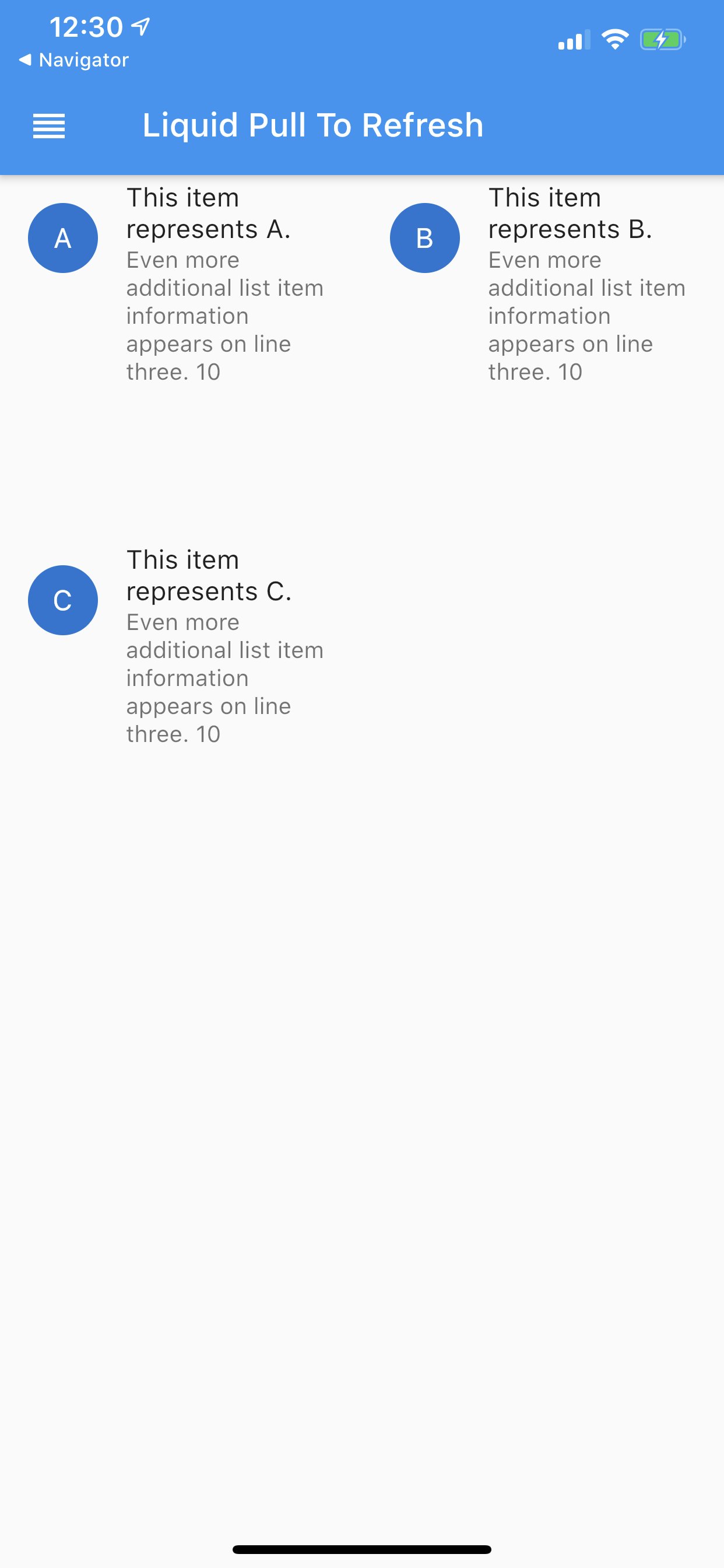
To Reproduce
I attached your sample code edited to use a GridView with only 3 items in it. You can't pull down until you add more items into the list.
Sample Code
import 'dart:async';
import 'dart:math';
import 'package:flutter/material.dart';
import 'package:liquid_pull_to_refresh/liquid_pull_to_refresh.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
// This widget is the root of your application.
@OverRide
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
debugShowCheckedModeBanner: false,
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: MyHomePage(title: 'Liquid Pull To Refresh'),
);
}
}
class MyHomePage extends StatefulWidget {
MyHomePage({Key key, this.title}) : super(key: key);
final String title;
@OverRide
_MyHomePageState createState() => _MyHomePageState();
}
class _MyHomePageState extends State {
final GlobalKey _scaffoldKey = GlobalKey();
final GlobalKey _refreshIndicatorKey =
GlobalKey();
static int refreshNum = 10; // number that changes when refreshed
Stream counterStream =
Stream.periodic(Duration(seconds: 3), (x) => refreshNum);
ScrollController _scrollController;
@OverRide
void initState() {
super.initState();
_scrollController = new ScrollController();
}
static final List _items = [
'A',
'B',
'C',
];
Future handleRefresh() {
final Completer completer = Completer();
Timer(const Duration(seconds: 3), () {
completer.complete();
});
setState(() {
refreshNum = new Random().nextInt(100);
});
return completer.future.then(() {
_scaffoldKey.currentState?.showSnackBar(SnackBar(
content: const Text('Refresh complete'),
action: SnackBarAction(
label: 'RETRY',
onPressed: () {
_refreshIndicatorKey.currentState.show();
})));
});
}
@OverRide
Widget build(BuildContext context) {
return Scaffold(
key: _scaffoldKey,
appBar: AppBar(
title: Stack(
children: [
Align(alignment: Alignment(-1.0, 0.0), child: Icon(Icons.reorder)),
Align(alignment: Alignment(-0.3, 0.0), child: Text(widget.title)),
],
),
),
body: LiquidPullToRefresh(
key: _refreshIndicatorKey,
onRefresh: _handleRefresh,
showChildOpacityTransition: false,
child: StreamBuilder(
stream: counterStream,
builder: (context, snapshot) {
return GridView.builder(
itemCount: _items.length,
controller: _scrollController,
itemBuilder: (BuildContext context, int index) {
final String item = _items[index];
return ListTile(
isThreeLine: true,
leading: CircleAvatar(child: Text(item)),
title: Text('This item represents $item.'),
subtitle: Text(
'Even more additional list item information appears on line three. ${snapshot.data}'),
);
},
gridDelegate: new SliverGridDelegateWithFixedCrossAxisCount(
crossAxisCount: 2),
);
}),
),
);
}
}
The text was updated successfully, but these errors were encountered: