-
Notifications
You must be signed in to change notification settings - Fork 0
/
Copy pathproblemset.jsonl
134 lines (134 loc) · 283 KB
/
problemset.jsonl
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
{"number": 3138, "title": "Minimum Length of Anagram Concatenation", "link": "https://leetcode.com/problems/minimum-length-of-anagram-concatenation/", "difficulty": "medium", "statement": "You are given a string `s`, which is known to be a concatenation of **anagrams** of some string `t`.\n\nReturn the **minimum** possible length of the string `t`.\n\nAn **anagram** is formed by rearranging the letters of a string. For example, \"aab\", \"aba\", and, \"baa\" are anagrams of \"aab\".\n\n**Example 1:**\n\n**Input:** s \\= \"abba\"\n\n**Output:** 2\n\n**Explanation:**\n\nOne possible string `t` could be `\"ba\"`.\n\n**Example 2:**\n\n**Input:** s \\= \"cdef\"\n\n**Output:** 4\n\n**Explanation:**\n\nOne possible string `t` could be `\"cdef\"`, notice that `t` can be equal to `s`.\n\n**Constraints:**\n\n* `1 <= s.length <= 105`\n* `s` consist only of lowercase English letters.", "templates": {"cpp": "class Solution {\npublic:\n int minAnagramLength(string s) {\n \n }\n};", "java": "class Solution {\n public int minAnagramLength(String s) {\n \n }\n}", "python": "class Solution:\n def minAnagramLength(self, s: str) -> int:\n "}, "hints": "1\\. The answer should be a divisor of `s.length`.\n2\\. Check each candidate naively.", "contains_png": false, "png_links": []}
{"number": 3139, "title": "Minimum Cost to Equalize Array", "link": "https://leetcode.com/problems/minimum-cost-to-equalize-array/", "difficulty": "hard", "statement": "You are given an integer array `nums` and two integers `cost1` and `cost2`. You are allowed to perform **either** of the following operations **any** number of times:\n\n* Choose an index `i` from `nums` and **increase** `nums[i]` by `1` for a cost of `cost1`.\n* Choose two **different** indices `i`, `j`, from `nums` and **increase** `nums[i]` and `nums[j]` by `1` for a cost of `cost2`.\n\nReturn the **minimum** **cost** required to make all elements in the array **equal***.* \n\nSince the answer may be very large, return it **modulo** `109 + 7`.\n\n**Example 1:**\n\n**Input:** nums \\= \\[4,1], cost1 \\= 5, cost2 \\= 2\n\n**Output:** 15\n\n**Explanation:** \n\nThe following operations can be performed to make the values equal:\n\n* Increase `nums[1]` by 1 for a cost of 5\\. `nums` becomes `[4,2]`.\n* Increase `nums[1]` by 1 for a cost of 5\\. `nums` becomes `[4,3]`.\n* Increase `nums[1]` by 1 for a cost of 5\\. `nums` becomes `[4,4]`.\n\nThe total cost is 15\\.\n\n**Example 2:**\n\n**Input:** nums \\= \\[2,3,3,3,5], cost1 \\= 2, cost2 \\= 1\n\n**Output:** 6\n\n**Explanation:** \n\nThe following operations can be performed to make the values equal:\n\n* Increase `nums[0]` and `nums[1]` by 1 for a cost of 1\\. `nums` becomes `[3,4,3,3,5]`.\n* Increase `nums[0]` and `nums[2]` by 1 for a cost of 1\\. `nums` becomes `[4,4,4,3,5]`.\n* Increase `nums[0]` and `nums[3]` by 1 for a cost of 1\\. `nums` becomes `[5,4,4,4,5]`.\n* Increase `nums[1]` and `nums[2]` by 1 for a cost of 1\\. `nums` becomes `[5,5,5,4,5]`.\n* Increase `nums[3]` by 1 for a cost of 2\\. `nums` becomes `[5,5,5,5,5]`.\n\nThe total cost is 6\\.\n\n**Example 3:**\n\n**Input:** nums \\= \\[3,5,3], cost1 \\= 1, cost2 \\= 3\n\n**Output:** 4\n\n**Explanation:**\n\nThe following operations can be performed to make the values equal:\n\n* Increase `nums[0]` by 1 for a cost of 1\\. `nums` becomes `[4,5,3]`.\n* Increase `nums[0]` by 1 for a cost of 1\\. `nums` becomes `[5,5,3]`.\n* Increase `nums[2]` by 1 for a cost of 1\\. `nums` becomes `[5,5,4]`.\n* Increase `nums[2]` by 1 for a cost of 1\\. `nums` becomes `[5,5,5]`.\n\nThe total cost is 4\\.\n\n**Constraints:**\n\n* `1 <= nums.length <= 105`\n* `1 <= nums[i] <= 106`\n* `1 <= cost1 <= 106`\n* `1 <= cost2 <= 106`", "templates": {"cpp": "class Solution {\npublic:\n int minCostToEqualizeArray(vector<int>& nums, int cost1, int cost2) {\n \n }\n};", "java": "class Solution {\n public int minCostToEqualizeArray(int[] nums, int cost1, int cost2) {\n \n }\n}", "python": "class Solution:\n def minCostToEqualizeArray(self, nums: List[int], cost1: int, cost2: int) -> int:\n "}, "hints": "1\\. How can you determine the minimum cost if you know the maximum value in the array once all values are made equal?\n2\\. If `cost2 > cost1 * 2`, we should just use `cost1` to change all the values to the maximum one.\n3\\. Otherwise, it's optimal to choose the smallest two values and use `cost2` to increase both of them.\n4\\. Since the maximum value is known, calculate the required increases to equalize all values, instead of naively simulating the operations.\n5\\. There are not a lot of candidates for the maximum; we can try all of them and choose which uses the minimum number of operations.", "contains_png": false, "png_links": []}
{"number": 3142, "title": "Check if Grid Satisfies Conditions", "link": "https://leetcode.com/problems/check-if-grid-satisfies-conditions/", "difficulty": "easy", "statement": "You are given a 2D matrix `grid` of size `m x n`. You need to check if each cell `grid[i][j]` is:\n\n* Equal to the cell below it, i.e. `grid[i][j] == grid[i + 1][j]` (if it exists).\n* Different from the cell to its right, i.e. `grid[i][j] != grid[i][j + 1]` (if it exists).\n\nReturn `true` if **all** the cells satisfy these conditions, otherwise, return `false`.\n\n**Example 1:**\n\n**Input:** grid \\= \\[\\[1,0,2],\\[1,0,2]]\n\n**Output:** true\n\n**Explanation:**\n\n**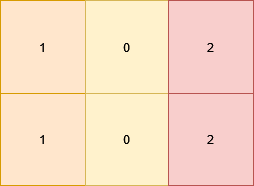**\n\nAll the cells in the grid satisfy the conditions.\n\n**Example 2:**\n\n**Input:** grid \\= \\[\\[1,1,1],\\[0,0,0]]\n\n**Output:** false\n\n**Explanation:**\n\n**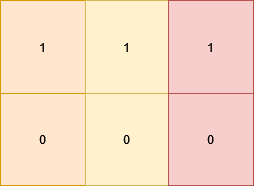**\n\nAll cells in the first row are equal.\n\n**Example 3:**\n\n**Input:** grid \\= \\[\\[1],\\[2],\\[3]]\n\n**Output:** false\n\n**Explanation:**\n\n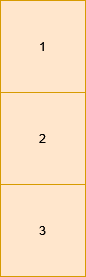\n\nCells in the first column have different values.\n\n**Constraints:**\n\n* `1 <= n, m <= 10`\n* `0 <= grid[i][j] <= 9`", "templates": {"cpp": "class Solution {\npublic:\n bool satisfiesConditions(vector<vector<int>>& grid) {\n \n }\n};", "java": "class Solution {\n public boolean satisfiesConditions(int[][] grid) {\n \n }\n}", "python": "class Solution:\n def satisfiesConditions(self, grid: List[List[int]]) -> bool:\n "}, "hints": "1\\. Check if each column has same value in each cell.\n2\\. If the previous condition is satisfied, we can simply check the first cells in adjacent columns.", "contains_png": true, "png_links": ["https://assets.leetcode.com/uploads/2024/04/15/examplechanged.png", "https://assets.leetcode.com/uploads/2024/03/27/example21.png", "https://assets.leetcode.com/uploads/2024/03/31/changed.png"]}
{"number": 3143, "title": "Maximum Points Inside the Square", "link": "https://leetcode.com/problems/maximum-points-inside-the-square/", "difficulty": "medium", "statement": "You are given a 2Darray `points` and a string `s` where, `points[i]` represents the coordinates of point `i`, and `s[i]` represents the **tag** of point `i`.\n\nA **valid** square is a square centered at the origin `(0, 0)`, has edges parallel to the axes, and **does not** contain two points with the same tag.\n\nReturn the **maximum** number of points contained in a **valid** square.\n\nNote:\n\n* A point is considered to be inside the square if it lies on or within the square's boundaries.\n* The side length of the square can be zero.\n\n**Example 1:**\n\n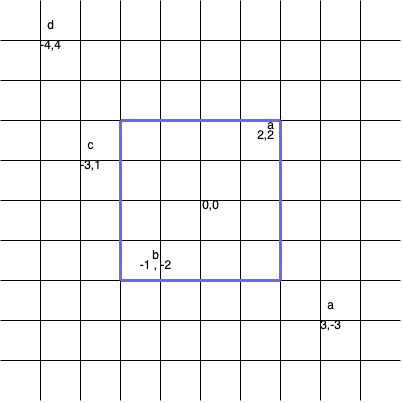\n\n**Input:** points \\= \\[\\[2,2],\\[\\-1,\\-2],\\[\\-4,4],\\[\\-3,1],\\[3,\\-3]], s \\= \"abdca\"\n\n**Output:** 2\n\n**Explanation:**\n\nThe square of side length 4 covers two points `points[0]` and `points[1]`.\n\n**Example 2:**\n\n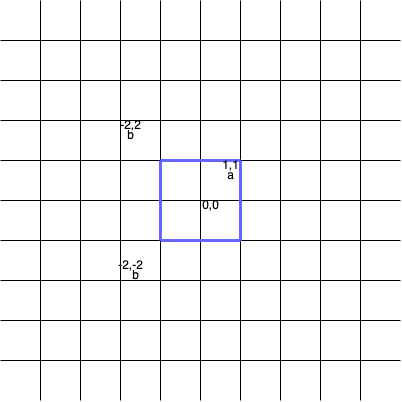\n\n**Input:** points \\= \\[\\[1,1],\\[\\-2,\\-2],\\[\\-2,2]], s \\= \"abb\"\n\n**Output:** 1\n\n**Explanation:**\n\nThe square of side length 2 covers one point, which is `points[0]`.\n\n**Example 3:**\n\n**Input:** points \\= \\[\\[1,1],\\[\\-1,\\-1],\\[2,\\-2]], s \\= \"ccd\"\n\n**Output:** 0\n\n**Explanation:**\n\nIt's impossible to make any valid squares centered at the origin such that it covers only one point among `points[0]` and `points[1]`.\n\n**Constraints:**\n\n* `1 <= s.length, points.length <= 105`\n* `points[i].length == 2`\n* `-109 <= points[i][0], points[i][1] <= 109`\n* `s.length == points.length`\n* `points` consists of distinct coordinates.\n* `s` consists only of lowercase English letters.", "templates": {"cpp": "class Solution {\npublic:\n int maxPointsInsideSquare(vector<vector<int>>& points, string s) {\n \n }\n};", "java": "class Solution {\n public int maxPointsInsideSquare(int[][] points, String s) {\n \n }\n}", "python": "class Solution:\n def maxPointsInsideSquare(self, points: List[List[int]], s: str) -> int:\n "}, "hints": "1\\. The smallest edge length of a square to include point `(x, y)` is `max(abs(x), abs(y)) * 2`.\n2\\. Sort the points by `max(abs(x), abs(y))` and try each edge length, check the included point tags.", "contains_png": true, "png_links": ["https://assets.leetcode.com/uploads/2024/03/29/3708-tc1.png", "https://assets.leetcode.com/uploads/2024/03/29/3708-tc2.png"]}
{"number": 3144, "title": "Minimum Substring Partition of Equal Character Frequency", "link": "https://leetcode.com/problems/minimum-substring-partition-of-equal-character-frequency/", "difficulty": "medium", "statement": "Given a string `s`, you need to partition it into one or more **balanced** substrings. For example, if `s == \"ababcc\"` then `(\"abab\", \"c\", \"c\")`, `(\"ab\", \"abc\", \"c\")`, and `(\"ababcc\")` are all valid partitions, but `(\"a\", \"bab\", \"cc\")`, `(\"aba\", \"bc\", \"c\")`, and `(\"ab\", \"abcc\")` are not. The unbalanced substrings are bolded.\n\nReturn the **minimum** number of substrings that you can partition `s` into.\n\n**Note:** A **balanced** string is a string where each character in the string occurs the same number of times.\n\n**Example 1:**\n\n**Input:** s \\= \"fabccddg\"\n\n**Output:** 3\n\n**Explanation:**\n\nWe can partition the string `s` into 3 substrings in one of the following ways: `(\"fab, \"ccdd\", \"g\")`, or `(\"fabc\", \"cd\", \"dg\")`.\n\n**Example 2:**\n\n**Input:** s \\= \"abababaccddb\"\n\n**Output:** 2\n\n**Explanation:**\n\nWe can partition the string `s` into 2 substrings like so: `(\"abab\", \"abaccddb\")`.\n\n**Constraints:**\n\n* `1 <= s.length <= 1000`\n* `s` consists only of English lowercase letters.", "templates": {"cpp": "class Solution {\npublic:\n int minimumSubstringsInPartition(string s) {\n \n }\n};", "java": "class Solution {\n public int minimumSubstringsInPartition(String s) {\n \n }\n}", "python": "class Solution:\n def minimumSubstringsInPartition(self, s: str) -> int:\n "}, "hints": "1\\. Let `dp[i]` be the minimum number of partitions for the prefix ending at index `i + 1`.\n2\\. `dp[i]` can be calculated as the `min(dp[j])` over all `j` such that `j < i` and `word[j+1\u2026i]` is valid.", "contains_png": false, "png_links": []}
{"number": 3145, "title": "Find Products of Elements of Big Array", "link": "https://leetcode.com/problems/find-products-of-elements-of-big-array/", "difficulty": "hard", "statement": "The **powerful array** of a non\\-negative integer `x` is defined as the shortest sorted array of powers of two that sum up to `x`. The table below illustrates examples of how the **powerful array** is determined. It can be proven that the powerful array of `x` is unique.\n\n| num | Binary Representation | powerful array |\n| --- | --- | --- |\n| 1 | 00001 | \\[1] |\n| 8 | 01000 | \\[8] |\n| 10 | 01010 | \\[2, 8] |\n| 13 | 01101 | \\[1, 4, 8] |\n| 23 | 10111 | \\[1, 2, 4, 16] |\n\nThe array `big_nums` is created by concatenating the **powerful arrays** for every positive integer `i` in ascending order: 1, 2, 3, and so on. Thus, `big_nums` begins as `[1, 2, 1, 2, 4, 1, 4, 2, 4, 1, 2, 4, 8, ...]`.\n\nYou are given a 2D integer matrix `queries`, where for `queries[i] = [fromi, toi, modi]` you should calculate `(big_nums[fromi] * big_nums[fromi + 1] * ... * big_nums[toi]) % modi`.\n\nReturn an integer array `answer` such that `answer[i]` is the answer to the `ith` query.\n\n**Example 1:**\n\n**Input:** queries \\= \\[\\[1,3,7]]\n\n**Output:** \\[4]\n\n**Explanation:**\n\nThere is one query.\n\n`big_nums[1..3] = [2,1,2]`. The product of them is 4\\. The result is `4 % 7 = 4.`\n\n**Example 2:**\n\n**Input:** queries \\= \\[\\[2,5,3],\\[7,7,4]]\n\n**Output:** \\[2,2]\n\n**Explanation:**\n\nThere are two queries.\n\nFirst query: `big_nums[2..5] = [1,2,4,1]`. The product of them is 8\\. The result is `8 % 3 = 2`.\n\nSecond query: `big_nums[7] = 2`. The result is `2 % 4 = 2`.\n\n**Constraints:**\n\n* `1 <= queries.length <= 500`\n* `queries[i].length == 3`\n* `0 <= queries[i][0] <= queries[i][1] <= 1015`\n* `1 <= queries[i][2] <= 105`", "templates": {"cpp": "class Solution {\npublic:\n vector<int> findProductsOfElements(vector<vector<long long>>& queries) {\n \n }\n};", "java": "class Solution {\n public int[] findProductsOfElements(long[][] queries) {\n \n }\n}", "python": "class Solution:\n def findProductsOfElements(self, queries: List[List[int]]) -> List[int]:\n "}, "hints": "1\\. Find a way to calculate `f(n, i)` which is the total number of numbers in `[1, n]` when the `ith` bit is set in `O(log(n))` time.\n2\\. Use binary search to find the last number for each query (and there might be one \u201cincomplete\u201d number for the query).\n3\\. Use a similar way to find the product (we only need to save the sum of exponents of power of `2`).", "contains_png": false, "png_links": []}
{"number": 3146, "title": "Permutation Difference between Two Strings", "link": "https://leetcode.com/problems/permutation-difference-between-two-strings/", "difficulty": "easy", "statement": "You are given two strings `s` and `t` such that every character occurs at most once in `s` and `t` is a permutation of `s`.\n\nThe **permutation difference** between `s` and `t` is defined as the **sum** of the absolute difference between the index of the occurrence of each character in `s` and the index of the occurrence of the same character in `t`.\n\nReturn the **permutation difference** between `s` and `t`.\n\n**Example 1:**\n\n**Input:** s \\= \"abc\", t \\= \"bac\"\n\n**Output:** 2\n\n**Explanation:**\n\nFor `s = \"abc\"` and `t = \"bac\"`, the permutation difference of `s` and `t` is equal to the sum of:\n\n* The absolute difference between the index of the occurrence of `\"a\"` in `s` and the index of the occurrence of `\"a\"` in `t`.\n* The absolute difference between the index of the occurrence of `\"b\"` in `s` and the index of the occurrence of `\"b\"` in `t`.\n* The absolute difference between the index of the occurrence of `\"c\"` in `s` and the index of the occurrence of `\"c\"` in `t`.\n\nThat is, the permutation difference between `s` and `t` is equal to `|0 - 1| + |1 - 0| + |2 - 2| = 2`.\n\n**Example 2:**\n\n**Input:** s \\= \"abcde\", t \\= \"edbac\"\n\n**Output:** 12\n\n**Explanation:** The permutation difference between `s` and `t` is equal to `|0 - 3| + |1 - 2| + |2 - 4| + |3 - 1| + |4 - 0| = 12`.\n\n**Constraints:**\n\n* `1 <= s.length <= 26`\n* Each character occurs at most once in `s`.\n* `t` is a permutation of `s`.\n* `s` consists only of lowercase English letters.", "templates": {"cpp": "class Solution {\npublic:\n int findPermutationDifference(string s, string t) {\n \n }\n};", "java": "class Solution {\n public int findPermutationDifference(String s, String t) {\n \n }\n}", "python": "class Solution:\n def findPermutationDifference(self, s: str, t: str) -> int:\n "}, "hints": "1\\. For each character, find the indices of its occurrences in string `s` then in string `t`.", "contains_png": false, "png_links": []}
{"number": 3147, "title": "Taking Maximum Energy From the Mystic Dungeon", "link": "https://leetcode.com/problems/taking-maximum-energy-from-the-mystic-dungeon/", "difficulty": "medium", "statement": "In a mystic dungeon, `n` magicians are standing in a line. Each magician has an attribute that gives you energy. Some magicians can give you negative energy, which means taking energy from you.\n\nYou have been cursed in such a way that after absorbing energy from magician `i`, you will be instantly transported to magician `(i + k)`. This process will be repeated until you reach the magician where `(i + k)` does not exist.\n\nIn other words, you will choose a starting point and then teleport with `k` jumps until you reach the end of the magicians' sequence, **absorbing all the energy** during the journey.\n\nYou are given an array `energy` and an integer `k`. Return the **maximum** possible energy you can gain.\n\n**Example 1:**\n\n**Input:** energy \\= \\[5,2,\\-10,\\-5,1], k \\= 3\n\n**Output:** 3\n\n**Explanation:** We can gain a total energy of 3 by starting from magician 1 absorbing 2 \\+ 1 \\= 3\\.\n\n**Example 2:**\n\n**Input:** energy \\= \\[\\-2,\\-3,\\-1], k \\= 2\n\n**Output:** \\-1\n\n**Explanation:** We can gain a total energy of \\-1 by starting from magician 2\\.\n\n**Constraints:**\n\n* `1 <= energy.length <= 105`\n* `-1000 <= energy[i] <= 1000`\n* `1 <= k <= energy.length - 1`\n\n\u200b\u200b\u200b\u200b\u200b\u200b", "templates": {"cpp": "class Solution {\npublic:\n int maximumEnergy(vector<int>& energy, int k) {\n \n }\n};", "java": "class Solution {\n public int maximumEnergy(int[] energy, int k) {\n \n }\n}", "python": "class Solution:\n def maximumEnergy(self, energy: List[int], k: int) -> int:\n "}, "hints": "1\\. Let `dp[i]` denote the energy we gain starting from index `i`.\n2\\. We can notice, that `dp[i] = dp[i + k] + energy[i]`.", "contains_png": false, "png_links": []}
{"number": 3148, "title": "Maximum Difference Score in a Grid", "link": "https://leetcode.com/problems/maximum-difference-score-in-a-grid/", "difficulty": "medium", "statement": "You are given an `m x n` matrix `grid` consisting of **positive** integers. You can move from a cell in the matrix to **any** other cell that is either to the bottom or to the right (not necessarily adjacent). The score of a move from a cell with the value `c1` to a cell with the value `c2` is `c2 - c1`.\n\nYou can start at **any** cell, and you have to make **at least** one move.\n\nReturn the **maximum** total score you can achieve.\n\n**Example 1:**\n\n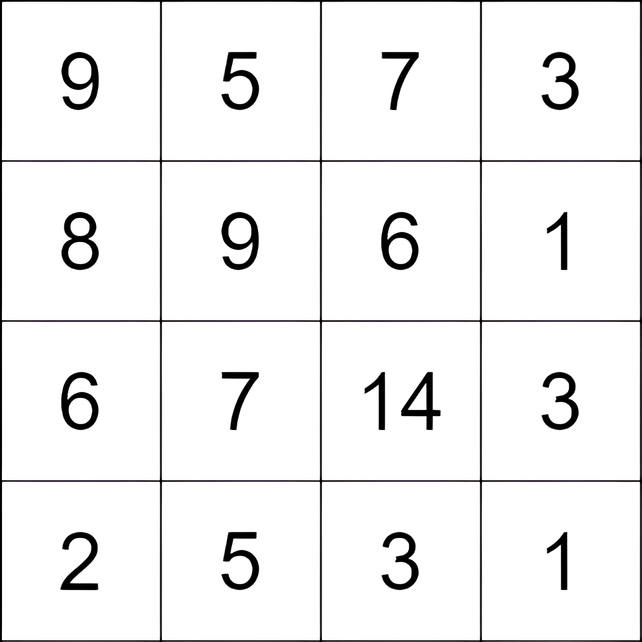\n\n**Input:** grid \\= \\[\\[9,5,7,3],\\[8,9,6,1],\\[6,7,14,3],\\[2,5,3,1]]\n\n**Output:** 9\n\n**Explanation:** We start at the cell `(0, 1)`, and we perform the following moves: \n\n\\- Move from the cell `(0, 1)` to `(2, 1)` with a score of `7 - 5 = 2`. \n\n\\- Move from the cell `(2, 1)` to `(2, 2)` with a score of `14 - 7 = 7`. \n\nThe total score is `2 + 7 = 9`.\n\n**Example 2:**\n\n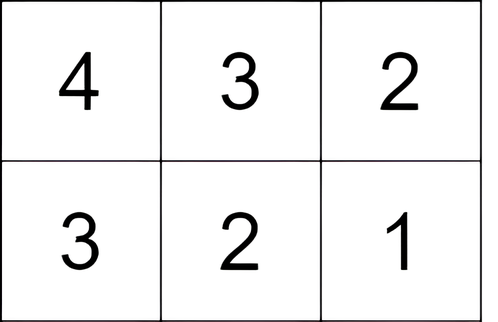\n\n**Input:** grid \\= \\[\\[4,3,2],\\[3,2,1]]\n\n**Output:** \\-1\n\n**Explanation:** We start at the cell `(0, 0)`, and we perform one move: `(0, 0)` to `(0, 1)`. The score is `3 - 4 = -1`.\n\n**Constraints:**\n\n* `m == grid.length`\n* `n == grid[i].length`\n* `2 <= m, n <= 1000`\n* `4 <= m * n <= 105`\n* `1 <= grid[i][j] <= 105`", "templates": {"cpp": "class Solution {\npublic:\n int maxScore(vector<vector<int>>& grid) {\n \n }\n};", "java": "class Solution {\n public int maxScore(List<List<Integer>> grid) {\n \n }\n}", "python": "class Solution:\n def maxScore(self, grid: List[List[int]]) -> int:\n "}, "hints": "1\\. Any path from a cell `(x1, y1)` to another cell `(x2, y2)` will always have a score of `grid[x2][y2] - grid[x1][y1]`.\n2\\. Let\u2019s say we fix the starting cell `(x1, y1)`, how to the find a cell `(x2, y2)` such that the value `grid[x2][y2] - grid[x1][y1]` is the maximum possible?", "contains_png": true, "png_links": ["https://assets.leetcode.com/uploads/2024/03/14/grid1.png", "https://assets.leetcode.com/uploads/2024/04/08/moregridsdrawio-1.png"]}
{"number": 3149, "title": "Find the Minimum Cost Array Permutation", "link": "https://leetcode.com/problems/find-the-minimum-cost-array-permutation/", "difficulty": "hard", "statement": "You are given an array `nums` which is a permutation of `[0, 1, 2, ..., n - 1]`. The **score** of any permutation of `[0, 1, 2, ..., n - 1]` named `perm` is defined as:\n\n`score(perm) = |perm[0] - nums[perm[1]]| + |perm[1] - nums[perm[2]]| + ... + |perm[n - 1] - nums[perm[0]]|`\n\nReturn the permutation `perm` which has the **minimum** possible score. If *multiple* permutations exist with this score, return the one that is lexicographically smallest among them.\n\n**Example 1:**\n\n**Input:** nums \\= \\[1,0,2]\n\n**Output:** \\[0,1,2]\n\n**Explanation:**\n\n**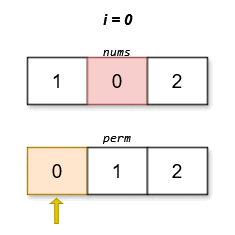**\n\nThe lexicographically smallest permutation with minimum cost is `[0,1,2]`. The cost of this permutation is `|0 - 0| + |1 - 2| + |2 - 1| = 2`.\n\n**Example 2:**\n\n**Input:** nums \\= \\[0,2,1]\n\n**Output:** \\[0,2,1]\n\n**Explanation:**\n\n**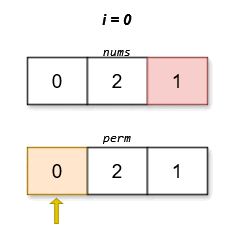**\n\nThe lexicographically smallest permutation with minimum cost is `[0,2,1]`. The cost of this permutation is `|0 - 1| + |2 - 2| + |1 - 0| = 2`.\n\n**Constraints:**\n\n* `2 <= n == nums.length <= 14`\n* `nums` is a permutation of `[0, 1, 2, ..., n - 1]`.", "templates": {"cpp": "class Solution {\npublic:\n vector<int> findPermutation(vector<int>& nums) {\n \n }\n};", "java": "class Solution {\n public int[] findPermutation(int[] nums) {\n \n }\n}", "python": "class Solution:\n def findPermutation(self, nums: List[int]) -> List[int]:\n "}, "hints": "1\\. The score function is cyclic, so we can always set `perm[0] = 0` for the smallest lexical order.\n2\\. It\u2019s similar to the Traveling Salesman Problem. Use Dynamic Programming.\n3\\. Use a bitmask to track which elements have been assigned to `perm`.", "contains_png": false, "png_links": []}
{"number": 3151, "title": "Special Array I", "link": "https://leetcode.com/problems/special-array-i/", "difficulty": "easy", "statement": "An array is considered **special** if every pair of its adjacent elements contains two numbers with different parity.\n\nYou are given an array of integers `nums`. Return `true` if `nums` is a **special** array, otherwise, return `false`.\n\n**Example 1:**\n\n**Input:** nums \\= \\[1]\n\n**Output:** true\n\n**Explanation:**\n\nThere is only one element. So the answer is `true`.\n\n**Example 2:**\n\n**Input:** nums \\= \\[2,1,4]\n\n**Output:** true\n\n**Explanation:**\n\nThere is only two pairs: `(2,1)` and `(1,4)`, and both of them contain numbers with different parity. So the answer is `true`.\n\n**Example 3:**\n\n**Input:** nums \\= \\[4,3,1,6]\n\n**Output:** false\n\n**Explanation:**\n\n`nums[1]` and `nums[2]` are both odd. So the answer is `false`.\n\n**Constraints:**\n\n* `1 <= nums.length <= 100`\n* `1 <= nums[i] <= 100`", "templates": {"cpp": "class Solution {\npublic:\n bool isArraySpecial(vector<int>& nums) {\n \n }\n};", "java": "class Solution {\n public boolean isArraySpecial(int[] nums) {\n \n }\n}", "python": "class Solution:\n def isArraySpecial(self, nums: List[int]) -> bool:\n "}, "hints": "1\\. Try to check the parity of each element and its previous element.", "contains_png": false, "png_links": []}
{"number": 3152, "title": "Special Array II", "link": "https://leetcode.com/problems/special-array-ii/", "difficulty": "medium", "statement": "An array is considered **special** if every pair of its adjacent elements contains two numbers with different parity.\n\nYou are given an array of integer `nums` and a 2D integer matrix `queries`, where for `queries[i] = [fromi, toi]` your task is to check that subarray `nums[fromi..toi]` is **special** or not.\n\nReturn an array of booleans `answer` such that `answer[i]` is `true` if `nums[fromi..toi]` is special.\n\n**Example 1:**\n\n**Input:** nums \\= \\[3,4,1,2,6], queries \\= \\[\\[0,4]]\n\n**Output:** \\[false]\n\n**Explanation:**\n\nThe subarray is `[3,4,1,2,6]`. 2 and 6 are both even.\n\n**Example 2:**\n\n**Input:** nums \\= \\[4,3,1,6], queries \\= \\[\\[0,2],\\[2,3]]\n\n**Output:** \\[false,true]\n\n**Explanation:**\n\n1. The subarray is `[4,3,1]`. 3 and 1 are both odd. So the answer to this query is `false`.\n2. The subarray is `[1,6]`. There is only one pair: `(1,6)` and it contains numbers with different parity. So the answer to this query is `true`.\n\n**Constraints:**\n\n* `1 <= nums.length <= 105`\n* `1 <= nums[i] <= 105`\n* `1 <= queries.length <= 105`\n* `queries[i].length == 2`\n* `0 <= queries[i][0] <= queries[i][1] <= nums.length - 1`", "templates": {"cpp": "class Solution {\npublic:\n vector<bool> isArraySpecial(vector<int>& nums, vector<vector<int>>& queries) {\n \n }\n};", "java": "class Solution {\n public boolean[] isArraySpecial(int[] nums, int[][] queries) {\n \n }\n}", "python": "class Solution:\n def isArraySpecial(self, nums: List[int], queries: List[List[int]]) -> List[bool]:\n "}, "hints": "1\\. Try to split the array into some non\\-intersected continuous special subarrays.\n2\\. For each query check that the first and the last elements of that query are in the same subarray or not.", "contains_png": false, "png_links": []}
{"number": 3153, "title": "Sum of Digit Differences of All Pairs", "link": "https://leetcode.com/problems/sum-of-digit-differences-of-all-pairs/", "difficulty": "medium", "statement": "You are given an array `nums` consisting of **positive** integers where all integers have the **same** number of digits.\n\nThe **digit difference** between two integers is the *count* of different digits that are in the **same** position in the two integers.\n\nReturn the **sum** of the **digit differences** between **all** pairs of integers in `nums`.\n\n**Example 1:**\n\n**Input:** nums \\= \\[13,23,12]\n\n**Output:** 4\n\n**Explanation:** \n\nWe have the following: \n\n\\- The digit difference between **1**3 and **2**3 is 1\\. \n\n\\- The digit difference between 1**3** and 1**2** is 1\\. \n\n\\- The digit difference between **23** and **12** is 2\\. \n\nSo the total sum of digit differences between all pairs of integers is `1 + 1 + 2 = 4`.\n\n**Example 2:**\n\n**Input:** nums \\= \\[10,10,10,10]\n\n**Output:** 0\n\n**Explanation:** \n\nAll the integers in the array are the same. So the total sum of digit differences between all pairs of integers will be 0\\.\n\n**Constraints:**\n\n* `2 <= nums.length <= 105`\n* `1 <= nums[i] < 109`\n* All integers in `nums` have the same number of digits.", "templates": {"cpp": "class Solution {\npublic:\n long long sumDigitDifferences(vector<int>& nums) {\n \n }\n};", "java": "class Solution {\n public long sumDigitDifferences(int[] nums) {\n \n }\n}", "python": "class Solution:\n def sumDigitDifferences(self, nums: List[int]) -> int:\n "}, "hints": "1\\. You can solve the problem for digits that are on the same position separately, and then sum up all the answers.\n2\\. For each position, count the number of occurences of each digit from 0 to 9 that appear on that position.\n3\\. Let `c` be the number of occurences of a digit on a position, that will contribute with `c * (n - c)` to the final answer, where `n` is the number of integers in `nums`.", "contains_png": false, "png_links": []}
{"number": 3154, "title": "Find Number of Ways to Reach the K-th Stair", "link": "https://leetcode.com/problems/find-number-of-ways-to-reach-the-k-th-stair/", "difficulty": "hard", "statement": "You are given a **non\\-negative** integer `k`. There exists a staircase with an infinite number of stairs, with the **lowest** stair numbered 0\\.\n\nAlice has an integer `jump`, with an initial value of 0\\. She starts on stair 1 and wants to reach stair `k` using **any** number of **operations**. If she is on stair `i`, in one **operation** she can:\n\n* Go down to stair `i - 1`. This operation **cannot** be used consecutively or on stair 0\\.\n* Go up to stair `i + 2jump`. And then, `jump` becomes `jump + 1`.\n\nReturn the *total* number of ways Alice can reach stair `k`.\n\n**Note** that it is possible that Alice reaches the stair `k`, and performs some operations to reach the stair `k` again.\n\n**Example 1:**\n\n**Input:** k \\= 0\n\n**Output:** 2\n\n**Explanation:**\n\nThe 2 possible ways of reaching stair 0 are:\n\n* Alice starts at stair 1\\.\n\t+ Using an operation of the first type, she goes down 1 stair to reach stair 0\\.\n* Alice starts at stair 1\\.\n\t+ Using an operation of the first type, she goes down 1 stair to reach stair 0\\.\n\t+ Using an operation of the second type, she goes up 20 stairs to reach stair 1\\.\n\t+ Using an operation of the first type, she goes down 1 stair to reach stair 0\\.\n\n**Example 2:**\n\n**Input:** k \\= 1\n\n**Output:** 4\n\n**Explanation:**\n\nThe 4 possible ways of reaching stair 1 are:\n\n* Alice starts at stair 1\\. Alice is at stair 1\\.\n* Alice starts at stair 1\\.\n\t+ Using an operation of the first type, she goes down 1 stair to reach stair 0\\.\n\t+ Using an operation of the second type, she goes up 20 stairs to reach stair 1\\.\n* Alice starts at stair 1\\.\n\t+ Using an operation of the second type, she goes up 20 stairs to reach stair 2\\.\n\t+ Using an operation of the first type, she goes down 1 stair to reach stair 1\\.\n* Alice starts at stair 1\\.\n\t+ Using an operation of the first type, she goes down 1 stair to reach stair 0\\.\n\t+ Using an operation of the second type, she goes up 20 stairs to reach stair 1\\.\n\t+ Using an operation of the first type, she goes down 1 stair to reach stair 0\\.\n\t+ Using an operation of the second type, she goes up 21 stairs to reach stair 2\\.\n\t+ Using an operation of the first type, she goes down 1 stair to reach stair 1\\.\n\n**Constraints:**\n\n* `0 <= k <= 109`", "templates": {"cpp": "class Solution {\npublic:\n int waysToReachStair(int k) {\n \n }\n};", "java": "class Solution {\n public int waysToReachStair(int k) {\n \n }\n}", "python": "class Solution:\n def waysToReachStair(self, k: int) -> int:\n "}, "hints": "1\\. On using `x` operations of the second type and `y` operations of the first type, the stair `2x - y` is reached.\n2\\. Since first operations cannot be consecutive, there are exactly `x + 1` positions (before and after each power of 2\\) to perform the second operation.\n3\\. Using combinatorics, we have x \\+ 1Cy number of ways to select the positions of second operations.", "contains_png": false, "png_links": []}
{"number": 3158, "title": "Find the XOR of Numbers Which Appear Twice", "link": "https://leetcode.com/problems/find-the-xor-of-numbers-which-appear-twice/", "difficulty": "easy", "statement": "You are given an array `nums`, where each number in the array appears **either**onceortwice.\n\nReturn the bitwise`XOR` of all the numbers that appear twice in the array, or 0 if no number appears twice.\n\n**Example 1:**\n\n**Input:** nums \\= \\[1,2,1,3]\n\n**Output:** 1\n\n**Explanation:**\n\nThe only number that appears twice in\u00a0`nums`\u00a0is 1\\.\n\n**Example 2:**\n\n**Input:** nums \\= \\[1,2,3]\n\n**Output:** 0\n\n**Explanation:**\n\nNo number appears twice in\u00a0`nums`.\n\n**Example 3:**\n\n**Input:** nums \\= \\[1,2,2,1]\n\n**Output:** 3\n\n**Explanation:**\n\nNumbers 1 and 2 appeared twice. `1 XOR 2 == 3`.\n\n**Constraints:**\n\n* `1 <= nums.length <= 50`\n* `1 <= nums[i] <= 50`\n* Each number in `nums` appears either once or twice.", "templates": {"cpp": "class Solution {\npublic:\n int duplicateNumbersXOR(vector<int>& nums) {\n \n }\n};", "java": "class Solution {\n public int duplicateNumbersXOR(int[] nums) {\n \n }\n}", "python": "class Solution:\n def duplicateNumbersXOR(self, nums: List[int]) -> int:\n "}, "hints": "1\\. The constraints are small. Brute force checking each value in the array.", "contains_png": false, "png_links": []}
{"number": 3159, "title": "Find Occurrences of an Element in an Array", "link": "https://leetcode.com/problems/find-occurrences-of-an-element-in-an-array/", "difficulty": "medium", "statement": "You are given an integer array `nums`, an integer array `queries`, and an integer `x`.\n\nFor each `queries[i]`, you need to find the index of the `queries[i]th` occurrence of `x` in the `nums` array. If there are fewer than `queries[i]` occurrences of `x`, the answer should be \\-1 for that query.\n\nReturn an integer array `answer` containing the answers to all queries.\n\n**Example 1:**\n\n**Input:** nums \\= \\[1,3,1,7], queries \\= \\[1,3,2,4], x \\= 1\n\n**Output:** \\[0,\\-1,2,\\-1]\n\n**Explanation:**\n\n* For the 1st query, the first occurrence of 1 is at index 0\\.\n* For the 2nd query, there are only two occurrences of 1 in `nums`, so the answer is \\-1\\.\n* For the 3rd query, the second occurrence of 1 is at index 2\\.\n* For the 4th query, there are only two occurrences of 1 in `nums`, so the answer is \\-1\\.\n\n**Example 2:**\n\n**Input:** nums \\= \\[1,2,3], queries \\= \\[10], x \\= 5\n\n**Output:** \\[\\-1]\n\n**Explanation:**\n\n* For the 1st query, 5 doesn't exist in `nums`, so the answer is \\-1\\.\n\n**Constraints:**\n\n* `1 <= nums.length, queries.length <= 105`\n* `1 <= queries[i] <= 105`\n* `1 <= nums[i], x <= 104`", "templates": {"cpp": "class Solution {\npublic:\n vector<int> occurrencesOfElement(vector<int>& nums, vector<int>& queries, int x) {\n \n }\n};", "java": "class Solution {\n public int[] occurrencesOfElement(int[] nums, int[] queries, int x) {\n \n }\n}", "python": "class Solution:\n def occurrencesOfElement(self, nums: List[int], queries: List[int], x: int) -> List[int]:\n "}, "hints": "1\\. Compress the array `nums` and save all the occurrences of each element in the separate arrays.", "contains_png": false, "png_links": []}
{"number": 3160, "title": "Find the Number of Distinct Colors Among the Balls", "link": "https://leetcode.com/problems/find-the-number-of-distinct-colors-among-the-balls/", "difficulty": "medium", "statement": "You are given an integer `limit` and a 2D array `queries` of size `n x 2`.\n\nThere are `limit + 1` balls with **distinct** labels in the range `[0, limit]`. Initially, all balls are uncolored. For every query in `queries` that is of the form `[x, y]`, you mark ball `x` with the color `y`. After each query, you need to find the number of **distinct** colors among the balls.\n\nReturn an array `result` of length `n`, where `result[i]` denotes the number of distinct colors *after* `ith` query.\n\n**Note** that when answering a query, lack of a color *will not* be considered as a color.\n\n**Example 1:**\n\n**Input:** limit \\= 4, queries \\= \\[\\[1,4],\\[2,5],\\[1,3],\\[3,4]]\n\n**Output:** \\[1,2,2,3]\n\n**Explanation:**\n\n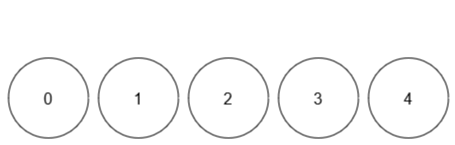\n\n* After query 0, ball 1 has color 4\\.\n* After query 1, ball 1 has color 4, and ball 2 has color 5\\.\n* After query 2, ball 1 has color 3, and ball 2 has color 5\\.\n* After query 3, ball 1 has color 3, ball 2 has color 5, and ball 3 has color 4\\.\n\n**Example 2:**\n\n**Input:** limit \\= 4, queries \\= \\[\\[0,1],\\[1,2],\\[2,2],\\[3,4],\\[4,5]]\n\n**Output:** \\[1,2,2,3,4]\n\n**Explanation:**\n\n**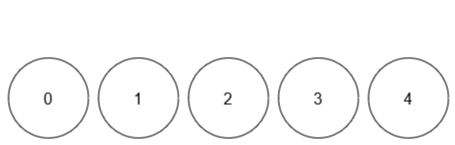**\n\n* After query 0, ball 0 has color 1\\.\n* After query 1, ball 0 has color 1, and ball 1 has color 2\\.\n* After query 2, ball 0 has color 1, and balls 1 and 2 have color 2\\.\n* After query 3, ball 0 has color 1, balls 1 and 2 have color 2, and ball 3 has color 4\\.\n* After query 4, ball 0 has color 1, balls 1 and 2 have color 2, ball 3 has color 4, and ball 4 has color 5\\.\n\n**Constraints:**\n\n* `1 <= limit <= 109`\n* `1 <= n == queries.length <= 105`\n* `queries[i].length == 2`\n* `0 <= queries[i][0] <= limit`\n* `1 <= queries[i][1] <= 109`", "templates": {"cpp": "class Solution {\npublic:\n vector<int> queryResults(int limit, vector<vector<int>>& queries) {\n \n }\n};", "java": "class Solution {\n public int[] queryResults(int limit, int[][] queries) {\n \n }\n}", "python": "class Solution:\n def queryResults(self, limit: int, queries: List[List[int]]) -> List[int]:\n "}, "hints": "1\\. Use two HashMaps to maintain the color of each ball and the set of balls with each color.", "contains_png": false, "png_links": []}
