diff --git a/README.md b/README.md
index 2cbad39..b3bcdb3 100644
--- a/README.md
+++ b/README.md
@@ -1,196 +1,75 @@
-# authing-go-sdk
+# SDK for Go
-[Authing](https://authing.cn) 身份云 `Go` 语言客户端,包含 [Authing Open API](https://api.authing.cn/openapi/) 所有 Management API 的请求方法。
+

+
-此模块一般用于后端服务器环境,以管理员(Administrator)的身份进行请求,用于管理 Authing 用户、角色、分组、组织机构等资源;一般来说,你在 Authing 控制台中能做的所有操作,都能用此模块完成。
+Authing Go SDK 目前支持 Golang 1.8+ 版本。
-如果你需要以终端用户(End User)的身份进行登录、注册、登出等操作,请使用 [Guard](https://www.authing.cn/learn/guard) .
-
-## 安装
+Authing Golang SDK 由两部分组成:`ManagementClient` 和 `AuthenticationClient`。
+`AuthenticationClient` 以终端用户(End User)的身份进行请求,提供了登录、注册、登出、管理用户资料、获取授权资源等所有管理用户身份的方法;此模块还提供了各种身份协议的 SDK,如 [OpenID Connect](/guides/federation/oidc.md), [OAuth 2.0](/guides/federation/oauth.md), [SAML](/guides/federation/saml.md) 和 [CAS](/guides/federation/cas.md)。此模块适合用于后端交互的服务器环境。
+`ManagementClient` 以管理员(Administrator)的身份进行请求,用于管理用户池资源和执行管理任务,提供了管理用户、角色、应用、资源等方法;一般来说,你在 [Authing 控制台](https://console.authing.cn/console/userpool) 中能做的所有操作,都能用此模块完成。此模块适合在后端环境下使用。
-```shell
-go get -u github.com/Authing/authing-go-sdk
-```
-
-## 初始化
+## GitHub 下载地址
-初始化 `ManagementClient` 需要使用 `accessKeyId` 和 `accessKeySecret` 参数:
+| 条目 | 说明 |
+| -------- | ------------------------------------------- |
+| 支持版本 | Golang 1.8 + |
+| 仓库地址 | [https://github.com/Authing/authing-go-sdk](https://github.com/Authing/authing-go-sdk) |
-```go
-import (
- "authing-go-sdk/client"
-)
+## 安装
-options := client.ManagementClientOptions{
- AccessKeyId: "AUTHING_USERPOOL_ID",
- AccessKeySecret: "AUTHING_USERPOOL_SECRET",
-}
-client, err := client.NewClient(&options)
-if err != nil {
- // The exception needs to be handled by the developer.
-}
+安装 golang sdk 库,请运行:
```
+go get github.com/Authing/authing-go-sdk
+```
-`ManagementClient` 会自动从 Authing 服务器获取 Management API Token,并通过返回的 Token 过期时间自动对 Token 进行缓存。
-
-完整的参数和释义如下:
-
-- `AccessKeyId`: Authing 用户池 ID;
-- `AccessKeySecret`: Authing 用户池密钥;
-- `Timeout`: 超时时间,单位为 ms,默认为 10000 ms;
-- `Host`: Authing 服务器地址,默认为 `https://api.authing.cn`。如果你使用的是 Authing 公有云版本,请忽略此参数。如果你使用的是私有化部署的版本,此参数必填,格式如下: https://authing-api.my-authing-service.com(最后不带斜杠 /)。
-- `Lang`: 接口 Message 返回语言格式(可选),可选值为 zh-CN 和 en-US,默认为 zh-CN。
-## 快速开始
+## 使用管理模块
-初始化完成 `ManagementClient` 之后,你可以获取 `ManagementClient` 的实例,然后调用此实例上的方法。例如:
+初始化 `ManagementClient` 需要 `userPoolId`(用户池 ID) 和 `secret`(用户池密钥):
-- 获取用户列表
+> 你可以在此[了解如何获取 UserPoolId 和 Secret](/guides/faqs/get-userpool-id-and-secret.md) .
```go
-package main
-
-import (
- "authing-go-sdk/client"
- "authing-go-sdk/dto"
- "fmt"
-)
-
func main() {
- options := client.ManagementClientOptions{
- AccessKeyId: "AUTHING_USERPOOL_ID",
- AccessKeySecret: "AUTHING_USERPOOL_SECRET",
- }
- client, err := client.NewClient(&options)
- request := dto.ListUsersDto{
- Page: 1,
- Limit: 10,
- }
- response := client.listUsers(request)
- fmt.Println(response)
+ client := management.NewClient(userPoolId, secret)
}
```
-- 创建角色
+现在 `managementClient` 实例就可以使用了。例如可以导出所有组织机构数据:
```go
-package main
-
-import (
- "authing-go-sdk/client"
- "authing-go-sdk/dto"
- "fmt"
-)
-
func main() {
- options := client.ManagementClientOptions{
- AccessKeyId: "AUTHING_USERPOOL_ID",
- AccessKeySecret: "AUTHING_USERPOOL_SECRET",
- }
- client, err := client.NewClient(&options)
- request := dto.CreateRoleDto{
- Code: "code",
- Namespace: "namespace",
- Description: "description",
- }
- response := client.createRole(request)
- fmt.Println(response)
+ client := management.NewClient(userPoolId, secret)
+ resp, err := client.ExportAll()
}
```
-完整的接口列表,你可以在 [Authing Open API](https://api.authing.cn/openapi/) 和 [SDK 文档](https://authing-open-api.readme.io/reference/go) 中获取。
-## 错误处理
-
-`ManagementClient` 中的每个方法,遵循统一的返回结构:
-
-- `StatusCode`: 请求是否成功状态码,当 `StatusCode` 为 200 时,表示操作成功,非 200 全部为失败。
-- `ApiCode`: 细分错误码,当 `ApiCode` 非 200 时,可通过此错误码得到具体的错误类型。
-- `Message`: 具体的错误信息。
-- `Data`: 具体返回的接口数据。
-
-一般情况下,如果你只需要判断操作是否成功,只需要对比一下 `Code` 是否为 200。如果非 200,可以在代码中通抛出异常或者任何你项目中使用的异常处理方式。
-
-```go
-package main
-
-import (
- "authing-go-sdk/client"
- "authing-go-sdk/dto"
- "fmt"
-)
-
-func main() {
- options := client.ManagementClientOptions{
- AccessKeyId: "AUTHING_USERPOOL_ID",
- AccessKeySecret: "AUTHING_USERPOOL_SECRET",
- }
- client, err := client.NewClient(&options)
- request := dto.CreateRoleDto{
- Code: "code",
- Namespace: "namespace",
- Description: "description",
- }
- response := client.createRole(request)
- fmt.Println(response)
-
- if response.Code != 200 {
- // 自定义错误处理逻辑
- }
-}
-```
## 私有化部署
-如果你使用的是私有化部署的 Authing IDaaS 服务,需要指定此 Authing 私有化实例的 `host`,如:
-
-```go
-package main
+**私有化部署**场景需要指定你私有化的 Authing 服务的 GraphQL 端点(**不带协议头和 Path**)以及密码加密公钥,如果你不清楚可以联系 Authing IDaaS 服务管理员。
-import (
- "authing-go-sdk/client"
- "authing-go-sdk/dto"
- "fmt"
-)
+如:
+```go
func main() {
- options := client.ManagementClientOptions{
- AccessKeyId: "AUTHING_USERPOOL_ID",
- AccessKeySecret: "AUTHING_USERPOOL_SECRET",
- Host: "YOUR_HOST", // 您的 Authing 私有化实例 HOST 地址,格式例如 https://core.authing.cn
- }
- client, err := client.NewClient(&options)
- if err != nil {
- // The exception needs to be handled by the developer.
- }
-
- request := dto.CreateRoleDto{
- Code: "code",
- Namespace: "namespace",
- Description: "description",
- }
- response := client.createRole(request)
- fmt.Println(response)
-
- if response.Code != 200 {
- // 自定义错误处理逻辑
- }
+ // 增加参数配置自定义域名
+ client := management.NewClient(userPoolId, secret, host)
}
```
-如果你不清楚如何获取,可以联系 Authing IDaaS 服务管理员。
-
-## 资源
-
-- [官网](https://authing.cn)
-- [开发者文档](https://docs.authing.cn/)
-- [Authing Open API](https://api.authing.cn/openapi/)
-- [SDK 文档](https://authing-open-api.readme.io/reference/nodejs)
-- [论坛社区](https://forum.authing.cn/)
+## 参与贡献
+- Fork it
+- Create your feature branch (git checkout -b my-new-feature)
+- Commit your changes (git commit -am 'Add some feature')
+- Push to the branch (git push origin my-new-feature)
+- Create new Pull Request
## 获取帮助
-有任何疑问,可以在 Authing 论坛提出: [#authing-forum](https://forum.authing.cn/)
+Join us on forum: [#authing-chat](https://forum.authing.cn/)
diff --git a/authing-golang-sdk b/authing-golang-sdk
new file mode 100755
index 0000000..025daeb
Binary files /dev/null and b/authing-golang-sdk differ
diff --git a/client/management_client.go b/client/management_client.go
deleted file mode 100644
index 7e16155..0000000
--- a/client/management_client.go
+++ /dev/null
@@ -1,1568 +0,0 @@
-package client
-
-import (
- "authing-go-sdk/dto"
- "encoding/json"
- "github.com/valyala/fasthttp"
-)
-
-/*
- * @summary 获取 Management API Token
- * @description 获取 Management API Token
- * @param requestBody
- * @returns GetManagementTokenRespDto
- */
-func (c *Client) GetManagementToken(reqDto *dto.GetManagementAccessTokenDto) *dto.GetManagementTokenRespDto {
- b, err := c.SendHttpRequest("/api/v3/get-management-token", fasthttp.MethodPost, reqDto)
- var response dto.GetManagementTokenRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 获取用户信息
- * @description 通过 id、username、email、phone、email、externalId 获取用户详情
- * @param userId 用户 ID
- * @param withCustomData 是否获取自定义数据
- * @param withIdentities 是否获取 identities
- * @param withDepartmentIds 是否获取部门 ID 列表
- * @param phone 手机号
- * @param email 邮箱
- * @param username 用户名
- * @param externalId 原系统 ID
- * @returns UserSingleRespDto
- */
-func (c *Client) GetUser(reqDto *dto.GetUserDto) *dto.UserSingleRespDto {
- b, err := c.SendHttpRequest("/api/v3/get-user", fasthttp.MethodGet, reqDto)
- var response dto.UserSingleRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 批量获取用户信息
- * @description 根据用户 id 批量获取用户信息
- * @param userIds 用户 ID 数组
- * @param withCustomData 是否获取自定义数据
- * @param withIdentities 是否获取 identities
- * @param withDepartmentIds 是否获取部门 ID 列表
- * @returns UserListRespDto
- */
-func (c *Client) GetUserBatch(reqDto *dto.GetUserBatchDto) *dto.UserListRespDto {
- b, err := c.SendHttpRequest("/api/v3/get-user-batch", fasthttp.MethodGet, reqDto)
- var response dto.UserListRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 获取用户列表
- * @description 获取用户列表接口,支持分页
- * @param page 当前页数,从 1 开始
- * @param limit 每页数目,最大不能超过 50,默认为 10
- * @param withCustomData 是否获取自定义数据
- * @param withIdentities 是否获取 identities
- * @param withDepartmentIds 是否获取部门 ID 列表
- * @returns UserPaginatedRespDto
- */
-func (c *Client) ListUsers(reqDto *dto.ListUsersDto) *dto.UserPaginatedRespDto {
- b, err := c.SendHttpRequest("/api/v3/list-users", fasthttp.MethodGet, reqDto)
- var response dto.UserPaginatedRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 获取用户的外部身份源
- * @description 获取用户的外部身份源
- * @param userId 用户 ID
- * @returns IdentityListRespDto
- */
-func (c *Client) GetUserIdentities(reqDto *dto.GetUserIdentitiesDto) *dto.IdentityListRespDto {
- b, err := c.SendHttpRequest("/api/v3/get-user-identities", fasthttp.MethodGet, reqDto)
- var response dto.IdentityListRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 获取用户角色列表
- * @description 获取用户角色列表
- * @param userId 用户 ID
- * @param namespace 所属权限分组的 code
- * @returns RolePaginatedRespDto
- */
-func (c *Client) GetUserRoles(reqDto *dto.GetUserRolesDto) *dto.RolePaginatedRespDto {
- b, err := c.SendHttpRequest("/api/v3/get-user-roles", fasthttp.MethodGet, reqDto)
- var response dto.RolePaginatedRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 获取用户实名认证信息
- * @description 获取用户实名认证信息
- * @param userId 用户 ID
- * @returns PrincipalAuthenticationInfoPaginatedRespDto
- */
-func (c *Client) GetPrincipalAuthenticationInfo(reqDto *dto.GetUserPrincipalAuthenticationInfoDto) *dto.PrincipalAuthenticationInfoPaginatedRespDto {
- b, err := c.SendHttpRequest("/api/v3/get-user-principal-authentication-info", fasthttp.MethodGet, reqDto)
- var response dto.PrincipalAuthenticationInfoPaginatedRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 删除用户实名认证信息
- * @description 删除用户实名认证信息
- * @param requestBody
- * @returns IsSuccessRespDto
- */
-func (c *Client) ResetPrincipalAuthenticationInfo(reqDto *dto.ResetUserPrincipalAuthenticationInfoDto) *dto.IsSuccessRespDto {
- b, err := c.SendHttpRequest("/api/v3/reset-user-principal-authentication-info", fasthttp.MethodPost, reqDto)
- var response dto.IsSuccessRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 获取用户部门列表
- * @description 获取用户部门列表
- * @param userId 用户 ID
- * @returns UserDepartmentPaginatedRespDto
- */
-func (c *Client) GetUserDepartments(reqDto *dto.GetUserDepartmentsDto) *dto.UserDepartmentPaginatedRespDto {
- b, err := c.SendHttpRequest("/api/v3/get-user-departments", fasthttp.MethodGet, reqDto)
- var response dto.UserDepartmentPaginatedRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 设置用户所在部门
- * @description 设置用户所在部门
- * @param requestBody
- * @returns IsSuccessRespDto
- */
-func (c *Client) SetUserDepartment(reqDto *dto.SetUserDepartmentsDto) *dto.IsSuccessRespDto {
- b, err := c.SendHttpRequest("/api/v3/set-user-departments", fasthttp.MethodPost, reqDto)
- var response dto.IsSuccessRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 获取用户分组列表
- * @description 获取用户分组列表
- * @param userId 用户 ID
- * @returns GroupPaginatedRespDto
- */
-func (c *Client) GetUserGroups(reqDto *dto.GetUserGroupsDto) *dto.GroupPaginatedRespDto {
- b, err := c.SendHttpRequest("/api/v3/get-user-groups", fasthttp.MethodGet, reqDto)
- var response dto.GroupPaginatedRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 删除用户
- * @description 删除用户(支持批量删除)
- * @param requestBody
- * @returns IsSuccessRespDto
- */
-func (c *Client) DeleteUserBatch(reqDto *dto.DeleteUsersBatchDto) *dto.IsSuccessRespDto {
- b, err := c.SendHttpRequest("/api/v3/delete-users-batch", fasthttp.MethodPost, reqDto)
- var response dto.IsSuccessRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 获取用户 MFA 绑定信息
- * @description 获取用户 MFA 绑定信息
- * @param userId 用户 ID
- * @returns UserMfaSingleRespDto
- */
-func (c *Client) GetUserMfaInfo(reqDto *dto.GetUserMfaInfoDto) *dto.UserMfaSingleRespDto {
- b, err := c.SendHttpRequest("/api/v3/get-user-mfa-info", fasthttp.MethodGet, reqDto)
- var response dto.UserMfaSingleRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 获取已归档的用户列表
- * @description 获取已归档的用户列表
- * @param page 当前页数,从 1 开始
- * @param limit 每页数目,最大不能超过 50,默认为 10
- * @returns ListArchivedUsersSingleRespDto
- */
-func (c *Client) ListArchivedUsers(reqDto *dto.ListArchivedUsersDto) *dto.ListArchivedUsersSingleRespDto {
- b, err := c.SendHttpRequest("/api/v3/list-archived-users", fasthttp.MethodGet, reqDto)
- var response dto.ListArchivedUsersSingleRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 强制下线用户
- * @description 强制下线用户
- * @param requestBody
- * @returns IsSuccessRespDto
- */
-func (c *Client) KickUsers(reqDto *dto.KickUsersDto) *dto.IsSuccessRespDto {
- b, err := c.SendHttpRequest("/api/v3/kick-users", fasthttp.MethodPost, reqDto)
- var response dto.IsSuccessRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 判断用户是否存在
- * @description 根据条件判断用户是否存在
- * @param requestBody
- * @returns IsUserExistsRespDto
- */
-func (c *Client) IsUserExists(reqDto *dto.IsUserExistsReqDto) *dto.IsUserExistsRespDto {
- b, err := c.SendHttpRequest("/api/v3/is-user-exists", fasthttp.MethodPost, reqDto)
- var response dto.IsUserExistsRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 创建用户
- * @description 创建用户,邮箱、手机号、用户名必须包含其中一个
- * @param requestBody
- * @returns UserSingleRespDto
- */
-func (c *Client) CreateUser(reqDto *dto.CreateUserReqDto) *dto.UserSingleRespDto {
- b, err := c.SendHttpRequest("/api/v3/create-user", fasthttp.MethodPost, reqDto)
- var response dto.UserSingleRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 批量创建用户
- * @description 此接口将以管理员身份批量创建用户,不需要进行手机号验证码检验等安全检测。用户的手机号、邮箱、用户名、externalId 用户池内唯一。
- * @param requestBody
- * @returns UserListRespDto
- */
-func (c *Client) CreateUserBatch(reqDto *dto.CreateUserBatchReqDto) *dto.UserListRespDto {
- b, err := c.SendHttpRequest("/api/v3/create-users-batch", fasthttp.MethodPost, reqDto)
- var response dto.UserListRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 修改用户资料
- * @description 修改用户资料
- * @param requestBody
- * @returns UserSingleRespDto
- */
-func (c *Client) UpdateUser(reqDto *dto.UpdateUserReqDto) *dto.UserSingleRespDto {
- b, err := c.SendHttpRequest("/api/v3/update-user", fasthttp.MethodPost, reqDto)
- var response dto.UserSingleRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 获取用户可访问应用
- * @description 获取用户可访问应用
- * @param userId 用户 ID
- * @returns AppListRespDto
- */
-func (c *Client) GetUserAccessibleApps(reqDto *dto.GetUserAccessibleAppsDto) *dto.AppListRespDto {
- b, err := c.SendHttpRequest("/api/v3/get-user-accessible-apps", fasthttp.MethodGet, reqDto)
- var response dto.AppListRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 获取用户授权的应用
- * @description 获取用户授权的应用
- * @param userId 用户 ID
- * @returns AppListRespDto
- */
-func (c *Client) GetUserAuthorizedApps(reqDto *dto.GetUserAuthorizedAppsDto) *dto.AppListRespDto {
- b, err := c.SendHttpRequest("/api/v3/get-user-authorized-apps", fasthttp.MethodGet, reqDto)
- var response dto.AppListRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 判断用户是否有某个角色
- * @description 判断用户是否有某个角色,支持同时传入多个角色进行判断
- * @param requestBody
- * @returns HasAnyRoleRespDto
- */
-func (c *Client) HasAnyRole(reqDto *dto.HasAnyRoleReqDto) *dto.HasAnyRoleRespDto {
- b, err := c.SendHttpRequest("/api/v3/has-any-role", fasthttp.MethodPost, reqDto)
- var response dto.HasAnyRoleRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 获取用户的登录历史记录
- * @description 获取用户登录历史记录
- * @param userId 用户 ID
- * @param appId 应用 ID
- * @param clientIp 客户端 IP
- * @param start 开始时间戳(毫秒)
- * @param end 结束时间戳(毫秒)
- * @param page 当前页数,从 1 开始
- * @param limit 每页数目,最大不能超过 50,默认为 10
- * @returns UserLoginHistoryPaginatedRespDto
- */
-func (c *Client) GetUserLoginHistory(reqDto *dto.GetUserLoginHistoryDto) *dto.UserLoginHistoryPaginatedRespDto {
- b, err := c.SendHttpRequest("/api/v3/get-user-login-history", fasthttp.MethodGet, reqDto)
- var response dto.UserLoginHistoryPaginatedRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 获取用户曾经登录过的应用
- * @description 获取用户曾经登录过的应用
- * @param userId 用户 ID
- * @returns UserLoggedInAppsListRespDto
- */
-func (c *Client) GetUserLoggedInApps(reqDto *dto.GetUserLoggedinAppsDto) *dto.UserLoggedInAppsListRespDto {
- b, err := c.SendHttpRequest("/api/v3/get-user-loggedin-apps", fasthttp.MethodGet, reqDto)
- var response dto.UserLoggedInAppsListRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 获取用户被授权的所有资源
- * @description 获取用户被授权的所有资源,用户被授权的资源是用户自身被授予、通过分组继承、通过角色继承、通过组织机构继承的集合
- * @param userId 用户 ID
- * @param namespace 所属权限分组的 code
- * @param resourceType 资源类型
- * @returns AuthorizedResourcePaginatedRespDto
- */
-func (c *Client) GetUserAuthorizedResources(reqDto *dto.GetUserAuthorizedResourcesDto) *dto.AuthorizedResourcePaginatedRespDto {
- b, err := c.SendHttpRequest("/api/v3/get-user-authorized-resources", fasthttp.MethodGet, reqDto)
- var response dto.AuthorizedResourcePaginatedRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 获取分组详情
- * @description 获取分组详情,通过 code 唯一标志用户池中的一个分组
- * @param code 分组 code
- * @returns GroupSingleRespDto
- */
-func (c *Client) GetGroup(reqDto *dto.GetGroupDto) *dto.GroupSingleRespDto {
- b, err := c.SendHttpRequest("/api/v3/get-group", fasthttp.MethodGet, reqDto)
- var response dto.GroupSingleRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 获取分组列表
- * @description 获取分组列表接口,支持分页
- * @param page 当前页数,从 1 开始
- * @param limit 每页数目,最大不能超过 50,默认为 10
- * @returns GroupPaginatedRespDto
- */
-func (c *Client) GetGroupList(reqDto *dto.ListGroupsDto) *dto.GroupPaginatedRespDto {
- b, err := c.SendHttpRequest("/api/v3/list-groups", fasthttp.MethodGet, reqDto)
- var response dto.GroupPaginatedRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 创建分组
- * @description 创建分组,一个分组必须包含一个用户池全局唯一的标志符(code),此标志符必须为一个合法的英文标志符,如 developers;以及分组名称
- * @param requestBody
- * @returns GroupSingleRespDto
- */
-func (c *Client) CreateGroup(reqDto *dto.CreateGroupReqDto) *dto.GroupSingleRespDto {
- b, err := c.SendHttpRequest("/api/v3/create-group", fasthttp.MethodPost, reqDto)
- var response dto.GroupSingleRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 批量创建分组
- * @description 批量创建分组
- * @param requestBody
- * @returns GroupListRespDto
- */
-func (c *Client) CreateGroupBatch(reqDto *dto.CreateGroupBatchReqDto) *dto.GroupListRespDto {
- b, err := c.SendHttpRequest("/api/v3/create-groups-batch", fasthttp.MethodPost, reqDto)
- var response dto.GroupListRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 修改分组
- * @description 修改分组,通过 code 唯一标志用户池中的一个分组。你可以修改此分组的 code
- * @param requestBody
- * @returns GroupSingleRespDto
- */
-func (c *Client) UpdateGroup(reqDto *dto.UpdateGroupReqDto) *dto.GroupSingleRespDto {
- b, err := c.SendHttpRequest("/api/v3/update-group", fasthttp.MethodPost, reqDto)
- var response dto.GroupSingleRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 批量删除分组
- * @description 批量删除分组
- * @param requestBody
- * @returns IsSuccessRespDto
- */
-func (c *Client) DeleteGroups(reqDto *dto.DeleteGroupsReqDto) *dto.IsSuccessRespDto {
- b, err := c.SendHttpRequest("/api/v3/delete-groups-batch", fasthttp.MethodPost, reqDto)
- var response dto.IsSuccessRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 添加分组成员
- * @description 添加分组成员
- * @param requestBody
- * @returns IsSuccessRespDto
- */
-func (c *Client) AddGroupMembers(reqDto *dto.AddGroupMembersReqDto) *dto.IsSuccessRespDto {
- b, err := c.SendHttpRequest("/api/v3/add-group-members", fasthttp.MethodPost, reqDto)
- var response dto.IsSuccessRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 批量移除分组成员
- * @description 批量移除分组成员
- * @param requestBody
- * @returns IsSuccessRespDto
- */
-func (c *Client) RemoveGroupMembers(reqDto *dto.RemoveGroupMembersReqDto) *dto.IsSuccessRespDto {
- b, err := c.SendHttpRequest("/api/v3/remove-group-members", fasthttp.MethodPost, reqDto)
- var response dto.IsSuccessRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 获取分组成员列表
- * @description 获取分组成员列表
- * @param code 分组 code
- * @param page 当前页数,从 1 开始
- * @param limit 每页数目,最大不能超过 50,默认为 10
- * @param withCustomData 是否获取自定义数据
- * @param withIdentities 是否获取 identities
- * @param withDepartmentIds 是否获取部门 ID 列表
- * @returns UserPaginatedRespDto
- */
-func (c *Client) ListGroupMembers(reqDto *dto.ListGroupMembersDto) *dto.UserPaginatedRespDto {
- b, err := c.SendHttpRequest("/api/v3/list-group-members", fasthttp.MethodGet, reqDto)
- var response dto.UserPaginatedRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 获取分组被授权的资源列表
- * @description 获取分组被授权的资源列表
- * @param code 分组 code
- * @param namespace 所属权限分组的 code
- * @param resourceType 资源类型
- * @returns AuthorizedResourceListRespDto
- */
-func (c *Client) GetGroupAuthorizedResources(reqDto *dto.GetGroupAuthorizedResourcesDto) *dto.AuthorizedResourceListRespDto {
- b, err := c.SendHttpRequest("/api/v3/get-group-authorized-resources", fasthttp.MethodGet, reqDto)
- var response dto.AuthorizedResourceListRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 获取角色详情
- * @description 获取角色详情
- * @param code 权限分组内角色的唯一标识符
- * @param namespace 所属权限分组的 code
- * @returns RoleSingleRespDto
- */
-func (c *Client) GetRole(reqDto *dto.GetRoleDto) *dto.RoleSingleRespDto {
- b, err := c.SendHttpRequest("/api/v3/get-role", fasthttp.MethodGet, reqDto)
- var response dto.RoleSingleRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 分配角色
- * @description 分配角色,被分配者可以是用户,可以是部门
- * @param requestBody
- * @returns IsSuccessRespDto
- */
-func (c *Client) AssignRole(reqDto *dto.AssignRoleDto) *dto.IsSuccessRespDto {
- b, err := c.SendHttpRequest("/api/v3/assign-role", fasthttp.MethodPost, reqDto)
- var response dto.IsSuccessRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 批量分配角色
- * @description 批量分配角色,被分配者可以是用户,可以是部门
- * @param requestBody
- * @returns IsSuccessRespDto
- */
-func (c *Client) AssignRoleBatch(reqDto *dto.AssignRoleBatchDto) *dto.IsSuccessRespDto {
- b, err := c.SendHttpRequest("/api/v3/assign-role-batch", fasthttp.MethodPost, reqDto)
- var response dto.IsSuccessRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 移除分配的角色
- * @description 移除分配的角色,被分配者可以是用户,可以是部门
- * @param requestBody
- * @returns IsSuccessRespDto
- */
-func (c *Client) RevokeRole(reqDto *dto.RevokeRoleDto) *dto.IsSuccessRespDto {
- b, err := c.SendHttpRequest("/api/v3/revoke-role", fasthttp.MethodPost, reqDto)
- var response dto.IsSuccessRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 移除分配的角色
- * @description 移除分配的角色,被分配者可以是用户,可以是部门
- * @param requestBody
- * @returns IsSuccessRespDto
- */
-func (c *Client) RevokeRoleBatch(reqDto *dto.RevokeRoleBatchDto) *dto.IsSuccessRespDto {
- b, err := c.SendHttpRequest("/api/v3/revoke-role-batch", fasthttp.MethodPost, reqDto)
- var response dto.IsSuccessRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 角色被授权的资源列表
- * @description 角色被授权的资源列表
- * @param code 权限分组内角色的唯一标识符
- * @param namespace 所属权限分组的 code
- * @param resourceType 资源类型
- * @returns RoleAuthorizedResourcePaginatedRespDto
- */
-func (c *Client) GetRoleAuthorizedResources(reqDto *dto.GetRoleAuthorizedResourcesDto) *dto.RoleAuthorizedResourcePaginatedRespDto {
- b, err := c.SendHttpRequest("/api/v3/get-role-authorized-resources", fasthttp.MethodGet, reqDto)
- var response dto.RoleAuthorizedResourcePaginatedRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 获取角色成员列表
- * @description 获取角色成员列表
- * @param code 权限分组内角色的唯一标识符
- * @param page 当前页数,从 1 开始
- * @param limit 每页数目,最大不能超过 50,默认为 10
- * @param withCustomData 是否获取自定义数据
- * @param withIdentities 是否获取 identities
- * @param withDepartmentIds 是否获取部门 ID 列表
- * @param namespace 所属权限分组的 code
- * @returns UserPaginatedRespDto
- */
-func (c *Client) ListRoleMembers(reqDto *dto.ListRoleMembersDto) *dto.UserPaginatedRespDto {
- b, err := c.SendHttpRequest("/api/v3/list-role-members", fasthttp.MethodGet, reqDto)
- var response dto.UserPaginatedRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 获取角色的部门列表
- * @description 获取角色的部门列表
- * @param code 权限分组内角色的唯一标识符
- * @param namespace 所属权限分组的 code
- * @param page 当前页数,从 1 开始
- * @param limit 每页数目,最大不能超过 50,默认为 10
- * @returns RoleDepartmentListPaginatedRespDto
- */
-func (c *Client) ListRoleDepartments(reqDto *dto.ListRoleDepartmentsDto) *dto.RoleDepartmentListPaginatedRespDto {
- b, err := c.SendHttpRequest("/api/v3/list-role-departments", fasthttp.MethodGet, reqDto)
- var response dto.RoleDepartmentListPaginatedRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 创建角色
- * @description 创建角色,可以指定不同的权限分组
- * @param requestBody
- * @returns RoleSingleRespDto
- */
-func (c *Client) CreateRole(reqDto *dto.CreateRoleDto) *dto.RoleSingleRespDto {
- b, err := c.SendHttpRequest("/api/v3/create-role", fasthttp.MethodPost, reqDto)
- var response dto.RoleSingleRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 获取角色列表
- * @description 获取角色列表
- * @param namespace 所属权限分组的 code
- * @param page 当前页数,从 1 开始
- * @param limit 每页数目,最大不能超过 50,默认为 10
- * @returns RolePaginatedRespDto
- */
-func (c *Client) ListRoles(reqDto *dto.ListRolesDto) *dto.RolePaginatedRespDto {
- b, err := c.SendHttpRequest("/api/v3/list-roles", fasthttp.MethodGet, reqDto)
- var response dto.RolePaginatedRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary (批量)删除角色
- * @description 删除角色
- * @param requestBody
- * @returns IsSuccessRespDto
- */
-func (c *Client) DeleteRolesBatch(reqDto *dto.DeleteRoleDto) *dto.IsSuccessRespDto {
- b, err := c.SendHttpRequest("/api/v3/delete-roles-batch", fasthttp.MethodPost, reqDto)
- var response dto.IsSuccessRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 批量创建角色
- * @description 批量创建角色
- * @param requestBody
- * @returns IsSuccessRespDto
- */
-func (c *Client) CreateRolesBatch(reqDto *dto.CreateRolesBatch) *dto.IsSuccessRespDto {
- b, err := c.SendHttpRequest("/api/v3/create-roles-batch", fasthttp.MethodPost, reqDto)
- var response dto.IsSuccessRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 修改角色
- * @description 修改角色
- * @param requestBody
- * @returns IsSuccessRespDto
- */
-func (c *Client) UpdateRole(reqDto *dto.UpdateRoleDto) *dto.IsSuccessRespDto {
- b, err := c.SendHttpRequest("/api/v3/update-role", fasthttp.MethodPost, reqDto)
- var response dto.IsSuccessRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 获取顶层组织机构列表
- * @description 获取顶层组织机构列表
- * @param page 当前页数,从 1 开始
- * @param limit 每页数目,最大不能超过 50,默认为 10
- * @returns OrganizationPaginatedRespDto
- */
-func (c *Client) ListOrganizations(reqDto *dto.ListOrganizationsDto) *dto.OrganizationPaginatedRespDto {
- b, err := c.SendHttpRequest("/api/v3/list-organizations", fasthttp.MethodGet, reqDto)
- var response dto.OrganizationPaginatedRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 创建顶层组织机构
- * @description 创建组织机构,会创建一个只有一个节点的组织机构
- * @param requestBody
- * @returns OrganizationSingleRespDto
- */
-func (c *Client) CreateOrganization(reqDto *dto.CreateOrganizationReqDto) *dto.OrganizationSingleRespDto {
- b, err := c.SendHttpRequest("/api/v3/create-organization", fasthttp.MethodPost, reqDto)
- var response dto.OrganizationSingleRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 修改顶层组织机构
- * @description 修改顶层组织机构
- * @param requestBody
- * @returns OrganizationSingleRespDto
- */
-func (c *Client) UpdateOrganization(reqDto *dto.UpdateOrganizationReqDto) *dto.OrganizationSingleRespDto {
- b, err := c.SendHttpRequest("/api/v3/update-organization", fasthttp.MethodPost, reqDto)
- var response dto.OrganizationSingleRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 删除组织机构
- * @description 删除组织机构树
- * @param requestBody
- * @returns IsSuccessRespDto
- */
-func (c *Client) DeleteOrganization(reqDto *dto.DeleteOrganizationReqDto) *dto.IsSuccessRespDto {
- b, err := c.SendHttpRequest("/api/v3/delete-organization", fasthttp.MethodPost, reqDto)
- var response dto.IsSuccessRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 获取部门信息
- * @description 获取部门信息
- * @param organizationCode 组织 code
- * @param departmentId 部门 id,根部门传 `root`
- * @param departmentIdType 此次调用中使用的部门 ID 的类型
- * @returns DepartmentSingleRespDto
- */
-func (c *Client) GetDepartment(reqDto *dto.GetDepartmentDto) *dto.DepartmentSingleRespDto {
- b, err := c.SendHttpRequest("/api/v3/get-department", fasthttp.MethodGet, reqDto)
- var response dto.DepartmentSingleRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 创建部门
- * @description 创建部门
- * @param requestBody
- * @returns DepartmentSingleRespDto
- */
-func (c *Client) CreateDepartment(reqDto *dto.CreateDepartmentReqDto) *dto.DepartmentSingleRespDto {
- b, err := c.SendHttpRequest("/api/v3/create-department", fasthttp.MethodPost, reqDto)
- var response dto.DepartmentSingleRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 修改部门
- * @description 修改部门
- * @param requestBody
- * @returns DepartmentSingleRespDto
- */
-func (c *Client) UpdateDepartment(reqDto *dto.UpdateDepartmentReqDto) *dto.DepartmentSingleRespDto {
- b, err := c.SendHttpRequest("/api/v3/update-department", fasthttp.MethodPost, reqDto)
- var response dto.DepartmentSingleRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 删除部门
- * @description 删除部门
- * @param requestBody
- * @returns IsSuccessRespDto
- */
-func (c *Client) DeleteDepartment(reqDto *dto.DeleteDepartmentReqDto) *dto.IsSuccessRespDto {
- b, err := c.SendHttpRequest("/api/v3/delete-department", fasthttp.MethodPost, reqDto)
- var response dto.IsSuccessRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 搜索部门
- * @description 搜索部门
- * @param requestBody
- * @returns DepartmentListRespDto
- */
-func (c *Client) SearchDepartments(reqDto *dto.SearchDepartmentsReqDto) *dto.DepartmentListRespDto {
- b, err := c.SendHttpRequest("/api/v3/search-departments", fasthttp.MethodPost, reqDto)
- var response dto.DepartmentListRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 获取子部门列表
- * @description 获取子部门列表
- * @param departmentId 需要获取的部门 ID
- * @param organizationCode 组织 code
- * @param departmentIdType 此次调用中使用的部门 ID 的类型
- * @returns DepartmentPaginatedRespDto
- */
-func (c *Client) ListChildrenDepartments(reqDto *dto.ListChildrenDepartmentsDto) *dto.DepartmentPaginatedRespDto {
- b, err := c.SendHttpRequest("/api/v3/list-children-departments", fasthttp.MethodGet, reqDto)
- var response dto.DepartmentPaginatedRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 获取部门直属成员列表
- * @description 获取部门直属成员列表
- * @param organizationCode 组织 code
- * @param departmentId 部门 id,根部门传 `root`
- * @param departmentIdType 此次调用中使用的部门 ID 的类型
- * @param page 当前页数,从 1 开始
- * @param limit 每页数目,最大不能超过 50,默认为 10
- * @param withCustomData 是否获取自定义数据
- * @param withIdentities 是否获取 identities
- * @param withDepartmentIds 是否获取部门 ID 列表
- * @returns UserListRespDto
- */
-func (c *Client) ListDepartmentMembers(reqDto *dto.ListDepartmentMembersDto) *dto.UserListRespDto {
- b, err := c.SendHttpRequest("/api/v3/list-department-members", fasthttp.MethodGet, reqDto)
- var response dto.UserListRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 获取部门直属成员 ID 列表
- * @description 获取部门直属成员 ID 列表
- * @param organizationCode 组织 code
- * @param departmentId 部门 id,根部门传 `root`
- * @param departmentIdType 此次调用中使用的部门 ID 的类型
- * @returns UserIdListRespDto
- */
-func (c *Client) ListDepartmentMemberIds(reqDto *dto.ListDepartmentMemberIdsDto) *dto.UserIdListRespDto {
- b, err := c.SendHttpRequest("/api/v3/list-department-member-ids", fasthttp.MethodGet, reqDto)
- var response dto.UserIdListRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 部门下添加成员
- * @description 部门下添加成员
- * @param requestBody
- * @returns IsSuccessRespDto
- */
-func (c *Client) AddDepartmentMembers(reqDto *dto.AddDepartmentMembersReqDto) *dto.IsSuccessRespDto {
- b, err := c.SendHttpRequest("/api/v3/add-department-members", fasthttp.MethodPost, reqDto)
- var response dto.IsSuccessRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 部门下删除成员
- * @description 部门下删除成员
- * @param requestBody
- * @returns IsSuccessRespDto
- */
-func (c *Client) RemoveDepartmentMembers(reqDto *dto.RemoveDepartmentMembersReqDto) *dto.IsSuccessRespDto {
- b, err := c.SendHttpRequest("/api/v3/remove-department-members", fasthttp.MethodPost, reqDto)
- var response dto.IsSuccessRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 获取父部门信息
- * @description 获取父部门信息
- * @param organizationCode 组织 code
- * @param departmentId 部门 id
- * @param departmentIdType 此次调用中使用的部门 ID 的类型
- * @returns DepartmentSingleRespDto
- */
-func (c *Client) GetParentDepartment(reqDto *dto.GetParentDepartmentDto) *dto.DepartmentSingleRespDto {
- b, err := c.SendHttpRequest("/api/v3/get-parent-department", fasthttp.MethodGet, reqDto)
- var response dto.DepartmentSingleRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 获取身份源列表
- * @description 获取身份源列表
- * @param tenantId 租户 ID
- * @returns ExtIdpListPaginatedRespDto
- */
-func (c *Client) ListExtIdp(reqDto *dto.ListExtIdpDto) *dto.ExtIdpListPaginatedRespDto {
- b, err := c.SendHttpRequest("/api/v3/list-ext-idp", fasthttp.MethodGet, reqDto)
- var response dto.ExtIdpListPaginatedRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 获取身份源详情
- * @description 获取身份源详情
- * @param id 身份源 id
- * @param tenantId 租户 ID
- * @returns ExtIdpDetailSingleRespDto
- */
-func (c *Client) GetExtIdp(reqDto *dto.GetExtIdpDto) *dto.ExtIdpDetailSingleRespDto {
- b, err := c.SendHttpRequest("/api/v3/get-ext-idp", fasthttp.MethodGet, reqDto)
- var response dto.ExtIdpDetailSingleRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 创建身份源
- * @description 创建身份源
- * @param requestBody
- * @returns ExtIdpSingleRespDto
- */
-func (c *Client) CreateExtIdp(reqDto *dto.CreateExtIdpDto) *dto.ExtIdpSingleRespDto {
- b, err := c.SendHttpRequest("/api/v3/create-ext-idp", fasthttp.MethodPost, reqDto)
- var response dto.ExtIdpSingleRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 更新身份源配置
- * @description 更新身份源配置
- * @param requestBody
- * @returns ExtIdpSingleRespDto
- */
-func (c *Client) UpdateExtIdp(reqDto *dto.UpdateExtIdpDto) *dto.ExtIdpSingleRespDto {
- b, err := c.SendHttpRequest("/api/v3/update-ext-idp", fasthttp.MethodPost, reqDto)
- var response dto.ExtIdpSingleRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 删除身份源
- * @description 删除身份源
- * @param requestBody
- * @returns IsSuccessRespDto
- */
-func (c *Client) DeleteExtIdp(reqDto *dto.DeleteExtIdpDto) *dto.IsSuccessRespDto {
- b, err := c.SendHttpRequest("/api/v3/delete-ext-idp", fasthttp.MethodPost, reqDto)
- var response dto.IsSuccessRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 在某个已有身份源下创建新连接
- * @description 在某个已有身份源下创建新连接
- * @param requestBody
- * @returns ExtIdpConnDetailSingleRespDto
- */
-func (c *Client) CreateExtIdpConn(reqDto *dto.CreateExtIdpConnDto) *dto.ExtIdpConnDetailSingleRespDto {
- b, err := c.SendHttpRequest("/api/v3/create-ext-idp-conn", fasthttp.MethodPost, reqDto)
- var response dto.ExtIdpConnDetailSingleRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 更新身份源连接
- * @description 更新身份源连接
- * @param requestBody
- * @returns ExtIdpConnDetailSingleRespDto
- */
-func (c *Client) UpdateExtIdpConn(reqDto *dto.UpdateExtIdpConnDto) *dto.ExtIdpConnDetailSingleRespDto {
- b, err := c.SendHttpRequest("/api/v3/update-ext-idp-conn", fasthttp.MethodPost, reqDto)
- var response dto.ExtIdpConnDetailSingleRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 删除身份源连接
- * @description 删除身份源连接
- * @param requestBody
- * @returns IsSuccessRespDto
- */
-func (c *Client) DeleteExtIdpConn(reqDto *dto.DeleteExtIdpConnDto) *dto.IsSuccessRespDto {
- b, err := c.SendHttpRequest("/api/v3/delete-ext-idp-conn", fasthttp.MethodPost, reqDto)
- var response dto.IsSuccessRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 身份源连接开关
- * @description 身份源连接开关
- * @param requestBody
- * @returns IsSuccessRespDto
- */
-func (c *Client) ChangeConnState(reqDto *dto.EnableExtIdpConnDto) *dto.IsSuccessRespDto {
- b, err := c.SendHttpRequest("/api/v3/enable-ext-idp-conn", fasthttp.MethodPost, reqDto)
- var response dto.IsSuccessRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 获取用户池配置的自定义字段列表
- * @description 获取用户池配置的自定义字段列表
- * @param targetType 主体类型,目前支持用户、角色、分组和部门
- * @returns CustomFieldListRespDto
- */
-func (c *Client) GetCustomFields(reqDto *dto.GetCustomFieldsDto) *dto.CustomFieldListRespDto {
- b, err := c.SendHttpRequest("/api/v3/get-custom-fields", fasthttp.MethodGet, reqDto)
- var response dto.CustomFieldListRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 创建/修改自定义字段定义
- * @description 创建/修改自定义字段定义,如果传入的 key 不存在则创建,存在则更新。
- * @param requestBody
- * @returns CustomFieldListRespDto
- */
-func (c *Client) SetCustomFields(reqDto *dto.SetCustomFieldsReqDto) *dto.CustomFieldListRespDto {
- b, err := c.SendHttpRequest("/api/v3/set-custom-fields", fasthttp.MethodPost, reqDto)
- var response dto.CustomFieldListRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 设置自定义字段的值
- * @description 给用户、角色、部门设置自定义字段的值,如果存在则更新,不存在则创建。
- * @param requestBody
- * @returns IsSuccessRespDto
- */
-func (c *Client) SetCustomData(reqDto *dto.SetCustomDataReqDto) *dto.IsSuccessRespDto {
- b, err := c.SendHttpRequest("/api/v3/set-custom-data", fasthttp.MethodPost, reqDto)
- var response dto.IsSuccessRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 获取用户、分组、角色、组织机构的自定义字段值
- * @description 获取用户、分组、角色、组织机构的自定义字段值
- * @param targetType 主体类型,目前支持用户、角色、分组和部门
- * @param targetIdentifier 目标对象唯一标志符
- * @param namespace 所属权限分组的 code,当 target_type 为角色的时候需要填写,否则可以忽略。
- * @returns GetCustomDataRespDto
- */
-func (c *Client) GetCustomData(reqDto *dto.GetCustomDataDto) *dto.GetCustomDataRespDto {
- b, err := c.SendHttpRequest("/api/v3/get-custom-data", fasthttp.MethodGet, reqDto)
- var response dto.GetCustomDataRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 创建资源
- * @description 创建资源
- * @param requestBody
- * @returns ResourceRespDto
- */
-func (c *Client) CreateResource(reqDto *dto.CreateResourceDto) *dto.ResourceRespDto {
- b, err := c.SendHttpRequest("/api/v3/create-resource", fasthttp.MethodPost, reqDto)
- var response dto.ResourceRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 批量创建资源
- * @description 批量创建资源
- * @param requestBody
- * @returns IsSuccessRespDto
- */
-func (c *Client) CreateResourcesBatch(reqDto *dto.CreateResourcesBatchDto) *dto.IsSuccessRespDto {
- b, err := c.SendHttpRequest("/api/v3/create-resources-batch", fasthttp.MethodPost, reqDto)
- var response dto.IsSuccessRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 获取资源详情
- * @description 获取资源详情
- * @param code 资源唯一标志符
- * @param namespace 所属权限分组的 code
- * @returns ResourceRespDto
- */
-func (c *Client) GetResource(reqDto *dto.GetResourceDto) *dto.ResourceRespDto {
- b, err := c.SendHttpRequest("/api/v3/get-resource", fasthttp.MethodGet, reqDto)
- var response dto.ResourceRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 批量获取资源详情
- * @description 批量获取资源详情
- * @param codeList 资源 code 列表,批量可以使用逗号分隔
- * @param namespace 所属权限分组的 code
- * @returns ResourceListRespDto
- */
-func (c *Client) GetResourcesBatch(reqDto *dto.GetResourcesBatchDto) *dto.ResourceListRespDto {
- b, err := c.SendHttpRequest("/api/v3/get-resources-batch", fasthttp.MethodGet, reqDto)
- var response dto.ResourceListRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 分页获取资源列表
- * @description 分页获取资源列表
- * @param namespace 所属权限分组的 code
- * @param type 资源类型
- * @param page 当前页数,从 1 开始
- * @param limit 每页数目,最大不能超过 50,默认为 10
- * @returns ResourcePaginatedRespDto
- */
-func (c *Client) ListResources(reqDto *dto.ListResourcesDto) *dto.ResourcePaginatedRespDto {
- b, err := c.SendHttpRequest("/api/v3/list-resources", fasthttp.MethodGet, reqDto)
- var response dto.ResourcePaginatedRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 修改资源
- * @description 修改资源(Pratial Update)
- * @param requestBody
- * @returns ResourceRespDto
- */
-func (c *Client) UpdateResource(reqDto *dto.UpdateResourceDto) *dto.ResourceRespDto {
- b, err := c.SendHttpRequest("/api/v3/update-resource", fasthttp.MethodPost, reqDto)
- var response dto.ResourceRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 删除资源
- * @description 删除资源
- * @param requestBody
- * @returns IsSuccessRespDto
- */
-func (c *Client) DeleteResource(reqDto *dto.DeleteResourceDto) *dto.IsSuccessRespDto {
- b, err := c.SendHttpRequest("/api/v3/delete-resource", fasthttp.MethodPost, reqDto)
- var response dto.IsSuccessRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 批量删除资源
- * @description 批量删除资源
- * @param requestBody
- * @returns IsSuccessRespDto
- */
-func (c *Client) DeleteResourcesBatch(reqDto *dto.DeleteResourcesBatchDto) *dto.IsSuccessRespDto {
- b, err := c.SendHttpRequest("/api/v3/delete-resources-batch", fasthttp.MethodPost, reqDto)
- var response dto.IsSuccessRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 创建权限分组
- * @description 创建权限分组
- * @param requestBody
- * @returns ResourceDto
- */
-func (c *Client) CreateNamespace(reqDto *dto.CreateNamespaceDto) *dto.ResourceDto {
- b, err := c.SendHttpRequest("/api/v3/create-namespace", fasthttp.MethodPost, reqDto)
- var response dto.ResourceDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 批量创建权限分组
- * @description 批量创建权限分组
- * @param requestBody
- * @returns IsSuccessRespDto
- */
-func (c *Client) CreateNamespacesBatch(reqDto *dto.CreateNamespacesBatchDto) *dto.IsSuccessRespDto {
- b, err := c.SendHttpRequest("/api/v3/create-namespaces-batch", fasthttp.MethodPost, reqDto)
- var response dto.IsSuccessRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 获取权限分组详情
- * @description 获取权限分组详情
- * @param code 权限分组唯一标志符
- * @returns NamespaceRespDto
- */
-func (c *Client) GetNamespace(reqDto *dto.GetNamespaceDto) *dto.NamespaceRespDto {
- b, err := c.SendHttpRequest("/api/v3/get-namespace", fasthttp.MethodGet, reqDto)
- var response dto.NamespaceRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 批量获取权限分组详情
- * @description 批量获取权限分组详情
- * @param codeList 资源 code 列表,批量可以使用逗号分隔
- * @returns NamespaceListRespDto
- */
-func (c *Client) GetNamespacesBatch(reqDto *dto.GetNamespacesBatchDto) *dto.NamespaceListRespDto {
- b, err := c.SendHttpRequest("/api/v3/get-namespaces-batch", fasthttp.MethodGet, reqDto)
- var response dto.NamespaceListRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 修改权限分组信息
- * @description 修改权限分组信息
- * @param requestBody
- * @returns UpdateNamespaceRespDto
- */
-func (c *Client) UpdateNamespace(reqDto *dto.UpdateNamespaceDto) *dto.UpdateNamespaceRespDto {
- b, err := c.SendHttpRequest("/api/v3/update-namespace", fasthttp.MethodPost, reqDto)
- var response dto.UpdateNamespaceRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 删除权限分组信息
- * @description 删除权限分组信息
- * @param requestBody
- * @returns IsSuccessRespDto
- */
-func (c *Client) DeleteNamespace(reqDto *dto.DeleteNamespaceDto) *dto.IsSuccessRespDto {
- b, err := c.SendHttpRequest("/api/v3/delete-namespace", fasthttp.MethodPost, reqDto)
- var response dto.IsSuccessRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 批量删除权限分组
- * @description 批量删除权限分组
- * @param requestBody
- * @returns IsSuccessRespDto
- */
-func (c *Client) DeleteNamespacesBatch(reqDto *dto.DeleteNamespacesBatchDto) *dto.IsSuccessRespDto {
- b, err := c.SendHttpRequest("/api/v3/delete-namespaces-batch", fasthttp.MethodPost, reqDto)
- var response dto.IsSuccessRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 授权资源
- * @description 给多个主体同时授权多个资源
- * @param requestBody
- * @returns IsSuccessRespDto
- */
-func (c *Client) AuthorizeResources(reqDto *dto.AuthorizeResourcesDto) *dto.IsSuccessRespDto {
- b, err := c.SendHttpRequest("/api/v3/authorize-resources", fasthttp.MethodPost, reqDto)
- var response dto.IsSuccessRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
-
-/*
- * @summary 获取某个主体被授权的资源列表
- * @description 获取某个主体被授权的资源列表
- * @param targetType 目标对象类型
- * @param targetIdentifier 目标对象唯一标志符
- * @param namespace 所属权限分组的 code
- * @param resourceType 资源类型,如数据、API、按钮、菜单
- * @returns IsSuccessRespDto
- */
-func (c *Client) GetTargetAuthorizedResources(reqDto *dto.GetAuthorizedResourcesDto) *dto.IsSuccessRespDto {
- b, err := c.SendHttpRequest("/api/v3/get-authorized-resources", fasthttp.MethodGet, reqDto)
- var response dto.IsSuccessRespDto
- if err != nil {
- return nil
- }
- json.Unmarshal(b, &response)
- return &response
-}
diff --git a/client/management_client_test.go b/client/management_client_test.go
deleted file mode 100644
index 00a6ca5..0000000
--- a/client/management_client_test.go
+++ /dev/null
@@ -1,1082 +0,0 @@
-package client
-
-import (
- "authing-go-sdk/dto"
- "fmt"
- "testing"
-)
-
-var client *Client
-
-func init() {
- options := ManagementClientOptions{
- AccessKeyId: "60e043f8cd91b87d712b6365",
- AccessKeySecret: "158c7679333bc196b524d78d745813e5",
- }
- var err error
- client, err = NewClient(&options)
- if err != nil {
- panic(err)
- }
-}
-
-func TestClient_GetResource(t *testing.T) {
- request := dto.GetResourceDto{
- Code: "code_3373",
- Namespace: "namespace_6254",
- }
- response := client.GetResource(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_GetNamespace(t *testing.T) {
- request := dto.GetNamespaceDto{
- Code: "code_1335",
- }
- response := client.GetNamespace(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_HasAnyRole(t *testing.T) {
- request := dto.HasAnyRoleReqDto{
- Roles: nil,
- UserId: "userId_3868",
- }
- response := client.HasAnyRole(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_SetCustomData(t *testing.T) {
- request := dto.SetCustomDataReqDto{
- List: nil,
- TargetIdentifier: "targetIdentifier_9468",
- TargetType: "",
- Namespace: "namespace_4136",
- }
- response := client.SetCustomData(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_GetCustomData(t *testing.T) {
- request := dto.GetCustomDataDto{
- TargetType: "targetType_4930",
- TargetIdentifier: "targetIdentifier_5664",
- Namespace: "namespace_6211",
- }
- response := client.GetCustomData(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_DeleteNamespace(t *testing.T) {
- request := dto.DeleteNamespaceDto{
- Code: "code_4611",
- }
- response := client.DeleteNamespace(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_AuthorizeResources(t *testing.T) {
- request := dto.AuthorizeResourcesDto{
- List: nil,
- Namespace: "namespace_3067",
- }
- response := client.AuthorizeResources(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_GetManagementToken(t *testing.T) {
- request := dto.GetManagementAccessTokenDto{
- AccessKeySecret: "accessKeySecret_7021",
- AccessKeyId: "accessKeyId_4717",
- }
- response := client.GetManagementToken(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_GetUser(t *testing.T) {
- request := dto.GetUserDto{
- UserId: "userId_6520",
- WithCustomData: false,
- WithIdentities: false,
- WithDepartmentIds: false,
- Phone: "phone_1889",
- Email: "email_1688",
- Username: "username_6931",
- ExternalId: "externalId_4495",
- }
- response := client.GetUser(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_ListUsers(t *testing.T) {
- request := dto.ListUsersDto{
- Page: 0,
- Limit: 0,
- WithCustomData: false,
- WithIdentities: false,
- WithDepartmentIds: false,
- }
- response := client.ListUsers(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_GetUserBatch(t *testing.T) {
- request := dto.GetUserBatchDto{
- UserIds: "userIds_7230",
- WithCustomData: false,
- WithIdentities: false,
- WithDepartmentIds: false,
- }
- response := client.GetUserBatch(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_GetUserIdentities(t *testing.T) {
- request := dto.GetUserIdentitiesDto{
- UserId: "userId_7225",
- }
- response := client.GetUserIdentities(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_CreateOrganization(t *testing.T) {
- request := dto.CreateOrganizationReqDto{
- OrganizationName: "organizationName_2027",
- OrganizationCode: "organizationCode_2270",
- OpenDepartmentId: "openDepartmentId_1674",
- }
- response := client.CreateOrganization(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_UpdateExtIdpConn(t *testing.T) {
- request := dto.UpdateExtIdpConnDto{
- Fields: nil,
- DisplayName: "displayName_8594",
- Id: "id_5185",
- Logo: "logo_8928",
- LoginOnly: false,
- }
- response := client.UpdateExtIdpConn(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_DeleteOrganization(t *testing.T) {
- request := dto.DeleteOrganizationReqDto{
- OrganizationCode: "organizationCode_7953",
- }
- response := client.DeleteOrganization(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_ChangeConnState(t *testing.T) {
- request := dto.EnableExtIdpConnDto{
- AppId: "appId_8921",
- Enabled: false,
- Id: "id_2921",
- TenantId: "tenantId_7497",
- }
- response := client.ChangeConnState(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_UpdateOrganization(t *testing.T) {
- request := dto.UpdateOrganizationReqDto{
- OrganizationCode: "organizationCode_3319",
- OpenDepartmentId: "openDepartmentId_8572",
- OrganizationNewCode: "organizationNewCode_530",
- OrganizationName: "organizationName_4419",
- }
- response := client.UpdateOrganization(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_SetCustomFields(t *testing.T) {
- request := dto.SetCustomFieldsReqDto{
- List: nil,
- }
- response := client.SetCustomFields(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_AssignRole(t *testing.T) {
- request := dto.AssignRoleDto{
- Targets: nil,
- Code: "code_1735",
- Namespace: "namespace_7758",
- }
- response := client.AssignRole(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_CreateGroupBatch(t *testing.T) {
- request := dto.CreateGroupBatchReqDto{
- List: nil,
- }
- response := client.CreateGroupBatch(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_GetUserMfaInfo(t *testing.T) {
- request := dto.GetUserMfaInfoDto{
- UserId: "userId_2063",
- }
- response := client.GetUserMfaInfo(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_GetRole(t *testing.T) {
- request := dto.GetRoleDto{
- Code: "code_3595",
- Namespace: "namespace_68",
- }
- response := client.GetRole(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_UpdateUser(t *testing.T) {
- request := dto.UpdateUserReqDto{
- UserId: "userId_8460",
- PhoneCountryCode: "phoneCountryCode_8091",
- Name: "name_8726",
- Nickname: "nickname_6206",
- Photo: "photo_7414",
- ExternalId: "externalId_3102",
- Status: "",
-
- EmailVerified: false,
- PhoneVerified: false,
- Birthdate: "birthdate_4429",
- Country: "country_7148",
- Province: "province_2823",
- City: "city_5141",
- Address: "address_1292",
- StreetAddress: "streetAddress_1482",
- PostalCode: "postalCode_2354",
- Gender: "",
-
- Username: "username_1579",
- PasswordEncryptType: "",
-
- Email: "email_358",
- Phone: "phone_5766",
- Password: "password_6386",
- CustomData: nil,
- }
- response := client.UpdateUser(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_AddGroupMembers(t *testing.T) {
