diff --git a/README.md b/README.md
index e542e65..264d192 100644
--- a/README.md
+++ b/README.md
@@ -1,9 +1,17 @@
-# Flutter Any Logo 💯
+
+
+
+
+
+
+
+ # Flutter Any Logo 💯
[](https://github.com/JordyHers/flutter_any_logo/actions/workflows/deploy_dev.yml)
-## Our aim is to implement 1000+ logos but keep the package as light as possible. 🪽
+### Our aim is to implement 1000+ logos but keep the package as light as possible. 🪽
+
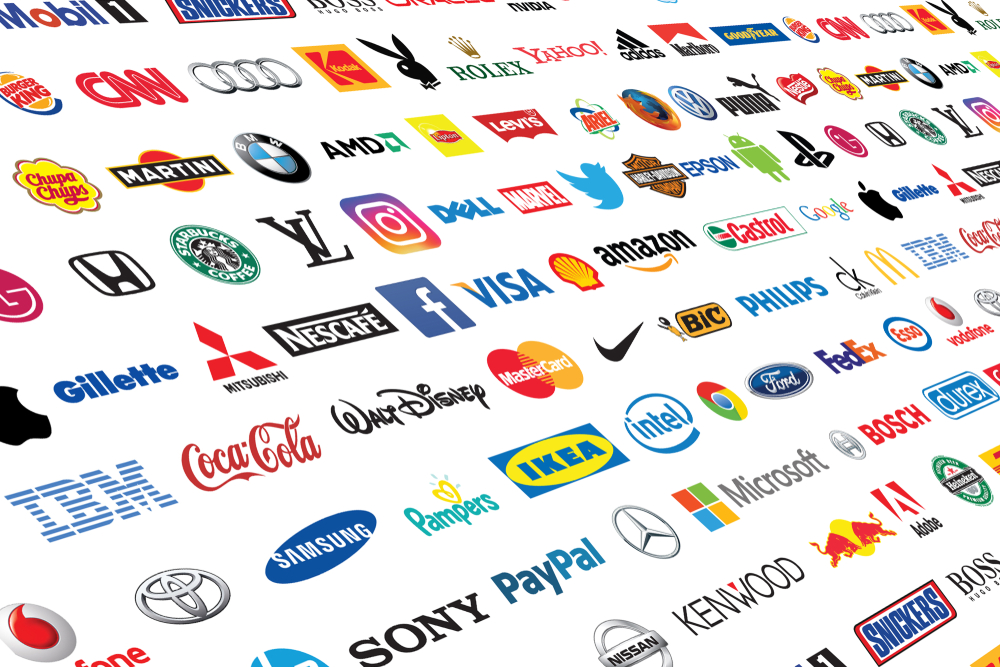
@@ -31,7 +39,7 @@ Add `flutter_any_logo` as a dependency in your `pubspec.yaml` file.
```
dependencies:
- flutter_any_logo: ^1.0.0
+ flutter_any_logo: ^1.0.3
```
Then, run `flutter pub get` in your terminal to install the plugin.
@@ -116,7 +124,7 @@ AnyLogo.uefa.manchesterUnited.image()
// int? cacheWidth,
// int? cacheHeight,
// All the variables are sill available
-AnyLogo.nba.atlantaHawks.image(height: 30, fit: BoxFit.contain);
+AnyLogo.nba.atlantaHawks.image(height: 30, width: 25, fit: BoxFit.contain);
//You can still pass just the asset image without calling [.image()]
@@ -251,3 +259,6 @@ Make sure you checkout and create a branch following this format:
## License
`flutter_any_logo` is released under the [MIT License](https://github.com/example/flutter_any_logo/blob/main/LICENSE).
