diff --git a/.eslintrc.js b/.eslintrc.js
index 26227a9129..9e0de5ac04 100644
--- a/.eslintrc.js
+++ b/.eslintrc.js
@@ -52,5 +52,5 @@ module.exports = {
'max-len': ['error', { code: 300 }],
'no-multiple-empty-lines': ['error', { max: 1, maxEOF: 1 }],
},
- ignorePatterns: ['*/docs/api/*', 'dist'],
+ ignorePatterns: ['*/docs/api/*', 'dist', 'packages/cli/src/template/**/*.*', '*/locale/*', 'stats.json'],
};
diff --git a/.github/workflows/quality.yml b/.github/workflows/quality.yml
index 89d87663e4..b23cbc3846 100644
--- a/.github/workflows/quality.yml
+++ b/.github/workflows/quality.yml
@@ -17,7 +17,5 @@ jobs:
run: pnpm lint
- name: Format Check
run: pnpm format:check
- - name: Test
- run: pnpm test
- name: Docs
run: pnpm docs:api
diff --git a/.github/workflows/test.yml b/.github/workflows/test.yml
new file mode 100644
index 0000000000..f14aa9d2a8
--- /dev/null
+++ b/.github/workflows/test.yml
@@ -0,0 +1,24 @@
+name: GrapesJS Tests
+on:
+ push:
+ branches: [dev]
+ pull_request:
+ branches: [dev]
+
+jobs:
+ test-core:
+ runs-on: ubuntu-latest
+ steps:
+ - uses: actions/checkout@v4
+ - uses: ./.github/actions/setup-project
+ - name: Core Tests
+ run: pnpm test
+ working-directory: ./packages/core
+ test-cli:
+ runs-on: ubuntu-latest
+ steps:
+ - uses: actions/checkout@v4
+ - uses: ./.github/actions/setup-project
+ - name: CLI Tests
+ run: pnpm test
+ working-directory: ./packages/cli
diff --git a/.prettierignore b/.prettierignore
index 19fba71ff9..2a75b23edf 100644
--- a/.prettierignore
+++ b/.prettierignore
@@ -1,3 +1,6 @@
docs/**/*.md
dist/
-pnpm-lock.yaml
\ No newline at end of file
+pnpm-lock.yaml
+packages/cli/src/template/**/*.*
+**/locale/**
+stats.json
\ No newline at end of file
diff --git a/package.json b/package.json
index 1a165f1802..094ae02bae 100644
--- a/package.json
+++ b/package.json
@@ -4,21 +4,23 @@
"packageManager": "pnpm@9.10.0",
"scripts": {
"start": "pnpm --filter grapesjs start",
- "test": "pnpm --filter grapesjs test",
+ "test": "pnpm -r run test",
"docs": "pnpm --filter @grapesjs/docs docs",
"docs:api": "pnpm --filter @grapesjs/docs docs:api",
"lint": "eslint .",
"build": "pnpm --filter \"!@grapesjs/docs\" build",
"ts:check": "pnpm --filter grapesjs ts:check",
"clean": "find . -type d \\( -name \"node_modules\" -o -name \"build\" -o -name \"dist\" \\) -exec rm -rf {} + && rm ./pnpm-lock.yaml",
- "format": "prettier . --write",
- "format:check": "prettier . --check"
+ "format": "prettier . --write --ignore-path .prettierignore",
+ "format:check": "prettier . --check --ignore-path .prettierignore"
},
"devDependencies": {
"@babel/cli": "7.24.8",
"@babel/core": "7.25.2",
"@babel/preset-env": "7.25.4",
"@babel/preset-typescript": "7.24.7",
+ "@babel/runtime": "7.25.6",
+ "babel-loader": "9.1.3",
"@jest/globals": "29.7.0",
"@types/jest": "29.5.12",
"@types/node": "22.4.1",
diff --git a/packages/cli/README.md b/packages/cli/README.md
new file mode 100644
index 0000000000..6a05f4b7ab
--- /dev/null
+++ b/packages/cli/README.md
@@ -0,0 +1,122 @@
+# GrapesJS CLI
+
+[](https://www.npmjs.com/package/grapesjs-cli)
+
+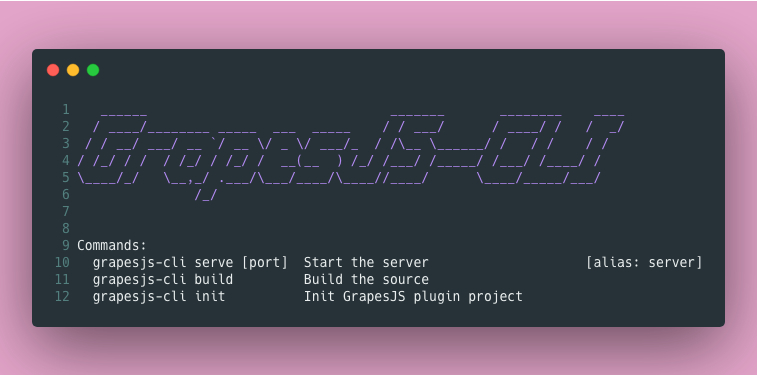
+
+A simple CLI library for helping in GrapesJS plugin development.
+
+The goal of this package is to avoid the hassle of setting up all the dependencies and configurations for the plugin development by centralizing and speeding up the necessary steps during the process.
+
+- Fast project scaffolding
+- No need to touch Babel and Webpack configurations
+
+## Plugin from 0 to 100
+
+Create a production-ready plugin in a few simple steps.
+
+- Create a folder for your plugin and init some preliminary steps
+
+```sh
+mkdir grapesjs-my-plugin
+cd grapesjs-my-plugin
+npm init -y
+git init
+```
+
+- Install the package
+
+```sh
+npm i -D grapesjs-cli
+```
+
+- Init your plugin project by following few steps
+
+```sh
+npx grapesjs-cli init
+```
+
+You can also skip all the questions with `-y` option or pass all the answers via options (to see all available options run `npx grapesjs-cli init --help`)
+
+```sh
+npx grapesjs-cli init -y --user=YOUR-GITHUB-USERNAME
+```
+
+- The command will scaffold the `src` directory and a bunch of other files inside your project. The `src/index.js` will be the entry point of your plugin. Before starting developing your plugin run the development server and open the printed URL (eg. the default is http://localhost:8080)
+
+```sh
+npx grapesjs-cli serve
+```
+
+If you need a custom port use the `-p` option
+
+```sh
+npx grapesjs-cli serve -p 8081
+```
+
+Under the hood we use `webpack-dev-server` and you can pass its option via CLI in this way
+
+```sh
+npx grapesjs-cli serve --devServer='{"https": true}'
+```
+
+- Once the development is finished you can build your plugin and generate the minified file ready for production
+
+```sh
+npx grapesjs-cli build
+```
+
+- Before publishing your package remember to complete your README.md file with all the available options, components, blocks and so on.
+ For a better user engagement create a simple live demo by using services like [JSFiddle](https://jsfiddle.net) [CodeSandbox](https://codesandbox.io) [CodePen](https://codepen.io) and link it in your README. To help you in this process we'll print all the necessary HTML/CSS/JS in your README, so it will be just a matter of copy-pasting on some of those services.
+
+## Customization
+
+### Customize webpack config
+
+If you need to customize the webpack configuration, you can create `webpack.config.js` file in the root dir of your project and export a function, which should return the new configuration object. Check the example below.
+
+```js
+// YOUR-PROJECT-DIR/webpack.config.js
+
+// config is the default configuration
+export default ({ config }) => {
+ // This is how you can distinguish the `build` command from the `serve`
+ const isBuild = config.mode === 'production';
+
+ return {
+ ...config,
+ module: {
+ rules: [
+ {
+ /* extra rule */
+ },
+ ...config.module.rules,
+ ],
+ },
+ };
+};
+```
+
+## Generic CLI usage
+
+Show all available commands
+
+```sh
+grapesjs-cli
+```
+
+Show available options for a command
+
+```sh
+grapesjs-cli COMMAND --help
+```
+
+Run the command
+
+```sh
+grapesjs-cli COMMAND --OPT1 --OPT2=VALUE
+```
+
+## License
+
+MIT
diff --git a/packages/cli/babel.config.js b/packages/cli/babel.config.js
new file mode 100644
index 0000000000..e6ffbd417e
--- /dev/null
+++ b/packages/cli/babel.config.js
@@ -0,0 +1,3 @@
+module.exports = {
+ presets: [['@babel/preset-env', { targets: { node: 'current' } }], '@babel/preset-typescript'],
+};
diff --git a/packages/cli/index.html b/packages/cli/index.html
new file mode 100644
index 0000000000..5a62789c49
--- /dev/null
+++ b/packages/cli/index.html
@@ -0,0 +1,42 @@
+
+
+
+
+ <%= title %>
+
+
+
+
+
+
+
+ This is a demo content generated from GrapesJS CLI . For the development, you should create a _index.html
+ template file (might be a copy of this one) and on the next server start the new file will be served, and it
+ will be ignored by git.
+
+
+
+
+
+
diff --git a/packages/cli/jest.config.ts b/packages/cli/jest.config.ts
new file mode 100644
index 0000000000..75b790de0f
--- /dev/null
+++ b/packages/cli/jest.config.ts
@@ -0,0 +1,10 @@
+import type { Config } from 'jest';
+
+const config: Config = {
+ testEnvironment: 'node',
+ verbose: true,
+ modulePaths: ['/src'],
+ testMatch: ['/test/**/*.(t|j)s'],
+};
+
+export default config;
diff --git a/packages/cli/package.json b/packages/cli/package.json
new file mode 100644
index 0000000000..db7878e35d
--- /dev/null
+++ b/packages/cli/package.json
@@ -0,0 +1,57 @@
+{
+ "name": "grapesjs-cli",
+ "version": "4.1.3",
+ "description": "GrapesJS CLI tool for the plugin development",
+ "bin": {
+ "grapesjs-cli": "dist/cli.js"
+ },
+ "files": [
+ "dist"
+ ],
+ "scripts": {
+ "build": "BUILD_MODE=production webpack --config ./webpack.cli.ts",
+ "build:watch": "webpack --config ./webpack.cli.ts --watch",
+ "lint": "eslint src",
+ "patch": "npm version patch -m 'Bump v%s'",
+ "test": "jest"
+ },
+ "repository": {
+ "type": "git",
+ "url": "https://github.com/GrapesJS/grapesjs.git"
+ },
+ "keywords": [
+ "grapesjs",
+ "plugin",
+ "dev",
+ "cli"
+ ],
+ "author": "Artur Arseniev",
+ "license": "BSD-3-Clause",
+ "dependencies": {
+ "@babel/core": "7.25.2",
+ "@babel/plugin-transform-runtime": "7.25.4",
+ "@babel/preset-env": "7.25.4",
+ "@babel/runtime": "7.25.6",
+ "babel-loader": "9.1.3",
+ "chalk": "^4.1.2",
+ "core-js": "3.38.1",
+ "dts-bundle-generator": "^8.0.1",
+ "html-webpack-plugin": "5.6.0",
+ "inquirer": "^8.2.5",
+ "listr": "^0.14.3",
+ "lodash.template": "^4.5.0",
+ "rimraf": "^4.1.2",
+ "spdx-license-list": "^6.6.0",
+ "terser-webpack-plugin": "^5.3.10",
+ "webpack": "5.94.0",
+ "webpack-cli": "5.1.4",
+ "webpack-dev-server": "5.1.0",
+ "yargs": "^17.6.2"
+ },
+ "devDependencies": {
+ "@types/webpack-node-externals": "^3.0.0",
+ "copy-webpack-plugin": "^11.0.0",
+ "fork-ts-checker-webpack-plugin": "^8.0.0",
+ "webpack-node-externals": "^3.0.0"
+ }
+}
diff --git a/packages/cli/src/banner.txt b/packages/cli/src/banner.txt
new file mode 100644
index 0000000000..85b626677b
--- /dev/null
+++ b/packages/cli/src/banner.txt
@@ -0,0 +1,6 @@
+ ______ _______ ________ ____
+ / ____/________ _____ ___ _____ / / ___/ / ____/ / / _/
+ / / __/ ___/ __ `/ __ \/ _ \/ ___/_ / /\__ \______/ / / / / /
+/ /_/ / / / /_/ / /_/ / __(__ ) /_/ /___/ /_____/ /___/ /____/ /
+\____/_/ \__,_/ .___/\___/____/\____//____/ \____/_____/___/
+ /_/
\ No newline at end of file
diff --git a/packages/cli/src/build.ts b/packages/cli/src/build.ts
new file mode 100644
index 0000000000..2afb77f0b3
--- /dev/null
+++ b/packages/cli/src/build.ts
@@ -0,0 +1,143 @@
+import {
+ printRow,
+ printError,
+ buildWebpackArgs,
+ normalizeJsonOpt,
+ copyRecursiveSync,
+ rootResolve,
+ babelConfig,
+ log,
+ writeFile,
+} from './utils';
+import { generateDtsBundle } from 'dts-bundle-generator';
+import webpack from 'webpack';
+import fs from 'fs';
+import webpackConfig from './webpack.config';
+import { exec } from 'child_process';
+import chalk from 'chalk';
+import rimraf from 'rimraf';
+import { transformFileSync } from '@babel/core';
+
+interface BuildOptions {
+ verbose?: boolean;
+ patch?: boolean;
+ statsOutput?: string;
+ localePath?: string;
+ dts?: 'include' | 'skip' | 'only';
+}
+
+/**
+ * Build locale files
+ * @param {Object} opts
+ */
+export const buildLocale = async (opts: BuildOptions = {}) => {
+ const { localePath } = opts;
+ if (!fs.existsSync(rootResolve(localePath))) return;
+ printRow('Start building locale files...', { lineDown: 0 });
+
+ await rimraf('locale');
+
+ const localDst = rootResolve('locale');
+ copyRecursiveSync(rootResolve(localePath), localDst);
+
+ // Create locale/index.js file
+ let result = '';
+ fs.readdirSync(localDst).forEach((file) => {
+ const name = file.split('.')[0];
+ result += `export { default as ${name} } from './${name}'\n`;
+ });
+ fs.writeFileSync(`${localDst}/index.js`, result);
+
+ // Compile files
+ const babelOpts = { ...babelConfig(buildWebpackArgs(opts) as any) };
+ fs.readdirSync(localDst).forEach((file) => {
+ const filePath = `${localDst}/${file}`;
+ const compiled = transformFileSync(filePath, babelOpts).code;
+ fs.writeFileSync(filePath, compiled);
+ });
+
+ printRow('Locale files building completed successfully!');
+};
+
+/**
+ * Build TS declaration file
+ * @param {Object} opts
+ */
+export const buildDeclaration = async (opts: BuildOptions = {}) => {
+ const filePath = rootResolve('src/index.ts');
+ if (!fs.existsSync(filePath)) return;
+
+ printRow('Start building TS declaration file...', { lineDown: 0 });
+
+ const entry = { filePath, output: { noBanner: true } };
+ const bundleOptions = { preferredConfigPath: rootResolve('tsconfig.json') };
+ const result = generateDtsBundle([entry], bundleOptions)[0];
+ await writeFile(rootResolve('dist/index.d.ts'), result);
+
+ printRow('TS declaration file building completed successfully!');
+};
+
+/**
+ * Build the library files
+ * @param {Object} opts
+ */
+export default (opts: BuildOptions = {}) => {
+ printRow('Start building the library...');
+ const isVerb = opts.verbose;
+ const { dts } = opts;
+ isVerb && log(chalk.yellow('Build config:\n'), opts, '\n');
+
+ const buildWebpack = () => {
+ const buildConf = {
+ ...webpackConfig({
+ production: 1,
+ args: buildWebpackArgs(opts),
+ cmdOpts: opts,
+ }),
+ ...normalizeJsonOpt(opts, 'config'),
+ };
+
+ if (dts === 'only') {
+ return buildDeclaration(opts);
+ }
+
+ webpack(buildConf, async (err, stats) => {
+ const errors = err || (stats ? stats.hasErrors() : false);
+ const statConf = {
+ hash: false,
+ colors: true,
+ builtAt: false,
+ entrypoints: false,
+ modules: false,
+ ...normalizeJsonOpt(opts, 'stats'),
+ };
+
+ if (stats) {
+ opts.statsOutput && fs.writeFileSync(rootResolve(opts.statsOutput), JSON.stringify(stats.toJson()));
+ isVerb && log(chalk.yellow('Stats config:\n'), statConf, '\n');
+ const result = stats.toString(statConf);
+ log(result, '\n');
+ }
+
+ await buildLocale(opts);
+
+ if (dts !== 'skip') {
+ await buildDeclaration(opts);
+ }
+
+ if (errors) {
+ printError(`Error during building`);
+ console.error(err);
+ } else {
+ printRow('Building completed successfully!');