- request := dto.AddGroupMembersReqDto{
- UserIds: nil,
- Code: "code_9093",
- }
- response := client.AddGroupMembers(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_RevokeRoleBatch(t *testing.T) {
- request := dto.RevokeRoleBatchDto{
- Targets: nil,
- Roles: nil,
- }
- response := client.RevokeRoleBatch(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_DeleteDepartment(t *testing.T) {
- request := dto.DeleteDepartmentReqDto{
- OrganizationCode: "organizationCode_7898",
- DepartmentId: "departmentId_8897",
- DepartmentIdType: "",
- }
- response := client.DeleteDepartment(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_GetResourcesBatch(t *testing.T) {
- request := dto.GetResourcesBatchDto{
- CodeList: "codeList_1627",
- Namespace: "namespace_2983",
- }
- response := client.GetResourcesBatch(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_CreateRole(t *testing.T) {
- request := dto.CreateRoleDto{
- Code: "code_70",
- Namespace: "namespace_5167",
- Description: "description_3228",
- }
- response := client.CreateRole(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_GetNamespacesBatch(t *testing.T) {
- request := dto.GetNamespacesBatchDto{
- CodeList: "codeList_1740",
- }
- response := client.GetNamespacesBatch(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_ListResources(t *testing.T) {
- request := dto.ListResourcesDto{
- Namespace: "namespace_3029",
- Type: "type_3240",
- Page: 0,
- Limit: 0,
- }
- response := client.ListResources(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_GetExtIdp(t *testing.T) {
- request := dto.GetExtIdpDto{
- Id: "id_8918",
- TenantId: "tenantId_7944",
- }
- response := client.GetExtIdp(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_ListArchivedUsers(t *testing.T) {
- request := dto.ListArchivedUsersDto{
- Page: 0,
- Limit: 0,
- }
- response := client.ListArchivedUsers(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_DeleteRolesBatch(t *testing.T) {
- request := dto.DeleteRoleDto{
- CodeList: nil,
- Namespace: "namespace_5144",
- }
- response := client.DeleteRolesBatch(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_CreateUser(t *testing.T) {
- request := dto.CreateUserReqDto{
- Status: "",
-
- Email: "email_5835",
- PasswordEncryptType: "",
-
- Phone: "phone_4953",
- PhoneCountryCode: "phoneCountryCode_6088",
- Username: "username_3276",
- Name: "name_3190",
- Nickname: "nickname_5535",
- Photo: "photo_1501",
- Gender: "",
-
- EmailVerified: false,
- PhoneVerified: false,
- Birthdate: "birthdate_2271",
- Country: "country_405",
- Province: "province_5583",
- City: "city_4082",
- Address: "address_7628",
- StreetAddress: "streetAddress_2665",
- PostalCode: "postalCode_2513",
- ExternalId: "externalId_784",
- DepartmentIds: nil,
- CustomData: nil,
- Password: "password_3785",
- TenantIds: nil,
- Identities: nil,
- Options: dto.CreateUserOptionsDto{
- KeepPassword: false,
- ResetPasswordOnFirstLogin: false,
- DepartmentIdType: "",
- },
- }
- response := client.CreateUser(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_SearchDepartments(t *testing.T) {
- request := dto.SearchDepartmentsReqDto{
- Search: "search_5854",
- OrganizationCode: "organizationCode_1752",
- }
- response := client.SearchDepartments(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_GetUserGroups(t *testing.T) {
- request := dto.GetUserGroupsDto{
- UserId: "userId_789",
- }
- response := client.GetUserGroups(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_IsUserExists(t *testing.T) {
- request := dto.IsUserExistsReqDto{
- Username: "username_9604",
- Email: "email_2780",
- Phone: "phone_7044",
- ExternalId: "externalId_6293",
- }
- response := client.IsUserExists(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_KickUsers(t *testing.T) {
- request := dto.KickUsersDto{
- AppIds: nil,
- UserId: "userId_5104",
- }
- response := client.KickUsers(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_CreateUserBatch(t *testing.T) {
- request := dto.CreateUserBatchReqDto{
- List: nil,
- Options: dto.CreateUserOptionsDto{},
- }
- response := client.CreateUserBatch(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_RemoveGroupMembers(t *testing.T) {
- request := dto.RemoveGroupMembersReqDto{
- UserIds: nil,
- Code: "code_9703",
- }
- response := client.RemoveGroupMembers(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_ListRoleMembers(t *testing.T) {
- request := dto.ListRoleMembersDto{
- Code: "code_2861",
- Page: 0,
- Limit: 0,
- WithCustomData: false,
- WithIdentities: false,
- WithDepartmentIds: false,
- Namespace: "namespace_872",
- }
- response := client.ListRoleMembers(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_CreateExtIdpConn(t *testing.T) {
- request := dto.CreateExtIdpConnDto{
- Fields: nil,
- DisplayName: "displayName_7799",
- Identifier: "identifier_6069",
- Type: "",
-
- ExtIdpId: "extIdpId_9458",
- LoginOnly: false,
- Logo: "logo_7996",
- }
- response := client.CreateExtIdpConn(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_AssignRoleBatch(t *testing.T) {
- request := dto.AssignRoleBatchDto{
- Targets: nil,
- Roles: nil,
- }
- response := client.AssignRoleBatch(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_DeleteExtIdpConn(t *testing.T) {
- request := dto.DeleteExtIdpConnDto{
- Id: "id_3553",
- }
- response := client.DeleteExtIdpConn(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_GetGroup(t *testing.T) {
- request := dto.GetGroupDto{
- Code: "code_9948",
- }
- response := client.GetGroup(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_GetCustomFields(t *testing.T) {
- request := dto.GetCustomFieldsDto{
- TargetType: "targetType_6791",
- }
- response := client.GetCustomFields(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_CreateResource(t *testing.T) {
- request := dto.CreateResourceDto{
- Type: "",
-
- Code: "code_6877",
- Description: "description_4762",
- Actions: nil,
- ApiIdentifier: "apiIdentifier_521",
- Namespace: "namespace_519",
- }
- response := client.CreateResource(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_UpdateResource(t *testing.T) {
- request := dto.UpdateResourceDto{
- Code: "code_3665",
- Description: "description_3254",
- Actions: nil,
- ApiIdentifier: "apiIdentifier_5164",
- Namespace: "namespace_2270",
- Type: "",
- }
- response := client.UpdateResource(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_ListRoles(t *testing.T) {
- request := dto.ListRolesDto{
- Namespace: "namespace_8667",
- Page: 0,
- Limit: 0,
- }
- response := client.ListRoles(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_CreateRolesBatch(t *testing.T) {
- request := dto.CreateRolesBatch{
- List: nil,
- }
- response := client.CreateRolesBatch(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_DeleteExtIdp(t *testing.T) {
- request := dto.DeleteExtIdpDto{
- Id: "id_5139",
- }
- response := client.DeleteExtIdp(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_CreateNamespace(t *testing.T) {
- request := dto.CreateNamespaceDto{
- Code: "code_7508",
- Name: "name_9713",
- Description: "description_8998",
- }
- response := client.CreateNamespace(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_DeleteResource(t *testing.T) {
- request := dto.DeleteResourceDto{
- Code: "code_2243",
- Namespace: "namespace_1961",
- }
- response := client.DeleteResource(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_GetDepartment(t *testing.T) {
- request := dto.GetDepartmentDto{
- OrganizationCode: "organizationCode_7418",
- DepartmentId: "departmentId_3675",
- DepartmentIdType: "departmentIdType_8967",
- }
- response := client.GetDepartment(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_RevokeRole(t *testing.T) {
- request := dto.RevokeRoleDto{
- Targets: nil,
- Code: "code_1746",
- Namespace: "namespace_9387",
- }
- response := client.RevokeRole(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_GetUserRoles(t *testing.T) {
- request := dto.GetUserRolesDto{
- UserId: "userId_8750",
- Namespace: "namespace_5251",
- }
- response := client.GetUserRoles(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_CreateGroup(t *testing.T) {
- request := dto.CreateGroupReqDto{
- Description: "description_715",
- Name: "name_1878",
- Code: "code_6657",
- }
- response := client.CreateGroup(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_SetUserDepartment(t *testing.T) {
- request := dto.SetUserDepartmentsDto{
- Departments: nil,
- UserId: "userId_8380",
- }
- response := client.SetUserDepartment(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_ListOrganizations(t *testing.T) {
- request := dto.ListOrganizationsDto{
- Page: 0,
- Limit: 0,
- }
- response := client.ListOrganizations(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_CreateExtIdp(t *testing.T) {
- request := dto.CreateExtIdpDto{
- Type: "",
-
- Name: "name_1550",
- TenantId: "tenantId_3102",
- }
- response := client.CreateExtIdp(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_UpdateNamespace(t *testing.T) {
- request := dto.UpdateNamespaceDto{
- Code: "code_8527",
- Description: "description_6479",
- Name: "name_4334",
- NewCode: "newCode_8628",
- }
- response := client.UpdateNamespace(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_DeleteUserBatch(t *testing.T) {
- request := dto.DeleteUsersBatchDto{
- UserIds: nil,
- }
- response := client.DeleteUserBatch(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_GetGroupList(t *testing.T) {
- request := dto.ListGroupsDto{
- Page: 0,
- Limit: 0,
- }
- response := client.GetGroupList(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_ListGroupMembers(t *testing.T) {
- request := dto.ListGroupMembersDto{
- Code: "code_9936",
- Page: 0,
- Limit: 0,
- WithCustomData: false,
- WithIdentities: false,
- WithDepartmentIds: false,
- }
- response := client.ListGroupMembers(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_ListExtIdp(t *testing.T) {
- request := dto.ListExtIdpDto{
- TenantId: "tenantId_1328",
- }
- response := client.ListExtIdp(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_UpdateDepartment(t *testing.T) {
- request := dto.UpdateDepartmentReqDto{
- OrganizationCode: "organizationCode_9222",
- ParentDepartmentId: "parentDepartmentId_9680",
- DepartmentId: "departmentId_4275",
- Code: "code_930",
- LeaderUserId: "leaderUserId_1549",
- Name: "name_9439",
- DepartmentIdType: "",
- }
- response := client.UpdateDepartment(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_GetUserDepartments(t *testing.T) {
- request := dto.GetUserDepartmentsDto{
- UserId: "userId_407",
- }
- response := client.GetUserDepartments(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_UpdateExtIdp(t *testing.T) {
- request := dto.UpdateExtIdpDto{
- Id: "id_9024",
- Name: "name_1707",
- }
- response := client.UpdateExtIdp(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_CreateDepartment(t *testing.T) {
- request := dto.CreateDepartmentReqDto{
- OrganizationCode: "organizationCode_1237",
- Name: "name_9915",
- ParentDepartmentId: "parentDepartmentId_4526",
- OpenDepartmentId: "openDepartmentId_5397",
- Code: "code_8456",
- LeaderUserId: "leaderUserId_870",
- DepartmentIdType: "",
- }
- response := client.CreateDepartment(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_UpdateGroup(t *testing.T) {
- request := dto.UpdateGroupReqDto{
- Description: "description_2466",
- Name: "name_9748",
- Code: "code_2809",
- NewCode: "newCode_1722",
- }
- response := client.UpdateGroup(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_DeleteGroups(t *testing.T) {
- request := dto.DeleteGroupsReqDto{
- CodeList: nil,
- }
- response := client.DeleteGroups(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_UpdateRole(t *testing.T) {
- request := dto.UpdateRoleDto{
- NewCode: "newCode_8752",
- Code: "code_3047",
- Namespace: "namespace_2695",
- Description: "description_376",
- }
- response := client.UpdateRole(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_GetUserLoginHistory(t *testing.T) {
- request := dto.GetUserLoginHistoryDto{
- UserId: "userId_7246",
- AppId: "appId_6638",
- ClientIp: "clientIp_3050",
- Start: 0,
- End: 0,
- Page: 0,
- Limit: 0,
- }
- response := client.GetUserLoginHistory(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_GetUserLoggedInApps(t *testing.T) {
- request := dto.GetUserLoggedinAppsDto{
- UserId: "userId_1810",
- }
- response := client.GetUserLoggedInApps(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_GetUserAuthorizedApps(t *testing.T) {
- request := dto.GetUserAuthorizedAppsDto{
- UserId: "userId_9193",
- }
- response := client.GetUserAuthorizedApps(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_GetGroupAuthorizedResources(t *testing.T) {
- request := dto.GetGroupAuthorizedResourcesDto{
- Code: "code_5382",
- Namespace: "namespace_107",
- ResourceType: "resourceType_5496",
- }
- response := client.GetGroupAuthorizedResources(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_GetUserAccessibleApps(t *testing.T) {
- request := dto.GetUserAccessibleAppsDto{
- UserId: "userId_7524",
- }
- response := client.GetUserAccessibleApps(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_GetUserAuthorizedResources(t *testing.T) {
- request := dto.GetUserAuthorizedResourcesDto{
- UserId: "userId_1642",
- Namespace: "namespace_8685",
- ResourceType: "resourceType_7457",
- }
- response := client.GetUserAuthorizedResources(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_GetPrincipalAuthenticationInfo(t *testing.T) {
- request := dto.GetUserPrincipalAuthenticationInfoDto{
- UserId: "userId_7829",
- }
- response := client.GetPrincipalAuthenticationInfo(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_ListChildrenDepartments(t *testing.T) {
- request := dto.ListChildrenDepartmentsDto{
- DepartmentId: "departmentId_2132",
- OrganizationCode: "organizationCode_5389",
- DepartmentIdType: "departmentIdType_4221",
- }
- response := client.ListChildrenDepartments(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_GetRoleAuthorizedResources(t *testing.T) {
- request := dto.GetRoleAuthorizedResourcesDto{
- Code: "code_20",
- Namespace: "namespace_7340",
- ResourceType: "resourceType_124",
- }
- response := client.GetRoleAuthorizedResources(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_ListDepartmentMembers(t *testing.T) {
- request := dto.ListDepartmentMembersDto{
- OrganizationCode: "organizationCode_5347",
- DepartmentId: "departmentId_2179",
- DepartmentIdType: "departmentIdType_6377",
- Page: 0,
- Limit: 0,
- WithCustomData: false,
- WithIdentities: false,
- WithDepartmentIds: false,
- }
- response := client.ListDepartmentMembers(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_AddDepartmentMembers(t *testing.T) {
- request := dto.AddDepartmentMembersReqDto{
- UserIds: nil,
- OrganizationCode: "organizationCode_8148",
- DepartmentId: "departmentId_6842",
- DepartmentIdType: "",
- }
- response := client.AddDepartmentMembers(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_RemoveDepartmentMembers(t *testing.T) {
- request := dto.RemoveDepartmentMembersReqDto{
- UserIds: nil,
- OrganizationCode: "organizationCode_7362",
- DepartmentId: "departmentId_6293",
- DepartmentIdType: "",
- }
- response := client.RemoveDepartmentMembers(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_ListRoleDepartments(t *testing.T) {
- request := dto.ListRoleDepartmentsDto{
- Code: "code_4901",
- Namespace: "namespace_5508",
- Page: 0,
- Limit: 0,
- }
- response := client.ListRoleDepartments(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_GetParentDepartment(t *testing.T) {
- request := dto.GetParentDepartmentDto{
- OrganizationCode: "organizationCode_2019",
- DepartmentId: "departmentId_3174",
- DepartmentIdType: "departmentIdType_7674",
- }
- response := client.GetParentDepartment(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_CreateResourcesBatch(t *testing.T) {
- request := dto.CreateResourcesBatchDto{
- List: nil,
- Namespace: "namespace_996",
- }
- response := client.CreateResourcesBatch(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_DeleteResourcesBatch(t *testing.T) {
- request := dto.DeleteResourcesBatchDto{
- CodeList: nil,
- Namespace: "namespace_9700",
- }
- response := client.DeleteResourcesBatch(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_ListDepartmentMemberIds(t *testing.T) {
- request := dto.ListDepartmentMemberIdsDto{
- OrganizationCode: "organizationCode_4878",
- DepartmentId: "departmentId_5224",
- DepartmentIdType: "departmentIdType_8269",
- }
- response := client.ListDepartmentMemberIds(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_CreateNamespacesBatch(t *testing.T) {
- request := dto.CreateNamespacesBatchDto{
- List: nil,
- }
- response := client.CreateNamespacesBatch(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_ResetPrincipalAuthenticationInfo(t *testing.T) {
- request := dto.ResetUserPrincipalAuthenticationInfoDto{
- UserId: "userId_7698",
- }
- response := client.ResetPrincipalAuthenticationInfo(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_DeleteNamespacesBatch(t *testing.T) {
- request := dto.DeleteNamespacesBatchDto{
- CodeList: nil,
- }
- response := client.DeleteNamespacesBatch(&request)
- fmt.Println(response)
-
-}
-
-func TestClient_GetTargetAuthorizedResources(t *testing.T) {
- request := dto.GetAuthorizedResourcesDto{
- TargetType: "targetType_9851",
- TargetIdentifier: "targetIdentifier_8941",
- Namespace: "namespace_7824",
- ResourceType: "resourceType_2521",
- }
- response := client.GetTargetAuthorizedResources(&request)
- fmt.Println(response)
-
-}
diff --git a/client/options.go b/client/options.go
deleted file mode 100644
index 2b128e7..0000000
--- a/client/options.go
+++ /dev/null
@@ -1,140 +0,0 @@
-package client
-
-import (
- "authing-go-sdk/constant"
- "authing-go-sdk/dto"
- "authing-go-sdk/util/cache"
- "bytes"
- "encoding/json"
- "github.com/dgrijalva/jwt-go"
- "github.com/valyala/fasthttp"
- "net/http"
- "sync"
- "time"
-)
-
-type Client struct {
- HttpClient *http.Client
- options *ManagementClientOptions
- userPoolId string
-}
-
-type ManagementClientOptions struct {
- AccessKeyId string
- AccessKeySecret string
- TenantId string
- Timeout int
- RequestFrom string
- Lang string
- Host string
- Headers fasthttp.RequestHeader
-}
-
-func NewClient(options *ManagementClientOptions) (*Client, error) {
- if options.Host == "" {
- options.Host = constant.ApiServiceUrl
- }
- c := &Client{
- options: options,
- }
- if c.HttpClient == nil {
- c.HttpClient = &http.Client{}
- _, err := GetAccessToken(c)
- if err != nil {
- return nil, err
- }
- /*src := oauth2.StaticTokenSource(
- &oauth2.Token{AccessToken: accessToken},
- )
- c.HttpClient = oauth2.NewClient(context.Background(), src)*/
- }
- return c, nil
-}
-
-type JwtClaims struct {
- *jwt.StandardClaims
- //用户编号
- UID string
- Username string
-}
-
-func GetAccessToken(client *Client) (string, error) {
- // 从缓存获取token
- cacheToken, b := cache.GetCache(constant.TokenCacheKeyPrefix + client.options.AccessKeyId)
- if b && cacheToken != nil {
- return cacheToken.(string), nil
- }
- // 从服务获取token,加锁
- var mutex sync.Mutex
- mutex.Lock()
- defer mutex.Unlock()
- cacheToken, b = cache.GetCache(constant.TokenCacheKeyPrefix + client.options.AccessKeyId)
- if b && cacheToken != nil {
- return cacheToken.(string), nil
- }
- resp, err := QueryAccessToken(client)
- if err != nil {
- return "", err
- }
- /*var jwtclaim = &JwtClaims{}
- _, err := jwt.ParseWithClaims(resp.Data.AccessToken, &jwtclaim, func(*jwt.Token) (interface{}, error) {
- //得到盐
- return secret, nil
- })*/
- if token, _ := jwt.Parse(resp.Data.AccessToken, nil); token != nil {
- userPoolId := token.Claims.(jwt.MapClaims)["scoped_userpool_id"]
- client.userPoolId = userPoolId.(string)
- }
- //fmt.Println(token)
- //var expire = (*(token.Exp) - time.Now().Unix() - 259200) * int64(time.Second)
- // TODO 时间戳类型转换
- cache.SetCache(constant.TokenCacheKeyPrefix+client.options.AccessKeyId, resp.Data.AccessToken, time.Duration(resp.Data.ExpiresIn*int(time.Second)))
- return resp.Data.AccessToken, nil
-}
-
-func QueryAccessToken(client *Client) (*dto.GetManagementTokenRespDto, error) {
- variables := map[string]interface{}{
- "accessKeyId": client.options.AccessKeyId,
- "accessKeySecret": client.options.AccessKeySecret,
- }
-
- b, err := client.SendHttpRequest("/api/v3/get-management-token", fasthttp.MethodPost, variables)
- if err != nil {
- return nil, err
- }
- var r dto.GetManagementTokenRespDto
- if b != nil {
- json.Unmarshal(b, &r)
- }
- return &r, nil
-}
-
-func (c *Client) SendHttpRequest(url string, method string, variables interface{}) ([]byte, error) {
- var buf bytes.Buffer
- err := json.NewEncoder(&buf).Encode(variables)
- if err != nil {
- return nil, err
- }
- req := fasthttp.AcquireRequest()
-
- req.SetRequestURI(c.options.Host + url)
-
- req.Header.Add("Content-Type", "application/json;charset=UTF-8")
- req.Header.Add("x-authing-app-tenant-id", ""+c.options.TenantId)
- req.Header.Add("x-authing-request-from", c.options.RequestFrom)
- req.Header.Add("x-authing-sdk-version", constant.SdkVersion)
- req.Header.Add("x-authing-lang", c.options.Lang)
- if url != "/api/v3/get-management-token" {
- token, _ := GetAccessToken(c)
- req.Header.Add("Authorization", "Bearer "+token)
- req.Header.Add("x-authing-userpool-id", c.userPoolId)
- }
- req.Header.SetMethod(method)
- req.SetBody(buf.Bytes())
-
- resp := fasthttp.AcquireResponse()
- client := &fasthttp.Client{}
- client.Do(req, resp)
- body := resp.Body()
- return body, err
-}
diff --git a/constant/base_constant.go b/constant/base_constant.go
deleted file mode 100644
index 7f804a2..0000000
--- a/constant/base_constant.go
+++ /dev/null
@@ -1,9 +0,0 @@
-package constant
-
-const (
- ApiServiceUrl = "https://api.authing.cn"
-
- TokenCacheKeyPrefix = "accessKeyId_token_"
-
- SdkVersion = "1.0.0"
-)
diff --git a/dto/AccessTokenDto.go b/dto/AccessTokenDto.go
deleted file mode 100644
index aebf654..0000000
--- a/dto/AccessTokenDto.go
+++ /dev/null
@@ -1,8 +0,0 @@
-package dto
-
-
-type AccessTokenDto struct{
- AccessToken string `json:"access_token"`
- ExpiresIn int `json:"expires_in"`
-}
-
diff --git a/dto/AddDepartmentMembersReqDto.go b/dto/AddDepartmentMembersReqDto.go
deleted file mode 100644
index f47f0f7..0000000
--- a/dto/AddDepartmentMembersReqDto.go
+++ /dev/null
@@ -1,10 +0,0 @@
-package dto
-
-
-type AddDepartmentMembersReqDto struct{
- UserIds []string `json:"userIds"`
- OrganizationCode string `json:"organizationCode"`
- DepartmentId string `json:"departmentId"`
- DepartmentIdType string `json:"departmentIdType,omitempty"`
-}
-
diff --git a/dto/AddGroupMembersReqDto.go b/dto/AddGroupMembersReqDto.go
deleted file mode 100644
index cb4650c..0000000
--- a/dto/AddGroupMembersReqDto.go
+++ /dev/null
@@ -1,8 +0,0 @@
-package dto
-
-
-type AddGroupMembersReqDto struct{
- UserIds []string `json:"userIds"`
- Code string `json:"code"`
-}
-
diff --git a/dto/AppDto.go b/dto/AppDto.go
deleted file mode 100644
index eed201b..0000000
--- a/dto/AppDto.go
+++ /dev/null
@@ -1,11 +0,0 @@
-package dto
-
-
-type AppDto struct{
- AppId string `json:"appId"`
- AppName string `json:"appName"`
- AppLogo string `json:"appLogo"`
- AppLoginUrl string `json:"appLoginUrl"`
- AppDefaultLoginStrategy string `json:"appDefaultLoginStrategy"`
-}
-
diff --git a/dto/AppListRespDto.go b/dto/AppListRespDto.go
deleted file mode 100644
index e02a379..0000000
--- a/dto/AppListRespDto.go
+++ /dev/null
@@ -1,10 +0,0 @@
-package dto
-
-
-type AppListRespDto struct{
- StatusCode int `json:"statusCode"`
- Message string `json:"message"`
- ApiCode int `json:"apiCode,omitempty"`
- Data []AppDto `json:"data"`
-}
-
diff --git a/dto/ArchivedUsersListPagingDto.go b/dto/ArchivedUsersListPagingDto.go
deleted file mode 100644
index 83400ae..0000000
--- a/dto/ArchivedUsersListPagingDto.go
+++ /dev/null
@@ -1,8 +0,0 @@
-package dto
-
-
-type ArchivedUsersListPagingDto struct{
- TotalCount int `json:"totalCount"`
- List []ListArchivedUsersRespDto `json:"list"`
-}
-
diff --git a/dto/AssignRoleBatchDto.go b/dto/AssignRoleBatchDto.go
deleted file mode 100644
index 728dbb7..0000000
--- a/dto/AssignRoleBatchDto.go
+++ /dev/null
@@ -1,8 +0,0 @@
-package dto
-
-
-type AssignRoleBatchDto struct{
- Targets []TargetDto `json:"targets"`
- Roles []RoleCodeDto `json:"roles"`
-}
-
diff --git a/dto/AssignRoleDto.go b/dto/AssignRoleDto.go
deleted file mode 100644
index 6ae487f..0000000
--- a/dto/AssignRoleDto.go
+++ /dev/null
@@ -1,9 +0,0 @@
-package dto
-
-
-type AssignRoleDto struct{
- Targets []TargetDto `json:"targets"`
- Code string `json:"code"`
- Namespace string `json:"namespace,omitempty"`
-}
-
diff --git a/dto/AuthorizeResourceItem.go b/dto/AuthorizeResourceItem.go
deleted file mode 100644
index efaa7cf..0000000
--- a/dto/AuthorizeResourceItem.go
+++ /dev/null
@@ -1,9 +0,0 @@
-package dto
-
-
-type AuthorizeResourceItem struct{
- TargetType string `json:"targetType"`
- TargetIdentifiers []string `json:"targetIdentifiers"`
- Resources []ResourceItemDto `json:"resources"`
-}
-
diff --git a/dto/AuthorizeResourcesDto.go b/dto/AuthorizeResourcesDto.go
deleted file mode 100644
index 833ee71..0000000
--- a/dto/AuthorizeResourcesDto.go
+++ /dev/null
@@ -1,8 +0,0 @@
-package dto
-
-
-type AuthorizeResourcesDto struct{
- List []AuthorizeResourceItem `json:"list"`
- Namespace string `json:"namespace,omitempty"`
-}
-
diff --git a/dto/AuthorizedResourceDto.go b/dto/AuthorizedResourceDto.go
deleted file mode 100644
index 983e827..0000000
--- a/dto/AuthorizedResourceDto.go
+++ /dev/null
@@ -1,10 +0,0 @@
-package dto
-
-
-type AuthorizedResourceDto struct{
- ResourceCode string `json:"resourceCode"`
- ResourceType string `json:"resourceType,omitempty"`
- Actions []string `json:"actions,omitempty"`
- ApiIdentifier string `json:"apiIdentifier,omitempty"`
-}
-
diff --git a/dto/AuthorizedResourceListRespDto.go b/dto/AuthorizedResourceListRespDto.go
deleted file mode 100644
index 8c892ba..0000000
--- a/dto/AuthorizedResourceListRespDto.go
+++ /dev/null
@@ -1,10 +0,0 @@
-package dto
-
-
-type AuthorizedResourceListRespDto struct{
- StatusCode int `json:"statusCode"`
- Message string `json:"message"`
- ApiCode int `json:"apiCode,omitempty"`
- Data []AuthorizedResourceDto `json:"data"`
-}
-
diff --git a/dto/AuthorizedResourcePaginatedRespDto.go b/dto/AuthorizedResourcePaginatedRespDto.go
deleted file mode 100644
index 61d8019..0000000
--- a/dto/AuthorizedResourcePaginatedRespDto.go
+++ /dev/null
@@ -1,10 +0,0 @@
-package dto
-
-
-type AuthorizedResourcePaginatedRespDto struct{
- StatusCode int `json:"statusCode"`
- Message string `json:"message"`
- ApiCode int `json:"apiCode,omitempty"`
- Data AuthorizedResourcePagingDto `json:"data"`
-}
-
diff --git a/dto/AuthorizedResourcePagingDto.go b/dto/AuthorizedResourcePagingDto.go
deleted file mode 100644
index 1f9bb65..0000000
--- a/dto/AuthorizedResourcePagingDto.go
+++ /dev/null
@@ -1,8 +0,0 @@
-package dto
-
-
-type AuthorizedResourcePagingDto struct{
- TotalCount int `json:"totalCount"`
- List []AuthorizedResourceDto `json:"list"`
-}
-
diff --git a/dto/CommonResponseDto.go b/dto/CommonResponseDto.go
deleted file mode 100644
index 4358070..0000000
--- a/dto/CommonResponseDto.go
+++ /dev/null
@@ -1,9 +0,0 @@
-package dto
-
-
-type CommonResponseDto struct{
- StatusCode int `json:"statusCode"`
- Message string `json:"message"`
- ApiCode int `json:"apiCode,omitempty"`
-}
-
diff --git a/dto/CreateDepartmentReqDto.go b/dto/CreateDepartmentReqDto.go
deleted file mode 100644
index 01b7f94..0000000
--- a/dto/CreateDepartmentReqDto.go
+++ /dev/null
@@ -1,13 +0,0 @@
-package dto
-
-
-type CreateDepartmentReqDto struct{
- OrganizationCode string `json:"organizationCode"`
- Name string `json:"name"`
- ParentDepartmentId string `json:"parentDepartmentId"`
- OpenDepartmentId string `json:"openDepartmentId,omitempty"`
- Code string `json:"code,omitempty"`
- LeaderUserId string `json:"leaderUserId,omitempty"`
- DepartmentIdType string `json:"departmentIdType,omitempty"`
-}
-
diff --git a/dto/CreateExtIdpConnDto.go b/dto/CreateExtIdpConnDto.go
deleted file mode 100644
index b57e83c..0000000
--- a/dto/CreateExtIdpConnDto.go
+++ /dev/null
@@ -1,13 +0,0 @@
-package dto
-
-
-type CreateExtIdpConnDto struct{
- Fields interface{} `json:"fields"`
- DisplayName string `json:"displayName"`
- Identifier string `json:"identifier"`
- Type string `json:"type"`
- ExtIdpId string `json:"extIdpId"`
- LoginOnly bool `json:"loginOnly,omitempty"`
- Logo string `json:"logo,omitempty"`
-}
-
diff --git a/dto/CreateExtIdpDto.go b/dto/CreateExtIdpDto.go
deleted file mode 100644
index 38e7cf7..0000000
--- a/dto/CreateExtIdpDto.go
+++ /dev/null
@@ -1,9 +0,0 @@
-package dto
-
-
-type CreateExtIdpDto struct{
- Type string `json:"type"`
- Name string `json:"name"`
- TenantId string `json:"tenantId,omitempty"`
-}
-
diff --git a/dto/CreateGroupBatchReqDto.go b/dto/CreateGroupBatchReqDto.go
deleted file mode 100644
index 95b9b1a..0000000
--- a/dto/CreateGroupBatchReqDto.go
+++ /dev/null
@@ -1,7 +0,0 @@
-package dto
-
-
-type CreateGroupBatchReqDto struct{
- List []CreateGroupReqDto `json:"list"`
-}
-
diff --git a/dto/CreateGroupReqDto.go b/dto/CreateGroupReqDto.go
deleted file mode 100644
index 48fc390..0000000
--- a/dto/CreateGroupReqDto.go
+++ /dev/null
@@ -1,9 +0,0 @@
-package dto
-
-
-type CreateGroupReqDto struct{
- Description string `json:"description"`
- Name string `json:"name"`
- Code string `json:"code"`
-}
-
diff --git a/dto/CreateIdentityDto.go b/dto/CreateIdentityDto.go
deleted file mode 100644
index 61db43d..0000000
--- a/dto/CreateIdentityDto.go
+++ /dev/null
@@ -1,10 +0,0 @@
-package dto
-
-
-type CreateIdentityDto struct{
- ExtIdpId string `json:"extIdpId"`
- Provider string `json:"provider"`
- Type string `json:"type"`
- UserIdInIdp string `json:"userIdInIdp"`
-}
-
diff --git a/dto/CreateNamespaceDto.go b/dto/CreateNamespaceDto.go
deleted file mode 100644
index 78bcaff..0000000
--- a/dto/CreateNamespaceDto.go
+++ /dev/null
@@ -1,9 +0,0 @@
-package dto
-
-
-type CreateNamespaceDto struct{
- Code string `json:"code"`
- Name string `json:"name,omitempty"`
- Description string `json:"description,omitempty"`
-}
-
diff --git a/dto/CreateNamespacesBatchDto.go b/dto/CreateNamespacesBatchDto.go
deleted file mode 100644
index cbe90bb..0000000
--- a/dto/CreateNamespacesBatchDto.go
+++ /dev/null
@@ -1,7 +0,0 @@
-package dto
-
-
-type CreateNamespacesBatchDto struct{
- List []CreateNamespacesBatchItemDto `json:"list"`
-}
-
diff --git a/dto/CreateNamespacesBatchItemDto.go b/dto/CreateNamespacesBatchItemDto.go
deleted file mode 100644
index 9f42a1a..0000000
--- a/dto/CreateNamespacesBatchItemDto.go
+++ /dev/null
@@ -1,9 +0,0 @@
-package dto
-
-
-type CreateNamespacesBatchItemDto struct{
- Code string `json:"code"`
- Name string `json:"name,omitempty"`
- Description string `json:"description,omitempty"`
-}
-
diff --git a/dto/CreateOrganizationReqDto.go b/dto/CreateOrganizationReqDto.go
deleted file mode 100644
index 9a58bea..0000000
--- a/dto/CreateOrganizationReqDto.go
+++ /dev/null
@@ -1,9 +0,0 @@
-package dto
-
-
-type CreateOrganizationReqDto struct{
- OrganizationName string `json:"organizationName"`
- OrganizationCode string `json:"organizationCode"`
- OpenDepartmentId string `json:"openDepartmentId,omitempty"`
-}
-
diff --git a/dto/CreateResourceBatchItemDto.go b/dto/CreateResourceBatchItemDto.go
deleted file mode 100644
index b2d52ea..0000000
--- a/dto/CreateResourceBatchItemDto.go
+++ /dev/null
@@ -1,11 +0,0 @@
-package dto
-
-
-type CreateResourceBatchItemDto struct{
- Code string `json:"code"`
- Description string `json:"description,omitempty"`
- Type string `json:"type"`
- Actions []ResourceAction `json:"actions,omitempty"`
- ApiIdentifier string `json:"apiIdentifier,omitempty"`
-}
-
diff --git a/dto/CreateResourceDto.go b/dto/CreateResourceDto.go
deleted file mode 100644
index 2916e74..0000000
--- a/dto/CreateResourceDto.go
+++ /dev/null
@@ -1,12 +0,0 @@
-package dto
-
-
-type CreateResourceDto struct{
- Type string `json:"type"`
- Code string `json:"code"`
- Description string `json:"description,omitempty"`
- Actions []ResourceAction `json:"actions,omitempty"`
- ApiIdentifier string `json:"apiIdentifier,omitempty"`
- Namespace string `json:"namespace,omitempty"`
-}
-
diff --git a/dto/CreateResourcesBatchDto.go b/dto/CreateResourcesBatchDto.go
deleted file mode 100644
index df4cefa..0000000
--- a/dto/CreateResourcesBatchDto.go
+++ /dev/null
@@ -1,8 +0,0 @@
-package dto
-
-
-type CreateResourcesBatchDto struct{
- List []CreateResourceBatchItemDto `json:"list"`
- Namespace string `json:"namespace,omitempty"`
-}
-
diff --git a/dto/CreateRoleDto.go b/dto/CreateRoleDto.go
deleted file mode 100644
index fc7e760..0000000
--- a/dto/CreateRoleDto.go
+++ /dev/null
@@ -1,9 +0,0 @@
-package dto
-
-
-type CreateRoleDto struct{
- Code string `json:"code"`
- Namespace string `json:"namespace,omitempty"`
- Description string `json:"description,omitempty"`
-}
-
diff --git a/dto/CreateRolesBatch.go b/dto/CreateRolesBatch.go
deleted file mode 100644
index 34f5107..0000000
--- a/dto/CreateRolesBatch.go
+++ /dev/null
@@ -1,7 +0,0 @@
-package dto
-
-
-type CreateRolesBatch struct{
- List []RoleListItem `json:"list"`
-}
-
diff --git a/dto/CreateUserBatchReqDto.go b/dto/CreateUserBatchReqDto.go
deleted file mode 100644
index eaa7e1c..0000000
--- a/dto/CreateUserBatchReqDto.go
+++ /dev/null
@@ -1,8 +0,0 @@
-package dto
-
-
-type CreateUserBatchReqDto struct{
- List []CreateUserInfoDto `json:"list"`
- Options CreateUserOptionsDto `json:"options,omitempty"`
-}
-
diff --git a/dto/CreateUserInfoDto.go b/dto/CreateUserInfoDto.go
deleted file mode 100644
index 6b45b6a..0000000
--- a/dto/CreateUserInfoDto.go
+++ /dev/null
@@ -1,31 +0,0 @@
-package dto
-
-
-type CreateUserInfoDto struct{
- Status string `json:"status,omitempty"`
- Email string `json:"email,omitempty"`
- PasswordEncryptType string `json:"passwordEncryptType,omitempty"`
- Phone string `json:"phone,omitempty"`
- PhoneCountryCode string `json:"phoneCountryCode,omitempty"`
- Username string `json:"username,omitempty"`
- Name string `json:"name,omitempty"`
- Nickname string `json:"nickname,omitempty"`
- Photo string `json:"photo,omitempty"`
- Gender string `json:"gender,omitempty"`
- EmailVerified bool `json:"emailVerified,omitempty"`
- PhoneVerified bool `json:"phoneVerified,omitempty"`
- Birthdate string `json:"birthdate,omitempty"`
- Country string `json:"country,omitempty"`
- Province string `json:"province,omitempty"`
- City string `json:"city,omitempty"`
- Address string `json:"address,omitempty"`
- StreetAddress string `json:"streetAddress,omitempty"`
- PostalCode string `json:"postalCode,omitempty"`
- ExternalId string `json:"externalId,omitempty"`
- DepartmentIds []string `json:"departmentIds,omitempty"`
- CustomData interface{} `json:"customData,omitempty"`
- Password string `json:"password,omitempty"`
- TenantIds []string `json:"tenantIds,omitempty"`
- Identities []CreateIdentityDto `json:"identities,omitempty"`
-}
-
diff --git a/dto/CreateUserOptionsDto.go b/dto/CreateUserOptionsDto.go
deleted file mode 100644
index a3d240a..0000000
--- a/dto/CreateUserOptionsDto.go
+++ /dev/null
@@ -1,9 +0,0 @@
-package dto
-
-
-type CreateUserOptionsDto struct{
- KeepPassword bool `json:"keepPassword,omitempty"`
- ResetPasswordOnFirstLogin bool `json:"resetPasswordOnFirstLogin,omitempty"`
- DepartmentIdType string `json:"departmentIdType,omitempty"`
-}
-
diff --git a/dto/CreateUserReqDto.go b/dto/CreateUserReqDto.go
deleted file mode 100644
index a9fb828..0000000
--- a/dto/CreateUserReqDto.go
+++ /dev/null
@@ -1,32 +0,0 @@
-package dto
-
-
-type CreateUserReqDto struct{
- Status string `json:"status,omitempty"`
- Email string `json:"email,omitempty"`
- PasswordEncryptType string `json:"passwordEncryptType,omitempty"`
- Phone string `json:"phone,omitempty"`
- PhoneCountryCode string `json:"phoneCountryCode,omitempty"`
- Username string `json:"username,omitempty"`
- Name string `json:"name,omitempty"`
- Nickname string `json:"nickname,omitempty"`
- Photo string `json:"photo,omitempty"`
- Gender string `json:"gender,omitempty"`
- EmailVerified bool `json:"emailVerified,omitempty"`
- PhoneVerified bool `json:"phoneVerified,omitempty"`
- Birthdate string `json:"birthdate,omitempty"`
- Country string `json:"country,omitempty"`
- Province string `json:"province,omitempty"`
- City string `json:"city,omitempty"`
- Address string `json:"address,omitempty"`
- StreetAddress string `json:"streetAddress,omitempty"`
- PostalCode string `json:"postalCode,omitempty"`
- ExternalId string `json:"externalId,omitempty"`
- DepartmentIds []string `json:"departmentIds,omitempty"`
- CustomData interface{} `json:"customData,omitempty"`
- Password string `json:"password,omitempty"`
- TenantIds []string `json:"tenantIds,omitempty"`
- Identities []CreateIdentityDto `json:"identities,omitempty"`
- Options CreateUserOptionsDto `json:"options,omitempty"`
-}
-
diff --git a/dto/CustomFieldDto.go b/dto/CustomFieldDto.go
deleted file mode 100644
index 1b5656f..0000000
--- a/dto/CustomFieldDto.go
+++ /dev/null
@@ -1,14 +0,0 @@
-package dto
-
-
-type CustomFieldDto struct{
- TargetType string `json:"targetType"`
- CreatedAt string `json:"createdAt"`
- DataType string `json:"dataType"`
- Key string `json:"key"`
- Label string `json:"label"`
- Description string `json:"description,omitempty"`
- Encrypted bool `json:"encrypted,omitempty"`
- Options []CustomFieldSelectOption `json:"options,omitempty"`
-}
-
diff --git a/dto/CustomFieldListRespDto.go b/dto/CustomFieldListRespDto.go
deleted file mode 100644
index f4cccc2..0000000
--- a/dto/CustomFieldListRespDto.go
+++ /dev/null
@@ -1,10 +0,0 @@
-package dto
-
-
-type CustomFieldListRespDto struct{
- StatusCode int `json:"statusCode"`
- Message string `json:"message"`
- ApiCode int `json:"apiCode,omitempty"`
- Data []CustomFieldDto `json:"data"`
-}
-
diff --git a/dto/CustomFieldSelectOption.go b/dto/CustomFieldSelectOption.go
deleted file mode 100644
index 209d3fe..0000000
--- a/dto/CustomFieldSelectOption.go
+++ /dev/null
@@ -1,8 +0,0 @@
-package dto
-
-
-type CustomFieldSelectOption struct{
- Value string `json:"value"`
- Label string `json:"label"`
-}
-
diff --git a/dto/DeleteDepartmentReqDto.go b/dto/DeleteDepartmentReqDto.go
deleted file mode 100644
index f4f24fe..0000000
--- a/dto/DeleteDepartmentReqDto.go
+++ /dev/null
@@ -1,9 +0,0 @@
-package dto
-
-
-type DeleteDepartmentReqDto struct{
- OrganizationCode string `json:"organizationCode"`
- DepartmentId string `json:"departmentId"`
- DepartmentIdType string `json:"departmentIdType,omitempty"`
-}
-
diff --git a/dto/DeleteExtIdpConnDto.go b/dto/DeleteExtIdpConnDto.go
deleted file mode 100644
index 1e653cc..0000000
--- a/dto/DeleteExtIdpConnDto.go
+++ /dev/null
@@ -1,7 +0,0 @@
-package dto
-
-
-type DeleteExtIdpConnDto struct{
- Id string `json:"id"`
-}
-
diff --git a/dto/DeleteExtIdpDto.go b/dto/DeleteExtIdpDto.go
deleted file mode 100644
index 6524371..0000000
--- a/dto/DeleteExtIdpDto.go
+++ /dev/null
@@ -1,7 +0,0 @@
-package dto
-
-
-type DeleteExtIdpDto struct{
- Id string `json:"id"`
-}
-
diff --git a/dto/DeleteGroupsReqDto.go b/dto/DeleteGroupsReqDto.go
deleted file mode 100644
index bfc629a..0000000
--- a/dto/DeleteGroupsReqDto.go
+++ /dev/null
@@ -1,7 +0,0 @@
-package dto
-
-
-type DeleteGroupsReqDto struct{
- CodeList []string `json:"codeList"`
-}
-
diff --git a/dto/DeleteNamespaceDto.go b/dto/DeleteNamespaceDto.go
deleted file mode 100644
index 6ed16e5..0000000
--- a/dto/DeleteNamespaceDto.go
+++ /dev/null
@@ -1,7 +0,0 @@
-package dto
-
-
-type DeleteNamespaceDto struct{
- Code string `json:"code"`
-}
-
diff --git a/dto/DeleteNamespacesBatchDto.go b/dto/DeleteNamespacesBatchDto.go
deleted file mode 100644
index c71e288..0000000
--- a/dto/DeleteNamespacesBatchDto.go
+++ /dev/null
@@ -1,7 +0,0 @@
-package dto
-
-
-type DeleteNamespacesBatchDto struct{
- CodeList []string `json:"codeList"`
-}
-
diff --git a/dto/DeleteOrganizationReqDto.go b/dto/DeleteOrganizationReqDto.go
deleted file mode 100644
index 2bad27e..0000000
--- a/dto/DeleteOrganizationReqDto.go
+++ /dev/null
@@ -1,7 +0,0 @@
-package dto
-
-
-type DeleteOrganizationReqDto struct{
- OrganizationCode string `json:"organizationCode"`
-}
-
diff --git a/dto/DeleteResourceDto.go b/dto/DeleteResourceDto.go
deleted file mode 100644
index 29a323e..0000000
--- a/dto/DeleteResourceDto.go
+++ /dev/null
@@ -1,8 +0,0 @@
-package dto
-
-
-type DeleteResourceDto struct{
- Code string `json:"code"`
- Namespace string `json:"namespace,omitempty"`
-}
-
diff --git a/dto/DeleteResourcesBatchDto.go b/dto/DeleteResourcesBatchDto.go
deleted file mode 100644
index baa061a..0000000
--- a/dto/DeleteResourcesBatchDto.go
+++ /dev/null
@@ -1,8 +0,0 @@
-package dto
-
-
-type DeleteResourcesBatchDto struct{
- CodeList []string `json:"codeList"`
- Namespace string `json:"namespace,omitempty"`
-}
-
diff --git a/dto/DeleteRoleDto.go b/dto/DeleteRoleDto.go
deleted file mode 100644
index 2d6e26b..0000000
--- a/dto/DeleteRoleDto.go
+++ /dev/null
@@ -1,8 +0,0 @@
-package dto
-
-
-type DeleteRoleDto struct{
- CodeList []string `json:"codeList"`
- Namespace string `json:"namespace,omitempty"`
-}
-
diff --git a/dto/DeleteUsersBatchDto.go b/dto/DeleteUsersBatchDto.go
deleted file mode 100644
index 9e45b14..0000000
--- a/dto/DeleteUsersBatchDto.go
+++ /dev/null
@@ -1,7 +0,0 @@
-package dto
-
-
-type DeleteUsersBatchDto struct{
- UserIds []string `json:"userIds"`
-}
-
diff --git a/dto/DepartmentDto.go b/dto/DepartmentDto.go
deleted file mode 100644
index c1965ab..0000000
--- a/dto/DepartmentDto.go
+++ /dev/null
@@ -1,16 +0,0 @@
-package dto
-
-
-type DepartmentDto struct{
- DepartmentId string `json:"departmentId"`
- OpenDepartmentId string `json:"openDepartmentId,omitempty"`
- ParentDepartmentId string `json:"parentDepartmentId"`
- ParentOpenDepartmentId string `json:"parentOpenDepartmentId,omitempty"`
- Name string `json:"name"`
- Description string `json:"description"`
- Code string `json:"code,omitempty"`
- LeaderUserId string `json:"leaderUserId,omitempty"`
- MembersCount int `json:"membersCount"`
- HasChildren bool `json:"hasChildren"`
-}
-
diff --git a/dto/DepartmentListRespDto.go b/dto/DepartmentListRespDto.go
deleted file mode 100644
index d6c55d7..0000000
--- a/dto/DepartmentListRespDto.go
+++ /dev/null
@@ -1,10 +0,0 @@
-package dto
-
-
-type DepartmentListRespDto struct{
- StatusCode int `json:"statusCode"`
- Message string `json:"message"`
- ApiCode int `json:"apiCode,omitempty"`
- Data []DepartmentDto `json:"data"`
-}
-
diff --git a/dto/DepartmentPaginatedRespDto.go b/dto/DepartmentPaginatedRespDto.go
deleted file mode 100644
index a863595..0000000
--- a/dto/DepartmentPaginatedRespDto.go
+++ /dev/null
@@ -1,10 +0,0 @@
-package dto
-
-
-type DepartmentPaginatedRespDto struct{
- StatusCode int `json:"statusCode"`
- Message string `json:"message"`
- ApiCode int `json:"apiCode,omitempty"`
- Data DepartmentPagingDto `json:"data"`
-}
-
diff --git a/dto/DepartmentPagingDto.go b/dto/DepartmentPagingDto.go
deleted file mode 100644
index c1c3655..0000000
--- a/dto/DepartmentPagingDto.go
+++ /dev/null
@@ -1,8 +0,0 @@
-package dto
-
-
-type DepartmentPagingDto struct{
- TotalCount bool `json:"totalCount"`
- List []DepartmentDto `json:"list"`
-}
-
diff --git a/dto/DepartmentSingleRespDto.go b/dto/DepartmentSingleRespDto.go
deleted file mode 100644
index 92ed2c0..0000000
--- a/dto/DepartmentSingleRespDto.go
+++ /dev/null
@@ -1,10 +0,0 @@
-package dto
-
-
-type DepartmentSingleRespDto struct{
- StatusCode int `json:"statusCode"`
- Message string `json:"message"`
- ApiCode int `json:"apiCode,omitempty"`
- Data DepartmentDto `json:"data"`
-}
-
diff --git a/dto/EnableExtIdpConnDto.go b/dto/EnableExtIdpConnDto.go
deleted file mode 100644
index 17d9031..0000000
--- a/dto/EnableExtIdpConnDto.go
+++ /dev/null
@@ -1,10 +0,0 @@
-package dto
-
-
-type EnableExtIdpConnDto struct{
- AppId string `json:"appId"`
- Enabled bool `json:"enabled"`
- Id string `json:"id"`
- TenantId string `json:"tenantId,omitempty"`
-}
-
diff --git a/dto/ExtIdpConnDetailSingleRespDto.go b/dto/ExtIdpConnDetailSingleRespDto.go
deleted file mode 100644
index 2b65e9e..0000000
--- a/dto/ExtIdpConnDetailSingleRespDto.go
+++ /dev/null
@@ -1,10 +0,0 @@
-package dto
-
-
-type ExtIdpConnDetailSingleRespDto struct{
- StatusCode int `json:"statusCode"`
- Message string `json:"message"`
- ApiCode int `json:"apiCode,omitempty"`
- Data ExtIdpConnDto `json:"data"`
-}
-
diff --git a/dto/ExtIdpConnDto.go b/dto/ExtIdpConnDto.go
deleted file mode 100644
index 3b13461..0000000
--- a/dto/ExtIdpConnDto.go
+++ /dev/null
@@ -1,11 +0,0 @@
-package dto
-
-
-type ExtIdpConnDto struct{
- Id string `json:"id"`
- Type string `json:"type"`
- Logo string `json:"logo"`
- Identifier string `json:"identifier,omitempty"`
- DisplayName string `json:"displayName,omitempty"`
-}
-
diff --git a/dto/ExtIdpDetail.go b/dto/ExtIdpDetail.go
deleted file mode 100644
index 7d90532..0000000
--- a/dto/ExtIdpDetail.go
+++ /dev/null
@@ -1,12 +0,0 @@
-package dto
-
-
-type ExtIdpDetail struct{
- Id string `json:"id"`
- Name string `json:"name"`
- TenantId string `json:"tenantId,omitempty"`
- Type string `json:"type"`
- Connections interface{} `json:"connections"`
- AutoJoin bool `json:"autoJoin"`
-}
-
diff --git a/dto/ExtIdpDetailSingleRespDto.go b/dto/ExtIdpDetailSingleRespDto.go
deleted file mode 100644
index dab2dc4..0000000
--- a/dto/ExtIdpDetailSingleRespDto.go
+++ /dev/null
@@ -1,10 +0,0 @@
-package dto
-
-
-type ExtIdpDetailSingleRespDto struct{
- StatusCode int `json:"statusCode"`
- Message string `json:"message"`
- ApiCode int `json:"apiCode,omitempty"`
- Data ExtIdpDetail `json:"data"`
-}
-
diff --git a/dto/ExtIdpDto.go b/dto/ExtIdpDto.go
deleted file mode 100644
index d13ca84..0000000
--- a/dto/ExtIdpDto.go
+++ /dev/null
@@ -1,10 +0,0 @@
-package dto
-
-
-type ExtIdpDto struct{
- Id string `json:"id"`
- Name string `json:"name"`
- TenantId string `json:"tenantId,omitempty"`
- Type string `json:"type"`
-}
-
diff --git a/dto/ExtIdpListPaginatedRespDto.go b/dto/ExtIdpListPaginatedRespDto.go
deleted file mode 100644
index e8b941e..0000000
--- a/dto/ExtIdpListPaginatedRespDto.go
+++ /dev/null
@@ -1,10 +0,0 @@
-package dto
-
-
-type ExtIdpListPaginatedRespDto struct{
- StatusCode int `json:"statusCode"`
- Message string `json:"message"`
- ApiCode int `json:"apiCode,omitempty"`
- Data ExtIdpListPagingDto `json:"data"`
-}
-
diff --git a/dto/ExtIdpListPagingDto.go b/dto/ExtIdpListPagingDto.go
deleted file mode 100644
index 28f57bb..0000000
--- a/dto/ExtIdpListPagingDto.go
+++ /dev/null
@@ -1,8 +0,0 @@
-package dto
-
-
-type ExtIdpListPagingDto struct{
- TotalCount int `json:"totalCount"`
- List []ExtIdpDto `json:"list"`
-}
-
diff --git a/dto/ExtIdpSingleRespDto.go b/dto/ExtIdpSingleRespDto.go
deleted file mode 100644
index 21ea871..0000000
--- a/dto/ExtIdpSingleRespDto.go
+++ /dev/null
@@ -1,10 +0,0 @@
-package dto
-
-
-type ExtIdpSingleRespDto struct{
- StatusCode int `json:"statusCode"`
- Message string `json:"message"`
- ApiCode int `json:"apiCode,omitempty"`
- Data ExtIdpDto `json:"data"`
-}
-
diff --git a/dto/GetAuthorizedResourcesDto.go b/dto/GetAuthorizedResourcesDto.go
deleted file mode 100644
index f2d0f3f..0000000
--- a/dto/GetAuthorizedResourcesDto.go
+++ /dev/null
@@ -1,10 +0,0 @@
-package dto
-
-
-type GetAuthorizedResourcesDto struct{
- TargetType string `json:"target_type,omitempty"`
- TargetIdentifier string `json:"target_identifier,omitempty"`
- Namespace string `json:"namespace,omitempty"`
- ResourceType string `json:"resource_type,omitempty"`
-}
-
diff --git a/dto/GetCustomDataDto.go b/dto/GetCustomDataDto.go
deleted file mode 100644
index b96134b..0000000
--- a/dto/GetCustomDataDto.go
+++ /dev/null
@@ -1,9 +0,0 @@
-package dto
-
-
-type GetCustomDataDto struct{
- TargetType string `json:"target_type,omitempty"`
- TargetIdentifier string `json:"target_identifier,omitempty"`
- Namespace string `json:"namespace,omitempty"`
-}
-
diff --git a/dto/GetCustomDataRespDto.go b/dto/GetCustomDataRespDto.go
deleted file mode 100644
index 27eee53..0000000
--- a/dto/GetCustomDataRespDto.go
+++ /dev/null
@@ -1,10 +0,0 @@
-package dto
-
-
-type GetCustomDataRespDto struct{
- StatusCode int `json:"statusCode"`
- Message string `json:"message"`
- ApiCode int `json:"apiCode,omitempty"`
- Data interface{} `json:"data"`
-}
-
diff --git a/dto/GetCustomFieldsDto.go b/dto/GetCustomFieldsDto.go
deleted file mode 100644
index 3c99b37..0000000
--- a/dto/GetCustomFieldsDto.go
+++ /dev/null
@@ -1,7 +0,0 @@
-package dto
-
-
-type GetCustomFieldsDto struct{
- TargetType string `json:"target_type,omitempty"`
-}
-
diff --git a/dto/GetDepartmentDto.go b/dto/GetDepartmentDto.go
deleted file mode 100644
index 5671877..0000000
--- a/dto/GetDepartmentDto.go
+++ /dev/null
@@ -1,9 +0,0 @@
-package dto
-
-
-type GetDepartmentDto struct{
- OrganizationCode string `json:"organization_code,omitempty"`
- DepartmentId string `json:"department_id,omitempty"`
- DepartmentIdType string `json:"department_id_type,omitempty"`
-}
-
diff --git a/dto/GetExtIdpDto.go b/dto/GetExtIdpDto.go
deleted file mode 100644
index 8fa05fa..0000000
--- a/dto/GetExtIdpDto.go
+++ /dev/null
@@ -1,8 +0,0 @@
-package dto
-
-
-type GetExtIdpDto struct{
- Id string `json:"id,omitempty"`
- TenantId string `json:"tenant_id,omitempty"`
-}
-
diff --git a/dto/GetGroupAuthorizedResourcesDto.go b/dto/GetGroupAuthorizedResourcesDto.go
deleted file mode 100644
index 5e73a0e..0000000
--- a/dto/GetGroupAuthorizedResourcesDto.go
+++ /dev/null
@@ -1,9 +0,0 @@
-package dto
-
-
-type GetGroupAuthorizedResourcesDto struct{
- Code string `json:"code,omitempty"`
- Namespace string `json:"namespace,omitempty"`
- ResourceType string `json:"resource_type,omitempty"`
-}
-
diff --git a/dto/GetGroupDto.go b/dto/GetGroupDto.go
deleted file mode 100644
index 140169d..0000000
--- a/dto/GetGroupDto.go
+++ /dev/null
@@ -1,7 +0,0 @@
-package dto
-
-
-type GetGroupDto struct{
- Code string `json:"code,omitempty"`
-}
-
diff --git a/dto/GetManagementAccessTokenDto.go b/dto/GetManagementAccessTokenDto.go
deleted file mode 100644
index 31fabe3..0000000
--- a/dto/GetManagementAccessTokenDto.go
+++ /dev/null
@@ -1,8 +0,0 @@
-package dto
-
-
-type GetManagementAccessTokenDto struct{
- AccessKeySecret string `json:"accessKeySecret"`
- AccessKeyId string `json:"accessKeyId"`
-}
-
diff --git a/dto/GetManagementTokenRespDto.go b/dto/GetManagementTokenRespDto.go
deleted file mode 100644
index 5a893bf..0000000
--- a/dto/GetManagementTokenRespDto.go
+++ /dev/null
@@ -1,10 +0,0 @@
-package dto
-
-
-type GetManagementTokenRespDto struct{