+
+
+
diff --git a/images/anyLogo.png b/images/anyLogo.png
new file mode 100644
index 0000000..04c1c3a
Binary files /dev/null and b/images/anyLogo.png differ
diff --git a/lib/src/model/class.dart b/lib/src/model/class.dart
index 17d77c5..66ca7ff 100644
--- a/lib/src/model/class.dart
+++ b/lib/src/model/class.dart
@@ -1,19 +1,108 @@
import 'package:flutter/material.dart';
import 'package:flutter_any_logo/gen/assets.gen.dart';
+/// [AnyLogo] class will help ypu access any logo
+/// you would like to display in your app; listed
+/// in the corresponding category
class AnyLogo {
+ /// You can still pass just the asset image without calling [.image()]
+ /// Image(image: AssetImage(AnyLogo.nba.atlanta.path)),
+ ///
+ ///
+ /// - Variables -
+ /// Key? key,
+ /// AssetBundle? bundle,
+ /// Widget Function(BuildContext, Widget, int?, bool)? frameBuilder,
+ /// Widget Function(BuildContext, Object, StackTrace?)? errorBuilder,
+ /// String? semanticLabel,
+ /// bool excludeFromSemantics = false,
+ /// double? scale,
+ /// double? width,
+ /// double? height,
+ /// Color? color,
+ /// Animation? opacity,
+ /// BlendMode? colorBlendMode,
+ /// BoxFit? fit,
+ /// AlignmentGeometry alignment = Alignment.center,
+ /// ImageRepeat repeat = ImageRepeat.noRepeat,
+ /// Rect? centerSlice,
+ /// bool matchTextDirection = false,
+ /// bool gaplessPlayback = false,
+ /// bool isAntiAlias = false,
+ /// String? package,
+ /// FilterQuality filterQuality = FilterQuality.low,
+ /// int? cacheWidth,
+ /// int? cacheHeight,
+ /// All the variables are sill available
+ /// AnyLogo.nba.atlantaHawks.image(height: 30, fit: BoxFit.contain);
+ ///
+ ///
+ /// - Single Assets Image NBA -
+ /// AnyLogo.daily.mcDonalds
+ ///
+ /// - Access all values in a type -
+ /// AnyLogo.nba.values
+ /// Access the Image
+ /// AnyLogo.uefa.manchesterUnited.image()
+
AnyLogo._();
+ /// Fashion section contains clothing / perfume / luxury / watches
+ /// AnyLogo.fashion.louisVuitton
+ /// AnyLogo.fashion.gucci
+ /// AnyLogo.fashion.prada
static const $AssetsFashionGen fashion = $AssetsFashionGen();
+
+ /// Daily section contains everyday brands food / travel /
+ /// plane / agencies related etc.. Basically if you don't
+ /// find a brand it could be in daily.
+ /// AnyLogo.daily.gillette
+ /// AnyLogo.daily.kellogs
+ /// AnyLogo.daily.lufthansa
static const $AssetsDailyGen daily = $AssetsDailyGen();
+
+ /// UEFA section contains football teams
+ /// AnyLogo.uefa.acMilan
+ /// AnyLogo.uefa.barcelona
static const $AssetsFootballGen uefa = $AssetsFootballGen();
+
+ /// Media section contains social media and area like
+ /// AnyLogo.media.instagram
+ /// AnyLogo.media.tiktok
+ /// AnyLogo.media.facebook
static const $AssetsMediaGen media = $AssetsMediaGen();
+
+ /// NBA section contains all nba teams
+ /// AnyLogo.nba.miamiHeat
+ /// AnyLogo.nba.losAngelesClippers
+ /// AnyLogo.nba.goldenStateWarriors
static const $AssetsNbaGen nba = $AssetsNbaGen();
+
+ /// Tech contains all brands and tech companies
+ /// AnyLogo.tech.playstation
+ /// AnyLogo.tech.siemens
+ /// AnyLogo.tech.visa
static const $AssetsTechGen tech = $AssetsTechGen();
+
+ /// NFL section contains nfl teams
+ /// AnyLogo.nfl.nflArizonaCardinals
+ /// AnyLogo.nfl.nflBuffaloBills
static const $AssetsNflGen nfl = $AssetsNflGen();
+
+ /// Cricket section contains social media and area like
+ /// AnyLogo.cricket.cscs
+ /// AnyLogo.cricket.gurajatLions
+ /// AnyLogo.cricket.mumbaiIndians
static const $AssetsCricketGen cricket = $AssetsCricketGen();
+
+ /// Auto section contains car brands and makes
+ /// AnyLogo.auto.bugatti
+ /// AnyLogo.auto.bmw
+ /// AnyLogo.auto.mercedesBenz
static const $AssetsAutoGen auto = $AssetsAutoGen();
+ /// This values variable can be accessed to display all logos
+ /// available in the plugin package.