{"number": 3161, "title": "Block Placement Queries", "link": "https://leetcode.com/problems/block-placement-queries/", "difficulty": "hard", "statement": "There exists an infinite number line, with its origin at 0 and extending towards the **positive** x\\-axis.\n\nYou are given a 2D array `queries`, which contains two types of queries:\n\n1. For a query of type 1, `queries[i] = [1, x]`. Build an obstacle at distance `x` from the origin. It is guaranteed that there is **no** obstacle at distance `x` when the query is asked.\n2. For a query of type 2, `queries[i] = [2, x, sz]`. Check if it is possible to place a block of size `sz` *anywhere* in the range `[0, x]` on the line, such that the block **entirely** lies in the range `[0, x]`. A block **cannot** be placed if it intersects with any obstacle, but it may touch it. Note that you do **not** actually place the block. Queries are separate.\n\nReturn a boolean array `results`, where `results[i]` is `true` if you can place the block specified in the `ith` query of type 2, and `false` otherwise.\n\n**Example 1:**\n\n**Input:** queries \\= \\[\\[1,2],\\[2,3,3],\\[2,3,1],\\[2,2,2]]\n\n**Output:** \\[false,true,true]\n\n**Explanation:**\n\n**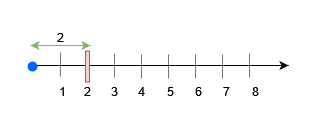**\n\nFor query 0, place an obstacle at `x = 2`. A block of size at most 2 can be placed before `x = 3`.\n\n**Example 2:**\n\n**Input:** queries \\= \\[\\[1,7],\\[2,7,6],\\[1,2],\\[2,7,5],\\[2,7,6]]\n\n**Output:** \\[true,true,false]\n\n**Explanation:**\n\n**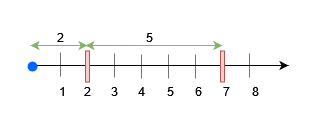**\n\n* Place an obstacle at `x = 7` for query 0\\. A block of size at most 7 can be placed before `x = 7`.\n* Place an obstacle at `x = 2` for query 2\\. Now, a block of size at most 5 can be placed before `x = 7`, and a block of size at most 2 before `x = 2`.\n\n**Constraints:**\n\n* `1 <= queries.length <= 15 * 104`\n* `2 <= queries[i].length <= 3`\n* `1 <= queries[i][0] <= 2`\n* `1 <= x, sz <= min(5 * 104, 3 * queries.length)`\n* The input is generated such that for queries of type 1, no obstacle exists at distance `x` when the query is asked.\n* The input is generated such that there is at least one query of type 2\\.", "templates": {"cpp": "class Solution {\npublic:\n vector<bool> getResults(vector<vector<int>>& queries) {\n \n }\n};", "java": "class Solution {\n public List<Boolean> getResults(int[][] queries) {\n \n }\n}", "python": "class Solution:\n def getResults(self, queries: List[List[int]]) -> List[bool]:\n "}, "hints": "1\\. Let `d[x]` be the distance of the next obstacle after `x`.\n2\\. For each query of type 2, we just need to check if `max(d[0], d[1], d[2], \u2026d[x - sz]) > sz`.\n3\\. Use segment tree to maintain `d[x]`.", "contains_png": true, "png_links": ["https://assets.leetcode.com/uploads/2024/04/22/example0block.png", "https://assets.leetcode.com/uploads/2024/04/22/example1block.png"]}
{"number": 3162, "title": "Find the Number of Good Pairs I", "link": "https://leetcode.com/problems/find-the-number-of-good-pairs-i/", "difficulty": "easy", "statement": "You are given 2 integer arrays `nums1` and `nums2` of lengths `n` and `m` respectively. You are also given a **positive** integer `k`.\n\nA pair `(i, j)` is called **good** if `nums1[i]` is divisible by `nums2[j] * k` (`0 <= i <= n - 1`, `0 <= j <= m - 1`).\n\nReturn the total number of **good** pairs.\n\n**Example 1:**\n\n**Input:** nums1 \\= \\[1,3,4], nums2 \\= \\[1,3,4], k \\= 1\n\n**Output:** 5\n\n**Explanation:**\n\nThe 5 good pairs are `(0, 0)`, `(1, 0)`, `(1, 1)`, `(2, 0)`, and `(2, 2)`.\n**Example 2:**\n\n**Input:** nums1 \\= \\[1,2,4,12], nums2 \\= \\[2,4], k \\= 3\n\n**Output:** 2\n\n**Explanation:**\n\nThe 2 good pairs are `(3, 0)` and `(3, 1)`.\n\n**Constraints:**\n\n* `1 <= n, m <= 50`\n* `1 <= nums1[i], nums2[j] <= 50`\n* `1 <= k <= 50`", "templates": {"cpp": "class Solution {\npublic:\n int numberOfPairs(vector<int>& nums1, vector<int>& nums2, int k) {\n \n }\n};", "java": "class Solution {\n public int numberOfPairs(int[] nums1, int[] nums2, int k) {\n \n }\n}", "python": "class Solution:\n def numberOfPairs(self, nums1: List[int], nums2: List[int], k: int) -> int:\n "}, "hints": "1\\. The constraints are small. Check all pairs.", "contains_png": false, "png_links": []}
{"number": 3163, "title": "String Compression III", "link": "https://leetcode.com/problems/string-compression-iii/", "difficulty": "medium", "statement": "Given a string `word`, compress it using the following algorithm:\n\n* Begin with an empty string `comp`. While `word` is **not** empty, use the following operation:\n\t+ Remove a maximum length prefix of `word` made of a *single character* `c` repeating **at most** 9 times.\n\t+ Append the length of the prefix followed by `c` to `comp`.\n\nReturn the string `comp`.\n\n**Example 1:**\n\n**Input:** word \\= \"abcde\"\n\n**Output:** \"1a1b1c1d1e\"\n\n**Explanation:**\n\nInitially, `comp = \"\"`. Apply the operation 5 times, choosing `\"a\"`, `\"b\"`, `\"c\"`, `\"d\"`, and `\"e\"` as the prefix in each operation.\n\nFor each prefix, append `\"1\"` followed by the character to `comp`.\n\n**Example 2:**\n\n**Input:** word \\= \"aaaaaaaaaaaaaabb\"\n\n**Output:** \"9a5a2b\"\n\n**Explanation:**\n\nInitially, `comp = \"\"`. Apply the operation 3 times, choosing `\"aaaaaaaaa\"`, `\"aaaaa\"`, and `\"bb\"` as the prefix in each operation.\n\n* For prefix `\"aaaaaaaaa\"`, append `\"9\"` followed by `\"a\"` to `comp`.\n* For prefix `\"aaaaa\"`, append `\"5\"` followed by `\"a\"` to `comp`.\n* For prefix `\"bb\"`, append `\"2\"` followed by `\"b\"` to `comp`.\n\n**Constraints:**\n\n* `1 <= word.length <= 2 * 105`\n* `word` consists only of lowercase English letters.", "templates": {"cpp": "class Solution {\npublic:\n string compressedString(string word) {\n \n }\n};", "java": "class Solution {\n public String compressedString(String word) {\n \n }\n}", "python": "class Solution:\n def compressedString(self, word: str) -> str:\n "}, "hints": "1\\. Each time, just cut the same character in prefix up to at max 9 times. It\u2019s always better to cut a bigger prefix.", "contains_png": false, "png_links": []}
{"number": 3164, "title": "Find the Number of Good Pairs II", "link": "https://leetcode.com/problems/find-the-number-of-good-pairs-ii/", "difficulty": "medium", "statement": "You are given 2 integer arrays `nums1` and `nums2` of lengths `n` and `m` respectively. You are also given a **positive** integer `k`.\n\nA pair `(i, j)` is called **good** if `nums1[i]` is divisible by `nums2[j] * k` (`0 <= i <= n - 1`, `0 <= j <= m - 1`).\n\nReturn the total number of **good** pairs.\n\n**Example 1:**\n\n**Input:** nums1 \\= \\[1,3,4], nums2 \\= \\[1,3,4], k \\= 1\n\n**Output:** 5\n\n**Explanation:**\n\nThe 5 good pairs are `(0, 0)`, `(1, 0)`, `(1, 1)`, `(2, 0)`, and `(2, 2)`.\n**Example 2:**\n\n**Input:** nums1 \\= \\[1,2,4,12], nums2 \\= \\[2,4], k \\= 3\n\n**Output:** 2\n\n**Explanation:**\n\nThe 2 good pairs are `(3, 0)` and `(3, 1)`.\n\n**Constraints:**\n\n* `1 <= n, m <= 105`\n* `1 <= nums1[i], nums2[j] <= 106`\n* `1 <= k <= 103`", "templates": {"cpp": "class Solution {\npublic:\n long long numberOfPairs(vector<int>& nums1, vector<int>& nums2, int k) {\n \n }\n};", "java": "class Solution {\n public long numberOfPairs(int[] nums1, int[] nums2, int k) {\n \n }\n}", "python": "class Solution:\n def numberOfPairs(self, nums1: List[int], nums2: List[int], k: int) -> int:\n "}, "hints": "1\\. Let `f[v]` be the number of occurrences of `v/k` in nums2\\.\n2\\. For each value `v` in nums1, enumerating all its factors `d` (in `sqrt(v)` time) and sum up all the `f[d]` to get the final answer.\n3\\. It is also possible to improve the complexity from `len(nums1) * sqrt(v)` to `len(nums1) * log(v)` \\- How?", "contains_png": false, "png_links": []}
{"number": 3165, "title": "Maximum Sum of Subsequence With Non-adjacent Elements", "link": "https://leetcode.com/problems/maximum-sum-of-subsequence-with-non-adjacent-elements/", "difficulty": "hard", "statement": "You are given an array `nums` consisting of integers. You are also given a 2D array `queries`, where `queries[i] = [posi, xi]`.\n\nFor query `i`, we first set `nums[posi]` equal to `xi`, then we calculate the answer to query `i` which is the **maximum** sum of a subsequence of `nums` where **no two adjacent elements are selected**.\n\nReturn the *sum* of the answers to all queries.\n\nSince the final answer may be very large, return it **modulo** `109 + 7`.\n\nA **subsequence** is an array that can be derived from another array by deleting some or no elements without changing the order of the remaining elements.\n\n**Example 1:**\n\n**Input:** nums \\= \\[3,5,9], queries \\= \\[\\[1,\\-2],\\[0,\\-3]]\n\n**Output:** 21\n\n**Explanation:** \n\nAfter the 1st query, `nums = [3,-2,9]` and the maximum sum of a subsequence with non\\-adjacent elements is `3 + 9 = 12`. \n\nAfter the 2nd query, `nums = [-3,-2,9]` and the maximum sum of a subsequence with non\\-adjacent elements is 9\\.\n\n**Example 2:**\n\n**Input:** nums \\= \\[0,\\-1], queries \\= \\[\\[0,\\-5]]\n\n**Output:** 0\n\n**Explanation:** \n\nAfter the 1st query, `nums = [-5,-1]` and the maximum sum of a subsequence with non\\-adjacent elements is 0 (choosing an empty subsequence).\n\n**Constraints:**\n\n* `1 <= nums.length <= 5 * 104`\n* `-105 <= nums[i] <= 105`\n* `1 <= queries.length <= 5 * 104`\n* `queries[i] == [posi, xi]`\n* `0 <= posi <= nums.length - 1`\n* `-105 <= xi <= 105`", "templates": {"cpp": "class Solution {\npublic:\n int maximumSumSubsequence(vector<int>& nums, vector<vector<int>>& queries) {\n \n }\n};", "java": "class Solution {\n public int maximumSumSubsequence(int[] nums, int[][] queries) {\n \n }\n}", "python": "class Solution:\n def maximumSumSubsequence(self, nums: List[int], queries: List[List[int]]) -> int:\n "}, "hints": "1\\. Can you solve each query in `O(nums.length)` with dynamic programming?\n2\\. In order to optimize, we will use segment tree where each node contains the maximum value of (front element has been chosen or not, back element has been chosen or not).", "contains_png": false, "png_links": []}
{"number": 3168, "title": "Minimum Number of Chairs in a Waiting Room", "link": "https://leetcode.com/problems/minimum-number-of-chairs-in-a-waiting-room/", "difficulty": "easy", "statement": "You are given a string `s`. Simulate events at each second `i`:\n\n* If `s[i] == 'E'`, a person enters the waiting room and takes one of the chairs in it.\n* If `s[i] == 'L'`, a person leaves the waiting room, freeing up a chair.\n\nReturn the **minimum** number of chairs needed so that a chair is available for every person who enters the waiting room given that it is initially **empty**.\n\n**Example 1:**\n\n**Input:** s \\= \"EEEEEEE\"\n\n**Output:** 7\n\n**Explanation:**\n\nAfter each second, a person enters the waiting room and no person leaves it. Therefore, a minimum of 7 chairs is needed.\n\n**Example 2:**\n\n**Input:** s \\= \"ELELEEL\"\n\n**Output:** 2\n\n**Explanation:**\n\nLet's consider that there are 2 chairs in the waiting room. The table below shows the state of the waiting room at each second.\n\n| Second | Event | People in the Waiting Room | Available Chairs |\n| --- | --- | --- | --- |\n| 0 | Enter | 1 | 1 |\n| 1 | Leave | 0 | 2 |\n| 2 | Enter | 1 | 1 |\n| 3 | Leave | 0 | 2 |\n| 4 | Enter | 1 | 1 |\n| 5 | Enter | 2 | 0 |\n| 6 | Leave | 1 | 1 |\n\n**Example 3:**\n\n**Input:** s \\= \"ELEELEELLL\"\n\n**Output:** 3\n\n**Explanation:**\n\nLet's consider that there are 3 chairs in the waiting room. The table below shows the state of the waiting room at each second.\n\n| Second | Event | People in the Waiting Room | Available Chairs |\n| --- | --- | --- | --- |\n| 0 | Enter | 1 | 2 |\n| 1 | Leave | 0 | 3 |\n| 2 | Enter | 1 | 2 |\n| 3 | Enter | 2 | 1 |\n| 4 | Leave | 1 | 2 |\n| 5 | Enter | 2 | 1 |\n| 6 | Enter | 3 | 0 |\n| 7 | Leave | 2 | 1 |\n| 8 | Leave | 1 | 2 |\n| 9 | Leave | 0 | 3 |\n\n**Constraints:**\n\n* `1 <= s.length <= 50`\n* `s` consists only of the letters `'E'` and `'L'`.\n* `s` represents a valid sequence of entries and exits.", "templates": {"cpp": "class Solution {\npublic:\n int minimumChairs(string s) {\n \n }\n};", "java": "class Solution {\n public int minimumChairs(String s) {\n \n }\n}", "python": "class Solution:\n def minimumChairs(self, s: str) -> int:\n "}, "hints": "1\\. Iterate from left to right over the string and keep track of the number of people in the waiting room using a variable that you will increment on every occurrence of \u2018E\u2019 and decrement on every occurrence of \u2018L\u2019.\n2\\. The answer is the maximum number of people in the waiting room at any instance.", "contains_png": false, "png_links": []}
{"number": 3169, "title": "Count Days Without Meetings", "link": "https://leetcode.com/problems/count-days-without-meetings/", "difficulty": "medium", "statement": "You are given a positive integer `days` representing the total number of days an employee is available for work (starting from day 1\\). You are also given a 2D array `meetings` of size `n` where, `meetings[i] = [start_i, end_i]` represents the starting and ending days of meeting `i` (inclusive).\n\nReturn the count of days when the employee is available for work but no meetings are scheduled.\n\n**Note:** The meetings may overlap.\n\n**Example 1:**\n\n**Input:** days \\= 10, meetings \\= \\[\\[5,7],\\[1,3],\\[9,10]]\n\n**Output:** 2\n\n**Explanation:**\n\nThere is no meeting scheduled on the 4th and 8th days.\n\n**Example 2:**\n\n**Input:** days \\= 5, meetings \\= \\[\\[2,4],\\[1,3]]\n\n**Output:** 1\n\n**Explanation:**\n\nThere is no meeting scheduled on the 5th day.\n\n**Example 3:**\n\n**Input:** days \\= 6, meetings \\= \\[\\[1,6]]\n\n**Output:** 0\n\n**Explanation:**\n\nMeetings are scheduled for all working days.\n\n**Constraints:**\n\n* `1 <= days <= 109`\n* `1 <= meetings.length <= 105`\n* `meetings[i].length == 2`\n* `1 <= meetings[i][0] <= meetings[i][1] <= days`", "templates": {"cpp": "class Solution {\npublic:\n int countDays(int days, vector<vector<int>>& meetings) {\n \n }\n};", "java": "class Solution {\n public int countDays(int days, int[][] meetings) {\n \n }\n}", "python": "class Solution:\n def countDays(self, days: int, meetings: List[List[int]]) -> int:\n "}, "hints": "1\\. Merge the overlapping meetings and sort the new meetings timings.\n2\\. Return the sum of difference between the end time of a meeting and the start time of the next meeting for all adjacent pairs.", "contains_png": false, "png_links": []}
{"number": 3170, "title": "Lexicographically Minimum String After Removing Stars", "link": "https://leetcode.com/problems/lexicographically-minimum-string-after-removing-stars/", "difficulty": "medium", "statement": "You are given a string `s`. It may contain any number of `'*'` characters. Your task is to remove all `'*'` characters.\n\nWhile there is a `'*'`, do the following operation:\n\n* Delete the leftmost `'*'` and the **smallest** non\\-`'*'` character to its *left*. If there are several smallest characters, you can delete any of them.\n\nReturn the lexicographically smallest resulting string after removing all `'*'` characters.\n\n**Example 1:**\n\n**Input:** s \\= \"aaba\\*\"\n\n**Output:** \"aab\"\n\n**Explanation:**\n\nWe should delete one of the `'a'` characters with `'*'`. If we choose `s[3]`, `s` becomes the lexicographically smallest.\n\n**Example 2:**\n\n**Input:** s \\= \"abc\"\n\n**Output:** \"abc\"\n\n**Explanation:**\n\nThere is no `'*'` in the string.\n\n**Constraints:**\n\n* `1 <= s.length <= 105`\n* `s` consists only of lowercase English letters and `'*'`.\n* The input is generated such that it is possible to delete all `'*'` characters.", "templates": {"cpp": "class Solution {\npublic:\n string clearStars(string s) {\n \n }\n};", "java": "class Solution {\n public String clearStars(String s) {\n \n }\n}", "python": "class Solution:\n def clearStars(self, s: str) -> str:\n "}, "hints": null, "contains_png": false, "png_links": []}
{"number": 3171, "title": "Find Subarray With Bitwise OR Closest to K", "link": "https://leetcode.com/problems/find-subarray-with-bitwise-or-closest-to-k/", "difficulty": "hard", "statement": "You are given an array `nums` and an integer `k`. You need to find a subarray of `nums` such that the **absolute difference** between `k` and the bitwise `OR` of the subarray elements is as **small** as possible. In other words, select a subarray `nums[l..r]` such that `|k - (nums[l] OR nums[l + 1] ... OR nums[r])|` is minimum.\n\nReturn the **minimum** possible value of the absolute difference.\n\nA **subarray** is a contiguous **non\\-empty** sequence of elements within an array.\n\n**Example 1:**\n\n**Input:** nums \\= \\[1,2,4,5], k \\= 3\n\n**Output:** 0\n\n**Explanation:**\n\nThe subarray `nums[0..1]` has `OR` value 3, which gives the minimum absolute difference `|3 - 3| = 0`.\n\n**Example 2:**\n\n**Input:** nums \\= \\[1,3,1,3], k \\= 2\n\n**Output:** 1\n\n**Explanation:**\n\nThe subarray `nums[1..1]` has `OR` value 3, which gives the minimum absolute difference `|3 - 2| = 1`.\n\n**Example 3:**\n\n**Input:** nums \\= \\[1], k \\= 10\n\n**Output:** 9\n\n**Explanation:**\n\nThere is a single subarray with `OR` value 1, which gives the minimum absolute difference `|10 - 1| = 9`.\n\n**Constraints:**\n\n* `1 <= nums.length <= 105`\n* `1 <= nums[i] <= 109`\n* `1 <= k <= 109`", "templates": {"cpp": "class Solution {\npublic:\n int minimumDifference(vector<int>& nums, int k) {\n \n }\n};", "java": "class Solution {\n public int minimumDifference(int[] nums, int k) {\n \n }\n}", "python": "class Solution:\n def minimumDifference(self, nums: List[int], k: int) -> int:\n "}, "hints": "1\\. Let `dp[i]` be the set of all the bitwise `OR` of all the subarrays ending at index `i`.\n2\\. We start from `nums[i]`, taking the bitwise `OR` result by including elements one by one from `i` towards left. Notice that only unset bits can become set on adding an element, and set bits never become unset again.\n3\\. Hence `dp[i]` can contain at most 30 elements.", "contains_png": false, "png_links": []}
{"number": 3174, "title": "Clear Digits", "link": "https://leetcode.com/problems/clear-digits/", "difficulty": "easy", "statement": "You are given a string `s`.\n\nYour task is to remove **all** digits by doing this operation repeatedly:\n\n* Delete the *first* digit and the **closest** **non\\-digit** character to its *left*.\n\nReturn the resulting string after removing all digits.\n\n**Example 1:**\n\n**Input:** s \\= \"abc\"\n\n**Output:** \"abc\"\n\n**Explanation:**\n\nThere is no digit in the string.\n\n**Example 2:**\n\n**Input:** s \\= \"cb34\"\n\n**Output:** \"\"\n\n**Explanation:**\n\nFirst, we apply the operation on `s[2]`, and `s` becomes `\"c4\"`.\n\nThen we apply the operation on `s[1]`, and `s` becomes `\"\"`.\n\n**Constraints:**\n\n* `1 <= s.length <= 100`\n* `s` consists only of lowercase English letters and digits.\n* The input is generated such that it is possible to delete all digits.", "templates": {"cpp": "class Solution {\npublic:\n string clearDigits(string s) {\n \n }\n};", "java": "class Solution {\n public String clearDigits(String s) {\n \n }\n}", "python": "class Solution:\n def clearDigits(self, s: str) -> str:\n "}, "hints": "1\\. Process string `s` from left to right, if `s[i]` is a digit, mark the nearest unmarked non\\-digit index to its left.\n2\\. Delete all digits and all marked characters.", "contains_png": false, "png_links": []}
{"number": 3175, "title": "Find The First Player to win K Games in a Row", "link": "https://leetcode.com/problems/find-the-first-player-to-win-k-games-in-a-row/", "difficulty": "medium", "statement": "A competition consists of `n` players numbered from `0` to `n - 1`.\n\nYou are given an integer array `skills` of size `n` and a **positive** integer `k`, where `skills[i]` is the skill level of player `i`. All integers in `skills` are **unique**.\n\nAll players are standing in a queue in order from player `0` to player `n - 1`.\n\nThe competition process is as follows:\n\n* The first two players in the queue play a game, and the player with the **higher** skill level wins.\n* After the game, the winner stays at the beginning of the queue, and the loser goes to the end of it.\n\nThe winner of the competition is the **first** player who wins `k` games **in a row**.\n\nReturn the initial index of the *winning* player.\n\n**Example 1:**\n\n**Input:** skills \\= \\[4,2,6,3,9], k \\= 2\n\n**Output:** 2\n\n**Explanation:**\n\nInitially, the queue of players is `[0,1,2,3,4]`. The following process happens:\n\n* Players 0 and 1 play a game, since the skill of player 0 is higher than that of player 1, player 0 wins. The resulting queue is `[0,2,3,4,1]`.\n* Players 0 and 2 play a game, since the skill of player 2 is higher than that of player 0, player 2 wins. The resulting queue is `[2,3,4,1,0]`.\n* Players 2 and 3 play a game, since the skill of player 2 is higher than that of player 3, player 2 wins. The resulting queue is `[2,4,1,0,3]`.\n\nPlayer 2 won `k = 2` games in a row, so the winner is player 2\\.\n\n**Example 2:**\n\n**Input:** skills \\= \\[2,5,4], k \\= 3\n\n**Output:** 1\n\n**Explanation:**\n\nInitially, the queue of players is `[0,1,2]`. The following process happens:\n\n* Players 0 and 1 play a game, since the skill of player 1 is higher than that of player 0, player 1 wins. The resulting queue is `[1,2,0]`.\n* Players 1 and 2 play a game, since the skill of player 1 is higher than that of player 2, player 1 wins. The resulting queue is `[1,0,2]`.\n* Players 1 and 0 play a game, since the skill of player 1 is higher than that of player 0, player 1 wins. The resulting queue is `[1,2,0]`.\n\nPlayer 1 won `k = 3` games in a row, so the winner is player 1\\.\n\n**Constraints:**\n\n* `n == skills.length`\n* `2 <= n <= 105`\n* `1 <= k <= 109`\n* `1 <= skills[i] <= 106`\n* All integers in `skills` are unique.", "templates": {"cpp": "class Solution {\npublic:\n int findWinningPlayer(vector<int>& skills, int k) {\n \n }\n};", "java": "class Solution {\n public int findWinningPlayer(int[] skills, int k) {\n \n }\n}", "python": "class Solution:\n def findWinningPlayer(self, skills: List[int], k: int) -> int:\n "}, "hints": "1\\. Suppose that `k \u2265 n`, there is exactly one player who can win `k` games in a row. Who is it?\n2\\. In case `k < n`, you can simulate the competition process described.", "contains_png": false, "png_links": []}
{"number": 3176, "title": "Find the Maximum Length of a Good Subsequence I", "link": "https://leetcode.com/problems/find-the-maximum-length-of-a-good-subsequence-i/", "difficulty": "medium", "statement": "You are given an integer array `nums` and a **non\\-negative** integer `k`. A sequence of integers `seq` is called **good** if there are **at most** `k` indices `i` in the range `[0, seq.length - 2]` such that `seq[i] != seq[i + 1]`.\n\nReturn the **maximum** possible length of a **good** subsequence of `nums`.\n\n**Example 1:**\n\n**Input:** nums \\= \\[1,2,1,1,3], k \\= 2\n\n**Output:** 4\n\n**Explanation:**\n\nThe maximum length subsequence is `[1,2,1,1,3]`.\n\n**Example 2:**\n\n**Input:** nums \\= \\[1,2,3,4,5,1], k \\= 0\n\n**Output:** 2\n\n**Explanation:**\n\nThe maximum length subsequence is `[1,2,3,4,5,1]`.\n\n**Constraints:**\n\n* `1 <= nums.length <= 500`\n* `1 <= nums[i] <= 109`\n* `0 <= k <= min(nums.length, 25)`", "templates": {"cpp": "class Solution {\npublic:\n int maximumLength(vector<int>& nums, int k) {\n \n }\n};", "java": "class Solution {\n public int maximumLength(int[] nums, int k) {\n \n }\n}", "python": "class Solution:\n def maximumLength(self, nums: List[int], k: int) -> int:\n "}, "hints": "1\\. The absolute values in `nums` don\u2019t really matter. So we can remap the set of values to the range `[0, n - 1]`.\n2\\. Let `dp[i][j]` be the length of the longest subsequence till index `j` with at most `i` positions such that `seq[i] != seq[i + 1]`.\n3\\. For each value `x` from left to right, update `dp[i][x] = max(dp[i][x] + 1, dp[i - 1][y] + 1)`, where `y != x`.", "contains_png": false, "png_links": []}
{"number": 3177, "title": "Find the Maximum Length of a Good Subsequence II", "link": "https://leetcode.com/problems/find-the-maximum-length-of-a-good-subsequence-ii/", "difficulty": "hard", "statement": "You are given an integer array `nums` and a **non\\-negative** integer `k`. A sequence of integers `seq` is called **good** if there are **at most** `k` indices `i` in the range `[0, seq.length - 2]` such that `seq[i] != seq[i + 1]`.\n\nReturn the **maximum** possible length of a **good** subsequence of `nums`.\n\n**Example 1:**\n\n**Input:** nums \\= \\[1,2,1,1,3], k \\= 2\n\n**Output:** 4\n\n**Explanation:**\n\nThe maximum length subsequence is `[1,2,1,1,3]`.\n\n**Example 2:**\n\n**Input:** nums \\= \\[1,2,3,4,5,1], k \\= 0\n\n**Output:** 2\n\n**Explanation:**\n\nThe maximum length subsequence is `[1,2,3,4,5,1]`.\n\n**Constraints:**\n\n* `1 <= nums.length <= 5 * 103`\n* `1 <= nums[i] <= 109`\n* `0 <= k <= min(50, nums.length)`", "templates": {"cpp": "class Solution {\npublic:\n int maximumLength(vector<int>& nums, int k) {\n \n }\n};", "java": "class Solution {\n public int maximumLength(int[] nums, int k) {\n \n }\n}", "python": "class Solution:\n def maximumLength(self, nums: List[int], k: int) -> int:\n "}, "hints": "1\\. The absolute values in `nums` don\u2019t really matter. So we can remap the set of values to the range `[0, n - 1]`.\n2\\. Let `dp[i][j]` be the length of the longest subsequence till index `j` with at most `i` positions such that `seq[i] != seq[i + 1]`.\n3\\. For each value `x` from left to right, update `dp[i][x] = max(dp[i][x] + 1, dp[i - 1][y] + 1)`, where `y != x`.", "contains_png": false, "png_links": []}
{"number": 3178, "title": "Find the Child Who Has the Ball After K Seconds", "link": "https://leetcode.com/problems/find-the-child-who-has-the-ball-after-k-seconds/", "difficulty": "easy", "statement": "You are given two **positive** integers `n` and `k`. There are `n` children numbered from `0` to `n - 1` standing in a queue *in order* from left to right.\n\nInitially, child 0 holds a ball and the direction of passing the ball is towards the right direction. After each second, the child holding the ball passes it to the child next to them. Once the ball reaches **either** end of the line, i.e. child 0 or child `n - 1`, the direction of passing is **reversed**.\n\nReturn the number of the child who receives the ball after `k` seconds.\n\n**Example 1:**\n\n**Input:** n \\= 3, k \\= 5\n\n**Output:** 1\n\n**Explanation:**\n\n| Time elapsed | Children |\n| --- | --- |\n| `0` | `[0, 1, 2]` |\n| `1` | `[0, 1, 2]` |\n| `2` | `[0, 1, 2]` |\n| `3` | `[0, 1, 2]` |\n| `4` | `[0, 1, 2]` |\n| `5` | `[0, 1, 2]` |\n\n**Example 2:**\n\n**Input:** n \\= 5, k \\= 6\n\n**Output:** 2\n\n**Explanation:**\n\n| Time elapsed | Children |\n| --- | --- |\n| `0` | `[0, 1, 2, 3, 4]` |\n| `1` | `[0, 1, 2, 3, 4]` |\n| `2` | `[0, 1, 2, 3, 4]` |\n| `3` | `[0, 1, 2, 3, 4]` |\n| `4` | `[0, 1, 2, 3, 4]` |\n| `5` | `[0, 1, 2, 3, 4]` |\n| `6` | `[0, 1, 2, 3, 4]` |\n\n**Example 3:**\n\n**Input:** n \\= 4, k \\= 2\n\n**Output:** 2\n\n**Explanation:**\n\n| Time elapsed | Children |\n| --- | --- |\n| `0` | `[0, 1, 2, 3]` |\n| `1` | `[0, 1, 2, 3]` |\n| `2` | `[0, 1, 2, 3]` |\n\n**Constraints:**\n\n* `2 <= n <= 50`\n* `1 <= k <= 50`\n\n**Note:** This question is the same as [2582: Pass the Pillow.](https://leetcode.com/problems/pass-the-pillow/description/)", "templates": {"cpp": "class Solution {\npublic:\n int numberOfChild(int n, int k) {\n \n }\n};", "java": "class Solution {\n public int numberOfChild(int n, int k) {\n \n }\n}", "python": "class Solution:\n def numberOfChild(self, n: int, k: int) -> int:\n "}, "hints": "1\\. The ball will go back to child 0 after `2 * (n - 1)` seconds and everything is the same as time 0\\.\n2\\. So the answer for `k` is the same as the answer for `k % (2 * (n - 1))`.", "contains_png": false, "png_links": []}
{"number": 3179, "title": "Find the N-th Value After K Seconds", "link": "https://leetcode.com/problems/find-the-n-th-value-after-k-seconds/", "difficulty": "medium", "statement": "You are given two integers `n` and `k`.\n\nInitially, you start with an array `a` of `n` integers where `a[i] = 1` for all `0 <= i <= n - 1`. After each second, you simultaneously update each element to be the sum of all its preceding elements plus the element itself. For example, after one second, `a[0]` remains the same, `a[1]` becomes `a[0] + a[1]`, `a[2]` becomes `a[0] + a[1] + a[2]`, and so on.\n\nReturn the **value** of `a[n - 1]` after `k` seconds.\n\nSince the answer may be very large, return it **modulo** `109 + 7`.\n\n**Example 1:**\n\n**Input:** n \\= 4, k \\= 5\n\n**Output:** 56\n\n**Explanation:**\n\n| Second | State After |\n| --- | --- |\n| 0 | \\[1,1,1,1] |\n| 1 | \\[1,2,3,4] |\n| 2 | \\[1,3,6,10] |\n| 3 | \\[1,4,10,20] |\n| 4 | \\[1,5,15,35] |\n| 5 | \\[1,6,21,56] |\n\n**Example 2:**\n\n**Input:** n \\= 5, k \\= 3\n\n**Output:** 35\n\n**Explanation:**\n\n| Second | State After |\n| --- | --- |\n| 0 | \\[1,1,1,1,1] |\n| 1 | \\[1,2,3,4,5] |\n| 2 | \\[1,3,6,10,15] |\n| 3 | \\[1,4,10,20,35] |\n\n**Constraints:**\n\n* `1 <= n, k <= 1000`", "templates": {"cpp": "class Solution {\npublic:\n int valueAfterKSeconds(int n, int k) {\n \n }\n};", "java": "class Solution {\n public int valueAfterKSeconds(int n, int k) {\n \n }\n}", "python": "class Solution:\n def valueAfterKSeconds(self, n: int, k: int) -> int:\n "}, "hints": "1\\. Calculate the prefix sum array of `nums`, `k` times.", "contains_png": false, "png_links": []}
{"number": 3180, "title": "Maximum Total Reward Using Operations I", "link": "https://leetcode.com/problems/maximum-total-reward-using-operations-i/", "difficulty": "medium", "statement": "You are given an integer array `rewardValues` of length `n`, representing the values of rewards.\n\nInitially, your total reward `x` is 0, and all indices are **unmarked**. You are allowed to perform the following operation **any** number of times:\n\n* Choose an **unmarked** index `i` from the range `[0, n - 1]`.\n* If `rewardValues[i]` is **greater** than your current total reward `x`, then add `rewardValues[i]` to `x` (i.e., `x = x + rewardValues[i]`), and **mark** the index `i`.\n\nReturn an integer denoting the **maximum** *total reward* you can collect by performing the operations optimally.\n\n**Example 1:**\n\n**Input:** rewardValues \\= \\[1,1,3,3]\n\n**Output:** 4\n\n**Explanation:**\n\nDuring the operations, we can choose to mark the indices 0 and 2 in order, and the total reward will be 4, which is the maximum.\n\n**Example 2:**\n\n**Input:** rewardValues \\= \\[1,6,4,3,2]\n\n**Output:** 11\n\n**Explanation:**\n\nMark the indices 0, 2, and 1 in order. The total reward will then be 11, which is the maximum.\n\n**Constraints:**\n\n* `1 <= rewardValues.length <= 2000`\n* `1 <= rewardValues[i] <= 2000`", "templates": {"cpp": "class Solution {\npublic:\n int maxTotalReward(vector<int>& rewardValues) {\n \n }\n};", "java": "class Solution {\n public int maxTotalReward(int[] rewardValues) {\n \n }\n}", "python": "class Solution:\n def maxTotalReward(self, rewardValues: List[int]) -> int:\n "}, "hints": "1\\. Sort the rewards array first.\n2\\. If we decide to apply some rewards, it's always optimal to apply them in order.\n3\\. Let `dp[i][j]` (true/false) be the state after the first `i` rewards, indicating whether we can get exactly `j` points.\n4\\. The transition is given by: `dp[i][j] = dp[i - 1][j \u2212 rewardValues[i]]` if `j \u2212 rewardValues[i] < rewardValues[i]`.", "contains_png": false, "png_links": []}
{"number": 3181, "title": "Maximum Total Reward Using Operations II", "link": "https://leetcode.com/problems/maximum-total-reward-using-operations-ii/", "difficulty": "hard", "statement": "You are given an integer array `rewardValues` of length `n`, representing the values of rewards.\n\nInitially, your total reward `x` is 0, and all indices are **unmarked**. You are allowed to perform the following operation **any** number of times:\n\n* Choose an **unmarked** index `i` from the range `[0, n - 1]`.\n* If `rewardValues[i]` is **greater** than your current total reward `x`, then add `rewardValues[i]` to `x` (i.e., `x = x + rewardValues[i]`), and **mark** the index `i`.\n\nReturn an integer denoting the **maximum** *total reward* you can collect by performing the operations optimally.\n\n**Example 1:**\n\n**Input:** rewardValues \\= \\[1,1,3,3]\n\n**Output:** 4\n\n**Explanation:**\n\nDuring the operations, we can choose to mark the indices 0 and 2 in order, and the total reward will be 4, which is the maximum.\n\n**Example 2:**\n\n**Input:** rewardValues \\= \\[1,6,4,3,2]\n\n**Output:** 11\n\n**Explanation:**\n\nMark the indices 0, 2, and 1 in order. The total reward will then be 11, which is the maximum.\n\n**Constraints:**\n\n* `1 <= rewardValues.length <= 5 * 104`\n* `1 <= rewardValues[i] <= 5 * 104`", "templates": {"cpp": "class Solution {\npublic:\n int maxTotalReward(vector<int>& rewardValues) {\n \n }\n};", "java": "class Solution {\n public int maxTotalReward(int[] rewardValues) {\n \n }\n}", "python": "class Solution:\n def maxTotalReward(self, rewardValues: List[int]) -> int:\n "}, "hints": "1\\. Sort the rewards array first.\n2\\. If we decide to apply some rewards, it's always optimal to apply them in order.\n3\\. The transition is given by: `dp[i][j] = dp[i - 1][j \u2212 rewardValues[i]]` if `j \u2212 rewardValues[i] < rewardValues[i]`.\n4\\. Note that the dp array is a boolean array. We just need 1 bit per element, so we can use a bitset or something similar. We just need a \"stream\" of bits and apply bitwise operations to optimize the computations by a constant factor.", "contains_png": false, "png_links": []}