- StatusCode int `json:"statusCode"`
- Message string `json:"message"`
- ApiCode int `json:"apiCode,omitempty"`
- Data AccessTokenDto `json:"data"`
-}
-
diff --git a/dto/GetNamespaceDto.go b/dto/GetNamespaceDto.go
deleted file mode 100644
index 353a56d..0000000
--- a/dto/GetNamespaceDto.go
+++ /dev/null
@@ -1,7 +0,0 @@
-package dto
-
-
-type GetNamespaceDto struct{
- Code string `json:"code,omitempty"`
-}
-
diff --git a/dto/GetNamespacesBatchDto.go b/dto/GetNamespacesBatchDto.go
deleted file mode 100644
index 8a819b4..0000000
--- a/dto/GetNamespacesBatchDto.go
+++ /dev/null
@@ -1,7 +0,0 @@
-package dto
-
-
-type GetNamespacesBatchDto struct{
- CodeList string `json:"code_list,omitempty"`
-}
-
diff --git a/dto/GetParentDepartmentDto.go b/dto/GetParentDepartmentDto.go
deleted file mode 100644
index 999ea90..0000000
--- a/dto/GetParentDepartmentDto.go
+++ /dev/null
@@ -1,9 +0,0 @@
-package dto
-
-
-type GetParentDepartmentDto struct{
- OrganizationCode string `json:"organization_code,omitempty"`
- DepartmentId string `json:"department_id,omitempty"`
- DepartmentIdType string `json:"department_id_type,omitempty"`
-}
-
diff --git a/dto/GetResourceDto.go b/dto/GetResourceDto.go
deleted file mode 100644
index 50702e4..0000000
--- a/dto/GetResourceDto.go
+++ /dev/null
@@ -1,8 +0,0 @@
-package dto
-
-
-type GetResourceDto struct{
- Code string `json:"code,omitempty"`
- Namespace string `json:"namespace,omitempty"`
-}
-
diff --git a/dto/GetResourcesBatchDto.go b/dto/GetResourcesBatchDto.go
deleted file mode 100644
index 0599eab..0000000
--- a/dto/GetResourcesBatchDto.go
+++ /dev/null
@@ -1,8 +0,0 @@
-package dto
-
-
-type GetResourcesBatchDto struct{
- CodeList string `json:"code_list,omitempty"`
- Namespace string `json:"namespace,omitempty"`
-}
-
diff --git a/dto/GetRoleAuthorizedResourcesDto.go b/dto/GetRoleAuthorizedResourcesDto.go
deleted file mode 100644
index b5e4ad0..0000000
--- a/dto/GetRoleAuthorizedResourcesDto.go
+++ /dev/null
@@ -1,9 +0,0 @@
-package dto
-
-
-type GetRoleAuthorizedResourcesDto struct{
- Code string `json:"code,omitempty"`
- Namespace string `json:"namespace,omitempty"`
- ResourceType string `json:"resource_type,omitempty"`
-}
-
diff --git a/dto/GetRoleDto.go b/dto/GetRoleDto.go
deleted file mode 100644
index beb0a0b..0000000
--- a/dto/GetRoleDto.go
+++ /dev/null
@@ -1,8 +0,0 @@
-package dto
-
-
-type GetRoleDto struct{
- Code string `json:"code,omitempty"`
- Namespace string `json:"namespace,omitempty"`
-}
-
diff --git a/dto/GetUserAccessibleAppsDto.go b/dto/GetUserAccessibleAppsDto.go
deleted file mode 100644
index 0de8d51..0000000
--- a/dto/GetUserAccessibleAppsDto.go
+++ /dev/null
@@ -1,7 +0,0 @@
-package dto
-
-
-type GetUserAccessibleAppsDto struct{
- UserId string `json:"user_id,omitempty"`
-}
-
diff --git a/dto/GetUserAuthorizedAppsDto.go b/dto/GetUserAuthorizedAppsDto.go
deleted file mode 100644
index 70d054e..0000000
--- a/dto/GetUserAuthorizedAppsDto.go
+++ /dev/null
@@ -1,7 +0,0 @@
-package dto
-
-
-type GetUserAuthorizedAppsDto struct{
- UserId string `json:"user_id,omitempty"`
-}
-
diff --git a/dto/GetUserAuthorizedResourcesDto.go b/dto/GetUserAuthorizedResourcesDto.go
deleted file mode 100644
index 0b2b0bc..0000000
--- a/dto/GetUserAuthorizedResourcesDto.go
+++ /dev/null
@@ -1,9 +0,0 @@
-package dto
-
-
-type GetUserAuthorizedResourcesDto struct{
- UserId string `json:"user_id,omitempty"`
- Namespace string `json:"namespace,omitempty"`
- ResourceType string `json:"resource_type,omitempty"`
-}
-
diff --git a/dto/GetUserBatchDto.go b/dto/GetUserBatchDto.go
deleted file mode 100644
index 48dd10a..0000000
--- a/dto/GetUserBatchDto.go
+++ /dev/null
@@ -1,10 +0,0 @@
-package dto
-
-
-type GetUserBatchDto struct{
- UserIds string `json:"user_ids,omitempty"`
- WithCustomData bool `json:"with_custom_data,omitempty"`
- WithIdentities bool `json:"with_identities,omitempty"`
- WithDepartmentIds bool `json:"with_department_ids,omitempty"`
-}
-
diff --git a/dto/GetUserDepartmentsDto.go b/dto/GetUserDepartmentsDto.go
deleted file mode 100644
index 2d0971c..0000000
--- a/dto/GetUserDepartmentsDto.go
+++ /dev/null
@@ -1,7 +0,0 @@
-package dto
-
-
-type GetUserDepartmentsDto struct{
- UserId string `json:"user_id,omitempty"`
-}
-
diff --git a/dto/GetUserDto.go b/dto/GetUserDto.go
deleted file mode 100644
index da25766..0000000
--- a/dto/GetUserDto.go
+++ /dev/null
@@ -1,14 +0,0 @@
-package dto
-
-
-type GetUserDto struct{
- UserId string `json:"user_id,omitempty"`
- WithCustomData bool `json:"with_custom_data,omitempty"`
- WithIdentities bool `json:"with_identities,omitempty"`
- WithDepartmentIds bool `json:"with_department_ids,omitempty"`
- Phone string `json:"phone,omitempty"`
- Email string `json:"email,omitempty"`
- Username string `json:"username,omitempty"`
- ExternalId string `json:"externalId,omitempty"`
-}
-
diff --git a/dto/GetUserGroupsDto.go b/dto/GetUserGroupsDto.go
deleted file mode 100644
index 94f1ee5..0000000
--- a/dto/GetUserGroupsDto.go
+++ /dev/null
@@ -1,7 +0,0 @@
-package dto
-
-
-type GetUserGroupsDto struct{
- UserId string `json:"user_id,omitempty"`
-}
-
diff --git a/dto/GetUserIdentitiesDto.go b/dto/GetUserIdentitiesDto.go
deleted file mode 100644
index 212246a..0000000
--- a/dto/GetUserIdentitiesDto.go
+++ /dev/null
@@ -1,7 +0,0 @@
-package dto
-
-
-type GetUserIdentitiesDto struct{
- UserId string `json:"user_id,omitempty"`
-}
-
diff --git a/dto/GetUserLoggedinAppsDto.go b/dto/GetUserLoggedinAppsDto.go
deleted file mode 100644
index 1c1a4e9..0000000
--- a/dto/GetUserLoggedinAppsDto.go
+++ /dev/null
@@ -1,7 +0,0 @@
-package dto
-
-
-type GetUserLoggedinAppsDto struct{
- UserId string `json:"user_id,omitempty"`
-}
-
diff --git a/dto/GetUserLoginHistoryDto.go b/dto/GetUserLoginHistoryDto.go
deleted file mode 100644
index ceea1f0..0000000
--- a/dto/GetUserLoginHistoryDto.go
+++ /dev/null
@@ -1,13 +0,0 @@
-package dto
-
-
-type GetUserLoginHistoryDto struct{
- UserId string `json:"user_id,omitempty"`
- AppId string `json:"app_id,omitempty"`
- ClientIp string `json:"client_ip,omitempty"`
- Start int `json:"start,omitempty"`
- End int `json:"end,omitempty"`
- Page int `json:"page,omitempty"`
- Limit int `json:"limit,omitempty"`
-}
-
diff --git a/dto/GetUserMfaInfoDto.go b/dto/GetUserMfaInfoDto.go
deleted file mode 100644
index 5e2897b..0000000
--- a/dto/GetUserMfaInfoDto.go
+++ /dev/null
@@ -1,7 +0,0 @@
-package dto
-
-
-type GetUserMfaInfoDto struct{
- UserId string `json:"user_id,omitempty"`
-}
-
diff --git a/dto/GetUserPrincipalAuthenticationInfoDto.go b/dto/GetUserPrincipalAuthenticationInfoDto.go
deleted file mode 100644
index 91f76bf..0000000
--- a/dto/GetUserPrincipalAuthenticationInfoDto.go
+++ /dev/null
@@ -1,7 +0,0 @@
-package dto
-
-
-type GetUserPrincipalAuthenticationInfoDto struct{
- UserId string `json:"user_id,omitempty"`
-}
-
diff --git a/dto/GetUserRolesDto.go b/dto/GetUserRolesDto.go
deleted file mode 100644
index 01bc891..0000000
--- a/dto/GetUserRolesDto.go
+++ /dev/null
@@ -1,8 +0,0 @@
-package dto
-
-
-type GetUserRolesDto struct{
- UserId string `json:"user_id,omitempty"`
- Namespace string `json:"namespace,omitempty"`
-}
-
diff --git a/dto/GroupDto.go b/dto/GroupDto.go
deleted file mode 100644
index 78d021c..0000000
--- a/dto/GroupDto.go
+++ /dev/null
@@ -1,9 +0,0 @@
-package dto
-
-
-type GroupDto struct{
- Code string `json:"code"`
- Name string `json:"name"`
- Description string `json:"description"`
-}
-
diff --git a/dto/GroupListRespDto.go b/dto/GroupListRespDto.go
deleted file mode 100644
index 8fff584..0000000
--- a/dto/GroupListRespDto.go
+++ /dev/null
@@ -1,10 +0,0 @@
-package dto
-
-
-type GroupListRespDto struct{
- StatusCode int `json:"statusCode"`
- Message string `json:"message"`
- ApiCode int `json:"apiCode,omitempty"`
- Data []GroupDto `json:"data"`
-}
-
diff --git a/dto/GroupPaginatedRespDto.go b/dto/GroupPaginatedRespDto.go
deleted file mode 100644
index 715e404..0000000
--- a/dto/GroupPaginatedRespDto.go
+++ /dev/null
@@ -1,10 +0,0 @@
-package dto
-
-
-type GroupPaginatedRespDto struct{
- StatusCode int `json:"statusCode"`
- Message string `json:"message"`
- ApiCode int `json:"apiCode,omitempty"`
- Data GroupPagingDto `json:"data"`
-}
-
diff --git a/dto/GroupPagingDto.go b/dto/GroupPagingDto.go
deleted file mode 100644
index 0b01040..0000000
--- a/dto/GroupPagingDto.go
+++ /dev/null
@@ -1,8 +0,0 @@
-package dto
-
-
-type GroupPagingDto struct{
- TotalCount int `json:"totalCount"`
- List []ResGroupDto `json:"list"`
-}
-
diff --git a/dto/GroupSingleRespDto.go b/dto/GroupSingleRespDto.go
deleted file mode 100644
index 2392669..0000000
--- a/dto/GroupSingleRespDto.go
+++ /dev/null
@@ -1,10 +0,0 @@
-package dto
-
-
-type GroupSingleRespDto struct{
- StatusCode int `json:"statusCode"`
- Message string `json:"message"`
- ApiCode int `json:"apiCode,omitempty"`
- Data GroupDto `json:"data"`
-}
-
diff --git a/dto/HasAnyRoleDto.go b/dto/HasAnyRoleDto.go
deleted file mode 100644
index 880d4d8..0000000
--- a/dto/HasAnyRoleDto.go
+++ /dev/null
@@ -1,7 +0,0 @@
-package dto
-
-
-type HasAnyRoleDto struct{
- HasAnyRole bool `json:"hasAnyRole"`
-}
-
diff --git a/dto/HasAnyRoleReqDto.go b/dto/HasAnyRoleReqDto.go
deleted file mode 100644
index 4feecdf..0000000
--- a/dto/HasAnyRoleReqDto.go
+++ /dev/null
@@ -1,8 +0,0 @@
-package dto
-
-
-type HasAnyRoleReqDto struct{
- Roles []HasRoleRolesDto `json:"roles"`
- UserId string `json:"userId"`
-}
-
diff --git a/dto/HasAnyRoleRespDto.go b/dto/HasAnyRoleRespDto.go
deleted file mode 100644
index 29f0a9b..0000000
--- a/dto/HasAnyRoleRespDto.go
+++ /dev/null
@@ -1,10 +0,0 @@
-package dto
-
-
-type HasAnyRoleRespDto struct{
- StatusCode int `json:"statusCode"`
- Message string `json:"message"`
- ApiCode int `json:"apiCode,omitempty"`
- Data HasAnyRoleDto `json:"data"`
-}
-
diff --git a/dto/HasRoleRolesDto.go b/dto/HasRoleRolesDto.go
deleted file mode 100644
index 6cba7bd..0000000
--- a/dto/HasRoleRolesDto.go
+++ /dev/null
@@ -1,8 +0,0 @@
-package dto
-
-
-type HasRoleRolesDto struct{
- Namespace string `json:"namespace,omitempty"`
- Code string `json:"code"`
-}
-
diff --git a/dto/IdentityDto.go b/dto/IdentityDto.go
deleted file mode 100644
index a5ff072..0000000
--- a/dto/IdentityDto.go
+++ /dev/null
@@ -1,11 +0,0 @@
-package dto
-
-
-type IdentityDto struct{
- IdentityId string `json:"identityId"`
- ExtIdpId string `json:"extIdpId"`
- Provider string `json:"provider"`
- Type string `json:"type"`
- UserIdInIdp string `json:"userIdInIdp"`
-}
-
diff --git a/dto/IdentityListRespDto.go b/dto/IdentityListRespDto.go
deleted file mode 100644
index 03df55b..0000000
--- a/dto/IdentityListRespDto.go
+++ /dev/null
@@ -1,10 +0,0 @@
-package dto
-
-
-type IdentityListRespDto struct{
- StatusCode int `json:"statusCode"`
- Message string `json:"message"`
- ApiCode int `json:"apiCode,omitempty"`
- Data []IdentityDto `json:"data"`
-}
-
diff --git a/dto/IsSuccessDto.go b/dto/IsSuccessDto.go
deleted file mode 100644
index a167859..0000000
--- a/dto/IsSuccessDto.go
+++ /dev/null
@@ -1,7 +0,0 @@
-package dto
-
-
-type IsSuccessDto struct{
- Success bool `json:"success"`
-}
-
diff --git a/dto/IsSuccessRespDto.go b/dto/IsSuccessRespDto.go
deleted file mode 100644
index c8aa5b4..0000000
--- a/dto/IsSuccessRespDto.go
+++ /dev/null
@@ -1,10 +0,0 @@
-package dto
-
-
-type IsSuccessRespDto struct{
- StatusCode int `json:"statusCode"`
- Message string `json:"message"`
- ApiCode int `json:"apiCode,omitempty"`
- Data IsSuccessDto `json:"data"`
-}
-
diff --git a/dto/IsUserExistsDto.go b/dto/IsUserExistsDto.go
deleted file mode 100644
index 079bed5..0000000
--- a/dto/IsUserExistsDto.go
+++ /dev/null
@@ -1,7 +0,0 @@
-package dto
-
-
-type IsUserExistsDto struct{
- Exists bool `json:"exists"`
-}
-
diff --git a/dto/IsUserExistsReqDto.go b/dto/IsUserExistsReqDto.go
deleted file mode 100644
index 7ce6f28..0000000
--- a/dto/IsUserExistsReqDto.go
+++ /dev/null
@@ -1,10 +0,0 @@
-package dto
-
-
-type IsUserExistsReqDto struct{
- Username string `json:"username,omitempty"`
- Email string `json:"email,omitempty"`
- Phone string `json:"phone,omitempty"`
- ExternalId string `json:"externalId,omitempty"`
-}
-
diff --git a/dto/IsUserExistsRespDto.go b/dto/IsUserExistsRespDto.go
deleted file mode 100644
index e8d06e9..0000000
--- a/dto/IsUserExistsRespDto.go
+++ /dev/null
@@ -1,10 +0,0 @@
-package dto
-
-
-type IsUserExistsRespDto struct{
- StatusCode int `json:"statusCode"`
- Message string `json:"message"`
- ApiCode int `json:"apiCode,omitempty"`
- Data IsUserExistsDto `json:"data"`
-}
-
diff --git a/dto/KickUsersDto.go b/dto/KickUsersDto.go
deleted file mode 100644
index 21930e9..0000000
--- a/dto/KickUsersDto.go
+++ /dev/null
@@ -1,8 +0,0 @@
-package dto
-
-
-type KickUsersDto struct{
- AppIds []string `json:"appIds"`
- UserId string `json:"userId"`
-}
-
diff --git a/dto/ListArchivedUsersDto.go b/dto/ListArchivedUsersDto.go
deleted file mode 100644
index b67dea0..0000000
--- a/dto/ListArchivedUsersDto.go
+++ /dev/null
@@ -1,8 +0,0 @@
-package dto
-
-
-type ListArchivedUsersDto struct{
- Page int `json:"page,omitempty"`
- Limit int `json:"limit,omitempty"`
-}
-
diff --git a/dto/ListArchivedUsersRespDto.go b/dto/ListArchivedUsersRespDto.go
deleted file mode 100644
index f104424..0000000
--- a/dto/ListArchivedUsersRespDto.go
+++ /dev/null
@@ -1,7 +0,0 @@
-package dto
-
-
-type ListArchivedUsersRespDto struct{
- UserId string `json:"userId"`
-}
-
diff --git a/dto/ListArchivedUsersSingleRespDto.go b/dto/ListArchivedUsersSingleRespDto.go
deleted file mode 100644
index a9603a0..0000000
--- a/dto/ListArchivedUsersSingleRespDto.go
+++ /dev/null
@@ -1,10 +0,0 @@
-package dto
-
-
-type ListArchivedUsersSingleRespDto struct{
- StatusCode int `json:"statusCode"`
- Message string `json:"message"`
- ApiCode int `json:"apiCode,omitempty"`
- Data ArchivedUsersListPagingDto `json:"data"`
-}
-
diff --git a/dto/ListChildrenDepartmentsDto.go b/dto/ListChildrenDepartmentsDto.go
deleted file mode 100644
index fd13cc8..0000000
--- a/dto/ListChildrenDepartmentsDto.go
+++ /dev/null
@@ -1,9 +0,0 @@
-package dto
-
-
-type ListChildrenDepartmentsDto struct{
- DepartmentId string `json:"department_id,omitempty"`
- OrganizationCode string `json:"organization_code,omitempty"`
- DepartmentIdType string `json:"department_id_type,omitempty"`
-}
-
diff --git a/dto/ListDepartmentMemberIdsDto.go b/dto/ListDepartmentMemberIdsDto.go
deleted file mode 100644
index 450f377..0000000
--- a/dto/ListDepartmentMemberIdsDto.go
+++ /dev/null
@@ -1,9 +0,0 @@
-package dto
-
-
-type ListDepartmentMemberIdsDto struct{
- OrganizationCode string `json:"organization_code,omitempty"`
- DepartmentId string `json:"department_id,omitempty"`
- DepartmentIdType string `json:"department_id_type,omitempty"`
-}
-
diff --git a/dto/ListDepartmentMembersDto.go b/dto/ListDepartmentMembersDto.go
deleted file mode 100644
index 300b48d..0000000
--- a/dto/ListDepartmentMembersDto.go
+++ /dev/null
@@ -1,14 +0,0 @@
-package dto
-
-
-type ListDepartmentMembersDto struct{
- OrganizationCode string `json:"organization_code,omitempty"`
- DepartmentId string `json:"department_id,omitempty"`
- DepartmentIdType string `json:"department_id_type,omitempty"`
- Page int `json:"page,omitempty"`
- Limit int `json:"limit,omitempty"`
- WithCustomData bool `json:"with_custom_data,omitempty"`
- WithIdentities bool `json:"with_identities,omitempty"`
- WithDepartmentIds bool `json:"with_department_ids,omitempty"`
-}
-
diff --git a/dto/ListExtIdpDto.go b/dto/ListExtIdpDto.go
deleted file mode 100644
index 76d0eb6..0000000
--- a/dto/ListExtIdpDto.go
+++ /dev/null
@@ -1,7 +0,0 @@
-package dto
-
-
-type ListExtIdpDto struct{
- TenantId string `json:"tenant_id,omitempty"`
-}
-
diff --git a/dto/ListGroupMembersDto.go b/dto/ListGroupMembersDto.go
deleted file mode 100644
index 37d0ea7..0000000
--- a/dto/ListGroupMembersDto.go
+++ /dev/null
@@ -1,12 +0,0 @@
-package dto
-
-
-type ListGroupMembersDto struct{
- Code string `json:"code,omitempty"`
- Page int `json:"page,omitempty"`
- Limit int `json:"limit,omitempty"`
- WithCustomData bool `json:"with_custom_data,omitempty"`
- WithIdentities bool `json:"with_identities,omitempty"`
- WithDepartmentIds bool `json:"with_department_ids,omitempty"`
-}
-
diff --git a/dto/ListGroupsDto.go b/dto/ListGroupsDto.go
deleted file mode 100644
index ce25bcb..0000000
--- a/dto/ListGroupsDto.go
+++ /dev/null
@@ -1,8 +0,0 @@
-package dto
-
-
-type ListGroupsDto struct{
- Page int `json:"page,omitempty"`
- Limit int `json:"limit,omitempty"`
-}
-
diff --git a/dto/ListOrganizationsDto.go b/dto/ListOrganizationsDto.go
deleted file mode 100644
index 4dcc40a..0000000
--- a/dto/ListOrganizationsDto.go
+++ /dev/null
@@ -1,8 +0,0 @@
-package dto
-
-
-type ListOrganizationsDto struct{
- Page int `json:"page,omitempty"`
- Limit int `json:"limit,omitempty"`
-}
-
diff --git a/dto/ListResourcesDto.go b/dto/ListResourcesDto.go
deleted file mode 100644
index c16c1a7..0000000
--- a/dto/ListResourcesDto.go
+++ /dev/null
@@ -1,10 +0,0 @@
-package dto
-
-
-type ListResourcesDto struct{
- Namespace string `json:"namespace,omitempty"`
- Type string `json:"type,omitempty"`
- Page int `json:"page,omitempty"`
- Limit int `json:"limit,omitempty"`
-}
-
diff --git a/dto/ListRoleDepartmentsDto.go b/dto/ListRoleDepartmentsDto.go
deleted file mode 100644
index 061eedb..0000000
--- a/dto/ListRoleDepartmentsDto.go
+++ /dev/null
@@ -1,10 +0,0 @@
-package dto
-
-
-type ListRoleDepartmentsDto struct{
- Code string `json:"code,omitempty"`
- Namespace string `json:"namespace,omitempty"`
- Page int `json:"page,omitempty"`
- Limit int `json:"limit,omitempty"`
-}
-
diff --git a/dto/ListRoleMembersDto.go b/dto/ListRoleMembersDto.go
deleted file mode 100644
index d6d6e6b..0000000
--- a/dto/ListRoleMembersDto.go
+++ /dev/null
@@ -1,13 +0,0 @@
-package dto
-
-
-type ListRoleMembersDto struct{
- Code string `json:"code,omitempty"`
- Page int `json:"page,omitempty"`
- Limit int `json:"limit,omitempty"`
- WithCustomData bool `json:"with_custom_data,omitempty"`
- WithIdentities bool `json:"with_identities,omitempty"`
- WithDepartmentIds bool `json:"with_department_ids,omitempty"`
- Namespace string `json:"namespace,omitempty"`
-}
-
diff --git a/dto/ListRolesDto.go b/dto/ListRolesDto.go
deleted file mode 100644
index b5d39b0..0000000
--- a/dto/ListRolesDto.go
+++ /dev/null
@@ -1,9 +0,0 @@
-package dto
-
-
-type ListRolesDto struct{
- Namespace string `json:"namespace,omitempty"`
- Page int `json:"page,omitempty"`
- Limit int `json:"limit,omitempty"`
-}
-
diff --git a/dto/ListUsersDto.go b/dto/ListUsersDto.go
deleted file mode 100644
index 999ff71..0000000
--- a/dto/ListUsersDto.go
+++ /dev/null
@@ -1,11 +0,0 @@
-package dto
-
-
-type ListUsersDto struct{
- Page int `json:"page,omitempty"`
- Limit int `json:"limit,omitempty"`
- WithCustomData bool `json:"with_custom_data,omitempty"`
- WithIdentities bool `json:"with_identities,omitempty"`
- WithDepartmentIds bool `json:"with_department_ids,omitempty"`
-}
-
diff --git a/dto/NamespaceDto.go b/dto/NamespaceDto.go
deleted file mode 100644
index a9d4b16..0000000
--- a/dto/NamespaceDto.go
+++ /dev/null
@@ -1,9 +0,0 @@
-package dto
-
-
-type NamespaceDto struct{
- Code string `json:"code"`
- Name string `json:"name,omitempty"`
- Description string `json:"description,omitempty"`
-}
-
diff --git a/dto/NamespaceListRespDto.go b/dto/NamespaceListRespDto.go
deleted file mode 100644
index 45160c5..0000000
--- a/dto/NamespaceListRespDto.go
+++ /dev/null
@@ -1,7 +0,0 @@
-package dto
-
-
-type NamespaceListRespDto struct{
- Data []NamespaceDto `json:"data"`
-}
-
diff --git a/dto/NamespaceRespDto.go b/dto/NamespaceRespDto.go
deleted file mode 100644
index 53a4327..0000000
--- a/dto/NamespaceRespDto.go
+++ /dev/null
@@ -1,7 +0,0 @@
-package dto
-
-
-type NamespaceRespDto struct{
- Data NamespaceDto `json:"data"`
-}
-
diff --git a/dto/OrganizationDto.go b/dto/OrganizationDto.go
deleted file mode 100644
index a8c4295..0000000
--- a/dto/OrganizationDto.go
+++ /dev/null
@@ -1,13 +0,0 @@
-package dto
-
-
-type OrganizationDto struct{
- OrganizationCode string `json:"organizationCode"`
- OrganizationName string `json:"organizationName"`
- DepartmentId string `json:"departmentId"`
- OpenDepartmentId string `json:"openDepartmentId,omitempty"`
- HasChildren bool `json:"hasChildren"`
- LeaderUserId string `json:"leaderUserId"`
- MembersCount int `json:"membersCount"`
-}
-
diff --git a/dto/OrganizationPaginatedRespDto.go b/dto/OrganizationPaginatedRespDto.go
deleted file mode 100644
index 8e0f16f..0000000
--- a/dto/OrganizationPaginatedRespDto.go
+++ /dev/null
@@ -1,10 +0,0 @@
-package dto
-
-
-type OrganizationPaginatedRespDto struct{
- StatusCode int `json:"statusCode"`
- Message string `json:"message"`
- ApiCode int `json:"apiCode,omitempty"`
- Data OrganizationPagingDto `json:"data"`
-}
-
diff --git a/dto/OrganizationPagingDto.go b/dto/OrganizationPagingDto.go
deleted file mode 100644
index cf89c66..0000000
--- a/dto/OrganizationPagingDto.go
+++ /dev/null
@@ -1,8 +0,0 @@
-package dto
-
-
-type OrganizationPagingDto struct{
- TotalCount int `json:"totalCount"`
- List []OrganizationDto `json:"list"`
-}
-
diff --git a/dto/OrganizationSingleRespDto.go b/dto/OrganizationSingleRespDto.go
deleted file mode 100644
index adb4c89..0000000
--- a/dto/OrganizationSingleRespDto.go
+++ /dev/null
@@ -1,10 +0,0 @@
-package dto
-
-
-type OrganizationSingleRespDto struct{
- StatusCode int `json:"statusCode"`
- Message string `json:"message"`
- ApiCode int `json:"apiCode,omitempty"`
- Data OrganizationDto `json:"data"`
-}
-
diff --git a/dto/PrincipalAuthenticationInfoDto.go b/dto/PrincipalAuthenticationInfoDto.go
deleted file mode 100644
index fdb47cd..0000000
--- a/dto/PrincipalAuthenticationInfoDto.go
+++ /dev/null
@@ -1,11 +0,0 @@
-package dto
-
-
-type PrincipalAuthenticationInfoDto struct{
- Authenticated bool `json:"authenticated"`
- PrincipalType string `json:"principalType"`
- PrincipalCode string `json:"principalCode"`
- PrincipalName string `json:"principalName"`
- AuthenticatedAt string `json:"authenticatedAt"`
-}
-
diff --git a/dto/PrincipalAuthenticationInfoPaginatedRespDto.go b/dto/PrincipalAuthenticationInfoPaginatedRespDto.go
deleted file mode 100644
index a6ebd0f..0000000
--- a/dto/PrincipalAuthenticationInfoPaginatedRespDto.go
+++ /dev/null
@@ -1,10 +0,0 @@
-package dto
-
-
-type PrincipalAuthenticationInfoPaginatedRespDto struct{
- StatusCode int `json:"statusCode"`
- Message string `json:"message"`
- ApiCode int `json:"apiCode,omitempty"`
- Data PrincipalAuthenticationInfoPagingDto `json:"data"`
-}
-
diff --git a/dto/PrincipalAuthenticationInfoPagingDto.go b/dto/PrincipalAuthenticationInfoPagingDto.go
deleted file mode 100644
index 5a3e593..0000000
--- a/dto/PrincipalAuthenticationInfoPagingDto.go
+++ /dev/null
@@ -1,8 +0,0 @@
-package dto
-
-
-type PrincipalAuthenticationInfoPagingDto struct{
- TotalCount int `json:"totalCount"`
- List []PrincipalAuthenticationInfoDto `json:"list"`
-}
-
diff --git a/dto/RemoveDepartmentMembersReqDto.go b/dto/RemoveDepartmentMembersReqDto.go
deleted file mode 100644
index ae99149..0000000
--- a/dto/RemoveDepartmentMembersReqDto.go
+++ /dev/null
@@ -1,10 +0,0 @@
-package dto
-
-
-type RemoveDepartmentMembersReqDto struct{
- UserIds []string `json:"userIds"`
- OrganizationCode string `json:"organizationCode"`
- DepartmentId string `json:"departmentId"`
- DepartmentIdType string `json:"departmentIdType,omitempty"`
-}
-
diff --git a/dto/RemoveGroupMembersReqDto.go b/dto/RemoveGroupMembersReqDto.go
deleted file mode 100644
index 6d471b0..0000000
--- a/dto/RemoveGroupMembersReqDto.go
+++ /dev/null
@@ -1,8 +0,0 @@
-package dto
-
-
-type RemoveGroupMembersReqDto struct{
- UserIds []string `json:"userIds"`
- Code string `json:"code"`
-}
-
diff --git a/dto/ResGroupDto.go b/dto/ResGroupDto.go
deleted file mode 100644
index b57d777..0000000
--- a/dto/ResGroupDto.go
+++ /dev/null
@@ -1,9 +0,0 @@
-package dto
-
-
-type ResGroupDto struct{
- Code string `json:"code"`
- Name string `json:"name"`
- Description string `json:"description"`
-}
-
diff --git a/dto/ResetPrincipalAuthenticationInfoRespDto.go b/dto/ResetPrincipalAuthenticationInfoRespDto.go
deleted file mode 100644
index 7ddcf1a..0000000
--- a/dto/ResetPrincipalAuthenticationInfoRespDto.go
+++ /dev/null
@@ -1,7 +0,0 @@
-package dto
-
-
-type ResetPrincipalAuthenticationInfoRespDto struct{
- Success bool `json:"success"`
-}
-
diff --git a/dto/ResetUserPrincipalAuthenticationInfoDto.go b/dto/ResetUserPrincipalAuthenticationInfoDto.go
deleted file mode 100644
index 1769293..0000000
--- a/dto/ResetUserPrincipalAuthenticationInfoDto.go
+++ /dev/null
@@ -1,7 +0,0 @@
-package dto
-
-
-type ResetUserPrincipalAuthenticationInfoDto struct{
- UserId string `json:"userId"`
-}
-
diff --git a/dto/ResourceAction.go b/dto/ResourceAction.go
deleted file mode 100644
index a7c393c..0000000
--- a/dto/ResourceAction.go
+++ /dev/null
@@ -1,8 +0,0 @@
-package dto
-
-
-type ResourceAction struct{
- Name string `json:"name"`
- Description string `json:"description"`
-}
-
diff --git a/dto/ResourceDto.go b/dto/ResourceDto.go
deleted file mode 100644
index 068abdf..0000000
--- a/dto/ResourceDto.go
+++ /dev/null
@@ -1,12 +0,0 @@
-package dto
-
-
-type ResourceDto struct{
- Code string `json:"code"`
- Description string `json:"description,omitempty"`
- Type string `json:"type"`
- Actions []ResourceAction `json:"actions,omitempty"`
- ApiIdentifier string `json:"apiIdentifier,omitempty"`
- Namespace string `json:"namespace,omitempty"`
-}
-
diff --git a/dto/ResourceItemDto.go b/dto/ResourceItemDto.go
deleted file mode 100644
index 84fc00e..0000000
--- a/dto/ResourceItemDto.go
+++ /dev/null
@@ -1,9 +0,0 @@
-package dto
-
-
-type ResourceItemDto struct{
- Code string `json:"code"`
- Actions []string `json:"actions"`
- ResourceType string `json:"resourceType"`
-}
-
diff --git a/dto/ResourceListRespDto.go b/dto/ResourceListRespDto.go
deleted file mode 100644
index 106340b..0000000
--- a/dto/ResourceListRespDto.go
+++ /dev/null
@@ -1,10 +0,0 @@
-package dto
-
-
-type ResourceListRespDto struct{
- StatusCode int `json:"statusCode"`
- Message string `json:"message"`
- ApiCode int `json:"apiCode,omitempty"`
- Data []ResourceDto `json:"data"`
-}
-
diff --git a/dto/ResourcePaginatedRespDto.go b/dto/ResourcePaginatedRespDto.go
deleted file mode 100644
index c81cc1b..0000000
--- a/dto/ResourcePaginatedRespDto.go
+++ /dev/null
@@ -1,10 +0,0 @@
-package dto
-
-
-type ResourcePaginatedRespDto struct{
- StatusCode int `json:"statusCode"`
- Message string `json:"message"`
- ApiCode int `json:"apiCode,omitempty"`
- Data ResourcePagingDto `json:"data"`
-}
-
diff --git a/dto/ResourcePagingDto.go b/dto/ResourcePagingDto.go
deleted file mode 100644
index 52e8077..0000000
--- a/dto/ResourcePagingDto.go
+++ /dev/null
@@ -1,11 +0,0 @@
-package dto
-
-
-type ResourcePagingDto struct{
- StatusCode int `json:"statusCode"`
- Message string `json:"message"`
- ApiCode int `json:"apiCode,omitempty"`
- TotalCount int `json:"totalCount"`
- List []ResourceDto `json:"list"`
-}
-
diff --git a/dto/ResourceRespDto.go b/dto/ResourceRespDto.go
deleted file mode 100644
index 61c93eb..0000000
--- a/dto/ResourceRespDto.go
+++ /dev/null
@@ -1,10 +0,0 @@
-package dto
-
-
-type ResourceRespDto struct{
- StatusCode int `json:"statusCode"`
- Message string `json:"message"`
- ApiCode int `json:"apiCode,omitempty"`
- Data ResourceDto `json:"data"`
-}
-
diff --git a/dto/RevokeRoleBatchDto.go b/dto/RevokeRoleBatchDto.go
deleted file mode 100644
index 6e5c843..0000000
--- a/dto/RevokeRoleBatchDto.go
+++ /dev/null
@@ -1,8 +0,0 @@
-package dto
-
-
-type RevokeRoleBatchDto struct{
- Targets []TargetDto `json:"targets"`
- Roles []RoleCodeDto `json:"roles"`
-}
-
diff --git a/dto/RevokeRoleDto.go b/dto/RevokeRoleDto.go
deleted file mode 100644
index 44690e4..0000000
--- a/dto/RevokeRoleDto.go
+++ /dev/null
@@ -1,9 +0,0 @@
-package dto
-
-
-type RevokeRoleDto struct{
- Targets []TargetDto `json:"targets"`
- Code string `json:"code"`
- Namespace string `json:"namespace,omitempty"`
-}
-
diff --git a/dto/Role.go b/dto/Role.go
deleted file mode 100644
index e43e8c2..0000000
--- a/dto/Role.go
+++ /dev/null
@@ -1,6 +0,0 @@
-package dto
-
-
-type Role struct{
-}
-
diff --git a/dto/RoleAuthorizedResourcePaginatedRespDto.go b/dto/RoleAuthorizedResourcePaginatedRespDto.go
deleted file mode 100644
index f2ac1b8..0000000
--- a/dto/RoleAuthorizedResourcePaginatedRespDto.go
+++ /dev/null
@@ -1,10 +0,0 @@
-package dto
-
-
-type RoleAuthorizedResourcePaginatedRespDto struct{
- StatusCode int `json:"statusCode"`
- Message string `json:"message"`
- ApiCode int `json:"apiCode,omitempty"`
- Data RoleAuthorizedResourcePagingDto `json:"data"`
-}
-
diff --git a/dto/RoleAuthorizedResourcePagingDto.go b/dto/RoleAuthorizedResourcePagingDto.go
deleted file mode 100644
index 89df242..0000000
--- a/dto/RoleAuthorizedResourcePagingDto.go
+++ /dev/null
@@ -1,8 +0,0 @@
-package dto
-
-
-type RoleAuthorizedResourcePagingDto struct{
- TotalCount int `json:"totalCount"`
- List []RoleAuthorizedResourcesRespDto `json:"list"`
-}
-
diff --git a/dto/RoleAuthorizedResourcesRespDto.go b/dto/RoleAuthorizedResourcesRespDto.go
deleted file mode 100644
index 23a9043..0000000
--- a/dto/RoleAuthorizedResourcesRespDto.go
+++ /dev/null
@@ -1,10 +0,0 @@
-package dto
-
-
-type RoleAuthorizedResourcesRespDto struct{
- ResourceCode string `json:"resourceCode"`
- ResourceType string `json:"resourceType"`
- Actions []string `json:"actions"`
- ApiIdentifier string `json:"apiIdentifier"`
-}
-
diff --git a/dto/RoleCodeDto.go b/dto/RoleCodeDto.go
deleted file mode 100644
index 5f027aa..0000000
--- a/dto/RoleCodeDto.go
+++ /dev/null
@@ -1,8 +0,0 @@
-package dto
-
-
-type RoleCodeDto struct{
- Code string `json:"code"`
- Namespace string `json:"namespace,omitempty"`
-}
-
diff --git a/dto/RoleDepartmentListPaginatedRespDto.go b/dto/RoleDepartmentListPaginatedRespDto.go
deleted file mode 100644
index b8753ea..0000000
--- a/dto/RoleDepartmentListPaginatedRespDto.go
+++ /dev/null
@@ -1,10 +0,0 @@
-package dto
-
-
-type RoleDepartmentListPaginatedRespDto struct{
- StatusCode int `json:"statusCode"`
- Message string `json:"message"`
- ApiCode int `json:"apiCode,omitempty"`
- Data RoleDepartmentListPagingDto `json:"data"`
-}
-
diff --git a/dto/RoleDepartmentListPagingDto.go b/dto/RoleDepartmentListPagingDto.go
deleted file mode 100644
index bb8c3dd..0000000
--- a/dto/RoleDepartmentListPagingDto.go
+++ /dev/null
@@ -1,8 +0,0 @@
-package dto
-
-
-type RoleDepartmentListPagingDto struct{
- TotalCount int `json:"totalCount"`
- List []RoleDepartmentRespDto `json:"list"`
-}
-
diff --git a/dto/RoleDepartmentRespDto.go b/dto/RoleDepartmentRespDto.go
deleted file mode 100644
index 8574934..0000000
--- a/dto/RoleDepartmentRespDto.go
+++ /dev/null
@@ -1,10 +0,0 @@
-package dto
-
-
-type RoleDepartmentRespDto struct{
- Id string `json:"id"`
- Code string `json:"code"`
- Name string `json:"name"`
- Description string `json:"description"`
-}
-
diff --git a/dto/RoleDto.go b/dto/RoleDto.go
deleted file mode 100644
index 0b0b2da..0000000
--- a/dto/RoleDto.go
+++ /dev/null
@@ -1,9 +0,0 @@
-package dto
-
-
-type RoleDto struct{
- Code string `json:"code"`
- Description string `json:"description"`
- Namespace string `json:"namespace"`
-}
-
diff --git a/dto/RoleListItem.go b/dto/RoleListItem.go
deleted file mode 100644
index de2e996..0000000
--- a/dto/RoleListItem.go
+++ /dev/null
@@ -1,9 +0,0 @@
-package dto
-
-
-type RoleListItem struct{
- Code string `json:"code"`
- Description string `json:"description,omitempty"`
- Namespace string `json:"namespace,omitempty"`
-}
-
diff --git a/dto/RolePaginatedRespDto.go b/dto/RolePaginatedRespDto.go
deleted file mode 100644
index cc2af65..0000000
--- a/dto/RolePaginatedRespDto.go
+++ /dev/null
@@ -1,10 +0,0 @@
-package dto
-
-
-type RolePaginatedRespDto struct{
- StatusCode int `json:"statusCode"`
- Message string `json:"message"`
- ApiCode int `json:"apiCode,omitempty"`
- Data RolePagingDto `json:"data"`
-}
-
diff --git a/dto/RolePagingDto.go b/dto/RolePagingDto.go
deleted file mode 100644
index a7800e8..0000000
--- a/dto/RolePagingDto.go
+++ /dev/null
@@ -1,8 +0,0 @@
-package dto
-
-
-type RolePagingDto struct{
- TotalCount int `json:"totalCount"`
- List []RoleDto `json:"list"`
-}
-
diff --git a/dto/RoleSingleRespDto.go b/dto/RoleSingleRespDto.go
deleted file mode 100644
index 0a5d055..0000000
--- a/dto/RoleSingleRespDto.go
+++ /dev/null
@@ -1,10 +0,0 @@
-package dto
-
-
-type RoleSingleRespDto struct{
- StatusCode int `json:"statusCode"`
- Message string `json:"message"`
- ApiCode int `json:"apiCode,omitempty"`
- Data RoleDto `json:"data"`
-}
-
diff --git a/dto/RolesDto.go b/dto/RolesDto.go
deleted file mode 100644
index e4c4b86..0000000
--- a/dto/RolesDto.go
+++ /dev/null
@@ -1,9 +0,0 @@
-package dto
-
-
-type RolesDto struct{
- Description string `json:"description"`
- Code string `json:"code"`
- Namespace string `json:"namespace"`
-}
-
diff --git a/dto/SearchDepartmentsReqDto.go b/dto/SearchDepartmentsReqDto.go
deleted file mode 100644
index ba9dc56..0000000
--- a/dto/SearchDepartmentsReqDto.go
+++ /dev/null
@@ -1,8 +0,0 @@
-package dto
-
-
-type SearchDepartmentsReqDto struct{
- Search string `json:"search"`
- OrganizationCode string `json:"organizationCode"`
-}
-
diff --git a/dto/SetCustomDataDto.go b/dto/SetCustomDataDto.go
deleted file mode 100644
index bad810e..0000000
--- a/dto/SetCustomDataDto.go
+++ /dev/null
@@ -1,8 +0,0 @@
-package dto
-
-
-type SetCustomDataDto struct{
- Key string `json:"key"`
- Value string `json:"value"`
-}
-
diff --git a/dto/SetCustomDataReqDto.go b/dto/SetCustomDataReqDto.go
deleted file mode 100644
index 2173b74..0000000
--- a/dto/SetCustomDataReqDto.go
+++ /dev/null
@@ -1,10 +0,0 @@
-package dto
-
-
-type SetCustomDataReqDto struct{
- List []SetCustomDataDto `json:"list"`
- TargetIdentifier string `json:"targetIdentifier"`
- TargetType string `json:"targetType"`
- Namespace string `json:"namespace,omitempty"`
-}
-
diff --git a/dto/SetCustomFieldDto.go b/dto/SetCustomFieldDto.go
deleted file mode 100644
index 2937443..0000000
--- a/dto/SetCustomFieldDto.go
+++ /dev/null
@@ -1,13 +0,0 @@
-package dto
-
-
-type SetCustomFieldDto struct{
- TargetType string `json:"targetType"`
- DataType string `json:"dataType"`
- Key string `json:"key"`
- Label string `json:"label"`
- Description string `json:"description,omitempty"`
- Encrypted bool `json:"encrypted,omitempty"`
- Options []CustomFieldSelectOption `json:"options,omitempty"`
-}
-
diff --git a/dto/SetCustomFieldsReqDto.go b/dto/SetCustomFieldsReqDto.go
deleted file mode 100644
index d931960..0000000
--- a/dto/SetCustomFieldsReqDto.go
+++ /dev/null
@@ -1,7 +0,0 @@
-package dto
-
-
-type SetCustomFieldsReqDto struct{
- List []SetCustomFieldDto `json:"list"`
-}
-
diff --git a/dto/SetUserCustomDataDto.go b/dto/SetUserCustomDataDto.go
deleted file mode 100644
index 747758b..0000000
--- a/dto/SetUserCustomDataDto.go
+++ /dev/null
@@ -1,7 +0,0 @@
-package dto
-
-
-type SetUserCustomDataDto struct{
- Success bool `json:"success"`
-}
-
diff --git a/dto/SetUserCustomDataRespDto.go b/dto/SetUserCustomDataRespDto.go
deleted file mode 100644
index 14e3743..0000000
--- a/dto/SetUserCustomDataRespDto.go
+++ /dev/null
@@ -1,10 +0,0 @@
-package dto
-
-
-type SetUserCustomDataRespDto struct{
- StatusCode int `json:"statusCode"`
- Message string `json:"message"`
- ApiCode int `json:"apiCode,omitempty"`
- Data SetUserCustomDataDto `json:"data"`
-}
-
diff --git a/dto/SetUserDepartmentDto.go b/dto/SetUserDepartmentDto.go
deleted file mode 100644
index 6a20c14..0000000
--- a/dto/SetUserDepartmentDto.go
+++ /dev/null
@@ -1,9 +0,0 @@
-package dto
-
-
-type SetUserDepartmentDto struct{
- DepartmentId string `json:"departmentId"`
- IsLeader bool `json:"isLeader,omitempty"`
- IsMainDepartment bool `json:"isMainDepartment,omitempty"`
-}
-
diff --git a/dto/SetUserDepartmentsDto.go b/dto/SetUserDepartmentsDto.go
deleted file mode 100644
index eb5b9c9..0000000
--- a/dto/SetUserDepartmentsDto.go
+++ /dev/null
@@ -1,8 +0,0 @@
-package dto
-
-
-type SetUserDepartmentsDto struct{
- Departments []SetUserDepartmentDto `json:"departments"`
- UserId string `json:"userId"`
-}
-
diff --git a/dto/TargetDto.go b/dto/TargetDto.go
deleted file mode 100644
index 80a4be9..0000000
--- a/dto/TargetDto.go
+++ /dev/null
@@ -1,8 +0,0 @@
-package dto
-
-
-type TargetDto struct{
- TargetType string `json:"targetType"`
- TargetIdentifier string `json:"targetIdentifier"`
-}
-
diff --git a/dto/UpdateDepartmentReqDto.go b/dto/UpdateDepartmentReqDto.go
deleted file mode 100644
index dcabf23..0000000
--- a/dto/UpdateDepartmentReqDto.go
+++ /dev/null
@@ -1,13 +0,0 @@
-package dto
-
-
-type UpdateDepartmentReqDto struct{
- OrganizationCode string `json:"organizationCode"`
- ParentDepartmentId string `json:"parentDepartmentId"`
- DepartmentId string `json:"departmentId"`
- Code string `json:"code,omitempty"`
- LeaderUserId string `json:"leaderUserId,omitempty"`
- Name string `json:"name,omitempty"`
- DepartmentIdType string `json:"departmentIdType,omitempty"`
-}
-
diff --git a/dto/UpdateExtIdpConnDto.go b/dto/UpdateExtIdpConnDto.go
deleted file mode 100644
index d7af8d9..0000000
--- a/dto/UpdateExtIdpConnDto.go
+++ /dev/null
@@ -1,11 +0,0 @@
-package dto
-
-
-type UpdateExtIdpConnDto struct{
- Fields interface{} `json:"fields"`
- DisplayName string `json:"displayName"`
- Id string `json:"id"`
- Logo string `json:"logo,omitempty"`
- LoginOnly bool `json:"loginOnly,omitempty"`
-}
-
diff --git a/dto/UpdateExtIdpDto.go b/dto/UpdateExtIdpDto.go
deleted file mode 100644
index eeae02c..0000000
--- a/dto/UpdateExtIdpDto.go
+++ /dev/null
@@ -1,8 +0,0 @@
-package dto
-
-
-type UpdateExtIdpDto struct{
- Id string `json:"id"`
- Name string `json:"name"`
-}
-
diff --git a/dto/UpdateGroupReqDto.go b/dto/UpdateGroupReqDto.go
deleted file mode 100644
index 2278bb4..0000000
--- a/dto/UpdateGroupReqDto.go
+++ /dev/null
@@ -1,10 +0,0 @@
-package dto
-
-
-type UpdateGroupReqDto struct{
- Description string `json:"description"`
- Name string `json:"name"`
- Code string `json:"code"`
- NewCode string `json:"newCode,omitempty"`
-}
-
diff --git a/dto/UpdateNamespaceDto.go b/dto/UpdateNamespaceDto.go
deleted file mode 100644
index a82f76f..0000000
--- a/dto/UpdateNamespaceDto.go
+++ /dev/null
@@ -1,10 +0,0 @@
-package dto
-
-
-type UpdateNamespaceDto struct{
- Code string `json:"code"`
- Description string `json:"description,omitempty"`
- Name string `json:"name,omitempty"`
- NewCode string `json:"newCode,omitempty"`
-}
-
diff --git a/dto/UpdateNamespaceRespDto.go b/dto/UpdateNamespaceRespDto.go
deleted file mode 100644
index 1dab353..0000000
--- a/dto/UpdateNamespaceRespDto.go
+++ /dev/null
@@ -1,10 +0,0 @@
-package dto
-
-
-type UpdateNamespaceRespDto struct{
- StatusCode int `json:"statusCode"`
- Message string `json:"message"`
- ApiCode int `json:"apiCode,omitempty"`
- Data UpdateNamespaceDto `json:"data"`
-}
-
diff --git a/dto/UpdateOrganizationReqDto.go b/dto/UpdateOrganizationReqDto.go
deleted file mode 100644
index c85a6c1..0000000
--- a/dto/UpdateOrganizationReqDto.go
+++ /dev/null
@@ -1,10 +0,0 @@
-package dto
-
-
-type UpdateOrganizationReqDto struct{
- OrganizationCode string `json:"organizationCode"`
- OpenDepartmentId string `json:"openDepartmentId,omitempty"`
- OrganizationNewCode string `json:"organizationNewCode,omitempty"`
- OrganizationName string `json:"organizationName,omitempty"`
-}
-
diff --git a/dto/UpdateResourceDto.go b/dto/UpdateResourceDto.go
deleted file mode 100644
index 1749bac..0000000
--- a/dto/UpdateResourceDto.go
+++ /dev/null
@@ -1,12 +0,0 @@
-package dto
-
-
-type UpdateResourceDto struct{
- Code string `json:"code"`
- Description string `json:"description,omitempty"`
- Actions []ResourceAction `json:"actions,omitempty"`
- ApiIdentifier string `json:"apiIdentifier,omitempty"`
- Namespace string `json:"namespace,omitempty"`
- Type string `json:"type,omitempty"`
-}
-
diff --git a/dto/UpdateRoleDto.go b/dto/UpdateRoleDto.go
deleted file mode 100644
index ff7f13b..0000000
--- a/dto/UpdateRoleDto.go
+++ /dev/null
@@ -1,10 +0,0 @@
-package dto
-
-
-type UpdateRoleDto struct{
- NewCode string `json:"newCode"`
- Code string `json:"code"`
- Namespace string `json:"namespace,omitempty"`
- Description string `json:"description,omitempty"`
-}
-
diff --git a/dto/UpdateUserReqDto.go b/dto/UpdateUserReqDto.go
deleted file mode 100644
index 456608d..0000000
--- a/dto/UpdateUserReqDto.go
+++ /dev/null
@@ -1,29 +0,0 @@
-package dto
-
-
-type UpdateUserReqDto struct{
- UserId string `json:"userId"`
- PhoneCountryCode string `json:"phoneCountryCode,omitempty"`
- Name string `json:"name,omitempty"`
- Nickname string `json:"nickname,omitempty"`
- Photo string `json:"photo,omitempty"`
- ExternalId string `json:"externalId,omitempty"`
- Status string `json:"status,omitempty"`
- EmailVerified bool `json:"emailVerified,omitempty"`
- PhoneVerified bool `json:"phoneVerified,omitempty"`
- Birthdate string `json:"birthdate,omitempty"`
- Country string `json:"country,omitempty"`
- Province string `json:"province,omitempty"`
- City string `json:"city,omitempty"`
- Address string `json:"address,omitempty"`
- StreetAddress string `json:"streetAddress,omitempty"`
- PostalCode string `json:"postalCode,omitempty"`
- Gender string `json:"gender,omitempty"`
- Username string `json:"username,omitempty"`
- PasswordEncryptType string `json:"passwordEncryptType,omitempty"`
- Email string `json:"email,omitempty"`
- Phone string `json:"phone,omitempty"`
- Password string `json:"password,omitempty"`
- CustomData interface{} `json:"customData,omitempty"`
-}
-
diff --git a/dto/UserDepartmentPaginatedRespDto.go b/dto/UserDepartmentPaginatedRespDto.go
deleted file mode 100644
index aa2d578..0000000
--- a/dto/UserDepartmentPaginatedRespDto.go
+++ /dev/null
@@ -1,10 +0,0 @@
-package dto
-
-
-type UserDepartmentPaginatedRespDto struct{
- StatusCode int `json:"statusCode"`
- Message string `json:"message"`
- ApiCode int `json:"apiCode,omitempty"`
- Data UserDepartmentPagingDto `json:"data"`
-}
-
diff --git a/dto/UserDepartmentPagingDto.go b/dto/UserDepartmentPagingDto.go
deleted file mode 100644
index cb26f97..0000000
--- a/dto/UserDepartmentPagingDto.go
+++ /dev/null
@@ -1,8 +0,0 @@
-package dto
-
-
-type UserDepartmentPagingDto struct{
- TotalCount int `json:"totalCount"`
- List []UserDepartmentRespDto `json:"list"`
-}
-
diff --git a/dto/UserDepartmentRespDto.go b/dto/UserDepartmentRespDto.go
deleted file mode 100644
index 23a01af..0000000
--- a/dto/UserDepartmentRespDto.go
+++ /dev/null
@@ -1,12 +0,0 @@
-package dto
-
-
-type UserDepartmentRespDto struct{
- DepartmentId string `json:"departmentId"`
- Name string `json:"name"`
- Description string `json:"description"`
- IsLeader bool `json:"isLeader"`
- Code string `json:"code"`
- IsMainDepartment bool `json:"isMainDepartment"`
-}
-
diff --git a/dto/UserDto.go b/dto/UserDto.go
deleted file mode 100644
index 7abed37..0000000
--- a/dto/UserDto.go
+++ /dev/null
@@ -1,32 +0,0 @@
-package dto
-
-
-type UserDto struct{
- UserId string `json:"userId"`
- Status string `json:"status"`
- Email string `json:"email,omitempty"`
- Phone string `json:"phone,omitempty"`
- PhoneCountryCode string `json:"phoneCountryCode,omitempty"`
- Username string `json:"username,omitempty"`
- Name string `json:"name,omitempty"`
- Nickname string `json:"nickname,omitempty"`
- Photo string `json:"photo,omitempty"`
- LoginsCount int `json:"loginsCount,omitempty"`
- LastLogin string `json:"lastLogin,omitempty"`
- LastIp string `json:"lastIp,omitempty"`
- Gender string `json:"gender"`
- EmailVerified bool `json:"emailVerified"`
- PhoneVerified bool `json:"phoneVerified"`
- Birthdate string `json:"birthdate,omitempty"`
- Country string `json:"country,omitempty"`
- Province string `json:"province,omitempty"`
- City string `json:"city,omitempty"`
- Address string `json:"address,omitempty"`
- StreetAddress string `json:"streetAddress,omitempty"`
- PostalCode string `json:"postalCode,omitempty"`
- ExternalId string `json:"externalId,omitempty"`
- DepartmentIds []string `json:"departmentIds,omitempty"`
- Identities []IdentityDto `json:"identities,omitempty"`
- CustomData interface{} `json:"customData,omitempty"`
-}
-
diff --git a/dto/UserIdListRespDto.go b/dto/UserIdListRespDto.go
deleted file mode 100644
index 76c2833..0000000
--- a/dto/UserIdListRespDto.go
+++ /dev/null
@@ -1,10 +0,0 @@
-package dto
-
-
-type UserIdListRespDto struct{
- StatusCode int `json:"statusCode"`
- Message string `json:"message"`
- ApiCode int `json:"apiCode,omitempty"`
- Data []string `json:"data"`
-}
-
diff --git a/dto/UserListRespDto.go b/dto/UserListRespDto.go
deleted file mode 100644
index 8829db9..0000000
--- a/dto/UserListRespDto.go
+++ /dev/null
@@ -1,10 +0,0 @@
-package dto
-
-
-type UserListRespDto struct{
- StatusCode int `json:"statusCode"`
- Message string `json:"message"`
- ApiCode int `json:"apiCode,omitempty"`
- Data UsersListPagingDto `json:"data"`
-}
-
diff --git a/dto/UserLoggedInAppsDto.go b/dto/UserLoggedInAppsDto.go
deleted file mode 100644
index 82a9e72..0000000
--- a/dto/UserLoggedInAppsDto.go
+++ /dev/null
@@ -1,10 +0,0 @@
-package dto
-
-
-type UserLoggedInAppsDto struct{
- AppId string `json:"appId"`
- AppName string `json:"appName"`
- AppLogo string `json:"appLogo"`
- AppLoginUrl string `json:"appLoginUrl"`
-}
-
diff --git a/dto/UserLoggedInAppsListRespDto.go b/dto/UserLoggedInAppsListRespDto.go
deleted file mode 100644
index e52281b..0000000
--- a/dto/UserLoggedInAppsListRespDto.go
+++ /dev/null
@@ -1,10 +0,0 @@
-package dto
-
-
-type UserLoggedInAppsListRespDto struct{
- StatusCode int `json:"statusCode"`
- Message string `json:"message"`
- ApiCode int `json:"apiCode,omitempty"`
- Data []UserLoggedInAppsDto `json:"data"`
-}
-
diff --git a/dto/UserLoginHistoryDto.go b/dto/UserLoginHistoryDto.go
deleted file mode 100644
index 73a838d..0000000
--- a/dto/UserLoginHistoryDto.go
+++ /dev/null
@@ -1,13 +0,0 @@
-package dto
-
-
-type UserLoginHistoryDto struct{
- AppId string `json:"appId"`
- AppName string `json:"appName"`
- AppLogo string `json:"appLogo"`
- AppLoginUrl string `json:"appLoginUrl"`
- ClientIp string `json:"clientIp"`
- UserAgent string `json:"userAgent,omitempty"`
- Time string `json:"time"`
-}
-
diff --git a/dto/UserLoginHistoryPaginatedRespDto.go b/dto/UserLoginHistoryPaginatedRespDto.go
deleted file mode 100644
index c70a6d8..0000000
--- a/dto/UserLoginHistoryPaginatedRespDto.go
+++ /dev/null
@@ -1,10 +0,0 @@
-package dto
-
-
-type UserLoginHistoryPaginatedRespDto struct{
- StatusCode int `json:"statusCode"`
- Message string `json:"message"`
- ApiCode int `json:"apiCode,omitempty"`
- Data UserLoginHistoryPagingDto `json:"data"`
-}
-
diff --git a/dto/UserLoginHistoryPagingDto.go b/dto/UserLoginHistoryPagingDto.go
deleted file mode 100644
index 5b77bb6..0000000
--- a/dto/UserLoginHistoryPagingDto.go
+++ /dev/null
@@ -1,8 +0,0 @@
-package dto
-
-
-type UserLoginHistoryPagingDto struct{
- TotalCount int `json:"totalCount"`
- List []UserLoginHistoryDto `json:"list"`
-}
-
diff --git a/dto/UserMfaRespDto.go b/dto/UserMfaRespDto.go
deleted file mode 100644
index 0d420f1..0000000
--- a/dto/UserMfaRespDto.go
+++ /dev/null
@@ -1,8 +0,0 @@
-package dto
-
-
-type UserMfaRespDto struct{
- TotpStatus string `json:"totpStatus"`
- FaceMfaStatus string `json:"faceMfaStatus"`
-}
-
diff --git a/dto/UserMfaSingleRespDto.go b/dto/UserMfaSingleRespDto.go
deleted file mode 100644
index f3e7877..0000000
--- a/dto/UserMfaSingleRespDto.go
+++ /dev/null
@@ -1,10 +0,0 @@
-package dto
-
-
-type UserMfaSingleRespDto struct{
- StatusCode int `json:"statusCode"`
- Message string `json:"message"`
- ApiCode int `json:"apiCode,omitempty"`
- Data UserMfaRespDto `json:"data"`
-}
-
diff --git a/dto/UserPaginatedRespDto.go b/dto/UserPaginatedRespDto.go
deleted file mode 100644
index de48190..0000000
--- a/dto/UserPaginatedRespDto.go
+++ /dev/null
@@ -1,10 +0,0 @@
-package dto
-
-
-type UserPaginatedRespDto struct{
- StatusCode int `json:"statusCode"`
- Message string `json:"message"`
- ApiCode int `json:"apiCode,omitempty"`
- Data UserPagingDto `json:"data"`
-}
-
diff --git a/dto/UserPagingDto.go b/dto/UserPagingDto.go
deleted file mode 100644
index eacbd8b..0000000
--- a/dto/UserPagingDto.go
+++ /dev/null
@@ -1,8 +0,0 @@
-package dto
-
-
-type UserPagingDto struct{
- TotalCount int `json:"totalCount"`
- List []UserDto `json:"list"`
-}
-
diff --git a/dto/UserSingleRespDto.go b/dto/UserSingleRespDto.go
deleted file mode 100644
index bd070bc..0000000
--- a/dto/UserSingleRespDto.go
+++ /dev/null
@@ -1,10 +0,0 @@
-package dto
-
-
-type UserSingleRespDto struct{
- StatusCode int `json:"statusCode"`
- Message string `json:"message"`
- ApiCode int `json:"apiCode,omitempty"`
- Data UserDto `json:"data"`
-}
-
diff --git a/dto/UsersListPagingDto.go b/dto/UsersListPagingDto.go
deleted file mode 100644
index 9090f73..0000000
--- a/dto/UsersListPagingDto.go
+++ /dev/null
@@ -1,8 +0,0 @@
-package dto
-
-
-type UsersListPagingDto struct{
- TotalCount int `json:"totalCount"`
- List []UserDto `json:"list"`
-}
-
diff --git a/go.mod b/go.mod
index 546b9c7..bd654f9 100644
--- a/go.mod
+++ b/go.mod
@@ -1,10 +1,16 @@
-module authing-go-sdk
-
-go 1.8
+module github.com/Authing/authing-go-sdk
require (
- github.com/dgrijalva/jwt-go v3.2.0+incompatible // indirect
- github.com/klauspost/compress v1.15.2 // indirect
+ github.com/bitly/go-simplejson v0.5.0
+ github.com/bmizerany/assert v0.0.0-20160611221934-b7ed37b82869 // indirect
+ github.com/json-iterator/go v1.1.11
+ github.com/kr/pretty v0.2.0 // indirect
github.com/patrickmn/go-cache v2.1.0+incompatible
- github.com/valyala/fasthttp v1.36.0
+ github.com/valyala/fasthttp v1.26.0
+ golang.org/x/net v0.0.0-20210525063256-abc453219eb5 // indirect
+ golang.org/x/oauth2 v0.0.0-20210514164344-f6687ab2804c
+ gopkg.in/check.v1 v1.0.0-20190902080502-41f04d3bba15 // indirect
+ gopkg.in/yaml.v2 v2.4.0 // indirect
)
+
+go 1.8
diff --git a/gql/schemas.gql b/gql/schemas.gql
new file mode 100644
index 0000000..b179658
--- /dev/null
+++ b/gql/schemas.gql
@@ -0,0 +1,2115 @@
+directive @date(format: String) on FIELD_DEFINITION
+
+directive @constraint(minLength: Int, maxLength: Int, startsWith: String, endsWith: String, contains: String, notContains: String, pattern: String, format: String, min: Int, max: Int, exclusiveMin: Int, exclusiveMax: Int, multipleOf: Int) on INPUT_FIELD_DEFINITION | FIELD_DEFINITION
+
+type AccessTokenRes {
+ accessToken: String
+ exp: Int
+ iat: Int
+}
+
+type App2WxappLoginStrategy {
+ ticketExpriresAfter: Int
+ ticketExchangeUserInfoNeedSecret: Boolean
+}
+
+input App2WxappLoginStrategyInput {
+ ticketExpriresAfter: Int
+ ticketExchangeUserInfoNeedSecret: Boolean
+}
+
+type AuthorizedResource {
+ code: String!