static List values = [
...AnyLogo.cricket.values.map((AssetGenImage e) => e.image()).toList(),
...AnyLogo.nba.values.map((AssetGenImage e) => e.image()).toList(),
diff --git a/pubspec.yaml b/pubspec.yaml
index 213fc50..887bfa9 100644
--- a/pubspec.yaml
+++ b/pubspec.yaml
@@ -24,12 +24,9 @@ environment:
#screenshots
screenshots:
- - description: ' All logos intro '
- path: images/logos.jpeg
- - description: 'First part of demo'
- path: images/demo1.png
- - description: 'Last part of demo'
- path: images/demo2.png
+ - description: ' Any Logo '
+ path: images/anyLogo.png
+
# dependencies
diff --git a/test/categories/automobile_test.dart b/test/categories/automobile_test.dart
index 602494a..2143364 100644
--- a/test/categories/automobile_test.dart
+++ b/test/categories/automobile_test.dart
@@ -1,4 +1,3 @@
-import 'package:flutter/material.dart';
import 'package:flutter_any_logo/src/model/class.dart';
import 'package:flutter_test/flutter_test.dart';
@@ -10,9 +9,7 @@ void main() {
group('Automobile List Tests', () {
testWidgets('Verify All Logos Should load', (WidgetTester tester) async {
await tester.pumpWidget(PumpWidget.auto);
- // Verify that all logo launch
- final int number = AnyLogoTest.numberOfLogos(Const.auto);
- expect(find.byType(Image, skipOffstage: false), findsNWidgets(number));
+ AnyLogoTest.testLogosRendered(Const.auto);
});
testWidgets('Verify no exception is thrown', (WidgetTester tester) async {
diff --git a/test/categories/basketball_test.dart b/test/categories/basketball_test.dart
index 25a52d6..6661182 100644
--- a/test/categories/basketball_test.dart
+++ b/test/categories/basketball_test.dart
@@ -1,4 +1,3 @@
-import 'package:flutter/material.dart';
import 'package:flutter_any_logo/src/model/class.dart';
import 'package:flutter_test/flutter_test.dart';
@@ -10,9 +9,7 @@ void main() {
group('Nba List Tests', () {
testWidgets('Verify All Logos Should load', (WidgetTester tester) async {
await tester.pumpWidget(PumpWidget.nba);
- // Verify that all logo launch
- final int number = AnyLogoTest.numberOfLogos(Const.nba);
- expect(find.byType(Image, skipOffstage: false), findsNWidgets(number));
+ AnyLogoTest.testLogosRendered(Const.nba);
});
testWidgets('Verify no exception is thrown', (WidgetTester tester) async {
diff --git a/test/categories/cricket_test.dart b/test/categories/cricket_test.dart
index eb343d9..8cf2ccc 100644
--- a/test/categories/cricket_test.dart
+++ b/test/categories/cricket_test.dart
@@ -1,4 +1,3 @@
-import 'package:flutter/material.dart';
import 'package:flutter_any_logo/src/model/class.dart';
import 'package:flutter_test/flutter_test.dart';
@@ -10,9 +9,7 @@ void main() {
group('Cricket List Tests', () {
testWidgets('Verify All Logos Should load', (WidgetTester tester) async {
await tester.pumpWidget(PumpWidget.cricket);
- // Verify that all logo launch
- final int number = AnyLogoTest.numberOfLogos(Const.cricket);
- expect(find.byType(Image, skipOffstage: false), findsNWidgets(number));
+ AnyLogoTest.testLogosRendered(Const.cricket);
});
testWidgets('Verify no exception is thrown', (WidgetTester tester) async {
diff --git a/test/categories/fashion_test.dart b/test/categories/fashion_test.dart
index 617a301..eb13e9e 100644
--- a/test/categories/fashion_test.dart
+++ b/test/categories/fashion_test.dart
@@ -1,4 +1,3 @@
-import 'package:flutter/material.dart';
import 'package:flutter_any_logo/src/model/class.dart';
import 'package:flutter_test/flutter_test.dart';
@@ -10,9 +9,7 @@ void main() {
group('Fashion List Tests', () {
testWidgets('Verify All Logos Should load', (WidgetTester tester) async {
await tester.pumpWidget(PumpWidget.fashion);
- // Verify that all logo launch
- final int number = AnyLogoTest.numberOfLogos(Const.fashion);
- expect(find.byType(Image, skipOffstage: false), findsNWidgets(number));
+ AnyLogoTest.testLogosRendered(Const.fashion);
});