+ }
+ });
+ };
+
+ if (opts.patch) {
+ isVerb && log(chalk.yellow('Patch the version'), '\n');
+ exec('npm version --no-git-tag-version patch', buildWebpack);
+ } else {
+ buildWebpack();
+ }
+};
diff --git a/packages/cli/src/cli.ts b/packages/cli/src/cli.ts
new file mode 100644
index 0000000000..20ecd18039
--- /dev/null
+++ b/packages/cli/src/cli.ts
@@ -0,0 +1,174 @@
+import yargs from 'yargs';
+import fs from 'fs';
+import path from 'path';
+import { serve, build, init } from './main';
+import chalk from 'chalk';
+import { printError } from './utils';
+import { version } from '../package.json';
+
+yargs.usage(chalk.green.bold(fs.readFileSync(path.resolve(__dirname, './banner.txt'), 'utf8') + `\nv${version}`));
+
+const webpackOptions = (yargs) => {
+ yargs
+ .positional('config', {
+ describe: 'webpack configuration options',
+ type: 'string',
+ default: '{}',
+ })
+ .positional('babel', {
+ describe: 'Babel configuration object',
+ type: 'string',
+ default: '{}',
+ })
+ .positional('targets', {
+ describe: 'Browser targets in browserslist query',
+ type: 'string',
+ default: '> 0.25%, not dead',
+ })
+ .positional('entry', {
+ describe: 'Library entry point',
+ type: 'string',
+ default: 'src/index',
+ })
+ .positional('output', {
+ describe: 'Build destination directory',
+ type: 'string',
+ default: 'dist',
+ });
+};
+
+export const createCommands = (yargs) => {
+ return yargs
+ .command(
+ ['serve [port]', 'server'],
+ 'Start the server',
+ (yargs) => {
+ yargs
+ .positional('devServer', {
+ describe: 'webpack-dev-server options',
+ type: 'string',
+ default: '{}',
+ })
+ .positional('host', {
+ alias: 'h',
+ describe: 'Host to bind on',
+ type: 'string',
+ default: 'localhost',
+ })
+ .positional('port', {
+ alias: 'p',
+ describe: 'Port to bind on',
+ type: 'number',
+ default: 8080,
+ })
+ .positional('htmlWebpack', {
+ describe: 'html-webpack-plugin options',
+ type: 'string',
+ default: '{}',
+ });
+ webpackOptions(yargs);
+ },
+ (argv) => serve(argv),
+ )
+ .command(
+ 'build',
+ 'Build the source',
+ (yargs) => {
+ yargs
+ .positional('stats', {
+ describe: 'Options for webpack Stats instance',
+ type: 'string',
+ default: '{}',
+ })
+ .positional('statsOutput', {
+ describe: 'Specify the path where to output webpack stats file (eg. "stats.json")',
+ type: 'string',
+ default: '',
+ })
+ .positional('patch', {
+ describe: 'Increase automatically the patch version',
+ type: 'boolean',
+ default: true,
+ })
+ .positional('localePath', {
+ describe: 'Path to the directory containing locale files',
+ type: 'string',
+ default: 'src/locale',
+ })
+ .positional('dts', {
+ describe: 'Generate typescript dts file ("include", "skip", "only")',
+ type: 'string',
+ default: 'include',
+ });
+ webpackOptions(yargs);
+ },
+ (argv) => build(argv),
+ )
+ .command(
+ 'init',
+ 'Init GrapesJS plugin project',
+ (yargs) => {
+ yargs
+ .positional('yes', {
+ alias: 'y',
+ describe: 'All default answers',
+ type: 'boolean',
+ default: false,
+ })
+ .positional('name', {
+ describe: 'Name of the project',
+ type: 'string',
+ })
+ .positional('rName', {
+ describe: 'Repository name',
+ type: 'string',
+ })
+ .positional('user', {
+ describe: 'Repository username',
+ type: 'string',
+ })
+ .positional('components', {
+ describe: 'Indicate to include custom component types API',
+ type: 'boolean',
+ })
+ .positional('blocks', {
+ describe: 'Indicate to include blocks API',
+ type: 'boolean',
+ })
+ .positional('i18n', {
+ describe: 'Indicate to include the support for i18n',
+ type: 'boolean',
+ })
+ .positional('license', {
+ describe: 'License of the project',
+ type: 'string',
+ });
+ },
+ (argv) => init(argv),
+ )
+ .options({
+ verbose: {
+ alias: 'v',
+ description: 'Run with verbose logging',
+ type: 'boolean', // boolean | number | string
+ default: false,
+ },
+ })
+ .recommendCommands()
+ .strict();
+};
+
+export const argsToOpts = async () => {
+ return await createCommands(yargs).parse();
+};
+
+export const run = async (opts = {}) => {
+ try {
+ let options = await argsToOpts();
+ if (!options._.length) yargs.showHelp();
+ } catch (error) {
+ printError((error.stack || error).toString());
+ }
+};
+
+run();
diff --git a/packages/cli/src/init.ts b/packages/cli/src/init.ts
new file mode 100644
index 0000000000..95e223dbe8
--- /dev/null
+++ b/packages/cli/src/init.ts
@@ -0,0 +1,217 @@
+import inquirer from 'inquirer';
+import { printRow, isUndefined, log, ensureDir } from './utils';
+import Listr from 'listr';
+import path from 'path';
+import fs from 'fs';
+import spdxLicenseList from 'spdx-license-list/full';
+import template from 'lodash.template';
+import { version } from '../package.json';
+
+interface InitOptions {
+ license?: string;
+ name?: string;
+ components?: boolean;
+ blocks?: boolean;
+ i18n?: boolean;
+ verbose?: boolean;
+ rName?: string;
+ user?: string;
+ yes?: boolean;
+}
+
+const tmpPath = './template';
+const rootPath = process.cwd();
+
+const getName = (str: string) =>
+ str
+ .replace(/\_/g, '-')
+ .split('-')
+ .filter((i) => i)
+ .map((i) => i[0].toUpperCase() + i.slice(1))
+ .join(' ');
+
+const getTemplateFileContent = (pth: string) => {
+ const pt = path.resolve(__dirname, `${tmpPath}/${pth}`);
+ return fs.readFileSync(pt, 'utf8');
+};
+
+const resolveRoot = (pth: string) => {
+ return path.resolve(rootPath, pth);
+};
+
+const resolveLocal = (pth: string) => {
+ return path.resolve(__dirname, `${tmpPath}/${pth}`);
+};
+
+const createSourceFiles = async (opts: InitOptions = {}) => {
+ const rdmSrc = getTemplateFileContent('README.md');
+ const rdmDst = resolveRoot('README.md');
+ const indxSrc = getTemplateFileContent('src/index.js');
+ const indxDst = resolveRoot('src/index.js');
+ const indexCnt = getTemplateFileContent('_index.html');
+ const indexDst = resolveRoot('_index.html');
+ const license = spdxLicenseList[opts.license];
+ const licenseTxt =
+ license &&
+ (license.licenseText || '')
+ .replace('', `${new Date().getFullYear()}-current`)
+ .replace('', opts.name);
+ ensureDir(indxDst);
+ // write src/_index.html
+ fs.writeFileSync(indxDst, template(indxSrc)(opts).trim());
+ // write _index.html
+ fs.writeFileSync(indexDst, template(indexCnt)(opts));
+ // Write README.md
+ fs.writeFileSync(rdmDst, template(rdmSrc)(opts));
+ // write LICENSE
+ licenseTxt && fs.writeFileSync(resolveRoot('LICENSE'), licenseTxt);
+ // Copy files
+ fs.copyFileSync(resolveLocal('.gitignore-t'), resolveRoot('.gitignore'));
+ fs.copyFileSync(resolveLocal('.npmignore-t'), resolveRoot('.npmignore'));
+ fs.copyFileSync(resolveLocal('tsconfig.json'), resolveRoot('tsconfig.json'));
+};
+
+const createFileComponents = (opts: InitOptions = {}) => {
+ const filepath = 'src/components.js';
+ const cmpSrc = resolveLocal(filepath);
+ const cmpDst = resolveRoot(filepath);
+ opts.components && fs.copyFileSync(cmpSrc, cmpDst);
+};
+
+const createFileBlocks = (opts: InitOptions = {}) => {
+ const filepath = 'src/blocks.js';
+ const blkSrc = resolveLocal(filepath);
+ const blkDst = resolveRoot(filepath);
+ opts.blocks && fs.copyFileSync(blkSrc, blkDst);
+};
+
+const createI18n = (opts = {}) => {
+ const enPath = 'src/locale/en.js';
+ const tmpEn = getTemplateFileContent(enPath);
+ const dstEn = resolveRoot(enPath);
+ ensureDir(dstEn);
+ fs.writeFileSync(dstEn, template(tmpEn)(opts));
+};
+
+const createPackage = (opts = {}) => {
+ const filepath = 'package.json';
+ const cnt = getTemplateFileContent(filepath);
+ const dst = resolveRoot(filepath);
+ fs.writeFileSync(
+ dst,
+ template(cnt)({
+ ...opts,
+ version,
+ }),
+ );
+};
+
+const checkBoolean = (value) => (value && value !== 'false' ? true : false);
+
+export const initPlugin = async (opts: InitOptions = {}) => {
+ printRow('Start project creation...');
+ opts.components = checkBoolean(opts.components);
+ opts.blocks = checkBoolean(opts.blocks);
+ opts.i18n = checkBoolean(opts.i18n);
+
+ const tasks = new Listr([
+ {
+ title: 'Creating initial source files',
+ task: () => createSourceFiles(opts),
+ },
+ {
+ title: 'Creating custom Component Type file',
+ task: () => createFileComponents(opts),
+ enabled: () => opts.components,
+ },
+ {
+ title: 'Creating Blocks file',
+ task: () => createFileBlocks(opts),
+ enabled: () => opts.blocks,
+ },
+ {
+ title: 'Creating i18n structure',
+ task: () => createI18n(opts),
+ enabled: () => opts.i18n,
+ },
+ {
+ title: 'Update package.json',
+ task: () => createPackage(opts),
+ },
+ ]);
+ await tasks.run();
+};
+
+export default async (opts: InitOptions = {}) => {
+ const rootDir = path.basename(process.cwd());
+ const questions = [];
+ const { verbose, name, rName, user, yes, components, blocks, i18n, license } = opts;
+ let results = {
+ name: name || getName(rootDir),
+ rName: rName || rootDir,
+ user: user || 'YOUR-USERNAME',
+ components: isUndefined(components) ? true : components,
+ blocks: isUndefined(blocks) ? true : blocks,
+ i18n: isUndefined(i18n) ? true : i18n,
+ license: license || 'MIT',
+ };
+ printRow(`Init the project${verbose ? ' (verbose)' : ''}...`);
+
+ if (!yes) {
+ !name &&
+ questions.push({
+ name: 'name',
+ message: 'Name of the project',
+ default: results.name,
+ });
+ !rName &&
+ questions.push({
+ name: 'rName',
+ message: 'Repository name (used also as the plugin name)',
+ default: results.rName,
+ });
+ !user &&
+ questions.push({
+ name: 'user',
+ message: 'Repository username (eg. on GitHub/Bitbucket)',
+ default: results.user,
+ });
+ isUndefined(components) &&
+ questions.push({
+ type: 'boolean',
+ name: 'components',
+ message: 'Will you need to add custom Component Types?',
+ default: results.components,
+ });
+ isUndefined(blocks) &&
+ questions.push({
+ type: 'boolean',
+ name: 'blocks',
+ message: 'Will you need to add Blocks?',
+ default: results.blocks,
+ });
+ isUndefined(i18n) &&
+ questions.push({
+ type: 'boolean',
+ name: 'i18n',
+ message: 'Do you want to setup i18n structure in this plugin?',
+ default: results.i18n,
+ });
+ !license &&
+ questions.push({
+ name: 'license',
+ message: 'License of the project',
+ default: results.license,
+ });
+ }
+
+ const answers = await inquirer.prompt(questions);
+ results = {
+ ...results,
+ ...answers,
+ };
+
+ verbose && log({ results, opts });
+ await initPlugin(results);
+ printRow('Project created! Happy coding');
+};
diff --git a/packages/cli/src/main.ts b/packages/cli/src/main.ts
new file mode 100644
index 0000000000..2cfdd33967
--- /dev/null
+++ b/packages/cli/src/main.ts
@@ -0,0 +1,3 @@
+export { default as init } from './init';
+export { default as build } from './build';
+export { default as serve } from './serve';
diff --git a/packages/cli/src/serve.ts b/packages/cli/src/serve.ts
new file mode 100644
index 0000000000..3dfba4ae9f
--- /dev/null
+++ b/packages/cli/src/serve.ts
@@ -0,0 +1,48 @@
+import { printRow, buildWebpackArgs, log, normalizeJsonOpt } from './utils';
+import webpack from 'webpack';
+import webpackDevServer from 'webpack-dev-server';
+import webpackConfig from './webpack.config';
+import chalk from 'chalk';
+
+interface ServeOptions {
+ host?: string;
+ port?: number;
+ verbose?: boolean;
+}
+
+/**
+ * Start up the development server
+ * @param {Object} opts
+ */
+export default (opts: ServeOptions = {}) => {
+ printRow('Start the development server...');
+ const { host, port } = opts;
+ const isVerb = opts.verbose;
+ const resultWebpackConf = {
+ ...webpackConfig({ args: buildWebpackArgs(opts), cmdOpts: opts }),
+ ...normalizeJsonOpt(opts, 'webpack'),
+ };
+ const devServerConf = {
+ ...resultWebpackConf.devServer,
+ open: true,
+ ...normalizeJsonOpt(opts, 'devServer'),
+ };
+
+ if (host !== 'localhost') {
+ devServerConf.host = host;
+ }
+
+ if (port !== 8080) {
+ devServerConf.port = port;
+ }
+
+ if (isVerb) {
+ log(chalk.yellow('Server config:\n'), opts, '\n');
+ log(chalk.yellow('DevServer config:\n'), devServerConf, '\n');
+ }
+
+ const compiler = webpack(resultWebpackConf);
+ const server = new webpackDevServer(devServerConf, compiler);
+
+ server.start();
+};
diff --git a/packages/cli/src/template/.gitignore-t b/packages/cli/src/template/.gitignore-t
new file mode 100644
index 0000000000..e5fb224315
--- /dev/null
+++ b/packages/cli/src/template/.gitignore-t
@@ -0,0 +1,8 @@
+.DS_Store
+private/
+/locale
+node_modules/
+*.log
+_index.html
+dist/
+stats.json
\ No newline at end of file
diff --git a/packages/cli/src/template/.npmignore-t b/packages/cli/src/template/.npmignore-t
new file mode 100644
index 0000000000..46cbb4ab22
--- /dev/null
+++ b/packages/cli/src/template/.npmignore-t
@@ -0,0 +1,7 @@
+.*
+*.log
+*.html
+**/tsconfig.json
+**/webpack.config.js
+node_modules
+src
\ No newline at end of file
diff --git a/packages/cli/src/template/README.md b/packages/cli/src/template/README.md
new file mode 100644
index 0000000000..311b16fec5
--- /dev/null
+++ b/packages/cli/src/template/README.md
@@ -0,0 +1,145 @@
+# <%= name %>
+
+## Live Demo
+
+> **Show a live example of your plugin**
+
+To make your plugin more engaging, create a simple live demo using online tools like [JSFiddle](https://jsfiddle.net), [CodeSandbox](https://codesandbox.io), or [CodePen](https://codepen.io). Include the demo link in your README. Adding a screenshot or GIF of the demo is a bonus.