{"number": 3184, "title": "Count Pairs That Form a Complete Day I", "link": "https://leetcode.com/problems/count-pairs-that-form-a-complete-day-i/", "difficulty": "easy", "statement": "Given an integer array `hours` representing times in **hours**, return an integer denoting the number of pairs `i`, `j` where `i < j` and `hours[i] + hours[j]` forms a **complete day**.\n\nA **complete day** is defined as a time duration that is an **exact** **multiple** of 24 hours.\n\nFor example, 1 day is 24 hours, 2 days is 48 hours, 3 days is 72 hours, and so on.\n\n**Example 1:**\n\n**Input:** hours \\= \\[12,12,30,24,24]\n\n**Output:** 2\n\n**Explanation:**\n\nThe pairs of indices that form a complete day are `(0, 1)` and `(3, 4)`.\n\n**Example 2:**\n\n**Input:** hours \\= \\[72,48,24,3]\n\n**Output:** 3\n\n**Explanation:**\n\nThe pairs of indices that form a complete day are `(0, 1)`, `(0, 2)`, and `(1, 2)`.\n\n**Constraints:**\n\n* `1 <= hours.length <= 100`\n* `1 <= hours[i] <= 109`", "templates": {"cpp": "class Solution {\npublic:\n int countCompleteDayPairs(vector<int>& hours) {\n \n }\n};", "java": "class Solution {\n public int countCompleteDayPairs(int[] hours) {\n \n }\n}", "python": "class Solution:\n def countCompleteDayPairs(self, hours: List[int]) -> int:\n "}, "hints": "1\\. Brute force all pairs `(i, j)` and check if they form a valid complete day. It is considered a complete day if `(hours[i] + hours[j]) % 24 == 0`.", "contains_png": false, "png_links": []}
{"number": 3185, "title": "Count Pairs That Form a Complete Day II", "link": "https://leetcode.com/problems/count-pairs-that-form-a-complete-day-ii/", "difficulty": "medium", "statement": "Given an integer array `hours` representing times in **hours**, return an integer denoting the number of pairs `i`, `j` where `i < j` and `hours[i] + hours[j]` forms a **complete day**.\n\nA **complete day** is defined as a time duration that is an **exact** **multiple** of 24 hours.\n\nFor example, 1 day is 24 hours, 2 days is 48 hours, 3 days is 72 hours, and so on.\n\n**Example 1:**\n\n**Input:** hours \\= \\[12,12,30,24,24]\n\n**Output:** 2\n\n**Explanation:** The pairs of indices that form a complete day are `(0, 1)` and `(3, 4)`.\n\n**Example 2:**\n\n**Input:** hours \\= \\[72,48,24,3]\n\n**Output:** 3\n\n**Explanation:** The pairs of indices that form a complete day are `(0, 1)`, `(0, 2)`, and `(1, 2)`.\n\n**Constraints:**\n\n* `1 <= hours.length <= 5 * 105`\n* `1 <= hours[i] <= 109`", "templates": {"cpp": "class Solution {\npublic:\n long long countCompleteDayPairs(vector<int>& hours) {\n \n }\n};", "java": "class Solution {\n public long countCompleteDayPairs(int[] hours) {\n \n }\n}", "python": "class Solution:\n def countCompleteDayPairs(self, hours: List[int]) -> int:\n "}, "hints": "1\\. A pair `(i, j)` forms a valid complete day if `(hours[i] + hours[j]) % 24 == 0`.\n2\\. Using an array or a map, for each index `j` moving from left to right, increase the answer by the count of `(24 - hours[j]) % 24`, and then increase the count of `hours[j]`.", "contains_png": false, "png_links": []}
{"number": 3186, "title": "Maximum Total Damage With Spell Casting", "link": "https://leetcode.com/problems/maximum-total-damage-with-spell-casting/", "difficulty": "medium", "statement": "A magician has various spells.\n\nYou are given an array `power`, where each element represents the damage of a spell. Multiple spells can have the same damage value.\n\nIt is a known fact that if a magician decides to cast a spell with a damage of `power[i]`, they **cannot** cast any spell with a damage of `power[i] - 2`, `power[i] - 1`, `power[i] + 1`, or `power[i] + 2`.\n\nEach spell can be cast **only once**.\n\nReturn the **maximum** possible *total damage* that a magician can cast.\n\n**Example 1:**\n\n**Input:** power \\= \\[1,1,3,4]\n\n**Output:** 6\n\n**Explanation:**\n\nThe maximum possible damage of 6 is produced by casting spells 0, 1, 3 with damage 1, 1, 4\\.\n\n**Example 2:**\n\n**Input:** power \\= \\[7,1,6,6]\n\n**Output:** 13\n\n**Explanation:**\n\nThe maximum possible damage of 13 is produced by casting spells 1, 2, 3 with damage 1, 6, 6\\.\n\n**Constraints:**\n\n* `1 <= power.length <= 105`\n* `1 <= power[i] <= 109`", "templates": {"cpp": "class Solution {\npublic:\n long long maximumTotalDamage(vector<int>& power) {\n \n }\n};", "java": "class Solution {\n public long maximumTotalDamage(int[] power) {\n \n }\n}", "python": "class Solution:\n def maximumTotalDamage(self, power: List[int]) -> int:\n "}, "hints": "1\\. If we ever decide to use some spell with power `x`, then we will use all spells with power `x`.\n2\\. Think of dynamic programming.\n3\\. `dp[i][j]` represents the maximum damage considering up to the `i`\\-th unique spell and `j` represents the number of spells skipped (up to 3 as per constraints).", "contains_png": false, "png_links": []}
{"number": 3187, "title": "Peaks in Array", "link": "https://leetcode.com/problems/peaks-in-array/", "difficulty": "hard", "statement": "A **peak** in an array `arr` is an element that is **greater** than its previous and next element in `arr`.\n\nYou are given an integer array `nums` and a 2D integer array `queries`.\n\nYou have to process queries of two types:\n\n* `queries[i] = [1, li, ri]`, determine the count of **peak** elements in the subarray `nums[li..ri]`.\n* `queries[i] = [2, indexi, vali]`, change `nums[indexi]` to `vali`.\n\nReturn an array `answer` containing the results of the queries of the first type in order.\n\n**Notes:**\n\n* The **first** and the **last** element of an array or a subarray **cannot** be a peak.\n\n**Example 1:**\n\n**Input:** nums \\= \\[3,1,4,2,5], queries \\= \\[\\[2,3,4],\\[1,0,4]]\n\n**Output:** \\[0]\n\n**Explanation:**\n\nFirst query: We change `nums[3]` to 4 and `nums` becomes `[3,1,4,4,5]`.\n\nSecond query: The number of peaks in the `[3,1,4,4,5]` is 0\\.\n\n**Example 2:**\n\n**Input:** nums \\= \\[4,1,4,2,1,5], queries \\= \\[\\[2,2,4],\\[1,0,2],\\[1,0,4]]\n\n**Output:** \\[0,1]\n\n**Explanation:**\n\nFirst query: `nums[2]` should become 4, but it is already set to 4\\.\n\nSecond query: The number of peaks in the `[4,1,4]` is 0\\.\n\nThird query: The second 4 is a peak in the `[4,1,4,2,1]`.\n\n**Constraints:**\n\n* `3 <= nums.length <= 105`\n* `1 <= nums[i] <= 105`\n* `1 <= queries.length <= 105`\n* `queries[i][0] == 1` or `queries[i][0] == 2`\n* For all `i` that:\n\t+ `queries[i][0] == 1`: `0 <= queries[i][1] <= queries[i][2] <= nums.length - 1`\n\t+ `queries[i][0] == 2`: `0 <= queries[i][1] <= nums.length - 1`, `1 <= queries[i][2] <= 105`", "templates": {"cpp": "class Solution {\npublic:\n vector<int> countOfPeaks(vector<int>& nums, vector<vector<int>>& queries) {\n \n }\n};", "java": "class Solution {\n public List<Integer> countOfPeaks(int[] nums, int[][] queries) {\n \n }\n}", "python": "class Solution:\n def countOfPeaks(self, nums: List[int], queries: List[List[int]]) -> List[int]:\n "}, "hints": "1\\. Let `p[i]` be whether `nums[i]` is a peak in the original array. Namely `p[i] = nums[i] > nums[i - 1] && nums[i] > nums[i + 1]`.\n2\\. Updating `nums[i]`, only affects `p[i]`, `p[i - 1]` and `p[i + 1]`. We can recalculate the 3 values in constant time.\n3\\. The answer for `[li, ri]` is `p[li + 1] + p[li + 2] + \u2026 + p[ri - 1]` (note that `li` and `ri` are not included).\n4\\. Use some data structures (i.e. segment tree or binary indexed tree) to maintain the subarray sum efficiently.", "contains_png": false, "png_links": []}
{"number": 3190, "title": "Find Minimum Operations to Make All Elements Divisible by Three", "link": "https://leetcode.com/problems/find-minimum-operations-to-make-all-elements-divisible-by-three/", "difficulty": "easy", "statement": "You are given an integer array `nums`. In one operation, you can add or subtract 1 from **any** element of `nums`.\n\nReturn the **minimum** number of operations to make all elements of `nums` divisible by 3\\.\n\n**Example 1:**\n\n**Input:** nums \\= \\[1,2,3,4]\n\n**Output:** 3\n\n**Explanation:**\n\nAll array elements can be made divisible by 3 using 3 operations:\n\n* Subtract 1 from 1\\.\n* Add 1 to 2\\.\n* Subtract 1 from 4\\.\n\n**Example 2:**\n\n**Input:** nums \\= \\[3,6,9]\n\n**Output:** 0\n\n**Constraints:**\n\n* `1 <= nums.length <= 50`\n* `1 <= nums[i] <= 50`", "templates": {"cpp": "class Solution {\npublic:\n int minimumOperations(vector<int>& nums) {\n \n }\n};", "java": "class Solution {\n public int minimumOperations(int[] nums) {\n \n }\n}", "python": "class Solution:\n def minimumOperations(self, nums: List[int]) -> int:\n "}, "hints": "1\\. If `x % 3 != 0` we can always increment or decrement `x` such that we only need 1 operation.\n2\\. Add `min(nums[i] % 3, 3 - (num[i] % 3))` to the count of operations.", "contains_png": false, "png_links": []}
{"number": 3191, "title": "Minimum Operations to Make Binary Array Elements Equal to One I", "link": "https://leetcode.com/problems/minimum-operations-to-make-binary-array-elements-equal-to-one-i/", "difficulty": "medium", "statement": "You are given a binary array `nums`.\n\nYou can do the following operation on the array **any** number of times (possibly zero):\n\n* Choose **any** 3 **consecutive** elements from the array and **flip** **all** of them.\n\n**Flipping** an element means changing its value from 0 to 1, and from 1 to 0\\.\n\nReturn the **minimum** number of operations required to make all elements in `nums` equal to 1\\. If it is impossible, return \\-1\\.\n\n**Example 1:**\n\n**Input:** nums \\= \\[0,1,1,1,0,0]\n\n**Output:** 3\n\n**Explanation:** \n\nWe can do the following operations:\n\n* Choose the elements at indices 0, 1 and 2\\. The resulting array is `nums = [1,0,0,1,0,0]`.\n* Choose the elements at indices 1, 2 and 3\\. The resulting array is `nums = [1,1,1,0,0,0]`.\n* Choose the elements at indices 3, 4 and 5\\. The resulting array is `nums = [1,1,1,1,1,1]`.\n\n**Example 2:**\n\n**Input:** nums \\= \\[0,1,1,1]\n\n**Output:** \\-1\n\n**Explanation:** \n\nIt is impossible to make all elements equal to 1\\.\n\n**Constraints:**\n\n* `3 <= nums.length <= 105`\n* `0 <= nums[i] <= 1`", "templates": {"cpp": "class Solution {\npublic:\n int minOperations(vector<int>& nums) {\n \n }\n};", "java": "class Solution {\n public int minOperations(int[] nums) {\n \n }\n}", "python": "class Solution:\n def minOperations(self, nums: List[int]) -> int:\n "}, "hints": "1\\. If `nums[0]` is 0, then the only way to change it to 1 is by doing an operation on the first 3 elements of the array.\n2\\. After Changing `nums[0]` to 1, use the same logic on the remaining array.", "contains_png": false, "png_links": []}
{"number": 3192, "title": "Minimum Operations to Make Binary Array Elements Equal to One II", "link": "https://leetcode.com/problems/minimum-operations-to-make-binary-array-elements-equal-to-one-ii/", "difficulty": "medium", "statement": "You are given a binary array `nums`.\n\nYou can do the following operation on the array **any** number of times (possibly zero):\n\n* Choose **any** index `i` from the array and **flip** **all** the elements from index `i` to the end of the array.\n\n**Flipping** an element means changing its value from 0 to 1, and from 1 to 0\\.\n\nReturn the **minimum** number of operations required to make all elements in `nums` equal to 1\\.\n\n**Example 1:**\n\n**Input:** nums \\= \\[0,1,1,0,1]\n\n**Output:** 4\n\n**Explanation:** \n\nWe can do the following operations:\n\n* Choose the index `i = 1`. The resulting array will be `nums = [0,0,0,1,0]`.\n* Choose the index `i = 0`. The resulting array will be `nums = [1,1,1,0,1]`.\n* Choose the index `i = 4`. The resulting array will be `nums = [1,1,1,0,0]`.\n* Choose the index `i = 3`. The resulting array will be `nums = [1,1,1,1,1]`.\n\n**Example 2:**\n\n**Input:** nums \\= \\[1,0,0,0]\n\n**Output:** 1\n\n**Explanation:** \n\nWe can do the following operation:\n\n* Choose the index `i = 1`. The resulting array will be `nums = [1,1,1,1]`.\n\n**Constraints:**\n\n* `1 <= nums.length <= 105`\n* `0 <= nums[i] <= 1`", "templates": {"cpp": "class Solution {\npublic:\n int minOperations(vector<int>& nums) {\n \n }\n};", "java": "class Solution {\n public int minOperations(int[] nums) {\n \n }\n}", "python": "class Solution:\n def minOperations(self, nums: List[int]) -> int:\n "}, "hints": "1\\. The only way to change `nums[0]` to 1 is by performing an operation with index `i = 0`.\n2\\. Iterate from left to right and perform an operation at each index i where nums\\[i] is 0, and keep track of how many operations are currently performed on the suffix.", "contains_png": false, "png_links": []}
{"number": 3193, "title": "Count the Number of Inversions", "link": "https://leetcode.com/problems/count-the-number-of-inversions/", "difficulty": "hard", "statement": "You are given an integer `n` and a 2D array `requirements`, where `requirements[i] = [endi, cnti]` represents the end index and the **inversion** count of each requirement.\n\nA pair of indices `(i, j)` from an integer array `nums` is called an **inversion** if:\n\n* `i < j` and `nums[i] > nums[j]`\n\nReturn the number of permutations `perm` of `[0, 1, 2, ..., n - 1]` such that for **all** `requirements[i]`, `perm[0..endi]` has exactly `cnti` inversions.\n\nSince the answer may be very large, return it **modulo** `109 + 7`.\n\n**Example 1:**\n\n**Input:** n \\= 3, requirements \\= \\[\\[2,2],\\[0,0]]\n\n**Output:** 2\n\n**Explanation:**\n\nThe two permutations are:\n\n* `[2, 0, 1]`\n\t+ Prefix `[2, 0, 1]` has inversions `(0, 1)` and `(0, 2)`.\n\t+ Prefix `[2]` has 0 inversions.\n* `[1, 2, 0]`\n\t+ Prefix `[1, 2, 0]` has inversions `(0, 2)` and `(1, 2)`.\n\t+ Prefix `[1]` has 0 inversions.\n\n**Example 2:**\n\n**Input:** n \\= 3, requirements \\= \\[\\[2,2],\\[1,1],\\[0,0]]\n\n**Output:** 1\n\n**Explanation:**\n\nThe only satisfying permutation is `[2, 0, 1]`:\n\n* Prefix `[2, 0, 1]` has inversions `(0, 1)` and `(0, 2)`.\n* Prefix `[2, 0]` has an inversion `(0, 1)`.\n* Prefix `[2]` has 0 inversions.\n\n**Example 3:**\n\n**Input:** n \\= 2, requirements \\= \\[\\[0,0],\\[1,0]]\n\n**Output:** 1\n\n**Explanation:**\n\nThe only satisfying permutation is `[0, 1]`:\n\n* Prefix `[0]` has 0 inversions.\n* Prefix `[0, 1]` has an inversion `(0, 1)`.\n\n**Constraints:**\n\n* `2 <= n <= 300`\n* `1 <= requirements.length <= n`\n* `requirements[i] = [endi, cnti]`\n* `0 <= endi <= n - 1`\n* `0 <= cnti <= 400`\n* The input is generated such that there is at least one `i` such that `endi == n - 1`.\n* The input is generated such that all `endi` are unique.", "templates": {"cpp": "class Solution {\npublic:\n int numberOfPermutations(int n, vector<vector<int>>& requirements) {\n \n }\n};", "java": "class Solution {\n public int numberOfPermutations(int n, int[][] requirements) {\n \n }\n}", "python": "class Solution:\n def numberOfPermutations(self, n: int, requirements: List[List[int]]) -> int:\n "}, "hints": "1\\. Let `dp[i][j]` denote the number of arrays of length `i` with `j` inversions.\n2\\. `dp[i][j] = dp[i - 1][j] + dp[i - 1][j - 1] + \u2026 + dp[i - 1][0]`.\n3\\. `dp[i][j] = 0` if for some `x`, `requirements[x][0] == i` and `requirements[x][1] != j`.", "contains_png": false, "png_links": []}
{"number": 3194, "title": "Minimum Average of Smallest and Largest Elements", "link": "https://leetcode.com/problems/minimum-average-of-smallest-and-largest-elements/", "difficulty": "easy", "statement": "You have an array of floating point numbers `averages` which is initially empty. You are given an array `nums` of `n` integers where `n` is even.\n\nYou repeat the following procedure `n / 2` times:\n\n* Remove the **smallest** element, `minElement`, and the **largest** element `maxElement`,\u00a0from `nums`.\n* Add `(minElement + maxElement) / 2` to `averages`.\n\nReturn the **minimum** element in `averages`.\n\n**Example 1:**\n\n**Input:** nums \\= \\[7,8,3,4,15,13,4,1]\n\n**Output:** 5\\.5\n\n**Explanation:**\n\n| step | nums | averages |\n| --- | --- | --- |\n| 0 | \\[7,8,3,4,15,13,4,1] | \\[] |\n| 1 | \\[7,8,3,4,13,4] | \\[8] |\n| 2 | \\[7,8,4,4] | \\[8,8] |\n| 3 | \\[7,4] | \\[8,8,6] |\n| 4 | \\[] | \\[8,8,6,5\\.5] |\n\nThe smallest element of averages, 5\\.5, is returned.\n**Example 2:**\n\n**Input:** nums \\= \\[1,9,8,3,10,5]\n\n**Output:** 5\\.5\n\n**Explanation:**\n\n| step | nums | averages |\n| --- | --- | --- |\n| 0 | \\[1,9,8,3,10,5] | \\[] |\n| 1 | \\[9,8,3,5] | \\[5\\.5] |\n| 2 | \\[8,5] | \\[5\\.5,6] |\n| 3 | \\[] | \\[5\\.5,6,6\\.5] |\n\n**Example 3:**\n\n**Input:** nums \\= \\[1,2,3,7,8,9]\n\n**Output:** 5\\.0\n\n**Explanation:**\n\n| step | nums | averages |\n| --- | --- | --- |\n| 0 | \\[1,2,3,7,8,9] | \\[] |\n| 1 | \\[2,3,7,8] | \\[5] |\n| 2 | \\[3,7] | \\[5,5] |\n| 3 | \\[] | \\[5,5,5] |\n\n**Constraints:**\n\n* `2 <= n == nums.length <= 50`\n* `n` is even.\n* `1 <= nums[i] <= 50`", "templates": {"cpp": "class Solution {\npublic:\n double minimumAverage(vector<int>& nums) {\n \n }\n};", "java": "class Solution {\n public double minimumAverage(int[] nums) {\n \n }\n}", "python": "class Solution:\n def minimumAverage(self, nums: List[int]) -> float:\n "}, "hints": "1\\. If `nums` is sorted, then the elements of `averages` are `(nums[i] + nums[n - i - 1]) / 2` for all `i < n / 2`.", "contains_png": false, "png_links": []}
{"number": 3195, "title": "Find the Minimum Area to Cover All Ones I", "link": "https://leetcode.com/problems/find-the-minimum-area-to-cover-all-ones-i/", "difficulty": "medium", "statement": "You are given a 2D **binary** array `grid`. Find a rectangle with horizontal and vertical sides with the **smallest** area, such that all the 1's in `grid` lie inside this rectangle.\n\nReturn the **minimum** possible area of the rectangle.\n\n**Example 1:**\n\n**Input:** grid \\= \\[\\[0,1,0],\\[1,0,1]]\n\n**Output:** 6\n\n**Explanation:**\n\n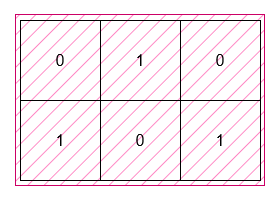\n\nThe smallest rectangle has a height of 2 and a width of 3, so it has an area of `2 * 3 = 6`.\n\n**Example 2:**\n\n**Input:** grid \\= \\[\\[1,0],\\[0,0]]\n\n**Output:** 1\n\n**Explanation:**\n\n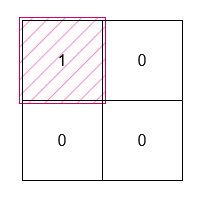\n\nThe smallest rectangle has both height and width 1, so its area is `1 * 1 = 1`.\n\n**Constraints:**\n\n* `1 <= grid.length, grid[i].length <= 1000`\n* `grid[i][j]` is either 0 or 1\\.\n* The input is generated such that there is at least one 1 in `grid`.", "templates": {"cpp": "class Solution {\npublic:\n int minimumArea(vector<vector<int>>& grid) {\n \n }\n};", "java": "class Solution {\n public int minimumArea(int[][] grid) {\n \n }\n}", "python": "class Solution:\n def minimumArea(self, grid: List[List[int]]) -> int:\n "}, "hints": "1\\. Find the minimum and maximum coordinates of a cell with a value of 1 in both directions.", "contains_png": true, "png_links": ["https://assets.leetcode.com/uploads/2024/05/08/examplerect0.png", "https://assets.leetcode.com/uploads/2024/05/08/examplerect1.png"]}
{"number": 3196, "title": "Maximize Total Cost of Alternating Subarrays", "link": "https://leetcode.com/problems/maximize-total-cost-of-alternating-subarrays/", "difficulty": "medium", "statement": "You are given an integer array `nums` with length `n`.\n\nThe **cost** of a subarray `nums[l..r]`, where `0 <= l <= r < n`, is defined as:\n\n`cost(l, r) = nums[l] - nums[l + 1] + ... + nums[r] * (\u22121)r \u2212 l`\n\nYour task is to **split** `nums` into subarrays such that the **total** **cost** of the subarrays is **maximized**, ensuring each element belongs to **exactly one** subarray.\n\nFormally, if `nums` is split into `k` subarrays, where `k > 1`, at indices `i1, i2, ..., ik \u2212 1`, where `0 <= i1 < i2 < ... < ik - 1 < n - 1`, then the total cost will be:\n\n`cost(0, i1) + cost(i1 + 1, i2) + ... + cost(ik \u2212 1 + 1, n \u2212 1)`\n\nReturn an integer denoting the *maximum total cost* of the subarrays after splitting the array optimally.\n\n**Note:** If `nums` is not split into subarrays, i.e. `k = 1`, the total cost is simply `cost(0, n - 1)`.\n\n**Example 1:**\n\n**Input:** nums \\= \\[1,\\-2,3,4]\n\n**Output:** 10\n\n**Explanation:**\n\nOne way to maximize the total cost is by splitting `[1, -2, 3, 4]` into subarrays `[1, -2, 3]` and `[4]`. The total cost will be `(1 + 2 + 3) + 4 = 10`.\n\n**Example 2:**\n\n**Input:** nums \\= \\[1,\\-1,1,\\-1]\n\n**Output:** 4\n\n**Explanation:**\n\nOne way to maximize the total cost is by splitting `[1, -1, 1, -1]` into subarrays `[1, -1]` and `[1, -1]`. The total cost will be `(1 + 1) + (1 + 1) = 4`.\n\n**Example 3:**\n\n**Input:** nums \\= \\[0]\n\n**Output:** 0\n\n**Explanation:**\n\nWe cannot split the array further, so the answer is 0\\.\n\n**Example 4:**\n\n**Input:** nums \\= \\[1,\\-1]\n\n**Output:** 2\n\n**Explanation:**\n\nSelecting the whole array gives a total cost of `1 + 1 = 2`, which is the maximum.\n\n**Constraints:**\n\n* `1 <= nums.length <= 105`\n* `-109 <= nums[i] <= 109`", "templates": {"cpp": "class Solution {\npublic:\n long long maximumTotalCost(vector<int>& nums) {\n \n }\n};", "java": "class Solution {\n public long maximumTotalCost(int[] nums) {\n \n }\n}", "python": "class Solution:\n def maximumTotalCost(self, nums: List[int]) -> int:\n "}, "hints": "1\\. The problem can be solved using dynamic programming.\n2\\. Since we can always start a new subarray, the problem is the same as selecting some elements in the array and flipping their signs to negative to maximize the sum. However, we cannot flip the signs of 2 consecutive elements, and the first element in the array cannot be negative.\n3\\. Let `dp[i][0/1]` be the largest sum we can get for prefix `nums[0..i]`, where `dp[i][0]` is the maximum if the `ith` element wasn't flipped, and `dp[i][1]` is the maximum if the `ith` element was flipped.\n4\\. Based on the restriction: \n\n`dp[i][0] = max(dp[i - 1][0], dp[i - 1][1]) + nums[i]` \n\n`dp[i][1] = dp[i - 1][0] - nums[i]`\n5\\. The initial state is: \n\n`dp[1][0] = nums[0] + nums[1]` \n\n`dp[1][1] = nums[0] - nums[1]` \n\nand the answer is `max(dp[n - 1][0], dp[n - 1][1])`.\n6\\. Can you optimize the space complexity?", "contains_png": false, "png_links": []}
{"number": 3197, "title": "Find the Minimum Area to Cover All Ones II", "link": "https://leetcode.com/problems/find-the-minimum-area-to-cover-all-ones-ii/", "difficulty": "hard", "statement": "You are given a 2D **binary** array `grid`. You need to find 3 **non\\-overlapping** rectangles having **non\\-zero** areas with horizontal and vertical sides such that all the 1's in `grid` lie inside these rectangles.\n\nReturn the **minimum** possible sum of the area of these rectangles.\n\n**Note** that the rectangles are allowed to touch.\n\n**Example 1:**\n\n**Input:** grid \\= \\[\\[1,0,1],\\[1,1,1]]\n\n**Output:** 5\n\n**Explanation:**\n\n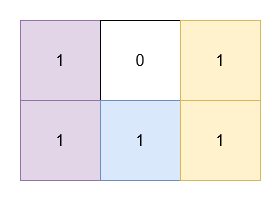\n\n* The 1's at `(0, 0)` and `(1, 0)` are covered by a rectangle of area 2\\.\n* The 1's at `(0, 2)` and `(1, 2)` are covered by a rectangle of area 2\\.\n* The 1 at `(1, 1)` is covered by a rectangle of area 1\\.\n\n**Example 2:**\n\n**Input:** grid \\= \\[\\[1,0,1,0],\\[0,1,0,1]]\n\n**Output:** 5\n\n**Explanation:**\n\n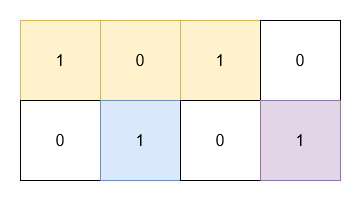\n\n* The 1's at `(0, 0)` and `(0, 2)` are covered by a rectangle of area 3\\.\n* The 1 at `(1, 1)` is covered by a rectangle of area 1\\.\n* The 1 at `(1, 3)` is covered by a rectangle of area 1\\.\n\n**Constraints:**\n\n* `1 <= grid.length, grid[i].length <= 30`\n* `grid[i][j]` is either 0 or 1\\.\n* The input is generated such that there are at least three 1's in `grid`.", "templates": {"cpp": "class Solution {\npublic:\n int minimumSum(vector<vector<int>>& grid) {\n \n }\n};", "java": "class Solution {\n public int minimumSum(int[][] grid) {\n \n }\n}", "python": "class Solution:\n def minimumSum(self, grid: List[List[int]]) -> int:\n "}, "hints": "1\\. Consider covering using 2 rectangles. As the rectangles don\u2019t overlap, one of the rectangles must either be vertically above or horizontally left to the other.\n2\\. To find the minimum area, check all possible vertical and horizontal splits.\n3\\. For 3 rectangles, extend the idea to first covering using one rectangle, and then try splitting leftover ones both horizontally and vertically.", "contains_png": true, "png_links": ["https://assets.leetcode.com/uploads/2024/05/14/example0rect21.png", "https://assets.leetcode.com/uploads/2024/05/14/example1rect2.png"]}
{"number": 3200, "title": "Maximum Height of a Triangle", "link": "https://leetcode.com/problems/maximum-height-of-a-triangle/", "difficulty": "easy", "statement": "You are given two integers `red` and `blue` representing the count of red and blue colored balls. You have to arrange these balls to form a triangle such that the 1st row will have 1 ball, the 2nd row will have 2 balls, the 3rd row will have 3 balls, and so on.\n\nAll the balls in a particular row should be the **same** color, and adjacent rows should have **different** colors.\n\nReturn the **maximum** *height of the triangle* that can be achieved.\n\n**Example 1:**\n\n**Input:** red \\= 2, blue \\= 4\n\n**Output:** 3\n\n**Explanation:**\n\n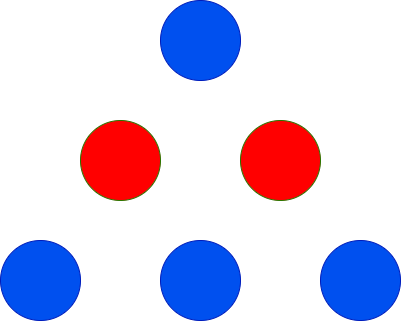\n\nThe only possible arrangement is shown above.\n\n**Example 2:**\n\n**Input:** red \\= 2, blue \\= 1\n\n**Output:** 2\n\n**Explanation:**\n\n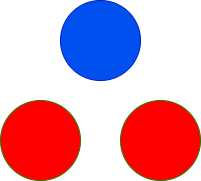 \n\nThe only possible arrangement is shown above.\n\n**Example 3:**\n\n**Input:** red \\= 1, blue \\= 1\n\n**Output:** 1\n\n**Example 4:**\n\n**Input:** red \\= 10, blue \\= 1\n\n**Output:** 2\n\n**Explanation:**\n\n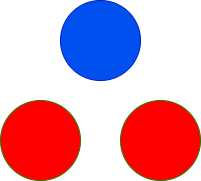 \n\nThe only possible arrangement is shown above.\n\n**Constraints:**\n\n* `1 <= red, blue <= 100`", "templates": {"cpp": "class Solution {\npublic:\n int maxHeightOfTriangle(int red, int blue) {\n \n }\n};", "java": "class Solution {\n public int maxHeightOfTriangle(int red, int blue) {\n \n }\n}", "python": "class Solution:\n def maxHeightOfTriangle(self, red: int, blue: int) -> int:\n "}, "hints": "1\\. Count the max height using both possibilities. That is, red ball as top and blue ball as top.\n2\\. For counting the max height, use a simple for loop and remove the number of balls required at this level.", "contains_png": true, "png_links": ["https://assets.leetcode.com/uploads/2024/06/16/brb.png", "https://assets.leetcode.com/uploads/2024/06/16/br.png", "https://assets.leetcode.com/uploads/2024/06/16/br.png"]}
{"number": 3201, "title": "Find the Maximum Length of Valid Subsequence I", "link": "https://leetcode.com/problems/find-the-maximum-length-of-valid-subsequence-i/", "difficulty": "medium", "statement": "You are given an integer array `nums`.\nA subsequence `sub` of `nums` with length `x` is called **valid** if it satisfies:\n\n* `(sub[0] + sub[1]) % 2 == (sub[1] + sub[2]) % 2 == ... == (sub[x - 2] + sub[x - 1]) % 2.`\n\nReturn the length of the **longest** **valid** subsequence of `nums`.\n\nA **subsequence** is an array that can be derived from another array by deleting some or no elements without changing the order of the remaining elements.\n\n**Example 1:**\n\n**Input:** nums \\= \\[1,2,3,4]\n\n**Output:** 4\n\n**Explanation:**\n\nThe longest valid subsequence is `[1, 2, 3, 4]`.\n\n**Example 2:**\n\n**Input:** nums \\= \\[1,2,1,1,2,1,2]\n\n**Output:** 6\n\n**Explanation:**\n\nThe longest valid subsequence is `[1, 2, 1, 2, 1, 2]`.\n\n**Example 3:**\n\n**Input:** nums \\= \\[1,3]\n\n**Output:** 2\n\n**Explanation:**\n\nThe longest valid subsequence is `[1, 3]`.\n\n**Constraints:**\n\n* `2 <= nums.length <= 2 * 105`\n* `1 <= nums[i] <= 107`", "templates": {"cpp": "class Solution {\npublic:\n int maximumLength(vector<int>& nums) {\n \n }\n};", "java": "class Solution {\n public int maximumLength(int[] nums) {\n \n }\n}", "python": "class Solution:\n def maximumLength(self, nums: List[int]) -> int:\n "}, "hints": "1\\. The possible sequence either contains all even elements, all odd elements, alternate even odd, or alternate odd even elements.\n2\\. Considering only the parity of elements, there are only 4 possibilities and we can try all of them.\n3\\. When selecting an element with any parity, try to select the earliest one.", "contains_png": false, "png_links": []}
{"number": 3202, "title": "Find the Maximum Length of Valid Subsequence II", "link": "https://leetcode.com/problems/find-the-maximum-length-of-valid-subsequence-ii/", "difficulty": "medium", "statement": "You are given an integer array `nums` and a **positive** integer `k`.\nA subsequence `sub` of `nums` with length `x` is called **valid** if it satisfies:\n\n* `(sub[0] + sub[1]) % k == (sub[1] + sub[2]) % k == ... == (sub[x - 2] + sub[x - 1]) % k.`\n\nReturn the length of the **longest** **valid** subsequence of `nums`.\n\n**Example 1:**\n\n**Input:** nums \\= \\[1,2,3,4,5], k \\= 2\n\n**Output:** 5\n\n**Explanation:**\n\nThe longest valid subsequence is `[1, 2, 3, 4, 5]`.\n\n**Example 2:**\n\n**Input:** nums \\= \\[1,4,2,3,1,4], k \\= 3\n\n**Output:** 4\n\n**Explanation:**\n\nThe longest valid subsequence is `[1, 4, 1, 4]`.\n\n**Constraints:**\n\n* `2 <= nums.length <= 103`\n* `1 <= nums[i] <= 107`\n* `1 <= k <= 103`", "templates": {"cpp": "class Solution {\npublic:\n int maximumLength(vector<int>& nums, int k) {\n \n }\n};", "java": "class Solution {\n public int maximumLength(int[] nums, int k) {\n \n }\n}", "python": "class Solution:\n def maximumLength(self, nums: List[int], k: int) -> int:\n "}, "hints": "1\\. Fix the value of `(subs[0] + subs[1]) % k` from the `k` possible values. Let it be `val`.\n2\\. Let `dp[i]` store the maximum length of a subsequence with its last element `x` such that `x % k == i`.\n3\\. Answer for a subsequence ending at index `y` is `dp[(k + val - (y % k)) % k] + 1`.", "contains_png": false, "png_links": []}
{"number": 3203, "title": "Find Minimum Diameter After Merging Two Trees", "link": "https://leetcode.com/problems/find-minimum-diameter-after-merging-two-trees/", "difficulty": "hard", "statement": "There exist two **undirected** trees with `n` and `m` nodes, numbered from `0` to `n - 1` and from `0` to `m - 1`, respectively. You are given two 2D integer arrays `edges1` and `edges2` of lengths `n - 1` and `m - 1`, respectively, where `edges1[i] = [ai, bi]` indicates that there is an edge between nodes `ai` and `bi` in the first tree and `edges2[i] = [ui, vi]` indicates that there is an edge between nodes `ui` and `vi` in the second tree.\n\nYou must connect one node from the first tree with another node from the second tree with an edge.\n\nReturn the **minimum** possible **diameter** of the resulting tree.\n\nThe **diameter** of a tree is the length of the *longest* path between any two nodes in the tree.\n\n**Example 1:**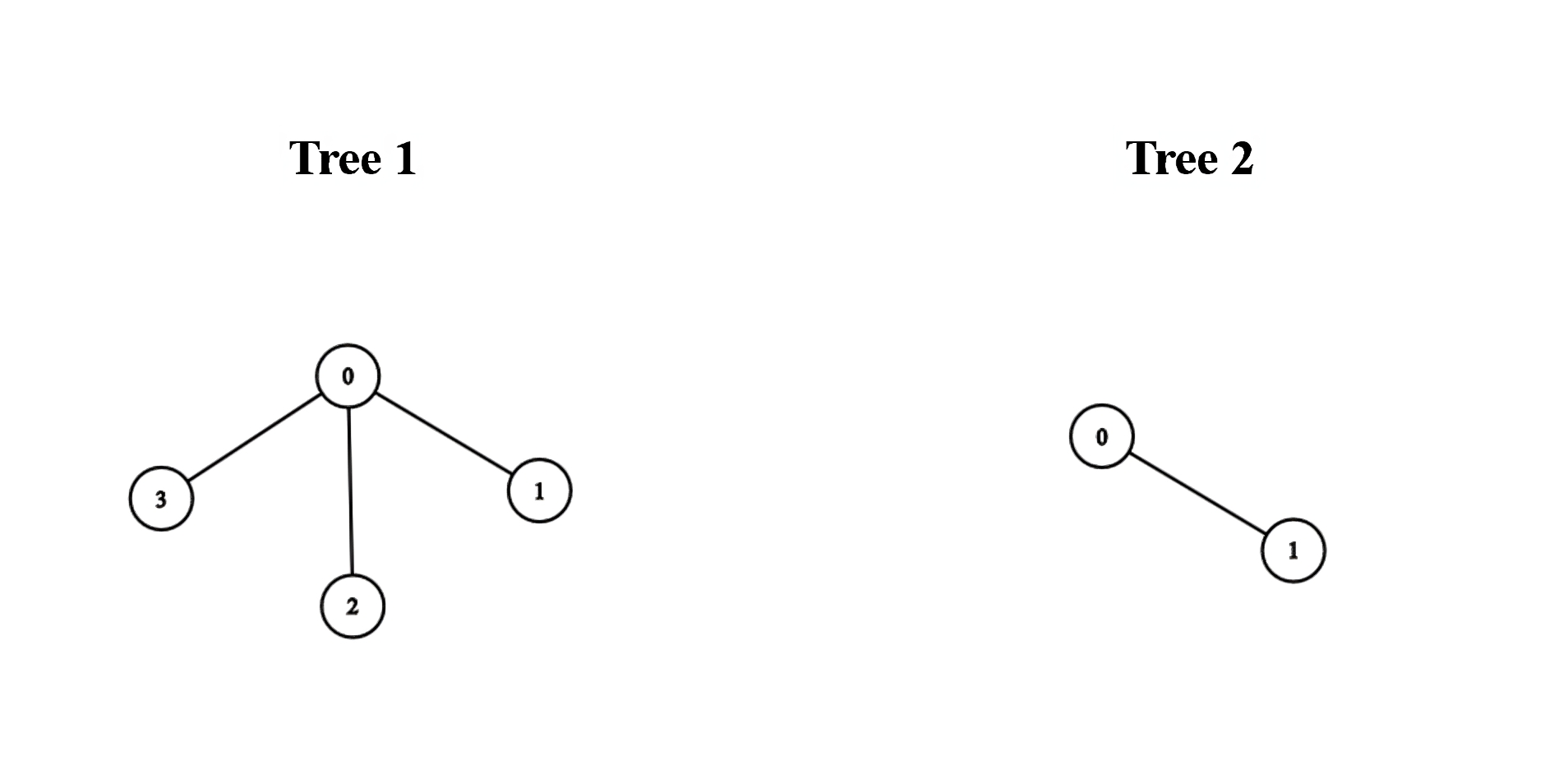\n\n**Input:** edges1 \\= \\[\\[0,1],\\[0,2],\\[0,3]], edges2 \\= \\[\\[0,1]]\n\n**Output:** 3\n\n**Explanation:**\n\nWe can obtain a tree of diameter 3 by connecting node 0 from the first tree with any node from the second tree.\n\n**Example 2:**\n\n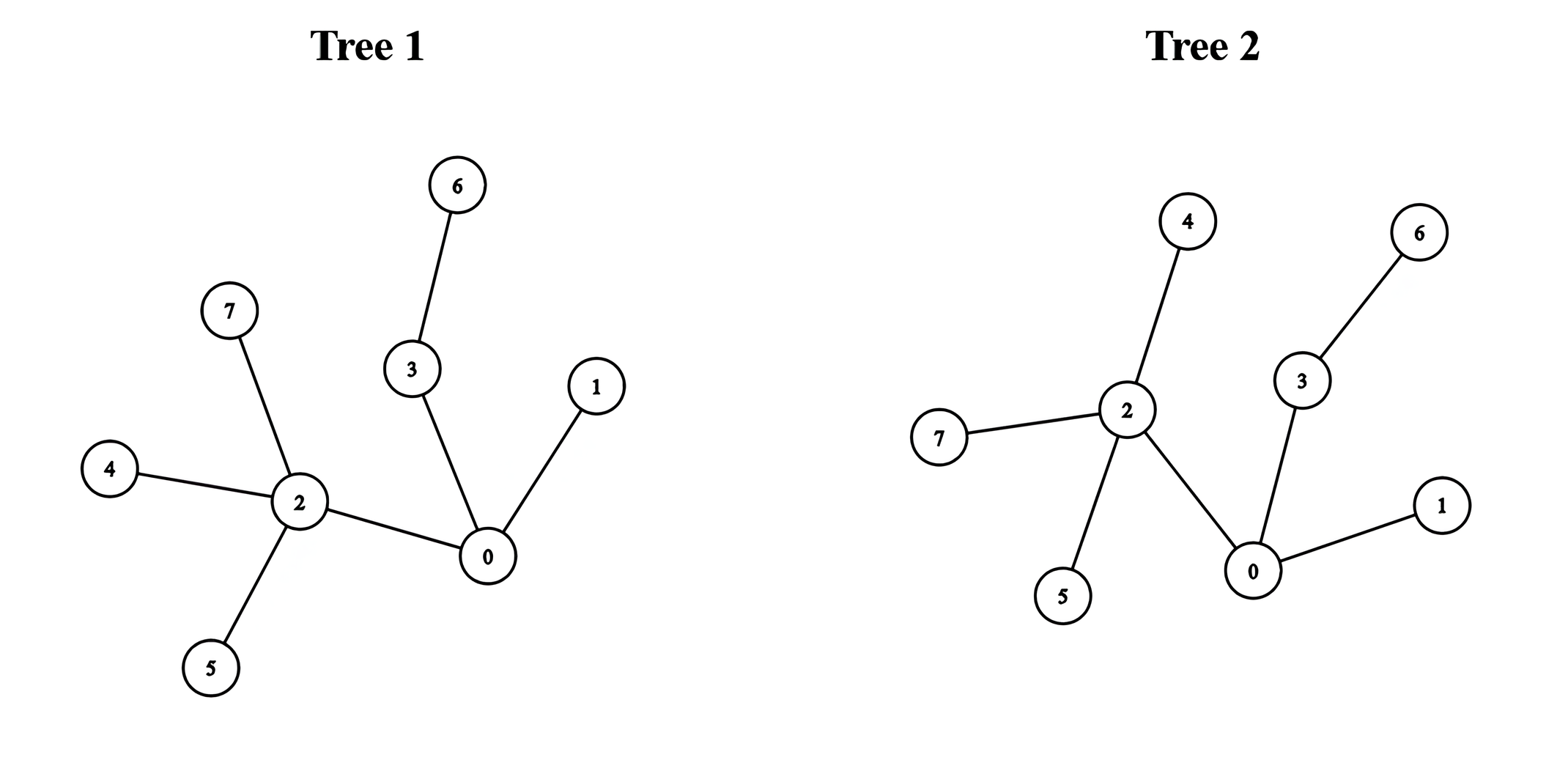\n\n**Input:** edges1 \\= \\[\\[0,1],\\[0,2],\\[0,3],\\[2,4],\\[2,5],\\[3,6],\\[2,7]], edges2 \\= \\[\\[0,1],\\[0,2],\\[0,3],\\[2,4],\\[2,5],\\[3,6],\\[2,7]]\n\n**Output:** 5\n\n**Explanation:**\n\nWe can obtain a tree of diameter 5 by connecting node 0 from the first tree with node 0 from the second tree.\n\n**Constraints:**\n\n* `1 <= n, m <= 105`\n* `edges1.length == n - 1`\n* `edges2.length == m - 1`\n* `edges1[i].length == edges2[i].length == 2`\n* `edges1[i] = [ai, bi]`\n* `0 <= ai, bi < n`\n* `edges2[i] = [ui, vi]`\n* `0 <= ui, vi < m`\n* The input is generated such that `edges1` and `edges2` represent valid trees.", "templates": {"cpp": "class Solution {\npublic:\n int minimumDiameterAfterMerge(vector<vector<int>>& edges1, vector<vector<int>>& edges2) {\n \n }\n};", "java": "class Solution {\n public int minimumDiameterAfterMerge(int[][] edges1, int[][] edges2) {\n \n }\n}", "python": "class Solution:\n def minimumDiameterAfterMerge(self, edges1: List[List[int]], edges2: List[List[int]]) -> int:\n "}, "hints": "1\\. Suppose that we connected node `a` in tree1 with node `b` in tree2\\. The diameter length of the resulting tree will be the largest of the following 3 values: \n1. The diameter of tree 1\\.\n2. The diameter of tree 2\\.\n3. The length of the longest path that starts at node `a` and that is completely within Tree 1 \\+ The length of the longest path that starts at node `b` and that is completely within Tree 2 \\+ 1\\.\n\nThe added one in the third value is due to the additional edge that we have added between trees 1 and 2\\.\n2\\. Values 1 and 2 are constant regardless of our choice of `a` and `b`. Therefore, we need to pick `a` and `b` in such a way that minimizes value 3\\.\n3\\. If we pick `a` and `b` optimally, they will be in the diameters of Tree 1 and Tree 2, respectively. Exactly which nodes of the diameter should we pick?\n4\\. `a` is the center of the diameter of tree 1, and `b` is the center of the diameter of tree 2\\.", "contains_png": true, "png_links": ["https://assets.leetcode.com/uploads/2024/04/22/example11-transformed.png", "https://assets.leetcode.com/uploads/2024/04/22/example211.png"]}
{"number": 3206, "title": "Alternating Groups I", "link": "https://leetcode.com/problems/alternating-groups-i/", "difficulty": "easy", "statement": "There is a circle of red and blue tiles. You are given an array of integers `colors`. The color of tile `i` is represented by `colors[i]`:\n\n* `colors[i] == 0` means that tile `i` is **red**.\n* `colors[i] == 1` means that tile `i` is **blue**.\n\nEvery 3 contiguous tiles in the circle with **alternating** colors (the middle tile has a different color from its **left** and **right** tiles) is called an **alternating** group.\n\nReturn the number of **alternating** groups.\n\n**Note** that since `colors` represents a **circle**, the **first** and the **last** tiles are considered to be next to each other.\n\n**Example 1:**\n\n**Input:** colors \\= \\[1,1,1]\n\n**Output:** 0\n\n**Explanation:**\n\n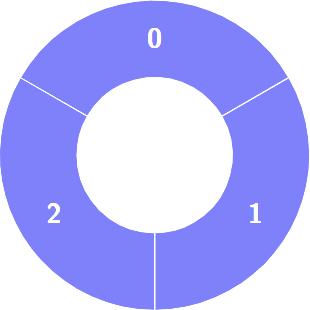\n\n**Example 2:**\n\n**Input:** colors \\= \\[0,1,0,0,1]\n\n**Output:** 3\n\n**Explanation:**\n\n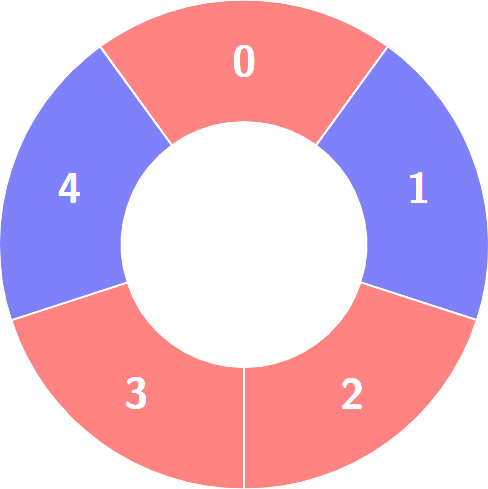\n\nAlternating groups:\n\n**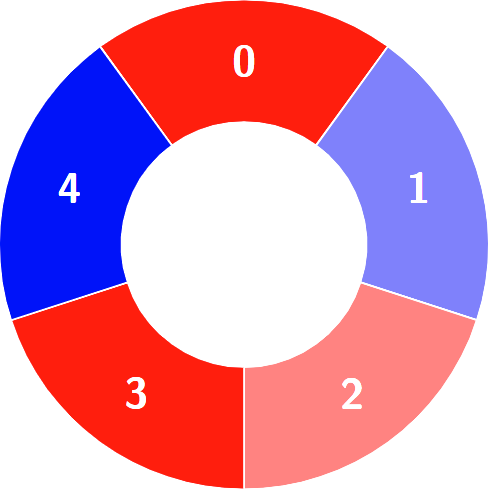**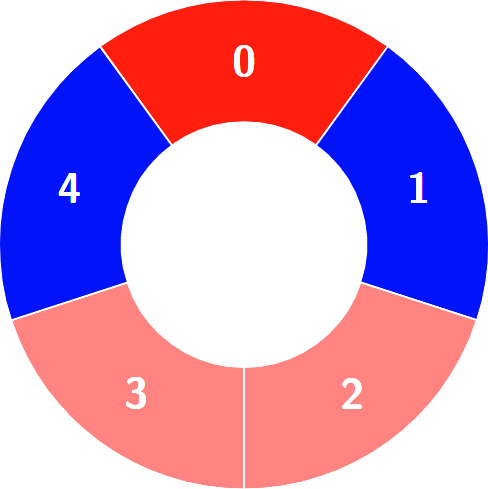**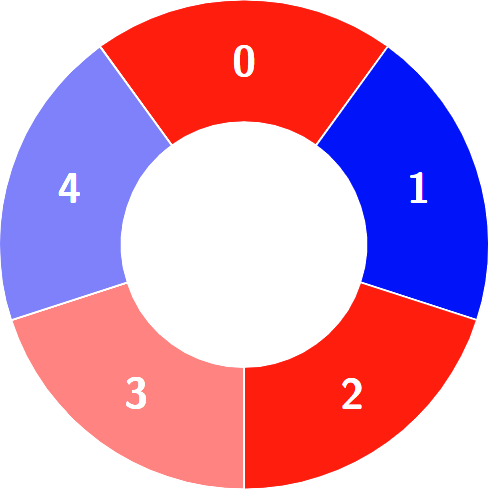**\n\n**Constraints:**\n\n* `3 <= colors.length <= 100`\n* `0 <= colors[i] <= 1`", "templates": {"cpp": "class Solution {\npublic:\n int numberOfAlternatingGroups(vector<int>& colors) {\n \n }\n};", "java": "class Solution {\n public int numberOfAlternatingGroups(int[] colors) {\n \n }\n}", "python": "class Solution:\n def numberOfAlternatingGroups(self, colors: List[int]) -> int:\n "}, "hints": "1\\. For each tile, check that the previous and the next tile have different colors from that tile or not.", "contains_png": true, "png_links": ["https://assets.leetcode.com/uploads/2024/05/16/image_2024-05-16_23-53-171.png", "https://assets.leetcode.com/uploads/2024/05/16/image_2024-05-16_23-47-491.png", "https://assets.leetcode.com/uploads/2024/05/16/image_2024-05-16_23-50-441.png", "https://assets.leetcode.com/uploads/2024/05/16/image_2024-05-16_23-48-211.png", "https://assets.leetcode.com/uploads/2024/05/16/image_2024-05-16_23-49-351.png"]}