+ type: ResourceType
+ actions: [String!]
+}
+
+input AuthorizedTargetsActionsInput {
+ op: Operator!
+ list: [String]!
+}
+
+input AuthorizeResourceOpt {
+ targetType: PolicyAssignmentTargetType!
+ targetIdentifier: String!
+ actions: [String!]
+}
+
+"""批量删除返回结果"""
+type BatchOperationResult {
+ """删除成功的个数"""
+ succeedCount: Int!
+
+ """删除失败的个数"""
+ failedCount: Int!
+ message: String
+ errors: [String!]
+}
+
+type ChangeEmailStrategy {
+ verifyOldEmail: Boolean
+}
+
+input ChangeEmailStrategyInput {
+ verifyOldEmail: Boolean
+}
+
+type ChangePhoneStrategy {
+ verifyOldPhone: Boolean
+}
+
+input ChangePhoneStrategyInput {
+ verifyOldPhone: Boolean
+}
+
+type CheckPasswordStrengthResult {
+ valid: Boolean!
+ message: String
+}
+
+type CommonMessage {
+ """可读的接口响应说明,请以业务状态码 code 作为判断业务是否成功的标志"""
+ message: String
+
+ """
+ 业务状态码(与 HTTP 响应码不同),但且仅当为 200 的时候表示操作成功表示,详细说明请见:
+ [Authing 错误代码列表](https://docs.authing.co/advanced/error-code.html)
+ """
+ code: Int
+}
+
+input ConfigEmailTemplateInput {
+ """邮件模版类型"""
+ type: EmailTemplateType!
+
+ """模版名称"""
+ name: String!
+
+ """邮件主题"""
+ subject: String!
+
+ """显示的邮件发送人"""
+ sender: String!
+
+ """邮件模版内容"""
+ content: String!
+
+ """重定向链接,操作成功后,用户将被重定向到此 URL。"""
+ redirectTo: String
+ hasURL: Boolean
+
+ """验证码过期时间(单位为秒)"""
+ expiresIn: Int
+}
+
+input CreateFunctionInput {
+ """函数名称"""
+ name: String!
+
+ """源代码"""
+ sourceCode: String!
+
+ """描述信息"""
+ description: String
+
+ """云函数链接"""
+ url: String
+}
+
+input CreateSocialConnectionInput {
+ provider: String!
+ name: String!
+ logo: String!
+ description: String
+ fields: [SocialConnectionFieldInput!]
+}
+
+input CreateSocialConnectionInstanceFieldInput {
+ key: String!
+ value: String!
+}
+
+input CreateSocialConnectionInstanceInput {
+ """社会化登录 provider"""
+ provider: String!
+ fields: [CreateSocialConnectionInstanceFieldInput]
+}
+
+input CreateUserInput {
+ """用户名,用户池内唯一"""
+ username: String
+
+ """邮箱,不区分大小写,如 Bob@example.com 和 bob@example.com 会识别为同一个邮箱。用户池内唯一。"""
+ email: String
+
+ """邮箱是否已验证"""
+ emailVerified: Boolean
+
+ """手机号,用户池内唯一"""
+ phone: String
+
+ """手机号是否已验证"""
+ phoneVerified: Boolean
+ unionid: String
+ openid: String
+
+ """昵称,该字段不唯一。"""
+ nickname: String
+
+ """头像链接,默认为 https://usercontents.authing.cn/authing-avatar.png"""
+ photo: String
+ password: String
+
+ """注册方式"""
+ registerSource: [String!]
+ browser: String
+
+ """用户社会化登录第三方身份提供商返回的原始用户信息,非社会化登录方式注册的用户此字段为空。"""
+ oauth: String
+
+ """用户累计登录次数,当你从你原有用户系统向 Authing 迁移的时候可以设置此字段。"""
+ loginsCount: Int
+ lastLogin: String
+ company: String
+ lastIP: String
+
+ """用户注册时间,当你从你原有用户系统向 Authing 迁移的时候可以设置此字段。"""
+ signedUp: String
+ blocked: Boolean
+ isDeleted: Boolean
+ device: String
+ name: String
+ givenName: String
+ familyName: String
+ middleName: String
+ profile: String
+ preferredUsername: String
+ website: String
+ gender: String
+ birthdate: String
+ zoneinfo: String
+ locale: String
+ address: String
+ formatted: String
+ streetAddress: String
+ locality: String
+ region: String
+ postalCode: String
+ country: String
+ externalId: String
+}
+
+type CustomSMSProvider {
+ enabled: Boolean
+ provider: String
+ config: String
+}
+
+input CustomSMSProviderInput {
+ enabled: Boolean
+ provider: String
+ config: String
+}
+
+"""邮件使用场景"""
+enum EmailScene {
+ """发送重置密码邮件,邮件中包含验证码"""
+ RESET_PASSWORD
+
+ """发送验证邮箱的邮件"""
+ VERIFY_EMAIL
+
+ """发送修改邮箱邮件,邮件中包含验证码"""
+ CHANGE_EMAIL
+
+ """发送 MFA 验证邮件"""
+ MFA_VERIFY
+}
+
+type EmailTemplate {
+ """邮件模版类型"""
+ type: EmailTemplateType!
+
+ """模版名称"""
+ name: String!
+
+ """邮件主题"""
+ subject: String!
+
+ """显示的邮件发送人"""
+ sender: String!
+
+ """邮件模版内容"""
+ content: String!
+
+ """重定向链接,操作成功后,用户将被重定向到此 URL。"""
+ redirectTo: String
+ hasURL: Boolean
+
+ """验证码过期时间(单位为秒)"""
+ expiresIn: Int
+
+ """是否开启(自定义模版)"""
+ enabled: Boolean
+
+ """是否是系统默认模版"""
+ isSystem: Boolean
+}
+
+enum EmailTemplateType {
+ """重置密码确认"""
+ RESET_PASSWORD
+
+ """重置密码通知"""
+ PASSWORD_RESETED_NOTIFICATION
+
+ """修改密码验证码"""
+ CHANGE_PASSWORD
+
+ """注册欢迎邮件"""
+ WELCOME
+
+ """验证邮箱"""
+ VERIFY_EMAIL
+
+ """修改绑定邮箱"""
+ CHANGE_EMAIL
+}
+
+type FrequentRegisterCheckConfig {
+ timeInterval: Int
+ limit: Int
+ enabled: Boolean
+}
+
+input FrequentRegisterCheckConfigInput {
+ timeInterval: Int
+ limit: Int
+ enabled: Boolean
+}
+
+"""函数"""
+type Function {
+ """ID"""
+ id: String!
+
+ """函数名称"""
+ name: String!
+
+ """源代码"""
+ sourceCode: String!
+
+ """描述信息"""
+ description: String
+
+ """云函数链接"""
+ url: String
+}
+
+type Group {
+ """唯一标志 code"""
+ code: String!
+
+ """名称"""
+ name: String!
+
+ """描述"""
+ description: String
+
+ """创建时间"""
+ createdAt: String
+
+ """修改时间"""
+ updatedAt: String
+
+ """包含的用户列表"""
+ users(page: Int, limit: Int): PaginatedUsers!
+
+ """被授权访问的所有资源"""
+ authorizedResources(namespace: String, resourceType: String): PaginatedAuthorizedResources
+}
+
+type Identity {
+ openid: String
+ userIdInIdp: String
+ userId: String
+ extIdpId: String
+ isSocial: Boolean
+ provider: String
+ userPoolId: String
+ refreshToken: String
+ accessToken: String
+}
+
+type JWTTokenStatus {
+ code: Int
+ message: String
+ status: Boolean
+ exp: Int
+ iat: Int
+ data: JWTTokenStatusDetail
+}
+
+type JWTTokenStatusDetail {
+ id: String
+ userPoolId: String
+ arn: String
+}
+
+type KeyValuePair {
+ key: String!
+ value: String!
+}
+
+input LoginByEmailInput {
+ email: String!
+ password: String!
+
+ """图形验证码"""
+ captchaCode: String
+
+ """如果用户不存在,是否自动创建一个账号"""
+ autoRegister: Boolean
+ clientIp: String
+
+ """设置用户自定义字段,要求符合 Array<{ key: string; value: string }> 格式"""
+ params: String
+
+ """请求上下文信息,将会传递到 pipeline 中"""
+ context: String
+}
+
+input LoginByPhoneCodeInput {
+ phone: String!
+ code: String!
+
+ """如果用户不存在,是否自动创建一个账号"""
+ autoRegister: Boolean
+ clientIp: String
+
+ """设置用户自定义字段,要求符合 Array<{ key: string; value: string }> 格式"""
+ params: String
+
+ """请求上下文信息,将会传递到 pipeline 中"""
+ context: String
+}
+
+input LoginByPhonePasswordInput {
+ phone: String!
+ password: String!
+
+ """图形验证码"""
+ captchaCode: String
+
+ """如果用户不存在,是否自动创建一个账号"""
+ autoRegister: Boolean
+ clientIp: String
+
+ """设置用户自定义字段,要求符合 Array<{ key: string; value: string }> 格式"""
+ params: String
+
+ """请求上下文信息,将会传递到 pipeline 中"""
+ context: String
+}
+
+input LoginByUsernameInput {
+ username: String!
+ password: String!
+
+ """图形验证码"""
+ captchaCode: String
+
+ """如果用户不存在,是否自动创建一个账号"""
+ autoRegister: Boolean
+ clientIp: String
+
+ """设置用户自定义字段,要求符合 Array<{ key: string; value: string }> 格式"""
+ params: String
+
+ """请求上下文信息,将会传递到 pipeline 中"""
+ context: String
+}
+
+type LoginFailCheckConfig {
+ timeInterval: Int
+ limit: Int
+ enabled: Boolean
+}
+
+input LoginFailCheckConfigInput {
+ timeInterval: Int
+ limit: Int
+ enabled: Boolean
+}
+
+type LoginPasswordFailCheckConfig {
+ timeInterval: Int
+ limit: Int
+ enabled: Boolean
+}
+
+input LoginPasswordFailCheckConfigInput {
+ timeInterval: Int
+ limit: Int
+ enabled: Boolean
+}
+
+type Mfa {
+ """MFA ID"""
+ id: String!
+
+ """用户 ID"""
+ userId: String!
+
+ """用户池 ID"""
+ userPoolId: String!
+
+ """是否开启 MFA"""
+ enable: Boolean!
+
+ """密钥"""
+ secret: String
+}
+
+type Mutation {
+ """允许操作某个资源"""
+ allow(
+ resource: String!
+ action: String!
+ userId: String
+ userIds: [String!]
+ roleCode: String
+ roleCodes: [String!]
+
+ """权限组 code"""
+ namespace: String
+ ): CommonMessage!
+
+ """将一个(类)资源授权给用户、角色、分组、组织机构,且可以分别指定不同的操作权限。"""
+ authorizeResource(
+ """权限分组"""
+ namespace: String
+
+ """资源类型"""
+ resourceType: ResourceType
+
+ """资源 code"""
+ resource: String
+ opts: [AuthorizeResourceOpt]
+ ): CommonMessage!
+
+ """配置社会化登录"""
+ createSocialConnectionInstance(input: CreateSocialConnectionInstanceInput!): SocialConnectionInstance!
+
+ """开启社会化登录"""
+ enableSocialConnectionInstance(provider: String!): SocialConnectionInstance!
+
+ """关闭社会化登录"""
+ disableSocialConnectionInstance(provider: String!): SocialConnectionInstance!
+
+ """设置用户在某个组织机构内所在的主部门"""
+ setMainDepartment(userId: String!, departmentId: String): CommonMessage!
+
+ """配置自定义邮件模版"""
+ configEmailTemplate(input: ConfigEmailTemplateInput!): EmailTemplate!
+
+ """启用自定义邮件模版"""
+ enableEmailTemplate(
+ """邮件模版类型"""
+ type: EmailTemplateType!
+ ): EmailTemplate!
+
+ """停用自定义邮件模版(将会使用系统默认邮件模版)"""
+ disableEmailTemplate(
+ """邮件模版类型"""
+ type: EmailTemplateType!
+ ): EmailTemplate!
+
+ """发送邮件"""
+ sendEmail(email: String!, scene: EmailScene!): CommonMessage!
+
+ """管理员发送首次登录验证邮件"""
+ sendFirstLoginVerifyEmail(userId: String!, appId: String!): CommonMessage!
+
+ """创建函数"""
+ createFunction(input: CreateFunctionInput!): Function
+
+ """修改函数"""
+ updateFunction(input: UpdateFunctionInput!): Function!
+ deleteFunction(id: String!): CommonMessage!
+ addUserToGroup(
+ """用户 ID,如果不填返回用户池的权限列表"""
+ userIds: [String!]!
+ code: String
+ ): CommonMessage!
+ removeUserFromGroup(
+ """用户 ID,如果不填返回用户池的权限列表"""
+ userIds: [String!]!
+ code: String
+ ): CommonMessage!
+
+ """创建角色"""
+ createGroup(
+ """唯一标志"""
+ code: String!
+
+ """名称"""
+ name: String!
+
+ """描述"""
+ description: String
+ ): Group!
+
+ """修改角色"""
+ updateGroup(code: String!, name: String, description: String, newCode: String): Group!
+
+ """批量删除角色"""
+ deleteGroups(codeList: [String!]!): CommonMessage!
+ loginByEmail(input: LoginByEmailInput!): User
+ loginByUsername(input: LoginByUsernameInput!): User
+ loginByPhoneCode(input: LoginByPhoneCodeInput!): User
+ loginByPhonePassword(input: LoginByPhonePasswordInput!): User
+
+ """修改 MFA 信息"""
+ changeMfa(
+ """是否开启 MFA"""
+ enable: Boolean
+
+ """MFA ID"""
+ id: String
+
+ """用户 ID"""
+ userId: String
+
+ """用户池 ID"""
+ userPoolId: String
+
+ """是否刷新密钥"""
+ refresh: Boolean
+ ): Mfa
+
+ """创建组织机构"""
+ createOrg(
+ """组织机构名称"""
+ name: String!
+
+ """唯一标志,会作为根节点的 code"""
+ code: String
+
+ """描述信息,可选。"""
+ description: String
+ ): Org!
+
+ """删除组织机构"""
+ deleteOrg(
+ """组织机构 ID"""
+ id: String!
+ ): CommonMessage!
+
+ """添加子节点"""
+ addNode(
+ """组织机构 ID"""
+ orgId: String!
+
+ """父节点 ID,不填默认为根节点"""
+ parentNodeId: String
+
+ """节点名称"""
+ name: String!
+
+ """多语言名称,**key** 为标准 **i18n** 语言编码,**value** 为对应语言的名称。"""
+ nameI18n: String
+
+ """描述信息"""
+ description: String
+
+ """多语言描述信息"""
+ descriptionI18n: String
+
+ """在父节点中的次序值。**order** 值大的排序靠前。有效的值范围是[0, 2^32)"""
+ order: Int
+
+ """节点唯一标志码,可以通过 code 进行搜索"""
+ code: String
+ ): Org!
+
+ """添加子节点"""
+ addNodeV2(
+ """组织机构 ID"""
+ orgId: String!
+
+ """父节点 ID,不填默认为根节点"""
+ parentNodeId: String
+
+ """节点名称"""
+ name: String!
+
+ """多语言名称,**key** 为标准 **i18n** 语言编码,**value** 为对应语言的名称。"""
+ nameI18n: String
+
+ """描述信息"""
+ description: String
+
+ """多语言描述信息"""
+ descriptionI18n: String
+
+ """在父节点中的次序值。**order** 值大的排序靠前。有效的值范围是[0, 2^32)"""
+ order: Int
+
+ """节点唯一标志码,可以通过 code 进行搜索"""
+ code: String
+ ): Node!
+
+ """修改节点"""
+ updateNode(id: String!, name: String, code: String, description: String): Node!
+
+ """删除节点(会一并删掉子节点)"""
+ deleteNode(
+ """组织机构 ID"""
+ orgId: String!
+
+ """节点 ID"""
+ nodeId: String!
+ ): CommonMessage!
+
+ """(批量)将成员添加到节点中"""
+ addMember(
+ """节点 ID"""
+ nodeId: String
+
+ """组织机构 ID"""
+ orgId: String
+
+ """节点 Code"""
+ nodeCode: String
+
+ """用户 ID 列表"""
+ userIds: [String!]!
+
+ """是否设置为 Leade"""
+ isLeader: Boolean = false
+ ): Node!
+
+ """(批量)将成员从节点中移除"""
+ removeMember(
+ """节点 ID"""
+ nodeId: String
+
+ """组织机构 ID"""
+ orgId: String
+
+ """节点 Code"""
+ nodeCode: String
+
+ """用户 ID 列表"""
+ userIds: [String!]!
+ ): Node!
+ moveMembers(userIds: [String!]!, sourceNodeId: String!, targetNodeId: String!): CommonMessage
+ moveNode(
+ """组织机构 ID"""
+ orgId: String!
+
+ """需要移动的节点 ID"""
+ nodeId: String!
+
+ """目标父节点 ID"""
+ targetParentId: String!
+ ): Org!
+ resetPassword(
+ """手机号"""
+ phone: String
+
+ """邮箱"""
+ email: String
+
+ """手机号验证码 / 邮件验证码"""
+ code: String!
+
+ """加密过后的新密码"""
+ newPassword: String!
+ ): CommonMessage
+
+ """通过首次登录的 Token 重置密码"""
+ resetPasswordByFirstLoginToken(token: String!, password: String!): CommonMessage
+ createPolicy(
+ """权限组 code"""
+ namespace: String
+ code: String!
+ description: String
+ statements: [PolicyStatementInput!]!
+ ): Policy!
+ updatePolicy(
+ """权限组 code"""
+ namespace: String
+ code: String!
+ description: String
+ statements: [PolicyStatementInput!]
+ newCode: String
+ ): Policy!
+ deletePolicy(code: String!, namespace: String): CommonMessage!
+ deletePolicies(codeList: [String!]!, namespace: String): CommonMessage!
+ addPolicyAssignments(
+ policies: [String!]!
+ targetType: PolicyAssignmentTargetType!
+ targetIdentifiers: [String!]
+
+ """是否被子节点继承(此参数只在授权对象为组织机构时有效)"""
+ inheritByChildren: Boolean
+
+ """权限组 code"""
+ namespace: String
+ ): CommonMessage!
+
+ """开启授权"""
+ enablePolicyAssignment(
+ """策略的 code"""
+ policy: String!
+
+ """目标对象类型"""
+ targetType: PolicyAssignmentTargetType!
+
+ """目标对象的唯一标志符"""
+ targetIdentifier: String!
+
+ """权限组 code"""
+ namespace: String
+ ): CommonMessage!
+
+ """开启授权"""
+ disbalePolicyAssignment(
+ """策略的 code"""
+ policy: String!
+
+ """目标对象类型"""
+ targetType: PolicyAssignmentTargetType!
+
+ """目标对象的唯一标志符"""
+ targetIdentifier: String!
+
+ """权限组 code"""
+ namespace: String
+ ): CommonMessage!
+ removePolicyAssignments(
+ policies: [String!]!
+ targetType: PolicyAssignmentTargetType!
+ targetIdentifiers: [String!]
+
+ """权限组 code"""
+ namespace: String
+ ): CommonMessage!
+ registerByUsername(input: RegisterByUsernameInput!): User
+ registerByEmail(input: RegisterByEmailInput!): User
+ registerByPhoneCode(input: RegisterByPhoneCodeInput!): User
+
+ """创建角色"""
+ createRole(
+ """权限组 code"""
+ namespace: String
+
+ """唯一标志"""
+ code: String!
+
+ """描述"""
+ description: String
+
+ """父角色代码"""
+ parent: String
+ ): Role!
+
+ """修改角色"""
+ updateRole(code: String!, description: String, newCode: String, namespace: String): Role!
+
+ """删除角色"""
+ deleteRole(code: String!, namespace: String): CommonMessage!
+
+ """批量删除角色"""
+ deleteRoles(codeList: [String!]!, namespace: String): CommonMessage!
+
+ """给用户授权角色"""
+ assignRole(
+ """权限组 code"""
+ namespace: String
+
+ """角色 code"""
+ roleCode: String
+
+ """角色 code 列表"""
+ roleCodes: [String]
+
+ """用户 ID 列表"""
+ userIds: [String!]
+
+ """角色列表"""
+ groupCodes: [String!]
+
+ """组织机构节点列表"""
+ nodeCodes: [String!]
+ ): CommonMessage
+
+ """撤销角色"""
+ revokeRole(
+ """权限组 code"""
+ namespace: String
+
+ """角色 code"""
+ roleCode: String
+
+ """角色 code 列表"""
+ roleCodes: [String]
+
+ """用户 ID 列表"""
+ userIds: [String!]
+
+ """分组列表"""
+ groupCodes: [String!]
+
+ """组织机构节点列表"""
+ nodeCodes: [String!]
+ ): CommonMessage
+
+ """使用子账号登录"""
+ loginBySubAccount(
+ """子账号用户名"""
+ account: String!
+
+ """子账号密码"""
+ password: String!
+
+ """图形验证码"""
+ captchaCode: String
+
+ """客户端真实 IP"""
+ clientIp: String
+ ): User!
+ setUdf(targetType: UDFTargetType!, key: String!, dataType: UDFDataType!, label: String!, options: String): UserDefinedField!
+ removeUdf(targetType: UDFTargetType!, key: String!): CommonMessage
+ setUdv(targetType: UDFTargetType!, targetId: String!, key: String!, value: String!): [UserDefinedData!]
+ setUdfValueBatch(targetType: UDFTargetType!, input: [SetUdfValueBatchInput!]!): CommonMessage
+ removeUdv(targetType: UDFTargetType!, targetId: String!, key: String!): [UserDefinedData!]
+ setUdvBatch(targetType: UDFTargetType!, targetId: String!, udvList: [UserDefinedDataInput!]): [UserDefinedData!]
+ refreshToken(id: String): RefreshToken
+
+ """创建用户。此接口需要管理员权限,普通用户注册请使用 **register** 接口。"""
+ createUser(
+ userInfo: CreateUserInput!
+
+ """
+ 是否保留密码,不使用 Authing 默认的加密方式。当你希望使用[自定义密码加密函数](https://docs.authing.co/security/config-pwd-encrypt-function.html)或不希望加密密码(不推荐)时可以设置此参数为 true。
+ """
+ keepPassword: Boolean
+
+ """初次登录要求重置密码"""
+ resetPasswordOnFirstLogin: Boolean
+
+ """设置用户自定义字段,要求符合 Array<{ key: string; value: string }> 格式"""
+ params: String
+ ): User!
+
+ """更新用户信息。"""
+ updateUser(
+ """用户 ID"""
+ id: String
+
+ """需要修改的用户字段"""
+ input: UpdateUserInput!
+ ): User!
+
+ """修改用户密码,此接口需要验证原始密码,管理员直接修改请使用 **updateUser** 接口。"""
+ updatePassword(
+ """需要设置的新密码"""
+ newPassword: String!
+
+ """原始密码"""
+ oldPassword: String
+ ): User!
+
+ """绑定手机号,调用此接口需要当前用户未绑定手机号"""
+ bindPhone(
+ """手机号"""
+ phone: String!
+
+ """验证码"""
+ phoneCode: String!
+ ): User!
+
+ """绑定邮箱"""
+ bindEmail(
+ """邮箱"""
+ email: String!
+
+ """验证码"""
+ emailCode: String!
+ ): User!
+
+ """解绑定手机号,调用此接口需要当前用户已绑定手机号并且绑定了其他登录方式"""
+ unbindPhone: User!
+
+ """修改手机号。此接口需要验证手机号验证码,管理员直接修改请使用 **updateUser** 接口。"""
+ updatePhone(
+ """需要更换为的手机号"""
+ phone: String!
+
+ """需要更换为手机号的短信验证码"""
+ phoneCode: String!
+
+ """
+ 原始手机号。如果用户关闭了「修改邮箱时是否验证旧手机」选项,此项可以不填 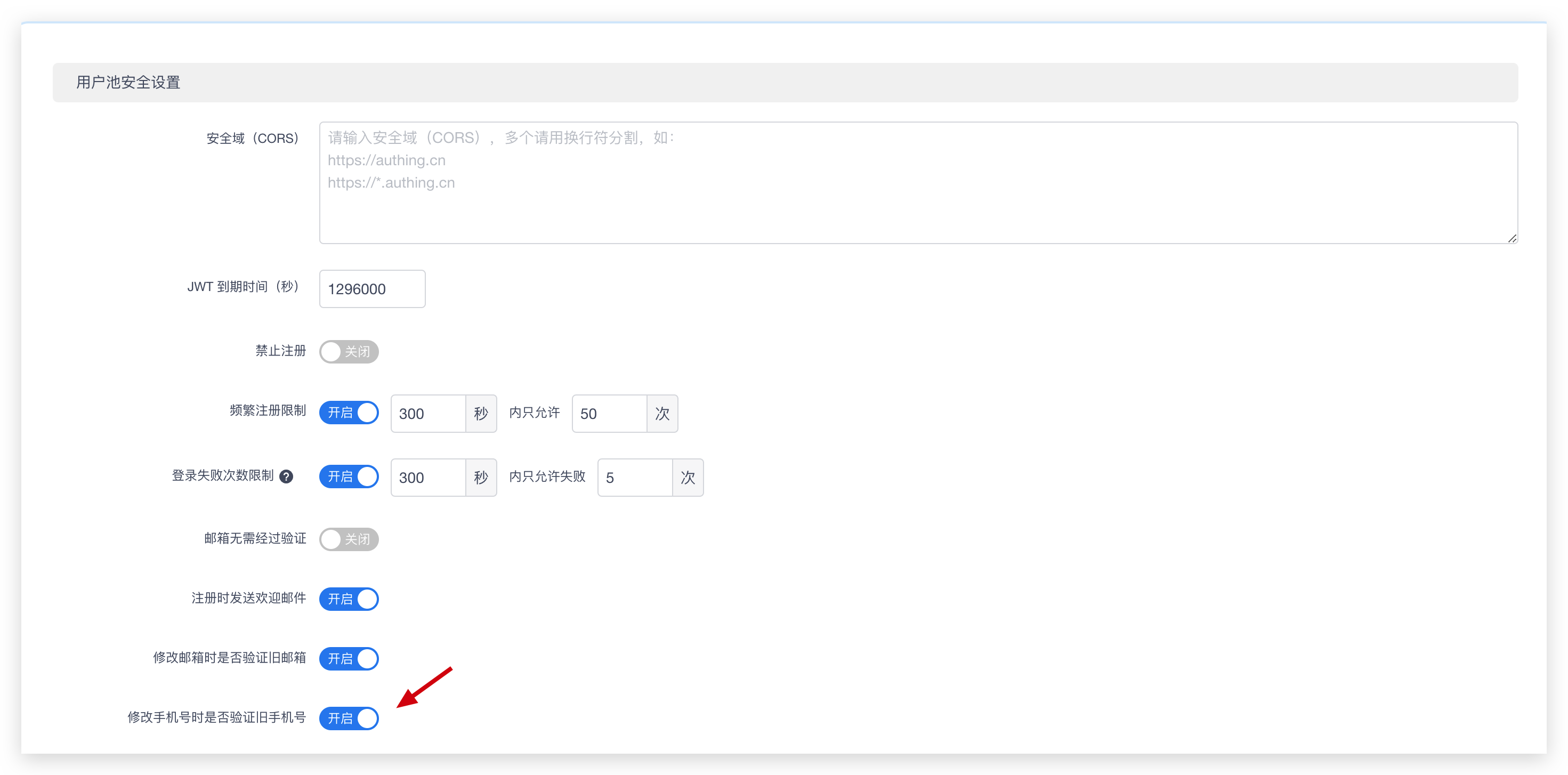
+ """
+ oldPhone: String
+
+ """
+ 原始手机号验证码。如果用户关闭了「修改邮箱时是否验证旧手机」选项,此项可以不填 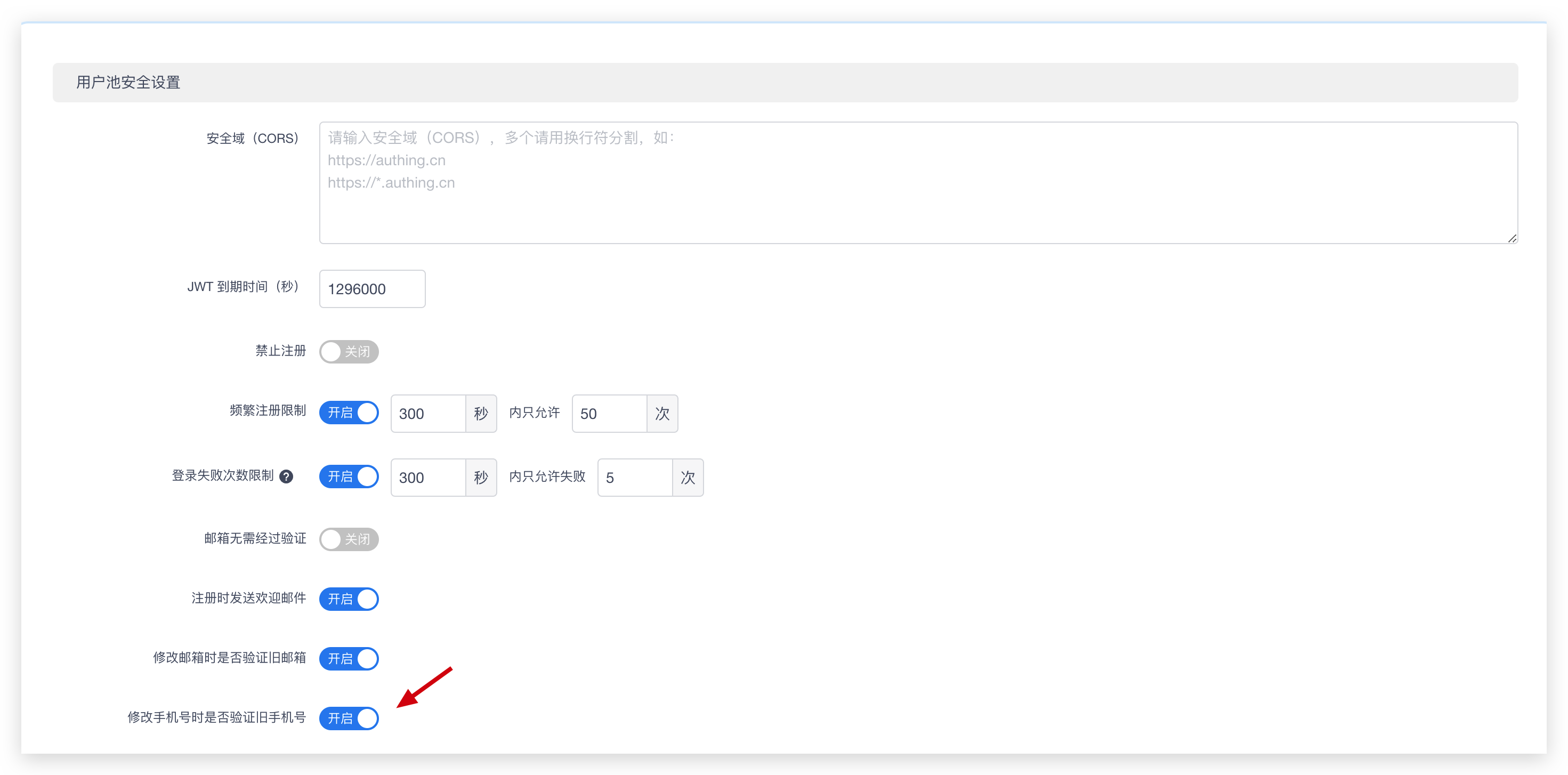
+ """
+ oldPhoneCode: String
+ ): User!
+
+ """修改邮箱。此接口需要验证邮箱验证码,管理员直接修改请使用 updateUser 接口。"""
+ updateEmail(
+ email: String!
+ emailCode: String!
+
+ """
+ 原始邮箱。如果用户关闭了「修改邮箱时是否验证旧邮箱」选项,此项可以不填 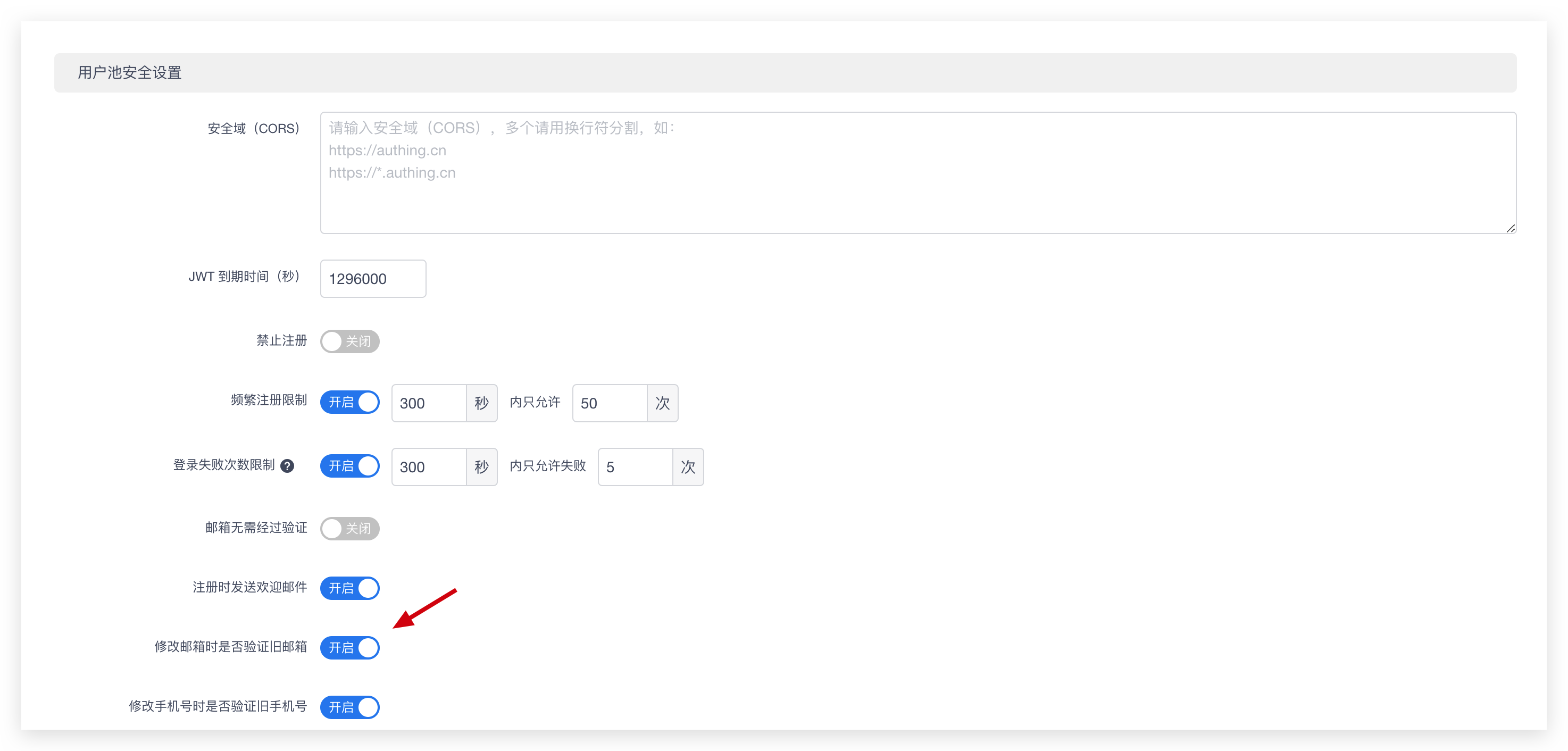
+ """
+ oldEmail: String
+
+ """
+ 原始邮箱验证码。果用户关闭了「修改邮箱时是否验证旧邮箱」选项,此项可以不填 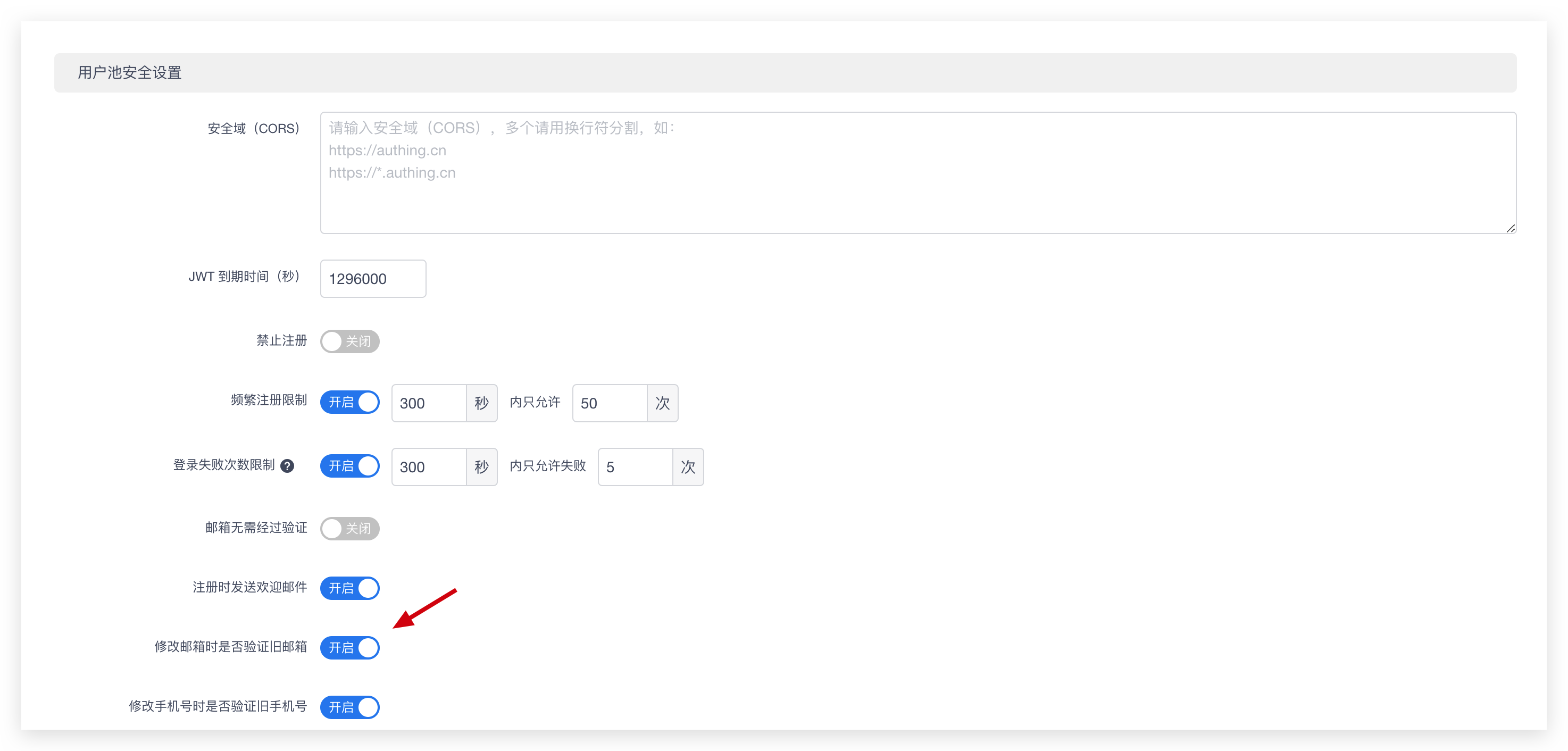
+ """
+ oldEmailCode: String
+ ): User!
+
+ """解绑定邮箱"""
+ unbindEmail: User!
+
+ """删除用户"""
+ deleteUser(
+ """用户 ID"""
+ id: String!
+ ): CommonMessage
+
+ """批量删除用户"""
+ deleteUsers(
+ """用户 ID 列表"""
+ ids: [String!]!
+ ): CommonMessage
+
+ """创建用户池"""
+ createUserpool(
+ """名称"""
+ name: String!
+
+ """二级域名"""
+ domain: String!
+
+ """描述"""
+ description: String
+
+ """用户池 logo"""
+ logo: String
+
+ """用户池类型列表"""
+ userpoolTypes: [String!]
+ ): UserPool!
+ updateUserpool(
+ """更新字段"""
+ input: UpdateUserpoolInput!
+ ): UserPool!
+ refreshUserpoolSecret: String!
+ deleteUserpool: CommonMessage!
+ refreshAccessToken(accessToken: String): RefreshAccessTokenRes!
+ addWhitelist(type: WhitelistType!, list: [String!]!): [WhiteList]!
+ removeWhitelist(type: WhitelistType!, list: [String!]!): [WhiteList]!
+}
+
+type Node {
+ id: String!
+
+ """组织机构 ID"""
+ orgId: String
+
+ """节点名称"""
+ name: String!
+
+ """多语言名称,**key** 为标准 **i18n** 语言编码,**value** 为对应语言的名称。"""
+ nameI18n: String
+
+ """描述信息"""
+ description: String
+
+ """多语言描述信息"""
+ descriptionI18n: String
+
+ """在父节点中的次序值。**order** 值大的排序靠前。有效的值范围是[0, 2^32)"""
+ order: Int
+
+ """节点唯一标志码,可以通过 code 进行搜索"""
+ code: String
+
+ """是否为根节点"""
+ root: Boolean
+
+ """
+ 距离父节点的深度(如果是查询整棵树,返回的 **depth** 为距离根节点的深度,如果是查询某个节点的子节点,返回的 **depth** 指的是距离该节点的深度。)
+ """
+ depth: Int
+ path: [String!]!
+ codePath: [String]!
+ namePath: [String!]!
+ createdAt: String
+ updatedAt: String
+
+ """该节点的子节点 **ID** 列表"""
+ children: [String!]
+
+ """节点的用户列表"""
+ users(
+ """页码数(one-based),默认为 1"""
+ page: Int = 1
+
+ """每页数目,默认为 10"""
+ limit: Int = 10
+
+ """排序方式,默认为 CREATEDAT_DESC(按照创建时间降序)"""
+ sortBy: SortByEnum = CREATEDAT_DESC
+
+ """是否包含子节点的用户,默认为 false"""
+ includeChildrenNodes: Boolean = true
+ ): PaginatedUsers!
+
+ """被授权访问的所有资源"""
+ authorizedResources(namespace: String, resourceType: String): PaginatedAuthorizedResources
+}
+
+scalar Object
+
+enum Operator {
+ AND
+ OR
+}
+
+type Org {
+ """组织机构 ID"""
+ id: String!
+
+ """根节点"""
+ rootNode: Node!
+
+ """组织机构节点列表"""
+ nodes: [Node!]!
+}
+
+type PaginatedAuthorizedResources {
+ totalCount: Int!
+ list: [AuthorizedResource!]!
+}
+
+type PaginatedAuthorizedTargets {
+ list: [ResourcePermissionAssignment]
+ totalCount: Int
+}
+
+type PaginatedDepartments {
+ list: [UserDepartment!]!
+ totalCount: Int!
+}
+
+type PaginatedFunctions {
+ list: [Function!]!
+ totalCount: Int!
+}
+
+type PaginatedGroups {
+ totalCount: Int!
+ list: [Group!]!
+}
+
+type PaginatedOrgs {
+ totalCount: Int!
+ list: [Org!]!
+}
+
+type PaginatedPolicies {
+ totalCount: Int!
+ list: [Policy!]!
+}
+
+type PaginatedPolicyAssignments {
+ totalCount: Int!
+ list: [PolicyAssignment!]!
+}
+
+type PaginatedRoles {
+ totalCount: Int!
+ list: [Role!]!
+}
+
+type PaginatedUserpool {
+ totalCount: Int!
+ list: [UserPool!]!
+}
+
+type PaginatedUsers {
+ totalCount: Int!
+ list: [User!]!
+}
+
+type Policy {
+ """权限组 code"""
+ namespace: String!
+ code: String!
+ isDefault: Boolean!
+ description: String
+ statements: [PolicyStatement!]!
+ createdAt: String
+ updatedAt: String
+
+ """被授权次数"""
+ assignmentsCount: Int!
+
+ """授权记录"""
+ assignments(page: Int, limit: Int, namespace: String): [PolicyAssignment!]!
+}
+
+type PolicyAssignment {
+ code: String!
+ targetType: PolicyAssignmentTargetType!
+ targetIdentifier: String!
+}
+
+enum PolicyAssignmentTargetType {
+ USER
+ ROLE
+ GROUP
+ ORG
+ AK_SK
+}
+
+enum PolicyEffect {
+ ALLOW
+ DENY
+}
+
+type PolicyStatement {
+ resource: String!
+ actions: [String!]!
+ effect: PolicyEffect
+ condition: [PolicyStatementCondition!]
+}
+
+type PolicyStatementCondition {
+ param: String!
+ operator: String!
+ value: Object!
+}
+
+input PolicyStatementConditionInput {
+ param: String!
+ operator: String!
+ value: Object!
+}
+
+input PolicyStatementInput {
+ resource: String!
+ actions: [String!]!
+ effect: PolicyEffect
+ condition: [PolicyStatementConditionInput!]
+}
+
+type QrcodeLoginStrategy {
+ qrcodeExpiresAfter: Int
+ returnFullUserInfo: Boolean
+ allowExchangeUserInfoFromBrowser: Boolean
+ ticketExpiresAfter: Int
+}
+
+input QrcodeLoginStrategyInput {
+ qrcodeExpiresAfter: Int
+ returnFullUserInfo: Boolean
+ allowExchangeUserInfoFromBrowser: Boolean
+ ticketExpiresAfter: Int
+}
+
+type Query {
+ isActionAllowed(
+ resource: String!
+ action: String!
+ userId: String!
+
+ """权限组 code"""
+ namespace: String
+ ): Boolean!
+ isActionDenied(
+ resource: String!
+ action: String!
+ userId: String!
+
+ """权限组 code"""
+ namespace: String
+ ): Boolean!
+ authorizedTargets(
+ namespace: String!
+ resourceType: ResourceType!
+
+ """资源 code"""
+ resource: String!
+ targetType: PolicyAssignmentTargetType
+ actions: AuthorizedTargetsActionsInput
+ ): PaginatedAuthorizedTargets
+ qiniuUptoken(type: String): String
+ isDomainAvaliable(domain: String!): Boolean
+
+ """获取社会化登录定义"""
+ socialConnection(provider: String!): SocialConnection
+
+ """获取所有社会化登录定义"""
+ socialConnections: [SocialConnection!]!
+
+ """获取当前用户池的社会化登录配置"""
+ socialConnectionInstance(provider: String!): SocialConnectionInstance!
+
+ """获取当前用户池的所有社会化登录配置"""
+ socialConnectionInstances: [SocialConnectionInstance!]!
+ emailTemplates: [EmailTemplate!]!
+ previewEmail(type: EmailTemplateType!): String!
+
+ """获取函数模版"""
+ templateCode: String!
+ function(id: String): Function
+ functions(
+ """页码数(one-based),默认为 1"""
+ page: Int = 1
+
+ """每页数目,默认为 10"""
+ limit: Int = 10
+
+ """排序方式,默认为 CREATEDAT_DESC(按照创建时间降序)"""
+ sortBy: SortByEnum = CREATEDAT_DESC
+ ): PaginatedFunctions!
+ group(code: String!): Group
+ groups(
+ """用户 ID,如果不填返回用户池的权限列表"""
+ userId: String
+
+ """页码数(one-based),默认为 1"""
+ page: Int = 1
+
+ """每页数目,默认为 10"""
+ limit: Int = 10
+
+ """排序方式,默认为 CREATEDAT_DESC(按照创建时间降序)"""
+ sortBy: SortByEnum = CREATEDAT_DESC
+ ): PaginatedGroups!
+
+ """查询 MFA 信息"""
+ queryMfa(
+ """MFA ID"""
+ id: String
+
+ """用户 ID"""
+ userId: String
+
+ """用户池 ID"""
+ userPoolId: String
+ ): Mfa
+ nodeById(id: String!): Node
+
+ """通过 code 查询节点"""
+ nodeByCode(
+ """组织机构 ID"""
+ orgId: String!
+
+ """节点在组织机构内的唯一标志"""
+ code: String!
+ ): Node
+
+ """查询组织机构详情"""
+ org(id: String!): Org!
+
+ """查询用户池组织机构列表"""
+ orgs(
+ """页码数(one-based),默认为 1"""
+ page: Int = 1
+
+ """每页数目,默认为 10"""
+ limit: Int = 10
+
+ """排序方式,默认为 CREATEDAT_DESC(按照创建时间降序)"""
+ sortBy: SortByEnum = CREATEDAT_DESC
+ ): PaginatedOrgs!
+
+ """查询子节点列表"""
+ childrenNodes(
+ """组织机构 ID(已废弃)"""
+ orgId: String
+
+ """节点 ID"""
+ nodeId: String!
+ ): [Node!]!
+ rootNode(orgId: String!): Node!
+ isRootNode(nodeId: String!, orgId: String!): Boolean
+ searchNodes(keyword: String!): [Node!]!
+ checkPasswordStrength(password: String!): CheckPasswordStrengthResult!
+ policy(code: String!, namespace: String): Policy
+ policies(page: Int, limit: Int, namespace: String, excludeDefault: Boolean): PaginatedPolicies!
+ policyAssignments(
+ """权限组 code"""
+ namespace: String
+ code: String
+ targetType: PolicyAssignmentTargetType
+ targetIdentifier: String
+ page: Int
+ limit: Int
+ ): PaginatedPolicyAssignments!
+
+ """获取一个对象被授权的资源列表"""
+ authorizedResources(targetType: PolicyAssignmentTargetType, targetIdentifier: String, namespace: String, resourceType: String): PaginatedAuthorizedResources
+
+ """通过 **code** 查询角色详情"""
+ role(code: String!, namespace: String): Role
+
+ """获取角色列表"""
+ roles(
+ """权限组 code"""
+ namespace: String
+
+ """页码数(one-based),默认为 1"""
+ page: Int = 1
+
+ """每页数目,默认为 10"""
+ limit: Int = 10
+
+ """排序方式,默认为 CREATEDAT_DESC(按照创建时间降序)"""
+ sortBy: SortByEnum = CREATEDAT_DESC
+ ): PaginatedRoles!
+
+ """查询某个实体定义的自定义数据"""
+ udv(targetType: UDFTargetType!, targetId: String!): [UserDefinedData!]!
+
+ """查询用户池定义的自定义字段"""
+ udf(targetType: UDFTargetType!): [UserDefinedField!]!
+
+ """批量查询多个对象的自定义数据"""
+ udfValueBatch(targetType: UDFTargetType!, targetIds: [String]!): [UserDefinedDataMap!]!
+ user(
+ """用户 ID"""
+ id: String
+ ): User
+ userBatch(
+ """用户 ID 列表"""
+ ids: [String!]!
+ type: String
+ ): [User!]!
+ users(
+ """页码数(one-based),默认为 1"""
+ page: Int
+
+ """每页数目,默认为 10"""
+ limit: Int
+
+ """排序方式,默认为 CREATEDAT_DESC(按照创建时间降序)"""
+ sortBy: SortByEnum = CREATEDAT_DESC
+ ): PaginatedUsers!
+
+ """已归档的用户列表"""
+ archivedUsers(
+ """页码数(one-based),默认为 1"""
+ page: Int
+
+ """每页数目,默认为 10"""
+ limit: Int
+ ): PaginatedUsers!
+ searchUser(
+ """查询内容"""
+ query: String!
+
+ """搜索的字段"""
+ fields: [String]
+
+ """页码数(one-based),默认为 1"""
+ page: Int
+
+ """每页数目,默认为 10"""
+ limit: Int
+
+ """所在的部门 ID 列表"""
+ departmentOpts: [SearchUserDepartmentOpt]
+
+ """所在的分组列表"""
+ groupOpts: [SearchUserGroupOpt]
+
+ """所在的角色列表"""
+ roleOpts: [SearchUserRoleOpt]
+ ): PaginatedUsers!
+ checkLoginStatus(token: String): JWTTokenStatus
+ isUserExists(email: String, phone: String, username: String, externalId: String): Boolean
+ findUser(email: String, phone: String, username: String, externalId: String): User
+
+ """查询用户池详情"""
+ userpool: UserPool!
+
+ """查询用户池列表"""
+ userpools(
+ """页码数(one-based),默认为 1"""
+ page: Int
+
+ """每页数目,默认为 10"""
+ limit: Int
+
+ """排序方式,默认为 CREATEDAT_DESC(按照创建时间降序)"""
+ sortBy: SortByEnum = CREATEDAT_DESC
+ ): PaginatedUserpool!
+ userpoolTypes: [UserPoolType!]!
+
+ """获取 accessToken ,如 SDK 初始化"""
+ accessToken(userPoolId: String!, secret: String!): AccessTokenRes!
+
+ """用户池注册白名单列表"""
+ whitelist(
+ """白名单类型"""
+ type: WhitelistType!
+ ): [WhiteList!]!
+}
+
+type RefreshAccessTokenRes {
+ accessToken: String
+ exp: Int
+ iat: Int
+}
+
+type RefreshToken {
+ token: String
+ iat: Int
+ exp: Int
+}
+
+input RegisterByEmailInput {
+ email: String!
+ password: String!
+ profile: RegisterProfile
+ forceLogin: Boolean
+ generateToken: Boolean
+ clientIp: String
+
+ """设置用户自定义字段,要求符合 Array<{ key: string; value: string }> 格式"""
+ params: String
+
+ """请求上下文信息,将会传递到 pipeline 中"""
+ context: String
+}
+
+input RegisterByPhoneCodeInput {
+ phone: String!