+
+Below, you'll find the necessary HTML, CSS, and JavaScript. Copy and paste this code into one of the tools mentioned. Once you're done, delete this section and update the link at the top with your demo.
+
+### HTML
+
+```html
+
+
+
+
+
+```
+
+### JS
+
+```js
+const editor = grapesjs.init({
+ container: '#gjs',
+ height: '100%',
+ fromElement: true,
+ storageManager: false,
+ plugins: ['<%= rName %>'],
+});
+```
+
+### CSS
+
+```css
+body,
+html {
+ margin: 0;
+ height: 100%;
+}
+```
+
+## Summary
+
+- Plugin name: `<%= rName %>`
+- Components
+ - `component-id-1`
+ - `component-id-2`
+ - ...
+- Blocks
+ - `block-id-1`
+ - `block-id-2`
+ - ...
+
+## Options
+
+| Option | Description | Default |
+| --------- | ------------------ | --------------- |
+| `option1` | Description option | `default value` |
+
+## Download
+
+- CDN
+ - `https://unpkg.com/<%= rName %>`
+- NPM
+ - `npm i <%= rName %>`
+- GIT
+ - `git clone https://github.com/<%= user %>/<%= rName %>.git`
+
+## Usage
+
+Directly in the browser
+
+```html
+
+
+
+
+
+
+
+```
+
+Modern javascript
+
+```js
+import grapesjs from 'grapesjs';
+import plugin from '<%= rName %>';
+import 'grapesjs/dist/css/grapes.min.css';
+
+const editor = grapesjs.init({
+ container : '#gjs',
+ // ...
+ plugins: [plugin],
+ pluginsOpts: {
+ [plugin]: { /* options */ }
+ }
+ // or
+ plugins: [
+ editor => plugin(editor, { /* options */ }),
+ ],
+});
+```
+
+## Development
+
+Clone the repository
+
+```sh
+$ git clone https://github.com/<%= user %>/<%= rName %>.git
+$ cd <%= rName %>
+```
+
+Install dependencies
+
+```sh
+npm i
+```
+
+Start the dev server
+
+```sh
+npm start
+```
+
+Build the source
+
+```sh
+npm run build
+```
+
+## License
+
+MIT
diff --git a/packages/cli/src/template/package.json b/packages/cli/src/template/package.json
new file mode 100644
index 0000000000..274ce01b63
--- /dev/null
+++ b/packages/cli/src/template/package.json
@@ -0,0 +1,23 @@
+{
+ "name": "<%= rName %>",
+ "version": "1.0.0",
+ "description": "<%= name %>",
+ "main": "dist/index.js",
+ "repository": {
+ "type": "git",
+ "url": "https://github.com/<%= user %>/<%= rName %>.git"
+ },
+ "scripts": {
+ "start": "grapesjs-cli serve",
+ "build": "grapesjs-cli build",
+ "bump": "npm version patch -m 'Bump v%s'"
+ },
+ "keywords": [
+ "grapesjs",
+ "plugin"
+ ],
+ "devDependencies": {
+ "grapesjs-cli": "^<%= version %>"
+ },
+ "license": "<%= license %>"
+}
diff --git a/packages/cli/src/template/src/blocks.js b/packages/cli/src/template/src/blocks.js
new file mode 100644
index 0000000000..9a889ec4d7
--- /dev/null
+++ b/packages/cli/src/template/src/blocks.js
@@ -0,0 +1,9 @@
+export default (editor, opts = {}) => {
+ const bm = editor.BlockManager;
+
+ bm.add('MY-BLOCK', {
+ label: 'My block',
+ content: { type: 'MY-COMPONENT' },
+ // media: '... ',
+ });
+};
diff --git a/packages/cli/src/template/src/components.js b/packages/cli/src/template/src/components.js
new file mode 100644
index 0000000000..78ef54ca0e
--- /dev/null
+++ b/packages/cli/src/template/src/components.js
@@ -0,0 +1,12 @@
+export default (editor, opts = {}) => {
+ const domc = editor.DomComponents;
+
+ domc.addType('MY-COMPONENT', {
+ model: {
+ defaults: {
+ // Default props
+ },
+ },
+ view: {},
+ });
+};
diff --git a/packages/cli/src/template/src/index.js b/packages/cli/src/template/src/index.js
new file mode 100644
index 0000000000..030d6764bf
--- /dev/null
+++ b/packages/cli/src/template/src/index.js
@@ -0,0 +1,29 @@
+<% if(components){ %>import loadComponents from './components';<% } %>
+<% if(blocks){ %>import loadBlocks from './blocks';<% } %>
+<% if(i18n){ %>import en from './locale/en';<% } %>
+
+export default (editor, opts = {}) => {
+ const options = { ...{
+ <% if(i18n){ %>i18n: {},<% } %>
+ // default options
+ }, ...opts };
+
+ <% if(components){ %>// Add components
+ loadComponents(editor, options);<% } %>
+ <% if(blocks){ %>// Add blocks
+ loadBlocks(editor, options);<% } %>
+ <% if(i18n){ %>// Load i18n files
+ editor.I18n && editor.I18n.addMessages({
+ en,
+ ...options.i18n,
+ });<% } %>
+
+ // TODO Remove
+ editor.on('load', () =>
+ editor.addComponents(
+ `
+ Content loaded from the plugin
+
`,
+ { at: 0 }
+ ))
+};
diff --git a/packages/cli/src/template/tsconfig.json b/packages/cli/src/template/tsconfig.json
new file mode 100644
index 0000000000..f9599dd335
--- /dev/null
+++ b/packages/cli/src/template/tsconfig.json
@@ -0,0 +1,20 @@
+{
+ "compilerOptions": {
+ "target": "es5",
+ "lib": ["dom", "dom.iterable", "esnext"],
+ "allowJs": true,
+ "sourceMap": true,
+ "skipLibCheck": true,
+ "esModuleInterop": true,
+ "allowSyntheticDefaultImports": true,
+ "strict": true,
+ "forceConsistentCasingInFileNames": true,
+ "noFallthroughCasesInSwitch": true,
+ "module": "esnext",
+ "moduleResolution": "node",
+ "resolveJsonModule": true,
+ "isolatedModules": true,
+ "noEmit": false
+ },
+ "include": ["src"]
+}
diff --git a/packages/cli/src/utils.ts b/packages/cli/src/utils.ts
new file mode 100644
index 0000000000..a3dfcb4ac8
--- /dev/null
+++ b/packages/cli/src/utils.ts
@@ -0,0 +1,123 @@
+import chalk from 'chalk';
+import path from 'path';
+import fs from 'fs';
+import fsp from 'fs/promises';
+
+export const isString = (val: any): val is string => typeof val === 'string';
+
+export const isUndefined = (value: any) => typeof value === 'undefined';
+
+export const isFunction = (value: any): value is Function => typeof value === 'function';
+
+export const isObject = (val: any) => val !== null && !Array.isArray(val) && typeof val === 'object';
+
+export const printRow = (str: string, { color = 'green', lineDown = 1 } = {}) => {
+ console.log('');
+ console.log(chalk[color].bold(str));
+ lineDown && console.log('');
+};
+
+export const printError = (str: string) => {
+ printRow(str, { color: 'red' });
+};
+
+export const log = (...args: any[]) => console.log.apply(this, args);
+
+export const ensureDir = (filePath: string) => {
+ const dirname = path.dirname(filePath);
+ if (fs.existsSync(dirname)) return true;
+ fs.mkdirSync(dirname);
+ return ensureDir(dirname);
+};
+
+/**
+ * Normalize JSON options
+ * @param opts Options
+ * @param key Options name to normalize
+ * @returns {Object}
+ */
+export const normalizeJsonOpt = (opts: Record, key: string) => {
+ let devServerOpt = opts[key] || {};
+
+ if (isString(devServerOpt)) {
+ try {
+ devServerOpt = JSON.parse(devServerOpt);
+ } catch (e) {
+ printError(`Error while parsing "${key}" option`);
+ printError(e);
+ devServerOpt = {};
+ }
+ }
+
+ return devServerOpt;
+};
+
+export const buildWebpackArgs = (opts: Record) => {
+ return {
+ ...opts,
+ babel: normalizeJsonOpt(opts, 'babel'),
+ htmlWebpack: normalizeJsonOpt(opts, 'htmlWebpack'),
+ };
+};
+
+export const copyRecursiveSync = (src: string, dest: string) => {
+ const exists = fs.existsSync(src);
+ const isDir = exists && fs.statSync(src).isDirectory();
+
+ if (isDir) {
+ fs.mkdirSync(dest);
+ fs.readdirSync(src).forEach((file) => {
+ copyRecursiveSync(path.join(src, file), path.join(dest, file));
+ });
+ } else if (exists) {
+ fs.copyFileSync(src, dest);
+ }
+};
+
+export const isPathExists = async (path: string) => {
+ try {
+ await fsp.access(path);
+ return true;
+ } catch {
+ return false;
+ }
+};
+
+export const writeFile = async (filePath: string, data: string) => {
+ try {
+ const dirname = path.dirname(filePath);
+ const exist = await isPathExists(dirname);
+ if (!exist) {
+ await fsp.mkdir(dirname, { recursive: true });
+ }
+
+ await fsp.writeFile(filePath, data, 'utf8');
+ } catch (err) {
+ throw new Error(err);
+ }
+};
+
+export const rootResolve = (val: string) => path.resolve(process.cwd(), val);
+
+export const originalRequire = () => {
+ // @ts-ignore need this to use the original 'require.resolve' as it's replaced by webpack
+ return __non_webpack_require__;
+};
+
+export const resolve = (value: string) => {
+ return originalRequire().resolve(value);
+};
+
+export const babelConfig = (opts: { targets?: string } = {}) => ({
+ presets: [
+ [
+ resolve('@babel/preset-env'),
+ {
+ targets: opts.targets,
+ // useBuiltIns: 'usage', // this makes the build much bigger
+ // corejs: 3,
+ },
+ ],
+ ],
+ plugins: [resolve('@babel/plugin-transform-runtime')],
+});
diff --git a/packages/cli/src/webpack.config.ts b/packages/cli/src/webpack.config.ts
new file mode 100644
index 0000000000..175ba71d25
--- /dev/null
+++ b/packages/cli/src/webpack.config.ts
@@ -0,0 +1,129 @@
+import { babelConfig, rootResolve, isFunction, isObject, log, resolve, originalRequire } from './utils';
+import HtmlWebpackPlugin from 'html-webpack-plugin';
+import TerserPlugin from 'terser-webpack-plugin';
+import chalk from 'chalk';
+import path from 'path';
+import fs from 'fs';
+import webpack from 'webpack';
+
+const dirCwd = process.cwd();
+let plugins = [];
+
+export default (opts: Record = {}): any => {
+ const pkgPath = path.join(dirCwd, 'package.json');
+ const rawPackageJson = fs.readFileSync(pkgPath) as unknown as string;
+ const pkg = JSON.parse(rawPackageJson);
+ const { args, cmdOpts = {} } = opts;
+ const { htmlWebpack = {} } = args;
+ const name = pkg.name;
+ const isProd = opts.production;
+ const banner = `/*! ${name} - ${pkg.version} */`;
+
+ if (!isProd) {
+ const fname = 'index.html';
+ const index = `${dirCwd}/${fname}`;
+ const indexDev = `${dirCwd}/_${fname}`;
+ let template = path.resolve(__dirname, `./../${fname}`);
+
+ if (fs.existsSync(indexDev)) {
+ template = indexDev;
+ } else if (fs.existsSync(index)) {
+ template = index;
+ }
+
+ plugins.push(
+ new HtmlWebpackPlugin({
+ inject: 'head',
+ template,
+ ...htmlWebpack,
+ templateParameters: {
+ name,
+ title: name,
+ gjsVersion: 'latest',
+ pathGjs: '',
+ pathGjsCss: '',
+ ...(htmlWebpack.templateParameters || {}),
+ },
+ }),
+ );
+ }
+
+ const outPath = path.resolve(dirCwd, args.output);
+ const modulesPaths = ['node_modules', path.join(__dirname, '../node_modules')];
+
+ let config = {
+ entry: path.resolve(dirCwd, args.entry),
+ mode: isProd ? 'production' : 'development',
+ devtool: isProd ? 'source-map' : 'eval',
+ optimization: {
+ minimizer: [
+ new TerserPlugin({
+ extractComments: false,
+ terserOptions: {
+ compress: {
+ evaluate: false, // Avoid breaking gjs scripts
+ },
+ output: {
+ comments: false,
+ quote_style: 3, // Preserve original quotes