{"number": 3207, "title": "Maximum Points After Enemy Battles", "link": "https://leetcode.com/problems/maximum-points-after-enemy-battles/", "difficulty": "medium", "statement": "You are given an integer array `enemyEnergies` denoting the energy values of various enemies.\n\nYou are also given an integer `currentEnergy` denoting the amount of energy you have initially.\n\nYou start with 0 points, and all the enemies are unmarked initially.\n\nYou can perform **either** of the following operations **zero** or multiple times to gain points:\n\n* Choose an **unmarked** enemy, `i`, such that `currentEnergy >= enemyEnergies[i]`. By choosing this option:\n\t+ You gain 1 point.\n\t+ Your energy is reduced by the enemy's energy, i.e. `currentEnergy = currentEnergy - enemyEnergies[i]`.\n* If you have **at least** 1 point, you can choose an **unmarked** enemy, `i`. By choosing this option:\n\t+ Your energy increases by the enemy's energy, i.e. `currentEnergy = currentEnergy + enemyEnergies[i]`.\n\t+ The enemy `i` is **marked**.\n\nReturn an integer denoting the **maximum** points you can get in the end by optimally performing operations.\n\n**Example 1:**\n\n**Input:** enemyEnergies \\= \\[3,2,2], currentEnergy \\= 2\n\n**Output:** 3\n\n**Explanation:**\n\nThe following operations can be performed to get 3 points, which is the maximum:\n\n* First operation on enemy 1: `points` increases by 1, and `currentEnergy` decreases by 2\\. So, `points = 1`, and `currentEnergy = 0`.\n* Second operation on enemy 0: `currentEnergy` increases by 3, and enemy 0 is marked. So, `points = 1`, `currentEnergy = 3`, and marked enemies \\= `[0]`.\n* First operation on enemy 2: `points` increases by 1, and `currentEnergy` decreases by 2\\. So, `points = 2`, `currentEnergy = 1`, and marked enemies \\= `[0]`.\n* Second operation on enemy 2: `currentEnergy` increases by 2, and enemy 2 is marked. So, `points = 2`, `currentEnergy = 3`, and marked enemies \\= `[0, 2]`.\n* First operation on enemy 1: `points` increases by 1, and `currentEnergy` decreases by 2\\. So, `points = 3`, `currentEnergy = 1`, and marked enemies \\= `[0, 2]`.\n\n**Example 2:**\n\n**Input:** enemyEnergies \\= \\[2], currentEnergy \\= 10\n\n**Output:** 5\n\n**Explanation:** \n\nPerforming the first operation 5 times on enemy 0 results in the maximum number of points.\n\n**Constraints:**\n\n* `1 <= enemyEnergies.length <= 105`\n* `1 <= enemyEnergies[i] <= 109`\n* `0 <= currentEnergy <= 109`", "templates": {"cpp": "class Solution {\npublic:\n long long maximumPoints(vector<int>& enemyEnergies, int currentEnergy) {\n \n }\n};", "java": "class Solution {\n public long maximumPoints(int[] enemyEnergies, int currentEnergy) {\n \n }\n}", "python": "class Solution:\n def maximumPoints(self, enemyEnergies: List[int], currentEnergy: int) -> int:\n "}, "hints": "1\\. The problem can be solved greedily.\n2\\. Mark all the others except the smallest one first.\n3\\. Use the smallest one to increase the energy.\n4\\. Note that the initial energy should be no less than the smallest enemy.", "contains_png": false, "png_links": []}
{"number": 3208, "title": "Alternating Groups II", "link": "https://leetcode.com/problems/alternating-groups-ii/", "difficulty": "medium", "statement": "There is a circle of red and blue tiles. You are given an array of integers `colors` and an integer `k`. The color of tile `i` is represented by `colors[i]`:\n\n* `colors[i] == 0` means that tile `i` is **red**.\n* `colors[i] == 1` means that tile `i` is **blue**.\n\nAn **alternating** group is every `k` contiguous tiles in the circle with **alternating** colors (each tile in the group except the first and last one has a different color from its **left** and **right** tiles).\n\nReturn the number of **alternating** groups.\n\n**Note** that since `colors` represents a **circle**, the **first** and the **last** tiles are considered to be next to each other.\n\n**Example 1:**\n\n**Input:** colors \\= \\[0,1,0,1,0], k \\= 3\n\n**Output:** 3\n\n**Explanation:**\n\n**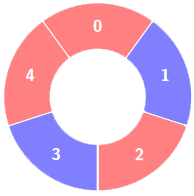**\n\nAlternating groups:\n\n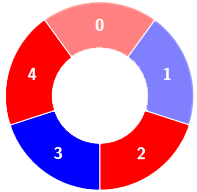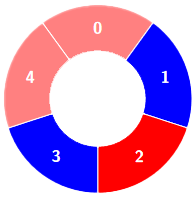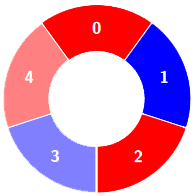\n\n**Example 2:**\n\n**Input:** colors \\= \\[0,1,0,0,1,0,1], k \\= 6\n\n**Output:** 2\n\n**Explanation:**\n\n**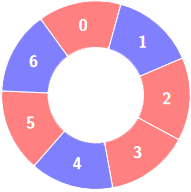**\n\nAlternating groups:\n\n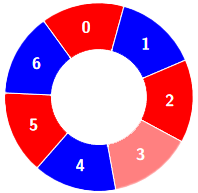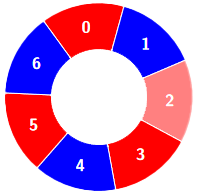\n\n**Example 3:**\n\n**Input:** colors \\= \\[1,1,0,1], k \\= 4\n\n**Output:** 0\n\n**Explanation:**\n\n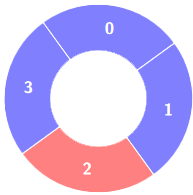\n\n**Constraints:**\n\n* `3 <= colors.length <= 105`\n* `0 <= colors[i] <= 1`\n* `3 <= k <= colors.length`", "templates": {"cpp": "class Solution {\npublic:\n int numberOfAlternatingGroups(vector<int>& colors, int k) {\n \n }\n};", "java": "class Solution {\n public int numberOfAlternatingGroups(int[] colors, int k) {\n \n }\n}", "python": "class Solution:\n def numberOfAlternatingGroups(self, colors: List[int], k: int) -> int:\n "}, "hints": "1\\. Try to find a tile that has the same color as its next tile (if it exists).\n2\\. Then try to find maximal alternating groups by starting a single for loop from that tile.", "contains_png": true, "png_links": ["https://assets.leetcode.com/uploads/2024/06/19/screenshot-2024-05-28-183519.png", "https://assets.leetcode.com/uploads/2024/05/28/screenshot-2024-05-28-182448.png", "https://assets.leetcode.com/uploads/2024/05/28/screenshot-2024-05-28-182844.png", "https://assets.leetcode.com/uploads/2024/05/28/screenshot-2024-05-28-183057.png", "https://assets.leetcode.com/uploads/2024/06/19/screenshot-2024-05-28-183907.png", "https://assets.leetcode.com/uploads/2024/06/19/screenshot-2024-05-28-184128.png", "https://assets.leetcode.com/uploads/2024/06/19/screenshot-2024-05-28-184240.png", "https://assets.leetcode.com/uploads/2024/06/19/screenshot-2024-05-28-184516.png"]}
{"number": 3209, "title": "Number of Subarrays With AND Value of K", "link": "https://leetcode.com/problems/number-of-subarrays-with-and-value-of-k/", "difficulty": "hard", "statement": "Given an array of integers `nums` and an integer `k`, return the number of subarrays of `nums` where the bitwise `AND` of the elements of the subarray equals `k`.\n\n**Example 1:**\n\n**Input:** nums \\= \\[1,1,1], k \\= 1\n\n**Output:** 6\n\n**Explanation:**\n\nAll subarrays contain only 1's.\n\n**Example 2:**\n\n**Input:** nums \\= \\[1,1,2], k \\= 1\n\n**Output:** 3\n\n**Explanation:**\n\nSubarrays having an `AND` value of 1 are: `[1,1,2]`, `[1,1,2]`, `[1,1,2]`.\n\n**Example 3:**\n\n**Input:** nums \\= \\[1,2,3], k \\= 2\n\n**Output:** 2\n\n**Explanation:**\n\nSubarrays having an `AND` value of 2 are: `[1,2,3]`, `[1,2,3]`.\n\n**Constraints:**\n\n* `1 <= nums.length <= 105`\n* `0 <= nums[i], k <= 109`", "templates": {"cpp": "class Solution {\npublic:\n long long countSubarrays(vector<int>& nums, int k) {\n \n }\n};", "java": "class Solution {\n public long countSubarrays(int[] nums, int k) {\n \n }\n}", "python": "class Solution:\n def countSubarrays(self, nums: List[int], k: int) -> int:\n "}, "hints": "1\\. Let\u2019s say we want to count the number of pairs `(l, r)` such that `nums[l] & nums[l + 1] & \u2026 & nums[r] == k`.\n2\\. Fix the left index `l`.\n3\\. Note that if you increase `r` for a fixed `l`, then the AND value of the subarray either decreases or remains unchanged.\n4\\. Therefore, consider using binary search.\n5\\. To calculate the AND value of a subarray, use sparse tables.", "contains_png": false, "png_links": []}
{"number": 3210, "title": "Find the Encrypted String", "link": "https://leetcode.com/problems/find-the-encrypted-string/", "difficulty": "easy", "statement": "You are given a string `s` and an integer `k`. Encrypt the string using the following algorithm:\n\n* For each character `c` in `s`, replace `c` with the `kth` character after `c` in the string (in a cyclic manner).\n\nReturn the *encrypted string*.\n\n**Example 1:**\n\n**Input:** s \\= \"dart\", k \\= 3\n\n**Output:** \"tdar\"\n\n**Explanation:**\n\n* For `i = 0`, the 3rd character after `'d'` is `'t'`.\n* For `i = 1`, the 3rd character after `'a'` is `'d'`.\n* For `i = 2`, the 3rd character after `'r'` is `'a'`.\n* For `i = 3`, the 3rd character after `'t'` is `'r'`.\n\n**Example 2:**\n\n**Input:** s \\= \"aaa\", k \\= 1\n\n**Output:** \"aaa\"\n\n**Explanation:**\n\nAs all the characters are the same, the encrypted string will also be the same.\n\n**Constraints:**\n\n* `1 <= s.length <= 100`\n* `1 <= k <= 104`\n* `s` consists only of lowercase English letters.", "templates": {"cpp": "class Solution {\npublic:\n string getEncryptedString(string s, int k) {\n \n }\n};", "java": "class Solution {\n public String getEncryptedString(String s, int k) {\n \n }\n}", "python": "class Solution:\n def getEncryptedString(self, s: str, k: int) -> str:\n "}, "hints": "1\\. Make a new string such that for each character in `s`, character `i` will correspond to `(i + k) % s.length` character in the original string.", "contains_png": false, "png_links": []}
{"number": 3211, "title": "Generate Binary Strings Without Adjacent Zeros", "link": "https://leetcode.com/problems/generate-binary-strings-without-adjacent-zeros/", "difficulty": "medium", "statement": "You are given a positive integer `n`.\n\nA binary string `x` is **valid** if all substrings of `x` of length 2 contain **at least** one `\"1\"`.\n\nReturn all **valid** strings with length `n`**,** in *any* order.\n\n**Example 1:**\n\n**Input:** n \\= 3\n\n**Output:** \\[\"010\",\"011\",\"101\",\"110\",\"111\"]\n\n**Explanation:**\n\nThe valid strings of length 3 are: `\"010\"`, `\"011\"`, `\"101\"`, `\"110\"`, and `\"111\"`.\n\n**Example 2:**\n\n**Input:** n \\= 1\n\n**Output:** \\[\"0\",\"1\"]\n\n**Explanation:**\n\nThe valid strings of length 1 are: `\"0\"` and `\"1\"`.\n\n**Constraints:**\n\n* `1 <= n <= 18`", "templates": {"cpp": "class Solution {\npublic:\n vector<string> validStrings(int n) {\n \n }\n};", "java": "class Solution {\n public List<String> validStrings(int n) {\n \n }\n}", "python": "class Solution:\n def validStrings(self, n: int) -> List[str]:\n "}, "hints": "1\\. If we have a string `s` of length `x`, we can generate all strings of length `x + 1`.\n2\\. If `s` has 0 as the last character, we can only append 1, whereas if the last character is 1, we can append both 0 and 1\\.\n3\\. We can use recursion and backtracking to generate all such strings.", "contains_png": false, "png_links": []}
{"number": 3212, "title": "Count Submatrices With Equal Frequency of X and Y", "link": "https://leetcode.com/problems/count-submatrices-with-equal-frequency-of-x-and-y/", "difficulty": "medium", "statement": "Given a 2D character matrix `grid`, where `grid[i][j]` is either `'X'`, `'Y'`, or `'.'`, return the number of submatrices that contain:\n\n* `grid[0][0]`\n* an **equal** frequency of `'X'` and `'Y'`.\n* **at least** one `'X'`.\n\n**Example 1:**\n\n**Input:** grid \\= \\[\\[\"X\",\"Y\",\".\"],\\[\"Y\",\".\",\".\"]]\n\n**Output:** 3\n\n**Explanation:**\n\n**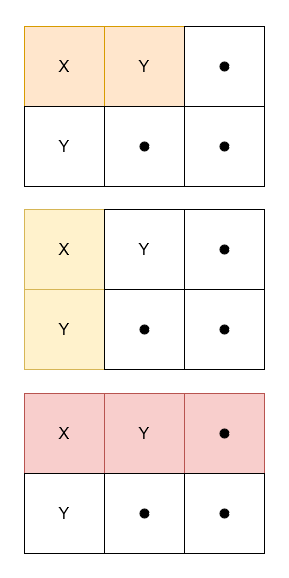**\n\n**Example 2:**\n\n**Input:** grid \\= \\[\\[\"X\",\"X\"],\\[\"X\",\"Y\"]]\n\n**Output:** 0\n\n**Explanation:**\n\nNo submatrix has an equal frequency of `'X'` and `'Y'`.\n\n**Example 3:**\n\n**Input:** grid \\= \\[\\[\".\",\".\"],\\[\".\",\".\"]]\n\n**Output:** 0\n\n**Explanation:**\n\nNo submatrix has at least one `'X'`.\n\n**Constraints:**\n\n* `1 <= grid.length, grid[i].length <= 1000`\n* `grid[i][j]` is either `'X'`, `'Y'`, or `'.'`.", "templates": {"cpp": "class Solution {\npublic:\n int numberOfSubmatrices(vector<vector<char>>& grid) {\n \n }\n};", "java": "class Solution {\n public int numberOfSubmatrices(char[][] grid) {\n \n }\n}", "python": "class Solution:\n def numberOfSubmatrices(self, grid: List[List[str]]) -> int:\n "}, "hints": "1\\. Replace `\u2019X\u2019` with 1, `\u2019Y\u2019` with \\-1 and `\u2019.\u2019` with 0\\.\n2\\. You need to find how many submatrices `grid[0..x][0..y]` have a sum of 0 and at least one `\u2019X\u2019`.\n3\\. Use prefix sum to calculate submatrices sum.", "contains_png": true, "png_links": ["https://assets.leetcode.com/uploads/2024/06/07/examplems.png"]}
{"number": 3213, "title": "Construct String with Minimum Cost", "link": "https://leetcode.com/problems/construct-string-with-minimum-cost/", "difficulty": "hard", "statement": "You are given a string `target`, an array of strings `words`, and an integer array `costs`, both arrays of the same length.\n\nImagine an empty string `s`.\n\nYou can perform the following operation any number of times (including **zero**):\n\n* Choose an index `i` in the range `[0, words.length - 1]`.\n* Append `words[i]` to `s`.\n* The cost of operation is `costs[i]`.\n\nReturn the **minimum** cost to make `s` equal to `target`. If it's not possible, return `-1`.\n\n**Example 1:**\n\n**Input:** target \\= \"abcdef\", words \\= \\[\"abdef\",\"abc\",\"d\",\"def\",\"ef\"], costs \\= \\[100,1,1,10,5]\n\n**Output:** 7\n\n**Explanation:**\n\nThe minimum cost can be achieved by performing the following operations:\n\n* Select index 1 and append `\"abc\"` to `s` at a cost of 1, resulting in `s = \"abc\"`.\n* Select index 2 and append `\"d\"` to `s` at a cost of 1, resulting in `s = \"abcd\"`.\n* Select index 4 and append `\"ef\"` to `s` at a cost of 5, resulting in `s = \"abcdef\"`.\n\n**Example 2:**\n\n**Input:** target \\= \"aaaa\", words \\= \\[\"z\",\"zz\",\"zzz\"], costs \\= \\[1,10,100]\n\n**Output:** \\-1\n\n**Explanation:**\n\nIt is impossible to make `s` equal to `target`, so we return \\-1\\.\n\n**Constraints:**\n\n* `1 <= target.length <= 5 * 104`\n* `1 <= words.length == costs.length <= 5 * 104`\n* `1 <= words[i].length <= target.length`\n* The total sum of `words[i].length` is less than or equal to `5 * 104`.\n* `target` and `words[i]` consist only of lowercase English letters.\n* `1 <= costs[i] <= 104`", "templates": {"cpp": "class Solution {\npublic:\n int minimumCost(string target, vector<string>& words, vector<int>& costs) {\n \n }\n};", "java": "class Solution {\n public int minimumCost(String target, String[] words, int[] costs) {\n \n }\n}", "python": "class Solution:\n def minimumCost(self, target: str, words: List[str], costs: List[int]) -> int:\n "}, "hints": "1\\. Use Dynamic Programming along with Aho\\-Corasick or Hashing.", "contains_png": false, "png_links": []}
{"number": 3216, "title": "Lexicographically Smallest String After a Swap", "link": "https://leetcode.com/problems/lexicographically-smallest-string-after-a-swap/", "difficulty": "easy", "statement": "Given a string `s` containing only digits, return the lexicographically smallest string that can be obtained after swapping **adjacent** digits in `s` with the same **parity** at most **once**.\n\nDigits have the same parity if both are odd or both are even. For example, 5 and 9, as well as 2 and 4, have the same parity, while 6 and 9 do not.\n\n**Example 1:**\n\n**Input:** s \\= \"45320\"\n\n**Output:** \"43520\"\n\n**Explanation:** \n\n`s[1] == '5'` and `s[2] == '3'` both have the same parity, and swapping them results in the lexicographically smallest string.\n\n**Example 2:**\n\n**Input:** s \\= \"001\"\n\n**Output:** \"001\"\n\n**Explanation:**\n\nThere is no need to perform a swap because `s` is already the lexicographically smallest.\n\n**Constraints:**\n\n* `2 <= s.length <= 100`\n* `s` consists only of digits.", "templates": {"cpp": "class Solution {\npublic:\n string getSmallestString(string s) {\n \n }\n};", "java": "class Solution {\n public String getSmallestString(String s) {\n \n }\n}", "python": "class Solution:\n def getSmallestString(self, s: str) -> str:\n "}, "hints": "1\\. Try all possible swaps satisfying the constraints and find the one that results in the lexicographically smallest string.", "contains_png": false, "png_links": []}
{"number": 3217, "title": "Delete Nodes From Linked List Present in Array", "link": "https://leetcode.com/problems/delete-nodes-from-linked-list-present-in-array/", "difficulty": "medium", "statement": "You are given an array of integers `nums` and the `head` of a linked list. Return the `head` of the modified linked list after **removing** all nodes from the linked list that have a value that exists in `nums`.\n\n**Example 1:**\n\n**Input:** nums \\= \\[1,2,3], head \\= \\[1,2,3,4,5]\n\n**Output:** \\[4,5]\n\n**Explanation:**\n\n****\n\nRemove the nodes with values 1, 2, and 3\\.\n\n**Example 2:**\n\n**Input:** nums \\= \\[1], head \\= \\[1,2,1,2,1,2]\n\n**Output:** \\[2,2,2]\n\n**Explanation:**\n\n\n\nRemove the nodes with value 1\\.\n\n**Example 3:**\n\n**Input:** nums \\= \\[5], head \\= \\[1,2,3,4]\n\n**Output:** \\[1,2,3,4]\n\n**Explanation:**\n\n**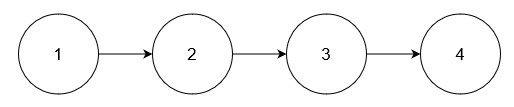**\n\nNo node has value 5\\.\n\n**Constraints:**\n\n* `1 <= nums.length <= 105`\n* `1 <= nums[i] <= 105`\n* All elements in `nums` are unique.\n* The number of nodes in the given list is in the range `[1, 105]`.\n* `1 <= Node.val <= 105`\n* The input is generated such that there is at least one node in the linked list that has a value not present in `nums`.", "templates": {"cpp": "/**\n * Definition for singly-linked list.\n * struct ListNode {\n * int val;\n * ListNode *next;\n * ListNode() : val(0), next(nullptr) {}\n * ListNode(int x) : val(x), next(nullptr) {}\n * ListNode(int x, ListNode *next) : val(x), next(next) {}\n * };\n */\nclass Solution {\npublic:\n ListNode* modifiedList(vector<int>& nums, ListNode* head) {\n \n }\n};", "java": "/**\n * Definition for singly-linked list.\n * public class ListNode {\n * int val;\n * ListNode next;\n * ListNode() {}\n * ListNode(int val) { this.val = val; }\n * ListNode(int val, ListNode next) { this.val = val; this.next = next; }\n * }\n */\nclass Solution {\n public ListNode modifiedList(int[] nums, ListNode head) {\n \n }\n}", "python": "# Definition for singly-linked list.\n# class ListNode:\n# def __init__(self, val=0, next=None):\n# self.val = val\n# self.next = next\nclass Solution:\n def modifiedList(self, nums: List[int], head: Optional[ListNode]) -> Optional[ListNode]:\n "}, "hints": "1\\. Add all elements of `nums` into a Set.\n2\\. Scan the list to check if the current element should be deleted by checking the Set.", "contains_png": true, "png_links": ["https://assets.leetcode.com/uploads/2024/06/11/linkedlistexample0.png", "https://assets.leetcode.com/uploads/2024/06/11/linkedlistexample1.png", "https://assets.leetcode.com/uploads/2024/06/11/linkedlistexample2.png"]}
{"number": 3218, "title": "Minimum Cost for Cutting Cake I", "link": "https://leetcode.com/problems/minimum-cost-for-cutting-cake-i/", "difficulty": "medium", "statement": "There is an `m x n` cake that needs to be cut into `1 x 1` pieces.\n\nYou are given integers `m`, `n`, and two arrays:\n\n* `horizontalCut` of size `m - 1`, where `horizontalCut[i]` represents the cost to cut along the horizontal line `i`.\n* `verticalCut` of size `n - 1`, where `verticalCut[j]` represents the cost to cut along the vertical line `j`.\n\nIn one operation, you can choose any piece of cake that is not yet a `1 x 1` square and perform one of the following cuts:\n\n1. Cut along a horizontal line `i` at a cost of `horizontalCut[i]`.\n2. Cut along a vertical line `j` at a cost of `verticalCut[j]`.\n\nAfter the cut, the piece of cake is divided into two distinct pieces.\n\nThe cost of a cut depends only on the initial cost of the line and does not change.\n\nReturn the **minimum** total cost to cut the entire cake into `1 x 1` pieces.\n\n**Example 1:**\n\n**Input:** m \\= 3, n \\= 2, horizontalCut \\= \\[1,3], verticalCut \\= \\[5]\n\n**Output:** 13\n\n**Explanation:**\n\n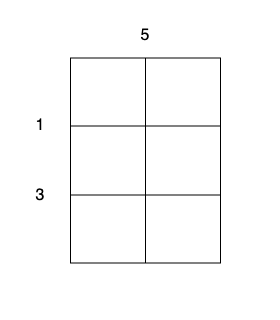\n\n* Perform a cut on the vertical line 0 with cost 5, current total cost is 5\\.\n* Perform a cut on the horizontal line 0 on `3 x 1` subgrid with cost 1\\.\n* Perform a cut on the horizontal line 0 on `3 x 1` subgrid with cost 1\\.\n* Perform a cut on the horizontal line 1 on `2 x 1` subgrid with cost 3\\.\n* Perform a cut on the horizontal line 1 on `2 x 1` subgrid with cost 3\\.\n\nThe total cost is `5 + 1 + 1 + 3 + 3 = 13`.\n\n**Example 2:**\n\n**Input:** m \\= 2, n \\= 2, horizontalCut \\= \\[7], verticalCut \\= \\[4]\n\n**Output:** 15\n\n**Explanation:**\n\n* Perform a cut on the horizontal line 0 with cost 7\\.\n* Perform a cut on the vertical line 0 on `1 x 2` subgrid with cost 4\\.\n* Perform a cut on the vertical line 0 on `1 x 2` subgrid with cost 4\\.\n\nThe total cost is `7 + 4 + 4 = 15`.\n\n**Constraints:**\n\n* `1 <= m, n <= 20`\n* `horizontalCut.length == m - 1`\n* `verticalCut.length == n - 1`\n* `1 <= horizontalCut[i], verticalCut[i] <= 103`", "templates": {"cpp": "class Solution {\npublic:\n int minimumCost(int m, int n, vector<int>& horizontalCut, vector<int>& verticalCut) {\n \n }\n};", "java": "class Solution {\n public int minimumCost(int m, int n, int[] horizontalCut, int[] verticalCut) {\n \n }\n}", "python": "class Solution:\n def minimumCost(self, m: int, n: int, horizontalCut: List[int], verticalCut: List[int]) -> int:\n "}, "hints": "1\\. The intended solution uses Dynamic Programming.\n2\\. Let `dp[sx][sy][tx][ty]` denote the minimum cost to cut the rectangle into `1 x 1` pieces.\n3\\. Iterate on the row or column on which you will perform the next cut, after the cut, the current rectangle will be decomposed into two sub\\-rectangles.", "contains_png": false, "png_links": []}
{"number": 3219, "title": "Minimum Cost for Cutting Cake II", "link": "https://leetcode.com/problems/minimum-cost-for-cutting-cake-ii/", "difficulty": "hard", "statement": "There is an `m x n` cake that needs to be cut into `1 x 1` pieces.\n\nYou are given integers `m`, `n`, and two arrays:\n\n* `horizontalCut` of size `m - 1`, where `horizontalCut[i]` represents the cost to cut along the horizontal line `i`.\n* `verticalCut` of size `n - 1`, where `verticalCut[j]` represents the cost to cut along the vertical line `j`.\n\nIn one operation, you can choose any piece of cake that is not yet a `1 x 1` square and perform one of the following cuts:\n\n1. Cut along a horizontal line `i` at a cost of `horizontalCut[i]`.\n2. Cut along a vertical line `j` at a cost of `verticalCut[j]`.\n\nAfter the cut, the piece of cake is divided into two distinct pieces.\n\nThe cost of a cut depends only on the initial cost of the line and does not change.\n\nReturn the **minimum** total cost to cut the entire cake into `1 x 1` pieces.\n\n**Example 1:**\n\n**Input:** m \\= 3, n \\= 2, horizontalCut \\= \\[1,3], verticalCut \\= \\[5]\n\n**Output:** 13\n\n**Explanation:**\n\n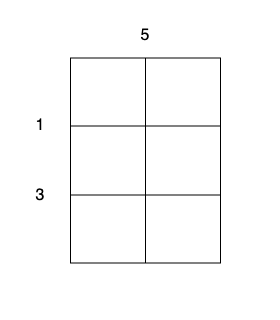\n\n* Perform a cut on the vertical line 0 with cost 5, current total cost is 5\\.\n* Perform a cut on the horizontal line 0 on `3 x 1` subgrid with cost 1\\.\n* Perform a cut on the horizontal line 0 on `3 x 1` subgrid with cost 1\\.\n* Perform a cut on the horizontal line 1 on `2 x 1` subgrid with cost 3\\.\n* Perform a cut on the horizontal line 1 on `2 x 1` subgrid with cost 3\\.\n\nThe total cost is `5 + 1 + 1 + 3 + 3 = 13`.\n\n**Example 2:**\n\n**Input:** m \\= 2, n \\= 2, horizontalCut \\= \\[7], verticalCut \\= \\[4]\n\n**Output:** 15\n\n**Explanation:**\n\n* Perform a cut on the horizontal line 0 with cost 7\\.\n* Perform a cut on the vertical line 0 on `1 x 2` subgrid with cost 4\\.\n* Perform a cut on the vertical line 0 on `1 x 2` subgrid with cost 4\\.\n\nThe total cost is `7 + 4 + 4 = 15`.\n\n**Constraints:**\n\n* `1 <= m, n <= 105`\n* `horizontalCut.length == m - 1`\n* `verticalCut.length == n - 1`\n* `1 <= horizontalCut[i], verticalCut[i] <= 103`", "templates": {"cpp": "class Solution {\npublic:\n long long minimumCost(int m, int n, vector<int>& horizontalCut, vector<int>& verticalCut) {\n \n }\n};", "java": "class Solution {\n public long minimumCost(int m, int n, int[] horizontalCut, int[] verticalCut) {\n \n }\n}", "python": "class Solution:\n def minimumCost(self, m: int, n: int, horizontalCut: List[int], verticalCut: List[int]) -> int:\n "}, "hints": "1\\. The intended solution uses a Greedy approach.\n2\\. At each step, we will perform a cut on the line with the highest cost.\n3\\. If you perform a horizontal cut, can you count the contribution that it adds to each row cut that comes afterward?", "contains_png": false, "png_links": []}
{"number": 3222, "title": "Find the Winning Player in Coin Game", "link": "https://leetcode.com/problems/find-the-winning-player-in-coin-game/", "difficulty": "easy", "statement": "You are given two **positive** integers `x` and `y`, denoting the number of coins with values 75 and 10 *respectively*.\n\nAlice and Bob are playing a game. Each turn, starting with **Alice**, the player must pick up coins with a **total** value 115\\. If the player is unable to do so, they **lose** the game.\n\nReturn the *name* of the player who wins the game if both players play **optimally**.\n\n**Example 1:**\n\n**Input:** x \\= 2, y \\= 7\n\n**Output:** \"Alice\"\n\n**Explanation:**\n\nThe game ends in a single turn:\n\n* Alice picks 1 coin with a value of 75 and 4 coins with a value of 10\\.\n\n**Example 2:**\n\n**Input:** x \\= 4, y \\= 11\n\n**Output:** \"Bob\"\n\n**Explanation:**\n\nThe game ends in 2 turns:\n\n* Alice picks 1 coin with a value of 75 and 4 coins with a value of 10\\.\n* Bob picks 1 coin with a value of 75 and 4 coins with a value of 10\\.\n\n**Constraints:**\n\n* `1 <= x, y <= 100`", "templates": {"cpp": "class Solution {\npublic:\n string losingPlayer(int x, int y) {\n \n }\n};", "java": "class Solution {\n public String losingPlayer(int x, int y) {\n \n }\n}", "python": "class Solution:\n def losingPlayer(self, x: int, y: int) -> str:\n "}, "hints": "1\\. The only way to make 115 is to use one coin of value 75 and four coins of value 10\\. Each turn uses up these many coins.\n2\\. Hence the number of turns is `min(x, y / 4)`.\n3\\. Determine the winner from its parity.", "contains_png": false, "png_links": []}
{"number": 3223, "title": "Minimum Length of String After Operations", "link": "https://leetcode.com/problems/minimum-length-of-string-after-operations/", "difficulty": "medium", "statement": "You are given a string `s`.\n\nYou can perform the following process on `s` **any** number of times:\n\n* Choose an index `i` in the string such that there is **at least** one character to the left of index `i` that is equal to `s[i]`, and **at least** one character to the right that is also equal to `s[i]`.\n* Delete the **closest** character to the **left** of index `i` that is equal to `s[i]`.\n* Delete the **closest** character to the **right** of index `i` that is equal to `s[i]`.\n\nReturn the **minimum** length of the final string `s` that you can achieve.\n\n**Example 1:**\n\n**Input:** s \\= \"abaacbcbb\"\n\n**Output:** 5\n\n**Explanation:** \n\nWe do the following operations:\n\n* Choose index 2, then remove the characters at indices 0 and 3\\. The resulting string is `s = \"bacbcbb\"`.\n* Choose index 3, then remove the characters at indices 0 and 5\\. The resulting string is `s = \"acbcb\"`.\n\n**Example 2:**\n\n**Input:** s \\= \"aa\"\n\n**Output:** 2\n\n**Explanation:** \n\nWe cannot perform any operations, so we return the length of the original string.\n\n**Constraints:**\n\n* `1 <= s.length <= 2 * 105`\n* `s` consists only of lowercase English letters.", "templates": {"cpp": "class Solution {\npublic:\n int minimumLength(string s) {\n \n }\n};", "java": "class Solution {\n public int minimumLength(String s) {\n \n }\n}", "python": "class Solution:\n def minimumLength(self, s: str) -> int:\n "}, "hints": "1\\. Only the frequency of each character matters in finding the final answer.\n2\\. If a character occurs less than 3 times, we cannot perform any process with it.\n3\\. Suppose there is a character that occurs at least 3 times in the string, we can repeatedly delete two of these characters until there are at most 2 occurrences left of it.", "contains_png": false, "png_links": []}
{"number": 3224, "title": "Minimum Array Changes to Make Differences Equal", "link": "https://leetcode.com/problems/minimum-array-changes-to-make-differences-equal/", "difficulty": "medium", "statement": "You are given an integer array `nums` of size `n` where `n` is **even**, and an integer `k`.\n\nYou can perform some changes on the array, where in one change you can replace **any** element in the array with **any** integer in the range from `0` to `k`.\n\nYou need to perform some changes (possibly none) such that the final array satisfies the following condition:\n\n* There exists an integer `X` such that `abs(a[i] - a[n - i - 1]) = X` for all `(0 <= i < n)`.\n\nReturn the **minimum** number of changes required to satisfy the above condition.\n\n**Example 1:**\n\n**Input:** nums \\= \\[1,0,1,2,4,3], k \\= 4\n\n**Output:** 2\n\n**Explanation:** \n\nWe can perform the following changes:\n\n* Replace `nums[1]` by 2\\. The resulting array is `nums = [1,2,1,2,4,3]`.\n* Replace `nums[3]` by 3\\. The resulting array is `nums = [1,2,1,3,4,3]`.\n\nThe integer `X` will be 2\\.\n\n**Example 2:**\n\n**Input:** nums \\= \\[0,1,2,3,3,6,5,4], k \\= 6\n\n**Output:** 2\n\n**Explanation:** \n\nWe can perform the following operations:\n\n* Replace `nums[3]` by 0\\. The resulting array is `nums = [0,1,2,0,3,6,5,4]`.\n* Replace `nums[4]` by 4\\. The resulting array is `nums = [0,1,2,0,4,6,5,4]`.\n\nThe integer `X` will be 4\\.\n\n**Constraints:**\n\n* `2 <= n == nums.length <= 105`\n* `n` is even.\n* `0 <= nums[i] <= k <= 105`", "templates": {"cpp": "class Solution {\npublic:\n int minChanges(vector<int>& nums, int k) {\n \n }\n};", "java": "class Solution {\n public int minChanges(int[] nums, int k) {\n \n }\n}", "python": "class Solution:\n def minChanges(self, nums: List[int], k: int) -> int:\n "}, "hints": "1\\. There are at most `k + 1` possible values of the integer `X`.\n2\\. How do we calculate the minimum number of changes efficiently if we fix the value of `X` before applying any changes?", "contains_png": false, "png_links": []}
{"number": 3225, "title": "Maximum Score From Grid Operations", "link": "https://leetcode.com/problems/maximum-score-from-grid-operations/", "difficulty": "hard", "statement": "You are given a 2D matrix `grid` of size `n x n`. Initially, all cells of the grid are colored white. In one operation, you can select any cell of indices `(i, j)`, and color black all the cells of the `jth` column starting from the top row down to the `ith` row.\n\nThe grid score is the sum of all `grid[i][j]` such that cell `(i, j)` is white and it has a horizontally adjacent black cell.\n\nReturn the **maximum** score that can be achieved after some number of operations.\n\n**Example 1:**\n\n**Input:** grid \\= \\[\\[0,0,0,0,0],\\[0,0,3,0,0],\\[0,1,0,0,0],\\[5,0,0,3,0],\\[0,0,0,0,2]]\n\n**Output:** 11\n\n**Explanation:**\n\n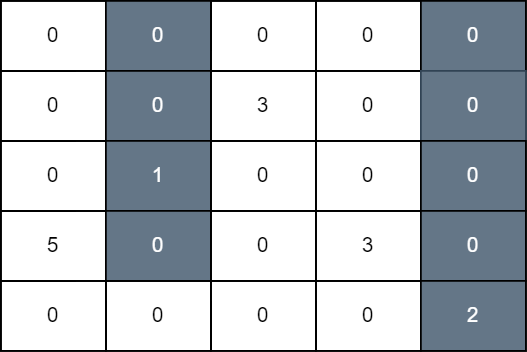\nIn the first operation, we color all cells in column 1 down to row 3, and in the second operation, we color all cells in column 4 down to the last row. The score of the resulting grid is `grid[3][0] + grid[1][2] + grid[3][3]` which is equal to 11\\.\n\n**Example 2:**\n\n**Input:** grid \\= \\[\\[10,9,0,0,15],\\[7,1,0,8,0],\\[5,20,0,11,0],\\[0,0,0,1,2],\\[8,12,1,10,3]]\n\n**Output:** 94\n\n**Explanation:**\n\n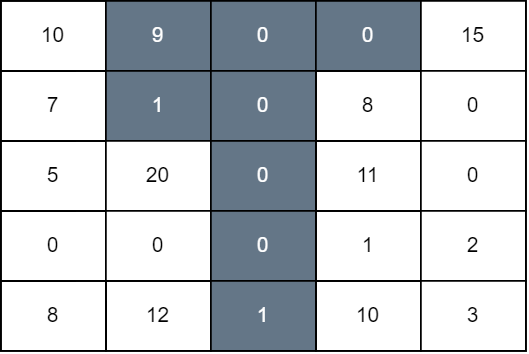\nWe perform operations on 1, 2, and 3 down to rows 1, 4, and 0, respectively. The score of the resulting grid is `grid[0][0] + grid[1][0] + grid[2][1] + grid[4][1] + grid[1][3] + grid[2][3] + grid[3][3] + grid[4][3] + grid[0][4]` which is equal to 94\\.\n\n**Constraints:**\n\n* `1 <=\u00a0n == grid.length <= 100`\n* `n == grid[i].length`\n* `0 <= grid[i][j] <= 109`", "templates": {"cpp": "class Solution {\npublic:\n long long maximumScore(vector<vector<int>>& grid) {\n \n }\n};", "java": "class Solution {\n public long maximumScore(int[][] grid) {\n \n }\n}", "python": "class Solution:\n def maximumScore(self, grid: List[List[int]]) -> int:\n "}, "hints": "1\\. Use dynamic programming.\n2\\. Solve the problem in O(N^4\\) using a 3\\-states dp.\n3\\. Let `dp[i][lastHeight][beforeLastHeight]` denote the maximum score if the grid was limited to column `i`, and the height of column `i - 1` is `lastHeight` and the height of column `i - 2` is `beforeLastHeight`.\n4\\. The third state, `beforeLastHeight`, is used to determine which values of column `i - 1` will be added to the score. We can replace this state with another state that only takes two values 0 or 1\\.\n5\\. Let `dp[i][lastHeight][isBigger]` denote the maximum score if the grid was limited to column `i`, and where the height of column `i - 1` is `lastHeight`. Additionally, if `isBigger == 1`, the number of black cells in column `i` is assumed to be larger than the number of black cells in column `i - 2`, and vice versa. Note that if our assumption is wrong, it would lead to a suboptimal score and, therefore, it would not be considered as the final answer.", "contains_png": true, "png_links": ["https://assets.leetcode.com/uploads/2024/05/11/one.png", "https://assets.leetcode.com/uploads/2024/05/11/two-1.png"]}
{"number": 3226, "title": "Number of Bit Changes to Make Two Integers Equal", "link": "https://leetcode.com/problems/number-of-bit-changes-to-make-two-integers-equal/", "difficulty": "easy", "statement": "You are given two positive integers `n` and `k`.\n\nYou can choose **any** bit in the **binary representation** of `n` that is equal to 1 and change it to 0\\.\n\nReturn the *number of changes* needed to make `n` equal to `k`. If it is impossible, return \\-1\\.\n\n**Example 1:**\n\n**Input:** n \\= 13, k \\= 4\n\n**Output:** 2\n\n**Explanation:** \n\nInitially, the binary representations of `n` and `k` are `n = (1101)2` and `k = (0100)2`. \n\nWe can change the first and fourth bits of `n`. The resulting integer is `n = (0100)2 = k`.\n\n**Example 2:**\n\n**Input:** n \\= 21, k \\= 21\n\n**Output:** 0\n\n**Explanation:** \n\n`n` and `k` are already equal, so no changes are needed.\n\n**Example 3:**\n\n**Input:** n \\= 14, k \\= 13\n\n**Output:** \\-1\n\n**Explanation:** \n\nIt is not possible to make `n` equal to `k`.\n\n**Constraints:**\n\n* `1 <= n, k <= 106`", "templates": {"cpp": "class Solution {\npublic:\n int minChanges(int n, int k) {\n \n }\n};", "java": "class Solution {\n public int minChanges(int n, int k) {\n \n }\n}", "python": "class Solution:\n def minChanges(self, n: int, k: int) -> int:\n "}, "hints": "1\\. Find the binary representations of `n` and `k`.\n2\\. Any bit that is equal to 1 in `n` and equal to 0 in `k` needs to be changed.", "contains_png": false, "png_links": []}