testWidgets('Verify no exception is thrown', (WidgetTester tester) async {
diff --git a/test/categories/football_test.dart b/test/categories/football_test.dart
index 30c5d93..82e408a 100644
--- a/test/categories/football_test.dart
+++ b/test/categories/football_test.dart
@@ -1,4 +1,3 @@
-import 'package:flutter/material.dart';
import 'package:flutter_any_logo/src/model/class.dart';
import 'package:flutter_test/flutter_test.dart';
@@ -10,9 +9,7 @@ void main() {
group('Football List Tests', () {
testWidgets('Verify All Logos Should load', (WidgetTester tester) async {
await tester.pumpWidget(PumpWidget.football);
- // Verify that all logo launch
- final int number = AnyLogoTest.numberOfLogos(Const.football);
- expect(find.byType(Image, skipOffstage: false), findsNWidgets(number));
+ AnyLogoTest.testLogosRendered(Const.football);
});
testWidgets('Verify no exception is thrown', (WidgetTester tester) async {
diff --git a/test/categories/media_test.dart b/test/categories/media_test.dart
index af12376..70e4dae 100644
--- a/test/categories/media_test.dart
+++ b/test/categories/media_test.dart
@@ -1,4 +1,3 @@
-import 'package:flutter/material.dart';
import 'package:flutter_any_logo/src/model/class.dart';
import 'package:flutter_test/flutter_test.dart';
@@ -10,9 +9,7 @@ void main() {
group('Media Logos Tests', () {
testWidgets('Verify All Logos Should load', (WidgetTester tester) async {
await tester.pumpWidget(PumpWidget.media);
- // Verify that all logo launch
- final int number = AnyLogoTest.numberOfLogos(Const.media);
- expect(find.byType(Image, skipOffstage: false), findsNWidgets(number));
+ AnyLogoTest.testLogosRendered(Const.media);
});
testWidgets('Verify no exception is thrown', (WidgetTester tester) async {
diff --git a/test/categories/nfl_test.dart b/test/categories/nfl_test.dart
index b98d920..393d8e8 100644
--- a/test/categories/nfl_test.dart
+++ b/test/categories/nfl_test.dart
@@ -1,4 +1,3 @@
-import 'package:flutter/material.dart';
import 'package:flutter_any_logo/src/model/class.dart';
import 'package:flutter_test/flutter_test.dart';
@@ -10,9 +9,7 @@ void main() {
group('NFL List Tests', () {
testWidgets('Verify All Logos Should load', (WidgetTester tester) async {
await tester.pumpWidget(PumpWidget.nfl);
- // Verify that all logo launch
- final int number = AnyLogoTest.numberOfLogos(Const.nfl);
- expect(find.byType(Image, skipOffstage: false), findsNWidgets(number));
+ AnyLogoTest.testLogosRendered(Const.nfl);
});
testWidgets('Verify no exception is thrown', (WidgetTester tester) async {
diff --git a/test/categories/tech_test.dart b/test/categories/tech_test.dart
index 21330d8..e8f4bfb 100644
--- a/test/categories/tech_test.dart
+++ b/test/categories/tech_test.dart
@@ -1,4 +1,3 @@
-import 'package:flutter/material.dart';
import 'package:flutter_any_logo/src/model/class.dart';
import 'package:flutter_test/flutter_test.dart';
@@ -10,9 +9,7 @@ void main() {
group('Tech logos Tests', () {
testWidgets('Verify All Logos Should load', (WidgetTester tester) async {
await tester.pumpWidget(PumpWidget.tech);
- // Verify that all logo launch
- final int number = AnyLogoTest.numberOfLogos(Const.tech);
- expect(find.byType(Image, skipOffstage: false), findsNWidgets(number));
+ AnyLogoTest.testLogosRendered(Const.tech);
});
testWidgets('Verify no exception is thrown', (WidgetTester tester) async {
diff --git a/test/helpers/helpers.dart b/test/helpers/helpers.dart
index d005cbd..3a3a584 100644
--- a/test/helpers/helpers.dart
+++ b/test/helpers/helpers.dart
@@ -5,12 +5,12 @@ import 'package:flutter/material.dart';
import 'package:flutter_any_logo/gen/assets.gen.dart';
import 'package:flutter_test/flutter_test.dart';
+/// This test will always make sure all the files in
+/// the directory are properly loaded. if the folder
+/// contains 30 files and 28 are loaded and error has
+/// occurred somewhere.