+ preamble: banner, // banner here instead of BannerPlugin
+ },
+ },
+ }),
+ ],
+ },
+ output: {
+ path: outPath,
+ filename: 'index.js',
+ library: name,
+ libraryTarget: 'umd',
+ globalObject: `typeof globalThis !== 'undefined' ? globalThis : (typeof window !== 'undefined' ? window : this)`,
+ },
+ module: {
+ rules: [
+ {
+ test: /\.tsx?$/,
+ loader: resolve('ts-loader'),
+ exclude: /node_modules/,
+ options: {
+ context: rootResolve(''),
+ configFile: rootResolve('tsconfig.json'),
+ },
+ },
+ {
+ test: /\.js$/,
+ loader: resolve('babel-loader'),
+ include: /src/,
+ options: {
+ ...babelConfig(args),
+ cacheDirectory: true,
+ ...args.babel,
+ },
+ },
+ ],
+ },
+ resolve: {
+ extensions: ['.tsx', '.ts', '.js'],
+ modules: modulesPaths,
+ },
+ plugins,
+ };
+
+ // Try to load local webpack config
+ const localWebpackPath = rootResolve('webpack.config.js');
+ let localWebpackConf: any;
+
+ if (fs.existsSync(localWebpackPath)) {
+ const customWebpack = originalRequire()(localWebpackPath);
+ localWebpackConf = customWebpack.default || customWebpack;
+ }
+
+ if (isFunction(localWebpackConf)) {
+ const fnRes = localWebpackConf({ config, webpack, pkg });
+ config = isObject(fnRes) ? fnRes : config;
+ }
+
+ cmdOpts.verbose && log(chalk.yellow('Webpack config:\n'), config, '\n');
+
+ return config;
+};
diff --git a/packages/cli/test/utils.spec.ts b/packages/cli/test/utils.spec.ts
new file mode 100644
index 0000000000..7caee590e9
--- /dev/null
+++ b/packages/cli/test/utils.spec.ts
@@ -0,0 +1,359 @@
+import {
+ isFunction,
+ isObject,
+ isString,
+ isUndefined,
+ printRow,
+ printError,
+ log,
+ ensureDir,
+ normalizeJsonOpt,
+ buildWebpackArgs,
+ copyRecursiveSync,
+ babelConfig,
+ originalRequire,
+ resolve,
+ rootResolve,
+} from '../src/utils';
+import chalk from 'chalk';
+import fs from 'fs';
+import path from 'path';
+import * as process from 'process';
+
+const typeTestValues = {
+ undefinedValue: undefined,
+ nullValue: null,
+ stringValue: 'hello',
+ emptyObject: {},
+ nonEmptyObject: { key: 'value' },
+ emptyArray: [],
+ functionValue: () => {},
+ numberValue: 42,
+ booleanValue: true,
+ dateValue: new Date(),
+};
+
+function runTypeCheck(typeCheckFunction: (value: any) => boolean) {
+ const keysWithPassingTypeChecks = Object.keys(typeTestValues).filter((key) => {
+ const value = typeTestValues[key];
+ return typeCheckFunction(value);
+ });
+
+ return keysWithPassingTypeChecks;
+}
+
+jest.mock('fs');
+jest.mock('fs/promises');
+
+describe('utils', () => {
+ afterEach(() => {
+ jest.clearAllMocks();
+ });
+
+ describe('isString', () => {
+ it('should correctly identify strings', () => {
+ const result = runTypeCheck(isString);
+ expect(result).toEqual(['stringValue']);
+ });
+ });
+
+ describe('isUndefined', () => {
+ it('should correctly identify undefined values', () => {
+ const result = runTypeCheck(isUndefined);
+ expect(result).toEqual(['undefinedValue']);
+ });
+ });
+
+ describe('isFunction', () => {
+ it('should correctly identify functions', () => {
+ const result = runTypeCheck(isFunction);
+ expect(result).toEqual(['functionValue']);
+ });
+ });
+
+ describe('isObject', () => {
+ it('should correctly identify objects', () => {
+ const result = runTypeCheck(isObject);
+ expect(result).toEqual(['emptyObject', 'nonEmptyObject', 'dateValue']);
+ });
+ });
+
+ describe('printRow', () => {
+ // TODO: We should refactor the function to make lineDown a boolean not a number
+ it('should console.log the given string with the specified color and line breaks', () => {
+ const str = 'Test string';
+ const color = 'blue';
+ const lineDown = 1;
+
+ console.log = jest.fn() as jest.Mock;
+
+ printRow(str, { color, lineDown });
+
+ expect(console.log).toHaveBeenCalledTimes(3); // 1 for empty line, 1 for colored string, 1 for line break
+ expect((console.log as jest.Mock).mock.calls[1][0]).toEqual(chalk[color].bold(str));
+ });
+
+ it('should not add a line break if lineDown is false', () => {
+ const str = 'Test string';
+ const color = 'green';
+ const lineDown = 0;
+
+ console.log = jest.fn();
+
+ printRow(str, { color, lineDown });
+
+ expect(console.log).toHaveBeenCalledTimes(2); // 1 for empty line, 1 for colored string
+ });
+ });
+
+ describe('printError', () => {
+ it('should print the given string in red', () => {
+ const str = 'Error message';
+
+ (console.log as jest.Mock).mockImplementation(() => {});
+
+ printError(str);
+
+ expect(console.log).toHaveBeenCalledTimes(3); // 1 for empty line, 1 for red string, 1 for line break
+ expect((console.log as jest.Mock).mock.calls[1][0]).toEqual(chalk.red.bold(str));
+ });
+ });
+
+ describe('log', () => {
+ it('should call console.log with the given arguments', () => {
+ const arg1 = 'Argument 1';
+ const arg2 = 'Argument 2';
+
+ console.log = jest.fn();
+
+ log(arg1, arg2);
+
+ expect(console.log).toHaveBeenCalledWith(arg1, arg2);
+ });
+ });
+
+ describe('ensureDir', () => {
+ it('should return true when the directory already exists', () => {
+ (fs.existsSync as jest.Mock).mockReturnValue(true);
+
+ const result = ensureDir('/path/to/file.txt');
+ expect(result).toBe(true);
+ expect(fs.existsSync).toHaveBeenCalledWith('/path/to');
+ expect(fs.mkdirSync).not.toHaveBeenCalled();
+ });
+
+ it('should create the directory when it does not exist', () => {
+ (fs.existsSync as jest.Mock).mockReturnValueOnce(false).mockReturnValueOnce(true);
+
+ const result = ensureDir('/path/to/file.txt');
+ expect(result).toBe(true);
+ expect(fs.existsSync).toHaveBeenCalledWith('/path/to');
+ expect(fs.mkdirSync).toHaveBeenCalledWith('/path/to');
+ });
+
+ it('should create parent directories recursively when they do not exist', () => {
+ (fs.existsSync as jest.Mock)
+ .mockReturnValueOnce(false) // Check /path/to (does not exist)
+ .mockReturnValueOnce(false) // Check /path (does not exist)
+ .mockReturnValueOnce(true); // Check / (root, exists)
+
+ const result = ensureDir('/path/to/file.txt');
+ expect(result).toBe(true);
+ expect(fs.existsSync).toHaveBeenCalledTimes(3); // /path/to, /path, /
+ expect(fs.mkdirSync).toHaveBeenCalledTimes(2); // /path, /path/to
+ });
+ });
+
+ describe('normalizeJsonOpt', () => {
+ it('should return the object if the option is already an object', () => {
+ const opts = { babel: { presets: ['@babel/preset-env'] } };
+ const result = normalizeJsonOpt(opts, 'babel');
+ expect(result).toEqual(opts.babel);
+ });
+
+ it('should parse and return the object if the option is a valid JSON string', () => {
+ const opts = { babel: '{"presets":["@babel/preset-env"]}' };
+ const result = normalizeJsonOpt(opts, 'babel');
+ expect(result).toEqual({ presets: ['@babel/preset-env'] });
+ });
+
+ it('should return an empty object if the option is an invalid JSON string', () => {
+ const opts = { babel: '{"presets":["@babel/preset-env"]' }; // Invalid JSON
+ const result = normalizeJsonOpt(opts, 'babel');
+ expect(result).toEqual({});
+ });
+
+ it('should return an empty object if the option is not provided', () => {
+ const opts = {};
+ const result = normalizeJsonOpt(opts, 'babel');
+ expect(result).toEqual({});
+ });
+ });
+
+ describe('buildWebpackArgs', () => {
+ it('should return the options with normalized JSON options for babel and htmlWebpack', () => {
+ const opts = {
+ babel: '{"presets":["@babel/preset-env"]}',
+ htmlWebpack: '{"template":"./src/index.html"}',
+ otherOption: 'someValue',
+ };
+
+ const result = buildWebpackArgs(opts);
+ expect(result).toEqual({
+ babel: { presets: ['@babel/preset-env'] },
+ htmlWebpack: { template: './src/index.html' },
+ otherOption: 'someValue',
+ });
+ });
+
+ it('should return empty objects for babel and htmlWebpack if they are invalid JSON strings', () => {
+ const opts = {
+ babel: '{"presets":["@babel/preset-env"]', // Invalid JSON
+ htmlWebpack: '{"template":"./src/index.html', // Invalid JSON
+ };
+
+ const result = buildWebpackArgs(opts);
+ expect(result).toEqual({
+ babel: {},
+ htmlWebpack: {},
+ });
+ });
+
+ it('should return the original objects if babel and htmlWebpack are already objects', () => {
+ const opts = {
+ babel: { presets: ['@babel/preset-env'] },
+ htmlWebpack: { template: './src/index.html' },
+ };
+
+ const result = buildWebpackArgs(opts);
+ expect(result).toEqual({
+ babel: opts.babel,
+ htmlWebpack: opts.htmlWebpack,
+ });
+ });
+
+ it('should handle missing babel and htmlWebpack keys gracefully', () => {
+ const opts = { otherOption: 'someValue' };
+
+ const result = buildWebpackArgs(opts);
+ expect(result).toEqual({
+ babel: {},
+ htmlWebpack: {},
+ otherOption: 'someValue',
+ });
+ });
+ });
+
+ describe('copyRecursiveSync', () => {
+ // TODO: Maybe this test case is a bit complex and we should think of an easier solution
+ it('should copy a directory and its contents recursively', () => {
+ /**
+ * First call: Mock as a directory with two files
+ * Subsequent calls: Mock as a file
+ */
+ const existsSyncMock = (fs.existsSync as jest.Mock).mockReturnValue(true);
+ const statSyncMock = (fs.statSync as jest.Mock)
+ .mockReturnValueOnce({ isDirectory: () => true })
+ .mockReturnValue({ isDirectory: () => false });
+ const readdirSyncMock = (fs.readdirSync as jest.Mock)
+ .mockReturnValueOnce(['file1.txt', 'file2.txt'])
+ .mockReturnValue([]);
+ const copyFileSyncMock = (fs.copyFileSync as jest.Mock).mockImplementation(() => {});
+
+ copyRecursiveSync('/src', '/dest');
+
+ expect(existsSyncMock).toHaveBeenCalledWith('/src');
+ expect(statSyncMock).toHaveBeenCalledWith('/src');
+ expect(fs.mkdirSync).toHaveBeenCalledWith('/dest');
+ expect(readdirSyncMock).toHaveBeenCalledWith('/src');
+ expect(copyFileSyncMock).toHaveBeenCalledWith(
+ path.normalize('/src/file1.txt'),
+ path.normalize('/dest/file1.txt'),
+ );
+ expect(copyFileSyncMock).toHaveBeenCalledWith(
+ path.normalize('/src/file2.txt'),
+ path.normalize('/dest/file2.txt'),
+ );
+ });
+
+ it('should copy a file when source is a file', () => {
+ (fs.existsSync as jest.Mock).mockReturnValue(true);
+ (fs.statSync as jest.Mock).mockReturnValue({ isDirectory: () => false });
+
+ copyRecursiveSync('/src/file.txt', '/dest/file.txt');
+
+ expect(fs.existsSync).toHaveBeenCalledWith('/src/file.txt');
+ expect(fs.statSync).toHaveBeenCalledWith('/src/file.txt');
+ expect(fs.copyFileSync).toHaveBeenCalledWith('/src/file.txt', '/dest/file.txt');