{"number": 3227, "title": "Vowels Game in a String", "link": "https://leetcode.com/problems/vowels-game-in-a-string/", "difficulty": "medium", "statement": "Alice and Bob are playing a game on a string.\n\nYou are given a string `s`, Alice and Bob will take turns playing the following game where Alice starts **first**:\n\n* On Alice's turn, she has to remove any **non\\-empty** substring from `s` that contains an **odd** number of vowels.\n* On Bob's turn, he has to remove any **non\\-empty** substring from `s` that contains an **even** number of vowels.\n\nThe first player who cannot make a move on their turn loses the game. We assume that both Alice and Bob play **optimally**.\n\nReturn `true` if Alice wins the game, and `false` otherwise.\n\nThe English vowels are: `a`, `e`, `i`, `o`, and `u`.\n\n**Example 1:**\n\n**Input:** s \\= \"leetcoder\"\n\n**Output:** true\n\n**Explanation:** \n\nAlice can win the game as follows:\n\n* Alice plays first, she can delete the underlined substring in `s = \"leetcoder\"` which contains 3 vowels. The resulting string is `s = \"der\"`.\n* Bob plays second, he can delete the underlined substring in `s = \"der\"` which contains 0 vowels. The resulting string is `s = \"er\"`.\n* Alice plays third, she can delete the whole string `s = \"er\"` which contains 1 vowel.\n* Bob plays fourth, since the string is empty, there is no valid play for Bob. So Alice wins the game.\n\n**Example 2:**\n\n**Input:** s \\= \"bbcd\"\n\n**Output:** false\n\n**Explanation:** \n\nThere is no valid play for Alice in her first turn, so Alice loses the game.\n\n**Constraints:**\n\n* `1 <= s.length <= 105`\n* `s` consists only of lowercase English letters.", "templates": {"cpp": "class Solution {\npublic:\n bool doesAliceWin(string s) {\n \n }\n};", "java": "class Solution {\n public boolean doesAliceWin(String s) {\n \n }\n}", "python": "class Solution:\n def doesAliceWin(self, s: str) -> bool:\n "}, "hints": "1\\. If there are no vowels in the initial string, then Bob wins.\n2\\. If the number of vowels in the initial string is odd, then Alice can remove the whole string on her first turn and win.\n3\\. What if the number of vowels in the initial string is even? What\u2019s the optimal play for Alice\u2019s first turn?", "contains_png": false, "png_links": []}
{"number": 3228, "title": "Maximum Number of Operations to Move Ones to the End", "link": "https://leetcode.com/problems/maximum-number-of-operations-to-move-ones-to-the-end/", "difficulty": "medium", "statement": "You are given a binary string `s`.\n\nYou can perform the following operation on the string **any** number of times:\n\n* Choose **any** index `i` from the string where `i + 1 < s.length` such that `s[i] == '1'` and `s[i + 1] == '0'`.\n* Move the character `s[i]` to the **right** until it reaches the end of the string or another `'1'`. For example, for `s = \"010010\"`, if we choose `i = 1`, the resulting string will be `s = \"000110\"`.\n\nReturn the **maximum** number of operations that you can perform.\n\n**Example 1:**\n\n**Input:** s \\= \"1001101\"\n\n**Output:** 4\n\n**Explanation:**\n\nWe can perform the following operations:\n\n* Choose index `i = 0`. The resulting string is `s = \"0011101\"`.\n* Choose index `i = 4`. The resulting string is `s = \"0011011\"`.\n* Choose index `i = 3`. The resulting string is `s = \"0010111\"`.\n* Choose index `i = 2`. The resulting string is `s = \"0001111\"`.\n\n**Example 2:**\n\n**Input:** s \\= \"00111\"\n\n**Output:** 0\n\n**Constraints:**\n\n* `1 <= s.length <= 105`\n* `s[i]` is either `'0'` or `'1'`.", "templates": {"cpp": "class Solution {\npublic:\n int maxOperations(string s) {\n \n }\n};", "java": "class Solution {\n public int maxOperations(String s) {\n \n }\n}", "python": "class Solution:\n def maxOperations(self, s: str) -> int:\n "}, "hints": "1\\. It is optimal to perform the operation on the lowest index possible each time.\n2\\. Traverse the string from left to right and perform the operation every time it is possible.", "contains_png": false, "png_links": []}
{"number": 3229, "title": "Minimum Operations to Make Array Equal to Target", "link": "https://leetcode.com/problems/minimum-operations-to-make-array-equal-to-target/", "difficulty": "hard", "statement": "You are given two positive integer arrays `nums` and `target`, of the same length.\n\nIn a single operation, you can select any subarray of `nums` and increment each element within that subarray by 1 or decrement each element within that subarray by 1\\.\n\nReturn the **minimum** number of operations required to make `nums` equal to the array `target`.\n\n**Example 1:**\n\n**Input:** nums \\= \\[3,5,1,2], target \\= \\[4,6,2,4]\n\n**Output:** 2\n\n**Explanation:**\n\nWe will perform the following operations to make `nums` equal to `target`: \n\n\\- Increment\u00a0`nums[0..3]` by 1, `nums = [4,6,2,3]`. \n\n\\- Increment\u00a0`nums[3..3]` by 1, `nums = [4,6,2,4]`.\n\n**Example 2:**\n\n**Input:** nums \\= \\[1,3,2], target \\= \\[2,1,4]\n\n**Output:** 5\n\n**Explanation:**\n\nWe will perform the following operations to make `nums` equal to `target`: \n\n\\- Increment\u00a0`nums[0..0]` by 1, `nums = [2,3,2]`. \n\n\\- Decrement\u00a0`nums[1..1]` by 1, `nums = [2,2,2]`. \n\n\\- Decrement\u00a0`nums[1..1]` by 1, `nums = [2,1,2]`. \n\n\\- Increment\u00a0`nums[2..2]` by 1, `nums = [2,1,3]`. \n\n\\- Increment\u00a0`nums[2..2]` by 1, `nums = [2,1,4]`.\n\n**Constraints:**\n\n* `1 <= nums.length == target.length <= 105`\n* `1 <= nums[i], target[i] <= 108`", "templates": {"cpp": "class Solution {\npublic:\n long long minimumOperations(vector<int>& nums, vector<int>& target) {\n \n }\n};", "java": "class Solution {\n public long minimumOperations(int[] nums, int[] target) {\n \n }\n}", "python": "class Solution:\n def minimumOperations(self, nums: List[int], target: List[int]) -> int:\n "}, "hints": "1\\. Change `nums'[i] = nums[i] - target[i]`, so our goal is to make `nums'` into all 0s.\n2\\. Divide and conquer.", "contains_png": false, "png_links": []}
{"number": 3232, "title": "Find if Digit Game Can Be Won", "link": "https://leetcode.com/problems/find-if-digit-game-can-be-won/", "difficulty": "easy", "statement": "You are given an array of **positive** integers `nums`.\n\nAlice and Bob are playing a game. In the game, Alice can choose **either** all single\\-digit numbers or all double\\-digit numbers from `nums`, and the rest of the numbers are given to Bob. Alice wins if the sum of her numbers is **strictly greater** than the sum of Bob's numbers.\n\nReturn `true` if Alice can win this game, otherwise, return `false`.\n\n**Example 1:**\n\n**Input:** nums \\= \\[1,2,3,4,10]\n\n**Output:** false\n\n**Explanation:**\n\nAlice cannot win by choosing either single\\-digit or double\\-digit numbers.\n\n**Example 2:**\n\n**Input:** nums \\= \\[1,2,3,4,5,14]\n\n**Output:** true\n\n**Explanation:**\n\nAlice can win by choosing single\\-digit numbers which have a sum equal to 15\\.\n\n**Example 3:**\n\n**Input:** nums \\= \\[5,5,5,25]\n\n**Output:** true\n\n**Explanation:**\n\nAlice can win by choosing double\\-digit numbers which have a sum equal to 25\\.\n\n**Constraints:**\n\n* `1 <= nums.length <= 100`\n* `1 <= nums[i] <= 99`", "templates": {"cpp": "class Solution {\npublic:\n bool canAliceWin(vector<int>& nums) {\n \n }\n};", "java": "class Solution {\n public boolean canAliceWin(int[] nums) {\n \n }\n}", "python": "class Solution:\n def canAliceWin(self, nums: List[int]) -> bool:\n "}, "hints": "1\\. Alice wins if the sum of all single\\-digit numbers and the sum of all double\\-digit numbers are different.", "contains_png": false, "png_links": []}
{"number": 3233, "title": "Find the Count of Numbers Which Are Not Special", "link": "https://leetcode.com/problems/find-the-count-of-numbers-which-are-not-special/", "difficulty": "medium", "statement": "You are given 2 **positive** integers `l` and `r`. For any number `x`, all positive divisors of `x` *except* `x` are called the **proper divisors** of `x`.\n\nA number is called **special** if it has exactly 2 **proper divisors**. For example:\n\n* The number 4 is *special* because it has proper divisors 1 and 2\\.\n* The number 6 is *not special* because it has proper divisors 1, 2, and 3\\.\n\nReturn the count of numbers in the range `[l, r]` that are **not** **special**.\n\n**Example 1:**\n\n**Input:** l \\= 5, r \\= 7\n\n**Output:** 3\n\n**Explanation:**\n\nThere are no special numbers in the range `[5, 7]`.\n\n**Example 2:**\n\n**Input:** l \\= 4, r \\= 16\n\n**Output:** 11\n\n**Explanation:**\n\nThe special numbers in the range `[4, 16]` are 4 and 9\\.\n\n**Constraints:**\n\n* `1 <= l <= r <= 109`", "templates": {"cpp": "class Solution {\npublic:\n int nonSpecialCount(int l, int r) {\n \n }\n};", "java": "class Solution {\n public int nonSpecialCount(int l, int r) {\n \n }\n}", "python": "class Solution:\n def nonSpecialCount(self, l: int, r: int) -> int:\n "}, "hints": "1\\. A special number must be a square of a prime number.\n2\\. We need to find all primes in the range `[sqrt(l), sqrt(r)]`.\n3\\. Use sieve to find primes till `sqrt(109)`.", "contains_png": false, "png_links": []}
{"number": 3234, "title": "Count the Number of Substrings With Dominant Ones", "link": "https://leetcode.com/problems/count-the-number-of-substrings-with-dominant-ones/", "difficulty": "medium", "statement": "You are given a binary string `s`.\n\nReturn the number of substrings with **dominant** ones.\n\nA string has **dominant** ones if the number of ones in the string is **greater than or equal to** the **square** of the number of zeros in the string.\n\n**Example 1:**\n\n**Input:** s \\= \"00011\"\n\n**Output:** 5\n\n**Explanation:**\n\nThe substrings with dominant ones are shown in the table below.\n\n| i | j | s\\[i..j] | Number of Zeros | Number of Ones |\n| --- | --- | --- | --- | --- |\n| 3 | 3 | 1 | 0 | 1 |\n| 4 | 4 | 1 | 0 | 1 |\n| 2 | 3 | 01 | 1 | 1 |\n| 3 | 4 | 11 | 0 | 2 |\n| 2 | 4 | 011 | 1 | 2 |\n\n**Example 2:**\n\n**Input:** s \\= \"101101\"\n\n**Output:** 16\n\n**Explanation:**\n\nThe substrings with **non\\-dominant** ones are shown in the table below.\n\nSince there are 21 substrings total and 5 of them have non\\-dominant ones, it follows that there are 16 substrings with dominant ones.\n\n| i | j | s\\[i..j] | Number of Zeros | Number of Ones |\n| --- | --- | --- | --- | --- |\n| 1 | 1 | 0 | 1 | 0 |\n| 4 | 4 | 0 | 1 | 0 |\n| 1 | 4 | 0110 | 2 | 2 |\n| 0 | 4 | 10110 | 2 | 3 |\n| 1 | 5 | 01101 | 2 | 3 |\n\n**Constraints:**\n\n* `1 <= s.length <= 4 * 104`\n* `s` consists only of characters `'0'` and `'1'`.", "templates": {"cpp": "class Solution {\npublic:\n int numberOfSubstrings(string s) {\n \n }\n};", "java": "class Solution {\n public int numberOfSubstrings(String s) {\n \n }\n}", "python": "class Solution:\n def numberOfSubstrings(self, s: str) -> int:\n "}, "hints": "1\\. Let us fix the starting index `l` of the substring and count the number of indices `r` such that `l <= r` and the substring `s[l..r]` has dominant ones.\n2\\. A substring with dominant ones has at most `sqrt(n)` zeros.\n3\\. We cannot iterate over every `r` and check if the `s[l..r]` has dominant ones. Instead, we iterate over the next `sqrt(n)` zeros to the left of `l` and count the number of substrings with dominant ones where the current zero is the rightmost zero of the substring.", "contains_png": false, "png_links": []}
{"number": 3235, "title": "Check if the Rectangle Corner Is Reachable", "link": "https://leetcode.com/problems/check-if-the-rectangle-corner-is-reachable/", "difficulty": "hard", "statement": "You are given two positive integers `xCorner` and `yCorner`, and a 2D array `circles`, where `circles[i] = [xi, yi, ri]` denotes a circle with center at `(xi, yi)` and radius `ri`.\n\nThere is a rectangle in the coordinate plane with its bottom left corner at the origin and top right corner at the coordinate `(xCorner, yCorner)`. You need to check whether there is a path from the bottom left corner to the top right corner such that the **entire path** lies inside the rectangle, **does not** touch or lie inside **any** circle, and touches the rectangle **only** at the two corners.\n\nReturn `true` if such a path exists, and `false` otherwise.\n\n**Example 1:**\n\n**Input:** xCorner \\= 3, yCorner \\= 4, circles \\= \\[\\[2,1,1]]\n\n**Output:** true\n\n**Explanation:**\n\n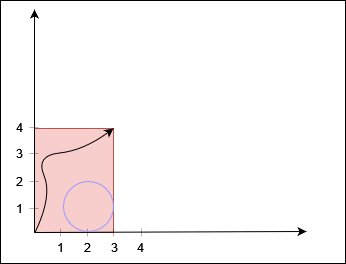\n\nThe black curve shows a possible path between `(0, 0)` and `(3, 4)`.\n\n**Example 2:**\n\n**Input:** xCorner \\= 3, yCorner \\= 3, circles \\= \\[\\[1,1,2]]\n\n**Output:** false\n\n**Explanation:**\n\n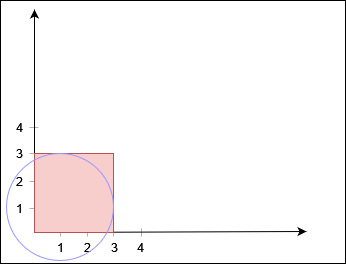\n\nNo path exists from `(0, 0)` to `(3, 3)`.\n\n**Example 3:**\n\n**Input:** xCorner \\= 3, yCorner \\= 3, circles \\= \\[\\[2,1,1],\\[1,2,1]]\n\n**Output:** false\n\n**Explanation:**\n\n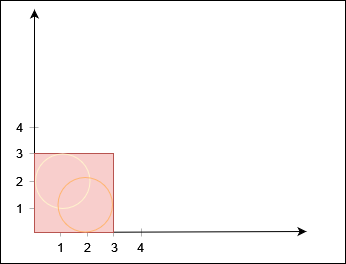\n\nNo path exists from `(0, 0)` to `(3, 3)`.\n\n**Example 4:**\n\n**Input:** xCorner \\= 4, yCorner \\= 4, circles \\= \\[\\[5,5,1]]\n\n**Output:** true\n\n**Explanation:**\n\n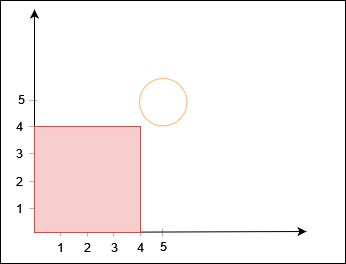\n\n**Constraints:**\n\n* `3 <= xCorner, yCorner <= 109`\n* `1 <= circles.length <= 1000`\n* `circles[i].length == 3`\n* `1 <= xi, yi, ri <= 109`", "templates": {"cpp": "class Solution {\npublic:\n bool canReachCorner(int xCorner, int yCorner, vector<vector<int>>& circles) {\n \n }\n};", "java": "class Solution {\n public boolean canReachCorner(int xCorner, int yCorner, int[][] circles) {\n \n }\n}", "python": "class Solution:\n def canReachCorner(self, xCorner: int, yCorner: int, circles: List[List[int]]) -> bool:\n "}, "hints": "1\\. Create a graph with `n + 4` vertices.\n2\\. Vertices 0 to `n - 1` represent the circles, vertex `n` represents upper edge, vertex `n + 1` represents right edge, vertex `n + 2` represents lower edge, and vertex `n + 3` represents left edge.\n3\\. Add an edge between these vertices if they intersect or touch.\n4\\. Answer will be `false` when any of two sides left\\-right, left\\-bottom, right\\-top or top\\-bottom are reachable using the edges.", "contains_png": true, "png_links": ["https://assets.leetcode.com/uploads/2024/05/18/example2circle1.png", "https://assets.leetcode.com/uploads/2024/05/18/example1circle.png", "https://assets.leetcode.com/uploads/2024/05/18/example0circle.png", "https://assets.leetcode.com/uploads/2024/08/04/rectangles.png"]}
{"number": 3238, "title": "Find the Number of Winning Players", "link": "https://leetcode.com/problems/find-the-number-of-winning-players/", "difficulty": "easy", "statement": "You are given an integer `n` representing the number of players in a game and a 2D array `pick` where `pick[i] = [xi, yi]` represents that the player `xi` picked a ball of color `yi`.\n\nPlayer `i` **wins** the game if they pick **strictly more** than `i` balls of the **same** color. In other words,\n\n* Player 0 wins if they pick any ball.\n* Player 1 wins if they pick at least two balls of the *same* color.\n* ...\n* Player `i` wins if they pick at least`i + 1` balls of the *same* color.\n\nReturn the number of players who **win** the game.\n\n**Note** that *multiple* players can win the game.\n\n**Example 1:**\n\n**Input:** n \\= 4, pick \\= \\[\\[0,0],\\[1,0],\\[1,0],\\[2,1],\\[2,1],\\[2,0]]\n\n**Output:** 2\n\n**Explanation:**\n\nPlayer 0 and player 1 win the game, while players 2 and 3 do not win.\n\n**Example 2:**\n\n**Input:** n \\= 5, pick \\= \\[\\[1,1],\\[1,2],\\[1,3],\\[1,4]]\n\n**Output:** 0\n\n**Explanation:**\n\nNo player wins the game.\n\n**Example 3:**\n\n**Input:** n \\= 5, pick \\= \\[\\[1,1],\\[2,4],\\[2,4],\\[2,4]]\n\n**Output:** 1\n\n**Explanation:**\n\nPlayer 2 wins the game by picking 3 balls with color 4\\.\n\n**Constraints:**\n\n* `2 <= n <= 10`\n* `1 <= pick.length <= 100`\n* `pick[i].length == 2`\n* `0 <= xi <= n - 1`\n* `0 <= yi <= 10`", "templates": {"cpp": "class Solution {\npublic:\n int winningPlayerCount(int n, vector<vector<int>>& pick) {\n \n }\n};", "java": "class Solution {\n public int winningPlayerCount(int n, int[][] pick) {\n \n }\n}", "python": "class Solution:\n def winningPlayerCount(self, n: int, pick: List[List[int]]) -> int:\n "}, "hints": "1\\. Keep track of the number of balls of each color for each user using hashing.\n2\\. Find the maximum color that occurred for each player.", "contains_png": false, "png_links": []}
{"number": 3239, "title": "Minimum Number of Flips to Make Binary Grid Palindromic I", "link": "https://leetcode.com/problems/minimum-number-of-flips-to-make-binary-grid-palindromic-i/", "difficulty": "medium", "statement": "You are given an `m x n` binary matrix `grid`.\n\nA row or column is considered **palindromic** if its values read the same forward and backward.\n\nYou can **flip** any number of cells in `grid` from `0` to `1`, or from `1` to `0`.\n\nReturn the **minimum** number of cells that need to be flipped to make **either** all rows **palindromic** or all columns **palindromic**.\n\n**Example 1:**\n\n**Input:** grid \\= \\[\\[1,0,0],\\[0,0,0],\\[0,0,1]]\n\n**Output:** 2\n\n**Explanation:**\n\n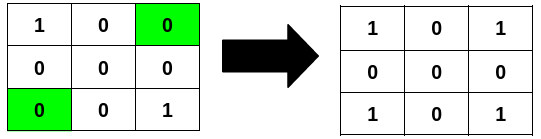\n\nFlipping the highlighted cells makes all the rows palindromic.\n\n**Example 2:**\n\n**Input:** grid \\= \\[\\[0,1],\\[0,1],\\[0,0]]\n\n**Output:** 1\n\n**Explanation:**\n\n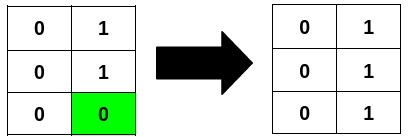\n\nFlipping the highlighted cell makes all the columns palindromic.\n\n**Example 3:**\n\n**Input:** grid \\= \\[\\[1],\\[0]]\n\n**Output:** 0\n\n**Explanation:**\n\nAll rows are already palindromic.\n\n**Constraints:**\n\n* `m == grid.length`\n* `n == grid[i].length`\n* `1 <= m * n <= 2 * 105`\n* `0 <= grid[i][j] <= 1`", "templates": {"cpp": "class Solution {\npublic:\n int minFlips(vector<vector<int>>& grid) {\n \n }\n};", "java": "class Solution {\n public int minFlips(int[][] grid) {\n \n }\n}", "python": "class Solution:\n def minFlips(self, grid: List[List[int]]) -> int:\n "}, "hints": "1\\. We need to perform the operation only when the equivalent element of `i` from the back is not equal.", "contains_png": true, "png_links": ["https://assets.leetcode.com/uploads/2024/07/07/screenshot-from-2024-07-08-00-20-10.png", "https://assets.leetcode.com/uploads/2024/07/07/screenshot-from-2024-07-08-00-31-23.png"]}
{"number": 3240, "title": "Minimum Number of Flips to Make Binary Grid Palindromic II", "link": "https://leetcode.com/problems/minimum-number-of-flips-to-make-binary-grid-palindromic-ii/", "difficulty": "medium", "statement": "You are given an `m x n` binary matrix `grid`.\n\nA row or column is considered **palindromic** if its values read the same forward and backward.\n\nYou can **flip** any number of cells in `grid` from `0` to `1`, or from `1` to `0`.\n\nReturn the **minimum** number of cells that need to be flipped to make **all** rows and columns **palindromic**, and the total number of `1`'s in `grid` **divisible** by `4`.\n\n**Example 1:**\n\n**Input:** grid \\= \\[\\[1,0,0],\\[0,1,0],\\[0,0,1]]\n\n**Output:** 3\n\n**Explanation:**\n\n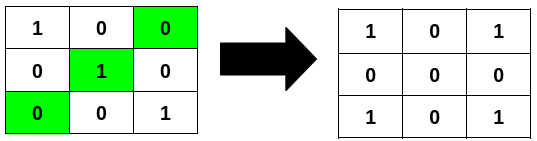\n\n**Example 2:**\n\n**Input:** grid \\= \\[\\[0,1],\\[0,1],\\[0,0]]\n\n**Output:** 2\n\n**Explanation:**\n\n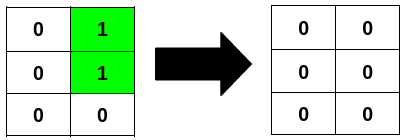\n\n**Example 3:**\n\n**Input:** grid \\= \\[\\[1],\\[1]]\n\n**Output:** 2\n\n**Explanation:**\n\n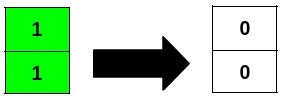\n\n**Constraints:**\n\n* `m == grid.length`\n* `n == grid[i].length`\n* `1 <= m * n <= 2 * 105`\n* `0 <= grid[i][j] <= 1`", "templates": {"cpp": "class Solution {\npublic:\n int minFlips(vector<vector<int>>& grid) {\n \n }\n};", "java": "class Solution {\n public int minFlips(int[][] grid) {\n \n }\n}", "python": "class Solution:\n def minFlips(self, grid: List[List[int]]) -> int:\n "}, "hints": "1\\. For each `(x, y)`, find `(m - 1 - x, y)`, `(m - 1 - x, n - 1 - y)`, and `(x, n - 1 - y)`; they should be the same.\n2\\. Note that we need to specially handle the middle row (column) if the number of rows (columns) is odd.", "contains_png": true, "png_links": ["https://assets.leetcode.com/uploads/2024/08/01/image.png", "https://assets.leetcode.com/uploads/2024/07/08/screenshot-from-2024-07-09-01-37-48.png", "https://assets.leetcode.com/uploads/2024/08/01/screenshot-from-2024-08-01-23-05-26.png"]}
{"number": 3241, "title": "Time Taken to Mark All Nodes", "link": "https://leetcode.com/problems/time-taken-to-mark-all-nodes/", "difficulty": "hard", "statement": "There exists an **undirected** tree with `n` nodes numbered `0` to `n - 1`. You are given a 2D integer array `edges` of length `n - 1`, where `edges[i] = [ui, vi]` indicates that there is an edge between nodes `ui` and `vi` in the tree.\n\nInitially, **all** nodes are **unmarked**. For each node `i`:\n\n* If `i` is odd, the node will get marked at time `x` if there is **at least** one node *adjacent* to it which was marked at time `x - 1`.\n* If `i` is even, the node will get marked at time `x` if there is **at least** one node *adjacent* to it which was marked at time `x - 2`.\n\nReturn an array `times` where `times[i]` is the time when all nodes get marked in the tree, if you mark node `i` at time `t = 0`.\n\n**Note** that the answer for each `times[i]` is **independent**, i.e. when you mark node `i` all other nodes are *unmarked*.\n\n**Example 1:**\n\n**Input:** edges \\= \\[\\[0,1],\\[0,2]]\n\n**Output:** \\[2,4,3]\n\n**Explanation:**\n\n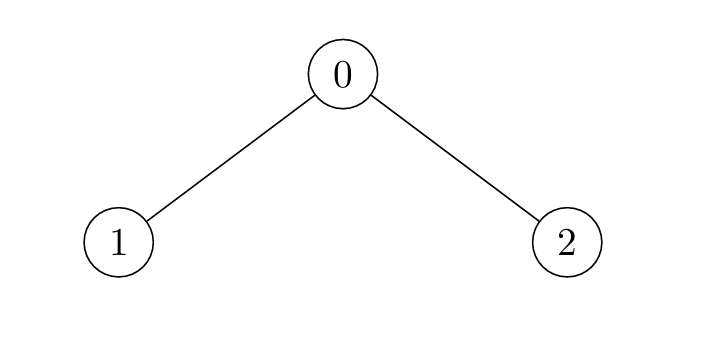\n\n* For `i = 0`:\n\t+ Node 1 is marked at `t = 1`, and Node 2 at `t = 2`.\n* For `i = 1`:\n\t+ Node 0 is marked at `t = 2`, and Node 2 at `t = 4`.\n* For `i = 2`:\n\t+ Node 0 is marked at `t = 2`, and Node 1 at `t = 3`.\n\n**Example 2:**\n\n**Input:** edges \\= \\[\\[0,1]]\n\n**Output:** \\[1,2]\n\n**Explanation:**\n\n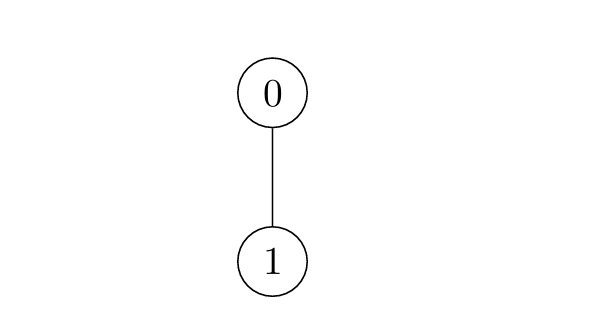\n\n* For `i = 0`:\n\t+ Node 1 is marked at `t = 1`.\n* For `i = 1`:\n\t+ Node 0 is marked at `t = 2`.\n\n**Example 3:**\n\n**Input:** edges \\= \\[\\[2,4],\\[0,1],\\[2,3],\\[0,2]]\n\n**Output:** \\[4,6,3,5,5]\n\n**Explanation:**\n\n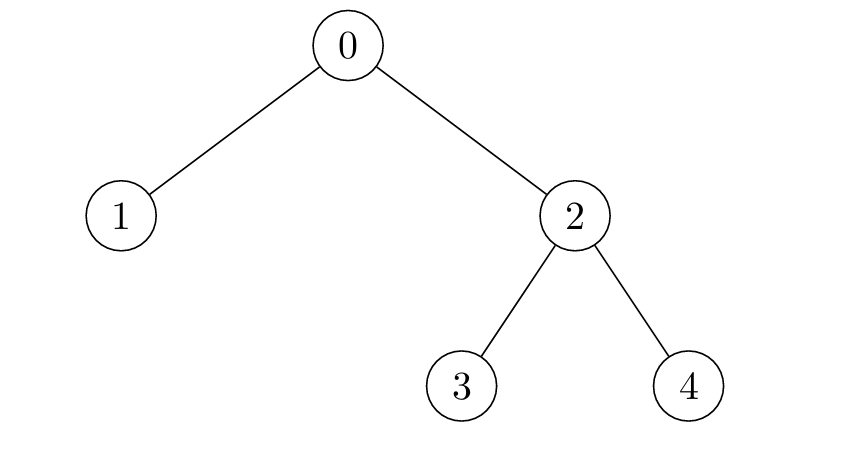\n\n**Constraints:**\n\n* `2 <= n <= 105`\n* `edges.length == n - 1`\n* `edges[i].length == 2`\n* `0 <= edges[i][0], edges[i][1] <= n - 1`\n* The input is generated such that `edges` represents a valid tree.", "templates": {"cpp": "class Solution {\npublic:\n vector<int> timeTaken(vector<vector<int>>& edges) {\n \n }\n};", "java": "class Solution {\n public int[] timeTaken(int[][] edges) {\n \n }\n}", "python": "class Solution:\n def timeTaken(self, edges: List[List[int]]) -> List[int]:\n "}, "hints": "1\\. Can we use dp on trees?\n2\\. Store the two most distant children for each node.\n3\\. When re\\-rooting the tree, keep a variable for distance to the root node.", "contains_png": true, "png_links": ["https://assets.leetcode.com/uploads/2024/06/01/screenshot-2024-06-02-122236.png", "https://assets.leetcode.com/uploads/2024/06/01/screenshot-2024-06-02-122249.png", "https://assets.leetcode.com/uploads/2024/06/03/screenshot-2024-06-03-210550.png"]}
{"number": 3242, "title": "Design Neighbor Sum Service", "link": "https://leetcode.com/problems/design-neighbor-sum-service/", "difficulty": "easy", "statement": "You are given a `n x n` 2D array `grid` containing **distinct** elements in the range `[0, n2 - 1]`.\n\nImplement the `NeighborSum` class:\n\n* `NeighborSum(int [][]grid)` initializes the object.\n* `int adjacentSum(int value)` returns the **sum** of elements which are adjacent neighbors of `value`, that is either to the top, left, right, or bottom of `value` in `grid`.\n* `int diagonalSum(int value)` returns the **sum** of elements which are diagonal neighbors of `value`, that is either to the top\\-left, top\\-right, bottom\\-left, or bottom\\-right of `value` in `grid`.\n\n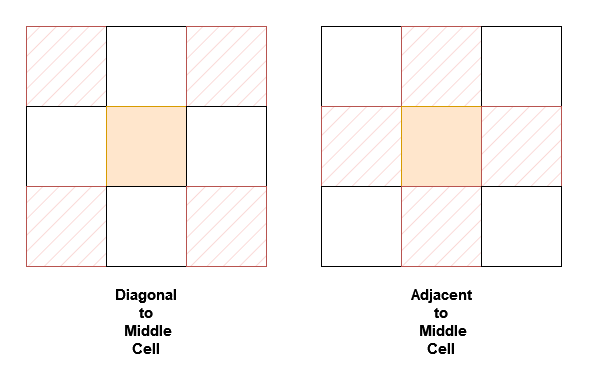\n\n**Example 1:**\n\n**Input:**\n\n\\[\"NeighborSum\", \"adjacentSum\", \"adjacentSum\", \"diagonalSum\", \"diagonalSum\"]\n\n\\[\\[\\[\\[0, 1, 2], \\[3, 4, 5], \\[6, 7, 8]]], \\[1], \\[4], \\[4], \\[8]]\n\n**Output:** \\[null, 6, 16, 16, 4]\n\n**Explanation:**\n\n**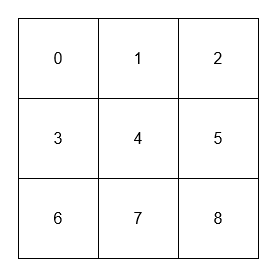**\n\n* The adjacent neighbors of 1 are 0, 2, and 4\\.\n* The adjacent neighbors of 4 are 1, 3, 5, and 7\\.\n* The diagonal neighbors of 4 are 0, 2, 6, and 8\\.\n* The diagonal neighbor of 8 is 4\\.\n\n**Example 2:**\n\n**Input:**\n\n\\[\"NeighborSum\", \"adjacentSum\", \"diagonalSum\"]\n\n\\[\\[\\[\\[1, 2, 0, 3], \\[4, 7, 15, 6], \\[8, 9, 10, 11], \\[12, 13, 14, 5]]], \\[15], \\[9]]\n\n**Output:** \\[null, 23, 45]\n\n**Explanation:**\n\n**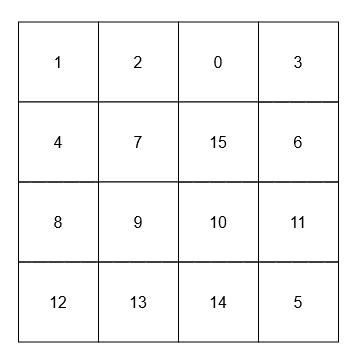**\n\n* The adjacent neighbors of 15 are 0, 10, 7, and 6\\.\n* The diagonal neighbors of 9 are 4, 12, 14, and 15\\.\n\n**Constraints:**\n\n* `3 <= n == grid.length == grid[0].length <= 10`\n* `0 <= grid[i][j] <= n2 - 1`\n* All `grid[i][j]` are distinct.\n* `value` in `adjacentSum` and `diagonalSum` will be in the range `[0, n2 - 1]`.\n* At most `2 * n2` calls will be made to `adjacentSum` and `diagonalSum`.", "templates": {"cpp": "class NeighborSum {\npublic:\n NeighborSum(vector<vector<int>>& grid) {\n \n }\n \n int adjacentSum(int value) {\n \n }\n \n int diagonalSum(int value) {\n \n }\n};\n\n/**\n * Your NeighborSum object will be instantiated and called as such:\n * NeighborSum* obj = new NeighborSum(grid);\n * int param_1 = obj->adjacentSum(value);\n * int param_2 = obj->diagonalSum(value);\n */", "java": "class NeighborSum {\n\n public NeighborSum(int[][] grid) {\n \n }\n \n public int adjacentSum(int value) {\n \n }\n \n public int diagonalSum(int value) {\n \n }\n}\n\n/**\n * Your NeighborSum object will be instantiated and called as such:\n * NeighborSum obj = new NeighborSum(grid);\n * int param_1 = obj.adjacentSum(value);\n * int param_2 = obj.diagonalSum(value);\n */", "python": "class NeighborSum:\n\n def __init__(self, grid: List[List[int]]):\n \n\n def adjacentSum(self, value: int) -> int:\n \n\n def diagonalSum(self, value: int) -> int:\n \n\n\n# Your NeighborSum object will be instantiated and called as such:\n# obj = NeighborSum(grid)\n# param_1 = obj.adjacentSum(value)\n# param_2 = obj.diagonalSum(value)"}, "hints": "1\\. Find the cell `(i, j)` in which the element is present.\n2\\. You can store the coordinates for each value.", "contains_png": true, "png_links": ["https://assets.leetcode.com/uploads/2024/06/24/design.png", "https://assets.leetcode.com/uploads/2024/06/24/designexample0.png", "https://assets.leetcode.com/uploads/2024/06/24/designexample2.png"]}
{"number": 3243, "title": "Shortest Distance After Road Addition Queries I", "link": "https://leetcode.com/problems/shortest-distance-after-road-addition-queries-i/", "difficulty": "medium", "statement": "You are given an integer `n` and a 2D integer array `queries`.\n\nThere are `n` cities numbered from `0` to `n - 1`. Initially, there is a **unidirectional** road from city `i` to city `i + 1` for all `0 <= i < n - 1`.\n\n`queries[i] = [ui, vi]` represents the addition of a new **unidirectional** road from city `ui` to city `vi`. After each query, you need to find the **length** of the **shortest path** from city `0` to city `n - 1`.\n\nReturn an array `answer` where for each `i` in the range `[0, queries.length - 1]`, `answer[i]` is the *length of the shortest path* from city `0` to city `n - 1` after processing the **first** `i + 1` queries.\n\n**Example 1:**\n\n**Input:** n \\= 5, queries \\= \\[\\[2,4],\\[0,2],\\[0,4]]\n\n**Output:** \\[3,2,1]\n\n**Explanation:** \n\n\n\nAfter the addition of the road from 2 to 4, the length of the shortest path from 0 to 4 is 3\\.\n\n\n\nAfter the addition of the road from 0 to 2, the length of the shortest path from 0 to 4 is 2\\.\n\n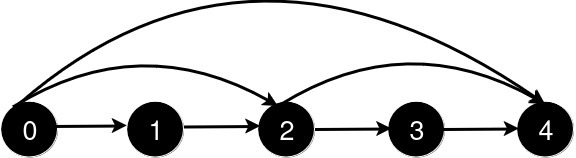\n\nAfter the addition of the road from 0 to 4, the length of the shortest path from 0 to 4 is 1\\.\n\n**Example 2:**\n\n**Input:** n \\= 4, queries \\= \\[\\[0,3],\\[0,2]]\n\n**Output:** \\[1,1]\n\n**Explanation:**\n\n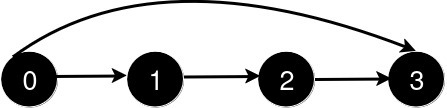\n\nAfter the addition of the road from 0 to 3, the length of the shortest path from 0 to 3 is 1\\.\n\n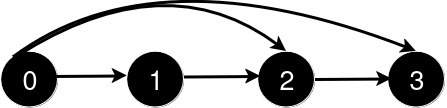\n\nAfter the addition of the road from 0 to 2, the length of the shortest path remains 1\\.\n\n**Constraints:**\n\n* `3 <= n <= 500`\n* `1 <= queries.length <= 500`\n* `queries[i].length == 2`\n* `0 <= queries[i][0] < queries[i][1] < n`\n* `1 < queries[i][1] - queries[i][0]`\n* There are no repeated roads among the queries.", "templates": {"cpp": "class Solution {\npublic:\n vector<int> shortestDistanceAfterQueries(int n, vector<vector<int>>& queries) {\n \n }\n};", "java": "class Solution {\n public int[] shortestDistanceAfterQueries(int n, int[][] queries) {\n \n }\n}", "python": "class Solution:\n def shortestDistanceAfterQueries(self, n: int, queries: List[List[int]]) -> List[int]:\n "}, "hints": "1\\. Maintain the graph and use an efficient shortest path algorithm after each update.\n2\\. We use BFS/Dijkstra for each query.", "contains_png": false, "png_links": []}
{"number": 3244, "title": "Shortest Distance After Road Addition Queries II", "link": "https://leetcode.com/problems/shortest-distance-after-road-addition-queries-ii/", "difficulty": "hard", "statement": "You are given an integer `n` and a 2D integer array `queries`.\n\nThere are `n` cities numbered from `0` to `n - 1`. Initially, there is a **unidirectional** road from city `i` to city `i + 1` for all `0 <= i < n - 1`.\n\n`queries[i] = [ui, vi]` represents the addition of a new **unidirectional** road from city `ui` to city `vi`. After each query, you need to find the **length** of the **shortest path** from city `0` to city `n - 1`.\n\nThere are no two queries such that `queries[i][0] < queries[j][0] < queries[i][1] < queries[j][1]`.\n\nReturn an array `answer` where for each `i` in the range `[0, queries.length - 1]`, `answer[i]` is the *length of the shortest path* from city `0` to city `n - 1` after processing the **first** `i + 1` queries.\n\n**Example 1:**\n\n**Input:** n \\= 5, queries \\= \\[\\[2,4],\\[0,2],\\[0,4]]\n\n**Output:** \\[3,2,1]\n\n**Explanation:** \n\n\n\nAfter the addition of the road from 2 to 4, the length of the shortest path from 0 to 4 is 3\\.\n\n\n\nAfter the addition of the road from 0 to 2, the length of the shortest path from 0 to 4 is 2\\.\n\n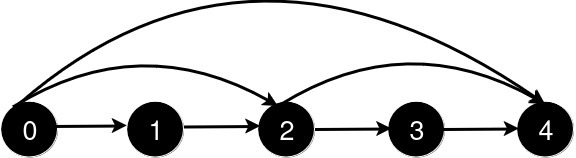\n\nAfter the addition of the road from 0 to 4, the length of the shortest path from 0 to 4 is 1\\.\n\n**Example 2:**\n\n**Input:** n \\= 4, queries \\= \\[\\[0,3],\\[0,2]]\n\n**Output:** \\[1,1]\n\n**Explanation:**\n\n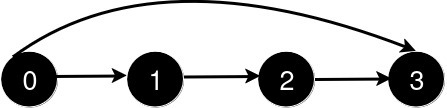\n\nAfter the addition of the road from 0 to 3, the length of the shortest path from 0 to 3 is 1\\.\n\n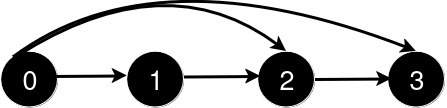\n\nAfter the addition of the road from 0 to 2, the length of the shortest path remains 1\\.\n\n**Constraints:**\n\n* `3 <= n <= 105`\n* `1 <= queries.length <= 105`\n* `queries[i].length == 2`\n* `0 <= queries[i][0] < queries[i][1] < n`\n* `1 < queries[i][1] - queries[i][0]`\n* There are no repeated roads among the queries.\n* There are no two queries such that `i != j` and `queries[i][0] < queries[j][0] < queries[i][1] < queries[j][1]`.", "templates": {"cpp": "class Solution {\npublic:\n vector<int> shortestDistanceAfterQueries(int n, vector<vector<int>>& queries) {\n \n }\n};", "java": "class Solution {\n public int[] shortestDistanceAfterQueries(int n, int[][] queries) {\n \n }\n}", "python": "class Solution:\n def shortestDistanceAfterQueries(self, n: int, queries: List[List[int]]) -> List[int]:\n "}, "hints": null, "contains_png": false, "png_links": []}
{"number": 3245, "title": "Alternating Groups III", "link": "https://leetcode.com/problems/alternating-groups-iii/", "difficulty": "hard", "statement": "There are some red and blue tiles arranged circularly. You are given an array of integers `colors` and a 2D integers array `queries`.\n\nThe color of tile `i` is represented by `colors[i]`:\n\n* `colors[i] == 0` means that tile `i` is **red**.\n* `colors[i] == 1` means that tile `i` is **blue**.\n\nAn **alternating** group is a contiguous subset of tiles in the circle with **alternating** colors (each tile in the group except the first and last one has a different color from its **adjacent** tiles in the group).\n\nYou have to process queries of two types:\n\n* `queries[i] = [1, sizei]`, determine the count of **alternating** groups with size `sizei`.\n* `queries[i] = [2, indexi, colori]`, change `colors[indexi]` to `colori`.\n\nReturn an array `answer` containing the results of the queries of the first type *in order*.\n\n**Note** that since `colors` represents a **circle**, the **first** and the **last** tiles are considered to be next to each other.\n\n**Example 1:**\n\n**Input:** colors \\= \\[0,1,1,0,1], queries \\= \\[\\[2,1,0],\\[1,4]]\n\n**Output:** \\[2]\n\n**Explanation:**\n\n**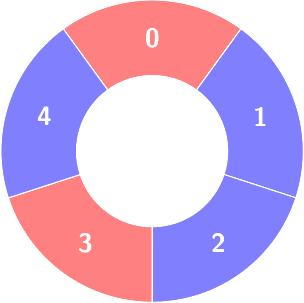**\n\nFirst query:\n\nChange `colors[1]` to 0\\.\n\n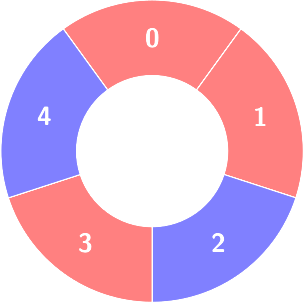\n\nSecond query:\n\nCount of the alternating groups with size 4:\n\n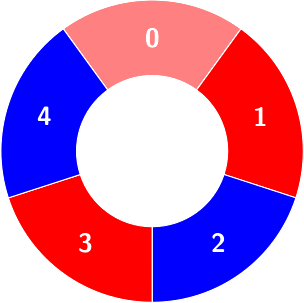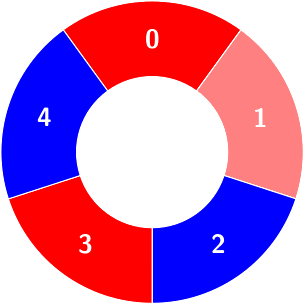\n\n**Example 2:**\n\n**Input:** colors \\= \\[0,0,1,0,1,1], queries \\= \\[\\[1,3],\\[2,3,0],\\[1,5]]\n\n**Output:** \\[2,0]\n\n**Explanation:**\n\n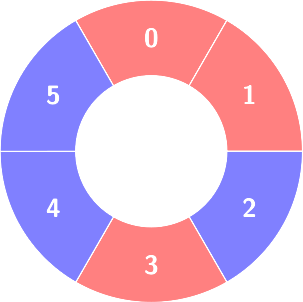\n\nFirst query:\n\nCount of the alternating groups with size 3:\n\n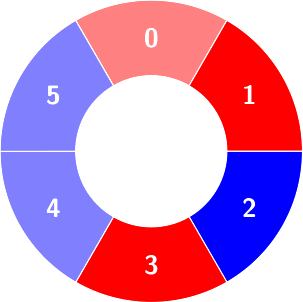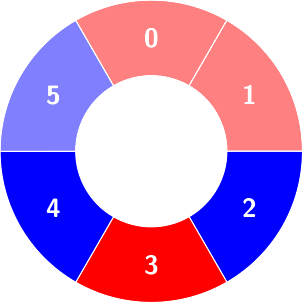\n\nSecond query: `colors` will not change.\n\nThird query: There is no alternating group with size 5\\.\n\n**Constraints:**\n\n* `4 <= colors.length <= 5 * 104`\n* `0 <= colors[i] <= 1`\n* `1 <= queries.length <= 5 * 104`\n* `queries[i][0] == 1` or `queries[i][0] == 2`\n* For all `i` that:\n\t+ `queries[i][0] == 1`: `queries[i].length == 2`, `3 <= queries[i][1] <= colors.length - 1`\n\t+ `queries[i][0] == 2`: `queries[i].length == 3`, `0 <= queries[i][1] <= colors.length - 1`, `0 <= queries[i][2] <= 1`", "templates": {"cpp": "class Solution {\npublic:\n vector<int> numberOfAlternatingGroups(vector<int>& colors, vector<vector<int>>& queries) {\n \n }\n};", "java": "class Solution {\n public List<Integer> numberOfAlternatingGroups(int[] colors, int[][] queries) {\n \n }\n}", "python": "class Solution:\n def numberOfAlternatingGroups(self, colors: List[int], queries: List[List[int]]) -> List[int]:\n "}, "hints": "1\\. Try using a segment tree to store the maximal alternating groups.\n2\\. Store the sizes of these maximal alternating groups in another data structure.\n3\\. Find the count of the alternating groups of size `k` with having the count of maximal alternating groups with size greater than or equal to `k` and the sum of their sizes.", "contains_png": true, "png_links": ["https://assets.leetcode.com/uploads/2024/06/03/screenshot-from-2024-06-03-20-14-44.png", "https://assets.leetcode.com/uploads/2024/06/03/screenshot-from-2024-06-03-20-20-25.png", "https://assets.leetcode.com/uploads/2024/06/03/screenshot-from-2024-06-03-20-25-02-2.png", "https://assets.leetcode.com/uploads/2024/06/03/screenshot-from-2024-06-03-20-24-12.png", "https://assets.leetcode.com/uploads/2024/06/03/screenshot-from-2024-06-03-20-35-50.png", "https://assets.leetcode.com/uploads/2024/06/03/screenshot-from-2024-06-03-20-37-13.png", "https://assets.leetcode.com/uploads/2024/06/03/screenshot-from-2024-06-03-20-36-40.png"]}