+ code: String!
+ password: String
+ profile: RegisterProfile
+ forceLogin: Boolean
+ generateToken: Boolean
+ clientIp: String
+
+ """设置用户自定义字段,要求符合 Array<{ key: string; value: string }> 格式"""
+ params: String
+
+ """请求上下文信息,将会传递到 pipeline 中"""
+ context: String
+}
+
+input RegisterByUsernameInput {
+ username: String!
+ password: String!
+ profile: RegisterProfile
+ forceLogin: Boolean
+ generateToken: Boolean
+ clientIp: String
+
+ """设置用户自定义字段,要求符合 Array<{ key: string; value: string }> 格式"""
+ params: String
+
+ """请求上下文信息,将会传递到 pipeline 中"""
+ context: String
+}
+
+input RegisterProfile {
+ ip: String
+ oauth: String
+ username: String
+ nickname: String
+ company: String
+ photo: String
+ device: String
+ browser: String
+ name: String
+ givenName: String
+ familyName: String
+ middleName: String
+ profile: String
+ preferredUsername: String
+ website: String
+ gender: String
+ birthdate: String
+ zoneinfo: String
+ locale: String
+ address: String
+ formatted: String
+ streetAddress: String
+ locality: String
+ region: String
+ postalCode: String
+ country: String
+ udf: [UserDdfInput!]
+}
+
+type RegisterWhiteListConfig {
+ """是否开启手机号注册白名单"""
+ phoneEnabled: Boolean
+
+ """是否开启邮箱注册白名单"""
+ emailEnabled: Boolean
+
+ """是否开用户名注册白名单"""
+ usernameEnabled: Boolean
+}
+
+input RegisterWhiteListConfigInput {
+ phoneEnabled: Boolean
+ emailEnabled: Boolean
+ usernameEnabled: Boolean
+}
+
+type ResourcePermissionAssignment {
+ targetType: PolicyAssignmentTargetType
+ targetIdentifier: String
+ actions: [String!]
+}
+
+enum ResourceType {
+ DATA
+ API
+ MENU
+ UI
+ BUTTON
+}
+
+type Role {
+ id: String!
+
+ """权限组 code"""
+ namespace: String!
+
+ """唯一标志 code"""
+ code: String!
+
+ """资源描述符 arn"""
+ arn: String!
+
+ """角色描述"""
+ description: String
+
+ """是否为系统内建,系统内建的角色不能删除"""
+ isSystem: Boolean
+
+ """创建时间"""
+ createdAt: String
+
+ """修改时间"""
+ updatedAt: String
+
+ """被授予此角色的用户列表"""
+ users(page: Int, limit: Int): PaginatedUsers!
+
+ """被授权访问的所有资源"""
+ authorizedResources(resourceType: String): PaginatedAuthorizedResources
+
+ """父角色"""
+ parent: Role
+}
+
+input SearchUserDepartmentOpt {
+ departmentId: String
+ includeChildrenDepartments: Boolean
+}
+
+input SearchUserGroupOpt {
+ code: String
+}
+
+input SearchUserRoleOpt {
+ namespace: String
+ code: String!
+}
+
+input SetUdfValueBatchInput {
+ targetId: String!
+ key: String!
+ value: Object!
+}
+
+type SocialConnection {
+ """社会化登录服务商唯一标志"""
+ provider: String!
+
+ """名称"""
+ name: String!
+
+ """logo"""
+ logo: String!
+
+ """描述信息"""
+ description: String
+
+ """表单字段"""
+ fields: [SocialConnectionField!]
+}
+
+type SocialConnectionField {
+ key: String
+ label: String
+ type: String
+ placeholder: String
+ children: [SocialConnectionField]
+}
+
+input SocialConnectionFieldInput {
+ key: String
+ label: String
+ type: String
+ placeholder: String
+ children: [SocialConnectionFieldInput]
+}
+
+type SocialConnectionInstance {
+ provider: String!
+ enabled: Boolean!
+ fields: [SocialConnectionInstanceField]
+}
+
+type SocialConnectionInstanceField {
+ key: String!
+ value: String!
+}
+
+enum SortByEnum {
+ """按照创建时间降序(后创建的在前面)"""
+ CREATEDAT_DESC
+
+ """按照创建时间升序(先创建的在前面)"""
+ CREATEDAT_ASC
+
+ """按照更新时间降序(最近更新的在前面)"""
+ UPDATEDAT_DESC
+
+ """按照更新时间升序(最近更新的在后面)"""
+ UPDATEDAT_ASC
+}
+
+enum UDFDataType {
+ STRING
+ NUMBER
+ DATETIME
+ BOOLEAN
+ OBJECT
+}
+
+enum UDFTargetType {
+ NODE
+ ORG
+ USER
+ USERPOOL
+ ROLE
+ PERMISSION
+ APPLICATION
+}
+
+input UpdateFunctionInput {
+ """ID"""
+ id: String!
+
+ """函数名称"""
+ name: String
+
+ """源代码"""
+ sourceCode: String
+
+ """描述信息"""
+ description: String
+
+ """云函数链接"""
+ url: String
+}
+
+input UpdateUserInput {
+ """邮箱。直接修改用户邮箱需要管理员权限,普通用户修改邮箱请使用 **updateEmail** 接口。"""
+ email: String
+ unionid: String
+ openid: String
+
+ """邮箱是否已验证。直接修改 emailVerified 需要管理员权限。"""
+ emailVerified: Boolean
+
+ """手机号。直接修改用户手机号需要管理员权限,普通用户修改邮箱请使用 **updatePhone** 接口。"""
+ phone: String
+
+ """手机号是否已验证。直接修改 **phoneVerified** 需要管理员权限。"""
+ phoneVerified: Boolean
+
+ """用户名,用户池内唯一"""
+ username: String
+
+ """昵称,该字段不唯一。"""
+ nickname: String
+
+ """密码。直接修改用户密码需要管理员权限,普通用户修改邮箱请使用 **updatePassword** 接口。"""
+ password: String
+
+ """头像链接,默认为 https://usercontents.authing.cn/authing-avatar.png"""
+ photo: String
+
+ """注册方式"""
+ company: String
+ browser: String
+ device: String
+ oauth: String
+ tokenExpiredAt: String
+
+ """用户累计登录次数,当你从你原有用户系统向 Authing 迁移的时候可以设置此字段。"""
+ loginsCount: Int
+ lastLogin: String
+ lastIP: String
+
+ """用户注册时间,当你从你原有用户系统向 Authing 迁移的时候可以设置此字段。"""
+ blocked: Boolean
+ name: String
+ givenName: String
+ familyName: String
+ middleName: String
+ profile: String
+ preferredUsername: String
+ website: String
+ gender: String
+ birthdate: String
+ zoneinfo: String
+ locale: String
+ address: String
+ formatted: String
+ streetAddress: String
+ locality: String
+ region: String
+ postalCode: String
+ city: String
+ province: String
+ country: String
+ externalId: String
+}
+
+input UpdateUserpoolInput {
+ name: String
+ logo: String
+ domain: String
+ description: String
+ userpoolTypes: [String!]
+ emailVerifiedDefault: Boolean
+ sendWelcomeEmail: Boolean
+ registerDisabled: Boolean
+
+ """@deprecated"""
+ appSsoEnabled: Boolean
+ allowedOrigins: String
+ tokenExpiresAfter: Int
+ frequentRegisterCheck: FrequentRegisterCheckConfigInput
+ loginFailCheck: LoginFailCheckConfigInput
+ loginFailStrategy: String
+ loginPasswordFailCheck: LoginPasswordFailCheckConfigInput
+ changePhoneStrategy: ChangePhoneStrategyInput
+ changeEmailStrategy: ChangeEmailStrategyInput
+ qrcodeLoginStrategy: QrcodeLoginStrategyInput
+ app2WxappLoginStrategy: App2WxappLoginStrategyInput
+ whitelist: RegisterWhiteListConfigInput
+
+ """自定义短信服务商配置"""
+ customSMSProvider: CustomSMSProviderInput
+
+ """是否要求邮箱必须验证才能登录(如果是通过邮箱登录的话)"""
+ loginRequireEmailVerified: Boolean
+ verifyCodeLength: Int
+}
+
+type User {
+ """用户 ID"""
+ id: String!
+ arn: String!
+
+ """用户在组织机构中的状态"""
+ status: UserStatus
+
+ """用户池 ID"""
+ userPoolId: String!
+
+ """用户名,用户池内唯一"""
+ username: String
+
+ """邮箱,用户池内唯一"""
+ email: String
+
+ """邮箱是否已验证"""
+ emailVerified: Boolean
+
+ """手机号,用户池内唯一"""
+ phone: String
+
+ """手机号是否已验证"""
+ phoneVerified: Boolean
+ unionid: String
+ openid: String
+
+ """用户的身份信息"""
+ identities: [Identity]
+
+ """昵称,该字段不唯一。"""
+ nickname: String
+
+ """注册方式"""
+ registerSource: [String!]
+
+ """头像链接,默认为 https://usercontents.authing.cn/authing-avatar.png"""
+ photo: String
+
+ """用户密码,数据库使用密钥加 salt 进行加密,非原文密码。"""
+ password: String
+
+ """用户社会化登录第三方身份提供商返回的原始用户信息,非社会化登录方式注册的用户此字段为空。"""
+ oauth: String
+
+ """
+ 用户登录凭证,开发者可以在后端检验该 token 的合法性,从而验证用户身份。详细文档请见:[验证 Token](https://docs.authing.co/advanced/verify-jwt-token.html)
+ """
+ token: String
+
+ """token 过期时间"""
+ tokenExpiredAt: String
+
+ """用户登录总次数"""
+ loginsCount: Int
+
+ """用户最近一次登录时间"""
+ lastLogin: String
+
+ """用户上一次登录时使用的 IP"""
+ lastIP: String
+
+ """用户注册时间"""
+ signedUp: String
+
+ """该账号是否被禁用"""
+ blocked: Boolean
+
+ """账号是否被软删除"""
+ isDeleted: Boolean
+ device: String
+ browser: String
+ company: String
+ name: String
+ givenName: String
+ familyName: String
+ middleName: String
+ profile: String
+ preferredUsername: String
+ website: String
+ gender: String
+ birthdate: String
+ zoneinfo: String
+ locale: String
+ address: String
+ formatted: String
+ streetAddress: String
+ locality: String
+ region: String
+ postalCode: String
+ city: String
+ province: String
+ country: String
+ createdAt: String
+ updatedAt: String
+
+ """用户所在的角色列表"""
+ roles(namespace: String): PaginatedRoles
+
+ """用户所在的分组列表"""
+ groups: PaginatedGroups
+
+ """用户所在的部门列表"""
+ departments(orgId: String): PaginatedDepartments
+
+ """被授权访问的所有资源"""
+ authorizedResources(namespace: String, resourceType: String): PaginatedAuthorizedResources
+
+ """用户外部 ID"""
+ externalId: String
+
+ """用户自定义数据"""
+ customData: [UserCustomData]
+}
+
+type UserCustomData {
+ key: String!
+ value: String
+ label: String
+ dataType: UDFDataType!
+}
+
+input UserDdfInput {
+ key: String!
+ value: String!
+}
+
+type UserDefinedData {
+ key: String!
+ dataType: UDFDataType!
+ value: String!
+ label: String
+}
+
+input UserDefinedDataInput {
+ key: String!
+ value: String
+}
+
+type UserDefinedDataMap {
+ targetId: String!
+ data: [UserDefinedData!]!
+}
+
+type UserDefinedField {
+ targetType: UDFTargetType!
+ dataType: UDFDataType!
+ key: String!
+ label: String
+ options: String
+}
+
+type UserDepartment {
+ department: Node!
+
+ """是否为主部门"""
+ isMainDepartment: Boolean!
+
+ """加入该部门的时间"""
+ joinedAt: String
+}
+
+type UserPool {
+ id: String!
+ name: String!
+ domain: String!
+ description: String
+ secret: String!
+ jwtSecret: String!
+ ownerId: String
+ userpoolTypes: [UserPoolType!]
+ logo: String!
+ createdAt: String
+ updatedAt: String
+
+ """用户邮箱是否验证(用户的 emailVerified 字段)默认值,默认为 false"""
+ emailVerifiedDefault: Boolean!
+
+ """用户注册之后是否发送欢迎邮件"""
+ sendWelcomeEmail: Boolean!
+
+ """是否关闭注册"""
+ registerDisabled: Boolean!
+
+ """@deprecated 是否开启用户池下应用间单点登录"""
+ appSsoEnabled: Boolean!
+
+ """
+ 用户池禁止注册后,是否还显示微信小程序扫码登录。当 **showWXMPQRCode** 为 **true** 时,
+ 前端显示小程序码,此时只有以前允许注册时,扫码登录过的用户可以继续登录;新用户扫码无法登录。
+ """
+ showWxQRCodeWhenRegisterDisabled: Boolean
+
+ """前端跨域请求白名单"""
+ allowedOrigins: String
+
+ """用户 **token** 有效时间,单位为秒,默认为 15 天。"""
+ tokenExpiresAfter: Int
+
+ """是否已删除"""
+ isDeleted: Boolean
+
+ """注册频繁检测"""
+ frequentRegisterCheck: FrequentRegisterCheckConfig
+
+ """登录失败检测"""
+ loginFailCheck: LoginFailCheckConfig
+
+ """登录失败检测"""
+ loginPasswordFailCheck: LoginPasswordFailCheckConfig
+
+ """密码安全策略"""
+ loginFailStrategy: String
+
+ """手机号修改策略"""
+ changePhoneStrategy: ChangePhoneStrategy
+
+ """邮箱修改策略"""
+ changeEmailStrategy: ChangeEmailStrategy
+
+ """APP 扫码登录配置"""
+ qrcodeLoginStrategy: QrcodeLoginStrategy
+
+ """APP 拉起小程序登录配置"""
+ app2WxappLoginStrategy: App2WxappLoginStrategy
+
+ """注册白名单配置"""
+ whitelist: RegisterWhiteListConfig
+
+ """自定义短信服务商配置"""
+ customSMSProvider: CustomSMSProvider
+
+ """用户池套餐类型"""
+ packageType: Int
+
+ """是否使用自定义数据库 CUSTOM_USER_STORE 模式"""
+ useCustomUserStore: Boolean
+
+ """是否要求邮箱必须验证才能登录(如果是通过邮箱登录的话)"""
+ loginRequireEmailVerified: Boolean
+
+ """短信验证码长度"""
+ verifyCodeLength: Int
+}
+
+type UserPoolType {
+ code: String
+ name: String
+ description: String
+ image: String
+ sdks: [String]
+}
+
+enum UserStatus {
+ """已停用"""
+ Suspended
+
+ """已离职"""
+ Resigned
+
+ """已激活(正常状态)"""
+ Activated
+
+ """已归档"""
+ Archived
+}
+
+type WhiteList {
+ createdAt: String
+ updatedAt: String
+ value: String!
+}
+
+enum WhitelistType {
+ USERNAME
+ EMAIL
+ PHONE
+}
+
diff --git a/lib/authentication/authentication_client.go b/lib/authentication/authentication_client.go
new file mode 100644
index 0000000..8115162
--- /dev/null
+++ b/lib/authentication/authentication_client.go
@@ -0,0 +1,1918 @@
+package authentication
+
+import (
+ "bytes"
+ "crypto/sha256"
+ "encoding/base64"
+ "encoding/json"
+ "errors"
+ "fmt"
+ "github.com/Authing/authing-go-sdk/lib/constant"
+ "github.com/Authing/authing-go-sdk/lib/model"
+ "github.com/Authing/authing-go-sdk/lib/util"
+ "github.com/Authing/authing-go-sdk/lib/util/cacheutil"
+ simplejson "github.com/bitly/go-simplejson"
+ jsoniter "github.com/json-iterator/go"
+ "io/ioutil"
+ "net/http"
+ "regexp"
+ "strings"
+ "sync"
+ "time"
+)
+
+type Client struct {
+ HttpClient *http.Client
+ AppId string
+ Protocol constant.ProtocolEnum
+ Secret string
+ Host string
+ RedirectUri string
+ UserPoolId string
+ TokenEndPointAuthMethod constant.AuthMethodEnum
+
+ ClientToken *string
+ ClientUser *model.User
+
+ Log func(s string)
+}
+
+func NewClient(appId string, secret string, host ...string) *Client {
+ var clientHost string
+ if len(host) == 0 {
+ clientHost = constant.CoreAuthingDefaultUrl
+ } else {
+ clientHost = host[0]
+ }
+ c := &Client{
+ HttpClient: nil,
+ AppId: appId,
+ Protocol: "",
+ Secret: secret,
+ Host: clientHost,
+ RedirectUri: "",
+ Log: nil,
+ }
+ if c.HttpClient == nil {
+ c.HttpClient = &http.Client{}
+ }
+
+ //c.AuthingRequest = util.NewAuthingRequest(appId,secret,clientHost)
+ return c
+}
+
+// TODO
+func (c *Client) BuildAuthorizeUrlByOidc(params model.OidcParams) (string, error) {
+ if c.AppId == "" {
+ return constant.StringEmpty, errors.New("请在初始化 AuthenticationClient 时传入 appId")
+ }
+ if c.Protocol != constant.OIDC {
+ return constant.StringEmpty, errors.New("初始化 AuthenticationClient 传入的 protocol 应为 ProtocolEnum.OIDC")
+ }
+ if params.RedirectUri == "" {
+ return constant.StringEmpty, errors.New("redirectUri 不能为空")
+ }
+ var scope = ""
+ if strings.Contains(params.Scope, "offline_access") {
+ scope = "consent"
+ }
+ dataMap := map[string]string{
+ "client_id": util.GetValidValue(params.AppId, c.AppId),
+ "scope": util.GetValidValue(params.Scope, "openid profile email phone address"),
+ "state": util.GetValidValue(params.State, util.RandomString(12)),
+ "nonce": util.GetValidValue(params.Nonce, util.RandomString(12)),
+ "response_mode": util.GetValidValue(params.ResponseMode, constant.StringEmpty),
+ "response_type": util.GetValidValue(params.ResponseType, "code"),
+ "redirect_uri": util.GetValidValue(params.RedirectUri, c.RedirectUri),
+ "prompt": util.GetValidValue(scope),
+ }
+ return c.Host + "/oidc/auth?" + util.GetQueryString(dataMap), nil
+}
+
+// GetAccessTokenByCode
+// code 换取 accessToken
+func (c *Client) GetAccessTokenByCode(code string) (string, error) {
+ if c.AppId == "" {
+ return constant.StringEmpty, errors.New("请在初始化 AuthenticationClient 时传入 appId")
+ }
+ if c.Secret == "" && c.TokenEndPointAuthMethod != constant.None {
+ return constant.StringEmpty, errors.New("请在初始化 AuthenticationClient 时传入 Secret")
+ }
+ url := c.Host + "/oidc/token"
+
+ header := map[string]string{
+ "Content-Type": "application/x-www-form-urlencoded",
+ }
+
+ body := map[string]string{
+ "client_id": c.AppId,
+ "client_secret": c.Secret,
+ "grant_type": "authorization_code",
+ "code": code,
+ "redirect_uri": c.RedirectUri,
+ }
+
+ switch c.TokenEndPointAuthMethod {
+ case constant.ClientSecretPost:
+ body["client_id"] = c.AppId
+ body["client_secret"] = c.Secret
+ case constant.ClientSecretBasic:
+ base64String := "Basic " + base64.StdEncoding.EncodeToString([]byte(fmt.Sprintf("%s:%s", c.AppId, c.Secret)))
+ header["Authorization"] = base64String
+ default:
+ body["client_id"] = c.AppId
+ }
+ resp, err := c.SendHttpRequest(url, constant.HttpMethodPost, header, body)
+
+ //resp, err := c.AuthingRequest.SendRequest(url, constant.HttpMethodPost, header, body)
+ return string(resp), err
+}
+
+// GetUserInfoByAccessToken
+// accessToken 换取用户信息
+func (c *Client) GetUserInfoByAccessToken(accessToken string) (string, error) {
+ if accessToken == constant.StringEmpty {
+ return constant.StringEmpty, errors.New("accessToken 不能为空")
+ }
+ url := c.Host + "/oidc/me?access_token=" + accessToken
+ resp, err := c.SendHttpRequest(url, constant.HttpMethodGet, nil, nil)
+ return string(resp), err
+}
+
+// GetNewAccessTokenByRefreshToken
+// 使用 Refresh token 获取新的 Access token
+func (c *Client) GetNewAccessTokenByRefreshToken(refreshToken string) (string, error) {
+ if c.Protocol != constant.OIDC && c.Protocol != constant.OAUTH {
+ return constant.StringEmpty, errors.New("初始化 AuthenticationClient 时传入的 protocol 参数必须为 ProtocolEnum.OAUTH 或 ProtocolEnum.OIDC,请检查参数")
+ }
+ if c.Secret == "" && c.TokenEndPointAuthMethod != constant.None {
+ return constant.StringEmpty, errors.New("请在初始化 AuthenticationClient 时传入 Secret")
+ }
+
+ url := c.Host + fmt.Sprintf("/%s/token", c.Protocol)
+
+ header := map[string]string{
+ "Content-Type": "application/x-www-form-urlencoded",
+ }
+
+ body := map[string]string{
+ "client_id": c.AppId,
+ "client_secret": c.Secret,
+ "grant_type": "refresh_token",
+ "refresh_token": refreshToken,
+ }
+
+ switch c.TokenEndPointAuthMethod {
+ case constant.ClientSecretPost:
+ body["client_id"] = c.AppId
+ body["client_secret"] = c.Secret
+ case constant.ClientSecretBasic:
+ base64String := "Basic " + base64.StdEncoding.EncodeToString([]byte(fmt.Sprintf("%s:%s", c.AppId, c.Secret)))
+ header["Authorization"] = base64String
+ default:
+ body["client_id"] = c.AppId
+ }
+ resp, err := c.SendHttpRequest(url, constant.HttpMethodPost, header, body)
+ return string(resp), err
+}
+
+// IntrospectToken
+// 检查 Access token 或 Refresh token 的状态
+func (c *Client) IntrospectToken(token string) (string, error) {
+ url := c.Host + fmt.Sprintf("/%s/token/introspection", c.Protocol)
+
+ header := map[string]string{
+ "Content-Type": "application/x-www-form-urlencoded",
+ }
+
+ body := map[string]string{
+ "token": token,
+ }
+
+ switch c.TokenEndPointAuthMethod {
+ case constant.ClientSecretPost:
+ body["client_id"] = c.AppId
+ body["client_secret"] = c.Secret
+ case constant.ClientSecretBasic:
+ base64String := "Basic " + base64.StdEncoding.EncodeToString([]byte(fmt.Sprintf("%s:%s", c.AppId, c.Secret)))
+ header["Authorization"] = base64String
+ default:
+ body["client_id"] = c.AppId
+ }
+ resp, err := c.SendHttpRequest(url, constant.HttpMethodPost, header, body)
+ return string(resp), err
+}
+
+// ValidateToken
+// 效验Token合法性
+func (c *Client) ValidateToken(req model.ValidateTokenRequest) (string, error) {
+ if req.IdToken == constant.StringEmpty && req.AccessToken == constant.StringEmpty {
+ return constant.StringEmpty, errors.New("请传入 AccessToken 或 IdToken")
+ }
+ if req.IdToken != constant.StringEmpty && req.AccessToken != constant.StringEmpty {
+ return constant.StringEmpty, errors.New("AccessToken 和 IdToken 不能同时传入")
+ }
+
+ url := c.Host + "/api/v2/oidc/validate_token?"
+ if req.IdToken != constant.StringEmpty {
+ url += "id_token=" + req.IdToken
+ } else if req.AccessToken != constant.StringEmpty {
+ url += "access_token=" + req.AccessToken
+ }
+
+ resp, err := c.SendHttpRequest(url, constant.HttpMethodGet, nil, nil)
+ return string(resp), err
+}
+
+// RevokeToken
+// 撤回 Access token 或 Refresh token
+func (c *Client) RevokeToken(token string) (string, error) {
+ if c.Protocol != constant.OIDC && c.Protocol != constant.OAUTH {
+ return constant.StringEmpty, errors.New("初始化 AuthenticationClient 时传入的 protocol 参数必须为 ProtocolEnum.OAUTH 或 ProtocolEnum.OIDC,请检查参数")
+ }
+ if c.Secret == "" && c.TokenEndPointAuthMethod != constant.None {
+ return constant.StringEmpty, errors.New("请在初始化 AuthenticationClient 时传入 Secret")
+ }
+
+ url := c.Host + fmt.Sprintf("/%s/token/revocation", c.Protocol)
+
+ header := map[string]string{
+ "Content-Type": "application/x-www-form-urlencoded",
+ }
+
+ body := map[string]string{
+ "client_id": c.AppId,
+ "token": token,
+ }
+
+ switch c.TokenEndPointAuthMethod {
+ case constant.ClientSecretPost:
+ body["client_id"] = c.AppId
+ body["client_secret"] = c.Secret
+ case constant.ClientSecretBasic:
+ base64String := "Basic " + base64.StdEncoding.EncodeToString([]byte(fmt.Sprintf("%s:%s", c.AppId, c.Secret)))
+ header["Authorization"] = base64String
+ default:
+ body["client_id"] = c.AppId
+ }
+ resp, err := c.SendHttpRequest(url, constant.HttpMethodPost, header, body)
+ return string(resp), err
+}
+
+// GetAccessTokenByClientCredentials
+// Client Credentials 模式获取 Access Token
+func (c *Client) GetAccessTokenByClientCredentials(req model.GetAccessTokenByClientCredentialsRequest) (string, error) {
+ if req.Scope == constant.StringEmpty {
+ return constant.StringEmpty, errors.New("请传入 scope 参数,请看文档:https://docs.authing.cn/v2/guides/authorization/m2m-authz.html")
+ }
+ if req.ClientCredentialInput == nil {
+ return constant.StringEmpty, errors.New("请在调用本方法时传入 ClientCredentialInput 参数,请看文档:https://docs.authing.cn/v2/guides/authorization/m2m-authz.html")
+ }
+
+ url := c.Host + "/oidc/token"
+
+ header := map[string]string{
+ "Content-Type": "application/x-www-form-urlencoded",
+ }
+
+ body := map[string]string{
+ "client_id": req.ClientCredentialInput.AccessKey,
+ "client_secret": req.ClientCredentialInput.SecretKey,
+ "grant_type": "client_credentials",
+ "scope": req.Scope,
+ }
+
+ resp, err := c.SendHttpRequest(url, constant.HttpMethodPost, header, body)
+ return string(resp), err
+}
+
+// LoginByUserName
+// 使用用户名登录
+func (c *Client) LoginByUserName(request model.LoginByUsernameInput) (*model.User, error) {
+ request.Password = util.RsaEncrypt(request.Password)
+ reqParam := make(map[string]interface{})
+ reqParam["input"] = request
+ data, _ := json.Marshal(&reqParam)
+ variables := make(map[string]interface{})
+ json.Unmarshal(data, &variables)
+ b, err := c.SendHttpRequestManage(c.Host+constant.CoreAuthingGraphqlPath, constant.HttpMethodPost, constant.LoginByUsernameDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ return c.loginGetUserInfo(b, "loginByUsername")
+}
+
+// LoginByEmail
+// 使用邮箱登录
+func (c *Client) LoginByEmail(request model.LoginByEmailInput) (*model.User, error) {
+ request.Password = util.RsaEncrypt(request.Password)
+ reqParam := make(map[string]interface{})
+ reqParam["input"] = request
+ data, _ := json.Marshal(&reqParam)
+ variables := make(map[string]interface{})
+ json.Unmarshal(data, &variables)
+ b, err := c.SendHttpRequestManage(c.Host+constant.CoreAuthingGraphqlPath, constant.HttpMethodPost, constant.LoginByEmailDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ return c.loginGetUserInfo(b, "loginByEmail")
+}
+
+// LoginByPhonePassword
+// 使用手机号密码登录
+func (c *Client) LoginByPhonePassword(request model.LoginByPhonePasswordInput) (*model.User, error) {
+ request.Password = util.RsaEncrypt(request.Password)
+ reqParam := make(map[string]interface{})
+ reqParam["input"] = request
+ data, _ := json.Marshal(&reqParam)
+ variables := make(map[string]interface{})
+ json.Unmarshal(data, &variables)
+ b, err := c.SendHttpRequestManage(c.Host+constant.CoreAuthingGraphqlPath, constant.HttpMethodPost, constant.LoginByPhonePasswordDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ return c.loginGetUserInfo(b, "loginByPhonePassword")
+}
+
+//TODO
+func (c *Client) loginGetUserInfo(b []byte, userKey string) (*model.User, error) {
+ var result *simplejson.Json
+ result, err := simplejson.NewJson(b)
+ if _, r := result.CheckGet("errors"); r {
+ msg, err := result.Get("errors").GetIndex(0).Get("message").Get("message").String()
+ if err != nil {
+ return nil, err
+ }
+ return nil, errors.New(msg)
+ }
+ byteUser, err := result.Get("data").Get(userKey).MarshalJSON()
+ if err != nil {
+ return nil, err
+ }
+ resultUser := model.User{}
+ err = json.Unmarshal(byteUser, &resultUser)
+ if err != nil {
+ return nil, err
+ }
+ c.SetCurrentUser(&resultUser)
+ return &resultUser, nil
+}
+func (c *Client) SendHttpRequest(url string, method string, header map[string]string, body map[string]string) ([]byte, error) {
+ var form http.Request
+ form.ParseForm()
+ if body != nil && len(body) != 0 {
+ for key, value := range body {
+ form.Form.Add(key, value)
+ }
+ }
+ reqBody := strings.TrimSpace(form.Form.Encode())
+ req, err := http.NewRequest(method, url, strings.NewReader(reqBody))
+ if err != nil {
+ fmt.Println(err)
+ }
+ //req.Header.Add("Content-Type", "application/x-www-form-urlencoded")
+ //增加header选项
+ if header != nil && len(header) != 0 {
+ for key, value := range header {
+ req.Header.Add(key, value)
+ }
+ }
+ res, err := c.HttpClient.Do(req)
+ defer res.Body.Close()
+ respBody, err := ioutil.ReadAll(res.Body)
+ return respBody, nil
+}
+
+func (c *Client) SendHttpRequestManage(url string, method string, query string, variables map[string]interface{}) ([]byte, error) {
+ var req *http.Request
+ if method == constant.HttpMethodGet {
+ req, _ = http.NewRequest(http.MethodGet, url, nil)
+ if variables != nil && len(variables) > 0 {
+ q := req.URL.Query()
+ for key, value := range variables {
+ q.Add(key, fmt.Sprintf("%v", value))
+ }
+ req.URL.RawQuery = q.Encode()
+ }
+
+ } else {
+ in := struct {
+ Query string `json:"query"`
+ Variables map[string]interface{} `json:"variables,omitempty"`
+ }{
+ Query: query,
+ Variables: variables,
+ }
+ var buf bytes.Buffer
+ err := json.NewEncoder(&buf).Encode(in)
+ if err != nil {
+ return nil, err
+ }
+ req, err = http.NewRequest(method, url, &buf)
+ req.Header.Add("Content-Type", "application/json")
+ }
+
+ //增加header选项
+ if !strings.HasPrefix(query, "query accessToken") && c.ClientToken != nil {
+ token := c.ClientToken
+ req.Header.Add("Authorization", "Bearer "+*token)
+ }
+ req.Header.Add("x-authing-userpool-id", ""+c.UserPoolId)
+ req.Header.Add("x-authing-request-from", constant.SdkType)
+ req.Header.Add("x-authing-sdk-version", constant.SdkVersion)
+ req.Header.Add("x-authing-app-id", ""+c.AppId)
+
+ res, err := c.HttpClient.Do(req)
+ if err != nil {
+ return nil, err
+ }
+ defer res.Body.Close()
+ body, err := ioutil.ReadAll(res.Body)
+ return body, nil
+}
+
+//TODO
+func QueryAccessToken(client *Client) (*model.AccessTokenRes, error) {
+ type Data struct {
+ AccessToken model.AccessTokenRes `json:"accessToken"`
+ }
+ type Result struct {
+ Data Data `json:"data"`
+ }
+
+ variables := map[string]interface{}{
+ "userPoolId": client.UserPoolId,
+ "secret": client.Secret,
+ }
+
+ b, err := client.SendHttpRequestManage(client.Host+constant.CoreAuthingGraphqlPath, constant.HttpMethodPost, constant.AccessTokenDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ var r Result
+ if b != nil {
+ json.Unmarshal(b, &r)
+ }
+ return &r.Data.AccessToken, nil
+}
+
+// GetAccessToken
+// 获取访问Token
+func GetAccessToken(client *Client) (string, error) {
+ // 从缓存获取token
+ cacheToken, b := cacheutil.GetCache(constant.TokenCacheKeyPrefix + client.UserPoolId)
+ if b && cacheToken != nil {
+ return cacheToken.(string), nil
+ }
+ // 从服务获取token,加锁
+ var mutex sync.Mutex
+ mutex.Lock()
+ defer mutex.Unlock()
+ cacheToken, b = cacheutil.GetCache(constant.TokenCacheKeyPrefix + client.UserPoolId)
+ if b && cacheToken != nil {
+ return cacheToken.(string), nil
+ }
+ token, err := QueryAccessToken(client)
+ if err != nil {
+ return "", err
+ }
+ var expire = *(token.Exp) - time.Now().Unix() - 43200
+ cacheutil.SetCache(constant.TokenCacheKeyPrefix+client.UserPoolId, *token.AccessToken, time.Duration(expire*int64(time.Second)))
+ return *token.AccessToken, nil
+}
+
+func (c *Client) SendHttpRestRequest(url string, method string, token *string, variables map[string]interface{}) ([]byte, error) {
+ var req *http.Request
+ if method == constant.HttpMethodGet {
+ req, _ = http.NewRequest(http.MethodGet, url, nil)
+ if variables != nil && len(variables) > 0 {
+ q := req.URL.Query()
+ for key, value := range variables {
+ q.Add(key, fmt.Sprintf("%v", value))
+ }
+ req.URL.RawQuery = q.Encode()
+ }
+ } else {
+ var buf bytes.Buffer
+ var err error
+ if variables != nil {
+ err = json.NewEncoder(&buf).Encode(variables)
+ }
+ if err != nil {
+ return nil, err
+ }
+ req, err = http.NewRequest(method, url, &buf)
+ req.Header.Add("Content-Type", "application/json")
+ }
+
+ if token == nil {
+ selfToken, _ := GetAccessToken(c)
+ token = &selfToken
+ }
+ req.Header.Add("Authorization", "Bearer "+*token)
+ req.Header.Add("x-authing-userpool-id", ""+c.UserPoolId)
+ req.Header.Add("x-authing-request-from", constant.SdkType)
+ req.Header.Add("x-authing-sdk-version", constant.SdkVersion)
+ req.Header.Add("x-authing-app-id", ""+c.AppId)
+ res, err := c.HttpClient.Do(req)
+ if err != nil {
+ return nil, err
+ }
+ defer res.Body.Close()
+ body, err := ioutil.ReadAll(res.Body)
+ return body, nil
+}
+
+func (c *Client) SendHttpRestRequestNotToken(url string, method string, variables map[string]interface{}) ([]byte, error) {
+ var req *http.Request
+ if method == constant.HttpMethodGet {
+ req, _ = http.NewRequest(http.MethodGet, url, nil)
+ if variables != nil && len(variables) > 0 {
+ q := req.URL.Query()
+ for key, value := range variables {
+ q.Add(key, fmt.Sprintf("%v", value))
+ }
+ req.URL.RawQuery = q.Encode()
+ }
+ } else {
+ var buf bytes.Buffer
+ var err error
+ if variables != nil {
+ err = json.NewEncoder(&buf).Encode(variables)
+ }
+ if err != nil {
+ return nil, err
+ }
+ req, err = http.NewRequest(method, url, &buf)
+ req.Header.Add("Content-Type", "application/json")
+ }
+
+ req.Header.Add("x-authing-userpool-id", ""+c.UserPoolId)
+ req.Header.Add("x-authing-request-from", constant.SdkType)
+ req.Header.Add("x-authing-sdk-version", constant.SdkVersion)
+ req.Header.Add("x-authing-app-id", ""+c.AppId)
+ res, err := c.HttpClient.Do(req)
+ if err != nil {
+ return nil, err
+ }
+ defer res.Body.Close()
+ body, err := ioutil.ReadAll(res.Body)
+ return body, nil
+}
+
+// GetCurrentUser
+// 获取资源列表
+func (c *Client) GetCurrentUser(token *string) (*model.User, error) {
+
+ url := fmt.Sprintf("%s/api/v2/users/me", c.Host)
+ b, err := c.SendHttpRestRequest(url, http.MethodGet, token, nil)
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data model.User `json:"data"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return &resp.Data, nil
+}
+
+func (c *Client) getCurrentUser() (*model.User, error) {
+ k, e := cacheutil.GetCache(constant.UserCacheKeyPrefix + c.UserPoolId)
+ if !e {
+ return nil, errors.New("未登录")
+ }
+ return k.(*model.User), nil
+}
+
+// SetCurrentUser
+// 设置当前用户
+func (c *Client) SetCurrentUser(user *model.User) (*model.User, error) {
+ c.ClientUser = user
+ c.ClientToken = user.Token
+ //cacheutil.SetDefaultCache(constant.UserCacheKeyPrefix+c.userPoolId, user)
+ //c.SetToken(*user.Token)
+
+ return user, nil
+}
+
+// SetToken
+// 设置 Token
+func (c *Client) SetToken(token string) {
+ c.ClientToken = &token
+ //cacheutil.SetDefaultCache(constant.TokenCacheKeyPrefix+c.userPoolId, token)
+}
+
+// RegisterByEmail
+// 使用邮箱注册
+func (c *Client) RegisterByEmail(request *model.RegisterByEmailInput) (*model.User, error) {
+ request.Password = util.RsaEncrypt(request.Password)
+ data, _ := jsoniter.Marshal(request)
+ variables := make(map[string]interface{})
+ jsoniter.Unmarshal(data, &variables)
+
+ b, err := c.SendHttpRequestManage(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.RegisterByEmailDocument,
+ map[string]interface{}{"input": variables})
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ RegisterByEmail model.User `json:"registerByEmail"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ c.SetCurrentUser(&response.Data.RegisterByEmail)
+ return &response.Data.RegisterByEmail, nil
+}
+
+// RegisterByUsername
+// 使用用户名注册
+func (c *Client) RegisterByUsername(request *model.RegisterByUsernameInput) (*model.User, error) {
+ request.Password = util.RsaEncrypt(request.Password)
+ data, _ := jsoniter.Marshal(request)
+ variables := make(map[string]interface{})
+ jsoniter.Unmarshal(data, &variables)
+
+ b, err := c.SendHttpRequestManage(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.RegisterByUsernameDocument,
+ map[string]interface{}{"input": variables})
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ RegisterByUsername model.User `json:"registerByUsername"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ c.SetCurrentUser(&response.Data.RegisterByUsername)
+ return &response.Data.RegisterByUsername, nil
+}
+
+// RegisterByPhoneCode
+// 使用手机号及验证码注册
+func (c *Client) RegisterByPhoneCode(request *model.RegisterByPhoneCodeInput) (*model.User, error) {
+
+ data, _ := jsoniter.Marshal(request)
+ variables := make(map[string]interface{})
+ jsoniter.Unmarshal(data, &variables)
+
+ b, err := c.SendHttpRequestManage(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.RegisterByPhoneCodeDocument,
+ map[string]interface{}{"input": variables})
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ RegisterByPhoneCode model.User `json:"registerByPhoneCode"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ c.SetCurrentUser(&response.Data.RegisterByPhoneCode)
+ return &response.Data.RegisterByPhoneCode, nil
+}
+
+// CheckPasswordStrength
+// 检查密码强度
+func (c *Client) CheckPasswordStrength(password string) (*struct {
+ Valid bool `json:"valid"`
+ Message string `json:"message"`
+}, error) {
+
+ b, err := c.SendHttpRequestManage(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.CheckPasswordStrengthDocument,
+ map[string]interface{}{"password": password})
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ CheckPasswordStrength struct {
+ Valid bool `json:"valid"`
+ Message string `json:"message"`
+ } `json:"checkPasswordStrength"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+
+ return &response.Data.CheckPasswordStrength, nil
+}
+
+// SendSmsCode
+// 发送短信验证码
+func (c *Client) SendSmsCode(phone string) (*struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+}, error) {
+
+ url := fmt.Sprintf("%s/api/v2/sms/send", c.Host)
+ b, err := c.SendHttpRestRequestNotToken(url, http.MethodPost, map[string]interface{}{
+ "phone": phone,
+ })
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return resp, nil
+}
+
+// LoginByPhoneCode
+// 使用手机号验证码登录
+func (c *Client) LoginByPhoneCode(req *model.LoginByPhoneCodeInput) (*model.User, error) {
+ data, _ := jsoniter.Marshal(req)
+ vars := make(map[string]interface{})
+ jsoniter.Unmarshal(data, &vars)
+
+ b, err := c.SendHttpRequestManage(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.LoginByPhoneCodeDocument, map[string]interface{}{
+ "input": vars,
+ })
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ LoginByPhoneCode model.User `json:"loginByPhoneCode"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+
+ return &response.Data.LoginByPhoneCode, nil
+}
+
+// CheckLoginStatus
+// 检测 Token 登录状态
+func (c *Client) CheckLoginStatus(token string) (*model.CheckLoginStatusResponse, error) {
+
+ b, err := c.SendHttpRequestManage(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.CheckLoginStatusDocument,
+ map[string]interface{}{"token": token})
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ CheckLoginStatus model.CheckLoginStatusResponse `json:"checkLoginStatus"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.CheckLoginStatus, nil
+}
+
+// SendEmail
+// 发送邮件
+func (c *Client) SendEmail(email string, scene model.EnumEmailScene) (*model.CommonMessageAndCode, error) {
+
+ b, err := c.SendHttpRequestManage(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.SendMailDocument,
+ map[string]interface{}{"email": email, "scene": scene})
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ SendMail model.CommonMessageAndCode `json:"sendEmail"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.SendMail, nil
+}
+
+// ResetPasswordByPhoneCode
+// 通过短信验证码重置密码
+func (c *Client) ResetPasswordByPhoneCode(phone, code, newPassword string) (*model.CommonMessageAndCode, error) {
+
+ b, err := c.SendHttpRequestManage(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.ResetPasswordDocument,
+ map[string]interface{}{"phone": phone, "code": code, "newPassword": util.RsaEncrypt(newPassword)})
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ ResetPassword model.CommonMessageAndCode `json:"resetPassword"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.ResetPassword, nil
+}
+
+// ResetPasswordByEmailCode
+// 通过邮件验证码重置密码
+func (c *Client) ResetPasswordByEmailCode(email, code, newPassword string) (*model.CommonMessageAndCode, error) {
+
+ b, err := c.SendHttpRequestManage(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.ResetPasswordDocument,
+ map[string]interface{}{"email": email, "code": code, "newPassword": util.RsaEncrypt(newPassword)})
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ ResetPassword model.CommonMessageAndCode `json:"resetPassword"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.ResetPassword, nil
+}
+
+// UpdateProfile
+// 修改用户资料
+func (c *Client) UpdateProfile(req *model.UpdateUserInput) (*model.User, error) {
+ vars := make(map[string]interface{})
+ currentUser, e := c.getCurrentUser()
+ if e != nil {
+ return nil, e
+ }
+ vars["id"] = currentUser.Id
+ vars["input"] = req
+
+ b, err := c.SendHttpRequestManage(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.UpdateProfileDocument,
+ vars)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ UpdateUser model.User `json:"updateUser"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ c.SetCurrentUser(&response.Data.UpdateUser)
+ return &response.Data.UpdateUser, nil
+}
+
+// UpdatePassword
+// 更新用户密码
+func (c *Client) UpdatePassword(oldPassword *string, newPassword string) (*model.User, error) {
+
+ vars := make(map[string]interface{})
+ vars["newPassword"] = util.RsaEncrypt(newPassword)
+ if oldPassword != nil {
+ vars["oldPassword"] = util.RsaEncrypt(*oldPassword)
+ }
+
+ b, err := c.SendHttpRequestManage(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.UpdatePasswordDocument, vars)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ UpdatePassword model.User `json:"updatePassword"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ c.SetCurrentUser(&response.Data.UpdatePassword)
+ return &response.Data.UpdatePassword, nil
+}
+
+// UpdatePhone
+// 更新用户手机号
+func (c *Client) UpdatePhone(phone, code string, oldPhone, oldPhoneCode *string) (*model.User, error) {
+
+ b, err := c.SendHttpRequestManage(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.UpdatePhoneDocument,
+ map[string]interface{}{
+ "phone": phone,
+ "phoneCode": code,
+ "oldPhone": oldPhone,
+ "oldPhoneCode": oldPhoneCode,
+ })
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ UpdatePhone model.User `json:"updatePhone"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ c.SetCurrentUser(&response.Data.UpdatePhone)
+ return &response.Data.UpdatePhone, nil
+}
+
+// UpdateEmail
+// 更新用户邮箱
+func (c *Client) UpdateEmail(email, code string, oldEmail, oldEmailCode *string) (*model.User, error) {
+
+ b, err := c.SendHttpRequestManage(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.UpdateEmailDocument,
+ map[string]interface{}{
+ "email": email,
+ "emailCode": code,
+ "oldEmail": oldEmail,
+ "oldEmailCode": oldEmailCode,
+ })
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ UpdateEmail model.User `json:"updateEmail"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ c.SetCurrentUser(&response.Data.UpdateEmail)
+ return &response.Data.UpdateEmail, nil
+}
+
+// RefreshToken
+// 刷新当前用户的 token
+func (c *Client) RefreshToken(token *string) (*model.RefreshToken, error) {
+
+ b, err := c.SendHttpRequestCustomTokenManage(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, token, constant.RefreshUserTokenDocument,
+ nil)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ RefreshToken model.RefreshToken `json:"refreshToken"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ c.SetToken(*response.Data.RefreshToken.Token)
+ return &response.Data.RefreshToken, nil
+}
+
+func (c *Client) SendHttpRequestCustomTokenManage(url string, method string, token *string, query string, variables map[string]interface{}) ([]byte, error) {
+ var req *http.Request
+ if method == constant.HttpMethodGet {
+ req, _ = http.NewRequest(http.MethodGet, url, nil)
+ if variables != nil && len(variables) > 0 {
+ q := req.URL.Query()
+ for key, value := range variables {
+ q.Add(key, fmt.Sprintf("%v", value))
+ }
+ req.URL.RawQuery = q.Encode()
+ }
+
+ } else {
+ in := struct {
+ Query string `json:"query"`
+ Variables map[string]interface{} `json:"variables,omitempty"`
+ }{
+ Query: query,
+ Variables: variables,
+ }
+ var buf bytes.Buffer
+ err := json.NewEncoder(&buf).Encode(in)
+ if err != nil {
+ return nil, err
+ }
+ req, err = http.NewRequest(method, url, &buf)
+ req.Header.Add("Content-Type", "application/json")
+ }
+
+ //增加header选项
+ if token == nil {
+ useToken, _ := GetAccessToken(c)
+ req.Header.Add("Authorization", "Bearer "+useToken)
+ } else {
+ req.Header.Add("Authorization", "Bearer "+*token)
+
+ }
+ req.Header.Add("x-authing-userpool-id", ""+c.UserPoolId)
+ req.Header.Add("x-authing-request-from", constant.SdkType)
+ req.Header.Add("x-authing-sdk-version", constant.SdkVersion)
+ req.Header.Add("x-authing-app-id", ""+c.AppId)
+
+ res, err := c.HttpClient.Do(req)
+ if err != nil {
+ return nil, err
+ }
+ defer res.Body.Close()
+ body, err := ioutil.ReadAll(res.Body)
+ return body, nil
+}
+
+// LinkAccount
+// 关联账号
+func (c *Client) LinkAccount(primaryUserToken, secondaryUserToken string) (*model.CommonMessageAndCode, error) {
+
+ url := fmt.Sprintf("%s/api/v2/users/link", c.Host)
+ b, err := c.SendHttpRestRequest(url, http.MethodPost, nil, map[string]interface{}{
+ "primaryUserToken": primaryUserToken,
+ "secondaryUserToken": secondaryUserToken,
+ })
+ if err != nil {
+ return nil, err
+ }
+ resp := model.CommonMessageAndCode{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return &resp, nil
+}
+
+// UnLinkAccount
+// 主账号解绑社会化登录账号
+func (c *Client) UnLinkAccount(primaryUserToken string, provider constant.SocialProviderType) (*model.CommonMessageAndCode, error) {
+
+ url := fmt.Sprintf("%s/api/v2/users/unlink", c.Host)
+ b, err := c.SendHttpRestRequest(url, http.MethodPost, nil, map[string]interface{}{
+ "primaryUserToken": primaryUserToken,
+ "provider": provider,
+ })
+ if err != nil {
+ return nil, err
+ }
+ resp := model.CommonMessageAndCode{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return &resp, nil
+}
+
+// BindPhone
+// 绑定手机号
+func (c *Client) BindPhone(phone, phoneCode string) (*model.User, error) {
+
+ b, err := c.SendHttpRequestManage(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.BindPhoneDocument,
+ map[string]interface{}{"phone": phone, "phoneCode": phoneCode})
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ BindPhone model.User `json:"bindPhone"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ c.SetCurrentUser(&response.Data.BindPhone)
+ return &response.Data.BindPhone, nil
+}
+
+// UnBindPhone
+// 绑定手机号
+func (c *Client) UnBindPhone() (*model.User, error) {
+ b, err := c.SendHttpRequestManage(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.UnBindPhoneDocument,
+ nil)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ UnbindPhone model.User `json:"unbindPhone"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ c.SetCurrentUser(&response.Data.UnbindPhone)
+ return &response.Data.UnbindPhone, nil
+}
+
+// BindEmail
+// 绑定邮箱号
+func (c *Client) BindEmail(email, emailCode string) (*model.User, error) {
+
+ b, err := c.SendHttpRequestManage(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.BindEmailDocument,
+ map[string]interface{}{
+ "email": email,
+ "emailCode": emailCode,
+ })
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ BindEmail model.User `json:"bindEmail"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ c.SetCurrentUser(&response.Data.BindEmail)
+ return &response.Data.BindEmail, nil
+}
+
+// UnBindEmail
+// 解绑邮箱号
+func (c *Client) UnBindEmail() (*model.User, error) {
+ b, err := c.SendHttpRequestManage(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.UnBindEmailDocument,
+ nil)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ UnbindEmail model.User `json:"unbindEmail"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ c.SetCurrentUser(&response.Data.UnbindEmail)
+ return &response.Data.UnbindEmail, nil
+}
+
+// Logout
+// 退出登录
+func (c *Client) Logout() (*model.CommonMessageAndCode, error) {
+ cacheToken, _ := cacheutil.GetCache(constant.TokenCacheKeyPrefix + c.UserPoolId)
+ if cacheToken == nil {
+ return nil, errors.New("Please login first")
+ }
+ token := cacheToken.(string)
+
+ url := fmt.Sprintf("%s/api/v2/logout?app_id=%s", c.Host, c.AppId)
+ b, err := c.SendHttpRestRequest(url, http.MethodGet, &token, nil)
+ if err != nil {
+ return nil, err
+ }
+ resp := model.CommonMessageAndCode{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ c.ClearUser()
+ return &resp, nil
+}
+
+func (c *Client) LogoutByToken(token string) (*model.CommonMessageAndCode, error) {
+ url := fmt.Sprintf("%s/api/v2/logout?app_id=%s", c.Host, c.AppId)
+ variables := make(map[string]interface{})
+
+ variables["withCredentials"] = true
+ fmt.Println(url)
+ b, err := c.SendHttpRestRequest(url, http.MethodGet, &token, variables)
+ if err != nil {
+ return nil, err
+ }
+ resp := model.CommonMessageAndCode{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return &resp, nil
+}
+
+func (c *Client) ClearUser() {
+ c.ClientUser = nil
+ c.ClientToken = nil
+ //cacheutil.DeleteCache(constant.TokenCacheKeyPrefix + c.userPoolId)
+ //cacheutil.DeleteCache(constant.UserCacheKeyPrefix + c.userPoolId)
+}
+
+func (c *Client) getCacheUser() (*model.User, error) {
+ //cache, _ := cacheutil.GetCache(constant.UserCacheKeyPrefix + c.userPoolId)
+ //if cache == nil {
+ // return nil, errors.New("Please login first")
+ //}
+ //cacheUser := cache.(*model.User)
+ if c.ClientUser == nil {
+ return nil, errors.New("Please login first")
+ }
+ return c.ClientUser, nil
+}
+
+// ListUdv
+// 获取当前用户的自定义数据列表
+func (c *Client) ListUdv() (*[]model.UserDefinedData, error) {
+ cacheUser, e := c.getCacheUser()
+ if e != nil {
+ return nil, e
+ }
+ b, err := c.SendHttpRequestManage(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.UdvDocument, map[string]interface{}{
+ "targetType": model.EnumUDFTargetTypeUSER,
+ "targetId": cacheUser.Id,
+ })
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ Udv []model.UserDefinedData `json:"udv"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.Udv, nil
+}
+
+// SetUdv
+// 添加自定义数据
+func (c *Client) SetUdv(udvList []model.KeyValuePair) (*[]model.UserDefinedData, error) {
+ cacheUser, e := c.getCacheUser()
+ if e != nil {
+ return nil, e
+ }
+ variables := make(map[string]interface{})
+
+ variables["targetType"] = model.EnumUDFTargetTypeUSER
+ variables["targetId"] = cacheUser.Id
+ variables["udvList"] = udvList
+
+ b, err := c.SendHttpRequestManage(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.SetRoleUdfValueDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ SetUdvBatch []model.UserDefinedData `json:"setUdvBatch"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.SetUdvBatch, nil
+}
+
+// RemoveUdv
+// 删除自定义数据
+func (c *Client) RemoveUdv(key string) (*[]model.UserDefinedData, error) {
+ cacheUser, e := c.getCacheUser()
+ if e != nil {
+ return nil, e
+ }
+ variables := make(map[string]interface{})
+ variables["targetType"] = constant.USER
+ variables["targetId"] = cacheUser.Id
+ variables["key"] = key
+
+ b, err := c.SendHttpRequestManage(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.RemoveUdfValueDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ RemoveUdv []model.UserDefinedData `json:"removeUdv"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.RemoveUdv, nil
+}
+
+// ListOrg
+// 获取用户所在组织机构
+func (c *Client) ListOrg() (*struct {
+ Code int64 `json:"code"`
+ Message string `json:"message"`
+ Data []model.UserOrgs `json:"data"`
+}, error) {
+
+ if c.ClientToken == nil {
+ return nil, errors.New("Please login first")
+ }
+ token := c.ClientToken
+
+ url := fmt.Sprintf("%s/api/v2/users/me/orgs", c.Host)
+ b, err := c.SendHttpRestRequest(url, http.MethodGet, token, nil)
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Code int64 `json:"code"`
+ Message string `json:"message"`
+ Data []model.UserOrgs `json:"data"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return resp, nil
+}
+
+// LoginByLdap
+// 使用 LDAP 用户名登录
+func (c *Client) LoginByLdap(username, password string) (*struct {
+ Code int64 `json:"code"`
+ Message string `json:"message"`
+ Data model.User `json:"data"`
+}, error) {
+
+ url := fmt.Sprintf("%s/api/v2/ldap/verify-user", c.Host)
+ b, err := c.SendHttpRestRequestNotToken(url, http.MethodPost, map[string]interface{}{
+ "username": username,
+ "password": password,
+ })
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Code int64 `json:"code"`
+ Message string `json:"message"`
+ Data model.User `json:"data"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return resp, nil
+}
+
+// LoginByAd
+// 使用 AD 用户名登录
+func (c *Client) LoginByAd(username, password string) (*struct {
+ Code int64 `json:"code"`
+ Message string `json:"message"`
+ Data model.User `json:"data"`
+}, error) {
+
+ com, _ := regexp.Compile("(?:http.*://)?(?P[^:/ ]+).?(?P[0-9]*).*")
+ domain := com.FindString(c.Host)
+
+ lis := strings.Split(domain, ".")
+ var wsHost string
+ if len(lis) > 2 {
+ wsHost = strings.Join(lis[1:], ".")