+ });
+
+ // Maybe we can change the behavior to throw an error if the `src` doesn't exist
+ it('should do nothing when source does not exist', () => {
+ (fs.existsSync as jest.Mock).mockReturnValue(false);
+
+ copyRecursiveSync('/src/file.txt', '/dest/file.txt');
+
+ expect(fs.existsSync).toHaveBeenCalledWith('/src/file.txt');
+ expect(fs.statSync).not.toHaveBeenCalled();
+ expect(fs.mkdirSync).not.toHaveBeenCalled();
+ expect(fs.copyFileSync).not.toHaveBeenCalled();
+ });
+ });
+
+ describe('rootResolve', () => {
+ it('should resolve a relative path to an absolute path', () => {
+ const result = rootResolve('src/index.js');
+
+ expect(result).toBe(path.join(process.cwd(), 'src/index.js'));
+ });
+ });
+
+ describe('originalRequire', () => {
+ it('should return the original require.resolve function', () => {
+ const originalRequireMock = jest.fn();
+ global.__non_webpack_require__ = originalRequireMock;
+
+ const result = originalRequire();
+
+ expect(result).toBe(originalRequireMock);
+ });
+ });
+
+ describe('resolve', () => {
+ it('should resolve a module path using the original require.resolve', () => {
+ const originalRequireMock = {
+ resolve: jest.fn().mockReturnValue('resolved/path'),
+ };
+ global.__non_webpack_require__ = originalRequireMock;
+
+ const result = resolve('my-module');
+
+ expect(result).toBe('resolved/path');
+ expect(originalRequireMock.resolve).toHaveBeenCalledWith('my-module');
+ });
+ });
+
+ describe('babelConfig', () => {
+ afterEach(() => {
+ jest.restoreAllMocks();
+ });
+
+ it('should return a Babel configuration object with specified presets and plugins', () => {
+ const result = babelConfig();
+
+ expect(result).toEqual({
+ presets: [[resolve('@babel/preset-env'), { targets: undefined }]],
+ plugins: [resolve('@babel/plugin-transform-runtime')],
+ });
+ });
+
+ it('should include the specified targets in the Babel configuration', () => {
+ const result = babelConfig({ targets: 'node 14' });
+
+ expect(result).toEqual({
+ presets: [[resolve('@babel/preset-env'), { targets: 'node 14' }]],
+ plugins: [resolve('@babel/plugin-transform-runtime')],
+ });
+ });
+ });
+});
diff --git a/packages/cli/tsconfig.json b/packages/cli/tsconfig.json
new file mode 100644
index 0000000000..5ff1b0865d
--- /dev/null
+++ b/packages/cli/tsconfig.json
@@ -0,0 +1,21 @@
+{
+ "compilerOptions": {
+ "allowJs": true,
+ "noImplicitThis": true,
+ "moduleResolution": "node",
+ "noUnusedLocals": true,
+ "allowUnreachableCode": false,
+ "module": "commonjs",
+ "target": "es2016",
+ "outDir": "dist",
+ "esModuleInterop": true,
+ "declaration": true,
+ "noImplicitReturns": false,
+ "noImplicitAny": false,
+ "strictNullChecks": false,
+ "resolveJsonModule": true,
+ "emitDecoratorMetadata": true,
+ "experimentalDecorators": true
+ },
+ "include": ["src/cli.ts"]
+}
diff --git a/packages/cli/webpack.cli.ts b/packages/cli/webpack.cli.ts
new file mode 100644
index 0000000000..3ef6c994f3
--- /dev/null
+++ b/packages/cli/webpack.cli.ts
@@ -0,0 +1,62 @@
+import webpack, { type Configuration } from 'webpack';
+import NodeExternals from 'webpack-node-externals';
+import CopyPlugin from 'copy-webpack-plugin';
+import ForkTsCheckerWebpackPlugin from 'fork-ts-checker-webpack-plugin';
+import { resolve } from 'path';
+
+const MODE = process.env.BUILD_MODE === 'production' ? 'production' : 'development';
+
+const config: Configuration = {
+ context: process.cwd(),
+ mode: MODE,
+ entry: './src/cli.ts',
+ output: {
+ filename: 'cli.js',
+ path: resolve(__dirname, 'dist'),
+ },
+ target: 'node',
+ stats: {
+ preset: 'minimal',
+ warnings: false,
+ },
+ module: {
+ rules: [
+ {
+ test: /\.(jsx?|tsx?)$/,
+ use: {
+ loader: 'babel-loader',
+ options: {
+ cacheDirectory: true,
+ presets: ['@babel/preset-typescript'],
+ assumptions: {
+ setPublicClassFields: false,
+ },
+ },
+ },
+ exclude: [/node_modules/],
+ },
+ ],
+ },
+ resolve: {
+ extensions: ['.ts', '.tsx', '.js', '.jsx', '.d.ts'],
+ },
+ plugins: [
+ new ForkTsCheckerWebpackPlugin(),
+ new webpack.BannerPlugin({ banner: '#!/usr/bin/env node', raw: true }),
+ new CopyPlugin({
+ patterns: [
+ { from: 'src/banner.txt', to: 'banner.txt' },
+ {
+ from: 'src/template',
+ to: 'template',
+ // Terser skip this file for minimization
+ info: { minimized: true },
+ },
+ ],
+ }),
+ ],
+ externalsPresets: { node: true },
+ externals: [NodeExternals()],
+};
+
+export default config;
diff --git a/packages/core/package.json b/packages/core/package.json
index ed4df321de..02657ddd67 100644
--- a/packages/core/package.json
+++ b/packages/core/package.json
@@ -41,7 +41,7 @@
},
"devDependencies": {
"@types/markdown-it": "14.1.2",
- "grapesjs-cli": "4.1.3",
+ "grapesjs-cli": "workspace:^",
"jest-environment-jsdom": "29.7.0",
"jsdom": "24.1.1",
"npm-run-all": "4.1.5",
@@ -67,13 +67,13 @@
"scripts": {
"build": "npm run build-all",
"build-all": "run-s build:*",
- "build:js": "grapesjs-cli build --patch=false --targets=\"> 1%, ie 11, safari 8, not dead\" --statsOutput=\"stats.json\" --localePath=\"src/i18n/locale\"",
- "build:mjs": "BUILD_MODULE=true grapesjs-cli build --dts='skip' --patch=false --targets=\"> 1%, ie 11, safari 8, not dead\"",
+ "build:js": "node node_modules/grapesjs-cli/dist/cli.js build --patch=false --targets=\"> 1%, ie 11, safari 8, not dead\" --statsOutput=\"stats.json\" --localePath=\"src/i18n/locale\"",
+ "build:mjs": "BUILD_MODULE=true node node_modules/grapesjs-cli/dist/cli.js build --dts='skip' --patch=false --targets=\"> 1%, ie 11, safari 8, not dead\"",
"build:css": "sass src/styles/scss/main.scss dist/css/grapes.min.css --no-source-map --style=compressed --load-path=node_modules",
- "ts:build": "grapesjs-cli build --dts='only' --patch=false",
+ "ts:build": "node node_modules/grapesjs-cli/dist/cli.js build --dts='only' --patch=false",
"ts:check": "tsc --noEmit --esModuleInterop dist/index.d.ts",
"start": "run-p start:*",
- "start:js": "grapesjs-cli serve",
+ "start:js": "node node_modules/grapesjs-cli/dist/cli.js serve",
"start:css": "npm run build:css -- --watch",
"test": "jest --forceExit",
"test:dev": "jest --watch"
diff --git a/pnpm-lock.yaml b/pnpm-lock.yaml
index 34ca0a54c9..5559d68de6 100644
--- a/pnpm-lock.yaml
+++ b/pnpm-lock.yaml
@@ -20,6 +20,9 @@ importers:
'@babel/preset-typescript':
specifier: 7.24.7
version: 7.24.7(@babel/core@7.25.2)
+ '@babel/runtime':
+ specifier: 7.25.6
+ version: 7.25.6
'@jest/globals':
specifier: 29.7.0
version: 29.7.0
@@ -38,6 +41,9 @@ importers:
'@typescript-eslint/parser':
specifier: 8.0.1
version: 8.0.1(eslint@8.57.0)(typescript@5.5.4)
+ babel-loader:
+ specifier: 9.1.3
+ version: 9.1.3(@babel/core@7.25.2)(webpack@5.94.0)
cross-env:
specifier: 7.0.3
version: 7.0.3
@@ -130,6 +136,79 @@ importers:
specifier: 3.6.20
version: 3.6.20
+ packages/cli:
+ dependencies:
+ '@babel/core':
+ specifier: 7.25.2
+ version: 7.25.2
+ '@babel/plugin-transform-runtime':
+ specifier: 7.25.4
+ version: 7.25.4(@babel/core@7.25.2)
+ '@babel/preset-env':
+ specifier: 7.25.4
+ version: 7.25.4(@babel/core@7.25.2)
+ '@babel/runtime':
+ specifier: 7.25.6
+ version: 7.25.6
+ babel-loader:
+ specifier: 9.1.3
+ version: 9.1.3(@babel/core@7.25.2)(webpack@5.94.0(webpack-cli@5.1.4))
+ chalk:
+ specifier: ^4.1.2
+ version: 4.1.2
+ core-js:
+ specifier: 3.38.1
+ version: 3.38.1
+ dts-bundle-generator:
+ specifier: ^8.0.1
+ version: 8.1.2
+ html-webpack-plugin:
+ specifier: 5.6.0
+ version: 5.6.0(webpack@5.94.0(webpack-cli@5.1.4))
+ inquirer:
+ specifier: ^8.2.5
+ version: 8.2.6
+ listr:
+ specifier: ^0.14.3
+ version: 0.14.3
+ lodash.template:
+ specifier: ^4.5.0
+ version: 4.5.0
+ rimraf:
+ specifier: ^4.1.2
+ version: 4.4.1
+ spdx-license-list:
+ specifier: ^6.6.0
+ version: 6.9.0
+ terser-webpack-plugin:
+ specifier: ^5.3.10
+ version: 5.3.10(webpack@5.94.0(webpack-cli@5.1.4))
+ webpack:
+ specifier: 5.94.0
+ version: 5.94.0(webpack-cli@5.1.4)
+ webpack-cli:
+ specifier: 5.1.4
+ version: 5.1.4(webpack-dev-server@5.1.0)(webpack@5.94.0)
+ webpack-dev-server:
+ specifier: 5.1.0
+ version: 5.1.0(webpack-cli@5.1.4)(webpack@5.94.0)
+ yargs:
+ specifier: ^17.6.2
+ version: 17.7.2
+ devDependencies:
+ '@types/webpack-node-externals':
+ specifier: ^3.0.0
+ version: 3.0.4(webpack-cli@5.1.4(webpack-dev-server@5.1.0)(webpack@5.94.0))
+ copy-webpack-plugin:
+ specifier: ^11.0.0
+ version: 11.0.0(webpack@5.94.0(webpack-cli@5.1.4))
+ fork-ts-checker-webpack-plugin:
+ specifier: ^8.0.0
+ version: 8.0.0(typescript@5.5.4)(webpack@5.94.0(webpack-cli@5.1.4))
+ webpack-node-externals:
+ specifier: ^3.0.0
+ version: 3.0.0
+
packages/core:
dependencies:
'@types/backbone':
@@ -161,8 +240,8 @@ importers:
specifier: 14.1.2
version: 14.1.2
grapesjs-cli:
- specifier: 4.1.3
- version: 4.1.3(@types/node@22.4.1)(typescript@5.5.4)
+ specifier: workspace:^
+ version: link:../cli
jest-environment-jsdom:
specifier: 29.7.0
version: 29.7.0
@@ -961,6 +1040,24 @@ packages:
'@jridgewell/trace-mapping@0.3.9':
resolution: {integrity: sha512-3Belt6tdc8bPgAtbcmdtNJlirVoTmEb5e2gC94PnkwEW9jI6CAHUeoG85tjWP5WquqfavoMtMwiG4P926ZKKuQ==}
+ '@jsonjoy.com/base64@1.1.2':
+ resolution: {integrity: sha512-q6XAnWQDIMA3+FTiOYajoYqySkO+JSat0ytXGSuRdq9uXE7o92gzuQwQM14xaCRlBLGq3v5miDGC4vkVTn54xA==}
+ engines: {node: '>=10.0'}
+ peerDependencies:
+ tslib: '2'
+
+ '@jsonjoy.com/json-pack@1.1.0':
+ resolution: {integrity: sha512-zlQONA+msXPPwHWZMKFVS78ewFczIll5lXiVPwFPCZUsrOKdxc2AvxU1HoNBmMRhqDZUR9HkC3UOm+6pME6Xsg==}
+ engines: {node: '>=10.0'}
+ peerDependencies:
+ tslib: '2'
+
+ '@jsonjoy.com/util@1.3.0':
+ resolution: {integrity: sha512-Cebt4Vk7k1xHy87kHY7KSPLT77A7Ev7IfOblyLZhtYEhrdQ6fX4EoLq3xOQ3O/DRMEh2ok5nyC180E+ABS8Wmw==}
+ engines: {node: '>=10.0'}
+ peerDependencies:
+ tslib: '2'
+
'@leichtgewicht/ip-codec@2.0.5':
resolution: {integrity: sha512-Vo+PSpZG2/fmgmiNzYK9qWRh8h/CHrwD0mo1h1DzL4yzHNSfWYujGTYsWGreD000gcgmZ7K4Ys6Tx9TxtsKdDw==}
@@ -1159,6 +1256,9 @@ packages:
'@types/normalize-package-data@2.4.4':
resolution: {integrity: sha512-37i+OaWTh9qeK4LSHPsyRC7NahnGotNuZvjLSgcPzblpHB3rrCJxAOgI5gCdKm7coonsaX1Of0ILiTcnZjbfxA==}
+ '@types/parse-json@4.0.2':
+ resolution: {integrity: sha512-dISoDXWWQwUquiKsyZ4Ng+HX2KsPL7LyHKHQwgGFEA3IaKac4Obd+h2a/a6waisAoepJlBcx9paWqjA8/HVjCw==}
+
'@types/parse5@6.0.3':
resolution: {integrity: sha512-SuT16Q1K51EAVPz1K29DJ/sXjhSQ0zjvsypYJ6tlwVsRV9jwW5Adq2ch8Dq8kDBCkYnELS7N7VNCSB5nC56t/g==}