{"number": 3248, "title": "Snake in Matrix", "link": "https://leetcode.com/problems/snake-in-matrix/", "difficulty": "easy", "statement": "There is a snake in an `n x n` matrix `grid` and can move in **four possible directions**. Each cell in the `grid` is identified by the position: `grid[i][j] = (i * n) + j`.\n\nThe snake starts at cell 0 and follows a sequence of commands.\n\nYou are given an integer `n` representing the size of the `grid` and an array of strings `commands` where each `command[i]` is either `\"UP\"`, `\"RIGHT\"`, `\"DOWN\"`, and `\"LEFT\"`. It's guaranteed that the snake will remain within the `grid` boundaries throughout its movement.\n\nReturn the position of the final cell where the snake ends up after executing `commands`.\n\n**Example 1:**\n\n**Input:** n \\= 2, commands \\= \\[\"RIGHT\",\"DOWN\"]\n\n**Output:** 3\n\n**Explanation:**\n\n| 0 | 1 |\n| --- | --- |\n| 2 | 3 |\n\n| 0 | 1 |\n| --- | --- |\n| 2 | 3 |\n\n| 0 | 1 |\n| --- | --- |\n| 2 | 3 |\n\n**Example 2:**\n\n**Input:** n \\= 3, commands \\= \\[\"DOWN\",\"RIGHT\",\"UP\"]\n\n**Output:** 1\n\n**Explanation:**\n\n| 0 | 1 | 2 |\n| --- | --- | --- |\n| 3 | 4 | 5 |\n| 6 | 7 | 8 |\n\n| 0 | 1 | 2 |\n| --- | --- | --- |\n| 3 | 4 | 5 |\n| 6 | 7 | 8 |\n\n| 0 | 1 | 2 |\n| --- | --- | --- |\n| 3 | 4 | 5 |\n| 6 | 7 | 8 |\n\n| 0 | 1 | 2 |\n| --- | --- | --- |\n| 3 | 4 | 5 |\n| 6 | 7 | 8 |\n\n**Constraints:**\n\n* `2 <= n <= 10`\n* `1 <= commands.length <= 100`\n* `commands` consists only of `\"UP\"`, `\"RIGHT\"`, `\"DOWN\"`, and `\"LEFT\"`.\n* The input is generated such the snake will not move outside of the boundaries.", "templates": {"cpp": "class Solution {\npublic:\n int finalPositionOfSnake(int n, vector<string>& commands) {\n \n }\n};", "java": "class Solution {\n public int finalPositionOfSnake(int n, List<String> commands) {\n \n }\n}", "python": "class Solution:\n def finalPositionOfSnake(self, n: int, commands: List[str]) -> int:\n "}, "hints": "1\\. Try to update the row and column of the snake after each command.", "contains_png": false, "png_links": []}
{"number": 3249, "title": "Count the Number of Good Nodes", "link": "https://leetcode.com/problems/count-the-number-of-good-nodes/", "difficulty": "medium", "statement": "There is an **undirected** tree with `n` nodes labeled from `0` to `n - 1`, and rooted at node `0`. You are given a 2D integer array `edges` of length `n - 1`, where `edges[i] = [ai, bi]` indicates that there is an edge between nodes `ai` and `bi` in the tree.\n\nA node is **good** if all the subtrees rooted at its children have the same size.\n\nReturn the number of **good** nodes in the given tree.\n\nA **subtree** of `treeName` is a tree consisting of a node in `treeName` and all of its descendants.\n\n**Example 1:**\n\n**Input:** edges \\= \\[\\[0,1],\\[0,2],\\[1,3],\\[1,4],\\[2,5],\\[2,6]]\n\n**Output:** 7\n\n**Explanation:**\n\n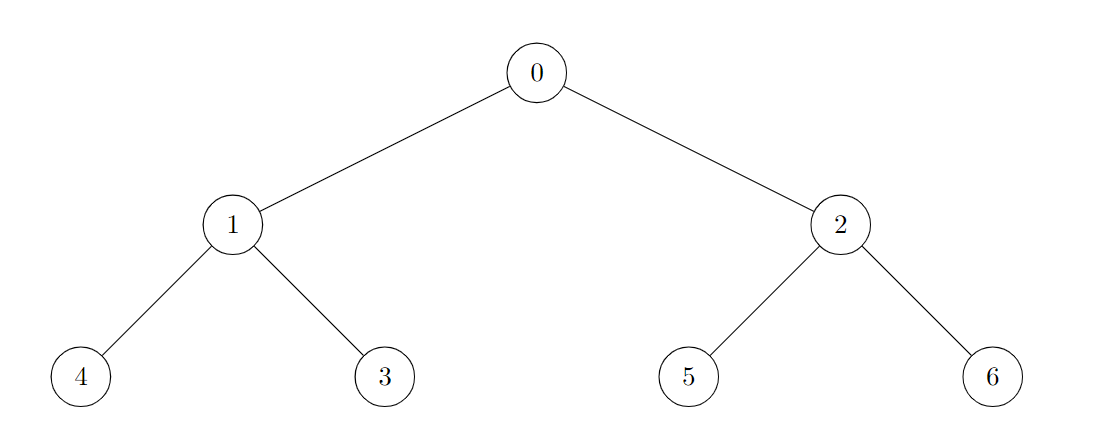\nAll of the nodes of the given tree are good.\n\n**Example 2:**\n\n**Input:** edges \\= \\[\\[0,1],\\[1,2],\\[2,3],\\[3,4],\\[0,5],\\[1,6],\\[2,7],\\[3,8]]\n\n**Output:** 6\n\n**Explanation:**\n\n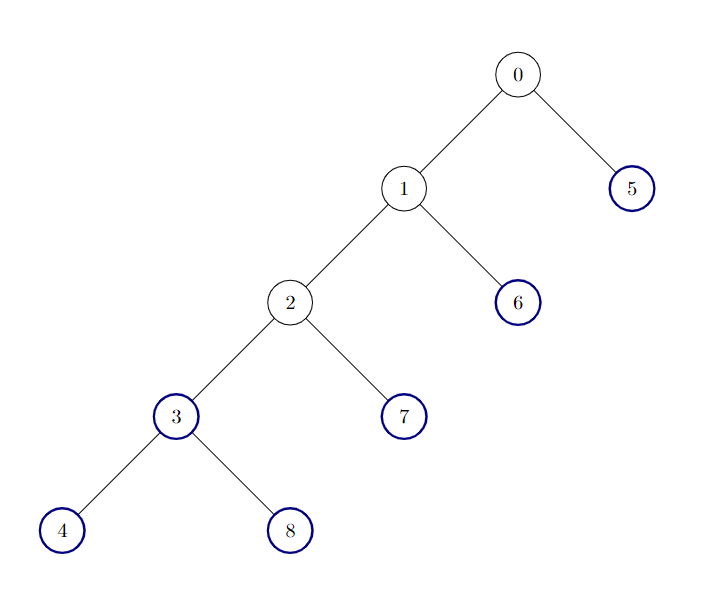\nThere are 6 good nodes in the given tree. They are colored in the image above.\n\n**Example 3:**\n\n**Input:** edges \\= \\[\\[0,1],\\[1,2],\\[1,3],\\[1,4],\\[0,5],\\[5,6],\\[6,7],\\[7,8],\\[0,9],\\[9,10],\\[9,12],\\[10,11]]\n\n**Output:** 12\n\n**Explanation:**\n\n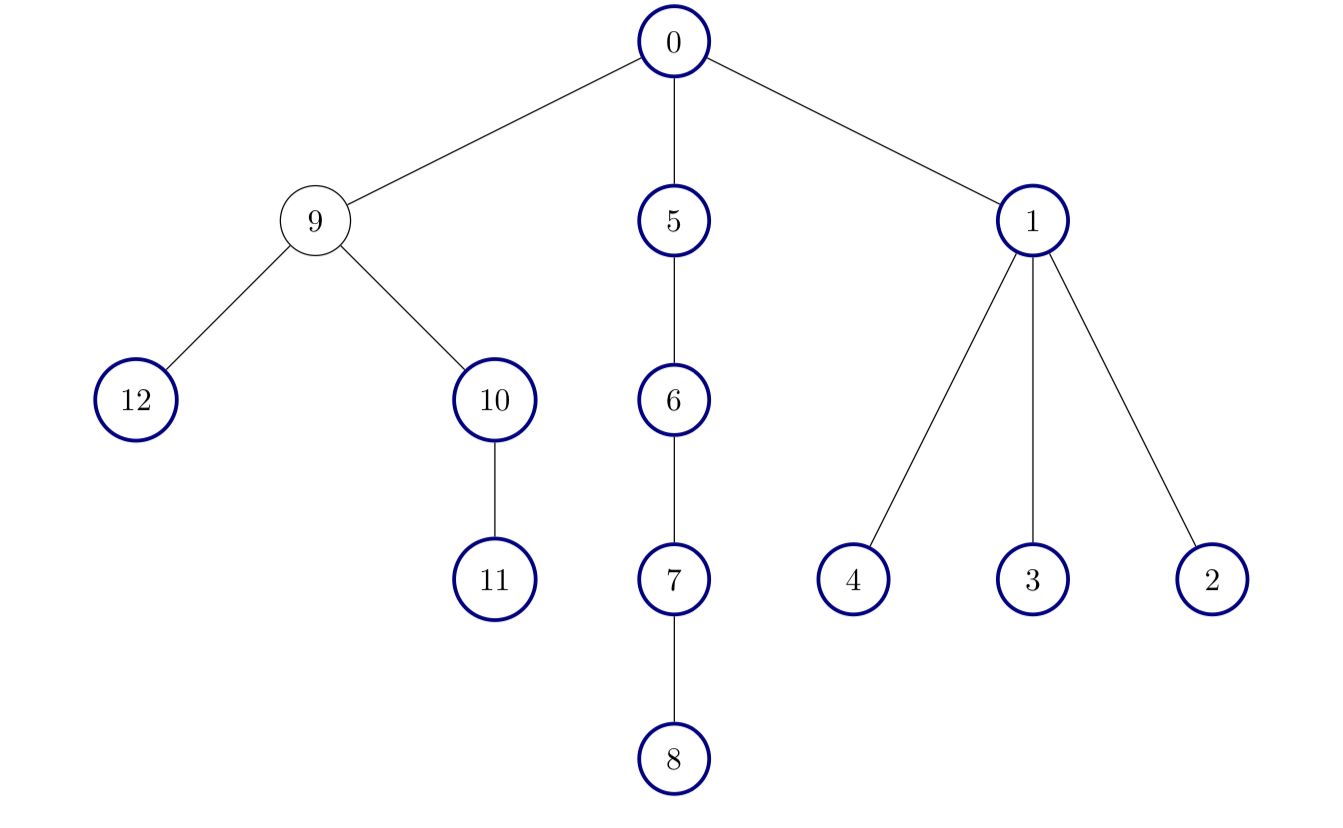\nAll nodes except node 9 are good.\n\n**Constraints:**\n\n* `2 <= n <= 105`\n* `edges.length == n - 1`\n* `edges[i].length == 2`\n* `0 <= ai, bi < n`\n* The input is generated such that `edges` represents a valid tree.", "templates": {"cpp": "class Solution {\npublic:\n int countGoodNodes(vector<vector<int>>& edges) {\n \n }\n};", "java": "class Solution {\n public int countGoodNodes(int[][] edges) {\n \n }\n}", "python": "class Solution:\n def countGoodNodes(self, edges: List[List[int]]) -> int:\n "}, "hints": "1\\. Use DFS.", "contains_png": true, "png_links": ["https://assets.leetcode.com/uploads/2024/05/26/tree1.png", "https://assets.leetcode.com/uploads/2024/06/03/screenshot-2024-06-03-193552.png"]}
{"number": 3250, "title": "Find the Count of Monotonic Pairs I", "link": "https://leetcode.com/problems/find-the-count-of-monotonic-pairs-i/", "difficulty": "hard", "statement": "You are given an array of **positive** integers `nums` of length `n`.\n\nWe call a pair of **non\\-negative** integer arrays `(arr1, arr2)` **monotonic** if:\n\n* The lengths of both arrays are `n`.\n* `arr1` is monotonically **non\\-decreasing**, in other words, `arr1[0] <= arr1[1] <= ... <= arr1[n - 1]`.\n* `arr2` is monotonically **non\\-increasing**, in other words, `arr2[0] >= arr2[1] >= ... >= arr2[n - 1]`.\n* `arr1[i] + arr2[i] == nums[i]` for all `0 <= i <= n - 1`.\n\nReturn the count of **monotonic** pairs.\n\nSince the answer may be very large, return it **modulo** `109 + 7`.\n\n**Example 1:**\n\n**Input:** nums \\= \\[2,3,2]\n\n**Output:** 4\n\n**Explanation:**\n\nThe good pairs are:\n\n1. `([0, 1, 1], [2, 2, 1])`\n2. `([0, 1, 2], [2, 2, 0])`\n3. `([0, 2, 2], [2, 1, 0])`\n4. `([1, 2, 2], [1, 1, 0])`\n\n**Example 2:**\n\n**Input:** nums \\= \\[5,5,5,5]\n\n**Output:** 126\n\n**Constraints:**\n\n* `1 <= n == nums.length <= 2000`\n* `1 <= nums[i] <= 50`", "templates": {"cpp": "class Solution {\npublic:\n int countOfPairs(vector<int>& nums) {\n \n }\n};", "java": "class Solution {\n public int countOfPairs(int[] nums) {\n \n }\n}", "python": "class Solution:\n def countOfPairs(self, nums: List[int]) -> int:\n "}, "hints": "1\\. Let `dp[i][s]` is the number of monotonic pairs of length `i` with the `arr1[i - 1] = s`.\n2\\. If `arr1[i - 1] = s`, `arr2[i - 1] = nums[i - 1] - s`.\n3\\. Check if the state in recurrence is valid.", "contains_png": false, "png_links": []}
{"number": 3251, "title": "Find the Count of Monotonic Pairs II", "link": "https://leetcode.com/problems/find-the-count-of-monotonic-pairs-ii/", "difficulty": "hard", "statement": "You are given an array of **positive** integers `nums` of length `n`.\n\nWe call a pair of **non\\-negative** integer arrays `(arr1, arr2)` **monotonic** if:\n\n* The lengths of both arrays are `n`.\n* `arr1` is monotonically **non\\-decreasing**, in other words, `arr1[0] <= arr1[1] <= ... <= arr1[n - 1]`.\n* `arr2` is monotonically **non\\-increasing**, in other words, `arr2[0] >= arr2[1] >= ... >= arr2[n - 1]`.\n* `arr1[i] + arr2[i] == nums[i]` for all `0 <= i <= n - 1`.\n\nReturn the count of **monotonic** pairs.\n\nSince the answer may be very large, return it **modulo** `109 + 7`.\n\n**Example 1:**\n\n**Input:** nums \\= \\[2,3,2]\n\n**Output:** 4\n\n**Explanation:**\n\nThe good pairs are:\n\n1. `([0, 1, 1], [2, 2, 1])`\n2. `([0, 1, 2], [2, 2, 0])`\n3. `([0, 2, 2], [2, 1, 0])`\n4. `([1, 2, 2], [1, 1, 0])`\n\n**Example 2:**\n\n**Input:** nums \\= \\[5,5,5,5]\n\n**Output:** 126\n\n**Constraints:**\n\n* `1 <= n == nums.length <= 2000`\n* `1 <= nums[i] <= 1000`", "templates": {"cpp": "class Solution {\npublic:\n int countOfPairs(vector<int>& nums) {\n \n }\n};", "java": "class Solution {\n public int countOfPairs(int[] nums) {\n \n }\n}", "python": "class Solution:\n def countOfPairs(self, nums: List[int]) -> int:\n "}, "hints": null, "contains_png": false, "png_links": []}
{"number": 3254, "title": "Find the Power of K-Size Subarrays I", "link": "https://leetcode.com/problems/find-the-power-of-k-size-subarrays-i/", "difficulty": "medium", "statement": "You are given an array of integers `nums` of length `n` and a *positive* integer `k`.\n\nThe **power** of an array is defined as:\n\n* Its **maximum** element if *all* of its elements are **consecutive** and **sorted** in **ascending** order.\n* \\-1 otherwise.\n\nYou need to find the **power** of all subarrays of `nums` of size `k`.\n\nReturn an integer array `results` of size `n - k + 1`, where `results[i]` is the *power* of `nums[i..(i + k - 1)]`.\n\n**Example 1:**\n\n**Input:** nums \\= \\[1,2,3,4,3,2,5], k \\= 3\n\n**Output:** \\[3,4,\\-1,\\-1,\\-1]\n\n**Explanation:**\n\nThere are 5 subarrays of `nums` of size 3:\n\n* `[1, 2, 3]` with the maximum element 3\\.\n* `[2, 3, 4]` with the maximum element 4\\.\n* `[3, 4, 3]` whose elements are **not** consecutive.\n* `[4, 3, 2]` whose elements are **not** sorted.\n* `[3, 2, 5]` whose elements are **not** consecutive.\n\n**Example 2:**\n\n**Input:** nums \\= \\[2,2,2,2,2], k \\= 4\n\n**Output:** \\[\\-1,\\-1]\n\n**Example 3:**\n\n**Input:** nums \\= \\[3,2,3,2,3,2], k \\= 2\n\n**Output:** \\[\\-1,3,\\-1,3,\\-1]\n\n**Constraints:**\n\n* `1 <= n == nums.length <= 500`\n* `1 <= nums[i] <= 105`\n* `1 <= k <= n`", "templates": {"cpp": "class Solution {\npublic:\n vector<int> resultsArray(vector<int>& nums, int k) {\n \n }\n};", "java": "class Solution {\n public int[] resultsArray(int[] nums, int k) {\n \n }\n}", "python": "class Solution:\n def resultsArray(self, nums: List[int], k: int) -> List[int]:\n "}, "hints": "1\\. Can we use a brute force solution with nested loops and HashSet?", "contains_png": false, "png_links": []}
{"number": 3255, "title": "Find the Power of K-Size Subarrays II", "link": "https://leetcode.com/problems/find-the-power-of-k-size-subarrays-ii/", "difficulty": "medium", "statement": "You are given an array of integers `nums` of length `n` and a *positive* integer `k`.\n\nThe **power** of an array is defined as:\n\n* Its **maximum** element if *all* of its elements are **consecutive** and **sorted** in **ascending** order.\n* \\-1 otherwise.\n\nYou need to find the **power** of all subarrays of `nums` of size `k`.\n\nReturn an integer array `results` of size `n - k + 1`, where `results[i]` is the *power* of `nums[i..(i + k - 1)]`.\n\n**Example 1:**\n\n**Input:** nums \\= \\[1,2,3,4,3,2,5], k \\= 3\n\n**Output:** \\[3,4,\\-1,\\-1,\\-1]\n\n**Explanation:**\n\nThere are 5 subarrays of `nums` of size 3:\n\n* `[1, 2, 3]` with the maximum element 3\\.\n* `[2, 3, 4]` with the maximum element 4\\.\n* `[3, 4, 3]` whose elements are **not** consecutive.\n* `[4, 3, 2]` whose elements are **not** sorted.\n* `[3, 2, 5]` whose elements are **not** consecutive.\n\n**Example 2:**\n\n**Input:** nums \\= \\[2,2,2,2,2], k \\= 4\n\n**Output:** \\[\\-1,\\-1]\n\n**Example 3:**\n\n**Input:** nums \\= \\[3,2,3,2,3,2], k \\= 2\n\n**Output:** \\[\\-1,3,\\-1,3,\\-1]\n\n**Constraints:**\n\n* `1 <= n == nums.length <= 105`\n* `1 <= nums[i] <= 106`\n* `1 <= k <= n`", "templates": {"cpp": "class Solution {\npublic:\n vector<int> resultsArray(vector<int>& nums, int k) {\n \n }\n};", "java": "class Solution {\n public int[] resultsArray(int[] nums, int k) {\n \n }\n}", "python": "class Solution:\n def resultsArray(self, nums: List[int], k: int) -> List[int]:\n "}, "hints": "1\\. Let `dp[i]` denote the length of the longest subarray ending at index `i` that has consecutive and sorted elements.\n2\\. Use a TreeMap with a sliding window to check if there are `k` elements in the subarray ending at index `i`.\n3\\. If TreeMap has less than `k` elements and `dp[i] < k`, the subarray has power equal to \\-1\\.\n4\\. Is it possible to achieve `O(nums.length)` using a Stack?", "contains_png": false, "png_links": []}
{"number": 3256, "title": "Maximum Value Sum by Placing Three Rooks I", "link": "https://leetcode.com/problems/maximum-value-sum-by-placing-three-rooks-i/", "difficulty": "hard", "statement": "You are given a `m x n` 2D array `board` representing a chessboard, where `board[i][j]` represents the **value** of the cell `(i, j)`.\n\nRooks in the **same** row or column **attack** each other. You need to place *three* rooks on the chessboard such that the rooks **do not** **attack** each other.\n\nReturn the **maximum** sum of the cell **values** on which the rooks are placed.\n\n**Example 1:**\n\n**Input:** board \\= \\[\\[\\-3,1,1,1],\\[\\-3,1,\\-3,1],\\[\\-3,2,1,1]]\n\n**Output:** 4\n\n**Explanation:**\n\n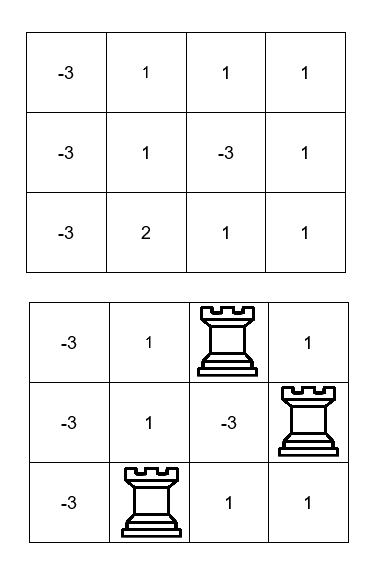\n\nWe can place the rooks in the cells `(0, 2)`, `(1, 3)`, and `(2, 1)` for a sum of `1 + 1 + 2 = 4`.\n\n**Example 2:**\n\n**Input:** board \\= \\[\\[1,2,3],\\[4,5,6],\\[7,8,9]]\n\n**Output:** 15\n\n**Explanation:**\n\nWe can place the rooks in the cells `(0, 0)`, `(1, 1)`, and `(2, 2)` for a sum of `1 + 5 + 9 = 15`.\n\n**Example 3:**\n\n**Input:** board \\= \\[\\[1,1,1],\\[1,1,1],\\[1,1,1]]\n\n**Output:** 3\n\n**Explanation:**\n\nWe can place the rooks in the cells `(0, 2)`, `(1, 1)`, and `(2, 0)` for a sum of `1 + 1 + 1 = 3`.\n\n**Constraints:**\n\n* `3 <= m == board.length <= 100`\n* `3 <= n == board[i].length <= 100`\n* `-109 <= board[i][j] <= 109`", "templates": {"cpp": "class Solution {\npublic:\n long long maximumValueSum(vector<vector<int>>& board) {\n \n }\n};", "java": "class Solution {\n public long maximumValueSum(int[][] board) {\n \n }\n}", "python": "class Solution:\n def maximumValueSum(self, board: List[List[int]]) -> int:\n "}, "hints": "1\\. Store the largest 3 values for each row.\n2\\. Select any 3 rows and brute force all combinations.", "contains_png": true, "png_links": ["https://assets.leetcode.com/uploads/2024/08/08/rooks2.png"]}
{"number": 3257, "title": "Maximum Value Sum by Placing Three Rooks II", "link": "https://leetcode.com/problems/maximum-value-sum-by-placing-three-rooks-ii/", "difficulty": "hard", "statement": "You are given a `m x n` 2D array `board` representing a chessboard, where `board[i][j]` represents the **value** of the cell `(i, j)`.\n\nRooks in the **same** row or column **attack** each other. You need to place *three* rooks on the chessboard such that the rooks **do not** **attack** each other.\n\nReturn the **maximum** sum of the cell **values** on which the rooks are placed.\n\n**Example 1:**\n\n**Input:** board \\= \\[\\[\\-3,1,1,1],\\[\\-3,1,\\-3,1],\\[\\-3,2,1,1]]\n\n**Output:** 4\n\n**Explanation:**\n\n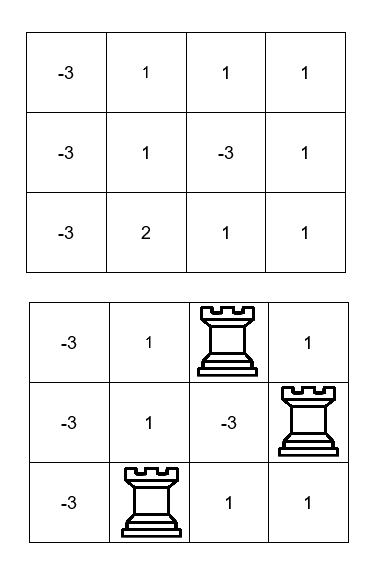\n\nWe can place the rooks in the cells `(0, 2)`, `(1, 3)`, and `(2, 1)` for a sum of `1 + 1 + 2 = 4`.\n\n**Example 2:**\n\n**Input:** board \\= \\[\\[1,2,3],\\[4,5,6],\\[7,8,9]]\n\n**Output:** 15\n\n**Explanation:**\n\nWe can place the rooks in the cells `(0, 0)`, `(1, 1)`, and `(2, 2)` for a sum of `1 + 5 + 9 = 15`.\n\n**Example 3:**\n\n**Input:** board \\= \\[\\[1,1,1],\\[1,1,1],\\[1,1,1]]\n\n**Output:** 3\n\n**Explanation:**\n\nWe can place the rooks in the cells `(0, 2)`, `(1, 1)`, and `(2, 0)` for a sum of `1 + 1 + 1 = 3`.\n\n**Constraints:**\n\n* `3 <= m == board.length <= 500`\n* `3 <= n == board[i].length <= 500`\n* `-109 <= board[i][j] <= 109`", "templates": {"cpp": "class Solution {\npublic:\n long long maximumValueSum(vector<vector<int>>& board) {\n \n }\n};", "java": "class Solution {\n public long maximumValueSum(int[][] board) {\n \n }\n}", "python": "class Solution:\n def maximumValueSum(self, board: List[List[int]]) -> int:\n "}, "hints": "1\\. Save the top 3 largest values in each row.\n2\\. Select any row, and select any of the three values stored in it.\n3\\. Get the top 4 values from all of the other 3 largest values of the other rows, which do not share the same column as the selected value.\n4\\. Brute force the selection of 2 positions from the top 4 now.", "contains_png": true, "png_links": ["https://assets.leetcode.com/uploads/2024/08/08/rooks2.png"]}
{"number": 3258, "title": "Count Substrings That Satisfy K-Constraint I", "link": "https://leetcode.com/problems/count-substrings-that-satisfy-k-constraint-i/", "difficulty": "easy", "statement": "You are given a **binary** string `s` and an integer `k`.\n\nA **binary string** satisfies the **k\\-constraint** if **either** of the following conditions holds:\n\n* The number of `0`'s in the string is at most `k`.\n* The number of `1`'s in the string is at most `k`.\n\nReturn an integer denoting the number of substrings of `s` that satisfy the **k\\-constraint**.\n\n**Example 1:**\n\n**Input:** s \\= \"10101\", k \\= 1\n\n**Output:** 12\n\n**Explanation:**\n\nEvery substring of `s` except the substrings `\"1010\"`, `\"10101\"`, and `\"0101\"` satisfies the k\\-constraint.\n\n**Example 2:**\n\n**Input:** s \\= \"1010101\", k \\= 2\n\n**Output:** 25\n\n**Explanation:**\n\nEvery substring of `s` except the substrings with a length greater than 5 satisfies the k\\-constraint.\n\n**Example 3:**\n\n**Input:** s \\= \"11111\", k \\= 1\n\n**Output:** 15\n\n**Explanation:**\n\nAll substrings of `s` satisfy the k\\-constraint.\n\n**Constraints:**\n\n* `1 <= s.length <= 50`\n* `1 <= k <= s.length`\n* `s[i]` is either `'0'` or `'1'`.", "templates": {"cpp": "class Solution {\npublic:\n int countKConstraintSubstrings(string s, int k) {\n \n }\n};", "java": "class Solution {\n public int countKConstraintSubstrings(String s, int k) {\n \n }\n}", "python": "class Solution:\n def countKConstraintSubstrings(self, s: str, k: int) -> int:\n "}, "hints": "1\\. Using a brute force approach, check each index until a substring satisfying the k\\-constraint is found, then increment.", "contains_png": false, "png_links": []}
{"number": 3259, "title": "Maximum Energy Boost From Two Drinks", "link": "https://leetcode.com/problems/maximum-energy-boost-from-two-drinks/", "difficulty": "medium", "statement": "You are given two integer arrays `energyDrinkA` and `energyDrinkB` of the same length `n` by a futuristic sports scientist. These arrays represent the energy boosts per hour provided by two different energy drinks, A and B, respectively.\n\nYou want to *maximize* your total energy boost by drinking one energy drink *per hour*. However, if you want to switch from consuming one energy drink to the other, you need to wait for *one hour* to cleanse your system (meaning you won't get any energy boost in that hour).\n\nReturn the **maximum** total energy boost you can gain in the next `n` hours.\n\n**Note** that you can start consuming *either* of the two energy drinks.\n\n**Example 1:**\n\n**Input:** energyDrinkA \\= \\[1,3,1], energyDrinkB \\= \\[3,1,1]\n\n**Output:** 5\n\n**Explanation:**\n\nTo gain an energy boost of 5, drink only the energy drink A (or only B).\n\n**Example 2:**\n\n**Input:** energyDrinkA \\= \\[4,1,1], energyDrinkB \\= \\[1,1,3]\n\n**Output:** 7\n\n**Explanation:**\n\nTo gain an energy boost of 7:\n\n* Drink the energy drink A for the first hour.\n* Switch to the energy drink B and we lose the energy boost of the second hour.\n* Gain the energy boost of the drink B in the third hour.\n\n**Constraints:**\n\n* `n == energyDrinkA.length == energyDrinkB.length`\n* `3 <= n <= 105`\n* `1 <= energyDrinkA[i], energyDrinkB[i] <= 105`", "templates": {"cpp": "class Solution {\npublic:\n long long maxEnergyBoost(vector<int>& energyDrinkA, vector<int>& energyDrinkB) {\n \n }\n};", "java": "class Solution {\n public long maxEnergyBoost(int[] energyDrinkA, int[] energyDrinkB) {\n \n }\n}", "python": "class Solution:\n def maxEnergyBoost(self, energyDrinkA: List[int], energyDrinkB: List[int]) -> int:\n "}, "hints": "1\\. Can we solve it using dynamic programming?\n2\\. Define `dpA[i]` as the maximum energy boost if we consider only the first `i + 1` hours such that in the last hour, we drink the energy drink A.\n3\\. Similarly define `dpB[i]`.\n4\\. `dpA[i] = max(dpA[i - 1], dpB[i - 2]) + energyDrinkA[i]`\n5\\. Similarly, fill `dpB`.\n6\\. The answer is `max(dpA[n - 1], dpB[n - 1])`.", "contains_png": false, "png_links": []}
{"number": 3260, "title": "Find the Largest Palindrome Divisible by K", "link": "https://leetcode.com/problems/find-the-largest-palindrome-divisible-by-k/", "difficulty": "hard", "statement": "You are given two **positive** integers `n` and `k`.\n\nAn integer `x` is called **k\\-palindromic** if:\n\n* `x` is a palindrome.\n* `x` is divisible by `k`.\n\nReturn the **largest** integer having `n` digits (as a string) that is **k\\-palindromic**.\n\n**Note** that the integer must **not** have leading zeros.\n\n**Example 1:**\n\n**Input:** n \\= 3, k \\= 5\n\n**Output:** \"595\"\n\n**Explanation:**\n\n595 is the largest k\\-palindromic integer with 3 digits.\n\n**Example 2:**\n\n**Input:** n \\= 1, k \\= 4\n\n**Output:** \"8\"\n\n**Explanation:**\n\n4 and 8 are the only k\\-palindromic integers with 1 digit.\n\n**Example 3:**\n\n**Input:** n \\= 5, k \\= 6\n\n**Output:** \"89898\"\n\n**Constraints:**\n\n* `1 <= n <= 105`\n* `1 <= k <= 9`", "templates": {"cpp": "class Solution {\npublic:\n string largestPalindrome(int n, int k) {\n \n }\n};", "java": "class Solution {\n public String largestPalindrome(int n, int k) {\n \n }\n}", "python": "class Solution:\n def largestPalindrome(self, n: int, k: int) -> str:\n "}, "hints": "1\\. It must have a solution since we can have all digits equal to `k`.\n2\\. Use string dp, store modulus along with length of number currently formed.\n3\\. Is it possible to solve greedily using divisibility rules?", "contains_png": false, "png_links": []}
{"number": 3261, "title": "Count Substrings That Satisfy K-Constraint II", "link": "https://leetcode.com/problems/count-substrings-that-satisfy-k-constraint-ii/", "difficulty": "hard", "statement": "You are given a **binary** string `s` and an integer `k`.\n\nYou are also given a 2D integer array `queries`, where `queries[i] = [li, ri]`.\n\nA **binary string** satisfies the **k\\-constraint** if **either** of the following conditions holds:\n\n* The number of `0`'s in the string is at most `k`.\n* The number of `1`'s in the string is at most `k`.\n\nReturn an integer array `answer`, where `answer[i]` is the number of substrings of `s[li..ri]` that satisfy the **k\\-constraint**.\n\n**Example 1:**\n\n**Input:** s \\= \"0001111\", k \\= 2, queries \\= \\[\\[0,6]]\n\n**Output:** \\[26]\n\n**Explanation:**\n\nFor the query `[0, 6]`, all substrings of `s[0..6] = \"0001111\"` satisfy the k\\-constraint except for the substrings `s[0..5] = \"000111\"` and `s[0..6] = \"0001111\"`.\n\n**Example 2:**\n\n**Input:** s \\= \"010101\", k \\= 1, queries \\= \\[\\[0,5],\\[1,4],\\[2,3]]\n\n**Output:** \\[15,9,3]\n\n**Explanation:**\n\nThe substrings of `s` with a length greater than 3 do not satisfy the k\\-constraint.\n\n**Constraints:**\n\n* `1 <= s.length <= 105`\n* `s[i]` is either `'0'` or `'1'`.\n* `1 <= k <= s.length`\n* `1 <= queries.length <= 105`\n* `queries[i] == [li, ri]`\n* `0 <= li <= ri < s.length`\n* All queries are distinct.", "templates": {"cpp": "class Solution {\npublic:\n vector<long long> countKConstraintSubstrings(string s, int k, vector<vector<int>>& queries) {\n \n }\n};", "java": "class Solution {\n public long[] countKConstraintSubstrings(String s, int k, int[][] queries) {\n \n }\n}", "python": "class Solution:\n def countKConstraintSubstrings(self, s: str, k: int, queries: List[List[int]]) -> List[int]:\n "}, "hints": "1\\. Answering online queries is tough. Try to answer them offline since the queries are known beforehand.\n2\\. For each index, how do you calculate the left boundary so that the given condition is satisfied?\n3\\. Using the precomputed left boundaries and a range data structure, you can now answer the queries optimally.", "contains_png": false, "png_links": []}
{"number": 3264, "title": "Final Array State After K Multiplication Operations I", "link": "https://leetcode.com/problems/final-array-state-after-k-multiplication-operations-i/", "difficulty": "easy", "statement": "You are given an integer array `nums`, an integer `k`, and an integer `multiplier`.\n\nYou need to perform `k` operations on `nums`. In each operation:\n\n* Find the **minimum** value `x` in `nums`. If there are multiple occurrences of the minimum value, select the one that appears **first**.\n* Replace the selected minimum value `x` with `x * multiplier`.\n\nReturn an integer array denoting the *final state* of `nums` after performing all `k` operations.\n\n**Example 1:**\n\n**Input:** nums \\= \\[2,1,3,5,6], k \\= 5, multiplier \\= 2\n\n**Output:** \\[8,4,6,5,6]\n\n**Explanation:**\n\n| Operation | Result |\n| --- | --- |\n| After operation 1 | \\[2, 2, 3, 5, 6] |\n| After operation 2 | \\[4, 2, 3, 5, 6] |\n| After operation 3 | \\[4, 4, 3, 5, 6] |\n| After operation 4 | \\[4, 4, 6, 5, 6] |\n| After operation 5 | \\[8, 4, 6, 5, 6] |\n\n**Example 2:**\n\n**Input:** nums \\= \\[1,2], k \\= 3, multiplier \\= 4\n\n**Output:** \\[16,8]\n\n**Explanation:**\n\n| Operation | Result |\n| --- | --- |\n| After operation 1 | \\[4, 2] |\n| After operation 2 | \\[4, 8] |\n| After operation 3 | \\[16, 8] |\n\n**Constraints:**\n\n* `1 <= nums.length <= 100`\n* `1 <= nums[i] <= 100`\n* `1 <= k <= 10`\n* `1 <= multiplier <= 5`", "templates": {"cpp": "class Solution {\npublic:\n vector<int> getFinalState(vector<int>& nums, int k, int multiplier) {\n \n }\n};", "java": "class Solution {\n public int[] getFinalState(int[] nums, int k, int multiplier) {\n \n }\n}", "python": "class Solution:\n def getFinalState(self, nums: List[int], k: int, multiplier: int) -> List[int]:\n "}, "hints": "1\\. Maintain sorted pairs `(nums[index], index)` in a priority queue.\n2\\. Simulate the operation `k` times.", "contains_png": false, "png_links": []}
{"number": 3265, "title": "Count Almost Equal Pairs I", "link": "https://leetcode.com/problems/count-almost-equal-pairs-i/", "difficulty": "medium", "statement": "You are given an array `nums` consisting of positive integers.\n\nWe call two integers `x` and `y` in this problem **almost equal** if both integers can become equal after performing the following operation **at most once**:\n\n* Choose **either** `x` or `y` and swap any two digits within the chosen number.\n\nReturn the number of indices `i` and `j` in `nums` where `i < j` such that `nums[i]` and `nums[j]` are **almost equal**.\n\n**Note** that it is allowed for an integer to have leading zeros after performing an operation.\n\n**Example 1:**\n\n**Input:** nums \\= \\[3,12,30,17,21]\n\n**Output:** 2\n\n**Explanation:**\n\nThe almost equal pairs of elements are:\n\n* 3 and 30\\. By swapping 3 and 0 in 30, you get 3\\.\n* 12 and 21\\. By swapping 1 and 2 in 12, you get 21\\.\n\n**Example 2:**\n\n**Input:** nums \\= \\[1,1,1,1,1]\n\n**Output:** 10\n\n**Explanation:**\n\nEvery two elements in the array are almost equal.\n\n**Example 3:**\n\n**Input:** nums \\= \\[123,231]\n\n**Output:** 0\n\n**Explanation:**\n\nWe cannot swap any two digits of 123 or 231 to reach the other.\n\n**Constraints:**\n\n* `2 <= nums.length <= 100`\n* `1 <= nums[i] <= 106`", "templates": {"cpp": "class Solution {\npublic:\n int countPairs(vector<int>& nums) {\n \n }\n};", "java": "class Solution {\n public int countPairs(int[] nums) {\n \n }\n}", "python": "class Solution:\n def countPairs(self, nums: List[int]) -> int:\n "}, "hints": "1\\. Since the constraint on the number of elements is small, you can check all pairs in the array.\n2\\. For each pair, perform an operation on one of the elements and check if it becomes equal to the other.", "contains_png": false, "png_links": []}
{"number": 3266, "title": "Final Array State After K Multiplication Operations II", "link": "https://leetcode.com/problems/final-array-state-after-k-multiplication-operations-ii/", "difficulty": "hard", "statement": "You are given an integer array `nums`, an integer `k`, and an integer `multiplier`.\n\nYou need to perform `k` operations on `nums`. In each operation:\n\n* Find the **minimum** value `x` in `nums`. If there are multiple occurrences of the minimum value, select the one that appears **first**.\n* Replace the selected minimum value `x` with `x * multiplier`.\n\nAfter the `k` operations, apply **modulo** `109 + 7` to every value in `nums`.\n\nReturn an integer array denoting the *final state* of `nums` after performing all `k` operations and then applying the modulo.\n\n**Example 1:**\n\n**Input:** nums \\= \\[2,1,3,5,6], k \\= 5, multiplier \\= 2\n\n**Output:** \\[8,4,6,5,6]\n\n**Explanation:**\n\n| Operation | Result |\n| --- | --- |\n| After operation 1 | \\[2, 2, 3, 5, 6] |\n| After operation 2 | \\[4, 2, 3, 5, 6] |\n| After operation 3 | \\[4, 4, 3, 5, 6] |\n| After operation 4 | \\[4, 4, 6, 5, 6] |\n| After operation 5 | \\[8, 4, 6, 5, 6] |\n| After applying modulo | \\[8, 4, 6, 5, 6] |\n\n**Example 2:**\n\n**Input:** nums \\= \\[100000,2000], k \\= 2, multiplier \\= 1000000\n\n**Output:** \\[999999307,999999993]\n\n**Explanation:**\n\n| Operation | Result |\n| --- | --- |\n| After operation 1 | \\[100000, 2000000000] |\n| After operation 2 | \\[100000000000, 2000000000] |\n| After applying modulo | \\[999999307, 999999993] |\n\n**Constraints:**\n\n* `1 <= nums.length <= 104`\n* `1 <= nums[i] <= 109`\n* `1 <= k <= 109`\n* `1 <= multiplier <= 106`", "templates": {"cpp": "class Solution {\npublic:\n vector<int> getFinalState(vector<int>& nums, int k, int multiplier) {\n \n }\n};", "java": "class Solution {\n public int[] getFinalState(int[] nums, int k, int multiplier) {\n \n }\n}", "python": "class Solution:\n def getFinalState(self, nums: List[int], k: int, multiplier: int) -> List[int]:\n "}, "hints": "1\\. What happens when `min(nums) * multiplier > max(nums)`?\n2\\. A cycle of operations begins.\n3\\. Simulate until `min(nums) * multiplier > max(nums)`, then greedily distribute remaining multiplications.", "contains_png": false, "png_links": []}
{"number": 3267, "title": "Count Almost Equal Pairs II", "link": "https://leetcode.com/problems/count-almost-equal-pairs-ii/", "difficulty": "hard", "statement": "**Attention**: In this version, the number of operations that can be performed, has been increased to **twice**.\n\nYou are given an array `nums` consisting of positive integers.\n\nWe call two integers `x` and `y` **almost equal** if both integers can become equal after performing the following operation **at most twice**:\n\n* Choose **either** `x` or `y` and swap any two digits within the chosen number.\n\nReturn the number of indices `i` and `j` in `nums` where `i < j` such that `nums[i]` and `nums[j]` are **almost equal**.\n\n**Note** that it is allowed for an integer to have leading zeros after performing an operation.\n\n**Example 1:**\n\n**Input:** nums \\= \\[1023,2310,2130,213]\n\n**Output:** 4\n\n**Explanation:**\n\nThe almost equal pairs of elements are:\n\n* 1023 and 2310\\. By swapping the digits 1 and 2, and then the digits 0 and 3 in 1023, you get 2310\\.\n* 1023 and 213\\. By swapping the digits 1 and 0, and then the digits 1 and 2 in 1023, you get 0213, which is 213\\.\n* 2310 and 213\\. By swapping the digits 2 and 0, and then the digits 3 and 2 in 2310, you get 0213, which is 213\\.\n* 2310 and 2130\\. By swapping the digits 3 and 1 in 2310, you get 2130\\.\n\n**Example 2:**\n\n**Input:** nums \\= \\[1,10,100]\n\n**Output:** 3\n\n**Explanation:**\n\nThe almost equal pairs of elements are:\n\n* 1 and 10\\. By swapping the digits 1 and 0 in 10, you get 01 which is 1\\.\n* 1 and 100\\. By swapping the second 0 with the digit 1 in 100, you get 001, which is 1\\.\n* 10 and 100\\. By swapping the first 0 with the digit 1 in 100, you get 010, which is 10\\.\n\n**Constraints:**\n\n* `2 <= nums.length <= 5000`\n* `1 <= nums[i] < 107`", "templates": {"cpp": "class Solution {\npublic:\n int countPairs(vector<int>& nums) {\n \n }\n};", "java": "class Solution {\n public int countPairs(int[] nums) {\n \n }\n}", "python": "class Solution:\n def countPairs(self, nums: List[int]) -> int:\n "}, "hints": "1\\. For each element, find all possible integers we can get by applying the operations.\n2\\. Store the frequencies of all the integers in a hashmap.", "contains_png": false, "png_links": []}
{"number": 3270, "title": "Find the Key of the Numbers", "link": "https://leetcode.com/problems/find-the-key-of-the-numbers/", "difficulty": "easy", "statement": "You are given three **positive** integers `num1`, `num2`, and `num3`.\n\nThe `key` of `num1`, `num2`, and `num3` is defined as a four\\-digit number such that:\n\n* Initially, if any number has **less than** four digits, it is padded with **leading zeros**.\n* The `ith` digit (`1 <= i <= 4`) of the `key` is generated by taking the **smallest** digit among the `ith` digits of `num1`, `num2`, and `num3`.\n\nReturn the `key` of the three numbers **without** leading zeros (*if any*).\n\n**Example 1:**\n\n**Input:** num1 \\= 1, num2 \\= 10, num3 \\= 1000\n\n**Output:** 0\n\n**Explanation:**\n\nOn padding, `num1` becomes `\"0001\"`, `num2` becomes `\"0010\"`, and `num3` remains `\"1000\"`.\n\n* The `1st` digit of the `key` is `min(0, 0, 1)`.\n* The `2nd` digit of the `key` is `min(0, 0, 0)`.\n* The `3rd` digit of the `key` is `min(0, 1, 0)`.\n* The `4th` digit of the `key` is `min(1, 0, 0)`.\n\nHence, the `key` is `\"0000\"`, i.e. 0\\.\n\n**Example 2:**\n\n**Input:** num1 \\= 987, num2 \\= 879, num3 \\= 798\n\n**Output:** 777\n\n**Example 3:**\n\n**Input:** num1 \\= 1, num2 \\= 2, num3 \\= 3\n\n**Output:** 1\n\n**Constraints:**\n\n* `1 <= num1, num2, num3 <= 9999`", "templates": {"cpp": "class Solution {\npublic:\n int generateKey(int num1, int num2, int num3) {\n \n }\n};", "java": "class Solution {\n public int generateKey(int num1, int num2, int num3) {\n \n }\n}", "python": "class Solution:\n def generateKey(self, num1: int, num2: int, num3: int) -> int:\n "}, "hints": null, "contains_png": false, "png_links": []}
{"number": 3271, "title": "Hash Divided String", "link": "https://leetcode.com/problems/hash-divided-string/", "difficulty": "medium", "statement": "You are given a string `s` of length `n` and an integer `k`, where `n` is a **multiple** of `k`. Your task is to hash the string `s` into a new string called `result`, which has a length of `n / k`.\n\nFirst, divide `s` into `n / k` **substrings**, each with a length of `k`. Then, initialize `result` as an **empty** string.\n\nFor each **substring** in order from the beginning:\n\n* The **hash value** of a character is the index of that character in the **English alphabet** (e.g., `'a' \u2192 0`, `'b' \u2192 1`, ..., `'z' \u2192 25`).\n* Calculate the *sum* of all the **hash values** of the characters in the substring.\n* Find the remainder of this sum when divided by 26, which is called `hashedChar`.\n* Identify the character in the English lowercase alphabet that corresponds to `hashedChar`.\n* Append that character to the end of `result`.\n\nReturn `result`.\n\n**Example 1:**\n\n**Input:** s \\= \"abcd\", k \\= 2\n\n**Output:** \"bf\"\n\n**Explanation:**\n\nFirst substring: `\"ab\"`, `0 + 1 = 1`, `1 % 26 = 1`, `result[0] = 'b'`.\n\nSecond substring: `\"cd\"`, `2 + 3 = 5`, `5 % 26 = 5`, `result[1] = 'f'`.\n\n**Example 2:**\n\n**Input:** s \\= \"mxz\", k \\= 3\n\n**Output:** \"i\"\n\n**Explanation:**\n\nThe only substring: `\"mxz\"`, `12 + 23 + 25 = 60`, `60 % 26 = 8`, `result[0] = 'i'`.\n\n**Constraints:**\n\n* `1 <= k <= 100`\n* `k <= s.length <= 1000`\n* `s.length` is divisible by `k`.\n* `s` consists only of lowercase English letters.", "templates": {"cpp": "class Solution {\npublic:\n string stringHash(string s, int k) {\n \n }\n};", "java": "class Solution {\n public String stringHash(String s, int k) {\n \n }\n}", "python": "class Solution:\n def stringHash(self, s: str, k: int) -> str:\n "}, "hints": "1\\. Try to find each substring.\n2\\. Use a for loop to find `hashedChar` of each substring.\n3\\. Find the answer using `hashedChar` of each substring.", "contains_png": false, "png_links": []}