+ } else {
+ wsHost = domain
+ }
+ url := fmt.Sprintf("https://ws.%s/api/v2/ad/verify-user", wsHost)
+ b, err := c.SendHttpRestRequestNotToken(url, http.MethodPost, map[string]interface{}{
+ "username": username,
+ "password": password,
+ })
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Code int64 `json:"code"`
+ Message string `json:"message"`
+ Data model.User `json:"data"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return resp, nil
+}
+
+// GetSecurityLevel
+// 用户安全等级
+func (c *Client) GetSecurityLevel() (*struct {
+ Code int64 `json:"code"`
+ Message string `json:"message"`
+ Data model.GetSecurityLevelResponse `json:"data"`
+}, error) {
+ //cacheToken, _ := cacheutil.GetCache(constant.TokenCacheKeyPrefix + c.userPoolId)
+ //if cacheToken == nil {
+ // return nil, errors.New("Please login first")
+ //}
+ //token := cacheToken.(string)
+ if c.ClientToken == nil {
+ return nil, errors.New("Please login first")
+ }
+ token := c.ClientToken
+ url := fmt.Sprintf("%s/api/v2/users/me/security-level", c.Host)
+ b, err := c.SendHttpRestRequest(url, http.MethodGet, token, nil)
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Code int64 `json:"code"`
+ Message string `json:"message"`
+ Data model.GetSecurityLevelResponse `json:"data"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return resp, nil
+}
+
+// ListAuthorizedResources
+// 获取用户被授权的所有资源
+func (c *Client) ListAuthorizedResources(namespace string, resourceType model.EnumResourceType) (*model.AuthorizedResources, error) {
+ cacheUser, e := c.getCacheUser()
+ if e != nil {
+ return nil, e
+ }
+ b, err := c.SendHttpRequestManage(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.ListUserAuthorizedResourcesDocument,
+ map[string]interface{}{
+ "id": cacheUser.Id,
+ "namespace": namespace,
+ "resourceType": resourceType,
+ })
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ User struct {
+ AuthorizedResources model.AuthorizedResources `json:"authorizedResources"`
+ } `json:"user"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.User.AuthorizedResources, nil
+}
+
+func (c *Client) BuildAuthorizeUrlByOauth(scope, redirectUri, state, responseType string) (string, error) {
+
+ if c.AppId == "" {
+ return constant.StringEmpty, errors.New("请在初始化 AuthenticationClient 时传入 appId")
+ }
+ if c.Protocol != constant.OAUTH {
+ return constant.StringEmpty, errors.New("初始化 AuthenticationClient 传入的 protocol 应为 ProtocolEnum.OAUTH")
+ }
+ if redirectUri == "" {
+ return constant.StringEmpty, errors.New("redirectUri 不能为空")
+ }
+
+ if strings.Contains(scope, "offline_access") {
+ scope = "consent"
+ }
+ dataMap := map[string]string{
+ "client_id": util.GetValidValue(c.AppId),
+ "scope": util.GetValidValue(scope, "openid profile email phone address"),
+ "state": util.GetValidValue(state, util.RandomString(12)),
+ "response_type": util.GetValidValue(responseType),
+ "redirect_uri": util.GetValidValue(redirectUri),
+ }
+ return c.Host + "/oauth/auth?" + util.GetQueryString(dataMap), nil
+}
+
+func (c *Client) BuildAuthorizeUrlBySaml() string {
+ return fmt.Sprintf("%s/api/v2/saml-idp/%s", c.Host, c.AppId)
+}
+
+func (c *Client) BuildAuthorizeUrlByCas(service *string) string {
+ if service != nil {
+ return fmt.Sprintf("%s/cas-idp/%s?service=%s", c.Host, c.AppId, *service)
+ } else {
+ return fmt.Sprintf("%s/cas-idp/%s?service", c.Host, c.AppId)
+ }
+}
+
+// ValidateTicketV1
+// 检验 CAS 1.0 Ticket 合法性
+func (c *Client) ValidateTicketV1(ticket, service string) (*struct {
+ Valid bool `json:"code"`
+ Message string `json:"message"`
+ Username string `json:"username"`
+}, error) {
+
+ url := fmt.Sprintf("%s/cas-idp/%s/validate?service=%s&ticket=%s", c.Host, c.AppId, service, ticket)
+ b, err := c.SendHttpRestRequestNotToken(url, http.MethodGet, nil)
+ if err != nil {
+ return nil, err
+ }
+ sps := strings.Split(string(b), "\n")
+ var username, message string
+
+ valid := (sps[0] == "yes")
+ username = sps[1]
+ if !valid {
+ message = "ticket is not valid"
+ }
+ resp := &struct {
+ Valid bool `json:"code"`
+ Message string `json:"message"`
+ Username string `json:"username"`
+ }{
+ Valid: valid,
+ Username: username,
+ Message: message,
+ }
+
+ return resp, nil
+}
+
+//BuildLogoutUrl
+//拼接登出 URL
+func (c *Client) BuildLogoutUrl(expert, redirectUri, idToken *string) string {
+ var url string
+ if c.Protocol == constant.OIDC {
+ if expert == nil {
+ if redirectUri != nil {
+ url = fmt.Sprintf("%s/login/profile/logout?redirect_uri=%s", c.Host, *redirectUri)
+ } else {
+ url = fmt.Sprintf("%s/login/profile/logout", c.Host)
+ }
+
+ } else {
+ if redirectUri != nil {
+ url = fmt.Sprintf("%s/oidc/session/end?id_token_hint=%s&post_logout_redirect_uri=%s", c.Host, *idToken, *redirectUri)
+ } else {
+ url = fmt.Sprintf("%s/oidc/session/end", c.Host)
+ }
+
+ }
+ }
+ if c.Protocol == constant.CAS {
+ if redirectUri != nil {
+ url = fmt.Sprintf("%s/cas-idp/logout?url=%s", c.Host, *redirectUri)
+ } else {
+ url = fmt.Sprintf("%s/cas-idp/logout", c.Host)
+ }
+ }
+ return url
+}
+
+// ListRole
+// 获取用户拥有的角色列表
+func (c *Client) ListRole(namespace string) (*struct {
+ TotalCount int `json:"totalCount"`
+ List []model.RoleModel `json:"list"`
+}, error) {
+ cacheUser, e := c.getCacheUser()
+ if e != nil {
+ return nil, e
+ }
+ b, err := c.SendHttpRequestManage(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.GetUserRolesDocument,
+ map[string]interface{}{
+ "id": cacheUser.Id,
+ "namespace": namespace,
+ })
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ User model.GetUserRolesResponse `json:"user"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.User.Roles, nil
+}
+
+// HasRole
+// 判断当前用户是否有某个角色
+func (c *Client) HasRole(code, namespace string) (*bool, error) {
+ r, e := c.ListRole(namespace)
+ if e != nil {
+ return nil, e
+ }
+ hasRole := true
+ notHas := false
+ if r.TotalCount == 0 {
+ return ¬Has, nil
+ }
+ for _, d := range r.List {
+ if d.Code == code {
+ return &hasRole, nil
+ }
+ }
+ return ¬Has, nil
+}
+
+// ListApplications
+// 获取当前用户能够访问的应用
+func (c *Client) ListApplications(page, limit int) (*struct {
+ Code int64 `json:"code"`
+ Message string `json:"message"`
+ Data struct {
+ TotalCount int64 `json:"totalCount"`
+ List []model.Application `json:"list"`
+ } `json:"data"`
+}, error) {
+ if c.ClientToken == nil {
+ return nil, errors.New("Please login first")
+ }
+ token := c.ClientToken
+ url := fmt.Sprintf("%s/api/v2/users/me/applications/allowed?page=%v&limit=%v", c.Host, page, limit)
+ b, err := c.SendHttpRestRequest(url, http.MethodGet, token, nil)
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Code int64 `json:"code"`
+ Message string `json:"message"`
+ Data struct {
+ TotalCount int64 `json:"totalCount"`
+ List []model.Application `json:"list"`
+ } `json:"data"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return resp, nil
+}
+
+// GenerateCodeChallenge
+// 生成一个 PKCE 校验码,长度必须大于等于 43。
+func (c *Client) GenerateCodeChallenge(size int) (string, error) {
+ if size < 43 {
+ return constant.StringEmpty, errors.New("code_challenge must be a string length grater than 43")
+ }
+ return util.RandomString(size), nil
+
+}
+
+// GetCodeChallengeDigest
+// 生成一个 PKCE 校验码摘要值
+func (c *Client) GetCodeChallengeDigest(codeChallenge string, method constant.GenerateCodeChallengeMethod) (string, error) {
+ if len(codeChallenge) < 43 {
+ return constant.StringEmpty, errors.New("code_challenge must be a string length grater than 43")
+ }
+ if method == constant.PLAIN {
+ return codeChallenge, nil
+ } else {
+ hasher := sha256.New()
+ hasher.Write([]byte(codeChallenge))
+ base64Str := base64.URLEncoding.EncodeToString(hasher.Sum(nil))
+ return strings.Replace(base64Str, "=", "", -1), nil
+ }
+
+}
+
+// LoginBySubAccount
+// 登录子账号
+func (c *Client) LoginBySubAccount(req *model.LoginBySubAccountRequest) (*model.User, error) {
+ req.Password = util.RsaEncrypt(req.Password)
+ data, _ := jsoniter.Marshal(req)
+ vars := make(map[string]interface{})
+ jsoniter.Unmarshal(data, &vars)
+ b, err := c.SendHttpRequestManage(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.LoginBySubAccountDocument, vars)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ LoginBySubAccount model.User `json:"loginBySubAccount"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ c.SetCurrentUser(&response.Data.LoginBySubAccount)
+ return &response.Data.LoginBySubAccount, nil
+}
+
+// ResetPasswordByFirstLoginToken
+// 通过首次登录的 Token 重置密码
+func (c *Client) ResetPasswordByFirstLoginToken(token, password string) (*model.CommonMessageAndCode, error) {
+ password = util.RsaEncrypt(password)
+
+ b, err := c.SendHttpRequestManage(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.ResetPasswordByTokenDocument,
+ map[string]interface{}{
+ "token": token,
+ "password": password,
+ })
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ ResetPasswordByFirstLoginToken model.CommonMessageAndCode `json:"resetPasswordByFirstLoginToken"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+
+ return &response.Data.ResetPasswordByFirstLoginToken, nil
+}
+
+// ResetPasswordByForceResetToken
+// 通过密码强制更新临时 Token 修改密码
+func (c *Client) ResetPasswordByForceResetToken(token, password, newPassword string) (*model.CommonMessageAndCode, error) {
+ password = util.RsaEncrypt(password)
+ newPassword = util.RsaEncrypt(newPassword)
+ b, err := c.SendHttpRequestManage(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.ResetPasswordByForceResetTokenDocument,
+ map[string]interface{}{
+ "token": token,
+ "oldPassword": password,
+ "newPassword": newPassword,
+ })
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ ResetPasswordByForceResetToken model.CommonMessageAndCode `json:"resetPasswordByForceResetToken"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+
+ return &response.Data.ResetPasswordByForceResetToken, nil
+}
+
+// ListDepartments
+// 获取用户所有部门
+func (c *Client) ListDepartments() (*model.PaginatedDepartments, error) {
+ cacheUser, e := c.getCacheUser()
+ if e != nil {
+ return nil, e
+ }
+ b, err := c.SendHttpRequestManage(c.Host+constant.CoreAuthingGraphqlPath, constant.HttpMethodPost, constant.GetUserDepartmentsDocument,
+ map[string]interface{}{"id": cacheUser.Id})
+ if err != nil {
+ return nil, err
+ }
+
+ var response = &struct {
+ Data model.UserDepartmentsData `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return response.Data.User.Departments, nil
+
+}
+
+// IsUserExists
+// 判断用户是否存在
+func (c *Client) IsUserExists(req *model.IsUserExistsRequest) (*bool, error) {
+
+ data, _ := jsoniter.Marshal(req)
+ vars := make(map[string]interface{})
+ jsoniter.Unmarshal(data, &vars)
+
+ b, err := c.SendHttpRequestManage(c.Host+constant.CoreAuthingGraphqlPath, constant.HttpMethodPost, constant.IsUserExistsDocument,
+ vars)
+ if err != nil {
+ return nil, err
+ }
+
+ var response = &struct {
+ Data struct {
+ IsUserExists *bool `json:"isUserExists"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return response.Data.IsUserExists, nil
+
+}
+
+// ValidateTicketV2
+// 通过远端服务验证票据合法性
+func (c *Client) ValidateTicketV2(ticket, service string, format constant.TicketFormat) (*struct {
+ Code int64 `json:"code"`
+ Message string `json:"message"`
+ Data interface{} `json:"data"`
+}, error) {
+
+ url := fmt.Sprintf("%s/cas-idp/%s/serviceValidate", c.Host, c.AppId)
+ b, err := c.SendHttpRestRequestNotToken(url, http.MethodGet, map[string]interface{}{
+ "service": service,
+ "ticket": ticket,
+ "format": format,
+ })
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Code int64 `json:"code"`
+ Message string `json:"message"`
+ Data interface{} `json:"data"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return resp, nil
+}
+
+// TrackSession
+// sso 检测登录态
+func (c *Client) TrackSession(code string, country, lang, state *string) (*struct {
+ Code int64 `json:"code"`
+ Message string `json:"message"`
+ Data interface{} `json:"data"`
+}, error) {
+
+ url := fmt.Sprintf("%s/connection/social/wechat:mobile/%s/callback?code=%s", c.Host, c.AppId, code)
+ if country != nil {
+ url = url + "&country=" + *country
+ }
+ if lang != nil {
+ url = url + "&lang=" + *lang
+ }
+ if state != nil {
+ url = url + "&state=" + *state
+ }
+ b, err := c.SendHttpRestRequestNotToken(url, http.MethodGet, nil)
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Code int64 `json:"code"`
+ Message string `json:"message"`
+ Data interface{} `json:"data"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return resp, nil
+}
diff --git a/lib/authentication/authentication_client_test.go b/lib/authentication/authentication_client_test.go
new file mode 100644
index 0000000..e6a1518
--- /dev/null
+++ b/lib/authentication/authentication_client_test.go
@@ -0,0 +1,734 @@
+package authentication
+
+import (
+ "fmt"
+ "github.com/Authing/authing-go-sdk/lib/constant"
+ "github.com/Authing/authing-go-sdk/lib/model"
+ jsoniter "github.com/json-iterator/go"
+ "log"
+ "testing"
+)
+
+const (
+ //UserPool=""
+ //Secret=""
+ //AppId =""
+ AppId = ""
+ Secret = ""
+ UserPool = ""
+)
+
+func TestClient_LogoutByToken(t *testing.T) {
+ authenticationClient := NewClient(AppId, Secret, "https://hfggf.authing.cn")
+ authenticationClient.UserPoolId = UserPool
+ model := model.LoginByEmailInput{}
+ model.Email = "zy@pm.com"
+ model.Password = "zy"
+ user, err := authenticationClient.LoginByEmail(model)
+ if err != nil {
+ fmt.Println(err)
+ }
+ fmt.Println(*user.Token)
+ res, err := authenticationClient.LogoutByToken(*(user.Token))
+ fmt.Println(res, err)
+ fmt.Println(authenticationClient.CheckLoginStatus(*(user.Token)))
+}
+
+func TestClient_BuildAuthorizeUrlByOidc(t *testing.T) {
+ authenticationClient := NewClient(AppId, Secret)
+ authenticationClient.Protocol = constant.OIDC
+ authenticationClient.TokenEndPointAuthMethod = constant.None
+ req := model.OidcParams{
+ AppId: AppId,
+ RedirectUri: "https://mvnrepository.com/",
+ Nonce: "test",
+ }
+ resp, err := authenticationClient.BuildAuthorizeUrlByOidc(req)
+ if err != nil {
+ fmt.Println(err)
+ } else {
+ fmt.Println(resp)
+ }
+}
+
+func TestClient_GetAccessTokenByCode(t *testing.T) {
+ authenticationClient := NewClient("60a6f980dd9a9a7642da768a", "5cd4ea7b3603b792aea9a00da9e18f44")
+ authenticationClient.Host = "https://32l5hb-demo.authing.cn"
+ authenticationClient.RedirectUri = "https://mvnrepository.com/"
+ authenticationClient.Protocol = constant.OIDC
+ authenticationClient.TokenEndPointAuthMethod = constant.None
+ resp, err := authenticationClient.GetAccessTokenByCode("vj-MWd4eRZdmakwobde53RaFZpBON3-khElsrlEZRGm")
+ if err != nil {
+ fmt.Println(err)
+ } else {
+ fmt.Println(resp)
+ }
+ // {"access_token":"eyJhbGciOiJSUzI1NiIsInR5cCI6IkpXVCIsImtpZCI6IjQ0bnJHU05YQ3NDLTByd1J5Q0hENjBzdmc0elpLNF9iV2VnQjluOFRhQzQifQ.eyJqdGkiOiJ3NjJmNkVieHYxd19wbEV3YWMwWlIiLCJzdWIiOiI2MGUyNmI2ZjdiMGRkN2MwYWY4M2VjZDkiLCJpYXQiOjE2MjU0OTI3NjUsImV4cCI6MTYyNjcwMjM2NSwic2NvcGUiOiJvcGVuaWQgcGhvbmUgYWRkcmVzcyBwcm9maWxlIGVtYWlsIiwiaXNzIjoiaHR0cHM6Ly8zMmw1aGItZGVtby5hdXRoaW5nLmNuL29pZGMiLCJhdWQiOiI2MGE2Zjk4MGRkOWE5YTc2NDJkYTc2OGEifQ.KOMWqEtbyH3qdBv_bHX3Dof2t_3XBQ7QDg4-x7fIr9W2YtCnwNnqVehOVYjWpcF-pkVyzBlpmKIc6_X9F8GA-oYbdUKJzhxfoAATj1JnRCRs6Wsxpo3U41up1pgXs5B7JS7gVbiw_IucMg4vLYw_QJ_aPgBTkjCkBZVsPf3NRYCd2cVwiZwvoa8GT6jGP9PJ908rJSSSdsqt6JNzydVbJ9a7p4mBhV3WxUAckXePjIE0QDNDe_GxFwFDktkTbLBIJZBL4bSg3pHGQKHiF9wabfjBRfWV8ChRe8i95n7pq-Gw9fw2fKNv7ieC5bK52D1j6R9L5h7wRvTstgiR7p8krQ","expires_in":1209600,"id_token":"eyJhbGciOiJSUzI1NiIsInR5cCI6IkpXVCIsImtpZCI6IjQ0bnJHU05YQ3NDLTByd1J5Q0hENjBzdmc0elpLNF9iV2VnQjluOFRhQzQifQ.eyJzdWIiOiI2MGUyNmI2ZjdiMGRkN2MwYWY4M2VjZDkiLCJwaG9uZV9udW1iZXIiOm51bGwsInBob25lX251bWJlcl92ZXJpZmllZCI6ZmFsc2UsImFkZHJlc3MiOnsiY291bnRyeSI6bnVsbCwicG9zdGFsX2NvZGUiOm51bGwsInJlZ2lvbiI6bnVsbCwiZm9ybWF0dGVkIjpudWxsfSwiYmlydGhkYXRlIjpudWxsLCJmYW1pbHlfbmFtZSI6bnVsbCwiZ2VuZGVyIjoiVSIsImdpdmVuX25hbWUiOm51bGwsImxvY2FsZSI6bnVsbCwibWlkZGxlX25hbWUiOm51bGwsIm5hbWUiOm51bGwsIm5pY2tuYW1lIjpudWxsLCJwaWN0dXJlIjoiaHR0cHM6Ly9maWxlcy5hdXRoaW5nLmNvL2F1dGhpbmctY29uc29sZS9kZWZhdWx0LXVzZXItYXZhdGFyLnBuZyIsInByZWZlcnJlZF91c2VybmFtZSI6bnVsbCwicHJvZmlsZSI6bnVsbCwidXBkYXRlZF9hdCI6IjIwMjEtMDctMDVUMTM6NDU6MjMuMTc0WiIsIndlYnNpdGUiOm51bGwsInpvbmVpbmZvIjpudWxsLCJlbWFpbCI6Imx1b2ppZWxpbkBhdXRoaW5nLmNuIiwiZW1haWxfdmVyaWZpZWQiOmZhbHNlLCJub25jZSI6InRlc3QiLCJhdF9oYXNoIjoiaFVfa2o5NzdjSGUxXzdhdWI3OWY3dyIsImF1ZCI6IjYwYTZmOTgwZGQ5YTlhNzY0MmRhNzY4YSIsImV4cCI6MTYyNjcwMjM2NSwiaWF0IjoxNjI1NDkyNzY1LCJpc3MiOiJodHRwczovLzMybDVoYi1kZW1vLmF1dGhpbmcuY24vb2lkYyJ9.XtLA_hQZqqwUW2GyVwEVhO2BMqNCFMWCkxQGd1FP37tclxnHKsa26wz8oBKNPXsGwEUBIlcyzi9SCTOibl_UlG4hNrHASNkk_2zQcsjO8fidHfXjEyw2UjhDfxsyh1B6xcJIiM8AJIQi5BHJ1FcFzCLxRK81v_kPqQMMHagYXEQhaFNf-otxrBrf9Yc66wuMLKlgKUgAZLyhTqJFpXPIayzss00vIOvbQNTc5XY27M_uUP2-TInIG8dxY-rcxe06PqTWVvLkDx1CMsEC7Ume1wf6lKqGU4kGnSLlXBxrl1-MRd-Q01gosvBvP2r2Tuxb30ZD0-yG4QY9yD9ytTYSPA","scope":"openid phone address profile email","token_type":"Bearer"}
+}
+
+func TestClient_GetUserInfoByAccessToken(t *testing.T) {
+ authenticationClient := NewClient("60a6f980dd9a9a7642da768a", "5cd4ea7b3603b792aea9a00da9e18f44")
+ authenticationClient.Host = "https://32l5hb-demo.authing.cn"
+ authenticationClient.RedirectUri = "https://mvnrepository.com/"
+ authenticationClient.Protocol = constant.OIDC
+ authenticationClient.TokenEndPointAuthMethod = constant.None
+ resp, err := authenticationClient.GetUserInfoByAccessToken("eyJhbGciOiJSUzI1NiIsInR5cCI6IkpXVCIsImtpZCI6IjQ0bnJHU05YQ3NDLTByd1J5Q0hENjBzdmc0elpLNF9iV2VnQjluOFRhQzQifQ.eyJqdGkiOiJ3NjJmNkVieHYxd19wbEV3YWMwWlIiLCJzdWIiOiI2MGUyNmI2ZjdiMGRkN2MwYWY4M2VjZDkiLCJpYXQiOjE2MjU0OTI3NjUsImV4cCI6MTYyNjcwMjM2NSwic2NvcGUiOiJvcGVuaWQgcGhvbmUgYWRkcmVzcyBwcm9maWxlIGVtYWlsIiwiaXNzIjoiaHR0cHM6Ly8zMmw1aGItZGVtby5hdXRoaW5nLmNuL29pZGMiLCJhdWQiOiI2MGE2Zjk4MGRkOWE5YTc2NDJkYTc2OGEifQ.KOMWqEtbyH3qdBv_bHX3Dof2t_3XBQ7QDg4-x7fIr9W2YtCnwNnqVehOVYjWpcF-pkVyzBlpmKIc6_X9F8GA-oYbdUKJzhxfoAATj1JnRCRs6Wsxpo3U41up1pgXs5B7JS7gVbiw_IucMg4vLYw_QJ_aPgBTkjCkBZVsPf3NRYCd2cVwiZwvoa8GT6jGP9PJ908rJSSSdsqt6JNzydVbJ9a7p4mBhV3WxUAckXePjIE0QDNDe_GxFwFDktkTbLBIJZBL4bSg3pHGQKHiF9wabfjBRfWV8ChRe8i95n7pq-Gw9fw2fKNv7ieC5bK52D1j6R9L5h7wRvTstgiR7p8krQ")
+ if err != nil {
+ fmt.Println(err)
+ } else {
+ fmt.Println(resp)
+ }
+}
+
+func TestClient_IntrospectToken(t *testing.T) {
+ authenticationClient := NewClient("60a6f980dd9a9a7642da768a", "5cd4ea7b3603b792aea9a00da9e18f44")
+ authenticationClient.Host = "https://32l5hb-demo.authing.cn"
+ authenticationClient.RedirectUri = "https://mvnrepository.com/"
+ authenticationClient.Protocol = constant.OIDC
+ authenticationClient.TokenEndPointAuthMethod = constant.None
+
+ resp, err := authenticationClient.IntrospectToken("eyJhbGciOiJSUzI1NiIsInR5cCI6IkpXVCIsImtpZCI6IjQ0bnJHU05YQ3NDLTByd1J5Q0hENjBzdmc0elpLNF9iV2VnQjluOFRhQzQifQ.eyJqdGkiOiJ3NjJmNkVieHYxd19wbEV3YWMwWlIiLCJzdWIiOiI2MGUyNmI2ZjdiMGRkN2MwYWY4M2VjZDkiLCJpYXQiOjE2MjU0OTI3NjUsImV4cCI6MTYyNjcwMjM2NSwic2NvcGUiOiJvcGVuaWQgcGhvbmUgYWRkcmVzcyBwcm9maWxlIGVtYWlsIiwiaXNzIjoiaHR0cHM6Ly8zMmw1aGItZGVtby5hdXRoaW5nLmNuL29pZGMiLCJhdWQiOiI2MGE2Zjk4MGRkOWE5YTc2NDJkYTc2OGEifQ.KOMWqEtbyH3qdBv_bHX3Dof2t_3XBQ7QDg4-x7fIr9W2YtCnwNnqVehOVYjWpcF-pkVyzBlpmKIc6_X9F8GA-oYbdUKJzhxfoAATj1JnRCRs6Wsxpo3U41up1pgXs5B7JS7gVbiw_IucMg4vLYw_QJ_aPgBTkjCkBZVsPf3NRYCd2cVwiZwvoa8GT6jGP9PJ908rJSSSdsqt6JNzydVbJ9a7p4mBhV3WxUAckXePjIE0QDNDe_GxFwFDktkTbLBIJZBL4bSg3pHGQKHiF9wabfjBRfWV8ChRe8i95n7pq-Gw9fw2fKNv7ieC5bK52D1j6R9L5h7wRvTstgiR7p8krQ")
+ if err != nil {
+ fmt.Println(err)
+ } else {
+ fmt.Println(resp)
+ }
+}
+
+func TestClient_ValidateToken(t *testing.T) {
+ authenticationClient := NewClient("60a6f980dd9a9a7642da768a", "5cd4ea7b3603b792aea9a00da9e18f44")
+ authenticationClient.Host = "https://32l5hb-demo.authing.cn"
+ authenticationClient.RedirectUri = "https://mvnrepository.com/"
+ authenticationClient.Protocol = constant.OIDC
+ authenticationClient.TokenEndPointAuthMethod = constant.None
+ req := model.ValidateTokenRequest{
+ AccessToken: "eyJhbGciOiJSUzI1NiIsInR5cCI6IkpXVCIsImtpZCI6IjQ0bnJHU05YQ3NDLTByd1J5Q0hENjBzdmc0elpLNF9iV2VnQjluOFRhQzQifQ.eyJqdGkiOiJ3NjJmNkVieHYxd19wbEV3YWMwWlIiLCJzdWIiOiI2MGUyNmI2ZjdiMGRkN2MwYWY4M2VjZDkiLCJpYXQiOjE2MjU0OTI3NjUsImV4cCI6MTYyNjcwMjM2NSwic2NvcGUiOiJvcGVuaWQgcGhvbmUgYWRkcmVzcyBwcm9maWxlIGVtYWlsIiwiaXNzIjoiaHR0cHM6Ly8zMmw1aGItZGVtby5hdXRoaW5nLmNuL29pZGMiLCJhdWQiOiI2MGE2Zjk4MGRkOWE5YTc2NDJkYTc2OGEifQ.KOMWqEtbyH3qdBv_bHX3Dof2t_3XBQ7QDg4-x7fIr9W2YtCnwNnqVehOVYjWpcF-pkVyzBlpmKIc6_X9F8GA-oYbdUKJzhxfoAATj1JnRCRs6Wsxpo3U41up1pgXs5B7JS7gVbiw_IucMg4vLYw_QJ_aPgBTkjCkBZVsPf3NRYCd2cVwiZwvoa8GT6jGP9PJ908rJSSSdsqt6JNzydVbJ9a7p4mBhV3WxUAckXePjIE0QDNDe_GxFwFDktkTbLBIJZBL4bSg3pHGQKHiF9wabfjBRfWV8ChRe8i95n7pq-Gw9fw2fKNv7ieC5bK52D1j6R9L5h7wRvTstgiR7p8krQ",
+ IdToken: "",
+ }
+ resp, err := authenticationClient.ValidateToken(req)
+ if err != nil {
+ fmt.Println(err)
+ } else {
+ fmt.Println(resp)
+ }
+}
+
+func TestClient_GetAccessTokenByClientCredentials(t *testing.T) {
+ authenticationClient := NewClient("60a6f980dd9a9a7642da768a", "5cd4ea7b3603b792aea9a00da9e18f44")
+ authenticationClient.Host = "https://32l5hb-demo.authing.cn"
+ authenticationClient.RedirectUri = "https://mvnrepository.com/"
+ authenticationClient.Protocol = constant.OIDC
+ authenticationClient.TokenEndPointAuthMethod = constant.None
+ input := model.ClientCredentialInput{
+ AccessKey: "",
+ SecretKey: "",
+ }
+ req := model.GetAccessTokenByClientCredentialsRequest{
+ Scope: "openid",
+ ClientCredentialInput: &input,
+ }
+ resp, err := authenticationClient.GetAccessTokenByClientCredentials(req)
+ if err != nil {
+ fmt.Println(err)
+ } else {
+ fmt.Println(resp)
+ }
+}
+
+func TestClient_RevokeToken(t *testing.T) {
+ authenticationClient := NewClient("60a6f980dd9a9a7642da768a", "5cd4ea7b3603b792aea9a00da9e18f44")
+ authenticationClient.Host = "https://32l5hb-demo.authing.cn"
+ authenticationClient.RedirectUri = "https://mvnrepository.com/"
+ authenticationClient.Protocol = constant.OIDC
+ authenticationClient.TokenEndPointAuthMethod = constant.None
+ resp, err := authenticationClient.RevokeToken("eyJhbGciOiJSUzI1NiIsInR5cCI6IkpXVCIsImtpZCI6IjQ0bnJHU05YQ3NDLTByd1J5Q0hENjBzdmc0elpLNF9iV2VnQjluOFRhQzQifQ.eyJqdGkiOiJ3NjJmNkVieHYxd19wbEV3YWMwWlIiLCJzdWIiOiI2MGUyNmI2ZjdiMGRkN2MwYWY4M2VjZDkiLCJpYXQiOjE2MjU0OTI3NjUsImV4cCI6MTYyNjcwMjM2NSwic2NvcGUiOiJvcGVuaWQgcGhvbmUgYWRkcmVzcyBwcm9maWxlIGVtYWlsIiwiaXNzIjoiaHR0cHM6Ly8zMmw1aGItZGVtby5hdXRoaW5nLmNuL29pZGMiLCJhdWQiOiI2MGE2Zjk4MGRkOWE5YTc2NDJkYTc2OGEifQ.KOMWqEtbyH3qdBv_bHX3Dof2t_3XBQ7QDg4-x7fIr9W2YtCnwNnqVehOVYjWpcF-pkVyzBlpmKIc6_X9F8GA-oYbdUKJzhxfoAATj1JnRCRs6Wsxpo3U41up1pgXs5B7JS7gVbiw_IucMg4vLYw_QJ_aPgBTkjCkBZVsPf3NRYCd2cVwiZwvoa8GT6jGP9PJ908rJSSSdsqt6JNzydVbJ9a7p4mBhV3WxUAckXePjIE0QDNDe_GxFwFDktkTbLBIJZBL4bSg3pHGQKHiF9wabfjBRfWV8ChRe8i95n7pq-Gw9fw2fKNv7ieC5bK52D1j6R9L5h7wRvTstgiR7p8krQ")
+ if err != nil {
+ fmt.Println(err)
+ } else {
+ fmt.Println(resp)
+ }
+}
+
+func TestClient_LoginByUserName(t *testing.T) {
+ authenticationClient := NewClient("60a6f980dd9a9a7642da768a", "5cd4ea7b3603b792aea9a00da9e18f44")
+ authenticationClient.UserPoolId = "60e043f8cd91b87d712b6365"
+ authenticationClient.Secret = "158c7679333bc196b524d78d745813e5"
+ req := model.LoginByUsernameInput{
+ Username: "luojielin",
+ Password: "12341",
+ CaptchaCode: nil,
+ AutoRegister: nil,
+ ClientIp: nil,
+ Params: nil,
+ Context: nil,
+ }
+ resp, err := authenticationClient.LoginByUserName(req)
+ log.Println(resp, err)
+}
+
+func TestClient_LoginByEmail(t *testing.T) {
+ authenticationClient := NewClient("60a6f980dd9a9a7642da768a", "5cd4ea7b3603b792aea9a00da9e18f44")
+ authenticationClient.UserPoolId = "60e043f8cd91b87d712b6365"
+ authenticationClient.Secret = "158c7679333bc196b524d78d745813e5"
+ req := model.LoginByEmailInput{
+ Email: "luojielin@authing.cn",
+ Password: "1234",
+ CaptchaCode: nil,
+ AutoRegister: nil,
+ ClientIp: nil,
+ Params: nil,
+ Context: nil,
+ }
+ resp, err := authenticationClient.LoginByEmail(req)
+ log.Println(resp, err)
+}
+
+func TestClient_LoginByPhonePassword(b *testing.T) {
+ authenticationClient := NewClient("60a6f980dd9a9a7642da768a", "5cd4ea7b3603b792aea9a00da9e18f44")
+ authenticationClient.UserPoolId = "60e043f8cd91b87d712b6365"
+ authenticationClient.Secret = "158c7679333bc196b524d78d745813e5"
+ req := model.LoginByPhonePasswordInput{
+ Phone: "18310641137",
+ Password: "1234",
+ CaptchaCode: nil,
+ AutoRegister: nil,
+ ClientIp: nil,
+ Params: nil,
+ Context: nil,
+ }
+ resp, err := authenticationClient.LoginByPhonePassword(req)
+ log.Println(resp, err)
+}
+
+/*func TestClient_LoginByPhoneCode(b *testing.T) {
+ authenticationClient := NewClient("60a6f980dd9a9a7642da768a","5cd4ea7b3603b792aea9a00da9e18f44")
+ authenticationClient.UserPoolId = "60e043f8cd91b87d712b6365"
+ authenticationClient.Secret = "158c7679333bc196b524d78d745813e5"
+ req := model.LoginByPhoneCodeInput{
+ Phone: "18310641137",
+ Code: "7458",
+ AutoRegister: nil,
+ ClientIp: nil,
+ Params: nil,
+ Context: nil,
+ }
+ resp,err := authenticationClient.LoginByPhoneCode(req)
+ log.Println(resp,err)
+}
+
+func TestClient_SendSmsCode(t *testing.T) {
+ authenticationClient := NewClient("60a6f980dd9a9a7642da768a","5cd4ea7b3603b792aea9a00da9e18f44")
+ authenticationClient.UserPoolId = "60e043f8cd91b87d712b6365"
+ authenticationClient.Secret = "158c7679333bc196b524d78d745813e5"
+ resp,err := authenticationClient.SendSmsCode("15566416161")
+ log.Println(resp,err)
+}*/
+
+func TestClient_GetCurrentUser(t *testing.T) {
+ authenticationClient := NewClient(AppId, Secret)
+ authenticationClient.UserPoolId = UserPool
+
+ req := &model.LoginByPhoneCodeInput{
+ Code: "3289",
+ Phone: "189xxxx1835",
+ }
+ authenticationClient.LoginByPhoneCode(req)
+ resp, err := authenticationClient.GetCurrentUser(nil)
+ log.Println(resp, err)
+}
+
+func TestClient_RegisterByEmail(t *testing.T) {
+ authenticationClient := NewClient(AppId, Secret)
+ authenticationClient.UserPoolId = UserPool
+ data, e := jsoniter.Marshal([]model.KeyValuePair{{Key: "custom", Value: "qq"}})
+ log.Println(data, e)
+ p := string(data)
+ userName := "username"
+ req := &model.RegisterByEmailInput{
+ Email: "5304950622@qq.com",
+ Password: "123456",
+ Profile: &model.RegisterProfile{
+ Username: &userName,
+ },
+ Params: &p,
+ }
+ resp, err := authenticationClient.RegisterByEmail(req)
+ log.Println(resp, err)
+}
+
+func TestClient_RegisterByUsername(t *testing.T) {
+ authenticationClient := NewClient(AppId, Secret)
+ authenticationClient.UserPoolId = UserPool
+ data, e := jsoniter.Marshal([]model.KeyValuePair{{Key: "custom", Value: "qq"}})
+ log.Println(data, e)
+ p := string(data)
+ req := &model.RegisterByUsernameInput{
+ Username: "gosdk",
+ Password: "123456",
+ Params: &p,
+ }
+ resp, err := authenticationClient.RegisterByUsername(req)
+ log.Println(resp, err)
+}
+
+func TestClient_RegisterByPhoneCode(t *testing.T) {
+ authenticationClient := NewClient(AppId, Secret)
+ authenticationClient.UserPoolId = UserPool
+ data, e := jsoniter.Marshal([]model.KeyValuePair{{Key: "custom", Value: "qq"}})
+ log.Println(data, e)
+ p := string(data)
+ company := "company"
+ nickName := "nickName"
+ req := &model.RegisterByPhoneCodeInput{
+ Phone: "15865561492",
+ Code: "123456",
+ Profile: &model.RegisterProfile{
+ Nickname: &nickName,
+ Company: &company,
+ },
+ Params: &p,
+ }
+ resp, err := authenticationClient.RegisterByPhoneCode(req)
+ log.Println(resp, err)
+}
+
+func TestClient_CheckPasswordStrength(t *testing.T) {
+ authenticationClient := NewClient(AppId, Secret)
+ authenticationClient.UserPoolId = UserPool
+ data, e := jsoniter.Marshal([]model.KeyValuePair{{Key: "custom", Value: "qq"}})
+ log.Println(data, e)
+
+ resp, err := authenticationClient.CheckPasswordStrength("12345678")
+ log.Println(resp, err)
+}
+
+func TestClient_SendSmsCode(t *testing.T) {
+ authenticationClient := NewClient(AppId, Secret)
+ authenticationClient.UserPoolId = UserPool
+
+ resp, err := authenticationClient.SendSmsCode("18515006338")
+ log.Println(resp, err)
+}
+
+func TestClient_LoginByPhoneCode(t *testing.T) {
+ authenticationClient := NewClient(AppId, Secret)
+ authenticationClient.UserPoolId = UserPool
+ req := &model.LoginByPhoneCodeInput{
+ Code: "3289",
+ Phone: "18910471835",
+ }
+ resp, err := authenticationClient.LoginByPhoneCode(req)
+ log.Println(resp, err)
+}
+
+func TestClient_CheckLoginStatus(t *testing.T) {
+ authenticationClient := NewClient(AppId, Secret)
+ authenticationClient.UserPoolId = UserPool
+ reginter := &model.RegisterByUsernameInput{
+ Username: "testGoSDK",
+ Password: "123456789",
+ }
+ ru, re := authenticationClient.RegisterByUsername(reginter)
+ log.Println(ru, re)
+ req := &model.LoginByUsernameInput{
+ Username: "testGoSDK",
+ Password: "123456789",
+ }
+ u, e := authenticationClient.LoginByUserName(*req)
+ log.Println(u, e)
+ resp, err := authenticationClient.CheckLoginStatus(*u.Token)
+ log.Println(resp, err)
+}
+
+func TestClient_SendEmail(t *testing.T) {
+ authenticationClient := NewClient(AppId, Secret)
+ authenticationClient.UserPoolId = UserPool
+
+ resp, err := authenticationClient.SendEmail(" mail@qq.com", model.EnumEmailSceneChangeEmail)
+ log.Println(resp, err)
+}
+
+func TestClient_UpdateProfile(t *testing.T) {
+ authenticationClient := NewClient("6139c4d24e78a4d706b7545b", Secret)
+ authenticationClient.UserPoolId = UserPool
+
+ req := &model.LoginByUsernameInput{
+ Username: "updateProfile",
+ Password: "123456",
+ }
+ resp, err := authenticationClient.LoginByUserName(*req)
+ log.Println(resp)
+ username := "goSdkTestUpdateProfile"
+ updateReq := &model.UpdateUserInput{
+ Username: &username,
+ }
+ resp1, err := authenticationClient.UpdateProfile(updateReq)
+ log.Println(resp1, err)
+}
+
+func TestClient_UpdatePassword(t *testing.T) {
+ authenticationClient := NewClient(AppId, Secret)
+ authenticationClient.UserPoolId = UserPool
+ req := &model.LoginByUsernameInput{
+ Username: "goSdkTestUpdateProfile",
+ Password: "654321",
+ }
+ authenticationClient.LoginByUserName(*req)
+ resp, err := authenticationClient.UpdatePassword(nil, "654321")
+
+ log.Println(resp, err)
+ loginResp, loginErr := authenticationClient.LoginByUserName(model.LoginByUsernameInput{
+ Username: "goSdkTestUpdateProfile",
+ Password: "654321",
+ })
+ log.Println(loginResp, loginErr)
+}
+
+func TestClient_UpdatePhone(t *testing.T) {
+ authenticationClient := NewClient(AppId, Secret)
+ authenticationClient.UserPoolId = UserPool
+ req := &model.LoginByUsernameInput{
+ Username: "goSdkTestUpdateProfile",
+ Password: "654321",
+ }
+ //authenticationClient.SendSmsCode("18515006338")
+ authenticationClient.LoginByUserName(*req)
+ resp, err := authenticationClient.UpdatePhone("18515006338", "7757", nil, nil)
+
+ log.Println(resp, err)
+
+}
+
+func TestClient_UpdateEmail(t *testing.T) {
+ authenticationClient := NewClient(AppId, Secret)
+ authenticationClient.UserPoolId = UserPool
+ req := &model.LoginByUsernameInput{
+ Username: "goSdkTestUpdateProfile",
+ Password: "654321",
+ }
+ //authenticationClient.SendSmsCode("18515006338")
+ authenticationClient.LoginByUserName(*req)
+ resp, err := authenticationClient.UpdateEmail("530495062@qq.com", "7757", nil, nil)
+
+ log.Println(resp, err)
+
+}
+
+func TestClient_RefreshToken(t *testing.T) {
+ authenticationClient := NewClient(AppId, Secret)
+ authenticationClient.UserPoolId = UserPool
+ req := &model.LoginByUsernameInput{
+ Username: "goSdkTestUpdateProfile",
+ Password: "654321",
+ }
+ //authenticationClient.SendSmsCode("18515006338")
+ user, _ := authenticationClient.LoginByUserName(*req)
+ oldToken := user.Token
+ log.Println(oldToken)
+ resp, err := authenticationClient.RefreshToken(user.Token)
+ log.Println(resp.Token)
+
+ log.Println(resp, err)
+
+}
+
+func TestClient_LinkAccount(t *testing.T) {
+ authenticationClient := NewClient(AppId, Secret)
+ authenticationClient.UserPoolId = UserPool
+ req := &model.LoginByUsernameInput{
+ Username: "goSdkTestUpdateProfile",
+ Password: "654321",
+ }
+
+ user, _ := authenticationClient.LoginByUserName(*req)
+
+ resp, err := authenticationClient.LinkAccount(*user.Token, "qqwe")
+
+ log.Println(resp, err)
+
+}
+
+func TestClient_UnLinkAccount(t *testing.T) {
+ authenticationClient := NewClient(AppId, Secret)
+ authenticationClient.UserPoolId = UserPool
+ req := &model.LoginByUsernameInput{
+ Username: "goSdkTestUpdateProfile",
+ Password: "654321",
+ }
+
+ user, _ := authenticationClient.LoginByUserName(*req)
+
+ resp, err := authenticationClient.UnLinkAccount(*user.Token, constant.WECHATPC)
+
+ log.Println(resp, err)
+
+}
+
+func TestClient_BindPhone(t *testing.T) {
+ authenticationClient := NewClient(AppId, Secret)
+ authenticationClient.UserPoolId = UserPool
+ req := &model.LoginByUsernameInput{
+ Username: "18515006338",
+ Password: "123456",
+ }
+ authenticationClient.LoginByUserName(*req)
+ resp, err := authenticationClient.BindPhone("18515006338", "1453")
+ log.Println(resp, err)
+
+}
+func TestClient_SendSmsCode2(t *testing.T) {
+ authenticationClient := NewClient(AppId, Secret)
+ authenticationClient.UserPoolId = UserPool
+ d, e := authenticationClient.SendSmsCode("18515006338")
+ log.Println(d, e)
+}
+
+func TestClient_UnBindPhone(t *testing.T) {
+ authenticationClient := NewClient(AppId, Secret)
+ authenticationClient.UserPoolId = UserPool
+ req := &model.LoginByUsernameInput{
+ Username: "18515006338",
+ Password: "123456",
+ }
+ authenticationClient.LoginByUserName(*req)
+ resp, err := authenticationClient.UnBindPhone()
+ log.Println(resp, err)
+
+}
+
+func TestClient_BindEmail(t *testing.T) {
+ authenticationClient := NewClient(AppId, Secret)
+ authenticationClient.UserPoolId = UserPool
+ req := &model.LoginByUsernameInput{
+ Username: "18515006338",
+ Password: "123456",
+ }
+ authenticationClient.LoginByUserName(*req)
+ resp, err := authenticationClient.BindEmail("email", "code")
+ log.Println(resp, err)
+
+}
+
+func TestClient_UnBindEmail(t *testing.T) {
+ authenticationClient := NewClient(AppId, Secret)
+ authenticationClient.UserPoolId = UserPool
+ req := &model.LoginByUsernameInput{
+ Username: "18515006338",
+ Password: "123456",
+ }
+ authenticationClient.LoginByUserName(*req)
+ resp, err := authenticationClient.UnBindEmail()
+ log.Println(resp, err)
+
+}
+
+func TestClient_Logout(t *testing.T) {
+ authenticationClient := NewClient(AppId, Secret)
+ authenticationClient.UserPoolId = UserPool
+ req := &model.LoginByUsernameInput{
+ Username: "18515006338",
+ Password: "123456",
+ }
+ authenticationClient.LoginByUserName(*req)
+ resp, err := authenticationClient.Logout()
+ log.Println(resp, err)
+
+}
+
+func TestClient_ListUdv(t *testing.T) {
+ authenticationClient := NewClient(AppId, Secret)
+ authenticationClient.UserPoolId = UserPool
+ req := &model.LoginByUsernameInput{
+ Username: "18515006338",
+ Password: "123456",
+ }
+ authenticationClient.LoginByUserName(*req)
+ resp, err := authenticationClient.ListUdv()
+ log.Println(resp, err)
+
+}
+
+func TestClient_SetUdv(t *testing.T) {
+ authenticationClient := NewClient(AppId, Secret)
+ authenticationClient.UserPoolId = UserPool
+ req := &model.LoginByUsernameInput{
+ Username: "18515006338",
+ Password: "123456",
+ }
+ authenticationClient.LoginByUserName(*req)
+ resp, err := authenticationClient.SetUdv([]model.KeyValuePair{
+ {Key: "age", Value: "18"},
+ })
+ log.Println(resp, err)
+
+}
+
+func TestClient_RemoveUdv(t *testing.T) {
+ authenticationClient := NewClient(AppId, Secret)
+ authenticationClient.UserPoolId = UserPool
+ req := &model.LoginByUsernameInput{
+ Username: "18515006338",
+ Password: "123456",
+ }
+ authenticationClient.LoginByUserName(*req)
+ resp, err := authenticationClient.RemoveUdv("school")
+ log.Println(resp, err)
+
+}
+
+func TestClient_ListOrg(t *testing.T) {
+ authenticationClient := NewClient(AppId, Secret)
+ authenticationClient.UserPoolId = UserPool
+ req := &model.LoginByUsernameInput{
+ Username: "18515006338",
+ Password: "123456",
+ }
+ authenticationClient.LoginByUserName(*req)
+ resp, err := authenticationClient.ListOrg()
+ log.Println(resp, err)
+
+}
+
+func TestClient_LoginByLdap(t *testing.T) {
+ authenticationClient := NewClient(AppId, Secret)
+ authenticationClient.UserPoolId = UserPool
+ resp, err := authenticationClient.LoginByLdap("18515006338", "123456")
+ log.Println(resp, err)
+}
+
+func TestClient_LoginByAd(t *testing.T) {
+ authenticationClient := NewClient(AppId, Secret)
+ authenticationClient.UserPoolId = UserPool
+ resp, err := authenticationClient.LoginByAd("18515006338", "123456")
+ log.Println(resp, err)
+}
+
+func TestClient_GetSecurityLevel(t *testing.T) {
+ authenticationClient := NewClient(AppId, Secret)
+ authenticationClient.UserPoolId = UserPool
+ req := &model.LoginByUsernameInput{
+ Username: "18515006338",
+ Password: "123456",
+ }
+ authenticationClient.LoginByUserName(*req)
+ resp, err := authenticationClient.GetSecurityLevel()
+ log.Println(resp, err)
+}
+
+func TestClient_ListAuthorizedResources(t *testing.T) {
+ authenticationClient := NewClient(AppId, Secret)
+ authenticationClient.UserPoolId = UserPool
+ req := &model.LoginByUsernameInput{
+ Username: "18515006338",
+ Password: "123456",
+ }
+ authenticationClient.LoginByUserName(*req)
+ resp, err := authenticationClient.ListAuthorizedResources("default", model.EnumResourceTypeDATA)
+ log.Println(resp, err)
+}
+
+func TestClient_BuildAuthorizeUrlByOauth(t *testing.T) {
+ authenticationClient := NewClient(AppId, Secret)
+ authenticationClient.UserPoolId = UserPool
+ authenticationClient.Protocol = constant.OAUTH
+ resp, ee := authenticationClient.BuildAuthorizeUrlByOauth("email", "qq", "ww", "cc")
+ log.Println(resp, ee)
+}
+
+func TestClient_ValidateTicketV1(t *testing.T) {
+ authenticationClient := NewClient(AppId, Secret)
+ authenticationClient.UserPoolId = UserPool
+ authenticationClient.Protocol = constant.OAUTH
+ resp, ee := authenticationClient.ValidateTicketV1("email", "qq")
+ log.Println(resp, ee)
+}
+
+func TestClient_ListRole(t *testing.T) {
+ authenticationClient := NewClient(AppId, Secret)
+ authenticationClient.UserPoolId = UserPool
+ req := &model.LoginByUsernameInput{
+ Username: "18515006338",
+ Password: "123456",
+ }
+ authenticationClient.LoginByUserName(*req)
+ resp, err := authenticationClient.ListRole("default")
+ log.Println(resp, err)
+}
+func TestClient_HasRole(t *testing.T) {
+ authenticationClient := NewClient(AppId, Secret)
+ authenticationClient.UserPoolId = UserPool
+ req := &model.LoginByUsernameInput{
+ Username: "18515006338",
+ Password: "123456",
+ }
+ authenticationClient.LoginByUserName(*req)
+ resp, err := authenticationClient.HasRole("NewCode", "default")
+ log.Println(resp, err)
+}
+func TestClient_ListApplications(t *testing.T) {
+ authenticationClient := NewClient(AppId, Secret)
+ authenticationClient.UserPoolId = UserPool
+ req := &model.LoginByUsernameInput{
+ Username: "18515006338",
+ Password: "123456",
+ }
+ authenticationClient.LoginByUserName(*req)
+ resp, err := authenticationClient.ListApplications(1, 10)
+ log.Println(resp, err)
+}
+
+func TestClient_GetCodeChallengeDigest(t *testing.T) {
+ authenticationClient := NewClient(AppId, Secret)
+ authenticationClient.UserPoolId = UserPool
+
+ resp, err := authenticationClient.GetCodeChallengeDigest("wpaiscposrovkquicztfmftripjocybgmphyqtucmoz", constant.S256)
+
+ log.Println(resp, err)
+}
+
+func TestClient_LoginBySubAccount(t *testing.T) {
+ authenticationClient := NewClient(AppId, Secret)
+ authenticationClient.UserPoolId = UserPool
+ req := &model.LoginBySubAccountRequest{
+ Account: "123456789",
+ Password: "8558781",
+ }
+ resp, err := authenticationClient.LoginBySubAccount(req)
+
+ log.Println(resp, err)
+}
+
+func TestClient_ListDepartments(t *testing.T) {
+ authenticationClient := NewClient(AppId, Secret)
+ authenticationClient.UserPoolId = UserPool
+ req := &model.LoginByUsernameInput{
+ Username: "18515006338",
+ Password: "123456",
+ }
+ authenticationClient.LoginByUserName(*req)
+ resp, err := authenticationClient.ListDepartments()
+ log.Println(resp, err)
+}
+
+func TestClient_IsUserExists(t *testing.T) {
+ authenticationClient := NewClient(AppId, Secret)
+ authenticationClient.UserPoolId = UserPool
+ req := &model.LoginByUsernameInput{
+ Username: "18515006338",
+ Password: "123456",
+ }
+ userName := "18515006338"
+ authenticationClient.LoginByUserName(*req)
+ resp, err := authenticationClient.IsUserExists(&model.IsUserExistsRequest{
+ Username: &userName,
+ })
+ log.Println(resp, err)
+}
+
+func TestClient_ValidateTicketV2(t *testing.T) {
+ authenticationClient := NewClient(AppId, Secret)
+ authenticationClient.UserPoolId = UserPool
+
+ resp, err := authenticationClient.ValidateTicketV2("ss", "ss", constant.XML)
+ log.Println(resp, err)
+}
diff --git a/lib/authentication/mfa_client.go b/lib/authentication/mfa_client.go
new file mode 100644
index 0000000..a0e8079
--- /dev/null
+++ b/lib/authentication/mfa_client.go
@@ -0,0 +1,343 @@
+package authentication
+
+import (
+ "errors"
+ "fmt"
+ "github.com/Authing/authing-go-sdk/lib/constant"
+ "github.com/Authing/authing-go-sdk/lib/model"
+ jsoniter "github.com/json-iterator/go"
+ "net/http"
+)
+
+// GetMfaAuthenticators
+// 获取 MFA 认证器
+func (c *Client) GetMfaAuthenticators(req *model.MfaInput) (*struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data []model.GetMfaAuthenticatorsResponse `json:"data"`
+}, error) {
+
+ vars := make(map[string]interface{})
+ if req.MfaType == nil {
+ vars["type"] = "totp"
+ } else {
+ vars["type"] = req.MfaType
+ }
+ if req.MfaSource == nil {
+ vars["source"] = constant.Self
+ } else {
+ vars["source"] = req.MfaSource
+ }
+ url := fmt.Sprintf("%s/api/v2/mfa/authenticator", c.Host)
+ b, err := c.SendHttpRestRequest(url, http.MethodGet, req.MfaToken, vars)
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data []model.GetMfaAuthenticatorsResponse `json:"data"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return resp, nil
+}
+
+// AssociateMfaAuthenticator
+// 请求 MFA 二维码和密钥信息
+func (c *Client) AssociateMfaAuthenticator(req *model.MfaInput) (*struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data model.AssociateMfaAuthenticatorResponse `json:"data"`
+}, error) {
+
+ vars := make(map[string]interface{})
+ if req.MfaType == nil {
+ vars["authenticatorType"] = "totp"
+ } else {
+ vars["authenticatorType"] = req.MfaType
+ }
+ if req.MfaSource == nil {
+ vars["source"] = constant.Self
+ } else {
+ vars["source"] = req.MfaSource
+ }
+ url := fmt.Sprintf("%s/api/v2/mfa/totp/associate", c.Host)
+ b, err := c.SendHttpRestRequest(url, http.MethodPost, req.MfaToken, vars)
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data model.AssociateMfaAuthenticatorResponse `json:"data"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return resp, nil
+}
+
+// DeleteMfaAuthenticator
+// 解绑 MFA
+func (c *Client) DeleteMfaAuthenticator() (*model.CommonMessageAndCode, error) {
+
+ url := fmt.Sprintf("%s/api/v2/mfa/totp/associate", c.Host)
+ b, err := c.SendHttpRestRequest(url, http.MethodDelete, nil, nil)
+ if err != nil {
+ return nil, err
+ }
+ var resp model.CommonMessageAndCode
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return &resp, nil
+}
+
+// ConfirmAssociateMfaAuthenticator
+// 确认绑定 MFA
+func (c *Client) ConfirmAssociateMfaAuthenticator(req *model.ConfirmAssociateMfaAuthenticatorRequest) (*struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data interface{} `json:"data"`
+}, error) {
+
+ vars := make(map[string]interface{})
+ if req.AuthenticatorType == nil {
+ vars["authenticatorType"] = "totp"
+ } else {
+ vars["authenticatorType"] = req.AuthenticatorType
+ }
+ if req.MfaSource == nil {
+ vars["source"] = constant.Self
+ } else {
+ vars["source"] = req.MfaSource
+ }
+ vars["totp"] = req.Totp
+ url := fmt.Sprintf("%s/api/v2/mfa/totp/associate/confirm", c.Host)
+ b, err := c.SendHttpRestRequest(url, http.MethodPost, req.MfaToken, vars)
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data interface{} `json:"data"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return resp, nil
+}
+
+// VerifyTotpMfa
+// 检验二次验证 MFA 口令
+func (c *Client) VerifyTotpMfa(totp, token string) (*struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data interface{} `json:"data"`
+}, error) {
+
+ vars := make(map[string]interface{})
+
+ vars["totp"] = totp
+ url := fmt.Sprintf("%s/api/v2/mfa/totp/verify", c.Host)
+ b, err := c.SendHttpRestRequest(url, http.MethodPost, &token, vars)
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data interface{} `json:"data"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return resp, nil
+}
+
+// VerifyAppSmsMfa
+// 检验二次验证 MFA 短信验证码
+func (c *Client) VerifyAppSmsMfa(phone, code, token string) (*struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data interface{} `json:"data"`
+}, error) {
+
+ vars := map[string]interface{}{
+ "code": code,
+ "phone": phone,
+ }
+
+ url := fmt.Sprintf("%s/api/v2/applications/mfa/sms/verify", c.Host)
+ b, err := c.SendHttpRestRequest(url, http.MethodPost, &token, vars)
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data interface{} `json:"data"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return resp, nil
+}
+
+// VerifyAppEmailMfa
+// 检验二次验证 MFA 邮箱验证码
+func (c *Client) VerifyAppEmailMfa(email, code, token string) (*struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data interface{} `json:"data"`
+}, error) {
+
+ vars := map[string]interface{}{
+ "code": code,
+ "email": email,
+ }
+
+ url := fmt.Sprintf("%s/api/v2/applications/mfa/email/verify", c.Host)
+ b, err := c.SendHttpRestRequest(url, http.MethodPost, &token, vars)
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data interface{} `json:"data"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return resp, nil
+}
+
+// PhoneOrEmailBindable
+// 检测手机号或邮箱是否已被绑定
+func (c *Client) PhoneOrEmailBindable(email, phone *string, token string) (*struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data interface{} `json:"data"`
+}, error) {
+
+ vars := make(map[string]interface{})
+ if email != nil {
+ vars["email"] = email
+ }
+ if phone != nil {
+ vars["phone"] = phone
+ }
+
+ url := fmt.Sprintf("%s/api/v2/applications/mfa/check", c.Host)
+ b, err := c.SendHttpRestRequest(url, http.MethodPost, &token, vars)
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data interface{} `json:"data"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return resp, nil
+}
+
+// VerifyTotpRecoveryCode
+// 检验二次验证 MFA 恢复代码
+func (c *Client) VerifyTotpRecoveryCode(code, token string) (*struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data interface{} `json:"data"`
+}, error) {
+
+ vars := make(map[string]interface{})
+
+ vars["recoveryCode"] = code
+ url := fmt.Sprintf("%s/api/v2/mfa/totp/recovery", c.Host)
+ b, err := c.SendHttpRestRequest(url, http.MethodPost, &token, vars)
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data interface{} `json:"data"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return resp, nil
+}
+
+// AssociateFaceByUrl
+// 通过图片 URL 绑定人脸
+func (c *Client) AssociateFaceByUrl(baseFaceUrl, CompareFaceUrl, token string) (*struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data interface{} `json:"data"`
+}, error) {
+
+ vars := map[string]interface{}{
+ "photoA": baseFaceUrl,
+ "photoB": CompareFaceUrl,
+ "isExternal": true,
+ }
+ url := fmt.Sprintf("%s/api/v2/mfa/face/associate", c.Host)
+ b, err := c.SendHttpRestRequest(url, http.MethodPost, &token, vars)
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data interface{} `json:"data"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return resp, nil
+}
+
+// VerifyFaceMfa
+// 人脸二次认证
+func (c *Client) VerifyFaceMfa(faceUrl, token string) (*struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data interface{} `json:"data"`
+}, error) {
+
+ vars := map[string]interface{}{
+ "photo": faceUrl,
+ }
+ url := fmt.Sprintf("%s/api/v2/mfa/face/associate", c.Host)
+ b, err := c.SendHttpRestRequest(url, http.MethodPost, &token, vars)
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data interface{} `json:"data"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return resp, nil
+}
diff --git a/lib/authentication/mfa_client_test.go b/lib/authentication/mfa_client_test.go
new file mode 100644
index 0000000..d79f124
--- /dev/null
+++ b/lib/authentication/mfa_client_test.go
@@ -0,0 +1,183 @@
+package authentication
+
+import (
+ "fmt"
+ "github.com/Authing/authing-go-sdk/lib/model"
+ "log"
+ "testing"
+)
+
+func TestClient_GetMfaAuthenticators(t *testing.T) {
+ authenticationClient := NewClient(AppId, Secret)
+ authenticationClient.UserPoolId = UserPool
+ loginReq := model.LoginByEmailInput{
+ Email: "fptvmzqyxn@authing.cn",
+ Password: "12345678",
+ }
+ u, e := authenticationClient.LoginByEmail(loginReq)
+ //log.Println(u)
+ log.Println(e)
+ resp, err := authenticationClient.GetMfaAuthenticators(&model.MfaInput{
+ MfaToken: u.Token,
+ })
+ if err != nil {
+ fmt.Println(err)
+ } else {
+ fmt.Println(resp)
+ }
+}
+
+func TestClient_AssociateMfaAuthenticator(t *testing.T) {
+ authenticationClient := NewClient(AppId, Secret)
+ authenticationClient.UserPoolId = UserPool
+ loginReq := model.LoginByEmailInput{
+ Email: "fptvmzqyxn@authing.cn",
+ Password: "12345678",
+ }
+ u, e := authenticationClient.LoginByEmail(loginReq)
+ log.Println(e)
+ resp, err := authenticationClient.AssociateMfaAuthenticator(&model.MfaInput{
+ MfaToken: u.Token,
+ })
+ if err != nil {
+ fmt.Println(err)
+ } else {
+ fmt.Println(resp)
+ }
+}
+
+func TestClient_DeleteMfaAuthenticator(t *testing.T) {
+ authenticationClient := NewClient(AppId, Secret)
+ authenticationClient.UserPoolId = UserPool
+ resp, err := authenticationClient.DeleteMfaAuthenticator()
+ if err != nil {