@@ -1174,8 +1274,8 @@ packages:
'@types/responselike@1.0.3':
resolution: {integrity: sha512-H/+L+UkTV33uf49PH5pCAUBVPNj2nDBXTN+qS1dOwyyg24l3CcicicCA7ca+HMvJBZcFgl5r8e+RR6elsb4Lyw==}
- '@types/retry@0.12.0':
- resolution: {integrity: sha512-wWKOClTTiizcZhXnPY4wikVAwmdYHp8q6DmC+EJUzAMsycb7HB32Kh9RN4+0gExjmPmZSAQjgURXIGATPegAvA==}
+ '@types/retry@0.12.2':
+ resolution: {integrity: sha512-XISRgDJ2Tc5q4TRqvgJtzsRkFYNJzZrhTdtMoGVBttwzzQJkPnS3WWTFc7kuDRoPtPakl+T+OfdEUjYJj7Jbow==}
'@types/send@0.17.4':
resolution: {integrity: sha512-x2EM6TJOybec7c52BX0ZspPodMsQUd5L6PRwOunVyVUhXiBSKf3AezDL8Dgvgt5o0UfKNfuA0eMLr2wLT4AiBA==}
@@ -1219,6 +1319,9 @@ packages:
'@types/webpack-dev-server@3.11.6':
resolution: {integrity: sha512-XCph0RiiqFGetukCTC3KVnY1jwLcZ84illFRMbyFzCcWl90B/76ew0tSqF46oBhnLC4obNDG7dMO0JfTN0MgMQ==}
+ '@types/webpack-node-externals@3.0.4':
+ resolution: {integrity: sha512-8Z3/edqxE3RRlOJwKSgOFxLZRt/i1qFlv/Bi308ZUKo9jh8oGngd9r8GR0ZNKW5AEJq8QNQE3b17CwghTjQ0Uw==}
+
'@types/webpack-sources@3.2.3':
resolution: {integrity: sha512-4nZOdMwSPHZ4pTEZzSp0AsTM4K7Qmu40UKW4tJDiOVs20UzYF9l+qUe4s0ftfN0pin06n+5cWWDJXH+sbhAiDw==}
@@ -2123,6 +2226,10 @@ packages:
builtin-status-codes@3.0.0:
resolution: {integrity: sha512-HpGFw18DgFWlncDfjTa2rcQ4W88O1mC8e8yZ2AvQY5KDaktSTwo+KRf6nHK6FRI5FyRyb/5T6+TSxfP7QyGsmQ==}
+ bundle-name@4.1.0:
+ resolution: {integrity: sha512-tjwM5exMg6BGRI+kNmTntNsvdZS1X8BFYS6tnJ2hdH0kVxM6/eVZ2xy+FqStSWvYmtfFMDLIxurorHwDKfDz5Q==}
+ engines: {node: '>=18'}
+
bundle-require@2.1.8:
resolution: {integrity: sha512-oOEg3A0hy/YzvNWNowtKD0pmhZKseOFweCbgyMqTIih4gRY1nJWsvrOCT27L9NbIyL5jMjTFrAUpGxxpW68Puw==}
peerDependencies:
@@ -2636,6 +2743,12 @@ packages:
resolution: {integrity: sha512-XgZ0pFcakEUlbwQEVNg3+QAis1FyTL3Qel9FYy8pSkQqoG3PNoT0bOCQtOXcOkur21r2Eq2kI+IE+gsmAEVlYw==}
engines: {node: '>=0.10.0'}
+ copy-webpack-plugin@11.0.0:
+ resolution: {integrity: sha512-fX2MWpamkW0hZxMEg0+mYnA40LTosOSa5TqZ9GYIBzyJa9C3QUaMPSE2xAi/buNr8u89SfD9wHSQVBzrRa/SOQ==}
+ engines: {node: '>= 14.15.0'}
+ peerDependencies:
+ webpack: ^5.1.0
+
copy-webpack-plugin@5.1.2:
resolution: {integrity: sha512-Uh7crJAco3AjBvgAy9Z75CjK8IG+gxaErro71THQ+vv/bl4HaQcpkexAY8KVW/T6D2W2IRr+couF/knIRkZMIQ==}
engines: {node: '>= 6.9.0'}
@@ -2658,6 +2771,10 @@ packages:
resolution: {integrity: sha512-H65gsXo1SKjf8zmrJ67eJk8aIRKV5ff2D4uKZIBZShbhGSpEmsQOPW/SKMKYhSTrqR7ufy6RP69rPogdaPh/kA==}
engines: {node: '>=4'}
+ cosmiconfig@7.1.0:
+ resolution: {integrity: sha512-AdmX6xUzdNASswsFtmwSt7Vj8po9IuqXm0UXz7QKPuEUmPB4XyjGfaAr2PSuELMwkRMVH1EpIkX5bTZGRB3eCA==}
+ engines: {node: '>=10'}
+
create-ecdh@4.0.4:
resolution: {integrity: sha512-mf+TCx8wWc9VpuxfP2ht0iSISLZnt0JgWlrOKZiNqyUZWnjIaCIVNQArMHnCZKfEYRg6IM7A+NeJoN8gf/Ws0A==}
@@ -2912,14 +3029,18 @@ packages:
resolution: {integrity: sha512-3sUqbMEc77XqpdNO7FRyRog+eW3ph+GYCbj+rK+uYyRMuwsVy0rMiVtPn+QJlKFvWP/1PYpapqYn0Me2knFn+A==}
engines: {node: '>=0.10.0'}
+ default-browser-id@5.0.0:
+ resolution: {integrity: sha512-A6p/pu/6fyBcA1TRz/GqWYPViplrftcW2gZC9q79ngNCKAeR/X3gcEdXQHl4KNXV+3wgIJ1CPkJQ3IHM6lcsyA==}
+ engines: {node: '>=18'}
+
+ default-browser@5.2.1:
+ resolution: {integrity: sha512-WY/3TUME0x3KPYdRRxEJJvXRHV4PyPoUsxtZa78lwItwRQRHhd2U9xOscaT/YTf8uCXIAjeJOFBVEh/7FtD8Xg==}
+ engines: {node: '>=18'}
+
default-gateway@4.2.0:
resolution: {integrity: sha512-h6sMrVB1VMWVrW13mSc6ia/DwYYw5MN6+exNu1OaJeFac5aSAvwM7lZ0NVfTABuSkQelr4h5oebg3KB1XPdjgA==}
engines: {node: '>=6'}
- default-gateway@6.0.3:
- resolution: {integrity: sha512-fwSOJsbbNzZ/CUFpqFBqYfYNLj1NbMPm8MMCIzHjC83iSJRBEGmDUxU+WP661BaBQImeC2yHwXtz+P/O9o+XEg==}
- engines: {node: '>= 10'}
-
defaults@1.0.4:
resolution: {integrity: sha512-eFuaLoy/Rxalv2kr+lqMlUnrDWV+3j4pljOIJgLIhI058IQfWJ7vXhyEIHu+HtC738klGALYxOKDO0bQP3tg8A==}
@@ -2930,9 +3051,9 @@ packages:
resolution: {integrity: sha512-rBMvIzlpA8v6E+SJZoo++HAYqsLrkg7MSfIinMPFhmkorw7X+dOXVJQs+QT69zGkzMyfDnIMN2Wid1+NbL3T+A==}
engines: {node: '>= 0.4'}
- define-lazy-prop@2.0.0:
- resolution: {integrity: sha512-Ds09qNh8yw3khSjiJjiUInaGX9xlqZDY7JVryGxdxV7NPeuqQfplOpQ66yJFZut3jLa5zOwkXw1g9EI2uKh4Og==}
- engines: {node: '>=8'}
+ define-lazy-prop@3.0.0:
+ resolution: {integrity: sha512-N+MeXYoqr3pOgn8xfyRPREN7gHakLYjhsHhWGT3fWAiL4IkAt0iDw14QiiEm2bE30c5XX5q0FtAA3CK5f9/BUg==}
+ engines: {node: '>=12'}
define-properties@1.2.1:
resolution: {integrity: sha512-8QmQKqEASLd5nx0U1B1okLElbUuuttJ/AnYmRXbbbGDWh6uS208EjD4Xqq/I9wK7u0v6O08XhTWnt5XtEbR6Dg==}
@@ -3727,6 +3848,13 @@ packages:
forever-agent@0.6.1:
resolution: {integrity: sha512-j0KLYPhm6zeac4lz3oJ3o65qvgQCcPubiyotZrXqEaG4hNagNYO8qdlUrX5vwqv9ohqeT/Z3j6+yW067yWWdUw==}
+ fork-ts-checker-webpack-plugin@8.0.0:
+ resolution: {integrity: sha512-mX3qW3idpueT2klaQXBzrIM/pHw+T0B/V9KHEvNrqijTq9NFnMZU6oreVxDYcf33P8a5cW+67PjodNHthGnNVg==}
+ engines: {node: '>=12.13.0', yarn: '>=1.0.0'}
+ peerDependencies:
+ typescript: '>3.6.0'
+ webpack: ^5.11.0
+
form-data@2.3.3:
resolution: {integrity: sha512-1lLKB2Mu3aGP1Q/2eCOx0fNbRMe7XdwktwOruhfqqd0rIJWwN4Dh+E3hrPSlDCXnSR7UtZ1N38rVXm+6+MEhJQ==}
engines: {node: '>= 0.12'}
@@ -3750,6 +3878,10 @@ packages:
from2@2.3.0:
resolution: {integrity: sha512-OMcX/4IC/uqEPVgGeyfN22LJk6AZrMkRZHxcHBMBvHScDGgwTm2GT2Wkgtocyd3JfZffjj2kYUDXXII0Fk9W0g==}
+ fs-extra@10.1.0:
+ resolution: {integrity: sha512-oRXApq54ETRj4eMiFzGnHWGy+zo5raudjuxN0b8H7s/RU2oW0Wvsx9O0ACRN/kRq9E8Vu/ReskGB5o3ji+FzHQ==}
+ engines: {node: '>=12'}
+
fs-extra@7.0.1:
resolution: {integrity: sha512-YJDaCJZEnBmcbw13fvdAM9AwNOJwOzrE4pqMqBq5nFiEqXUqHwlK4B+3pUw6JNvfSPtX05xFHtYy/1ni01eGCw==}
engines: {node: '>=6 <7 || >=8'}
@@ -3902,6 +4034,10 @@ packages:
resolution: {integrity: sha512-jhIXaOzy1sb8IyocaruWSn1TjmnBVs8Ayhcy83rmxNJ8q2uWKCAj3CnJY+KpGSXCueAPc0i05kVvVKtP1t9S3g==}
engines: {node: '>=10'}
+ globby@13.2.2:
+ resolution: {integrity: sha512-Y1zNGV+pzQdh7H39l9zgB4PJqjRNqydvdYCDG4HFXM4XuvSaQQlEc91IU1yALL8gUTDomgBAfz3XJdmUS+oo0w==}
+ engines: {node: ^12.20.0 || ^14.13.1 || >=16.0.0}
+
globby@6.1.0:
resolution: {integrity: sha512-KVbFv2TQtbzCoxAnfD6JcHZTYCzyliEaaeM/gH8qQdkKr5s0OP9scEgvdcngyk7AVdY6YVW/TJHd+lQ/Df3Daw==}
engines: {node: '>=0.10.0'}
@@ -3924,11 +4060,6 @@ packages:
graceful-fs@4.2.11:
resolution: {integrity: sha512-RbJ5/jmFcNNCcDV5o9eTnBLJ/HszWV0P73bc+Ff4nS/rJj+YaS6IGyiOL0VoBYX+l1Wrl3k63h/KrH+nhJ0XvQ==}
- grapesjs-cli@4.1.3:
- resolution: {integrity: sha512-T5Rbl2dHi6pD7RIS7WqE8fdt9CmZcy+jVFHEuBHd9CIFKN520E06cFIIE86bhFMymlYpbdmTsG7R2dVDqqKrWQ==}
- engines: {node: '>=14.15.0'}
- hasBin: true
-
graphemer@1.4.0:
resolution: {integrity: sha512-EtKwoO6kxCL9WO5xipiHTZlSzBm7WLT627TqC/uVRd0HKmq8NXyebnNYxDoBi7wt8eTWrUrKXCOVaFq9x1kgag==}
@@ -4203,6 +4334,10 @@ packages:
resolution: {integrity: sha512-B4FFZ6q/T2jhhksgkbEW3HBvWIfDW85snkQgawt07S7J5QXTk6BkNV+0yAeZrM5QpMAdYlocGoljn0sJ/WQkFw==}
engines: {node: '>=10.17.0'}
+ hyperdyperid@1.2.0:
+ resolution: {integrity: sha512-Y93lCzHYgGWdrJ66yIktxiaGULYc6oGiABxhcO5AufBeOyoIdZF7bIfLaOrbM0iGIOXQQgxxRrFEnb+Y6w1n4A==}
+ engines: {node: '>=10.18'}
+
iconv-lite@0.4.24:
resolution: {integrity: sha512-v3MXnZAcvnywkTUEZomIActle7RXXeedOR31wwl7VlyoXO4Qi9arvSenNQWne1TcRwhCL1HwLI21bEqdpj8/rA==}
engines: {node: '>=0.10.0'}
@@ -4430,9 +4565,9 @@ packages:
resolution: {integrity: sha512-yVChGzahRFvbkscn2MlwGismPO12i9+znNruC5gVEntG3qu0xQMzsGg/JFbrsqDOHtHFPci+V5aP5T9I+yeKqw==}
engines: {node: '>=0.10.0'}
- is-docker@2.2.1:
- resolution: {integrity: sha512-F+i2BKsFrH66iaUFc0woD8sLy8getkwTwtOBjvs56Cx4CgJDeKQeqfz8wAYiSb8JOprWhHH5p77PbmYCvvUuXQ==}
- engines: {node: '>=8'}
+ is-docker@3.0.0:
+ resolution: {integrity: sha512-eljcgEDlEns/7AXFosB5K/2nCM4P7FQPkGc/DWLy5rmFEWvZayGrik1d9/QIY5nJ4f9YsVvBkA6kJpHn9rISdQ==}
+ engines: {node: ^12.20.0 || ^14.13.1 || >=16.0.0}
hasBin: true
is-extendable@0.1.1:
@@ -4471,6 +4606,11 @@ packages:
resolution: {integrity: sha512-xelSayHH36ZgE7ZWhli7pW34hNbNl8Ojv5KVmkJD4hBdD3th8Tfk9vYasLM+mXWOZhFkgZfxhLSnrwRr4elSSg==}
engines: {node: '>=0.10.0'}
+ is-inside-container@1.0.0:
+ resolution: {integrity: sha512-KIYLCCJghfHZxqjYBE7rEy0OBuTd5xCHS7tHVgvCLkx7StIoaxwNW3hCALgEUjFfeRk+MG/Qxmp/vtETEF3tRA==}
+ engines: {node: '>=14.16'}
+ hasBin: true
+
is-installed-globally@0.3.2:
resolution: {integrity: sha512-wZ8x1js7Ia0kecP/CHM/3ABkAmujX7WPvQk6uu3Fly/Mk44pySulQpnHG46OMjHGXApINnV4QhY3SWnECO2z5g==}
engines: {node: '>=8'}
@@ -4483,6 +4623,10 @@ packages:
resolution: {integrity: sha512-5KoIu2Ngpyek75jXodFvnafB6DJgr3u8uuK0LEZJjrU19DrMD3EVERaR8sjz8CCGgpZvxPl9SuE1GMVPFHx1mw==}
engines: {node: '>= 0.4'}
+ is-network-error@1.1.0:
+ resolution: {integrity: sha512-tUdRRAnhT+OtCZR/LxZelH/C7QtjtFrTu5tXCA8pl55eTUElUHT+GPYV8MBMBvea/j+NxQqVt3LbWMRir7Gx9g==}
+ engines: {node: '>=16'}
+
is-npm@4.0.0:
resolution: {integrity: sha512-96ECIfh9xtDDlPylNPXhzjsykHsMJZ18ASpaWzQyBr4YRTcVjUvzaHayDAES2oU/3KpljhHUjtSRNiDwi0F0ig==}
engines: {node: '>=8'}
@@ -4605,9 +4749,9 @@ packages:
resolution: {integrity: sha512-gfygJYZ2gLTDlmbWMI0CE2MwnFzSN/2SZfkMlItC4K/JBlsWVDB0bO6XhqcY13YXE7iMcAJnzTCJjPiTeJJ0Mw==}
engines: {node: '>=4'}
- is-wsl@2.2.0:
- resolution: {integrity: sha512-fKzAra0rGJUUBwGBgNkHZuToZcn+TtXHpeCgmkMJMMYx1sQDYaCSyjJBSCa2nH1DGm7s3n1oBnohoVTBaN7Lww==}
- engines: {node: '>=8'}
+ is-wsl@3.1.0:
+ resolution: {integrity: sha512-UcVfVfaK4Sc4m7X3dUSoHoozQGBEFeDC+zVo06t98xe8CzHSZZBekNXH+tu0NalHolcJ/QAGqS46Hef7QXBIMw==}
+ engines: {node: '>=16'}
is-yarn-global@0.3.0:
resolution: {integrity: sha512-VjSeb/lHmkoyd8ryPVIKvOCn4D1koMqY+vqyjjUfc3xyKtP4dYOxM44sZrnqQSzSds3xyOrUTLTC9LVCVgLngw==}
@@ -4893,6 +5037,9 @@ packages:
jsonfile@4.0.0:
resolution: {integrity: sha512-m6F1R3z8jjlf2imQHS2Qez5sjKWQzbuuhuJ/FKYFRZvPE3PuHcSMVZzfsLhGVOkfd20obL5SWEBew5ShlquNxg==}
+ jsonfile@6.1.0:
+ resolution: {integrity: sha512-5dgndWOriYSm5cnYaJNhalLNDKOqFwyDB/rr1E9ZsGciGvKPs8R2xYGCacuf3z6K1YKDz182fd+fY3cn3pMqXQ==}
+
jsprim@1.4.2:
resolution: {integrity: sha512-P2bSOMAc/ciLz6DzgjVlGJP9+BrJWu5UDGK70C2iweC5QBIeFf0ZXRvGjEj2uYgrY2MkAAhsSWHDWlFtEroZWw==}
engines: {node: '>=0.6.0'}
@@ -5214,6 +5361,10 @@ packages:
resolution: {integrity: sha512-UERzLsxzllchadvbPs5aolHh65ISpKpM+ccLbOJ8/vvpBKmAWf+la7dXFy7Mr0ySHbdHrFv5kGFCUHHe6GFEmw==}
engines: {node: '>= 4.0.0'}
+ memfs@4.11.1:
+ resolution: {integrity: sha512-LZcMTBAgqUUKNXZagcZxvXXfgF1bHX7Y7nQ0QyEiNbRJgE29GhgPd8Yna1VQcLlPiHt/5RFJMWYN9Uv/VPNvjQ==}
+ engines: {node: '>= 4.0.0'}
+
memory-fs@0.4.1:
resolution: {integrity: sha512-cda4JKCxReDXFXRqOHPQscuIYg1PvxbE2S2GP45rnwfEK+vZaXC8C1OFvdHIbgw0DLzowXGVoxLaAmlgRy14GQ==}
@@ -5497,6 +5648,9 @@ packages:
no-case@3.0.4:
resolution: {integrity: sha512-fgAN3jGAh+RoxUGZHTSOLJIqUc2wmoBwGR4tbpNAKmmovFoWq0OdRkb0VkldReO2a2iBT/OEulG9XSUc10r3zg==}
+ node-abort-controller@3.1.1:
+ resolution: {integrity: sha512-AGK2yQKIjRuqnc6VkX2Xj5d+QW8xZ87pa1UK6yA6ouUyuxfHuMP6umE5QK7UmTeOAymo+Zx1Fxiuw9rVx8taHQ==}
+
node-forge@0.10.0:
resolution: {integrity: sha512-PPmu8eEeG9saEUvI97fm4OYxXVB6bFvyNTyiUOBichBpFG8A1Ljw3bY62+5oOjDEMHRnd0Y7HQ+x7uzxOzC6JA==}
engines: {node: '>= 6.0.0'}
@@ -5654,9 +5808,9 @@ packages:
resolution: {integrity: sha512-kbpaSSGJTWdAY5KPVeMOKXSrPtr8C8C7wodJbcsd51jRnmD+GZu8Y0VoU6Dm5Z4vWr0Ig/1NKuWRKf7j5aaYSg==}
engines: {node: '>=6'}
- open@8.4.2:
- resolution: {integrity: sha512-7x81NCL719oNbsq/3mh+hVrAWmFuEYUqrq/Iw3kUzH8ReypT9QQ0BLoJS7/G9k6N81XjW4qHWtjWwe/9eLy1EQ==}
- engines: {node: '>=12'}
+ open@10.1.0:
+ resolution: {integrity: sha512-mnkeQ1qP5Ue2wd+aivTD3NHd/lZ96Lu0jgf0pwktLPtx6cTZiH7tyeGRRHs0zX0rbrahXPnXlUnbeXyaBBuIaw==}
+ engines: {node: '>=18'}
opencollective-postinstall@2.0.3:
resolution: {integrity: sha512-8AV/sCtuzUeTo8gQK5qDZzARrulB3egtLzFgteqB2tcT4Mw7B8Kt7JcDHmltjz6FOAHsvTevk70gZEbhM4ZS9Q==}
@@ -5730,9 +5884,9 @@ packages:
resolution: {integrity: sha512-XE6G4+YTTkT2a0UWb2kjZe8xNwf8bIbnqpc/IS/idOBVhyves0mK5OJgeocjx7q5pvX/6m23xuzVPYT1uGM73w==}
engines: {node: '>=6'}
- p-retry@4.6.2:
- resolution: {integrity: sha512-312Id396EbJdvRONlngUx0NydfrIQ5lsYu0znKVUzVvArzEIt08V1qhtyESbGVd1FGX7UKtiFp5uwKZdM8wIuQ==}
- engines: {node: '>=8'}
+ p-retry@6.2.0:
+ resolution: {integrity: sha512-JA6nkq6hKyWLLasXQXUrO4z8BUZGUt/LjlJxx8Gb2+2ntodU/SS63YZ8b0LUTbQ8ZB9iwOfhEPhg4ykKnn2KsA==}
+ engines: {node: '>=16.17'}
p-try@2.2.0:
resolution: {integrity: sha512-R4nPAVTAU0B9D35/Gk3uJf/7XYbQcyohSKdvAxIRSNghFl4e71hVoGnBNQz9cWaXxO2I10KTC+3jMdvvoKw6dQ==}
@@ -6494,6 +6648,10 @@ packages:
rrweb-cssom@0.7.1:
resolution: {integrity: sha512-TrEMa7JGdVm0UThDJSx7ddw5nVm3UJS9o9CCIZ72B1vSyEZoziDqBYP3XIoi/12lKrJR8rE3jeFHMok2F/Mnsg==}
+ run-applescript@7.0.0:
+ resolution: {integrity: sha512-9by4Ij99JUr/MCFBUkDKLWK3G9HVXmabKz9U5MlIAIuvuzkiOicRYs8XJLxX+xahD+mLiiCYDqF9dKAgtzKP1A==}
+ engines: {node: '>=18'}
+
run-async@2.4.1:
resolution: {integrity: sha512-tvVnVv01b8c1RrA6Ep7JkStj85Guv/YrMcwqYQnwjsAS2cTmmPGBBjAjpCW7RrSodNSoE2/qg9O4bceNvUuDgQ==}
engines: {node: '>=0.12.0'}
@@ -6688,6 +6846,10 @@ packages:
resolution: {integrity: sha512-g9Q1haeby36OSStwb4ntCGGGaKsaVSjQ68fBxoQcutl5fS1vuY18H3wSt3jFyFtrkx+Kz0V1G85A4MyAdDMi2Q==}
engines: {node: '>=8'}
+ slash@4.0.0:
+ resolution: {integrity: sha512-3dOsAHXXUkQTpOYcoAxLIorMTp4gIQr5IW3iVb7A7lFIp0VHhnynm9izx6TssdrIcVIESAlVjtnO2K8bg+Coew==}
+ engines: {node: '>=12'}
+
slice-ansi@0.0.4:
resolution: {integrity: sha512-up04hB2hR92PgjpyU3y/eg91yIBILyjVY26NvvciY3EVVPjybkMszMpXQ9QAkcS3I5rtJBDLoTxxg+qvW8c7rw==}
engines: {node: '>=0.10.0'}
@@ -7051,6 +7213,12 @@ packages:
text-table@0.2.0:
resolution: {integrity: sha512-N+8UisAXDGk8PFXP4HAzVR9nbfmVJ3zYLAWiTIoqC5v5isinhr+r5uaO8+7r3BMfuNIufIsA7RdpVgacC2cSpw==}
+ thingies@1.21.0:
+ resolution: {integrity: sha512-hsqsJsFMsV+aD4s3CWKk85ep/3I9XzYV/IXaSouJMYIoDlgyi11cBhsqYe9/geRfB0YIikBQg6raRaM+nIMP9g==}
+ engines: {node: '>=10.18'}
+ peerDependencies:
+ tslib: ^2
+
through2@2.0.5:
resolution: {integrity: sha512-/mrRod8xqpA+IHSLyGCQ2s8SPHiCDEeQJSep1jqLYeEUClOFG2Qsh+4FU6G9VeqpZnGW/Su8LQGc4YKni5rYSQ==}
@@ -7130,6 +7298,12 @@ packages:
resolution: {integrity: sha512-tk2G5R2KRwBd+ZN0zaEXpmzdKyOYksXwywulIX95MBODjSzMIuQnQ3m8JxgbhnL1LeVo7lqQKsYa1O3Htl7K5g==}
engines: {node: '>=18'}
+ tree-dump@1.0.2:
+ resolution: {integrity: sha512-dpev9ABuLWdEubk+cIaI9cHwRNNDjkBBLXTwI4UCUFdQ5xXKqNXoK4FEciw/vxf+NQ7Cb7sGUyeUtORvHIdRXQ==}
+ engines: {node: '>=10.0'}
+ peerDependencies:
+ tslib: '2'
+
trim-lines@3.0.1:
resolution: {integrity: sha512-kRj8B+YHZCc9kQYdWfJB2/oUl9rA99qbowYYBtr4ui4mZyAQ2JpvVBd/6U2YloATfqBhBTSMhTpgBHtU0Mf3Rg==}
@@ -7349,6 +7523,10 @@ packages:
resolution: {integrity: sha512-CJ1QgKmNg3CwvAv/kOFmtnEN05f0D/cn9QntgNOQlQF9dgvVTHj3t+8JPdjqawCHk7V/KA+fbUqzZ9XWhcqPUg==}
engines: {node: '>= 4.0.0'}
+ universalify@2.0.1:
+ resolution: {integrity: sha512-gptHNQghINnc/vTGIk0SOFGFNXw7JVrlRUtConJRlvaw6DuX0wO5Jeko9sWrMBhh+PsYAZ7oXAiOnf/UKogyiw==}
+ engines: {node: '>= 10.0.0'}
+
unpipe@1.0.0:
resolution: {integrity: sha512-pjy2bYhSsufwWlKwPc+l3cN7+wuJlK6uz0YdJEOlQDbl6jo/YlPi4mb8agUkVC8BF7V8NuzeyPNqRksA3hztKQ==}
engines: {node: '>= 0.8'}
@@ -7609,11 +7787,14 @@ packages:
peerDependencies:
webpack: ^4.0.0 || ^5.0.0
- webpack-dev-middleware@5.3.4:
- resolution: {integrity: sha512-BVdTqhhs+0IfoeAf7EoH5WE+exCmqGerHfDM0IL096Px60Tq2Mn9MAbnaGUe6HiMa41KMCYF19gyzZmBcq/o4Q==}
- engines: {node: '>= 12.13.0'}
+ webpack-dev-middleware@7.4.2:
+ resolution: {integrity: sha512-xOO8n6eggxnwYpy1NlzUKpvrjfJTvae5/D6WOK0S2LSo7vjmo5gCM1DbLUmFqrMTJP+W/0YZNctm7jasWvLuBA==}
+ engines: {node: '>= 18.12.0'}
peerDependencies:
- webpack: ^4.0.0 || ^5.0.0
+ webpack: ^5.0.0
+ peerDependenciesMeta:
+ webpack:
+ optional: true
webpack-dev-server@3.11.3:
resolution: {integrity: sha512-3x31rjbEQWKMNzacUZRE6wXvUFuGpH7vr0lIEbYpMAG9BOxi0928QU1BBswOAP3kg3H1O4hiS+sq4YyAn6ANnA==}
@@ -7626,12 +7807,12 @@ packages:
webpack-cli:
optional: true
- webpack-dev-server@4.15.2:
- resolution: {integrity: sha512-0XavAZbNJ5sDrCbkpWL8mia0o5WPOd2YGtxrEiZkBK9FjLppIUK2TgxK6qGD2P3hUXTJNNPVibrerKcx5WkR1g==}
- engines: {node: '>= 12.13.0'}
+ webpack-dev-server@5.1.0:
+ resolution: {integrity: sha512-aQpaN81X6tXie1FoOB7xlMfCsN19pSvRAeYUHOdFWOlhpQ/LlbfTqYwwmEDFV0h8GGuqmCmKmT+pxcUV/Nt2gQ==}
+ engines: {node: '>= 18.12.0'}
hasBin: true
peerDependencies:
- webpack: ^4.37.0 || ^5.0.0
+ webpack: ^5.0.0
webpack-cli: '*'
peerDependenciesMeta:
webpack:
@@ -7650,6 +7831,10 @@ packages:
resolution: {integrity: sha512-+4zXKdx7UnO+1jaN4l2lHVD+mFvnlZQP/6ljaJVb4SZiwIKeUnrT5l0gkT8z+n4hKpC+jpOv6O9R+gLtag7pSA==}
engines: {node: '>=10.0.0'}
+ webpack-node-externals@3.0.0:
+ resolution: {integrity: sha512-LnL6Z3GGDPht/AigwRh2dvL9PQPFQ8skEpVrWZXLWBYmqcaojHNN0onvHzie6rq7EWKrrBfPYqNEzTJgiwEQDQ==}
+ engines: {node: '>=6'}
+
webpack-sources@1.4.3:
resolution: {integrity: sha512-lgTS3Xhv1lCOKo7SA5TjKXMjpSM4sBjNV5+q2bqesbSPs5FjGmU6jjtBSkX9b4qW87vDIsCIlUPOEhbZrMdjeQ==}
@@ -7841,6 +8026,10 @@ packages:
yallist@4.0.0:
resolution: {integrity: sha512-3wdGidZyq5PB084XLES5TpOSRA3wjXAlIWMhum2kRcv/41Sn2emQ0dycQW4uZXLejwKvg6EsvbdlVL+FYEct7A==}
+ yaml@1.10.2:
+ resolution: {integrity: sha512-r3vXyErRCYJ7wg28yvBY5VSoAF8ZvlcW9/BwUzEtUsjvX/DKs24dIkuwjtuprwJJHsbyUbLApepYTR1BN4uHrg==}
+ engines: {node: '>= 6'}
+
yargs-parser@13.1.2:
resolution: {integrity: sha512-3lbsNRf/j+A4QuSZfDRA7HRSfWrzO0YjqTJd5kjAq37Zep1CEgaYmrH9Q3GwPiB9cHyd1Y1UwggGhJGoxipbzg==}
@@ -8968,6 +9157,22 @@ snapshots:
'@jridgewell/resolve-uri': 3.1.2
'@jridgewell/sourcemap-codec': 1.5.0
+ '@jsonjoy.com/base64@1.1.2(tslib@2.7.0)':
+ dependencies:
+ tslib: 2.7.0
+
+ '@jsonjoy.com/json-pack@1.1.0(tslib@2.7.0)':
+ dependencies:
+ '@jsonjoy.com/base64': 1.1.2(tslib@2.7.0)
+ '@jsonjoy.com/util': 1.3.0(tslib@2.7.0)
+ hyperdyperid: 1.2.0
+ thingies: 1.21.0(tslib@2.7.0)
+ tslib: 2.7.0
+
+ '@jsonjoy.com/util@1.3.0(tslib@2.7.0)':
+ dependencies:
+ tslib: 2.7.0
+
'@leichtgewicht/ip-codec@2.0.5': {}
'@mrmlnc/readdir-enhanced@2.2.1':
@@ -9186,6 +9391,8 @@ snapshots:
'@types/normalize-package-data@2.4.4': {}
+ '@types/parse-json@4.0.2': {}
+
'@types/parse5@6.0.3': {}
'@types/q@1.5.8': {}
@@ -9198,7 +9405,7 @@ snapshots:
dependencies:
'@types/node': 22.4.1
- '@types/retry@0.12.0': {}
+ '@types/retry@0.12.2': {}
'@types/send@0.17.4':
dependencies:
@@ -9249,6 +9456,16 @@ snapshots:
transitivePeerDependencies:
- debug
+ '@types/webpack-node-externals@3.0.4(webpack-cli@5.1.4(webpack-dev-server@5.1.0)(webpack@5.94.0))':
+ dependencies:
+ '@types/node': 22.4.1
+ webpack: 5.94.0(webpack-cli@5.1.4)
+ transitivePeerDependencies:
+ - '@swc/core'
+ - esbuild
+ - uglify-js
+ - webpack-cli
+
'@types/webpack-sources@3.2.3':
dependencies:
'@types/node': 22.4.1
@@ -10061,22 +10278,22 @@ snapshots:
'@webassemblyjs/wast-parser': 1.9.0
'@xtuc/long': 4.2.2
- '@webpack-cli/configtest@2.1.1(webpack-cli@5.1.4(webpack-dev-server@4.15.2)(webpack@5.94.0))(webpack@5.94.0(webpack-cli@5.1.4))':
+ '@webpack-cli/configtest@2.1.1(webpack-cli@5.1.4(webpack-dev-server@5.1.0)(webpack@5.94.0))(webpack@5.94.0(webpack-cli@5.1.4))':
dependencies:
webpack: 5.94.0(webpack-cli@5.1.4)
- webpack-cli: 5.1.4(webpack-dev-server@4.15.2)(webpack@5.94.0)
+ webpack-cli: 5.1.4(webpack-dev-server@5.1.0)(webpack@5.94.0)
- '@webpack-cli/info@2.0.2(webpack-cli@5.1.4(webpack-dev-server@4.15.2)(webpack@5.94.0))(webpack@5.94.0(webpack-cli@5.1.4))':
+ '@webpack-cli/info@2.0.2(webpack-cli@5.1.4(webpack-dev-server@5.1.0)(webpack@5.94.0))(webpack@5.94.0(webpack-cli@5.1.4))':
dependencies:
webpack: 5.94.0(webpack-cli@5.1.4)
- webpack-cli: 5.1.4(webpack-dev-server@4.15.2)(webpack@5.94.0)
+ webpack-cli: 5.1.4(webpack-dev-server@5.1.0)(webpack@5.94.0)
- '@webpack-cli/serve@2.0.5(webpack-cli@5.1.4(webpack-dev-server@4.15.2)(webpack@5.94.0))(webpack-dev-server@4.15.2(webpack-cli@5.1.4)(webpack@5.94.0))(webpack@5.94.0(webpack-cli@5.1.4))':
+ '@webpack-cli/serve@2.0.5(webpack-cli@5.1.4(webpack-dev-server@5.1.0)(webpack@5.94.0))(webpack-dev-server@5.1.0(webpack-cli@5.1.4)(webpack@5.94.0))(webpack@5.94.0(webpack-cli@5.1.4))':
dependencies:
webpack: 5.94.0(webpack-cli@5.1.4)
- webpack-cli: 5.1.4(webpack-dev-server@4.15.2)(webpack@5.94.0)
+ webpack-cli: 5.1.4(webpack-dev-server@5.1.0)(webpack@5.94.0)
optionalDependencies:
- webpack-dev-server: 4.15.2(webpack-cli@5.1.4)(webpack@5.94.0)
+ webpack-dev-server: 5.1.0(webpack-cli@5.1.4)(webpack@5.94.0)
'@xtuc/ieee754@1.2.0': {}
@@ -10403,6 +10620,13 @@ snapshots:
schema-utils: 4.2.0
webpack: 5.94.0(webpack-cli@5.1.4)
+ babel-loader@9.1.3(@babel/core@7.25.2)(webpack@5.94.0):
+ dependencies:
+ '@babel/core': 7.25.2
+ find-cache-dir: 4.0.0
+ schema-utils: 4.2.0
+ webpack: 5.94.0
+
babel-plugin-dynamic-import-node@2.3.3:
dependencies:
object.assign: 4.1.5
@@ -10683,6 +10907,10 @@ snapshots:
builtin-status-codes@3.0.0: {}
+ bundle-name@4.1.0:
+ dependencies:
+ run-applescript: 7.0.0
+
bundle-require@2.1.8(esbuild@0.14.7):
dependencies:
esbuild: 0.14.7
@@ -11076,6 +11304,16 @@ snapshots:
copy-descriptor@0.1.1: {}
+ copy-webpack-plugin@11.0.0(webpack@5.94.0(webpack-cli@5.1.4)):