{"number": 3272, "title": "Find the Count of Good Integers", "link": "https://leetcode.com/problems/find-the-count-of-good-integers/", "difficulty": "hard", "statement": "You are given two **positive** integers `n` and `k`.\n\nAn integer `x` is called **k\\-palindromic** if:\n\n* `x` is a palindrome.\n* `x` is divisible by `k`.\n\nAn integer is called **good** if its digits can be *rearranged* to form a **k\\-palindromic** integer. For example, for `k = 2`, 2020 can be rearranged to form the *k\\-palindromic* integer 2002, whereas 1010 cannot be rearranged to form a *k\\-palindromic* integer.\n\nReturn the count of **good** integers containing `n` digits.\n\n**Note** that *any* integer must **not** have leading zeros, **neither** before **nor** after rearrangement. For example, 1010 *cannot* be rearranged to form 101\\.\n\n**Example 1:**\n\n**Input:** n \\= 3, k \\= 5\n\n**Output:** 27\n\n**Explanation:**\n\n*Some* of the good integers are:\n\n* 551 because it can be rearranged to form 515\\.\n* 525 because it is already k\\-palindromic.\n\n**Example 2:**\n\n**Input:** n \\= 1, k \\= 4\n\n**Output:** 2\n\n**Explanation:**\n\nThe two good integers are 4 and 8\\.\n\n**Example 3:**\n\n**Input:** n \\= 5, k \\= 6\n\n**Output:** 2468\n\n**Constraints:**\n\n* `1 <= n <= 10`\n* `1 <= k <= 9`", "templates": {"cpp": "class Solution {\npublic:\n long long countGoodIntegers(int n, int k) {\n \n }\n};", "java": "class Solution {\n public long countGoodIntegers(int n, int k) {\n \n }\n}", "python": "class Solution:\n def countGoodIntegers(self, n: int, k: int) -> int:\n "}, "hints": "1\\. How to generate all K\\-palindromic strings of length `n`? Do we need to go through all `n` digits?\n2\\. Use permutations to calculate the number of possible rearrangements.", "contains_png": false, "png_links": []}
{"number": 3273, "title": "Minimum Amount of Damage Dealt to Bob", "link": "https://leetcode.com/problems/minimum-amount-of-damage-dealt-to-bob/", "difficulty": "hard", "statement": "You are given an integer `power` and two integer arrays `damage` and `health`, both having length `n`.\n\nBob has `n` enemies, where enemy `i` will deal Bob `damage[i]` **points** of damage per second while they are *alive* (i.e. `health[i] > 0`).\n\nEvery second, **after** the enemies deal damage to Bob, he chooses **one** of the enemies that is still *alive* and deals `power` points of damage to them.\n\nDetermine the **minimum** total amount of damage points that will be dealt to Bob before **all** `n` enemies are *dead*.\n\n**Example 1:**\n\n**Input:** power \\= 4, damage \\= \\[1,2,3,4], health \\= \\[4,5,6,8]\n\n**Output:** 39\n\n**Explanation:**\n\n* Attack enemy 3 in the first two seconds, after which enemy 3 will go down, the number of damage points dealt to Bob is `10 + 10 = 20` points.\n* Attack enemy 2 in the next two seconds, after which enemy 2 will go down, the number of damage points dealt to Bob is `6 + 6 = 12` points.\n* Attack enemy 0 in the next second, after which enemy 0 will go down, the number of damage points dealt to Bob is `3` points.\n* Attack enemy 1 in the next two seconds, after which enemy 1 will go down, the number of damage points dealt to Bob is `2 + 2 = 4` points.\n\n**Example 2:**\n\n**Input:** power \\= 1, damage \\= \\[1,1,1,1], health \\= \\[1,2,3,4]\n\n**Output:** 20\n\n**Explanation:**\n\n* Attack enemy 0 in the first second, after which enemy 0 will go down, the number of damage points dealt to Bob is `4` points.\n* Attack enemy 1 in the next two seconds, after which enemy 1 will go down, the number of damage points dealt to Bob is `3 + 3 = 6` points.\n* Attack enemy 2 in the next three seconds, after which enemy 2 will go down, the number of damage points dealt to Bob is `2 + 2 + 2 = 6` points.\n* Attack enemy 3 in the next four seconds, after which enemy 3 will go down, the number of damage points dealt to Bob is `1 + 1 + 1 + 1 = 4` points.\n\n**Example 3:**\n\n**Input:** power \\= 8, damage \\= \\[40], health \\= \\[59]\n\n**Output:** 320\n\n**Constraints:**\n\n* `1 <= power <= 104`\n* `1 <= n == damage.length == health.length <= 105`\n* `1 <= damage[i], health[i] <= 104`", "templates": {"cpp": "class Solution {\npublic:\n long long minDamage(int power, vector<int>& damage, vector<int>& health) {\n \n }\n};", "java": "class Solution {\n public long minDamage(int power, int[] damage, int[] health) {\n \n }\n}", "python": "class Solution:\n def minDamage(self, power: int, damage: List[int], health: List[int]) -> int:\n "}, "hints": "1\\. Can we use sorting here along with a custom comparator?\n2\\. For any two enemies `i` and `j` with damages `damage[i]` and `damage[j]`, and time to take each of them down `ti` and `tj`, when is it better to choose enemy `i` over enemy `j` first?", "contains_png": false, "png_links": []}
{"number": 3274, "title": "Check if Two Chessboard Squares Have the Same Color", "link": "https://leetcode.com/problems/check-if-two-chessboard-squares-have-the-same-color/", "difficulty": "easy", "statement": "You are given two strings, `coordinate1` and `coordinate2`, representing the coordinates of a square on an `8 x 8` chessboard.\n\nBelow is the chessboard for reference.\n\n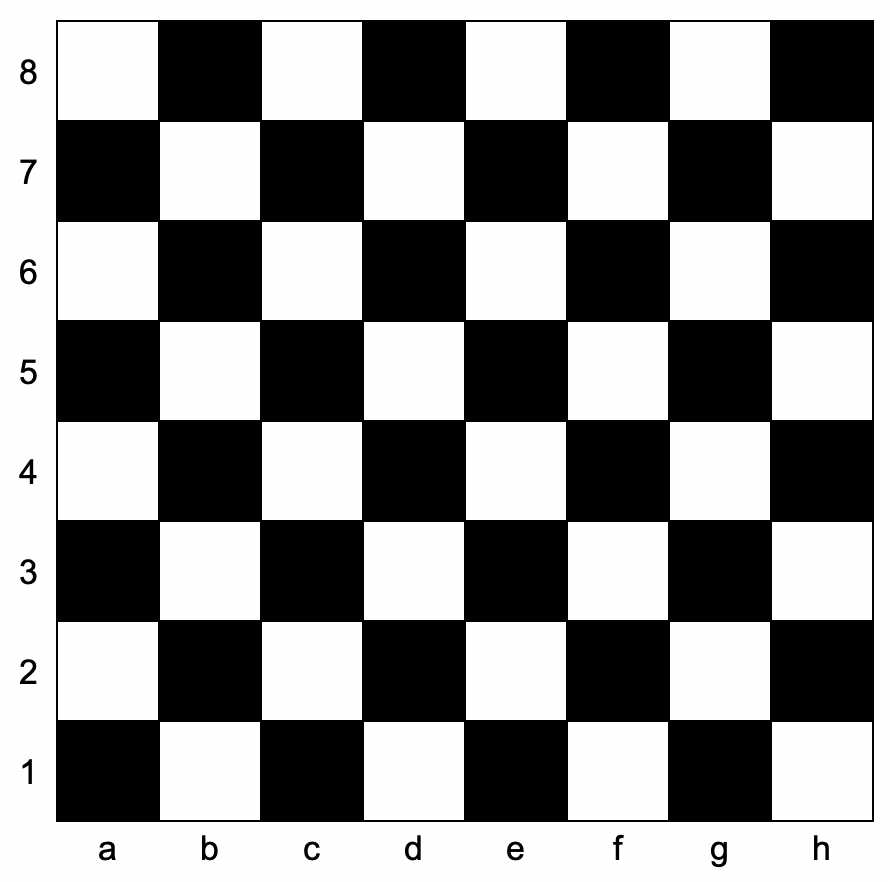\n\nReturn `true` if these two squares have the same color and `false` otherwise.\n\nThe coordinate will always represent a valid chessboard square. The coordinate will always have the letter first (indicating its column), and the number second (indicating its row).\n\n**Example 1:**\n\n**Input:** coordinate1 \\= \"a1\", coordinate2 \\= \"c3\"\n\n**Output:** true\n\n**Explanation:**\n\nBoth squares are black.\n\n**Example 2:**\n\n**Input:** coordinate1 \\= \"a1\", coordinate2 \\= \"h3\"\n\n**Output:** false\n\n**Explanation:**\n\nSquare `\"a1\"` is black and `\"h3\"` is white.\n\n**Constraints:**\n\n* `coordinate1.length == coordinate2.length == 2`\n* `'a' <= coordinate1[0], coordinate2[0] <= 'h'`\n* `'1' <= coordinate1[1], coordinate2[1] <= '8'`", "templates": {"cpp": "class Solution {\npublic:\n bool checkTwoChessboards(string coordinate1, string coordinate2) {\n \n }\n};", "java": "class Solution {\n public boolean checkTwoChessboards(String coordinate1, String coordinate2) {\n \n }\n}", "python": "class Solution:\n def checkTwoChessboards(self, coordinate1: str, coordinate2: str) -> bool:\n "}, "hints": "1\\. The color of the chessboard is black the sum of row coordinates and column coordinates is even. Otherwise, it's white.", "contains_png": true, "png_links": ["https://assets.leetcode.com/uploads/2024/07/17/screenshot-2021-02-20-at-22159-pm.png"]}
{"number": 3275, "title": "K-th Nearest Obstacle Queries", "link": "https://leetcode.com/problems/k-th-nearest-obstacle-queries/", "difficulty": "medium", "statement": "There is an infinite 2D plane.\n\nYou are given a positive integer `k`. You are also given a 2D array `queries`, which contains the following queries:\n\n* `queries[i] = [x, y]`: Build an obstacle at coordinate `(x, y)` in the plane. It is guaranteed that there is **no** obstacle at this coordinate when this query is made.\n\nAfter each query, you need to find the **distance** of the `kth` **nearest** obstacle from the origin.\n\nReturn an integer array `results` where `results[i]` denotes the `kth` nearest obstacle after query `i`, or `results[i] == -1` if there are less than `k` obstacles.\n\n**Note** that initially there are **no** obstacles anywhere.\n\nThe **distance** of an obstacle at coordinate `(x, y)` from the origin is given by `|x| + |y|`.\n\n**Example 1:**\n\n**Input:** queries \\= \\[\\[1,2],\\[3,4],\\[2,3],\\[\\-3,0]], k \\= 2\n\n**Output:** \\[\\-1,7,5,3]\n\n**Explanation:**\n\n* Initially, there are 0 obstacles.\n* After `queries[0]`, there are less than 2 obstacles.\n* After `queries[1]`, there are obstacles at distances 3 and 7\\.\n* After `queries[2]`, there are obstacles at distances 3, 5, and 7\\.\n* After `queries[3]`, there are obstacles at distances 3, 3, 5, and 7\\.\n\n**Example 2:**\n\n**Input:** queries \\= \\[\\[5,5],\\[4,4],\\[3,3]], k \\= 1\n\n**Output:** \\[10,8,6]\n\n**Explanation:**\n\n* After `queries[0]`, there is an obstacle at distance 10\\.\n* After `queries[1]`, there are obstacles at distances 8 and 10\\.\n* After `queries[2]`, there are obstacles at distances 6, 8, and 10\\.\n\n**Constraints:**\n\n* `1 <= queries.length <= 2 * 105`\n* All `queries[i]` are unique.\n* `-109 <= queries[i][0], queries[i][1] <= 109`\n* `1 <= k <= 105`", "templates": {"cpp": "class Solution {\npublic:\n vector<int> resultsArray(vector<vector<int>>& queries, int k) {\n \n }\n};", "java": "class Solution {\n public int[] resultsArray(int[][] queries, int k) {\n \n }\n}", "python": "class Solution:\n def resultsArray(self, queries: List[List[int]], k: int) -> List[int]:\n "}, "hints": "1\\. Consider if there are more than `k` obstacles. Can the `k + 1th` obstacle ever be the answer to any query?\n2\\. Maintain a max heap of size `k`, thus heap will contain minimum element at the top in that queue.\n3\\. Remove top element and insert new element from input array if current max is larger than this.", "contains_png": false, "png_links": []}
{"number": 3276, "title": "Select Cells in Grid With Maximum Score", "link": "https://leetcode.com/problems/select-cells-in-grid-with-maximum-score/", "difficulty": "hard", "statement": "You are given a 2D matrix `grid` consisting of positive integers.\n\nYou have to select *one or more* cells from the matrix such that the following conditions are satisfied:\n\n* No two selected cells are in the **same** row of the matrix.\n* The values in the set of selected cells are **unique**.\n\nYour score will be the **sum** of the values of the selected cells.\n\nReturn the **maximum** score you can achieve.\n\n**Example 1:**\n\n**Input:** grid \\= \\[\\[1,2,3],\\[4,3,2],\\[1,1,1]]\n\n**Output:** 8\n\n**Explanation:**\n\n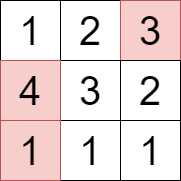\n\nWe can select the cells with values 1, 3, and 4 that are colored above.\n\n**Example 2:**\n\n**Input:** grid \\= \\[\\[8,7,6],\\[8,3,2]]\n\n**Output:** 15\n\n**Explanation:**\n\n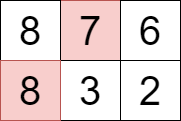\n\nWe can select the cells with values 7 and 8 that are colored above.\n\n**Constraints:**\n\n* `1 <= grid.length, grid[i].length <= 10`\n* `1 <= grid[i][j] <= 100`", "templates": {"cpp": "class Solution {\npublic:\n int maxScore(vector<vector<int>>& grid) {\n \n }\n};", "java": "class Solution {\n public int maxScore(List<List<Integer>> grid) {\n \n }\n}", "python": "class Solution:\n def maxScore(self, grid: List[List[int]]) -> int:\n "}, "hints": "1\\. Sort all the cells in the grid by their values and keep track of their original positions.\n2\\. Try dynamic programming with the following states: the current cell that we might select and a bitmask representing all the rows from which we have already selected a cell so far.", "contains_png": true, "png_links": ["https://assets.leetcode.com/uploads/2024/07/29/grid1drawio.png", "https://assets.leetcode.com/uploads/2024/07/29/grid8_8drawio.png"]}
{"number": 3277, "title": "Maximum XOR Score Subarray Queries", "link": "https://leetcode.com/problems/maximum-xor-score-subarray-queries/", "difficulty": "hard", "statement": "You are given an array `nums` of `n` integers, and a 2D integer array `queries` of size `q`, where `queries[i] = [li, ri]`.\n\nFor each query, you must find the **maximum XOR score** of any subarray of `nums[li..ri]`.\n\nThe **XOR score** of an array `a` is found by repeatedly applying the following operations on `a` so that only one element remains, that is the **score**:\n\n* Simultaneously replace `a[i]` with `a[i] XOR a[i + 1]` for all indices `i` except the last one.\n* Remove the last element of `a`.\n\nReturn an array `answer` of size `q` where `answer[i]` is the answer to query `i`.\n\n**Example 1:**\n\n**Input:** nums \\= \\[2,8,4,32,16,1], queries \\= \\[\\[0,2],\\[1,4],\\[0,5]]\n\n**Output:** \\[12,60,60]\n\n**Explanation:**\n\nIn the first query, `nums[0..2]` has 6 subarrays `[2]`, `[8]`, `[4]`, `[2, 8]`, `[8, 4]`, and `[2, 8, 4]` each with a respective XOR score of 2, 8, 4, 10, 12, and 6\\. The answer for the query is 12, the largest of all XOR scores.\n\nIn the second query, the subarray of `nums[1..4]` with the largest XOR score is `nums[1..4]` with a score of 60\\.\n\nIn the third query, the subarray of `nums[0..5]` with the largest XOR score is `nums[1..4]` with a score of 60\\.\n\n**Example 2:**\n\n**Input:** nums \\= \\[0,7,3,2,8,5,1], queries \\= \\[\\[0,3],\\[1,5],\\[2,4],\\[2,6],\\[5,6]]\n\n**Output:** \\[7,14,11,14,5]\n\n**Explanation:**\n\n| Index | nums\\[li..ri] | Maximum XOR Score Subarray | Maximum Subarray XOR Score |\n| --- | --- | --- | --- |\n| 0 | \\[0, 7, 3, 2] | \\[7] | 7 |\n| 1 | \\[7, 3, 2, 8, 5] | \\[7, 3, 2, 8] | 14 |\n| 2 | \\[3, 2, 8] | \\[3, 2, 8] | 11 |\n| 3 | \\[3, 2, 8, 5, 1] | \\[2, 8, 5, 1] | 14 |\n| 4 | \\[5, 1] | \\[5] | 5 |\n\n**Constraints:**\n\n* `1 <= n == nums.length <= 2000`\n* `0 <= nums[i] <= 231 - 1`\n* `1 <= q == queries.length <= 105`\n* `queries[i].length == 2`\n* `queries[i] = [li, ri]`\n* `0 <= li <= ri <= n - 1`", "templates": {"cpp": "class Solution {\npublic:\n vector<int> maximumSubarrayXor(vector<int>& nums, vector<vector<int>>& queries) {\n \n }\n};", "java": "class Solution {\n public int[] maximumSubarrayXor(int[] nums, int[][] queries) {\n \n }\n}", "python": "class Solution:\n def maximumSubarrayXor(self, nums: List[int], queries: List[List[int]]) -> List[int]:\n "}, "hints": "1\\. Precompute the XOR score of every subarray.\n2\\. Try to find a relationship between XOR score of `nums[i..j], nums[i..j + 1], nums[i..j + 2], \u2026`. Do you notice any pattern?\n3\\. If `dp[i][j]` is the XOR score of subarray `nums[i..j]`, `dp[i][j] = dp[i - 1][j] XOR dp[i - 1][j + 1]`.", "contains_png": false, "png_links": []}
{"number": 3280, "title": "Convert Date to Binary", "link": "https://leetcode.com/problems/convert-date-to-binary/", "difficulty": "easy", "statement": "You are given a string `date` representing a Gregorian calendar date in the `yyyy-mm-dd` format.\n\n`date` can be written in its binary representation obtained by converting year, month, and day to their binary representations without any leading zeroes and writing them down in `year-month-day` format.\n\nReturn the **binary** representation of `date`.\n\n**Example 1:**\n\n**Input:** date \\= \"2080\\-02\\-29\"\n\n**Output:** \"100000100000\\-10\\-11101\"\n\n**Explanation:**\n\n100000100000, 10, and 11101 are the binary representations of 2080, 02, and 29 respectively.\n\n**Example 2:**\n\n**Input:** date \\= \"1900\\-01\\-01\"\n\n**Output:** \"11101101100\\-1\\-1\"\n\n**Explanation:**\n\n11101101100, 1, and 1 are the binary representations of 1900, 1, and 1 respectively.\n\n**Constraints:**\n\n* `date.length == 10`\n* `date[4] == date[7] == '-'`, and all other `date[i]`'s are digits.\n* The input is generated such that `date` represents a valid Gregorian calendar date between Jan 1st, 1900 and Dec 31st, 2100 (both inclusive).", "templates": {"cpp": "class Solution {\npublic:\n string convertDateToBinary(string date) {\n \n }\n};", "java": "class Solution {\n public String convertDateToBinary(String date) {\n \n }\n}", "python": "class Solution:\n def convertDateToBinary(self, date: str) -> str:\n "}, "hints": null, "contains_png": false, "png_links": []}
{"number": 3281, "title": "Maximize Score of Numbers in Ranges", "link": "https://leetcode.com/problems/maximize-score-of-numbers-in-ranges/", "difficulty": "medium", "statement": "You are given an array of integers `start` and an integer `d`, representing `n` intervals `[start[i], start[i] + d]`.\n\nYou are asked to choose `n` integers where the `ith` integer must belong to the `ith` interval. The **score** of the chosen integers is defined as the **minimum** absolute difference between any two integers that have been chosen.\n\nReturn the **maximum** *possible score* of the chosen integers.\n\n**Example 1:**\n\n**Input:** start \\= \\[6,0,3], d \\= 2\n\n**Output:** 4\n\n**Explanation:**\n\nThe maximum possible score can be obtained by choosing integers: 8, 0, and 4\\. The score of these chosen integers is `min(|8 - 0|, |8 - 4|, |0 - 4|)` which equals 4\\.\n\n**Example 2:**\n\n**Input:** start \\= \\[2,6,13,13], d \\= 5\n\n**Output:** 5\n\n**Explanation:**\n\nThe maximum possible score can be obtained by choosing integers: 2, 7, 13, and 18\\. The score of these chosen integers is `min(|2 - 7|, |2 - 13|, |2 - 18|, |7 - 13|, |7 - 18|, |13 - 18|)` which equals 5\\.\n\n**Constraints:**\n\n* `2 <= start.length <= 105`\n* `0 <= start[i] <= 109`\n* `0 <= d <= 109`", "templates": {"cpp": "class Solution {\npublic:\n int maxPossibleScore(vector<int>& start, int d) {\n \n }\n};", "java": "class Solution {\n public int maxPossibleScore(int[] start, int d) {\n \n }\n}", "python": "class Solution:\n def maxPossibleScore(self, start: List[int], d: int) -> int:\n "}, "hints": "1\\. Can we use binary search here?\n2\\. Suppose that the answer is `x`. We can find a valid configuration of integers by sorting `start`, the first integer should be `start[0]`, then each subsequent integer should be the smallest one in `[start[i], start[i] + d]` that is greater than `last_chosen_value + x`.\n3\\. Binary search over `x`", "contains_png": false, "png_links": []}
{"number": 3282, "title": "Reach End of Array With Max Score", "link": "https://leetcode.com/problems/reach-end-of-array-with-max-score/", "difficulty": "medium", "statement": "You are given an integer array `nums` of length `n`.\n\nYour goal is to start at index `0` and reach index `n - 1`. You can only jump to indices **greater** than your current index.\n\nThe score for a jump from index `i` to index `j` is calculated as `(j - i) * nums[i]`.\n\nReturn the **maximum** possible **total score** by the time you reach the last index.\n\n**Example 1:**\n\n**Input:** nums \\= \\[1,3,1,5]\n\n**Output:** 7\n\n**Explanation:**\n\nFirst, jump to index 1 and then jump to the last index. The final score is `1 * 1 + 2 * 3 = 7`.\n\n**Example 2:**\n\n**Input:** nums \\= \\[4,3,1,3,2]\n\n**Output:** 16\n\n**Explanation:**\n\nJump directly to the last index. The final score is `4 * 4 = 16`.\n\n**Constraints:**\n\n* `1 <= nums.length <= 105`\n* `1 <= nums[i] <= 105`", "templates": {"cpp": "class Solution {\npublic:\n long long findMaximumScore(vector<int>& nums) {\n \n }\n};", "java": "class Solution {\n public long findMaximumScore(List<Integer> nums) {\n \n }\n}", "python": "class Solution:\n def findMaximumScore(self, nums: List[int]) -> int:\n "}, "hints": "1\\. It can be proven that from each index `i`, the optimal solution is to jump to the nearest index `j > i` such that `nums[j] > nums[i]`.", "contains_png": false, "png_links": []}
{"number": 3283, "title": "Maximum Number of Moves to Kill All Pawns", "link": "https://leetcode.com/problems/maximum-number-of-moves-to-kill-all-pawns/", "difficulty": "hard", "statement": "There is a `50 x 50` chessboard with **one** knight and some pawns on it. You are given two integers `kx` and `ky` where `(kx, ky)` denotes the position of the knight, and a 2D array `positions` where `positions[i] = [xi, yi]` denotes the position of the pawns on the chessboard.\n\nAlice and Bob play a *turn\\-based* game, where Alice goes first. In each player's turn:\n\n* The player *selects* a pawn that still exists on the board and captures it with the knight in the **fewest** possible **moves**. **Note** that the player can select **any** pawn, it **might not** be one that can be captured in the **least** number of moves.\n* In the process of capturing the *selected* pawn, the knight **may** pass other pawns **without** capturing them. **Only** the *selected* pawn can be captured in *this* turn.\n\nAlice is trying to **maximize** the **sum** of the number of moves made by *both* players until there are no more pawns on the board, whereas Bob tries to **minimize** them.\n\nReturn the **maximum** *total* number of moves made during the game that Alice can achieve, assuming both players play **optimally**.\n\nNote that in one **move,** a chess knight has eight possible positions it can move to, as illustrated below. Each move is two cells in a cardinal direction, then one cell in an orthogonal direction.\n\n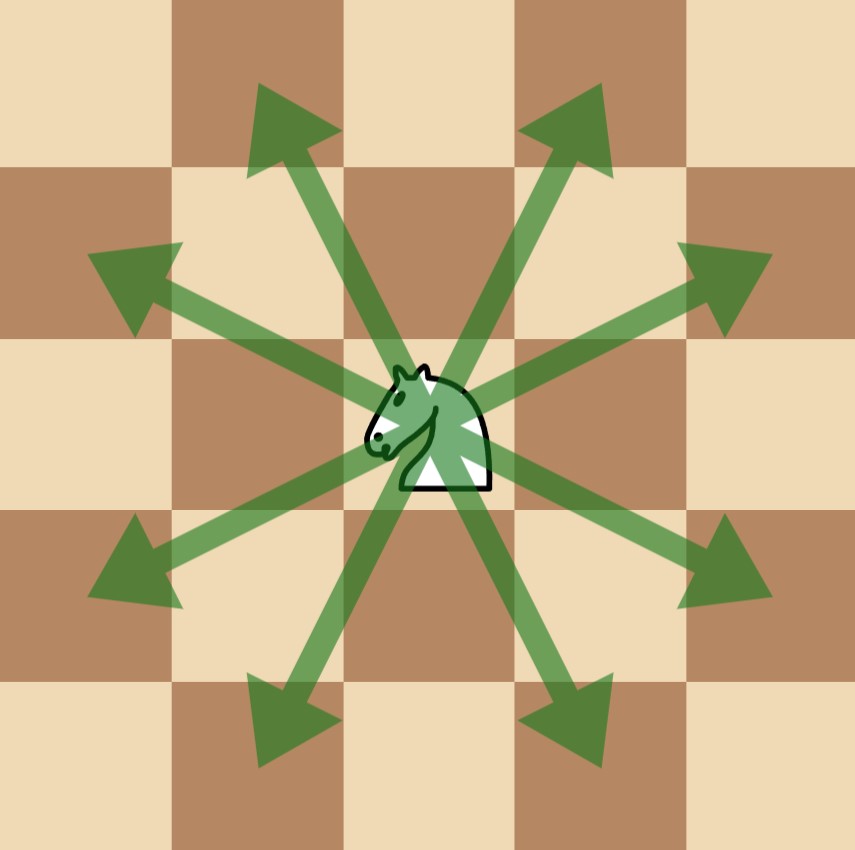\n\n**Example 1:**\n\n**Input:** kx \\= 1, ky \\= 1, positions \\= \\[\\[0,0]]\n\n**Output:** 4\n\n**Explanation:**\n\n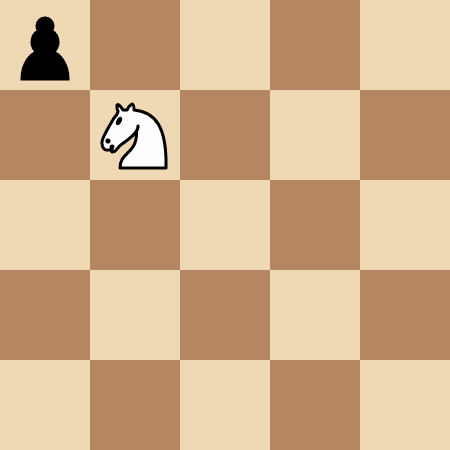\n\nThe knight takes 4 moves to reach the pawn at `(0, 0)`.\n\n**Example 2:**\n\n**Input:** kx \\= 0, ky \\= 2, positions \\= \\[\\[1,1],\\[2,2],\\[3,3]]\n\n**Output:** 8\n\n**Explanation:**\n\n**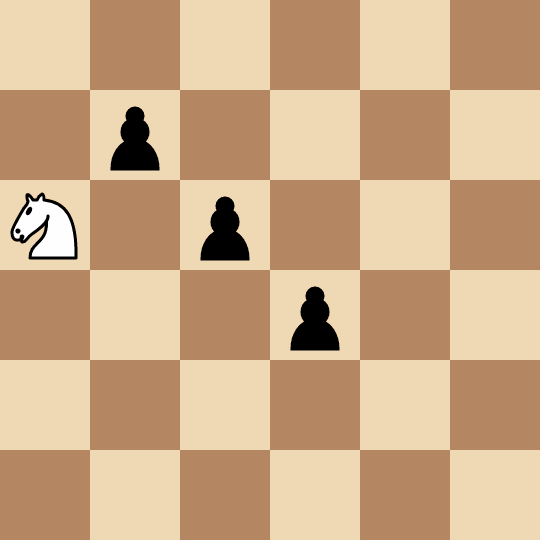**\n\n* Alice picks the pawn at `(2, 2)` and captures it in two moves: `(0, 2) -> (1, 4) -> (2, 2)`.\n* Bob picks the pawn at `(3, 3)` and captures it in two moves: `(2, 2) -> (4, 1) -> (3, 3)`.\n* Alice picks the pawn at `(1, 1)` and captures it in four moves: `(3, 3) -> (4, 1) -> (2, 2) -> (0, 3) -> (1, 1)`.\n\n**Example 3:**\n\n**Input:** kx \\= 0, ky \\= 0, positions \\= \\[\\[1,2],\\[2,4]]\n\n**Output:** 3\n\n**Explanation:**\n\n* Alice picks the pawn at `(2, 4)` and captures it in two moves: `(0, 0) -> (1, 2) -> (2, 4)`. Note that the pawn at `(1, 2)` is not captured.\n* Bob picks the pawn at `(1, 2)` and captures it in one move: `(2, 4) -> (1, 2)`.\n\n**Constraints:**\n\n* `0 <= kx, ky <= 49`\n* `1 <= positions.length <= 15`\n* `positions[i].length == 2`\n* `0 <= positions[i][0], positions[i][1] <= 49`\n* All `positions[i]` are unique.\n* The input is generated such that `positions[i] != [kx, ky]` for all `0 <= i < positions.length`.", "templates": {"cpp": "class Solution {\npublic:\n int maxMoves(int kx, int ky, vector<vector<int>>& positions) {\n \n }\n};", "java": "class Solution {\n public int maxMoves(int kx, int ky, int[][] positions) {\n \n }\n}", "python": "class Solution:\n def maxMoves(self, kx: int, ky: int, positions: List[List[int]]) -> int:\n "}, "hints": "1\\. Use BFS to preprocess the minimum number of moves to reach one pawn from the other pawns.\n2\\. Consider the knight\u2019s original position as another pawn.\n3\\. Use DP with a bitmask to store current pawns that have not been captured.", "contains_png": false, "png_links": []}
{"number": 3285, "title": "Find Indices of Stable Mountains", "link": "https://leetcode.com/problems/find-indices-of-stable-mountains/", "difficulty": "easy", "statement": "There are `n` mountains in a row, and each mountain has a height. You are given an integer array `height` where `height[i]` represents the height of mountain `i`, and an integer `threshold`.\n\nA mountain is called **stable** if the mountain just before it (**if it exists**) has a height **strictly greater** than `threshold`. **Note** that mountain 0 is **not** stable.\n\nReturn an array containing the indices of *all* **stable** mountains in **any** order.\n\n**Example 1:**\n\n**Input:** height \\= \\[1,2,3,4,5], threshold \\= 2\n\n**Output:** \\[3,4]\n\n**Explanation:**\n\n* Mountain 3 is stable because `height[2] == 3` is greater than `threshold == 2`.\n* Mountain 4 is stable because `height[3] == 4` is greater than `threshold == 2`.\n\n**Example 2:**\n\n**Input:** height \\= \\[10,1,10,1,10], threshold \\= 3\n\n**Output:** \\[1,3]\n\n**Example 3:**\n\n**Input:** height \\= \\[10,1,10,1,10], threshold \\= 10\n\n**Output:** \\[]\n\n**Constraints:**\n\n* `2 <= n == height.length <= 100`\n* `1 <= height[i] <= 100`\n* `1 <= threshold <= 100`", "templates": {"cpp": "class Solution {\npublic:\n vector<int> stableMountains(vector<int>& height, int threshold) {\n \n }\n};", "java": "class Solution {\n public List<Integer> stableMountains(int[] height, int threshold) {\n \n }\n}", "python": "class Solution:\n def stableMountains(self, height: List[int], threshold: int) -> List[int]:\n "}, "hints": null, "contains_png": false, "png_links": []}
{"number": 3286, "title": "Find a Safe Walk Through a Grid", "link": "https://leetcode.com/problems/find-a-safe-walk-through-a-grid/", "difficulty": "medium", "statement": "You are given an `m x n` binary matrix `grid` and an integer `health`.\n\nYou start on the upper\\-left corner `(0, 0)` and would like to get to the lower\\-right corner `(m - 1, n - 1)`.\n\nYou can move up, down, left, or right from one cell to another adjacent cell as long as your health *remains* **positive**.\n\nCells `(i, j)` with `grid[i][j] = 1` are considered **unsafe** and reduce your health by 1\\.\n\nReturn `true` if you can reach the final cell with a health value of 1 or more, and `false` otherwise.\n\n**Example 1:**\n\n**Input:** grid \\= \\[\\[0,1,0,0,0],\\[0,1,0,1,0],\\[0,0,0,1,0]], health \\= 1\n\n**Output:** true\n\n**Explanation:**\n\nThe final cell can be reached safely by walking along the gray cells below.\n\n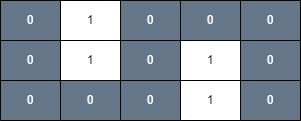\n**Example 2:**\n\n**Input:** grid \\= \\[\\[0,1,1,0,0,0],\\[1,0,1,0,0,0],\\[0,1,1,1,0,1],\\[0,0,1,0,1,0]], health \\= 3\n\n**Output:** false\n\n**Explanation:**\n\nA minimum of 4 health points is needed to reach the final cell safely.\n\n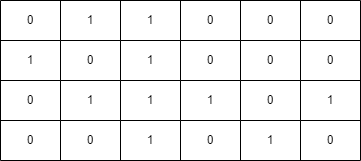\n**Example 3:**\n\n**Input:** grid \\= \\[\\[1,1,1],\\[1,0,1],\\[1,1,1]], health \\= 5\n\n**Output:** true\n\n**Explanation:**\n\nThe final cell can be reached safely by walking along the gray cells below.\n\n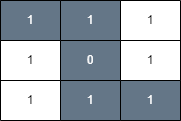\n\nAny path that does not go through the cell `(1, 1)` is unsafe since your health will drop to 0 when reaching the final cell.\n\n**Constraints:**\n\n* `m == grid.length`\n* `n == grid[i].length`\n* `1 <= m, n <= 50`\n* `2 <= m * n`\n* `1 <= health <= m + n`\n* `grid[i][j]` is either 0 or 1\\.", "templates": {"cpp": "class Solution {\npublic:\n bool findSafeWalk(vector<vector<int>>& grid, int health) {\n \n }\n};", "java": "class Solution {\n public boolean findSafeWalk(List<List<Integer>> grid, int health) {\n \n }\n}", "python": "class Solution:\n def findSafeWalk(self, grid: List[List[int]], health: int) -> bool:\n "}, "hints": "1\\. Use 01 BFS.", "contains_png": true, "png_links": ["https://assets.leetcode.com/uploads/2024/08/04/3868_examples_1drawio.png", "https://assets.leetcode.com/uploads/2024/08/04/3868_examples_2drawio.png", "https://assets.leetcode.com/uploads/2024/08/04/3868_examples_3drawio.png"]}
{"number": 3287, "title": "Find the Maximum Sequence Value of Array", "link": "https://leetcode.com/problems/find-the-maximum-sequence-value-of-array/", "difficulty": "hard", "statement": "You are given an integer array `nums` and a **positive** integer `k`.\n\nThe **value** of a sequence `seq` of size `2 * x` is defined as:\n\n* `(seq[0] OR seq[1] OR ... OR seq[x - 1]) XOR (seq[x] OR seq[x + 1] OR ... OR seq[2 * x - 1])`.\n\nReturn the **maximum** **value** of any subsequence of `nums` having size `2 * k`.\n\n**Example 1:**\n\n**Input:** nums \\= \\[2,6,7], k \\= 1\n\n**Output:** 5\n\n**Explanation:**\n\nThe subsequence `[2, 7]` has the maximum value of `2 XOR 7 = 5`.\n\n**Example 2:**\n\n**Input:** nums \\= \\[4,2,5,6,7], k \\= 2\n\n**Output:** 2\n\n**Explanation:**\n\nThe subsequence `[4, 5, 6, 7]` has the maximum value of `(4 OR 5) XOR (6 OR 7) = 2`.\n\n**Constraints:**\n\n* `2 <= nums.length <= 400`\n* `1 <= nums[i] < 27`\n* `1 <= k <= nums.length / 2`", "templates": {"cpp": "class Solution {\npublic:\n int maxValue(vector<int>& nums, int k) {\n \n }\n};", "java": "class Solution {\n public int maxValue(int[] nums, int k) {\n \n }\n}", "python": "class Solution:\n def maxValue(self, nums: List[int], k: int) -> int:\n "}, "hints": "1\\. Find all the possible `OR` till each `i` with `k` elements backward and forward.", "contains_png": false, "png_links": []}
{"number": 3288, "title": "Length of the Longest Increasing Path", "link": "https://leetcode.com/problems/length-of-the-longest-increasing-path/", "difficulty": "hard", "statement": "You are given a 2D array of integers `coordinates` of length `n` and an integer `k`, where `0 <= k < n`.\n\n`coordinates[i] = [xi, yi]` indicates the point `(xi, yi)` in a 2D plane.\n\nAn **increasing path** of length `m` is defined as a list of points `(x1, y1)`, `(x2, y2)`, `(x3, y3)`, ..., `(xm, ym)` such that:\n\n* `xi < xi + 1` and `yi < yi + 1` for all `i` where `1 <= i < m`.\n* `(xi, yi)` is in the given coordinates for all `i` where `1 <= i <= m`.\n\nReturn the **maximum** length of an **increasing path** that contains `coordinates[k]`.\n\n**Example 1:**\n\n**Input:** coordinates \\= \\[\\[3,1],\\[2,2],\\[4,1],\\[0,0],\\[5,3]], k \\= 1\n\n**Output:** 3\n\n**Explanation:**\n\n`(0, 0)`, `(2, 2)`, `(5, 3)` is the longest increasing path that contains `(2, 2)`.\n\n**Example 2:**\n\n**Input:** coordinates \\= \\[\\[2,1],\\[7,0],\\[5,6]], k \\= 2\n\n**Output:** 2\n\n**Explanation:**\n\n`(2, 1)`, `(5, 6)` is the longest increasing path that contains `(5, 6)`.\n\n**Constraints:**\n\n* `1 <= n == coordinates.length <= 105`\n* `coordinates[i].length == 2`\n* `0 <= coordinates[i][0], coordinates[i][1] <= 109`\n* All elements in `coordinates` are **distinct**.\n* `0 <= k <= n - 1`", "templates": {"cpp": "class Solution {\npublic:\n int maxPathLength(vector<vector<int>>& coordinates, int k) {\n \n }\n};", "java": "class Solution {\n public int maxPathLength(int[][] coordinates, int k) {\n \n }\n}", "python": "class Solution:\n def maxPathLength(self, coordinates: List[List[int]], k: int) -> int:\n "}, "hints": "1\\. Only keep coordinates with both `x` and `y` being strictly less than `coordinates[k]`.\n2\\. Sort them by `x`\u2019s, in the case of equal, the `y` values should be decreasing.\n3\\. Calculate LIS only using `y` values.\n4\\. Do the same for coordinates with both `x` and `y` being strictly larger than `coordinates[k]`.", "contains_png": false, "png_links": []}
{"number": 3289, "title": "The Two Sneaky Numbers of Digitville", "link": "https://leetcode.com/problems/the-two-sneaky-numbers-of-digitville/", "difficulty": "easy", "statement": "In the town of Digitville, there was a list of numbers called `nums` containing integers from `0` to `n - 1`. Each number was supposed to appear **exactly once** in the list, however, **two** mischievous numbers sneaked in an *additional time*, making the list longer than usual.\n\nAs the town detective, your task is to find these two sneaky numbers. Return an array of size **two** containing the two numbers (in *any order*), so peace can return to Digitville.\n\n**Example 1:**\n\n**Input:** nums \\= \\[0,1,1,0]\n\n**Output:** \\[0,1]\n\n**Explanation:**\n\nThe numbers 0 and 1 each appear twice in the array.\n\n**Example 2:**\n\n**Input:** nums \\= \\[0,3,2,1,3,2]\n\n**Output:** \\[2,3]\n\n**Explanation:** \n\nThe numbers 2 and 3 each appear twice in the array.\n\n**Example 3:**\n\n**Input:** nums \\= \\[7,1,5,4,3,4,6,0,9,5,8,2]\n\n**Output:** \\[4,5]\n\n**Explanation:** \n\nThe numbers 4 and 5 each appear twice in the array.\n\n**Constraints:**\n\n* `2 <= n <= 100`\n* `nums.length == n + 2`\n* `0 <= nums[i] < n`\n* The input is generated such that `nums` contains **exactly** two repeated elements.", "templates": {"cpp": "class Solution {\npublic:\n vector<int> getSneakyNumbers(vector<int>& nums) {\n \n }\n};", "java": "class Solution {\n public int[] getSneakyNumbers(int[] nums) {\n \n }\n}", "python": "class Solution:\n def getSneakyNumbers(self, nums: List[int]) -> List[int]:\n "}, "hints": "1\\. To solve the problem without the extra space, we need to think about how many times each number occurs in relation to the index.", "contains_png": false, "png_links": []}
{"number": 3290, "title": "Maximum Multiplication Score", "link": "https://leetcode.com/problems/maximum-multiplication-score/", "difficulty": "medium", "statement": "You are given an integer array `a` of size 4 and another integer array `b` of size **at least** 4\\.\n\nYou need to choose 4 indices `i0`, `i1`, `i2`, and `i3` from the array `b` such that `i0 < i1 < i2 < i3`. Your score will be equal to the value `a[0] * b[i0] + a[1] * b[i1] + a[2] * b[i2] + a[3] * b[i3]`.\n\nReturn the **maximum** score you can achieve.\n\n**Example 1:**\n\n**Input:** a \\= \\[3,2,5,6], b \\= \\[2,\\-6,4,\\-5,\\-3,2,\\-7]\n\n**Output:** 26\n\n**Explanation:** \n\nWe can choose the indices 0, 1, 2, and 5\\. The score will be `3 * 2 + 2 * (-6) + 5 * 4 + 6 * 2 = 26`.\n\n**Example 2:**\n\n**Input:** a \\= \\[\\-1,4,5,\\-2], b \\= \\[\\-5,\\-1,\\-3,\\-2,\\-4]\n\n**Output:** \\-1\n\n**Explanation:** \n\nWe can choose the indices 0, 1, 3, and 4\\. The score will be `(-1) * (-5) + 4 * (-1) + 5 * (-2) + (-2) * (-4) = -1`.\n\n**Constraints:**\n\n* `a.length == 4`\n* `4 <= b.length <= 105`\n* `-105 <= a[i], b[i] <= 105`", "templates": {"cpp": "class Solution {\npublic:\n long long maxScore(vector<int>& a, vector<int>& b) {\n \n }\n};", "java": "class Solution {\n public long maxScore(int[] a, int[] b) {\n \n }\n}", "python": "class Solution:\n def maxScore(self, a: List[int], b: List[int]) -> int:\n "}, "hints": "1\\. Try using dynamic programming.\n2\\. Consider a dp with the following states: The current position in the array b, and the number of indices considered.", "contains_png": false, "png_links": []}
{"number": 3291, "title": "Minimum Number of Valid Strings to Form Target I", "link": "https://leetcode.com/problems/minimum-number-of-valid-strings-to-form-target-i/", "difficulty": "medium", "statement": "You are given an array of strings `words` and a string `target`.\n\nA string `x` is called **valid** if `x` is a prefix of **any** string in `words`.\n\nReturn the **minimum** number of **valid** strings that can be *concatenated* to form `target`. If it is **not** possible to form `target`, return `-1`.\n\n**Example 1:**\n\n**Input:** words \\= \\[\"abc\",\"aaaaa\",\"bcdef\"], target \\= \"aabcdabc\"\n\n**Output:** 3\n\n**Explanation:**\n\nThe target string can be formed by concatenating:\n\n* Prefix of length 2 of `words[1]`, i.e. `\"aa\"`.\n* Prefix of length 3 of `words[2]`, i.e. `\"bcd\"`.\n* Prefix of length 3 of `words[0]`, i.e. `\"abc\"`.\n\n**Example 2:**\n\n**Input:** words \\= \\[\"abababab\",\"ab\"], target \\= \"ababaababa\"\n\n**Output:** 2\n\n**Explanation:**\n\nThe target string can be formed by concatenating:\n\n* Prefix of length 5 of `words[0]`, i.e. `\"ababa\"`.\n* Prefix of length 5 of `words[0]`, i.e. `\"ababa\"`.\n\n**Example 3:**\n\n**Input:** words \\= \\[\"abcdef\"], target \\= \"xyz\"\n\n**Output:** \\-1\n\n**Constraints:**\n\n* `1 <= words.length <= 100`\n* `1 <= words[i].length <= 5 * 103`\n* The input is generated such that `sum(words[i].length) <= 105`.\n* `words[i]` consists only of lowercase English letters.\n* `1 <= target.length <= 5 * 103`\n* `target` consists only of lowercase English letters.", "templates": {"cpp": "class Solution {\npublic:\n int minValidStrings(vector<string>& words, string target) {\n \n }\n};", "java": "class Solution {\n public int minValidStrings(String[] words, String target) {\n \n }\n}", "python": "class Solution:\n def minValidStrings(self, words: List[str], target: str) -> int:\n "}, "hints": "1\\. Let `dp[i]` be the minimum cost to form the prefix of length `i` of `target`.\n2\\. If `target[(i + 1)..j]` matches any prefix, update the range `dp[(i + 1)..j]` to minimum between original value and `dp[i] + 1`.\n3\\. Use a Trie to check prefix matching.", "contains_png": false, "png_links": []}