class AnyLogoTest {
- // This test will always make sure all the files in
- // the directory are properly loaded. if the folder
- // contains 30 files and 28 are loaded and error has
- // occurred somewhere.
- static int numberOfLogos(String path) {
+ static void testLogosRendered(String path) {
final Directory myDirectory = Directory(path);
final List myFiles = myDirectory.listSync();
@@ -18,26 +18,19 @@ class AnyLogoTest {
if (kDebugMode) {
print('Number of files: $fileCount');
}
-
- return fileCount;
+ expect(find.byType(Image, skipOffstage: false), findsNWidgets(fileCount));
}
static void testSize(String directoryPath) {
- // Get a list of all files in the directory (recursively)
final List files =
Directory(directoryPath).listSync(recursive: true);
- // Loop through each file and check if it's an image with size
- // less than 400KB
for (final FileSystemEntity file in files) {
if (file is File && (file.path.endsWith('.png'))) {
- // Load the image data from the file
final Uint8List imageData = file.readAsBytesSync();
- // Get the size of the image data
final double imageSizeInKB = imageData.lengthInBytes / 1024;
- // Check if the size is less than 400KB
expect(imageSizeInKB, lessThan(400),
reason: 'The image ${file.absolute} size of ${file.path} should be'
' less than 400KB');
@@ -46,11 +39,9 @@ class AnyLogoTest {
}
static void testType(String directoryPath) {
- // Get a list of all files in the directory (recursively)
final List files =
Directory(directoryPath).listSync(recursive: true);
- // Loop through each file and check if it's an image type
for (final FileSystemEntity file in files) {
if (file is File) {
expect(file.path.endsWith('.png'), isTrue,
@@ -60,40 +51,35 @@ class AnyLogoTest {
}
static void testException(WidgetTester tester) async {
- // Verify that no error is thrown
- expect(tester.takeException(), isNull);
+ expect(tester.takeException(), isNull,
+ reason: 'Verify that no error is thrown');
- // Scroll up
await tester.fling(find.byType(ListView), const Offset(0, -700), 500);
await tester.pumpAndSettle();
- // Verify that no error is thrown
- expect(tester.takeException(), isNull);
+ expect(tester.takeException(), isNull,
+ reason: 'Verify that no error is thrown during scrolling');
- // Scroll up
await tester.fling(find.byType(ListView), const Offset(0, -700), 500);
await tester.pumpAndSettle();
- // Verify that no error is thrown
- expect(tester.takeException(), isNull);
+ expect(tester.takeException(), isNull,
+ reason: 'Verify that no error is thrown');
}
static void testDisplayedLogos(
WidgetTester tester, List items) async {
- // Verify that no error is thrown
- expect(tester.takeException(), isNull);
+ expect(tester.takeException(), isNull,
+ reason: 'Verify that no error is thrown');
- // Scroll up
await tester.fling(find.byType(ListView), const Offset(0, -102000), 3000);
await tester.pumpAndSettle();
-
final Finder listViewFinder = find.byType(ListView);
expect(listViewFinder, findsOneWidget);
-
await tester.pumpAndSettle();
- // Verify that the last item displayed after scrolling up is the same as t
- // he item at the last index
+ expect(tester.takeException(), isNull,
+ reason: 'Verify that no error is thrown ');
final Finder lastItemFinder = find.byType(Image, skipOffstage: false).last;
final ImageProvider