+ fmt.Println(err)
+ } else {
+ fmt.Println(resp)
+ }
+}
+
+func TestClient_ConfirmAssociateMfaAuthenticator(t *testing.T) {
+ authenticationClient := NewClient(AppId, Secret)
+ authenticationClient.UserPoolId = UserPool
+ //loginReq:= model.LoginByEmailInput{
+ // Email: "fptvmzqyxn@authing.cn",
+ // Password: "12345678",
+ //}
+ //u,e:=authenticationClient.LoginByEmail(loginReq)
+ //log.Println(e)
+ resp, err := authenticationClient.ConfirmAssociateMfaAuthenticator(&model.ConfirmAssociateMfaAuthenticatorRequest{
+ Totp: "D5LH4GQQGEEWEHKX",
+ //Totp: "c833-cbb6-9180-7240-a048-ebe6",
+ //MfaToken: u.Token,
+ })
+ if err != nil {
+ fmt.Println(err)
+ } else {
+ fmt.Println(resp)
+ }
+}
+
+func TestClient_VerifyTotpMfa(t *testing.T) {
+ authenticationClient := NewClient(AppId, Secret)
+ authenticationClient.UserPoolId = UserPool
+ mfaToken := "eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJkYXRhIjp7InVzZXJQb29sSWQiOiI2MGMxN2IzZDcyYjkyNTA5N2E3MzhkODYiLCJ1c2VySWQiOiI2MTc2NWYxMDI5MThhOGZjNjUyNDU2NDAiLCJhcm4iOiJhcm46Y246YXV0aGluZzo2MGMxN2IzZDcyYjkyNTA5N2E3MzhkODY6dXNlcjo2MTc2NWYxMDI5MThhOGZjNjUyNDU2NDAiLCJzdGFnZSI6MX0sImlhdCI6MTYzNTE0OTQ2MiwiZXhwIjoxNjM1MTQ5ODIyfQ.2DbmVf1-JQeiRMpZBk-3y-uPIN15FL-ranE4UlMKMoM"
+
+ resp, err := authenticationClient.VerifyTotpMfa("q", mfaToken)
+ if err != nil {
+ fmt.Println(err)
+ } else {
+ fmt.Println(resp)
+ }
+}
+
+func TestClient_VerifyAppSmsMfa(t *testing.T) {
+ authenticationClient := NewClient(AppId, Secret)
+ authenticationClient.UserPoolId = UserPool
+ loginReq := model.LoginByEmailInput{
+ Email: "gosdk@mail.com",
+ Password: "123456789",
+ }
+ u, e := authenticationClient.LoginByEmail(loginReq)
+ log.Println(e)
+ resp, err := authenticationClient.VerifyAppSmsMfa("777777", "q", *u.Token)
+ if err != nil {
+ fmt.Println(err)
+ } else {
+ fmt.Println(resp)
+ }
+}
+
+func TestClient_VerifyAppEmailMfa(t *testing.T) {
+ authenticationClient := NewClient(AppId, Secret)
+ authenticationClient.UserPoolId = UserPool
+ loginReq := model.LoginByEmailInput{
+ Email: "gosdk@mail.com",
+ Password: "123456789",
+ }
+ u, e := authenticationClient.LoginByEmail(loginReq)
+ log.Println(u, e)
+ mfaToken := "eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJkYXRhIjp7InVzZXJQb29sSWQiOiI2MGMxN2IzZDcyYjkyNTA5N2E3MzhkODYiLCJ1c2VySWQiOiI2MTc2NWYxMDI5MThhOGZjNjUyNDU2NDAiLCJhcm4iOiJhcm46Y246YXV0aGluZzo2MGMxN2IzZDcyYjkyNTA5N2E3MzhkODY6dXNlcjo2MTc2NWYxMDI5MThhOGZjNjUyNDU2NDAiLCJzdGFnZSI6MX0sImlhdCI6MTYzNTE0OTQ2MiwiZXhwIjoxNjM1MTQ5ODIyfQ.2DbmVf1-JQeiRMpZBk-3y-uPIN15FL-ranE4UlMKMoM"
+
+ resp, err := authenticationClient.VerifyAppEmailMfa("gosdk@mail.com", "q", mfaToken)
+ if err != nil {
+ fmt.Println(err)
+ } else {
+ fmt.Println(resp)
+ }
+}
+
+func TestClient_PhoneOrEmailBindable(t *testing.T) {
+ authenticationClient := NewClient(AppId, Secret)
+ authenticationClient.UserPoolId = UserPool
+ mfaToken := "eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJkYXRhIjp7InVzZXJQb29sSWQiOiI2MGMxN2IzZDcyYjkyNTA5N2E3MzhkODYiLCJ1c2VySWQiOiI2MTc2NWYxMDI5MThhOGZjNjUyNDU2NDAiLCJhcm4iOiJhcm46Y246YXV0aGluZzo2MGMxN2IzZDcyYjkyNTA5N2E3MzhkODY6dXNlcjo2MTc2NWYxMDI5MThhOGZjNjUyNDU2NDAiLCJzdGFnZSI6MX0sImlhdCI6MTYzNTE0OTQ2MiwiZXhwIjoxNjM1MTQ5ODIyfQ.2DbmVf1-JQeiRMpZBk-3y-uPIN15FL-ranE4UlMKMoM"
+ email := "gosdk@mail.com"
+ resp, err := authenticationClient.PhoneOrEmailBindable(&email, nil, mfaToken)
+ if err != nil {
+ fmt.Println(err)
+ } else {
+ fmt.Println(resp)
+ }
+}
+
+func TestClient_VerifyFaceMfa(t *testing.T) {
+ authenticationClient := NewClient(AppId, Secret)
+ authenticationClient.UserPoolId = UserPool
+
+ mfaToken := "eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJkYXRhIjp7InVzZXJQb29sSWQiOiI2MGMxN2IzZDcyYjkyNTA5N2E3MzhkODYiLCJ1c2VySWQiOiI2MTc2NWYxMDI5MThhOGZjNjUyNDU2NDAiLCJhcm4iOiJhcm46Y246YXV0aGluZzo2MGMxN2IzZDcyYjkyNTA5N2E3MzhkODY6dXNlcjo2MTc2NWYxMDI5MThhOGZjNjUyNDU2NDAiLCJzdGFnZSI6MX0sImlhdCI6MTYzNTE0OTQ2MiwiZXhwIjoxNjM1MTQ5ODIyfQ.2DbmVf1-JQeiRMpZBk-3y-uPIN15FL-ranE4UlMKMoM"
+
+ resp, err := authenticationClient.VerifyFaceMfa("http://face", mfaToken)
+ if err != nil {
+ fmt.Println(err)
+ } else {
+ fmt.Println(resp)
+ }
+}
+
+func TestClient_AssociateFaceByUrl(t *testing.T) {
+ authenticationClient := NewClient(AppId, Secret)
+ authenticationClient.UserPoolId = UserPool
+
+ mfaToken := "eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJkYXRhIjp7InVzZXJQb29sSWQiOiI2MGMxN2IzZDcyYjkyNTA5N2E3MzhkODYiLCJ1c2VySWQiOiI2MTc2NWYxMDI5MThhOGZjNjUyNDU2NDAiLCJhcm4iOiJhcm46Y246YXV0aGluZzo2MGMxN2IzZDcyYjkyNTA5N2E3MzhkODY6dXNlcjo2MTc2NWYxMDI5MThhOGZjNjUyNDU2NDAiLCJzdGFnZSI6MX0sImlhdCI6MTYzNTE0OTQ2MiwiZXhwIjoxNjM1MTQ5ODIyfQ.2DbmVf1-JQeiRMpZBk-3y-uPIN15FL-ranE4UlMKMoM"
+
+ resp, err := authenticationClient.AssociateFaceByUrl("http://tp", "http://zp", mfaToken)
+ if err != nil {
+ fmt.Println(err)
+ } else {
+ fmt.Println(resp)
+ }
+}
+
+func TestClient_VerifyTotpRecoveryCode(t *testing.T) {
+ authenticationClient := NewClient(AppId, Secret)
+ authenticationClient.UserPoolId = UserPool
+
+ mfaToken := "eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJkYXRhIjp7InVzZXJQb29sSWQiOiI2MGMxN2IzZDcyYjkyNTA5N2E3MzhkODYiLCJ1c2VySWQiOiI2MTc2NWYxMDI5MThhOGZjNjUyNDU2NDAiLCJhcm4iOiJhcm46Y246YXV0aGluZzo2MGMxN2IzZDcyYjkyNTA5N2E3MzhkODY6dXNlcjo2MTc2NWYxMDI5MThhOGZjNjUyNDU2NDAiLCJzdGFnZSI6MX0sImlhdCI6MTYzNTE0OTQ2MiwiZXhwIjoxNjM1MTQ5ODIyfQ.2DbmVf1-JQeiRMpZBk-3y-uPIN15FL-ranE4UlMKMoM"
+
+ resp, err := authenticationClient.VerifyTotpMfa("eedc-58ed-931b-8967-a092-46ae", mfaToken)
+ if err != nil {
+ fmt.Println(err)
+ } else {
+ fmt.Println(resp)
+ }
+}
diff --git a/lib/constant/constant.go b/lib/constant/constant.go
new file mode 100644
index 0000000..0782c33
--- /dev/null
+++ b/lib/constant/constant.go
@@ -0,0 +1,5 @@
+package constant
+
+const StringEmpty = ""
+
+const PublicKey = "MIGfMA0GCSqGSIb3DQEBAQUAA4GNADCBiQKBgQC4xKeUgQ+Aoz7TLfAfs9+paePb5KIofVthEopwrXFkp8OCeocaTHt9ICjTT2QeJh6cZaDaArfZ873GPUn00eOIZ7Ae+TiA2BKHbCvloW3w5Lnqm70iSsUi5Fmu9/2+68GZRH9L7Mlh8cFksCicW2Y2W2uMGKl64GDcIq3au+aqJQIDAQAB"
diff --git a/lib/constant/enums.go b/lib/constant/enums.go
new file mode 100644
index 0000000..bccc64c
--- /dev/null
+++ b/lib/constant/enums.go
@@ -0,0 +1,145 @@
+package constant
+
+const (
+ HttpMethodGet = "GET"
+ HttpMethodPost = "POST"
+)
+
+const (
+ CoreAuthingDefaultUrl = "https://core.authing.cn"
+ CoreAuthingGraphqlPath = "/graphql/v2"
+
+ /**
+ * token 过期时间
+ */
+ AccessTokenExpiresAt int64 = 0
+
+ /**
+ * 应用 Id
+ */
+ AppId = ""
+
+ //应用密钥
+ Secret = ""
+ //应用身份协议
+ Protocol = "oidc"
+ //获取 token 端点认证方式
+ TokenEndPointAuthMethod = ClientSecretPost
+ //检查 token 端点认证方式
+ IntrospectionEndPointAuthMethod = ClientSecretPost
+ //撤回 token 端点认证方式
+ RevocationEndPointAuthMethod = ClientSecretPost
+
+ //应用回调地址
+ RedirectUri = ""
+ //Websocket 服务器域名
+ WebsocketHost = ""
+
+ SdkType = "SDK"
+ SdkVersion = "go:2.0.0"
+
+ // TokenCacheKeyPrefix token缓存key前缀
+ TokenCacheKeyPrefix = "token_"
+ UserCacheKeyPrefix = "user_"
+)
+
+type ProtocolEnum string
+
+const (
+ OAUTH ProtocolEnum = "oauth"
+ OIDC ProtocolEnum = "oidc"
+ CAS ProtocolEnum = "cas"
+ SAML ProtocolEnum = "saml"
+)
+
+type AuthMethodEnum string
+
+const (
+ ClientSecretPost = "client_secret_post"
+ ClientSecretBasic = "client_secret_basic"
+ None = "none"
+)
+
+type ResourceTargetTypeEnum string
+
+const (
+ USER ResourceTargetTypeEnum = "USER"
+ ROLE ResourceTargetTypeEnum = "ROLE"
+ GROUP ResourceTargetTypeEnum = "GROUP"
+ ORG ResourceTargetTypeEnum = "ORG"
+)
+
+type ApplicationDefaultAccessPolicies string
+
+const (
+ AllowAll ApplicationDefaultAccessPolicies = "ALLOW_ALL"
+ DenyAll ApplicationDefaultAccessPolicies = "DENY_ALL"
+)
+
+type GetAuthorizedTargetsOpt string
+
+const (
+ AND GetAuthorizedTargetsOpt = "AND"
+ OR GetAuthorizedTargetsOpt = "OR"
+)
+
+type ProviderTypeEnum string
+
+const (
+ DingTalk ProviderTypeEnum = "dingtalk"
+ WechatWork ProviderTypeEnum = "wechatwork"
+ AD ProviderTypeEnum = "ad"
+)
+
+type PrincipalAuthenticateType string
+
+const (
+ P PrincipalAuthenticateType = "P"
+ E PrincipalAuthenticateType = "E"
+)
+
+type MfaSource string
+
+const (
+ Self MfaSource = "SELF"
+ Application MfaSource = "APPLICATION"
+)
+
+type SocialProviderType string
+
+const (
+ WECHATPC SocialProviderType = "wechat:pc"
+ GITHUB SocialProviderType = "github"
+ GOOGLE SocialProviderType = "google"
+ QQ SocialProviderType = "qq"
+ APPLE SocialProviderType = "apple"
+ BAIDU SocialProviderType = "baidu"
+ ALIPAY SocialProviderType = "alipay"
+ LARK_APP_STORE SocialProviderType = "lark:app-store"
+ LARK_CUSTOM_APP SocialProviderType = "lark:custom-app"
+ WEIBO SocialProviderType = "weibo"
+ DINGTALK SocialProviderType = "dingtalk"
+ WECHAT_WEB SocialProviderType = "wechat:webpage-authorization"
+ ALIPAY_MOBILE SocialProviderType = "alipay"
+ WECHAT_MQ_DEFAULT SocialProviderType = "wechat:miniprogram:default"
+ WECHAT_MOBILE SocialProviderType = "wechat:mobile"
+ WECHATWORK_SP_AUTHZ SocialProviderType = "wechatwork:service-provider:authorization"
+ WECHATWORK_SP_QR SocialProviderType = "wechatwork:service-provider:qrconnect"
+ WECHATWORK_CORP_QR SocialProviderType = "wechatwork:corp:qrconnect"
+ WECHAT_MP_AL SocialProviderType = "wechat:miniprogram:app-launch"
+ WECHAT_MP_QR SocialProviderType = "wechat:miniprogram:qrconnect"
+)
+
+type GenerateCodeChallengeMethod string
+
+const (
+ PLAIN GenerateCodeChallengeMethod = "plain"
+ S256 GenerateCodeChallengeMethod = "S256"
+)
+
+type TicketFormat string
+
+const (
+ XML TicketFormat = "XML"
+ JSON TicketFormat = "JSON"
+)
diff --git a/lib/constant/gql.go b/lib/constant/gql.go
new file mode 100644
index 0000000..b0db1ab
--- /dev/null
+++ b/lib/constant/gql.go
@@ -0,0 +1,814 @@
+package constant
+
+const AccessTokenDocument = `query accessToken($userPoolId: String!, $secret: String!) {
+ accessToken(userPoolId: $userPoolId, secret: $secret) {
+ accessToken
+ exp
+ iat
+ }
+}`
+
+const NodeByIdWithMembersDocument = `
+ query nodeByIdWithMembers($page: Int, $limit: Int, $sortBy: SortByEnum, $includeChildrenNodes: Boolean, $id: String!) {
+ nodeById(id: $id) {
+ id
+ orgId
+ name
+ nameI18n
+ description
+ descriptionI18n
+ order
+ code
+ root
+ depth
+ createdAt
+ updatedAt
+ children
+ users(page: $page, limit: $limit, sortBy: $sortBy, includeChildrenNodes: $includeChildrenNodes) {
+ totalCount
+ list {
+ id
+ arn
+ userPoolId
+ status
+ username
+ email
+ emailVerified
+ phone
+ phoneVerified
+ unionid
+ openid
+ nickname
+ registerSource
+ photo
+ password
+ oauth
+ token
+ tokenExpiredAt
+ loginsCount
+ lastLogin
+ lastIP
+ signedUp
+ blocked
+ isDeleted
+ device
+ browser
+ company
+ name
+ givenName
+ familyName
+ middleName
+ profile
+ preferredUsername
+ website
+ gender
+ birthdate
+ zoneinfo
+ locale
+ address
+ formatted
+ streetAddress
+ locality
+ region
+ postalCode
+ city
+ province
+ country
+ createdAt
+ updatedAt
+ externalId
+ }
+ }
+ }
+}
+ `
+
+const UsersDocument = `
+ query users($page: Int, $limit: Int, $sortBy: SortByEnum) {
+ users(page: $page, limit: $limit, sortBy: $sortBy) {
+ totalCount
+ list {
+ id
+ arn
+ userPoolId
+ status
+ username
+ email
+ emailVerified
+ phone
+ phoneVerified
+ unionid
+ openid
+ nickname
+ registerSource
+ photo
+ password
+ oauth
+ token
+ tokenExpiredAt
+ loginsCount
+ lastLogin
+ lastIP
+ signedUp
+ blocked
+ isDeleted
+ device
+ browser
+ company
+ name
+ givenName
+ familyName
+ middleName
+ profile
+ preferredUsername
+ website
+ gender
+ birthdate
+ zoneinfo
+ locale
+ address
+ formatted
+ streetAddress
+ locality
+ region
+ postalCode
+ city
+ province
+ country
+ createdAt
+ updatedAt
+ externalId
+ }
+ }
+}
+ `
+
+const OrgDocument = `
+ query org($id: String!) {
+ org(id: $id) {
+ id
+ rootNode {
+ id
+ orgId
+ name
+ nameI18n
+ description
+ descriptionI18n
+ order
+ code
+ root
+ depth
+ path
+ createdAt
+ updatedAt
+ children
+ }
+ nodes {
+ id
+ orgId
+ name
+ nameI18n
+ description
+ descriptionI18n
+ order
+ code
+ root
+ depth
+ path
+ createdAt
+ updatedAt
+ children
+ }
+ }
+}
+ `
+
+const GetUserDepartmentsDocument = `
+ query getUserDepartments($id: String!, $orgId: String) {
+ user(id: $id) {
+ departments(orgId: $orgId) {
+ totalCount
+ list {
+ department {
+ id
+ orgId
+ name
+ nameI18n
+ description
+ descriptionI18n
+ order
+ code
+ root
+ depth
+ path
+ codePath
+ namePath
+ createdAt
+ updatedAt
+ children
+ }
+ isMainDepartment
+ joinedAt
+ }
+ }
+ }
+}
+ `
+
+const LoginByEmailDocument = `
+ mutation loginByEmail($input: LoginByEmailInput!) {
+ loginByEmail(input: $input) {
+ id
+ arn
+ status
+ userPoolId
+ username
+ email
+ emailVerified
+ phone
+ phoneVerified
+ unionid
+ openid
+ nickname
+ registerSource
+ photo
+ password
+ oauth
+ token
+ tokenExpiredAt
+ loginsCount
+ lastLogin
+ lastIP
+ signedUp
+ blocked
+ isDeleted
+ device
+ browser
+ company
+ name
+ givenName
+ familyName
+ middleName
+ profile
+ preferredUsername
+ website
+ gender
+ birthdate
+ zoneinfo
+ locale
+ address
+ formatted
+ streetAddress
+ locality
+ region
+ postalCode
+ city
+ province
+ country
+ createdAt
+ updatedAt
+ externalId
+ }
+}
+ `
+
+const LoginByPhoneCodeDocument = `
+ mutation loginByPhoneCode($input: LoginByPhoneCodeInput!) {
+ loginByPhoneCode(input: $input) {
+ id
+ arn
+ userPoolId
+ status
+ username
+ email
+ emailVerified
+ phone
+ phoneVerified
+ unionid
+ openid
+ nickname
+ registerSource
+ photo
+ password
+ oauth
+ token
+ tokenExpiredAt
+ loginsCount
+ lastLogin
+ lastIP
+ signedUp
+ blocked
+ isDeleted
+ device
+ browser
+ company
+ name
+ givenName
+ familyName
+ middleName
+ profile
+ preferredUsername
+ website
+ gender
+ birthdate
+ zoneinfo
+ locale
+ address
+ formatted
+ streetAddress
+ locality
+ region
+ postalCode
+ city
+ province
+ country
+ createdAt
+ updatedAt
+ externalId
+ }
+}
+ `
+
+const LoginByPhonePasswordDocument = `
+ mutation loginByPhonePassword($input: LoginByPhonePasswordInput!) {
+ loginByPhonePassword(input: $input) {
+ id
+ arn
+ userPoolId
+ status
+ username
+ email
+ emailVerified
+ phone
+ phoneVerified
+ unionid
+ openid
+ nickname
+ registerSource
+ photo
+ password
+ oauth
+ token
+ tokenExpiredAt
+ loginsCount
+ lastLogin
+ lastIP
+ signedUp
+ blocked
+ isDeleted
+ device
+ browser
+ company
+ name
+ givenName
+ familyName
+ middleName
+ profile
+ preferredUsername
+ website
+ gender
+ birthdate
+ zoneinfo
+ locale
+ address
+ formatted
+ streetAddress
+ locality
+ region
+ postalCode
+ city
+ province
+ country
+ createdAt
+ updatedAt
+ externalId
+ }
+}
+ `
+
+const LoginBySubAccountDocument = `
+ mutation loginBySubAccount($account: String!, $password: String!, $captchaCode: String, $clientIp: String) {
+ loginBySubAccount(account: $account, password: $password, captchaCode: $captchaCode, clientIp: $clientIp) {
+ id
+ arn
+ status
+ userPoolId
+ username
+ email
+ emailVerified
+ phone
+ phoneVerified
+ unionid
+ openid
+ nickname
+ registerSource
+ photo
+ password
+ oauth
+ token
+ tokenExpiredAt
+ loginsCount
+ lastLogin
+ lastIP
+ signedUp
+ blocked
+ isDeleted
+ device
+ browser
+ company
+ name
+ givenName
+ familyName
+ middleName
+ profile
+ preferredUsername
+ website
+ gender
+ birthdate
+ zoneinfo
+ locale
+ address
+ formatted
+ streetAddress
+ locality
+ region
+ postalCode
+ city
+ province
+ country
+ createdAt
+ updatedAt
+ externalId
+ }
+}
+ `
+
+const LoginByUsernameDocument = `
+ mutation loginByUsername($input: LoginByUsernameInput!) {
+ loginByUsername(input: $input) {
+ id
+ arn
+ userPoolId
+ status
+ username
+ email
+ emailVerified
+ phone
+ phoneVerified
+ unionid
+ openid
+ nickname
+ registerSource
+ photo
+ password
+ oauth
+ token
+ tokenExpiredAt
+ loginsCount
+ lastLogin
+ lastIP
+ signedUp
+ blocked
+ isDeleted
+ device
+ browser
+ company
+ name
+ givenName
+ familyName
+ middleName
+ profile
+ preferredUsername
+ website
+ gender
+ birthdate
+ zoneinfo
+ locale
+ address
+ formatted
+ streetAddress
+ locality
+ region
+ postalCode
+ city
+ province
+ country
+ createdAt
+ updatedAt
+ externalId
+ }
+}
+ `
+
+const UsersWithCustomDocument = `
+ query usersWithCustomData($page: Int, $limit: Int, $sortBy: SortByEnum, $excludeUsersInOrg: Boolean) {
+ users(page: $page, limit: $limit, sortBy: $sortBy, excludeUsersInOrg: $excludeUsersInOrg) {
+ totalCount
+ list {
+ id
+ identities {
+ openid
+ userIdInIdp
+ userId
+ extIdpId
+ isSocial
+ provider
+ type
+ userPoolId
+ }
+ arn
+ userPoolId
+ status
+ username
+ email
+ emailVerified
+ phone
+ phoneVerified
+ unionid
+ openid
+ nickname
+ registerSource
+ photo
+ password
+ oauth
+ token
+ tokenExpiredAt
+ loginsCount
+ lastLogin
+ lastIP
+ signedUp
+ blocked
+ isDeleted
+ device
+ browser
+ company
+ name
+ givenName
+ familyName
+ middleName
+ profile
+ preferredUsername
+ website
+ gender
+ birthdate
+ zoneinfo
+ locale
+ address
+ formatted
+ streetAddress
+ locality
+ region
+ postalCode
+ city
+ province
+ country
+ createdAt
+ updatedAt
+ externalId
+ customData {
+ key
+ value
+ dataType
+ label
+ }
+ }
+ }
+}
+
+ `
+
+const IsActionAllowedDocument = `
+ query isActionAllowed($resource: String!, $action: String!, $userId: String!, $namespace: String) {
+ isActionAllowed(resource: $resource, action: $action, userId: $userId, namespace: $namespace)
+}
+ `
+
+const AllowDocument = `
+ mutation allow($resource: String!, $action: String!, $userId: String, $userIds: [String!], $roleCode: String, $roleCodes: [String!], $namespace: String) {
+ allow(resource: $resource, action: $action, userId: $userId, userIds: $userIds, roleCode: $roleCode, roleCodes: $roleCodes, namespace: $namespace) {
+ message
+ code
+ }
+}
+ `
+
+const AuthorizeResourceDocument = `
+ mutation authorizeResource($namespace: String, $resource: String, $resourceType: ResourceType, $opts: [AuthorizeResourceOpt]) {
+ authorizeResource(namespace: $namespace, resource: $resource, resourceType: $resourceType, opts: $opts) {
+ code
+ message
+ }
+}
+ `
+
+const UpdateUserPoolDocument = `
+mutation updateUserpool($input: UpdateUserpoolInput!) {
+ updateUserpool(input: $input) {
+ id
+ name
+ domain
+ description
+ secret
+ jwtSecret
+ userpoolTypes {
+ code
+ name
+ description
+ image
+ sdks
+ }
+ logo
+ createdAt
+ updatedAt
+ emailVerifiedDefault
+ sendWelcomeEmail
+ registerDisabled
+ appSsoEnabled
+ showWxQRCodeWhenRegisterDisabled
+ allowedOrigins
+ tokenExpiresAfter
+ isDeleted
+ frequentRegisterCheck {
+ timeInterval
+ limit
+ enabled
+ }
+ loginFailCheck {
+ timeInterval
+ limit
+ enabled
+ }
+ loginFailStrategy
+ loginPasswordFailCheck {
+ timeInterval
+ limit
+ enabled
+ }
+ changePhoneStrategy {
+ verifyOldPhone
+ }
+ changeEmailStrategy {
+ verifyOldEmail
+ }
+ qrcodeLoginStrategy {
+ qrcodeExpiresAfter
+ returnFullUserInfo
+ allowExchangeUserInfoFromBrowser
+ ticketExpiresAfter
+ }
+ app2WxappLoginStrategy {
+ ticketExpriresAfter
+ ticketExchangeUserInfoNeedSecret
+ }
+ whitelist {
+ phoneEnabled
+ emailEnabled
+ usernameEnabled
+ }
+ customSMSProvider {
+ enabled
+ provider
+ config
+ }
+ packageType
+ useCustomUserStore
+ loginRequireEmailVerified
+ verifyCodeLength
+ }
+}
+
+`
+const WhileListDocument = `
+query whitelist($type: WhitelistType!) {
+ whitelist(type: $type) {
+ createdAt
+ updatedAt
+ value
+ }
+}
+`
+const AddWhileListDocument = `
+mutation addWhitelist($type: WhitelistType!, $list: [String!]!) {
+ addWhitelist(type: $type, list: $list) {
+ createdAt
+ updatedAt
+ value
+ }
+}
+`
+
+const RemoveWhileListDocument = `
+mutation removeWhitelist($type: WhitelistType!, $list: [String!]!) {
+ removeWhitelist(type: $type, list: $list) {
+ createdAt
+ updatedAt
+ value
+ }
+}
+`
+
+const ListAuthorizedResourcesDocument = `
+query authorizedResources($targetType: PolicyAssignmentTargetType, $targetIdentifier: String, $namespace: String, $resourceType: String) {
+ authorizedResources(targetType: $targetType, targetIdentifier: $targetIdentifier, namespace: $namespace, resourceType: $resourceType) {
+ totalCount
+ list {
+ code
+ type
+ actions
+ }
+ }
+}
+`
+const GetAuthorizedTargetsDocument = `
+query authorizedTargets($namespace: String!, $resourceType: ResourceType!, $resource: String!, $targetType: PolicyAssignmentTargetType, $actions: AuthorizedTargetsActionsInput) {
+ authorizedTargets(namespace: $namespace, resource: $resource, resourceType: $resourceType, targetType: $targetType, actions: $actions) {
+ totalCount
+ list {
+ targetType
+ targetIdentifier
+ actions
+ }
+ }
+}
+`
+
+const GetAuthorizedTargetsCodeDocument = `
+query authorizedTargetsCode($namespace: String!, $resourceType: ResourceType!, $resource: String!, $targetType: PolicyAssignmentTargetType, $actions: AuthorizedTargetsActionsInput) {
+ authorizedTargetsCode(namespace: $namespace, resource: $resource, resourceType: $resourceType, targetType: $targetType, actions: $actions) {
+ totalCount
+ list {
+ targetType
+ targetIdentifier
+ actions
+ }
+ }
+}
+`
+
+const SendMailDocument = `
+mutation sendEmail($email: String!, $scene: EmailScene!) {
+ sendEmail(email: $email, scene: $scene) {
+ message
+ code
+ }
+}
+`
+
+const CheckLoginStatusDocument = `
+query checkLoginStatus($token: String) {
+ checkLoginStatus(token: $token) {
+ code
+ message
+ status
+ exp
+ iat
+ data {
+ id
+ userPoolId
+ arn
+ }
+ }
+}
+`
+
+const ListUdfDocument = `
+query udf($targetType: UDFTargetType!) {
+ udf(targetType: $targetType) {
+ targetType
+ dataType
+ key
+ label
+ options
+ }
+}`
+
+const SetUdfDocument = `
+mutation setUdf($targetType: UDFTargetType!, $key: String!, $dataType: UDFDataType!, $label: String!, $options: String) {
+ setUdf(targetType: $targetType, key: $key, dataType: $dataType, label: $label, options: $options) {
+ targetType
+ dataType
+ key
+ label
+ options
+ }
+}
+`
+const RemoveUdfDocument = `
+mutation removeUdf($targetType: UDFTargetType!, $key: String!) {
+ removeUdf(targetType: $targetType, key: $key) {
+ message
+ code
+ }
+}
+`
+
+const UdvDocument = `
+query udv($targetType: UDFTargetType!, $targetId: String!) {
+ udv(targetType: $targetType, targetId: $targetId) {
+ key
+ dataType
+ value
+ label
+ }
+}
+`
diff --git a/lib/constant/gql_authentication.go b/lib/constant/gql_authentication.go
new file mode 100644
index 0000000..bae0e36
--- /dev/null
+++ b/lib/constant/gql_authentication.go
@@ -0,0 +1,664 @@
+package constant
+
+const RegisterByEmailDocument = `
+mutation registerByEmail($input: RegisterByEmailInput!) {
+ registerByEmail(input: $input) {
+ id
+ arn
+ userPoolId
+ status
+ username
+ email
+ emailVerified
+ phone
+ phoneVerified
+ unionid
+ openid
+ nickname
+ registerSource
+ photo
+ password
+ oauth
+ token
+ tokenExpiredAt
+ loginsCount
+ lastLogin
+ lastIP
+ signedUp
+ blocked
+ isDeleted
+ device
+ browser
+ company
+ name
+ givenName
+ familyName
+ middleName
+ profile
+ preferredUsername
+ website
+ gender
+ birthdate
+ zoneinfo
+ locale
+ address
+ formatted
+ streetAddress
+ locality
+ region
+ postalCode
+ city
+ province
+ country
+ createdAt
+ updatedAt
+ externalId
+ }
+}
+
+`
+
+const RegisterByUsernameDocument = `
+mutation registerByUsername($input: RegisterByUsernameInput!) {
+ registerByUsername(input: $input) {
+ id
+ arn
+ userPoolId
+ status
+ username
+ email
+ emailVerified
+ phone
+ phoneVerified
+ unionid
+ openid
+ nickname
+ registerSource
+ photo
+ password
+ oauth
+ token
+ tokenExpiredAt
+ loginsCount
+ lastLogin
+ lastIP
+ signedUp
+ blocked
+ isDeleted
+ device
+ browser
+ company
+ name
+ givenName
+ familyName
+ middleName
+ profile
+ preferredUsername
+ website
+ gender
+ birthdate
+ zoneinfo
+ locale
+ address
+ formatted
+ streetAddress
+ locality
+ region
+ postalCode
+ city
+ province
+ country
+ createdAt
+ updatedAt
+ externalId
+ }
+}
+
+`
+
+const RegisterByPhoneCodeDocument = `
+mutation registerByPhoneCode($input: RegisterByPhoneCodeInput!) {
+ registerByPhoneCode(input: $input) {
+ id
+ arn
+ userPoolId
+ status
+ username
+ email
+ emailVerified
+ phone
+ phoneVerified
+ unionid
+ openid
+ nickname
+ registerSource
+ photo
+ password
+ oauth
+ token
+ tokenExpiredAt
+ loginsCount
+ lastLogin
+ lastIP
+ signedUp
+ blocked
+ isDeleted
+ device
+ browser
+ company
+ name
+ givenName
+ familyName
+ middleName
+ profile
+ preferredUsername
+ website
+ gender
+ birthdate
+ zoneinfo
+ locale
+ address
+ formatted
+ streetAddress
+ locality
+ region
+ postalCode
+ city
+ province
+ country
+ createdAt
+ updatedAt
+ externalId
+ }
+}
+
+`
+
+const CheckPasswordStrengthDocument = `
+query checkPasswordStrength($password: String!) {
+ checkPasswordStrength(password: $password) {
+ valid
+ message
+ }
+}
+`
+
+const ResetPasswordDocument = `
+mutation resetPassword($phone: String, $email: String, $code: String!, $newPassword: String!) {
+ resetPassword(phone: $phone, email: $email, code: $code, newPassword: $newPassword) {
+ message
+ code
+ }
+}
+`
+
+const UpdateProfileDocument = `
+mutation updateUser($id: String, $input: UpdateUserInput!) {
+ updateUser(id: $id, input: $input) {
+ id
+ arn
+ userPoolId
+ status
+ username
+ email
+ emailVerified
+ phone
+ phoneVerified
+ unionid
+ openid
+ nickname
+ registerSource
+ photo
+ password
+ oauth
+ token
+ tokenExpiredAt
+ loginsCount
+ lastLogin
+ lastIP
+ signedUp
+ blocked
+ isDeleted
+ device
+ browser
+ company
+ name
+ givenName
+ familyName
+ middleName
+ profile
+ preferredUsername
+ website
+ gender
+ birthdate
+ zoneinfo
+ locale
+ address
+ formatted
+ streetAddress
+ locality
+ region
+ postalCode
+ city
+ province
+ country
+ createdAt
+ updatedAt
+ externalId
+ }
+}
+`
+
+const UpdatePasswordDocument = `
+mutation updatePassword($newPassword: String!, $oldPassword: String) {
+ updatePassword(newPassword: $newPassword, oldPassword: $oldPassword) {
+ id
+ arn
+ userPoolId
+ status
+ username
+ email
+ emailVerified
+ phone
+ phoneVerified
+ unionid
+ openid
+ nickname
+ registerSource
+ photo
+ password
+ oauth
+ token
+ tokenExpiredAt
+ loginsCount
+ lastLogin
+ lastIP
+ signedUp
+ blocked
+ isDeleted
+ device
+ browser
+ company
+ name
+ givenName
+ familyName
+ middleName
+ profile
+ preferredUsername
+ website
+ gender
+ birthdate
+ zoneinfo
+ locale
+ address
+ formatted
+ streetAddress
+ locality
+ region
+ postalCode
+ city
+ province
+ country
+ createdAt
+ updatedAt
+ }
+}
+
+`
+const UpdatePhoneDocument = `
+mutation updatePhone($phone: String!, $phoneCode: String!, $oldPhone: String, $oldPhoneCode: String) {
+ updatePhone(phone: $phone, phoneCode: $phoneCode, oldPhone: $oldPhone, oldPhoneCode: $oldPhoneCode) {
+ id
+ arn
+ userPoolId
+ status
+ username
+ email
+ emailVerified
+ phone
+ phoneVerified
+ unionid
+ openid
+ nickname
+ registerSource
+ photo
+ password
+ oauth
+ token
+ tokenExpiredAt
+ loginsCount
+ lastLogin
+ lastIP
+ signedUp
+ blocked
+ isDeleted
+ device
+ browser
+ company
+ name
+ givenName
+ familyName
+ middleName
+ profile
+ preferredUsername
+ website
+ gender
+ birthdate
+ zoneinfo
+ locale
+ address
+ formatted
+ streetAddress
+ locality
+ region
+ postalCode
+ city
+ province
+ country
+ createdAt
+ updatedAt
+ }
+}
+`
+
+const UpdateEmailDocument = `
+mutation updateEmail($email: String!, $emailCode: String!, $oldEmail: String, $oldEmailCode: String) {
+ updateEmail(email: $email, emailCode: $emailCode, oldEmail: $oldEmail, oldEmailCode: $oldEmailCode) {
+ id
+ arn
+ userPoolId
+ status
+ username
+ email
+ emailVerified
+ phone
+ phoneVerified
+ unionid
+ openid
+ nickname
+ registerSource
+ photo
+ password
+ oauth
+ token
+ tokenExpiredAt
+ loginsCount
+ lastLogin
+ lastIP
+ signedUp
+ blocked
+ isDeleted
+ device
+ browser
+ company
+ name
+ givenName
+ familyName
+ middleName
+ profile
+ preferredUsername
+ website
+ gender
+ birthdate
+ zoneinfo
+ locale
+ address
+ formatted
+ streetAddress
+ locality
+ region
+ postalCode
+ city
+ province
+ country
+ createdAt
+ updatedAt
+ }
+}
+
+`
+const BindPhoneDocument = `
+mutation bindPhone($phone: String!, $phoneCode: String!) {
+ bindPhone(phone: $phone, phoneCode: $phoneCode) {
+ id
+ arn
+ userPoolId
+ status
+ username
+ email
+ emailVerified
+ phone
+ phoneVerified
+ unionid
+ openid
+ nickname
+ registerSource
+ photo
+ password
+ oauth
+ token
+ tokenExpiredAt
+ loginsCount
+ lastLogin
+ lastIP
+ signedUp
+ blocked
+ isDeleted
+ device
+ browser
+ company
+ name
+ givenName
+ familyName
+ middleName
+ profile
+ preferredUsername
+ website
+ gender
+ birthdate
+ zoneinfo
+ locale
+ address
+ formatted
+ streetAddress
+ locality
+ region
+ postalCode
+ city
+ province
+ country
+ createdAt
+ updatedAt
+ }
+}
+
+`
+
+const UnBindPhoneDocument = `
+mutation unbindPhone {
+ unbindPhone {
+ id
+ arn
+ userPoolId
+ status
+ username
+ email
+ emailVerified
+ phone
+ phoneVerified
+ unionid
+ openid
+ nickname
+ registerSource
+ photo
+ password
+ oauth
+ token
+ tokenExpiredAt
+ loginsCount
+ lastLogin
+ lastIP
+ signedUp
+ blocked
+ isDeleted
+ device
+ browser
+ company
+ name
+ givenName
+ familyName
+ middleName
+ profile
+ preferredUsername
+ website
+ gender
+ birthdate
+ zoneinfo
+ locale
+ address
+ formatted
+ streetAddress
+ locality
+ region
+ postalCode
+ city
+ province
+ country
+ createdAt
+ updatedAt
+ }
+}
+
+`
+
+const BindEmailDocument = `
+mutation bindEmail($email: String!, $emailCode: String!) {
+ bindEmail(email: $email, emailCode: $emailCode) {
+ id
+ arn
+ userPoolId
+ status
+ username
+ email
+ emailVerified
+ phone
+ phoneVerified
+ unionid
+ openid
+ nickname
+ registerSource
+ photo
+ password
+ oauth
+ token
+ tokenExpiredAt
+ loginsCount
+ lastLogin
+ lastIP
+ signedUp
+ blocked
+ isDeleted
+ device
+ browser
+ company
+ name
+ givenName
+ familyName
+ middleName
+ profile
+ preferredUsername
+ website
+ gender
+ birthdate
+ zoneinfo
+ locale
+ address
+ formatted
+ streetAddress
+ locality
+ region
+ postalCode
+ city
+ province
+ country
+ createdAt
+ updatedAt
+ }
+}`
+
+const UnBindEmailDocument = `
+mutation unbindEmail {
+ unbindEmail {
+ id
+ arn
+ userPoolId
+ status
+ username
+ email
+ emailVerified
+ phone
+ phoneVerified
+ unionid
+ openid
+ nickname
+ registerSource
+ photo
+ password
+ oauth
+ token
+ tokenExpiredAt
+ loginsCount
+ lastLogin
+ lastIP
+ signedUp
+ blocked
+ isDeleted
+ device
+ browser
+ company
+ name
+ givenName
+ familyName
+ middleName
+ profile
+ preferredUsername
+ website
+ gender
+ birthdate
+ zoneinfo
+ locale
+ address
+ formatted
+ streetAddress
+ locality
+ region
+ postalCode
+ city
+ province
+ country
+ createdAt
+ updatedAt
+ }
+}
+`
+const ResetPasswordByTokenDocument = `
+mutation resetPasswordByFirstLoginToken($token: String!, $password: String!) {
+ resetPasswordByFirstLoginToken(token: $token, password: $password) {
+ message
+ code
+ }
+}
+`
+const ResetPasswordByForceResetTokenDocument = `
+mutation resetPasswordByForceResetToken($token: String!, $oldPassword: String!, $newPassword: String!) {
+ resetPasswordByForceResetToken(token: $token, oldPassword: $oldPassword, newPassword: $newPassword) {
+ message
+ code
+ }
+}
+`
+const IsUserExistsDocument = `
+query isUserExists($email: String, $phone: String, $username: String, $externalId: String) {
+ isUserExists(email: $email, phone: $phone, username: $username, externalId: $externalId)
+}
+`
diff --git a/lib/constant/gql_manage_groups.go b/lib/constant/gql_manage_groups.go
new file mode 100644
index 0000000..1be9946
--- /dev/null
+++ b/lib/constant/gql_manage_groups.go
@@ -0,0 +1,223 @@
+package constant
+
+const CreateGroupsDocument = `
+mutation createGroup($code: String!, $name: String!, $description: String) {
+ createGroup(code: $code, name: $name, description: $description) {
+ code
+ name
+ description
+ createdAt
+ updatedAt
+ }
+}
+`
+
+const UpdateGroupsDocument = `
+mutation updateGroup($code: String!, $name: String, $description: String, $newCode: String) {
+ updateGroup(code: $code, name: $name, description: $description, newCode: $newCode) {
+ code
+ name
+ description
+ createdAt
+ updatedAt
+ }
+}
+`
+
+const GroupsDocument = `
+ query groups($userId: String, $page: Int, $limit: Int, $sortBy: SortByEnum) {
+ groups(userId: $userId, page: $page, limit: $limit, sortBy: $sortBy) {
+ totalCount
+ list {
+ code
+ name
+ description
+ createdAt
+ updatedAt
+ }
+ }
+}
+`
+
+const DetailGroupsDocument = `
+query group($code: String!) {
+ group(code: $code) {
+ code
+ name
+ description
+ createdAt
+ updatedAt
+ }
+}
+`
+
+const DeleteGroupsDocument = `
+mutation deleteGroups($codeList: [String!]!) {
+ deleteGroups(codeList: $codeList) {
+ message
+ code
+ }
+}
+`
+
+const ListGroupsDocument = `
+query groups($userId: String, $page: Int, $limit: Int, $sortBy: SortByEnum) {
+ groups(userId: $userId, page: $page, limit: $limit, sortBy: $sortBy) {
+ totalCount
+ list {
+ code
+ name
+ description
+ createdAt
+ updatedAt
+ }
+ }
+}
+`
+
+const ListGroupUserDocument = `
+query groupWithUsers($code: String!, $page: Int, $limit: Int) {
+ group(code: $code) {
+ users(page: $page, limit: $limit) {
+ totalCount
+ list {
+ id
+ arn
+ status
+ userPoolId
+ username
+ email
+ emailVerified
+ phone
+ phoneVerified
+ unionid
+ openid
+ nickname
+ registerSource
+ photo
+ password
+ oauth
+ token
+ tokenExpiredAt
+ loginsCount
+ lastLogin
+ lastIP
+ signedUp
+ blocked
+ isDeleted
+ device
+ browser
+ company
+ name
+ givenName
+ familyName
+ middleName
+ profile
+ preferredUsername
+ website
+ gender
+ birthdate
+ zoneinfo
+ locale
+ address
+ formatted
+ streetAddress
+ locality
+ region
+ postalCode
+ city
+ province
+ country
+ createdAt
+ updatedAt
+ externalId
+ }
+ }
+ }
+}
+
+`
+
+const ListGroupUserWithCustomDocument = `
+query groupWithUsersWithCustomData($code: String!, $page: Int, $limit: Int) {
+ group(code: $code) {
+ users(page: $page, limit: $limit) {
+ totalCount
+ list {
+ id
+ arn
+ status
+ userPoolId
+ username
+ email
+ emailVerified
+ phone
+ phoneVerified
+ unionid
+ openid
+ nickname
+ registerSource
+ photo
+ password
+ oauth
+ token
+ tokenExpiredAt
+ loginsCount
+ lastLogin
+ lastIP
+ signedUp
+ blocked
+ isDeleted
+ device
+ browser
+ company
+ name
+ givenName
+ familyName
+ middleName
+ profile
+ preferredUsername
+ website
+ gender
+ birthdate
+ zoneinfo
+ locale
+ address
+ formatted
+ streetAddress
+ locality
+ region
+ postalCode
+ city
+ province
+ country
+ createdAt
+ updatedAt
+ externalId
+ customData {
+ key
+ value
+ dataType
+ label
+ }
+ }
+ }
+ }
+}
+
+`
+
+const ListGroupAuthorizedResourcesDocument = `
+query listGroupAuthorizedResources($code: String!, $namespace: String, $resourceType: String) {
+ group(code: $code) {
+ authorizedResources(namespace: $namespace, resourceType: $resourceType) {
+ totalCount
+ list {
+ code
+ type
+ actions
+ }
+ }
+ }
+}
+`
diff --git a/lib/constant/gql_manage_org.go b/lib/constant/gql_manage_org.go
new file mode 100644
index 0000000..2ce9038
--- /dev/null
+++ b/lib/constant/gql_manage_org.go
@@ -0,0 +1,480 @@
+package constant
+
+const CreateOrgDocument = `
+mutation createOrg($name: String!, $code: String, $description: String) {
+ createOrg(name: $name, code: $code, description: $description) {
+ id
+ rootNode {
+ id
+ orgId
+ name
+ nameI18n
+ description
+ descriptionI18n
+ order
+ code
+ root
+ depth
+ path
+ createdAt
+ updatedAt
+ children
+ }
+ nodes {
+ id
+ orgId
+ name
+ nameI18n
+ description
+ descriptionI18n
+ order
+ code
+ root
+ depth
+ path
+ createdAt
+ updatedAt
+ children
+ }
+ }
+}
+`
+
+const DeleteOrgDocument = `
+mutation deleteOrg($id: String!) {
+ deleteOrg(id: $id) {
+ message
+ code
+ }
+}
+`
+
+const ListOrgDocument = `
+query orgs($page: Int, $limit: Int, $sortBy: SortByEnum) {
+ orgs(page: $page, limit: $limit, sortBy: $sortBy) {
+ totalCount
+ list {
+ id
+ rootNode {
+ id
+ name
+ nameI18n
+ path
+ description
+ descriptionI18n
+ order
+ code
+ root
+ depth
+ createdAt
+ updatedAt
+ children
+ }
+ nodes {
+ id
+ name
+ path
+ nameI18n
+ description
+ descriptionI18n
+ order
+ code
+ root
+ depth
+ createdAt
+ updatedAt
+ children
+ }
+ }
+ }
+}
+
+`
+
+const AddOrgNodeDocument = `
+mutation addNode($orgId: String!, $parentNodeId: String, $name: String!, $nameI18n: String, $description: String, $descriptionI18n: String, $order: Int, $code: String) {
+ addNode(orgId: $orgId, parentNodeId: $parentNodeId, name: $name, nameI18n: $nameI18n, description: $description, descriptionI18n: $descriptionI18n, order: $order, code: $code) {
+ id
+ rootNode {
+ id
+ orgId
+ name
+ nameI18n
+ description
+ descriptionI18n
+ order
+ code
+ root
+ depth
+ path
+ createdAt
+ updatedAt
+ children
+ }
+ nodes {
+ id
+ orgId
+ name
+ nameI18n
+ description
+ descriptionI18n
+ order
+ code
+ root
+ depth
+ path
+ createdAt
+ updatedAt
+ children
+ }
+ }
+}
+`
+
+const GetOrgNodeDocument = `
+query nodeById($id: String!) {
+ nodeById(id: $id) {
+ id
+ orgId
+ name
+ nameI18n
+ description
+ descriptionI18n
+ order
+ code
+ root
+ depth
+ path
+ createdAt
+ updatedAt
+ children
+ }
+}
+
+`
+
+const UpdateOrgNodeDocument = `
+mutation updateNode($page: Int, $limit: Int, $sortBy: SortByEnum, $includeChildrenNodes: Boolean, $id: String!, $name: String, $code: String, $description: String) {
+ updateNode(id: $id, name: $name, code: $code, description: $description) {
+ id
+ orgId
+ name
+ nameI18n
+ description
+ descriptionI18n
+ order
+ code
+ root
+ depth
+ path
+ createdAt
+ updatedAt
+ children
+ users(page: $page, limit: $limit, sortBy: $sortBy, includeChildrenNodes: $includeChildrenNodes) {
+ totalCount
+ }
+ }
+}
+`
+
+const DeleteOrgNodeDocument = `
+mutation deleteNode($orgId: String!, $nodeId: String!) {
+ deleteNode(orgId: $orgId, nodeId: $nodeId) {
+ message
+ code
+ }
+}
+`
+
+const IsRootNodeDocument = `
+query isRootNode($nodeId: String!, $orgId: String!) {
+ isRootNode(nodeId: $nodeId, orgId: $orgId)
+}
+`
+
+const MoveNodeDocument = `
+
+mutation moveNode($orgId: String!, $nodeId: String!, $targetParentId: String!) {
+ moveNode(orgId: $orgId, nodeId: $nodeId, targetParentId: $targetParentId) {
+ id
+ rootNode {
+ id
+ orgId
+ name
+ nameI18n
+ description
+ descriptionI18n
+ order
+ code
+ root
+ depth
+ path
+ createdAt
+ updatedAt
+ children
+ }
+ nodes {
+ id
+ orgId
+ name
+ nameI18n
+ description
+ descriptionI18n
+ order
+ code
+ root
+ depth
+ path
+ createdAt
+ updatedAt
+ children
+ }
+ }
+}`
+
+const GetRootNodeDocument = `
+query rootNode($orgId: String!) {
+ rootNode(orgId: $orgId) {
+ id
+ orgId
+ name
+ nameI18n
+ description
+ descriptionI18n
+ order
+ code
+ root
+ depth
+ path
+ codePath
+ namePath
+ createdAt
+ updatedAt
+ children
+ }
+}
+
+`
+
+const AddMembersDocument = `
+mutation addMember($page: Int, $limit: Int, $sortBy: SortByEnum, $includeChildrenNodes: Boolean, $nodeId: String, $orgId: String, $nodeCode: String, $userIds: [String!]!, $isLeader: Boolean) {
+ addMember(nodeId: $nodeId, orgId: $orgId, nodeCode: $nodeCode, userIds: $userIds, isLeader: $isLeader) {
+ id
+ orgId
+ name
+ nameI18n
+ description
+ descriptionI18n
+ order
+ code
+ root
+ depth
+ path
+ createdAt
+ updatedAt
+ children
+ users(page: $page, limit: $limit, sortBy: $sortBy, includeChildrenNodes: $includeChildrenNodes) {
+ totalCount
+ list {
+ id
+ arn
+ userPoolId
+ username
+ status
+ email
+ emailVerified
+ phone
+ phoneVerified
+ unionid
+ openid
+ nickname
+ registerSource
+ photo
+ password
+ oauth
+ token
+ tokenExpiredAt
+ loginsCount
+ lastLogin
+ lastIP
+ signedUp
+ blocked
+ isDeleted
+ device
+ browser
+ company
+ name
+ givenName
+ familyName
+ middleName
+ profile
+ preferredUsername
+ website
+ gender
+ birthdate
+ zoneinfo
+ locale
+ address
+ formatted
+ streetAddress
+ locality
+ region
+ postalCode
+ city
+ province
+ country
+ createdAt
+ updatedAt
+ externalId
+ }
+ }
+ }
+}
+
+`
+
+const MoveNodeMembersDocument = `
+mutation moveMembers($userIds: [String!]!, $sourceNodeId: String!, $targetNodeId: String!) {
+ moveMembers(userIds: $userIds, sourceNodeId: $sourceNodeId, targetNodeId: $targetNodeId) {
+ code
+ message
+ }
+}
+
+`
+
+const RemoveNodeMembersDocument = `
+mutation removeMember($page: Int, $limit: Int, $sortBy: SortByEnum, $includeChildrenNodes: Boolean, $nodeId: String, $orgId: String, $nodeCode: String, $userIds: [String!]!) {
+ removeMember(nodeId: $nodeId, orgId: $orgId, nodeCode: $nodeCode, userIds: $userIds) {
+ id
+ name
+ nameI18n
+ description
+ descriptionI18n
+ order
+ code
+ root
+ depth
+ createdAt
+ updatedAt
+ children
+ users(page: $page, limit: $limit, sortBy: $sortBy, includeChildrenNodes: $includeChildrenNodes) {
+ totalCount
+ list {
+ id
+ arn
+ userPoolId
+ status
+ username
+ email
+ emailVerified
+ phone
+ phoneVerified
+ unionid
+ openid
+ nickname
+ registerSource
+ photo
+ password
+ oauth
+ token
+ tokenExpiredAt
+ loginsCount
+ lastLogin
+ lastIP
+ signedUp
+ blocked
+ isDeleted
+ device
+ browser
+ company
+ name
+ givenName
+ familyName
+ middleName
+ profile
+ preferredUsername
+ website
+ gender
+ birthdate
+ zoneinfo
+ locale
+ address
+ formatted
+ streetAddress
+ locality
+ region
+ postalCode
+ city
+ province
+ country
+ createdAt
+ updatedAt
+ }
+ }
+ }
+}
+`
+
+const SetUserMainDepartmentDocument = `
+mutation setMainDepartment($userId: String!, $departmentId: String) {
+ setMainDepartment(userId: $userId, departmentId: $departmentId) {
+ message
+ code
+ }
+}
+`
+
+const ListNodeByIdAuthorizedResourcesDocument = `
+query listNodeByIdAuthorizedResources($id: String!, $namespace: String, $resourceType: String) {
+ nodeById(id: $id) {
+ authorizedResources(namespace: $namespace, resourceType: $resourceType) {
+ totalCount
+ list {
+ code
+ type
+ actions
+ }
+ }
+ }
+}
+
+`
+
+const ListNodeByCodeAuthorizedResourcesDocument = `
+query listNodeByCodeAuthorizedResources($orgId: String!, $code: String!, $namespace: String, $resourceType: String) {
+ nodeByCode(orgId: $orgId, code: $code) {
+ authorizedResources(namespace: $namespace, resourceType: $resourceType) {
+ totalCount
+ list {
+ code
+ type
+ actions
+ }
+ }
+ }
+}
+`
+
+const SearchNodesDocument = `
+query searchNodes($keyword: String!) {
+ searchNodes(keyword: $keyword) {
+ id
+ orgId
+ name
+ nameI18n
+ description
+ descriptionI18n
+ order
+ code
+ root
+ depth
+ path
+ codePath
+ namePath
+ createdAt
+ updatedAt
+ children
+ }
+}
+`
diff --git a/lib/constant/gql_manage_policy.go b/lib/constant/gql_manage_policy.go
new file mode 100644
index 0000000..0cc620e
--- /dev/null
+++ b/lib/constant/gql_manage_policy.go
@@ -0,0 +1,162 @@
+package constant
+
+const CreatePolicyDocument = `
+mutation createPolicy($namespace: String, $code: String!, $description: String, $statements: [PolicyStatementInput!]!) {
+ createPolicy(namespace: $namespace, code: $code, description: $description, statements: $statements) {
+ namespace
+ code
+ isDefault
+ description
+ statements {
+ resource
+ actions
+ effect
+ condition {
+ param
+ operator
+ value
+ }
+ }
+ createdAt
+ updatedAt
+ assignmentsCount
+ }
+}
+
+`
+
+const ListPolicyDocument = `
+query policies($page: Int, $limit: Int, $namespace: String) {
+ policies(page: $page, limit: $limit, namespace: $namespace) {
+ totalCount
+ list {
+ namespace
+ code
+ description
+ createdAt
+ updatedAt
+ statements {
+ resource
+ actions
+ effect
+ condition {
+ param
+ operator
+ value
+ }
+ }
+ }
+ }
+}
+`
+
+const DetailPolicyDocument = `
+query policy($namespace: String, $code: String!) {
+ policy(code: $code, namespace: $namespace) {
+ namespace
+ code
+ isDefault
+ description
+ statements {
+ resource
+ actions
+ effect
+ condition {
+ param
+ operator
+ value
+ }
+ }
+ createdAt
+ updatedAt
+ }
+}
+
+`
+
+const UpdatePolicyDocument = `
+mutation updatePolicy($namespace: String, $code: String!, $description: String, $statements: [PolicyStatementInput!], $newCode: String) {
+ updatePolicy(namespace: $namespace, code: $code, description: $description, statements: $statements, newCode: $newCode) {
+ namespace
+ code
+ description
+ statements {
+ resource
+ actions
+ effect
+ condition {
+ param
+ operator
+ value
+ }
+ }
+ createdAt
+ updatedAt
+ }
+}
+
+`
+
+const DeletePolicyDocument = `
+mutation deletePolicy($code: String!, $namespace: String) {
+ deletePolicy(code: $code, namespace: $namespace) {
+ message
+ code
+ }
+}
+`
+
+const BatchDeletePolicyDocument = `
+mutation deletePolicies($codeList: [String!]!, $namespace: String) {
+ deletePolicies(codeList: $codeList, namespace: $namespace) {
+ message
+ code
+ }
+}
+`
+const PolicyAssignmentsDocument = `
+query policyAssignments($namespace: String, $code: String, $targetType: PolicyAssignmentTargetType, $targetIdentifier: String, $page: Int, $limit: Int) {
+ policyAssignments(namespace: $namespace, code: $code, targetType: $targetType, targetIdentifier: $targetIdentifier, page: $page, limit: $limit) {
+ totalCount
+ list {
+ code
+ targetType
+ targetIdentifier
+ }
+ }
+}
+`
+const AddAssignmentsDocument = `
+mutation addPolicyAssignments($policies: [String!]!, $targetType: PolicyAssignmentTargetType!, $targetIdentifiers: [String!], $inheritByChildren: Boolean, $namespace: String) {
+ addPolicyAssignments(policies: $policies, targetType: $targetType, targetIdentifiers: $targetIdentifiers, inheritByChildren: $inheritByChildren, namespace: $namespace) {
+ message
+ code
+ }
+}
+`
+const RemoveAssignmentsDocument = `
+mutation removePolicyAssignments($policies: [String!]!, $targetType: PolicyAssignmentTargetType!, $targetIdentifiers: [String!], $namespace: String) {
+ removePolicyAssignments(policies: $policies, targetType: $targetType, targetIdentifiers: $targetIdentifiers, namespace: $namespace) {
+ message
+ code
+ }
+}
+`
+
+const EnablePolicyAssignmentDocument = `
+mutation enablePolicyAssignment($policy: String!, $targetType: PolicyAssignmentTargetType!, $targetIdentifier: String!, $namespace: String) {
+ enablePolicyAssignment(policy: $policy, targetType: $targetType, targetIdentifier: $targetIdentifier, namespace: $namespace) {
+ message
+ code
+ }
+}
+`
+const DisablePolicyAssignmentDocument = `
+mutation disbalePolicyAssignment($policy: String!, $targetType: PolicyAssignmentTargetType!, $targetIdentifier: String!, $namespace: String) {
+ disbalePolicyAssignment(policy: $policy, targetType: $targetType, targetIdentifier: $targetIdentifier, namespace: $namespace) {
+ message
+ code
+ }
+}
+
+`
diff --git a/lib/constant/gql_manage_role.go b/lib/constant/gql_manage_role.go
new file mode 100644
index 0000000..c037975
--- /dev/null
+++ b/lib/constant/gql_manage_role.go
@@ -0,0 +1,278 @@
+package constant
+
+const RolesDocument = `
+query roles($namespace: String, $page: Int, $limit: Int, $sortBy: SortByEnum) {
+ roles(namespace: $namespace, page: $page, limit: $limit, sortBy: $sortBy) {
+ totalCount
+ list {
+ id
+ namespace
+ code
+ arn
+ description
+ createdAt
+ updatedAt
+ }
+ }
+}`
+
+const RoleWithUsersDocument = `
+query roleWithUsers($code: String!, $namespace: String, $page: Int, $limit: Int) {
+ role(code: $code, namespace: $namespace) {
+ users(page: $page, limit: $limit) {
+ totalCount
+ list {
+ id
+ arn
+ status
+ userPoolId
+ username
+ email
+ emailVerified
+ phone
+ phoneVerified
+ unionid
+ openid
+ nickname
+ registerSource
+ photo
+ password
+ oauth
+ token
+ tokenExpiredAt
+ loginsCount
+ lastLogin
+ lastIP
+ signedUp
+ blocked
+ isDeleted
+ device
+ browser
+ company
+ name
+ givenName
+ familyName
+ middleName
+ profile
+ preferredUsername
+ website
+ gender
+ birthdate
+ zoneinfo
+ locale
+ address
+ formatted
+ streetAddress
+ locality
+ region
+ postalCode
+ city
+ province
+ country
+ createdAt
+ updatedAt
+ externalId
+ }
+ }
+ }
+}`
+
+const CreateRoleDocument = `
+mutation createRole($namespace: String, $code: String!, $description: String, $parent: String) {
+ createRole(namespace: $namespace, code: $code, description: $description, parent: $parent) {
+ id
+ namespace
+ code
+ arn
+ description
+ createdAt
+ updatedAt
+ parent {
+ namespace
+ code
+ arn
+ description
+ createdAt
+ updatedAt
+ }
+ }
+}
+`
+
+const DeleteRoleDocument = `
+mutation deleteRole($code: String!, $namespace: String) {
+ deleteRole(code: $code, namespace: $namespace) {
+ message
+ code
+ }
+}
+`
+
+const BatchDeleteRoleDocument = `
+mutation deleteRoles($codeList: [String!]!, $namespace: String) {
+ deleteRoles(codeList: $codeList, namespace: $namespace) {
+ message
+ code
+ }
+}
+`
+
+const UpdateRoleDocument = `
+mutation updateRole($code: String!, $description: String, $newCode: String, $namespace: String) {
+ updateRole(code: $code, description: $description, newCode: $newCode, namespace: $namespace) {
+ id
+ namespace
+ code
+ arn
+ description
+ createdAt
+ updatedAt
+ parent {
+ namespace
+ code
+ arn
+ description
+ createdAt
+ updatedAt
+ }
+ }
+}
+`
+
+const RoleDetailDocument = `
+query role($code: String!, $namespace: String) {
+ role(code: $code, namespace: $namespace) {
+ id
+ namespace
+ code
+ arn
+ description
+ createdAt
+ updatedAt
+ parent {
+ namespace
+ code
+ arn
+ description
+ createdAt
+ updatedAt
+ }
+ }
+}`
+
+const AssignRoleDocument = `
+mutation assignRole($namespace: String, $roleCode: String, $roleCodes: [String], $userIds: [String!], $groupCodes: [String!], $nodeCodes: [String!]) {
+ assignRole(namespace: $namespace, roleCode: $roleCode, roleCodes: $roleCodes, userIds: $userIds, groupCodes: $groupCodes, nodeCodes: $nodeCodes) {
+ message
+ code
+ }
+}
+`
+
+const RevokeRoleDocument = `
+mutation revokeRole($namespace: String, $roleCode: String, $roleCodes: [String], $userIds: [String!], $groupCodes: [String!], $nodeCodes: [String!]) {
+ revokeRole(namespace: $namespace, roleCode: $roleCode, roleCodes: $roleCodes, userIds: $userIds, groupCodes: $groupCodes, nodeCodes: $nodeCodes) {
+ message
+ code
+ }
+}
+`
+
+const ListPoliciesDocument = `