+ dependencies:
+ fast-glob: 3.3.2
+ glob-parent: 6.0.2
+ globby: 13.2.2
+ normalize-path: 3.0.0
+ schema-utils: 4.2.0
+ serialize-javascript: 6.0.2
+ webpack: 5.94.0(webpack-cli@5.1.4)
+
copy-webpack-plugin@5.1.2(webpack@4.47.0):
dependencies:
cacache: 12.0.4
@@ -11109,6 +11347,14 @@ snapshots:
js-yaml: 3.14.1
parse-json: 4.0.0
+ cosmiconfig@7.1.0:
+ dependencies:
+ '@types/parse-json': 4.0.2
+ import-fresh: 3.3.0
+ parse-json: 5.2.0
+ path-type: 4.0.0
+ yaml: 1.10.2
+
create-ecdh@4.0.4:
dependencies:
bn.js: 4.12.0
@@ -11423,15 +11669,18 @@ snapshots:
deepmerge@4.3.1: {}
+ default-browser-id@5.0.0: {}
+
+ default-browser@5.2.1:
+ dependencies:
+ bundle-name: 4.1.0
+ default-browser-id: 5.0.0
+
default-gateway@4.2.0:
dependencies:
execa: 1.0.0
ip-regex: 2.1.0
- default-gateway@6.0.3:
- dependencies:
- execa: 5.1.1
-
defaults@1.0.4:
dependencies:
clone: 1.0.4
@@ -11444,7 +11693,7 @@ snapshots:
es-errors: 1.3.0
gopd: 1.0.1
- define-lazy-prop@2.0.0: {}
+ define-lazy-prop@3.0.0: {}
define-properties@1.2.1:
dependencies:
@@ -12411,6 +12660,23 @@ snapshots:
forever-agent@0.6.1: {}
+ fork-ts-checker-webpack-plugin@8.0.0(typescript@5.5.4)(webpack@5.94.0(webpack-cli@5.1.4)):
+ dependencies:
+ '@babel/code-frame': 7.24.7
+ chalk: 4.1.2
+ chokidar: 3.6.0
+ cosmiconfig: 7.1.0
+ deepmerge: 4.3.1
+ fs-extra: 10.1.0
+ memfs: 3.5.3
+ minimatch: 3.1.2
+ node-abort-controller: 3.1.1
+ schema-utils: 3.3.0
+ semver: 7.6.3
+ tapable: 2.2.1
+ typescript: 5.5.4
+ webpack: 5.94.0(webpack-cli@5.1.4)
+
form-data@2.3.3:
dependencies:
asynckit: 0.4.0
@@ -12436,6 +12702,12 @@ snapshots:
inherits: 2.0.4
readable-stream: 2.3.8
+ fs-extra@10.1.0:
+ dependencies:
+ graceful-fs: 4.2.11
+ jsonfile: 6.1.0
+ universalify: 2.0.1
+
fs-extra@7.0.1:
dependencies:
graceful-fs: 4.2.11
@@ -12602,6 +12874,14 @@ snapshots:
merge2: 1.4.1
slash: 3.0.0
+ globby@13.2.2:
+ dependencies:
+ dir-glob: 3.0.1
+ fast-glob: 3.3.2
+ ignore: 5.3.2
+ merge2: 1.4.1
+ slash: 4.0.0
+
globby@6.1.0:
dependencies:
array-union: 1.0.2
@@ -12654,45 +12934,6 @@ snapshots:
graceful-fs@4.2.11: {}
- grapesjs-cli@4.1.3(@types/node@22.4.1)(typescript@5.5.4):
- dependencies:
- '@babel/core': 7.25.2
- '@babel/plugin-transform-runtime': 7.25.4(@babel/core@7.25.2)
- '@babel/preset-env': 7.25.4(@babel/core@7.25.2)
- '@babel/runtime': 7.25.6
- babel-loader: 9.1.3(@babel/core@7.25.2)(webpack@5.94.0(webpack-cli@5.1.4))
- chalk: 4.1.2
- core-js: 3.38.1
- dts-bundle-generator: 8.1.2
- html-webpack-plugin: 5.6.0(webpack@5.94.0(webpack-cli@5.1.4))
- inquirer: 8.2.6
- listr: 0.14.3
- lodash.template: 4.5.0
- rimraf: 4.4.1
- spdx-license-list: 6.9.0
- ts-loader: 9.5.1(typescript@5.5.4)(webpack@5.94.0(webpack-cli@5.1.4))
- ts-node: 10.9.2(@types/node@22.4.1)(typescript@5.5.4)
- webpack: 5.94.0(webpack-cli@5.1.4)
- webpack-cli: 5.1.4(webpack-dev-server@4.15.2)(webpack@5.94.0)
- webpack-dev-server: 4.15.2(webpack-cli@5.1.4)(webpack@5.94.0)
- yargs: 17.7.2
- transitivePeerDependencies:
- - '@rspack/core'
- - '@swc/core'
- - '@swc/wasm'
- - '@types/node'
- - '@webpack-cli/generators'
- - bufferutil
- - debug
- - esbuild
- - supports-color
- - typescript
- - uglify-js
- - utf-8-validate
- - webpack-bundle-analyzer
- - zen-observable
- - zenObservable
-
graphemer@1.4.0: {}
gray-matter@4.0.3:
@@ -13038,6 +13279,8 @@ snapshots:
human-signals@2.1.0: {}
+ hyperdyperid@1.2.0: {}
+
iconv-lite@0.4.24:
dependencies:
safer-buffer: 2.1.2
@@ -13251,7 +13494,7 @@ snapshots:
is-directory@0.3.1: {}
- is-docker@2.2.1: {}
+ is-docker@3.0.0: {}
is-extendable@0.1.1: {}
@@ -13279,6 +13522,10 @@ snapshots:
dependencies:
is-extglob: 2.1.1
+ is-inside-container@1.0.0:
+ dependencies:
+ is-docker: 3.0.0
+
is-installed-globally@0.3.2:
dependencies:
global-dirs: 2.1.0
@@ -13288,6 +13535,8 @@ snapshots:
is-negative-zero@2.0.3: {}
+ is-network-error@1.1.0: {}
+
is-npm@4.0.0: {}
is-number-object@1.0.7:
@@ -13383,9 +13632,9 @@ snapshots:
is-wsl@1.1.0: {}
- is-wsl@2.2.0:
+ is-wsl@3.1.0:
dependencies:
- is-docker: 2.2.1
+ is-inside-container: 1.0.0
is-yarn-global@0.3.0: {}
@@ -13893,6 +14142,12 @@ snapshots:
optionalDependencies:
graceful-fs: 4.2.11
+ jsonfile@6.1.0:
+ dependencies:
+ universalify: 2.0.1
+ optionalDependencies:
+ graceful-fs: 4.2.11
+
jsprim@1.4.2:
dependencies:
assert-plus: 1.0.0
@@ -14305,6 +14560,13 @@ snapshots:
dependencies:
fs-monkey: 1.0.6
+ memfs@4.11.1:
+ dependencies:
+ '@jsonjoy.com/json-pack': 1.1.0(tslib@2.7.0)
+ '@jsonjoy.com/util': 1.3.0(tslib@2.7.0)
+ tree-dump: 1.0.2(tslib@2.7.0)
+ tslib: 2.7.0
+
memory-fs@0.4.1:
dependencies:
errno: 0.1.8
@@ -14701,6 +14963,8 @@ snapshots:
lower-case: 2.0.2
tslib: 2.7.0
+ node-abort-controller@3.1.1: {}
+
node-forge@0.10.0: {}
node-forge@1.3.1: {}
@@ -14890,11 +15154,12 @@ snapshots:
dependencies:
mimic-fn: 2.1.0
- open@8.4.2:
+ open@10.1.0:
dependencies:
- define-lazy-prop: 2.0.0
- is-docker: 2.2.1
- is-wsl: 2.2.0
+ default-browser: 5.2.1
+ define-lazy-prop: 3.0.0
+ is-inside-container: 1.0.0
+ is-wsl: 3.1.0
opencollective-postinstall@2.0.3: {}
@@ -14971,9 +15236,10 @@ snapshots:
dependencies:
retry: 0.12.0
- p-retry@4.6.2:
+ p-retry@6.2.0:
dependencies:
- '@types/retry': 0.12.0
+ '@types/retry': 0.12.2
+ is-network-error: 1.1.0
retry: 0.13.1
p-try@2.2.0: {}
@@ -15828,6 +16094,8 @@ snapshots:
rrweb-cssom@0.7.1: {}
+ run-applescript@7.0.0: {}
+
run-async@2.4.1: {}
run-parallel@1.2.0:
@@ -16056,6 +16324,8 @@ snapshots:
slash@3.0.0: {}
+ slash@4.0.0: {}
+
slice-ansi@0.0.4: {}
smoothscroll-polyfill@0.4.4: {}
@@ -16484,6 +16754,10 @@ snapshots:
text-table@0.2.0: {}
+ thingies@1.21.0(tslib@2.7.0):
+ dependencies:
+ tslib: 2.7.0
+
through2@2.0.5:
dependencies:
readable-stream: 2.3.8
@@ -16559,6 +16833,10 @@ snapshots:
dependencies:
punycode: 2.3.1
+ tree-dump@1.0.2(tslib@2.7.0):
+ dependencies:
+ tslib: 2.7.0
+
trim-lines@3.0.1: {}
trough@2.2.0: {}
@@ -16586,16 +16864,6 @@ snapshots:
'@jest/types': 29.6.3
babel-jest: 29.7.0(@babel/core@7.25.2)
- ts-loader@9.5.1(typescript@5.5.4)(webpack@5.94.0(webpack-cli@5.1.4)):
- dependencies:
- chalk: 4.1.2
- enhanced-resolve: 5.17.1
- micromatch: 4.0.8
- semver: 7.6.3
- source-map: 0.7.4
- typescript: 5.5.4
- webpack: 5.94.0(webpack-cli@5.1.4)
-
ts-loader@9.5.1(typescript@5.5.4)(webpack@5.94.0):
dependencies:
chalk: 4.1.2
@@ -16799,6 +17067,8 @@ snapshots:
universalify@0.2.0: {}
+ universalify@2.0.1: {}
+
unpipe@1.0.0: {}
unquote@1.1.1: {}
@@ -17216,12 +17486,12 @@ snapshots:
deepmerge: 1.5.2
javascript-stringify: 2.1.0
- webpack-cli@5.1.4(webpack-dev-server@4.15.2)(webpack@5.94.0):
+ webpack-cli@5.1.4(webpack-dev-server@5.1.0)(webpack@5.94.0):
dependencies:
'@discoveryjs/json-ext': 0.5.7
- '@webpack-cli/configtest': 2.1.1(webpack-cli@5.1.4(webpack-dev-server@4.15.2)(webpack@5.94.0))(webpack@5.94.0(webpack-cli@5.1.4))
- '@webpack-cli/info': 2.0.2(webpack-cli@5.1.4(webpack-dev-server@4.15.2)(webpack@5.94.0))(webpack@5.94.0(webpack-cli@5.1.4))
- '@webpack-cli/serve': 2.0.5(webpack-cli@5.1.4(webpack-dev-server@4.15.2)(webpack@5.94.0))(webpack-dev-server@4.15.2(webpack-cli@5.1.4)(webpack@5.94.0))(webpack@5.94.0(webpack-cli@5.1.4))
+ '@webpack-cli/configtest': 2.1.1(webpack-cli@5.1.4(webpack-dev-server@5.1.0)(webpack@5.94.0))(webpack@5.94.0(webpack-cli@5.1.4))
+ '@webpack-cli/info': 2.0.2(webpack-cli@5.1.4(webpack-dev-server@5.1.0)(webpack@5.94.0))(webpack@5.94.0(webpack-cli@5.1.4))
+ '@webpack-cli/serve': 2.0.5(webpack-cli@5.1.4(webpack-dev-server@5.1.0)(webpack@5.94.0))(webpack-dev-server@5.1.0(webpack-cli@5.1.4)(webpack@5.94.0))(webpack@5.94.0(webpack-cli@5.1.4))
colorette: 2.0.20
commander: 10.0.1
cross-spawn: 7.0.3
@@ -17233,7 +17503,7 @@ snapshots:
webpack: 5.94.0(webpack-cli@5.1.4)
webpack-merge: 5.10.0
optionalDependencies:
- webpack-dev-server: 4.15.2(webpack-cli@5.1.4)(webpack@5.94.0)
+ webpack-dev-server: 5.1.0(webpack-cli@5.1.4)(webpack@5.94.0)
webpack-dev-middleware@3.7.3(webpack@4.47.0):
dependencies:
@@ -17244,13 +17514,15 @@ snapshots:
webpack: 4.47.0
webpack-log: 2.0.0
- webpack-dev-middleware@5.3.4(webpack@5.94.0(webpack-cli@5.1.4)):
+ webpack-dev-middleware@7.4.2(webpack@5.94.0(webpack-cli@5.1.4)):
dependencies:
colorette: 2.0.20
- memfs: 3.5.3
+ memfs: 4.11.1
mime-types: 2.1.35
+ on-finished: 2.4.1
range-parser: 1.2.1
schema-utils: 4.2.0
+ optionalDependencies:
webpack: 5.94.0(webpack-cli@5.1.4)
webpack-dev-server@3.11.3(webpack@4.47.0):
@@ -17293,7 +17565,7 @@ snapshots:
- bufferutil
- utf-8-validate
- webpack-dev-server@4.15.2(webpack-cli@5.1.4)(webpack@5.94.0):
+ webpack-dev-server@5.1.0(webpack-cli@5.1.4)(webpack@5.94.0):
dependencies:
'@types/bonjour': 3.5.13
'@types/connect-history-api-fallback': 1.5.4
@@ -17308,26 +17580,24 @@ snapshots:
colorette: 2.0.20
compression: 1.7.4(supports-color@6.1.0)
connect-history-api-fallback: 2.0.0
- default-gateway: 6.0.3
express: 4.19.2(supports-color@6.1.0)
graceful-fs: 4.2.11
html-entities: 2.5.2
http-proxy-middleware: 2.0.6(@types/express@4.17.21)
ipaddr.js: 2.2.0
launch-editor: 2.9.1
- open: 8.4.2
- p-retry: 4.6.2
- rimraf: 3.0.2
+ open: 10.1.0
+ p-retry: 6.2.0
schema-utils: 4.2.0
selfsigned: 2.4.1
serve-index: 1.9.1(supports-color@6.1.0)
sockjs: 0.3.24
spdy: 4.0.2(supports-color@6.1.0)
- webpack-dev-middleware: 5.3.4(webpack@5.94.0(webpack-cli@5.1.4))
+ webpack-dev-middleware: 7.4.2(webpack@5.94.0(webpack-cli@5.1.4))
ws: 8.18.0
optionalDependencies:
webpack: 5.94.0(webpack-cli@5.1.4)
- webpack-cli: 5.1.4(webpack-dev-server@4.15.2)(webpack@5.94.0)
+ webpack-cli: 5.1.4(webpack-dev-server@5.1.0)(webpack@5.94.0)
transitivePeerDependencies:
- bufferutil
- debug
@@ -17349,6 +17619,8 @@ snapshots:
flat: 5.0.2
wildcard: 2.0.1
+ webpack-node-externals@3.0.0: {}
+
webpack-sources@1.4.3:
dependencies:
source-list-map: 2.0.1
@@ -17440,7 +17712,7 @@ snapshots:
watchpack: 2.4.2
webpack-sources: 3.2.3
optionalDependencies:
- webpack-cli: 5.1.4(webpack-dev-server@4.15.2)(webpack@5.94.0)
+ webpack-cli: 5.1.4(webpack-dev-server@5.1.0)(webpack@5.94.0)
transitivePeerDependencies:
- '@swc/core'
- esbuild
@@ -17593,6 +17865,8 @@ snapshots:
yallist@4.0.0: {}
+ yaml@1.10.2: {}
+
yargs-parser@13.1.2:
dependencies:
camelcase: 5.3.1
diff --git a/pnpm-workspace.yaml b/pnpm-workspace.yaml
index 81efe021e1..335a211285 100644
--- a/pnpm-workspace.yaml
+++ b/pnpm-workspace.yaml
@@ -1,3 +1,4 @@
packages:
- - 'packages/**'
- - 'docs/**'
+ - 'packages/cli'
+ - 'packages/core'
+ - 'docs/'