{"number": 3292, "title": "Minimum Number of Valid Strings to Form Target II", "link": "https://leetcode.com/problems/minimum-number-of-valid-strings-to-form-target-ii/", "difficulty": "hard", "statement": "You are given an array of strings `words` and a string `target`.\n\nA string `x` is called **valid** if `x` is a prefix of **any** string in `words`.\n\nReturn the **minimum** number of **valid** strings that can be *concatenated* to form `target`. If it is **not** possible to form `target`, return `-1`.\n\n**Example 1:**\n\n**Input:** words \\= \\[\"abc\",\"aaaaa\",\"bcdef\"], target \\= \"aabcdabc\"\n\n**Output:** 3\n\n**Explanation:**\n\nThe target string can be formed by concatenating:\n\n* Prefix of length 2 of `words[1]`, i.e. `\"aa\"`.\n* Prefix of length 3 of `words[2]`, i.e. `\"bcd\"`.\n* Prefix of length 3 of `words[0]`, i.e. `\"abc\"`.\n\n**Example 2:**\n\n**Input:** words \\= \\[\"abababab\",\"ab\"], target \\= \"ababaababa\"\n\n**Output:** 2\n\n**Explanation:**\n\nThe target string can be formed by concatenating:\n\n* Prefix of length 5 of `words[0]`, i.e. `\"ababa\"`.\n* Prefix of length 5 of `words[0]`, i.e. `\"ababa\"`.\n\n**Example 3:**\n\n**Input:** words \\= \\[\"abcdef\"], target \\= \"xyz\"\n\n**Output:** \\-1\n\n**Constraints:**\n\n* `1 <= words.length <= 100`\n* `1 <= words[i].length <= 5 * 104`\n* The input is generated such that `sum(words[i].length) <= 105`.\n* `words[i]` consists only of lowercase English letters.\n* `1 <= target.length <= 5 * 104`\n* `target` consists only of lowercase English letters.", "templates": {"cpp": "class Solution {\npublic:\n int minValidStrings(vector<string>& words, string target) {\n \n }\n};", "java": "class Solution {\n public int minValidStrings(String[] words, String target) {\n \n }\n}", "python": "class Solution:\n def minValidStrings(self, words: List[str], target: str) -> int:\n "}, "hints": "1\\. Let `dp[i]` be the minimum cost to form the prefix of length `i` of `target`.\n2\\. Use Rabin\\-Karp to hash every prefix and store it in a HashSet.\n3\\. Use Binary search to find the longest substring starting at index `i` (`target[i..j]`) that has a hash present in the HashSet.\n4\\. Inverse Modulo precomputation can optimise hash calculation.\n5\\. Use Lazy Segment Tree, or basic Segment Tree to update `dp[i..j]`.\n6\\. Is it possible to use two TreeSets to update `dp[i..j]`?", "contains_png": false, "png_links": []}
{"number": 3295, "title": "Report Spam Message", "link": "https://leetcode.com/problems/report-spam-message/", "difficulty": "medium", "statement": "You are given an array of strings `message` and an array of strings `bannedWords`.\n\nAn array of words is considered **spam** if there are **at least** two words in it that **exactly** match any word in `bannedWords`.\n\nReturn `true` if the array `message` is spam, and `false` otherwise.\n\n**Example 1:**\n\n**Input:** message \\= \\[\"hello\",\"world\",\"leetcode\"], bannedWords \\= \\[\"world\",\"hello\"]\n\n**Output:** true\n\n**Explanation:**\n\nThe words `\"hello\"` and `\"world\"` from the `message` array both appear in the `bannedWords` array.\n\n**Example 2:**\n\n**Input:** message \\= \\[\"hello\",\"programming\",\"fun\"], bannedWords \\= \\[\"world\",\"programming\",\"leetcode\"]\n\n**Output:** false\n\n**Explanation:**\n\nOnly one word from the `message` array (`\"programming\"`) appears in the `bannedWords` array.\n\n**Constraints:**\n\n* `1 <= message.length, bannedWords.length <= 105`\n* `1 <= message[i].length, bannedWords[i].length <= 15`\n* `message[i]` and `bannedWords[i]` consist only of lowercase English letters.", "templates": {"cpp": "class Solution {\npublic:\n bool reportSpam(vector<string>& message, vector<string>& bannedWords) {\n \n }\n};", "java": "class Solution {\n public boolean reportSpam(String[] message, String[] bannedWords) {\n \n }\n}", "python": "class Solution:\n def reportSpam(self, message: List[str], bannedWords: List[str]) -> bool:\n "}, "hints": "1\\. Use hash set.", "contains_png": false, "png_links": []}
{"number": 3296, "title": "Minimum Number of Seconds to Make Mountain Height Zero", "link": "https://leetcode.com/problems/minimum-number-of-seconds-to-make-mountain-height-zero/", "difficulty": "medium", "statement": "You are given an integer `mountainHeight` denoting the height of a mountain.\n\nYou are also given an integer array `workerTimes` representing the work time of workers in **seconds**.\n\nThe workers work **simultaneously** to **reduce** the height of the mountain. For worker `i`:\n\n* To decrease the mountain's height by `x`, it takes `workerTimes[i] + workerTimes[i] * 2 + ... + workerTimes[i] * x` seconds. For example:\n\t+ To reduce the height of the mountain by 1, it takes `workerTimes[i]` seconds.\n\t+ To reduce the height of the mountain by 2, it takes `workerTimes[i] + workerTimes[i] * 2` seconds, and so on.\n\nReturn an integer representing the **minimum** number of seconds required for the workers to make the height of the mountain 0\\.\n\n**Example 1:**\n\n**Input:** mountainHeight \\= 4, workerTimes \\= \\[2,1,1]\n\n**Output:** 3\n\n**Explanation:**\n\nOne way the height of the mountain can be reduced to 0 is:\n\n* Worker 0 reduces the height by 1, taking `workerTimes[0] = 2` seconds.\n* Worker 1 reduces the height by 2, taking `workerTimes[1] + workerTimes[1] * 2 = 3` seconds.\n* Worker 2 reduces the height by 1, taking `workerTimes[2] = 1` second.\n\nSince they work simultaneously, the minimum time needed is `max(2, 3, 1) = 3` seconds.\n\n**Example 2:**\n\n**Input:** mountainHeight \\= 10, workerTimes \\= \\[3,2,2,4]\n\n**Output:** 12\n\n**Explanation:**\n\n* Worker 0 reduces the height by 2, taking `workerTimes[0] + workerTimes[0] * 2 = 9` seconds.\n* Worker 1 reduces the height by 3, taking `workerTimes[1] + workerTimes[1] * 2 + workerTimes[1] * 3 = 12` seconds.\n* Worker 2 reduces the height by 3, taking `workerTimes[2] + workerTimes[2] * 2 + workerTimes[2] * 3 = 12` seconds.\n* Worker 3 reduces the height by 2, taking `workerTimes[3] + workerTimes[3] * 2 = 12` seconds.\n\nThe number of seconds needed is `max(9, 12, 12, 12) = 12` seconds.\n\n**Example 3:**\n\n**Input:** mountainHeight \\= 5, workerTimes \\= \\[1]\n\n**Output:** 15\n\n**Explanation:**\n\nThere is only one worker in this example, so the answer is `workerTimes[0] + workerTimes[0] * 2 + workerTimes[0] * 3 + workerTimes[0] * 4 + workerTimes[0] * 5 = 15`.\n\n**Constraints:**\n\n* `1 <= mountainHeight <= 105`\n* `1 <= workerTimes.length <= 104`\n* `1 <= workerTimes[i] <= 106`", "templates": {"cpp": "class Solution {\npublic:\n long long minNumberOfSeconds(int mountainHeight, vector<int>& workerTimes) {\n \n }\n};", "java": "class Solution {\n public long minNumberOfSeconds(int mountainHeight, int[] workerTimes) {\n \n }\n}", "python": "class Solution:\n def minNumberOfSeconds(self, mountainHeight: int, workerTimes: List[int]) -> int:\n "}, "hints": "1\\. Can we use binary search to solve this problem?\n2\\. Do a binary search on the number of seconds to check if it's enough to reduce the mountain height to 0 or less with all workers working simultaneously.", "contains_png": false, "png_links": []}
{"number": 3297, "title": "Count Substrings That Can Be Rearranged to Contain a String I", "link": "https://leetcode.com/problems/count-substrings-that-can-be-rearranged-to-contain-a-string-i/", "difficulty": "medium", "statement": "You are given two strings `word1` and `word2`.\n\nA string `x` is called **valid** if `x` can be rearranged to have `word2` as a prefix.\n\nReturn the total number of **valid** substrings of `word1`.\n\n**Example 1:**\n\n**Input:** word1 \\= \"bcca\", word2 \\= \"abc\"\n\n**Output:** 1\n\n**Explanation:**\n\nThe only valid substring is `\"bcca\"` which can be rearranged to `\"abcc\"` having `\"abc\"` as a prefix.\n\n**Example 2:**\n\n**Input:** word1 \\= \"abcabc\", word2 \\= \"abc\"\n\n**Output:** 10\n\n**Explanation:**\n\nAll the substrings except substrings of size 1 and size 2 are valid.\n\n**Example 3:**\n\n**Input:** word1 \\= \"abcabc\", word2 \\= \"aaabc\"\n\n**Output:** 0\n\n**Constraints:**\n\n* `1 <= word1.length <= 105`\n* `1 <= word2.length <= 104`\n* `word1` and `word2` consist only of lowercase English letters.", "templates": {"cpp": "class Solution {\npublic:\n long long validSubstringCount(string word1, string word2) {\n \n }\n};", "java": "class Solution {\n public long validSubstringCount(String word1, String word2) {\n \n }\n}", "python": "class Solution:\n def validSubstringCount(self, word1: str, word2: str) -> int:\n "}, "hints": "1\\. Store the frequency of each character for all prefixes.\n2\\. Use Binary Search.", "contains_png": false, "png_links": []}
{"number": 3298, "title": "Count Substrings That Can Be Rearranged to Contain a String II", "link": "https://leetcode.com/problems/count-substrings-that-can-be-rearranged-to-contain-a-string-ii/", "difficulty": "hard", "statement": "You are given two strings `word1` and `word2`.\n\nA string `x` is called **valid** if `x` can be rearranged to have `word2` as a prefix.\n\nReturn the total number of **valid** substrings of `word1`.\n\n**Note** that the memory limits in this problem are **smaller** than usual, so you **must** implement a solution with a *linear* runtime complexity.\n\n**Example 1:**\n\n**Input:** word1 \\= \"bcca\", word2 \\= \"abc\"\n\n**Output:** 1\n\n**Explanation:**\n\nThe only valid substring is `\"bcca\"` which can be rearranged to `\"abcc\"` having `\"abc\"` as a prefix.\n\n**Example 2:**\n\n**Input:** word1 \\= \"abcabc\", word2 \\= \"abc\"\n\n**Output:** 10\n\n**Explanation:**\n\nAll the substrings except substrings of size 1 and size 2 are valid.\n\n**Example 3:**\n\n**Input:** word1 \\= \"abcabc\", word2 \\= \"aaabc\"\n\n**Output:** 0\n\n**Constraints:**\n\n* `1 <= word1.length <= 106`\n* `1 <= word2.length <= 104`\n* `word1` and `word2` consist only of lowercase English letters.", "templates": {"cpp": "class Solution {\npublic:\n long long validSubstringCount(string word1, string word2) {\n \n }\n};", "java": "class Solution {\n public long validSubstringCount(String word1, String word2) {\n \n }\n}", "python": "class Solution:\n def validSubstringCount(self, word1: str, word2: str) -> int:\n "}, "hints": "1\\. Use sliding window along with two\\-pointer here.\n2\\. Use constant space to store the frequency of characters.", "contains_png": false, "png_links": []}
{"number": 3300, "title": "Minimum Element After Replacement With Digit Sum", "link": "https://leetcode.com/problems/minimum-element-after-replacement-with-digit-sum/", "difficulty": "easy", "statement": "You are given an integer array `nums`.\n\nYou replace each element in `nums` with the **sum** of its digits.\n\nReturn the **minimum** element in `nums` after all replacements.\n\n**Example 1:**\n\n**Input:** nums \\= \\[10,12,13,14]\n\n**Output:** 1\n\n**Explanation:**\n\n`nums` becomes `[1, 3, 4, 5]` after all replacements, with minimum element 1\\.\n\n**Example 2:**\n\n**Input:** nums \\= \\[1,2,3,4]\n\n**Output:** 1\n\n**Explanation:**\n\n`nums` becomes `[1, 2, 3, 4]` after all replacements, with minimum element 1\\.\n\n**Example 3:**\n\n**Input:** nums \\= \\[999,19,199]\n\n**Output:** 10\n\n**Explanation:**\n\n`nums` becomes `[27, 10, 19]` after all replacements, with minimum element 10\\.\n\n**Constraints:**\n\n* `1 <= nums.length <= 100`\n* `1 <= nums[i] <= 104`", "templates": {"cpp": "class Solution {\npublic:\n int minElement(vector<int>& nums) {\n \n }\n};", "java": "class Solution {\n public int minElement(int[] nums) {\n \n }\n}", "python": "class Solution:\n def minElement(self, nums: List[int]) -> int:\n "}, "hints": "1\\. Convert to string and calculate the sum for each element.", "contains_png": false, "png_links": []}
{"number": 3301, "title": "Maximize the Total Height of Unique Towers", "link": "https://leetcode.com/problems/maximize-the-total-height-of-unique-towers/", "difficulty": "medium", "statement": "You are given an array `maximumHeight`, where `maximumHeight[i]` denotes the **maximum** height the `ith` tower can be assigned.\n\nYour task is to assign a height to each tower so that:\n\n1. The height of the `ith` tower is a positive integer and does not exceed `maximumHeight[i]`.\n2. No two towers have the same height.\n\nReturn the **maximum** possible total sum of the tower heights. If it's not possible to assign heights, return `-1`.\n\n**Example 1:**\n\n**Input:** maximumHeight \\= \\[2,3,4,3]\n\n**Output:** 10\n\n**Explanation:**\n\nWe can assign heights in the following way: `[1, 2, 4, 3]`.\n\n**Example 2:**\n\n**Input:** maximumHeight \\= \\[15,10]\n\n**Output:** 25\n\n**Explanation:**\n\nWe can assign heights in the following way: `[15, 10]`.\n\n**Example 3:**\n\n**Input:** maximumHeight \\= \\[2,2,1]\n\n**Output:** \\-1\n\n**Explanation:**\n\nIt's impossible to assign positive heights to each index so that no two towers have the same height.\n\n**Constraints:**\n\n* `1 <= maximumHeight.length\u00a0<= 105`\n* `1 <= maximumHeight[i] <= 109`", "templates": {"cpp": "class Solution {\npublic:\n long long maximumTotalSum(vector<int>& maximumHeight) {\n \n }\n};", "java": "class Solution {\n public long maximumTotalSum(int[] maximumHeight) {\n \n }\n}", "python": "class Solution:\n def maximumTotalSum(self, maximumHeight: List[int]) -> int:\n "}, "hints": "1\\. Sort the array `maximumHeight` in descending order.\n2\\. After sorting, it can be seen that the maximum height that we can assign to the `ith` element is `min(maximumHeight[i], maximumHeight[i - 1] - 1)`.", "contains_png": false, "png_links": []}
{"number": 3302, "title": "Find the Lexicographically Smallest Valid Sequence", "link": "https://leetcode.com/problems/find-the-lexicographically-smallest-valid-sequence/", "difficulty": "medium", "statement": "You are given two strings `word1` and `word2`.\n\nA string `x` is called **almost equal** to `y` if you can change **at most** one character in `x` to make it *identical* to `y`.\n\nA sequence of indices `seq` is called **valid** if:\n\n* The indices are sorted in **ascending** order.\n* *Concatenating* the characters at these indices in `word1` in **the same** order results in a string that is **almost equal** to `word2`.\n\nReturn an array of size `word2.length` representing the lexicographically smallest **valid** sequence of indices. If no such sequence of indices exists, return an **empty** array.\n\n**Note** that the answer must represent the *lexicographically smallest array*, **not** the corresponding string formed by those indices.\n\n**Example 1:**\n\n**Input:** word1 \\= \"vbcca\", word2 \\= \"abc\"\n\n**Output:** \\[0,1,2]\n\n**Explanation:**\n\nThe lexicographically smallest valid sequence of indices is `[0, 1, 2]`:\n\n* Change `word1[0]` to `'a'`.\n* `word1[1]` is already `'b'`.\n* `word1[2]` is already `'c'`.\n\n**Example 2:**\n\n**Input:** word1 \\= \"bacdc\", word2 \\= \"abc\"\n\n**Output:** \\[1,2,4]\n\n**Explanation:**\n\nThe lexicographically smallest valid sequence of indices is `[1, 2, 4]`:\n\n* `word1[1]` is already `'a'`.\n* Change `word1[2]` to `'b'`.\n* `word1[4]` is already `'c'`.\n\n**Example 3:**\n\n**Input:** word1 \\= \"aaaaaa\", word2 \\= \"aaabc\"\n\n**Output:** \\[]\n\n**Explanation:**\n\nThere is no valid sequence of indices.\n\n**Example 4:**\n\n**Input:** word1 \\= \"abc\", word2 \\= \"ab\"\n\n**Output:** \\[0,1]\n\n**Constraints:**\n\n* `1 <= word2.length < word1.length <= 3 * 105`\n* `word1` and `word2` consist only of lowercase English letters.", "templates": {"cpp": "class Solution {\npublic:\n vector<int> validSequence(string word1, string word2) {\n \n }\n};", "java": "class Solution {\n public int[] validSequence(String word1, String word2) {\n \n }\n}", "python": "class Solution:\n def validSequence(self, word1: str, word2: str) -> List[int]:\n "}, "hints": "1\\. Let `dp[i]` be the longest suffix of `word2` that exists as a subsequence of suffix of the substring of `word1` starting at index `i`.\n2\\. If `dp[i + 1] < m` and `word1[i] == word2[m - dp[i + 1] - 1]`,`dp[i] = dp[i + 1] + 1`. Otherwise, `dp[i] = dp[i + 1]`.\n3\\. For each index `i`, greedily select characters using the `dp` array to know whether a solution exists.", "contains_png": false, "png_links": []}
{"number": 3303, "title": "Find the Occurrence of First Almost Equal Substring", "link": "https://leetcode.com/problems/find-the-occurrence-of-first-almost-equal-substring/", "difficulty": "hard", "statement": "You are given two strings `s` and `pattern`.\n\nA string `x` is called **almost equal** to `y` if you can change **at most** one character in `x` to make it *identical* to `y`.\n\nReturn the **smallest** *starting index* of a substring in `s` that is **almost equal** to `pattern`. If no such index exists, return `-1`.\n\nA **substring** is a contiguous **non\\-empty** sequence of characters within a string.\n\n**Example 1:**\n\n**Input:** s \\= \"abcdefg\", pattern \\= \"bcdffg\"\n\n**Output:** 1\n\n**Explanation:**\n\nThe substring `s[1..6] == \"bcdefg\"` can be converted to `\"bcdffg\"` by changing `s[4]` to `\"f\"`.\n\n**Example 2:**\n\n**Input:** s \\= \"ababbababa\", pattern \\= \"bacaba\"\n\n**Output:** 4\n\n**Explanation:**\n\nThe substring `s[4..9] == \"bababa\"` can be converted to `\"bacaba\"` by changing `s[6]` to `\"c\"`.\n\n**Example 3:**\n\n**Input:** s \\= \"abcd\", pattern \\= \"dba\"\n\n**Output:** \\-1\n\n**Example 4:**\n\n**Input:** s \\= \"dde\", pattern \\= \"d\"\n\n**Output:** 0\n\n**Constraints:**\n\n* `1 <= pattern.length < s.length <= 105`\n* `s` and `pattern` consist only of lowercase English letters.\n\n**Follow\\-up:** Could you solve the problem if **at most** `k` **consecutive** characters can be changed?", "templates": {"cpp": "class Solution {\npublic:\n int minStartingIndex(string s, string pattern) {\n \n }\n};", "java": "class Solution {\n public int minStartingIndex(String s, String pattern) {\n \n }\n}", "python": "class Solution:\n def minStartingIndex(self, s: str, pattern: str) -> int:\n "}, "hints": "1\\. Let `dp1[i]` represent the maximum length of a substring of `s` starting at index `i` that is also a prefix of `pattern`.\n2\\. Let `dp2[i]` represent the maximum length of a substring of `s` ending at index `i` that is also a suffix of `pattern`.\n3\\. Consider a window of size `pattern.length`. If `dp1[i] + i == i + pattern.length - 1 - dp2[i + pattern.length - 1]`, what does this signify?", "contains_png": false, "png_links": []}
{"number": 3304, "title": "Find the K-th Character in String Game I", "link": "https://leetcode.com/problems/find-the-k-th-character-in-string-game-i/", "difficulty": "easy", "statement": "Alice and Bob are playing a game. Initially, Alice has a string `word = \"a\"`.\n\nYou are given a **positive** integer `k`.\n\nNow Bob will ask Alice to perform the following operation **forever**:\n\n* Generate a new string by **changing** each character in `word` to its **next** character in the English alphabet, and **append** it to the *original* `word`.\n\nFor example, performing the operation on `\"c\"` generates `\"cd\"` and performing the operation on `\"zb\"` generates `\"zbac\"`.\n\nReturn the value of the `kth` character in `word`, after enough operations have been done for `word` to have **at least** `k` characters.\n\n**Note** that the character `'z'` can be changed to `'a'` in the operation.\n\n**Example 1:**\n\n**Input:** k \\= 5\n\n**Output:** \"b\"\n\n**Explanation:**\n\nInitially, `word = \"a\"`. We need to do the operation three times:\n\n* Generated string is `\"b\"`, `word` becomes `\"ab\"`.\n* Generated string is `\"bc\"`, `word` becomes `\"abbc\"`.\n* Generated string is `\"bccd\"`, `word` becomes `\"abbcbccd\"`.\n\n**Example 2:**\n\n**Input:** k \\= 10\n\n**Output:** \"c\"\n\n**Constraints:**\n\n* `1 <= k <= 500`", "templates": {"cpp": "class Solution {\npublic:\n char kthCharacter(int k) {\n \n }\n};", "java": "class Solution {\n public char kthCharacter(int k) {\n \n }\n}", "python": "class Solution:\n def kthCharacter(self, k: int) -> str:\n "}, "hints": "1\\. The constraints are small. Construct the string by simulating the operations.", "contains_png": false, "png_links": []}
{"number": 3305, "title": "Count of Substrings Containing Every Vowel and K Consonants I", "link": "https://leetcode.com/problems/count-of-substrings-containing-every-vowel-and-k-consonants-i/", "difficulty": "medium", "statement": "You are given a string `word` and a **non\\-negative** integer `k`.\n\nReturn the total number of substrings of `word` that contain every vowel (`'a'`, `'e'`, `'i'`, `'o'`, and `'u'`) **at least** once and **exactly** `k` consonants.\n\n**Example 1:**\n\n**Input:** word \\= \"aeioqq\", k \\= 1\n\n**Output:** 0\n\n**Explanation:**\n\nThere is no substring with every vowel.\n\n**Example 2:**\n\n**Input:** word \\= \"aeiou\", k \\= 0\n\n**Output:** 1\n\n**Explanation:**\n\nThe only substring with every vowel and zero consonants is `word[0..4]`, which is `\"aeiou\"`.\n\n**Example 3:**\n\n**Input:** word \\= \"ieaouqqieaouqq\", k \\= 1\n\n**Output:** 3\n\n**Explanation:**\n\nThe substrings with every vowel and one consonant are:\n\n* `word[0..5]`, which is `\"ieaouq\"`.\n* `word[6..11]`, which is `\"qieaou\"`.\n* `word[7..12]`, which is `\"ieaouq\"`.\n\n**Constraints:**\n\n* `5 <= word.length <= 250`\n* `word` consists only of lowercase English letters.\n* `0 <= k <= word.length - 5`", "templates": {"cpp": "class Solution {\npublic:\n int countOfSubstrings(string word, int k) {\n \n }\n};", "java": "class Solution {\n public int countOfSubstrings(String word, int k) {\n \n }\n}", "python": "class Solution:\n def countOfSubstrings(self, word: str, k: int) -> int:\n "}, "hints": "1\\. Use a HashMap and check all the substrings.", "contains_png": false, "png_links": []}
{"number": 3306, "title": "Count of Substrings Containing Every Vowel and K Consonants II", "link": "https://leetcode.com/problems/count-of-substrings-containing-every-vowel-and-k-consonants-ii/", "difficulty": "medium", "statement": "You are given a string `word` and a **non\\-negative** integer `k`.\n\nReturn the total number of substrings of `word` that contain every vowel (`'a'`, `'e'`, `'i'`, `'o'`, and `'u'`) **at least** once and **exactly** `k` consonants.\n\n**Example 1:**\n\n**Input:** word \\= \"aeioqq\", k \\= 1\n\n**Output:** 0\n\n**Explanation:**\n\nThere is no substring with every vowel.\n\n**Example 2:**\n\n**Input:** word \\= \"aeiou\", k \\= 0\n\n**Output:** 1\n\n**Explanation:**\n\nThe only substring with every vowel and zero consonants is `word[0..4]`, which is `\"aeiou\"`.\n\n**Example 3:**\n\n**Input:** word \\= \"ieaouqqieaouqq\", k \\= 1\n\n**Output:** 3\n\n**Explanation:**\n\nThe substrings with every vowel and one consonant are:\n\n* `word[0..5]`, which is `\"ieaouq\"`.\n* `word[6..11]`, which is `\"qieaou\"`.\n* `word[7..12]`, which is `\"ieaouq\"`.\n\n**Constraints:**\n\n* `5 <= word.length <= 2 * 105`\n* `word` consists only of lowercase English letters.\n* `0 <= k <= word.length - 5`", "templates": {"cpp": "class Solution {\npublic:\n long long countOfSubstrings(string word, int k) {\n \n }\n};", "java": "class Solution {\n public long countOfSubstrings(String word, int k) {\n \n }\n}", "python": "class Solution:\n def countOfSubstrings(self, word: str, k: int) -> int:\n "}, "hints": "1\\. We can use sliding window and binary search.\n2\\. For each index `r`, find the maximum `l` such that both conditions are satisfied using binary search.", "contains_png": false, "png_links": []}
{"number": 3307, "title": "Find the K-th Character in String Game II", "link": "https://leetcode.com/problems/find-the-k-th-character-in-string-game-ii/", "difficulty": "hard", "statement": "Alice and Bob are playing a game. Initially, Alice has a string `word = \"a\"`.\n\nYou are given a **positive** integer `k`. You are also given an integer array `operations`, where `operations[i]` represents the **type** of the `ith` operation.\n\nNow Bob will ask Alice to perform **all** operations in sequence:\n\n* If `operations[i] == 0`, **append** a copy of `word` to itself.\n* If `operations[i] == 1`, generate a new string by **changing** each character in `word` to its **next** character in the English alphabet, and **append** it to the *original* `word`. For example, performing the operation on `\"c\"` generates `\"cd\"` and performing the operation on `\"zb\"` generates `\"zbac\"`.\n\nReturn the value of the `kth` character in `word` after performing all the operations.\n\n**Note** that the character `'z'` can be changed to `'a'` in the second type of operation.\n\n**Example 1:**\n\n**Input:** k \\= 5, operations \\= \\[0,0,0]\n\n**Output:** \"a\"\n\n**Explanation:**\n\nInitially, `word == \"a\"`. Alice performs the three operations as follows:\n\n* Appends `\"a\"` to `\"a\"`, `word` becomes `\"aa\"`.\n* Appends `\"aa\"` to `\"aa\"`, `word` becomes `\"aaaa\"`.\n* Appends `\"aaaa\"` to `\"aaaa\"`, `word` becomes `\"aaaaaaaa\"`.\n\n**Example 2:**\n\n**Input:** k \\= 10, operations \\= \\[0,1,0,1]\n\n**Output:** \"b\"\n\n**Explanation:**\n\nInitially, `word == \"a\"`. Alice performs the four operations as follows:\n\n* Appends `\"a\"` to `\"a\"`, `word` becomes `\"aa\"`.\n* Appends `\"bb\"` to `\"aa\"`, `word` becomes `\"aabb\"`.\n* Appends `\"aabb\"` to `\"aabb\"`, `word` becomes `\"aabbaabb\"`.\n* Appends `\"bbccbbcc\"` to `\"aabbaabb\"`, `word` becomes `\"aabbaabbbbccbbcc\"`.\n\n**Constraints:**\n\n* `1 <= k <= 1014`\n* `1 <= operations.length <= 100`\n* `operations[i]` is either 0 or 1\\.\n* The input is generated such that `word` has **at least** `k` characters after all operations.", "templates": {"cpp": "class Solution {\npublic:\n char kthCharacter(long long k, vector<int>& operations) {\n \n }\n};", "java": "class Solution {\n public char kthCharacter(long k, int[] operations) {\n \n }\n}", "python": "class Solution:\n def kthCharacter(self, k: int, operations: List[int]) -> str:\n "}, "hints": "1\\. Try to replay the operations `kth` character was part of.\n2\\. The `kth` character is only affected if it is present in the first half of the string.", "contains_png": false, "png_links": []}
{"number": 3309, "title": "Maximum Possible Number by Binary Concatenation", "link": "https://leetcode.com/problems/maximum-possible-number-by-binary-concatenation/", "difficulty": "medium", "statement": "You are given an array of integers `nums` of size 3\\.\n\nReturn the **maximum** possible number whose *binary representation* can be formed by **concatenating** the *binary representation* of **all** elements in `nums` in some order.\n\n**Note** that the binary representation of any number *does not* contain leading zeros.\n\n**Example 1:**\n\n**Input:** nums \\= \\[1,2,3]\n\n**Output:** 30\n\n**Explanation:**\n\nConcatenate the numbers in the order `[3, 1, 2]` to get the result `\"11110\"`, which is the binary representation of 30\\.\n\n**Example 2:**\n\n**Input:** nums \\= \\[2,8,16]\n\n**Output:** 1296\n\n**Explanation:**\n\nConcatenate the numbers in the order `[2, 8, 16]` to get the result `\"10100010000\"`, which is the binary representation of 1296\\.\n\n**Constraints:**\n\n* `nums.length == 3`\n* `1 <= nums[i] <= 127`", "templates": {"cpp": "class Solution {\npublic:\n int maxGoodNumber(vector<int>& nums) {\n \n }\n};", "java": "class Solution {\n public int maxGoodNumber(int[] nums) {\n \n }\n}", "python": "class Solution:\n def maxGoodNumber(self, nums: List[int]) -> int:\n "}, "hints": "1\\. How many possible concatenation orders are there?", "contains_png": false, "png_links": []}
{"number": 3310, "title": "Remove Methods From Project", "link": "https://leetcode.com/problems/remove-methods-from-project/", "difficulty": "medium", "statement": "You are maintaining a project that has `n` methods numbered from `0` to `n - 1`.\n\nYou are given two integers `n` and `k`, and a 2D integer array `invocations`, where `invocations[i] = [ai, bi]` indicates that method `ai` invokes method `bi`.\n\nThere is a known bug in method `k`. Method `k`, along with any method invoked by it, either **directly** or **indirectly**, are considered **suspicious** and we aim to remove them.\n\nA group of methods can only be removed if no method **outside** the group invokes any methods **within** it.\n\nReturn an array containing all the remaining methods after removing all the **suspicious** methods. You may return the answer in *any order*. If it is not possible to remove **all** the suspicious methods, **none** should be removed.\n\n**Example 1:**\n\n**Input:** n \\= 4, k \\= 1, invocations \\= \\[\\[1,2],\\[0,1],\\[3,2]]\n\n**Output:** \\[0,1,2,3]\n\n**Explanation:**\n\n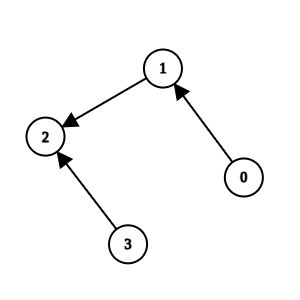\n\nMethod 2 and method 1 are suspicious, but they are directly invoked by methods 3 and 0, which are not suspicious. We return all elements without removing anything.\n\n**Example 2:**\n\n**Input:** n \\= 5, k \\= 0, invocations \\= \\[\\[1,2],\\[0,2],\\[0,1],\\[3,4]]\n\n**Output:** \\[3,4]\n\n**Explanation:**\n\n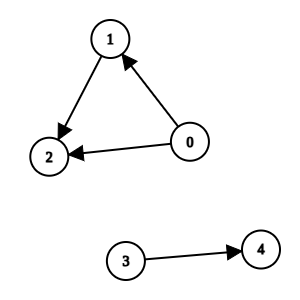\n\nMethods 0, 1, and 2 are suspicious and they are not directly invoked by any other method. We can remove them.\n\n**Example 3:**\n\n**Input:** n \\= 3, k \\= 2, invocations \\= \\[\\[1,2],\\[0,1],\\[2,0]]\n\n**Output:** \\[]\n\n**Explanation:**\n\n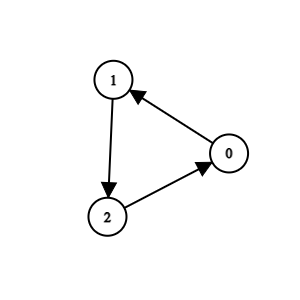\n\nAll methods are suspicious. We can remove them.\n\n**Constraints:**\n\n* `1 <= n <= 105`\n* `0 <= k <= n - 1`\n* `0 <= invocations.length <= 2 * 105`\n* `invocations[i] == [ai, bi]`\n* `0 <= ai, bi <= n - 1`\n* `ai != bi`\n* `invocations[i] != invocations[j]`", "templates": {"cpp": "class Solution {\npublic:\n vector<int> remainingMethods(int n, int k, vector<vector<int>>& invocations) {\n \n }\n};", "java": "class Solution {\n public List<Integer> remainingMethods(int n, int k, int[][] invocations) {\n \n }\n}", "python": "class Solution:\n def remainingMethods(self, n: int, k: int, invocations: List[List[int]]) -> List[int]:\n "}, "hints": "1\\. Use DFS from node `k`.\n2\\. Mark all the nodes visited from node `k`, and then check if they can be visited from the other nodes.", "contains_png": true, "png_links": ["https://assets.leetcode.com/uploads/2024/07/18/graph-2.png", "https://assets.leetcode.com/uploads/2024/07/18/graph-3.png", "https://assets.leetcode.com/uploads/2024/07/20/graph.png"]}
{"number": 3311, "title": "Construct 2D Grid Matching Graph Layout", "link": "https://leetcode.com/problems/construct-2d-grid-matching-graph-layout/", "difficulty": "hard", "statement": "You are given a 2D integer array `edges` representing an **undirected** graph having `n` nodes, where `edges[i] = [ui, vi]` denotes an edge between nodes `ui` and `vi`.\n\nConstruct a 2D grid that satisfies these conditions:\n\n* The grid contains **all nodes** from `0` to `n - 1` in its cells, with each node appearing exactly **once**.\n* Two nodes should be in adjacent grid cells (**horizontally** or **vertically**) **if and only if** there is an edge between them in `edges`.\n\nIt is guaranteed that `edges` can form a 2D grid that satisfies the conditions.\n\nReturn a 2D integer array satisfying the conditions above. If there are multiple solutions, return *any* of them.\n\n**Example 1:**\n\n**Input:** n \\= 4, edges \\= \\[\\[0,1],\\[0,2],\\[1,3],\\[2,3]]\n\n**Output:** \\[\\[3,1],\\[2,0]]\n\n**Explanation:**\n\n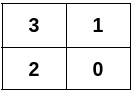\n\n**Example 2:**\n\n**Input:** n \\= 5, edges \\= \\[\\[0,1],\\[1,3],\\[2,3],\\[2,4]]\n\n**Output:** \\[\\[4,2,3,1,0]]\n\n**Explanation:**\n\n\n\n**Example 3:**\n\n**Input:** n \\= 9, edges \\= \\[\\[0,1],\\[0,4],\\[0,5],\\[1,7],\\[2,3],\\[2,4],\\[2,5],\\[3,6],\\[4,6],\\[4,7],\\[6,8],\\[7,8]]\n\n**Output:** \\[\\[8,6,3],\\[7,4,2],\\[1,0,5]]\n\n**Explanation:**\n\n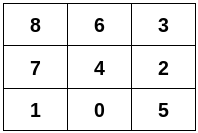\n\n**Constraints:**\n\n* `2 <= n <= 5 * 104`\n* `1 <= edges.length <= 105`\n* `edges[i] = [ui, vi]`\n* `0 <= ui < vi < n`\n* All the edges are distinct.\n* The input is generated such that `edges` can form a 2D grid that satisfies the conditions.", "templates": {"cpp": "class Solution {\npublic:\n vector<vector<int>> constructGridLayout(int n, vector<vector<int>>& edges) {\n \n }\n};", "java": "class Solution {\n public int[][] constructGridLayout(int n, int[][] edges) {\n \n }\n}", "python": "class Solution:\n def constructGridLayout(self, n: int, edges: List[List[int]]) -> List[List[int]]:\n "}, "hints": "1\\. Observe the indegrees of the nodes.\n2\\. The case where there are two nodes with an indegree of 1, and all the others have an indegree of 2 can be handled separately.\n3\\. The nodes with the smallest degrees are the corners.\n4\\. You can simulate the grid creation process using BFS or a similar approach after making some observations on the indegrees.", "contains_png": true, "png_links": ["https://assets.leetcode.com/uploads/2024/08/11/screenshot-from-2024-08-11-14-07-59.png", "https://assets.leetcode.com/uploads/2024/08/11/screenshot-from-2024-08-11-14-06-02.png", "https://assets.leetcode.com/uploads/2024/08/11/screenshot-from-2024-08-11-14-06-38.png"]}
{"number": 3312, "title": "Sorted GCD Pair Queries", "link": "https://leetcode.com/problems/sorted-gcd-pair-queries/", "difficulty": "hard", "statement": "You are given an integer array `nums` of length `n` and an integer array `queries`.\n\nLet `gcdPairs` denote an array obtained by calculating the GCD of all possible pairs `(nums[i], nums[j])`, where `0 <= i < j < n`, and then sorting these values in **ascending** order.\n\nFor each query `queries[i]`, you need to find the element at index `queries[i]` in `gcdPairs`.\n\nReturn an integer array `answer`, where `answer[i]` is the value at `gcdPairs[queries[i]]` for each query.\n\nThe term `gcd(a, b)` denotes the **greatest common divisor** of `a` and `b`.\n\n**Example 1:**\n\n**Input:** nums \\= \\[2,3,4], queries \\= \\[0,2,2]\n\n**Output:** \\[1,2,2]\n\n**Explanation:**\n\n`gcdPairs = [gcd(nums[0], nums[1]), gcd(nums[0], nums[2]), gcd(nums[1], nums[2])] = [1, 2, 1]`.\n\nAfter sorting in ascending order, `gcdPairs = [1, 1, 2]`.\n\nSo, the answer is `[gcdPairs[queries[0]], gcdPairs[queries[1]], gcdPairs[queries[2]]] = [1, 2, 2]`.\n\n**Example 2:**\n\n**Input:** nums \\= \\[4,4,2,1], queries \\= \\[5,3,1,0]\n\n**Output:** \\[4,2,1,1]\n\n**Explanation:**\n\n`gcdPairs` sorted in ascending order is `[1, 1, 1, 2, 2, 4]`.\n\n**Example 3:**\n\n**Input:** nums \\= \\[2,2], queries \\= \\[0,0]\n\n**Output:** \\[2,2]\n\n**Explanation:**\n\n`gcdPairs = [2]`.\n\n**Constraints:**\n\n* `2 <= n == nums.length <= 105`\n* `1 <= nums[i] <= 5 * 104`\n* `1 <= queries.length <= 105`\n* `0 <= queries[i] < n * (n - 1) / 2`", "templates": {"cpp": "class Solution {\npublic:\n vector<int> gcdValues(vector<int>& nums, vector<long long>& queries) {\n \n }\n};", "java": "class Solution {\n public int[] gcdValues(int[] nums, long[] queries) {\n \n }\n}", "python": "class Solution:\n def gcdValues(self, nums: List[int], queries: List[int]) -> List[int]:\n "}, "hints": "1\\. Try counting the number of pairs that have a GCD of `g</code.\n2. Use inclusion-exclusion.`", "contains_png": false, "png_links": []}