+query policyAssignments($namespace: String, $code: String, $targetType: PolicyAssignmentTargetType, $targetIdentifier: String, $page: Int, $limit: Int) {
+ policyAssignments(namespace: $namespace, code: $code, targetType: $targetType, targetIdentifier: $targetIdentifier, page: $page, limit: $limit) {
+ totalCount
+ list {
+ code
+ targetType
+ targetIdentifier
+ }
+ }
+}
+`
+
+const AddPoliciesDocument = `
+mutation addPolicyAssignments($policies: [String!]!, $targetType: PolicyAssignmentTargetType!, $targetIdentifiers: [String!], $inheritByChildren: Boolean, $namespace: String) {
+ addPolicyAssignments(policies: $policies, targetType: $targetType, targetIdentifiers: $targetIdentifiers, inheritByChildren: $inheritByChildren, namespace: $namespace) {
+ message
+ code
+ }
+}
+`
+const RemovePoliciesDocument = `
+mutation removePolicyAssignments($policies: [String!]!, $targetType: PolicyAssignmentTargetType!, $targetIdentifiers: [String!], $namespace: String) {
+ removePolicyAssignments(policies: $policies, targetType: $targetType, targetIdentifiers: $targetIdentifiers, namespace: $namespace) {
+ message
+ code
+ }
+}
+`
+
+const ListRoleAuthorizedResourcesDocument = `
+query listRoleAuthorizedResources($code: String!, $namespace: String, $resourceType: String) {
+ role(code: $code, namespace: $namespace) {
+ authorizedResources(resourceType: $resourceType) {
+ totalCount
+ list {
+ code
+ type
+ actions
+ }
+ }
+ }
+}
+`
+const GetRoleUdfValueDocument = `
+query udv($targetType: UDFTargetType!, $targetId: String!) {
+ udv(targetType: $targetType, targetId: $targetId) {
+ key
+ dataType
+ value
+ label
+ }
+}
+`
+
+const BatchGetRoleUdfValueDocument = `
+query udfValueBatch($targetType: UDFTargetType!, $targetIds: [String!]!) {
+ udfValueBatch(targetType: $targetType, targetIds: $targetIds) {
+ targetId
+ data {
+ key
+ dataType
+ value
+ label
+ }
+ }
+}
+`
+
+const SetRoleUdfValueDocument = `
+mutation setUdvBatch($targetType: UDFTargetType!, $targetId: String!, $udvList: [UserDefinedDataInput!]) {
+ setUdvBatch(targetType: $targetType, targetId: $targetId, udvList: $udvList) {
+ key
+ dataType
+ value
+ label
+ }
+}
+`
+
+const BatchSetUdfValueDocument = `
+mutation setUdfValueBatch($targetType: UDFTargetType!, $input: [SetUdfValueBatchInput!]!) {
+ setUdfValueBatch(targetType: $targetType, input: $input) {
+ code
+ message
+ }
+}
+`
+const RemoveUdfValueDocument = `
+mutation removeUdv($targetType: UDFTargetType!, $targetId: String!, $key: String!) {
+ removeUdv(targetType: $targetType, targetId: $targetId, key: $key) {
+ key
+ dataType
+ value
+ label
+ }
+}
+`
diff --git a/lib/constant/gql_manage_user.go b/lib/constant/gql_manage_user.go
new file mode 100644
index 0000000..f4ca58e
--- /dev/null
+++ b/lib/constant/gql_manage_user.go
@@ -0,0 +1,763 @@
+package constant
+
+const CreateUserDocument = `
+mutation createUser($userInfo: CreateUserInput!, $params: String, $identity: CreateUserIdentityInput, $keepPassword: Boolean, $resetPasswordOnFirstLogin: Boolean) {
+ createUser(userInfo: $userInfo, params: $params, identity: $identity, keepPassword: $keepPassword, resetPasswordOnFirstLogin: $resetPasswordOnFirstLogin) {
+ id
+ arn
+ userPoolId
+ status
+ username
+ email
+ emailVerified
+ phone
+ phoneVerified
+ unionid
+ openid
+ nickname
+ registerSource
+ photo
+ password
+ oauth
+ token
+ tokenExpiredAt
+ loginsCount
+ lastLogin
+ lastIP
+ signedUp
+ blocked
+ isDeleted
+ device
+ browser
+ company
+ name
+ givenName
+ familyName
+ middleName
+ profile
+ preferredUsername
+ website
+ gender
+ birthdate
+ zoneinfo
+ locale
+ address
+ formatted
+ streetAddress
+ locality
+ region
+ postalCode
+ city
+ province
+ country
+ createdAt
+ updatedAt
+ externalId
+ }
+}
+`
+const CreateUserWithCustomDataDocument = `
+mutation createUserWithCustomData($userInfo: CreateUserInput!, $keepPassword: Boolean, $params: String) {
+ createUser(userInfo: $userInfo, keepPassword: $keepPassword, params: $params) {
+ id
+ arn
+ userPoolId
+ status
+ username
+ email
+ emailVerified
+ phone
+ phoneVerified
+ unionid
+ openid
+ nickname
+ registerSource
+ photo
+ password
+ oauth
+ token
+ tokenExpiredAt
+ loginsCount
+ lastLogin
+ lastIP
+ signedUp
+ blocked
+ isDeleted
+ device
+ browser
+ company
+ name
+ givenName
+ familyName
+ middleName
+ profile
+ preferredUsername
+ website
+ gender
+ birthdate
+ zoneinfo
+ locale
+ address
+ formatted
+ streetAddress
+ locality
+ region
+ postalCode
+ city
+ province
+ country
+ createdAt
+ updatedAt
+ externalId
+ customData {
+ key
+ value
+ dataType
+ label
+ }
+ }
+}
+`
+
+const UpdateUserDocument = `
+mutation updateUser($id: String, $input: UpdateUserInput!) {
+ updateUser(id: $id, input: $input) {
+ id
+ arn
+ userPoolId
+ status
+ username
+ email
+ emailVerified
+ phone
+ phoneVerified
+ unionid
+ openid
+ nickname
+ registerSource
+ photo
+ password
+ oauth
+ token
+ tokenExpiredAt
+ loginsCount
+ lastLogin
+ lastIP
+ signedUp
+ blocked
+ isDeleted
+ device
+ browser
+ company
+ name
+ givenName
+ familyName
+ middleName
+ profile
+ preferredUsername
+ website
+ gender
+ birthdate
+ zoneinfo
+ locale
+ address
+ formatted
+ streetAddress
+ locality
+ region
+ postalCode
+ city
+ province
+ country
+ createdAt
+ updatedAt
+ externalId
+ }
+}
+`
+
+const DeleteUserDocument = `
+mutation deleteUser($id: String!) {
+ deleteUser(id: $id) {
+ message
+ code
+ }
+}
+`
+
+const BatchDeleteUserDocument = `
+mutation deleteUsers($ids: [String!]!) {
+ deleteUsers(ids: $ids) {
+ message
+ code
+ }
+}
+`
+
+const BatchGetUserDocument = `
+query userBatch($ids: [String!]!, $type: String) {
+ userBatch(ids: $ids, type: $type) {
+ identities {
+ openid
+ userIdInIdp
+ userId
+ extIdpId
+ isSocial
+ provider
+ type
+ userPoolId
+ }
+ id
+ arn
+ userPoolId
+ status
+ username
+ email
+ emailVerified
+ phone
+ phoneVerified
+ unionid
+ openid
+ nickname
+ registerSource
+ photo
+ password
+ oauth
+ token
+ tokenExpiredAt
+ loginsCount
+ lastLogin
+ lastIP
+ signedUp
+ blocked
+ isDeleted
+ device
+ browser
+ company
+ name
+ givenName
+ familyName
+ middleName
+ profile
+ preferredUsername
+ website
+ gender
+ birthdate
+ zoneinfo
+ locale
+ address
+ formatted
+ streetAddress
+ locality
+ region
+ postalCode
+ city
+ province
+ country
+ createdAt
+ updatedAt
+ externalId
+ }
+}
+`
+
+const BatchGetUserWithCustomDocument = `
+query userBatchWithCustomData($ids: [String!]!, $type: String) {
+ userBatch(ids: $ids, type: $type) {
+ identities {
+ openid
+ userIdInIdp
+ userId
+ extIdpId
+ isSocial
+ provider
+ type
+ userPoolId
+ }
+ id
+ arn
+ userPoolId
+ status
+ username
+ email
+ emailVerified
+ phone
+ phoneVerified
+ unionid
+ openid
+ nickname
+ registerSource
+ photo
+ password
+ oauth
+ token
+ tokenExpiredAt
+ loginsCount
+ lastLogin
+ lastIP
+ signedUp
+ blocked
+ isDeleted
+ device
+ browser
+ company
+ name
+ givenName
+ familyName
+ middleName
+ profile
+ preferredUsername
+ website
+ gender
+ birthdate
+ zoneinfo
+ locale
+ address
+ formatted
+ streetAddress
+ locality
+ region
+ postalCode
+ city
+ province
+ country
+ createdAt
+ updatedAt
+ externalId
+ customData {
+ key
+ value
+ dataType
+ label
+ }
+ }
+}
+
+`
+
+const ListArchivedUsersDocument = `
+query archivedUsers($page: Int, $limit: Int) {
+ archivedUsers(page: $page, limit: $limit) {
+ totalCount
+ list {
+ id
+ arn
+ status
+ userPoolId
+ username
+ email
+ emailVerified
+ phone
+ phoneVerified
+ unionid
+ openid
+ nickname
+ registerSource
+ photo
+ password
+ oauth
+ token
+ tokenExpiredAt
+ loginsCount
+ lastLogin
+ lastIP
+ signedUp
+ blocked
+ isDeleted
+ device
+ browser
+ company
+ name
+ givenName
+ familyName
+ middleName
+ profile
+ preferredUsername
+ website
+ gender
+ birthdate
+ zoneinfo
+ locale
+ address
+ formatted
+ streetAddress
+ locality
+ region
+ postalCode
+ city
+ province
+ country
+ createdAt
+ updatedAt
+ externalId
+ }
+ }
+}
+`
+const FindUserDocument = `
+query findUser($email: String, $phone: String, $username: String, $externalId: String, $identity: FindUserByIdentityInput) {
+ findUser(email: $email, phone: $phone, username: $username, externalId: $externalId, identity: $identity) {
+ id
+ arn
+ userPoolId
+ status
+ username
+ email
+ emailVerified
+ phone
+ phoneVerified
+ unionid
+ openid
+ nickname
+ registerSource
+ photo
+ password
+ oauth
+ token
+ tokenExpiredAt
+ loginsCount
+ lastLogin
+ lastIP
+ signedUp
+ blocked
+ isDeleted
+ device
+ browser
+ company
+ name
+ givenName
+ familyName
+ middleName
+ profile
+ preferredUsername
+ website
+ gender
+ birthdate
+ zoneinfo
+ locale
+ address
+ formatted
+ streetAddress
+ locality
+ region
+ postalCode
+ city
+ province
+ country
+ createdAt
+ updatedAt
+ externalId
+ }
+}
+`
+
+const FindUserWithCustomDocument = `
+query findUserWithCustomData($email: String, $phone: String, $username: String, $externalId: String) {
+ findUser(email: $email, phone: $phone, username: $username, externalId: $externalId) {
+ id
+ arn
+ userPoolId
+ status
+ username
+ email
+ emailVerified
+ phone
+ phoneVerified
+ unionid
+ openid
+ nickname
+ registerSource
+ photo
+ password
+ oauth
+ token
+ tokenExpiredAt
+ loginsCount
+ lastLogin
+ lastIP
+ signedUp
+ blocked
+ isDeleted
+ device
+ browser
+ company
+ name
+ givenName
+ familyName
+ middleName
+ profile
+ preferredUsername
+ website
+ gender
+ birthdate
+ zoneinfo
+ locale
+ address
+ formatted
+ streetAddress
+ locality
+ region
+ postalCode
+ city
+ province
+ country
+ createdAt
+ updatedAt
+ externalId
+ customData {
+ key
+ value
+ dataType
+ label
+ }
+ }
+}
+
+`
+
+const SearchUserDocument = `
+query searchUser($query: String!, $fields: [String], $page: Int, $limit: Int, $departmentOpts: [SearchUserDepartmentOpt], $groupOpts: [SearchUserGroupOpt], $roleOpts: [SearchUserRoleOpt]) {
+ searchUser(query: $query, fields: $fields, page: $page, limit: $limit, departmentOpts: $departmentOpts, groupOpts: $groupOpts, roleOpts: $roleOpts) {
+ totalCount
+ list {
+ id
+ arn
+ userPoolId
+ status
+ username
+ email
+ emailVerified
+ phone
+ phoneVerified
+ unionid
+ openid
+ nickname
+ registerSource
+ photo
+ password
+ oauth
+ token
+ tokenExpiredAt
+ loginsCount
+ lastLogin
+ lastIP
+ signedUp
+ blocked
+ isDeleted
+ device
+ browser
+ company
+ name
+ givenName
+ familyName
+ middleName
+ profile
+ preferredUsername
+ website
+ gender
+ birthdate
+ zoneinfo
+ locale
+ address
+ formatted
+ streetAddress
+ locality
+ region
+ postalCode
+ city
+ province
+ country
+ createdAt
+ updatedAt
+ externalId
+ }
+ }
+}
+`
+
+const SearchUserWithCustomDocument = `
+query searchUserWithCustomData($query: String!, $fields: [String], $page: Int, $limit: Int, $departmentOpts: [SearchUserDepartmentOpt], $groupOpts: [SearchUserGroupOpt], $roleOpts: [SearchUserRoleOpt]) {
+ searchUser(query: $query, fields: $fields, page: $page, limit: $limit, departmentOpts: $departmentOpts, groupOpts: $groupOpts, roleOpts: $roleOpts) {
+ totalCount
+ list {
+ id
+ arn
+ userPoolId
+ status
+ username
+ email
+ emailVerified
+ phone
+ phoneVerified
+ unionid
+ openid
+ nickname
+ registerSource
+ photo
+ password
+ oauth
+ token
+ tokenExpiredAt
+ loginsCount
+ lastLogin
+ lastIP
+ signedUp
+ blocked
+ isDeleted
+ device
+ browser
+ company
+ name
+ givenName
+ familyName
+ middleName
+ profile
+ preferredUsername
+ website
+ gender
+ birthdate
+ zoneinfo
+ locale
+ address
+ formatted
+ streetAddress
+ locality
+ region
+ postalCode
+ city
+ province
+ country
+ createdAt
+ updatedAt
+ externalId
+ customData {
+ key
+ value
+ dataType
+ label
+ }
+ }
+ }
+}
+`
+
+const RefreshUserTokenDocument = `
+mutation refreshToken($id: String) {
+ refreshToken(id: $id) {
+ token
+ iat
+ exp
+ }
+}
+`
+
+const GetUserGroupsDocument = `
+query getUserGroups($id: String!) {
+ user(id: $id) {
+ groups {
+ totalCount
+ list {
+ code
+ name
+ description
+ createdAt
+ updatedAt
+ }
+ }
+ }
+}
+`
+const AddUserToGroupDocument = `
+mutation addUserToGroup($userIds: [String!]!, $code: String) {
+ addUserToGroup(userIds: $userIds, code: $code) {
+ message
+ code
+ }
+}
+`
+
+const RemoveUserInGroupDocument = `
+mutation removeUserFromGroup($userIds: [String!]!, $code: String) {
+ removeUserFromGroup(userIds: $userIds, code: $code) {
+ message
+ code
+ }
+}`
+
+const GetUserRolesDocument = `
+query getUserRoles($id: String!, $namespace: String) {
+ user(id: $id) {
+ roles(namespace: $namespace) {
+ totalCount
+ list {
+ id
+ code
+ namespace
+ arn
+ description
+ createdAt
+ updatedAt
+ parent {
+ code
+ namespace
+ arn
+ description
+ createdAt
+ updatedAt
+ }
+ }
+ }
+ }
+}
+`
+const AddUserToRoleDocument = `
+mutation assignRole($namespace: String, $roleCode: String, $roleCodes: [String], $userIds: [String!], $groupCodes: [String!], $nodeCodes: [String!]) {
+ assignRole(namespace: $namespace, roleCode: $roleCode, roleCodes: $roleCodes, userIds: $userIds, groupCodes: $groupCodes, nodeCodes: $nodeCodes) {
+ message
+ code
+ }
+}
+`
+
+const RemoveUserInRoleDocument = `
+mutation revokeRole($namespace: String, $roleCode: String, $roleCodes: [String], $userIds: [String!], $groupCodes: [String!], $nodeCodes: [String!]) {
+ revokeRole(namespace: $namespace, roleCode: $roleCode, roleCodes: $roleCodes, userIds: $userIds, groupCodes: $groupCodes, nodeCodes: $nodeCodes) {
+ message
+ code
+ }
+}
+`
+
+const ListUserAuthorizedResourcesDocument = `
+query listUserAuthorizedResources($id: String!, $namespace: String, $resourceType: String) {
+ user(id: $id) {
+ authorizedResources(namespace: $namespace, resourceType: $resourceType) {
+ totalCount
+ list {
+ code
+ type
+ actions
+ }
+ }
+ }
+}
+`
+
+const SetUdvDocument = `
+mutation setUdv($targetType: UDFTargetType!, $targetId: String!, $key: String!, $value: String!) {
+ setUdv(targetType: $targetType, targetId: $targetId, key: $key, value: $value) {
+ key
+ dataType
+ value
+ label
+ }
+}
+`
+const SendFirstLoginVerifyEmailDocument = `
+mutation sendFirstLoginVerifyEmail($userId: String!, $appId: String!) {
+ sendFirstLoginVerifyEmail(userId: $userId, appId: $appId) {
+ message
+ code
+ }
+}
+`
diff --git a/lib/enum/Interface_enum.go b/lib/enum/Interface_enum.go
new file mode 100644
index 0000000..226077d
--- /dev/null
+++ b/lib/enum/Interface_enum.go
@@ -0,0 +1,10 @@
+package enum
+
+type SortByEnum string
+
+const (
+ SortByCreatedAtDesc SortByEnum = "CREATEDAT_DESC"
+ SortByCreatedAtAsc SortByEnum = "CREATEDAT_ASC"
+ SortByUpdatedAtDesc SortByEnum = "UPDATEDAT_DESC"
+ SortByUpdatedAtAsc SortByEnum = "UPDATEDAT_ASC"
+)
diff --git a/lib/management/acl_management_client.go b/lib/management/acl_management_client.go
new file mode 100644
index 0000000..3e38df9
--- /dev/null
+++ b/lib/management/acl_management_client.go
@@ -0,0 +1,665 @@
+package management
+
+import (
+ "encoding/json"
+ "errors"
+ "fmt"
+ "github.com/Authing/authing-go-sdk/lib/constant"
+ "github.com/Authing/authing-go-sdk/lib/model"
+ "github.com/Authing/authing-go-sdk/lib/util"
+ "github.com/bitly/go-simplejson"
+ jsoniter "github.com/json-iterator/go"
+ "net/http"
+)
+
+//IsAllowed
+//判断某个用户是否对某个资源有某个操作权限
+func (c *Client) IsAllowed(request model.IsAllowedRequest) (bool, error) {
+ data, _ := json.Marshal(&request)
+ variables := make(map[string]interface{})
+ json.Unmarshal(data, &variables)
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, constant.HttpMethodPost, constant.IsActionAllowedDocument, variables)
+ if err != nil {
+ return false, err
+ }
+ resultJson, err := simplejson.NewJson(b)
+ result, err := resultJson.Get("data").Get("isActionAllowed").Bool()
+ if err != nil {
+ return false, err
+ }
+ return result, nil
+}
+
+//Allow
+//允许某个用户对某个资源进行某个操作
+func (c *Client) Allow(request model.AllowRequest) (bool, error) {
+ data, _ := json.Marshal(&request)
+ variables := make(map[string]interface{})
+ json.Unmarshal(data, &variables)
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, constant.HttpMethodPost, constant.AllowDocument, variables)
+ if err != nil {
+ return false, err
+ }
+ resultJson, err := simplejson.NewJson(b)
+ result, err := resultJson.Get("data").Get("allow").Get("code").Int64()
+ if err != nil {
+ return false, err
+ }
+ return result == 200, nil
+
+}
+
+//AuthorizeResource
+//将一个(类)资源授权给用户、角色、分组、组织机构,且可以分别指定不同的操作权限。
+func (c *Client) AuthorizeResource(request model.AuthorizeResourceRequest) (bool, error) {
+ data, _ := json.Marshal(&request)
+ variables := make(map[string]interface{})
+ json.Unmarshal(data, &variables)
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, constant.HttpMethodPost, constant.AuthorizeResourceDocument, variables)
+ if err != nil {
+ return false, err
+ }
+ resultJson, err := simplejson.NewJson(b)
+ result, err := resultJson.Get("data").Get("authorizeResource").Get("code").Int64()
+ if err != nil {
+ return false, err
+ }
+ return result == 200, nil
+}
+
+//RevokeResource
+//批量撤销资源的授权
+func (c *Client) RevokeResource(request model.RevokeResourceRequest) (bool, error) {
+ data, _ := json.Marshal(&request)
+ variables := make(map[string]interface{})
+ json.Unmarshal(data, &variables)
+ b, err := c.SendHttpRequest(c.Host+"/api/v2/acl/revoke-resource", constant.HttpMethodPost, constant.StringEmpty, variables)
+ resultJson, err := simplejson.NewJson(b)
+ result, err := resultJson.Get("code").Int64()
+ if err != nil {
+ return false, err
+ }
+ return result == 200, nil
+}
+
+// ListAuthorizedResourcesForCustom
+// 获取某个主体(用户、角色、分组、组织机构节点)被授权的所有资源。
+func (c *Client) ListAuthorizedResourcesForCustom(request model.ListAuthorizedResourcesRequest) (*struct {
+ TotalCount int64 `json:"totalCount"`
+ List []model.AuthorizedResource `json:"list"`
+}, error) {
+
+ data, _ := json.Marshal(&request)
+ variables := make(map[string]interface{})
+ json.Unmarshal(data, &variables)
+
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.ListAuthorizedResourcesDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ AuthorizedResources struct {
+ TotalCount int64 `json:"totalCount"`
+ List []model.AuthorizedResource `json:"list"`
+ } `json:"authorizedResources"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.AuthorizedResources, nil
+}
+
+// ProgrammaticAccessAccountList
+// 编程访问账号列表
+func (c *Client) ProgrammaticAccessAccountList(appId string, page, limit int) (*struct {
+ TotalCount int64 `json:"totalCount"`
+ List []model.ProgrammaticAccessAccount `json:"list"`
+}, error) {
+
+ url := fmt.Sprintf("%s/api/v2/applications/%s/programmatic-access-accounts?limit=%v&page=%v", c.Host, appId, limit, page)
+ b, err := c.SendHttpRestRequest(url, http.MethodGet, nil)
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data struct {
+ TotalCount int64 `json:"totalCount"`
+ List []model.ProgrammaticAccessAccount `json:"list"`
+ } `json:"data"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return &resp.Data, nil
+}
+
+// CreateProgrammaticAccessAccount
+// 添加编程访问账号
+func (c *Client) CreateProgrammaticAccessAccount(appId string, remark *string, tokenLifetime *int) (*model.ProgrammaticAccessAccount, error) {
+
+ vars := make(map[string]interface{})
+ if tokenLifetime == nil {
+ vars["tokenLifetime"] = 600
+ } else {
+ vars["tokenLifetime"] = tokenLifetime
+ }
+ if remark != nil {
+ vars["remark"] = remark
+ }
+ url := fmt.Sprintf("%s/api/v2/applications/%s/programmatic-access-accounts", c.Host, appId)
+ b, err := c.SendHttpRestRequest(url, http.MethodPost, vars)
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data model.ProgrammaticAccessAccount `json:"data"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return &resp.Data, nil
+}
+
+// DisableProgrammaticAccessAccount
+// 禁用编程访问账号
+func (c *Client) DisableProgrammaticAccessAccount(programmaticAccessAccountId string) (*model.ProgrammaticAccessAccount, error) {
+
+ url := fmt.Sprintf("%s/api/v2/applications/programmatic-access-accounts", c.Host)
+ b, err := c.SendHttpRestRequest(url, http.MethodPatch, map[string]interface{}{
+ "id": programmaticAccessAccountId,
+ "enabled": false,
+ })
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data model.ProgrammaticAccessAccount `json:"data"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return &resp.Data, nil
+}
+
+// EnableProgrammaticAccessAccount
+// 启用编程访问账号
+func (c *Client) EnableProgrammaticAccessAccount(programmaticAccessAccountId string) (*model.ProgrammaticAccessAccount, error) {
+
+ url := fmt.Sprintf("%s/api/v2/applications/programmatic-access-accounts", c.Host)
+ b, err := c.SendHttpRestRequest(url, http.MethodPatch, map[string]interface{}{
+ "id": programmaticAccessAccountId,
+ "enabled": true,
+ })
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data model.ProgrammaticAccessAccount `json:"data"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return &resp.Data, nil
+}
+
+// RefreshProgrammaticAccessAccountSecret
+// 刷新编程访问账号密钥
+func (c *Client) RefreshProgrammaticAccessAccountSecret(programmaticAccessAccountId string, secret *string) (*model.ProgrammaticAccessAccount, error) {
+
+ vars := map[string]interface{}{
+ "id": programmaticAccessAccountId,
+ }
+ if secret == nil {
+ vars["secret"] = util.RandomString(32)
+ } else {
+ vars["secret"] = secret
+ }
+ url := fmt.Sprintf("%s/api/v2/applications/programmatic-access-accounts", c.Host)
+ b, err := c.SendHttpRestRequest(url, http.MethodPatch, vars)
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data model.ProgrammaticAccessAccount `json:"data"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return &resp.Data, nil
+}
+
+// DeleteProgrammaticAccessAccount
+// 删除编程访问账号
+func (c *Client) DeleteProgrammaticAccessAccount(programmaticAccessAccountId string) (*string, error) {
+
+ url := fmt.Sprintf("%s/api/v2/applications/programmatic-access-accounts?id=%s", c.Host, programmaticAccessAccountId)
+ b, err := c.SendHttpRestRequest(url, http.MethodDelete, nil)
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return &resp.Message, nil
+}
+
+// ListNamespaceResources
+// 获取资源列表
+func (c *Client) ListNamespaceResources(req model.ListResourceRequest) (*model.ListNamespaceResourceResponse, error) {
+ data, _ := json.Marshal(&req)
+ variables := make(map[string]interface{})
+ json.Unmarshal(data, &variables)
+
+ url := fmt.Sprintf("%s/api/v2/resources", c.Host)
+ b, err := c.SendHttpRestRequest(url, http.MethodGet, variables)
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data model.ListNamespaceResourceResponse `json:"data"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return &resp.Data, nil
+}
+
+// GetResourceById
+// 根据 ID 获取单个资源
+func (c *Client) GetResourceById(id string) (*model.ResourceResponse, error) {
+ url := fmt.Sprintf("%s/api/v2/resources/detail", c.Host)
+ b, err := c.SendHttpRestRequest(url, http.MethodGet, map[string]interface{}{"id": id})
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data model.ResourceResponse `json:"data"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return &resp.Data, nil
+}
+
+// GetResourceByCode
+// 根据 Code 获取单个资源
+func (c *Client) GetResourceByCode(code, namespace string) (*model.ResourceResponse, error) {
+ url := fmt.Sprintf("%s/api/v2/resources/detail", c.Host)
+ b, err := c.SendHttpRestRequest(url, http.MethodGet, map[string]interface{}{
+ "code": code,
+ "namespace": namespace,
+ })
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data model.ResourceResponse `json:"data"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return &resp.Data, nil
+}
+
+// CreateResource
+// 创建资源
+func (c *Client) CreateResource(req *model.CreateResourceRequest) (*model.ResourceResponse, error) {
+ data, _ := json.Marshal(&req)
+ variables := make(map[string]interface{})
+ json.Unmarshal(data, &variables)
+
+ url := fmt.Sprintf("%s/api/v2/resources", c.Host)
+ b, err := c.SendHttpRestRequest(url, http.MethodPost, variables)
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data model.ResourceResponse `json:"data"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return &resp.Data, nil
+}
+
+// UpdateResource
+// 更新资源
+func (c *Client) UpdateResource(code string, req *model.UpdateResourceRequest) (*model.ResourceResponse, error) {
+ data, _ := json.Marshal(&req)
+ variables := make(map[string]interface{})
+ json.Unmarshal(data, &variables)
+ url := fmt.Sprintf("%s/api/v2/resources/%s", c.Host, code)
+ b, err := c.SendHttpRestRequest(url, http.MethodPost, variables)
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data model.ResourceResponse `json:"data"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return &resp.Data, nil
+}
+
+// DeleteResource
+// 删除资源
+func (c *Client) DeleteResource(code, namespace string) (*string, error) {
+
+ url := fmt.Sprintf("%s/api/v2/resources/%s", c.Host, code)
+ b, err := c.SendHttpRestRequest(url, http.MethodDelete, map[string]interface{}{"namespace": namespace})
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return &resp.Message, nil
+}
+
+// GetApplicationAccessPolicies
+// 获取应用访问控制策略列表
+func (c *Client) GetApplicationAccessPolicies(appId string, page, limit int) (*model.GetApplicationAccessPoliciesResponse, error) {
+
+ url := fmt.Sprintf("%s/api/v2/applications/%s/authorization/records", c.Host, appId)
+ b, err := c.SendHttpRestRequest(url, http.MethodGet, map[string]interface{}{
+ "page": page,
+ "limit": limit,
+ })
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data model.GetApplicationAccessPoliciesResponse `json:"data"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return &resp.Data, nil
+}
+
+// EnableApplicationAccessPolicies
+// 启用应用访问控制策略
+func (c *Client) EnableApplicationAccessPolicies(appId string, req *model.ApplicationAccessPoliciesRequest) (*string, error) {
+ data, _ := json.Marshal(&req)
+ variables := make(map[string]interface{})
+ json.Unmarshal(data, &variables)
+ url := fmt.Sprintf("%s/api/v2/applications/%s/authorization/enable-effect", c.Host, appId)
+ b, err := c.SendHttpRestRequest(url, http.MethodPost, variables)
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return &resp.Message, nil
+}
+
+// DisableApplicationAccessPolicies
+// 停用应用访问控制策略
+func (c *Client) DisableApplicationAccessPolicies(appId string, req *model.ApplicationAccessPoliciesRequest) (*string, error) {
+ data, _ := json.Marshal(&req)
+ variables := make(map[string]interface{})
+ json.Unmarshal(data, &variables)
+ url := fmt.Sprintf("%s/api/v2/applications/%s/authorization/disable-effect", c.Host, appId)
+ b, err := c.SendHttpRestRequest(url, http.MethodPost, variables)
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return &resp.Message, nil
+}
+
+// DeleteApplicationAccessPolicies
+// 删除应用访问控制策略
+func (c *Client) DeleteApplicationAccessPolicies(appId string, req *model.ApplicationAccessPoliciesRequest) (*string, error) {
+ data, _ := json.Marshal(&req)
+ variables := make(map[string]interface{})
+ json.Unmarshal(data, &variables)
+ url := fmt.Sprintf("%s/api/v2/applications/%s/authorization/revoke", c.Host, appId)
+ b, err := c.SendHttpRestRequest(url, http.MethodPost, variables)
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return &resp.Message, nil
+}
+
+// AllowApplicationAccessPolicies
+// 配置「允许主体(用户、角色、分组、组织机构节点)访问应用」的控制策略
+func (c *Client) AllowApplicationAccessPolicies(appId string, req *model.ApplicationAccessPoliciesRequest) (*string, error) {
+ data, _ := json.Marshal(&req)
+ variables := make(map[string]interface{})
+ json.Unmarshal(data, &variables)
+ url := fmt.Sprintf("%s/api/v2/applications/%s/authorization/allow", c.Host, appId)
+ b, err := c.SendHttpRestRequest(url, http.MethodPost, variables)
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return &resp.Message, nil
+}
+
+// DenyApplicationAccessPolicies
+// 配置「拒绝主体(用户、角色、分组、组织机构节点)访问应用」的控制策略
+func (c *Client) DenyApplicationAccessPolicies(appId string, req *model.ApplicationAccessPoliciesRequest) (*string, error) {
+ data, _ := json.Marshal(&req)
+ variables := make(map[string]interface{})
+ json.Unmarshal(data, &variables)
+ url := fmt.Sprintf("%s/api/v2/applications/%s/authorization/deny", c.Host, appId)
+ b, err := c.SendHttpRestRequest(url, http.MethodPost, variables)
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return &resp.Message, nil
+}
+
+// UpdateDefaultApplicationAccessPolicy
+// 更改默认应用访问策略(默认拒绝所有用户访问应用、默认允许所有用户访问应用)
+func (c *Client) UpdateDefaultApplicationAccessPolicy(appId string, strategy constant.ApplicationDefaultAccessPolicies) (*model.Application, error) {
+
+ url := fmt.Sprintf("%s/api/v2/applications/%s", c.Host, appId)
+ b, err := c.SendHttpRestRequest(url, http.MethodPost, map[string]interface{}{
+ "permissionStrategy": map[string]interface{}{
+ "defaultStrategy": strategy,
+ },
+ })
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data model.Application `json:"data"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return &resp.Data, nil
+}
+
+// GetAuthorizedTargets
+// 获取具备某些资源操作权限的主体
+func (c *Client) GetAuthorizedTargets(req *model.GetAuthorizedTargetsRequest) (*struct {
+ TotalCount int64 `json:"totalCount"`
+ List []struct {
+ Actions []string `json:"actions"`
+ TargetType string `json:"targetType"`
+ TargetIdentifier string `json:"targetIdentifier"`
+ } `json:"list"`
+}, error) {
+ data, _ := json.Marshal(&req)
+ variables := make(map[string]interface{})
+ json.Unmarshal(data, &variables)
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.GetAuthorizedTargetsDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ AuthorizedTargets struct {
+ TotalCount int64 `json:"totalCount"`
+ List []struct {
+ Actions []string `json:"actions"`
+ TargetType string `json:"targetType"`
+ TargetIdentifier string `json:"targetIdentifier"`
+ } `json:"list"`
+ } `json:"authorizedTargets"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.AuthorizedTargets, nil
+}
+
+// GetAuthorizedTargetsCode
+// 获取具备某些资源操作权限的主体, 分组返回 Code
+func (c *Client) GetAuthorizedTargetsCode(req *model.GetAuthorizedTargetsRequest) (*struct {
+ TotalCount int64 `json:"totalCount"`
+ List []struct {
+ Actions []string `json:"actions"`
+ TargetType string `json:"targetType"`
+ TargetIdentifier string `json:"targetIdentifier"`
+ } `json:"list"`
+}, error) {
+ data, _ := json.Marshal(&req)
+ variables := make(map[string]interface{})
+ json.Unmarshal(data, &variables)
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.GetAuthorizedTargetsCodeDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ AuthorizedTargets struct {
+ TotalCount int64 `json:"totalCount"`
+ List []struct {
+ Actions []string `json:"actions"`
+ TargetType string `json:"targetType"`
+ TargetIdentifier string `json:"targetIdentifier"`
+ } `json:"list"`
+ } `json:"authorizedTargetsCode"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.AuthorizedTargets, nil
+}
+
+/*func (c *Client) CheckResourcePermissionBatch(request model.CheckResourcePermissionBatchRequest) (bool, error) {
+ data, _ := json.Marshal(&request)
+ variables := make(map[string]interface{})
+ json.Unmarshal(data, &variables)
+ b, err := c.SendHttpRequest(c.Host+"/api/v2/acl/check-resource-permission-batch", constant.HttpMethodPost, constant.StringEmpty, variables)
+ resultJson, err := simplejson.NewJson(b)
+ result, err := resultJson.Get("code").Int64()
+ if err != nil {
+ return false, err
+ }
+ return result == 200, nil
+}
+
+func (c *Client) GetAuthorizedResourcesOfResourceKind(request model.GetAuthorizedResourcesOfResourceKindRequest) (bool, error) {
+ data, _ := json.Marshal(&request)
+ variables := make(map[string]interface{})
+ json.Unmarshal(data, &variables)
+ b, err := c.SendHttpRequest(c.Host+"/api/v2/acl/get-authorized-resources-of-resource-kind", constant.HttpMethodPost, constant.StringEmpty, variables)
+ resultJson, err := simplejson.NewJson(b)
+ result, err := resultJson.Get("code").Int64()
+ if err != nil {
+ return false, err
+ }
+ return result == 200, nil
+}*/
diff --git a/lib/management/acl_management_client_test.go b/lib/management/acl_management_client_test.go
new file mode 100644
index 0000000..38021e4
--- /dev/null
+++ b/lib/management/acl_management_client_test.go
@@ -0,0 +1,310 @@
+package management
+
+import (
+ "fmt"
+ "github.com/Authing/authing-go-sdk/lib/constant"
+ "github.com/Authing/authing-go-sdk/lib/model"
+ "log"
+ "testing"
+)
+
+func TestClient_IsAllowed(t *testing.T) {
+ client, err := NewClientWithError(userPoolId, appSecret)
+ if err != nil {
+ fmt.Println(err)
+ return
+ }
+ log.Println("==========判断某个用户是否对某个资源有某个操作权限==========")
+
+ req := model.IsAllowedRequest{
+ Resource: "7629:read",
+ Action: "read",
+ UserId: "611b2ff477d701441c25e29e",
+ Namespace: nil,
+ }
+ resp, _ := client.IsAllowed(req)
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_Allow(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========允许某个用户对某个资源进行某个操作==========")
+ req := model.AllowRequest{
+ Resource: "7629:read",
+ Action: "add",
+ UserId: "611b2ff477d701441c25e29e",
+ Namespace: "6123528118b7794b2420b311",
+ }
+ resp, _ := client.Allow(req)
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_AuthorizeResource(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========将一个(类)资源批量授权给用户、角色、分组、组织机构==========")
+ var actions []string
+ actions = append(actions, "*")
+ opt := model.AuthorizeResourceOpt{
+ TargetType: model.EnumPolicyAssignmentTargetTypeUser,
+ TargetIdentifier: "611b2ff477d701441c25e29e",
+ Actions: actions,
+ }
+ var opts []model.AuthorizeResourceOpt
+ opts = append(opts, opt)
+ req := model.AuthorizeResourceRequest{
+ Namespace: "6123528118b7794b2420b311",
+ Resource: "7629:read",
+ ResourceType: model.EnumResourceTypeBUTTON,
+ Opts: opts,
+ }
+ resp, _ := client.AuthorizeResource(req)
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_RevokeResource(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========将一个(类)资源取消授权给用户、角色、分组、组织机构==========")
+ var actions []string
+ actions = append(actions, "*")
+ opt := model.AuthorizeResourceOpt{
+ TargetType: model.EnumPolicyAssignmentTargetTypeGroup,
+ TargetIdentifier: "74wr2RzVV0",
+ Actions: actions,
+ }
+ var opts []model.AuthorizeResourceOpt
+ opts = append(opts, opt)
+ req := model.RevokeResourceRequest{
+ Namespace: "default",
+ Resource: "open",
+ ResourceType: model.EnumResourceTypeAPI,
+ Opts: opts,
+ }
+ resp, _ := client.RevokeResource(req)
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_ListAuthorizedResourcesForCustom(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========将一个(类)资源取消授权给用户、角色、分组、组织机构==========")
+ rt := model.EnumResourceTypeAPI
+ req := model.ListAuthorizedResourcesRequest{
+ Namespace: "default",
+ ResourceType: &rt,
+ TargetIdentifier: "616d41b7410a33da0cb70e65",
+ TargetType: constant.USER,
+ }
+ resp, _ := client.ListAuthorizedResourcesForCustom(req)
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_ProgrammaticAccessAccountList(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========编程账号列表==========")
+
+ resp, _ := client.ProgrammaticAccessAccountList("6168f95e81d5e20f9cb72f22", 1, 10)
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_CreateProgrammaticAccessAccount(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========编程账号列表==========")
+
+ resp, _ := client.CreateProgrammaticAccessAccount("6168f95e81d5e20f9cb72f22", nil, nil)
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_DisableProgrammaticAccessAccount(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========禁用编程账号==========")
+
+ resp, _ := client.DisableProgrammaticAccessAccount("617109c03d185a5092395cab")
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_EnableProgrammaticAccessAccount(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========启用编程账号==========")
+
+ resp, _ := client.EnableProgrammaticAccessAccount("617109c03d185a5092395cab")
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_RefreshProgrammaticAccessAccountSecret(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========刷新编程账号访问秘钥==========")
+
+ resp, _ := client.RefreshProgrammaticAccessAccountSecret("617109c03d185a5092395cab", nil)
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_DeleteProgrammaticAccessAccount(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========刷新编程账号访问秘钥==========")
+
+ resp, _ := client.DeleteProgrammaticAccessAccount("617109c03d185a5092395cab")
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_ListNamespaceResources(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========获取Namespace下资源列表==========")
+
+ req := model.ListResourceRequest{
+ ResourceType: model.EnumResourceTypeAPI,
+ Namespace: "default",
+ Page: 1,
+ Limit: 10,
+ }
+ resp, _ := client.ListNamespaceResources(req)
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_GetResourceById(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========根据ID获取资源==========")
+
+ resp, _ := client.GetResourceById("616cdf9d1642b20d8c2ec555")
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_GetResourceByCode(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========根据 Code 获取资源==========")
+
+ resp, _ := client.GetResourceByCode("ddddd", "default")
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_CreateResource(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========创建资源==========")
+ req := &model.CreateResourceRequest{
+ Code: "nmw",
+ Namespace: "default",
+ Actions: []model.ActionsModel{{
+ Name: "qqw",
+ Description: "qwe",
+ }},
+ }
+ resp, _ := client.CreateResource(req)
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_UpdateResource(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========修改资源==========")
+ req := &model.UpdateResourceRequest{
+
+ Namespace: "default",
+ Actions: []model.ActionsModel{{
+ Name: "qqwcc",
+ Description: "qwe",
+ }},
+ }
+ resp, _ := client.UpdateResource("nmw", req)
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_DeleteResource(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========删除资源==========")
+
+ resp, _ := client.DeleteResource("nmw", "default")
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_GetApplicationAccessPolicies(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========获取应用访问策略==========")
+
+ resp, _ := client.GetApplicationAccessPolicies("6168f95e81d5e20f9cb72f22", 1, 10)
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_EnableApplicationAccessPolicies(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========启用应用访问策略==========")
+ req := &model.ApplicationAccessPoliciesRequest{
+ TargetType: constant.USER,
+ InheritByChildren: true,
+ TargetIdentifiers: []string{"616e905ebc18f0f106973a29"},
+ Namespace: "default",
+ }
+ resp, _ := client.EnableApplicationAccessPolicies("6168f95e81d5e20f9cb72f22", req)
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_DisableApplicationAccessPolicies(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========禁用应用访问策略==========")
+ req := &model.ApplicationAccessPoliciesRequest{
+ TargetType: constant.USER,
+ InheritByChildren: true,
+ TargetIdentifiers: []string{"616e905ebc18f0f106973a29"},
+ Namespace: "default",
+ }
+ resp, _ := client.DisableApplicationAccessPolicies("6168f95e81d5e20f9cb72f22", req)
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_AllowApplicationAccessPolicies(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========允许应用访问策略==========")
+ req := &model.ApplicationAccessPoliciesRequest{
+ TargetType: constant.USER,
+ InheritByChildren: true,
+ TargetIdentifiers: []string{"616e905ebc18f0f106973a29"},
+ Namespace: "default",
+ }
+ resp, _ := client.AllowApplicationAccessPolicies("6168f95e81d5e20f9cb72f22", req)
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_DenyApplicationAccessPolicies(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========拒绝应用访问策略==========")
+ req := &model.ApplicationAccessPoliciesRequest{
+ TargetType: constant.USER,
+ InheritByChildren: true,
+ TargetIdentifiers: []string{"616e905ebc18f0f106973a29"},
+ Namespace: "default",
+ }
+ resp, _ := client.DenyApplicationAccessPolicies("6168f95e81d5e20f9cb72f22", req)
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_UpdateDefaultApplicationAccessPolicy(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========修改应用默认访问策略==========")
+
+ resp, _ := client.UpdateDefaultApplicationAccessPolicy("6168f95e81d5e20f9cb72f22", constant.AllowAll)
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_GetAuthorizedTargets(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========获取拥有资源的对象列表==========")
+
+ req := &model.GetAuthorizedTargetsRequest{
+ TargetType: constant.GROUP,
+ Resource: "open",
+ Namespace: "default",
+ ResourceType: model.EnumResourceTypeAPI,
+ }
+ resp, _ := client.GetAuthorizedTargetsCode(req)
+ log.Printf("%+v\n", resp)
+}
+
+/*func TestClient_CheckResourcePermissionBatch(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========获取用户对某些资源的权限==========")
+ var resources []string
+ resources = append(resources, "7629:read")
+ req := model.CheckResourcePermissionBatchRequest{
+ UserId: "61436e13634d7bdc0fd7ce6e",
+ Namespace: "default",
+ Resources: resources,
+ }
+ resp, _ := client.CheckResourcePermissionBatch(req)
+ log.Printf("%+v\n", resp)
+}*/
diff --git a/lib/management/application_management_client.go b/lib/management/application_management_client.go
new file mode 100644
index 0000000..7d6c701
--- /dev/null
+++ b/lib/management/application_management_client.go
@@ -0,0 +1,302 @@
+package management
+
+import (
+ "errors"
+ "fmt"
+ "net/http"
+
+ "github.com/Authing/authing-go-sdk/lib/constant"
+ "github.com/Authing/authing-go-sdk/lib/model"
+ jsoniter "github.com/json-iterator/go"
+)
+
+// ListApplication
+// 获取应用列表
+func (c *Client) ListApplication(req *model.CommonPageRequest) (*struct {
+ List []model.Application `json:"list"`
+}, error) {
+
+ url := fmt.Sprintf("%v/api/v2/applications?page=%v&limit=%v", c.Host, req.Page, req.Limit)
+ b, err := c.SendHttpRequest(url, constant.HttpMethodGet, "", nil)
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data struct {
+ List []model.Application `json:"list"`
+ } `json:"data"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return &resp.Data, nil
+}
+
+// CreateApplication
+// 创建应用
+func (c *Client) CreateApplication(name, identifier, redirectUris string, logo *string) (*model.Application, error) {
+ vars := make(map[string]interface{})
+ vars["name"] = name
+ vars["identifier"] = identifier
+ vars["redirectUris"] = redirectUris
+ if logo != nil {
+ vars["logo"] = logo
+ }
+ url := fmt.Sprintf("%v/api/v2/applications", c.Host)
+ b, err := c.SendHttpRequest(url, constant.HttpMethodPost, "", vars)
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data model.Application `json:"data"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return &resp.Data, nil
+}
+
+// DeleteApplication
+// 删除应用
+func (c *Client) DeleteApplication(appId string) (*string, error) {
+ url := fmt.Sprintf("%v/api/v2/applications/%v", c.Host, appId)
+ b, err := c.SendHttpRestRequest(url, http.MethodDelete, nil)
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return &resp.Message, nil
+}
+
+// RefreshApplicationSecret
+// 刷新应用密钥
+func (c *Client) RefreshApplicationSecret(appId string) (*model.Application, error) {
+ url := fmt.Sprintf("%s/api/v2/application/%s/refresh-secret", c.Host, appId)
+ b, err := c.SendHttpRestRequest(url, http.MethodPatch, nil)
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data model.Application `json:"data"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return &resp.Data, nil
+}
+
+// ListApplicationActiveUsers
+// 查看应用下已登录用户
+func (c *Client) ListApplicationActiveUsers(appId string, page, limit int) (*struct {
+ List []model.ApplicationActiveUsers `json:"list"`
+ TotalCount int64 `json:"totalCount"`
+}, error) {
+ url := fmt.Sprintf("%s/api/v2/applications/%s/active-users?page=%v&limit=%v", c.Host, appId, page, limit)
+ b, err := c.SendHttpRestRequest(url, http.MethodGet, nil)
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data struct {
+ List []model.ApplicationActiveUsers `json:"list"`
+ TotalCount int64 `json:"totalCount"`
+ } `json:"data"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return &resp.Data, nil
+}
+
+// FindApplicationById
+// 通过应用 id 查找应用详情
+func (c *Client) FindApplicationById(appId string) (*model.Application, error) {
+ url := fmt.Sprintf("%s/api/v2/applications/%s", c.Host, appId)
+ b, err := c.SendHttpRestRequest(url, http.MethodGet, nil)
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data model.Application `json:"data"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return &resp.Data, nil
+}
+
+// CreateApplicationAgreement
+// 创建应用协议
+func (c *Client) CreateApplicationAgreement(appId, title string, lang *string, required *bool) (*model.ApplicationAgreement, error) {
+ if lang == nil {
+ var def = "zh-CN"
+ lang = &def
+ }
+ if required == nil {
+ var def = true
+ required = &def
+ }
+ vars := map[string]interface{}{
+ "title": title,
+ "lang": lang,
+ "required": required,
+ }
+ url := fmt.Sprintf("%s/api/v2/applications/%s/agreements", c.Host, appId)
+ b, err := c.SendHttpRestRequest(url, http.MethodPost, vars)
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data model.ApplicationAgreement `json:"data"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return &resp.Data, nil
+}
+
+// ListApplicationAgreement
+// 应用协议列表
+func (c *Client) ListApplicationAgreement(appId string) (*struct {
+ List []model.ApplicationAgreement `json:"list"`
+ TotalCount int64 `json:"totalCount"`
+}, error) {
+
+ url := fmt.Sprintf("%s/api/v2/applications/%s/agreements", c.Host, appId)
+ b, err := c.SendHttpRestRequest(url, http.MethodGet, nil)
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data struct {
+ List []model.ApplicationAgreement `json:"list"`
+ TotalCount int64 `json:"totalCount"`
+ } `json:"data"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return &resp.Data, nil
+}
+
+// ModifyApplicationAgreement
+// 修改应用协议
+func (c *Client) ModifyApplicationAgreement(appId, agreementId, title string, lang *string, required *bool) (*model.ApplicationAgreement, error) {
+ if lang == nil {
+ var def = "zh-CN"
+ lang = &def
+ }
+ if required == nil {
+ var def = true
+ required = &def
+ }
+ vars := map[string]interface{}{
+ "title": title,
+ "lang": lang,
+ "required": required,
+ }
+ url := fmt.Sprintf("%s/api/v2/applications/%s/agreements/%v", c.Host, appId, agreementId)
+ b, err := c.SendHttpRestRequest(url, http.MethodPut, vars)
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data model.ApplicationAgreement `json:"data"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return &resp.Data, nil
+}
+
+// DeleteApplicationAgreement
+// 删除应用协议
+func (c *Client) DeleteApplicationAgreement(appId, agreementId string) (*string, error) {
+
+ url := fmt.Sprintf("%s/api/v2/applications/%s/agreements/%v", c.Host, appId, agreementId)
+ b, err := c.SendHttpRestRequest(url, http.MethodDelete, nil)
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return &resp.Message, nil
+}
+
+// SortApplicationAgreement
+// 排序应用协议
+func (c *Client) SortApplicationAgreement(appId string, ids []string) (*string, error) {
+
+ url := fmt.Sprintf("%s/api/v2/applications/%s/agreements/sort", c.Host, appId)
+ b, err := c.SendHttpRestRequest(url, http.MethodPost, map[string]interface{}{"ids": ids})
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return &resp.Message, nil
+}
+
+// ApplicationTenants
+// 获取应用关联租户
+func (c *Client) ApplicationTenants(appId string) (*model.ApplicationTenantDetails, error) {
+
+ url := fmt.Sprintf("%s/api/v2/application/%v/tenants", c.Host, appId)
+ b, err := c.SendHttpRestRequest(url, http.MethodGet, nil)
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data model.ApplicationTenantDetails `json:"data"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return &resp.Data, nil
+}
diff --git a/lib/management/application_management_client_test.go b/lib/management/application_management_client_test.go
new file mode 100644
index 0000000..07cb627
--- /dev/null
+++ b/lib/management/application_management_client_test.go
@@ -0,0 +1,136 @@
+package management
+
+import (
+ "fmt"
+ "log"
+ "testing"
+
+ "github.com/Authing/authing-go-sdk/lib/model"
+)
+
+func TestClient_ListApplication(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========查询应用列表==========")
+
+ req := &model.CommonPageRequest{
+ Page: 1,
+ Limit: 10,
+ }
+ resp, err := client.ListApplication(req)
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_CreateApplication(t *testing.T) {
+ log.Println(userPoolId)
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========创建应用==========")
+ resp, err := client.CreateApplication("sqq12", "noww22", "http://locaqql", nil)
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_DeleteApplication(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========删除应用==========")
+ resp, err := client.DeleteApplication("616fbde39a4c5ce0518d87fc")
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_RefreshApplicationSecret(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========刷新应用秘钥==========")
+ resp, err := client.RefreshApplicationSecret("614bf4af279893d5ab645e58")
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_ListApplicationActiveUsers(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========获取应用下登录用户==========")
+ resp, err := client.ListApplicationActiveUsers("61527e0124a5f0df0eed7af2", 1, 100)
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+ log.Println(resp.TotalCount)
+}
+
+func TestClient_FindApplicationById(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========根据ID获取应用==========")
+ resp, err := client.FindApplicationById("614bf4af279893d5ab645e58")
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_CreateApplicationAgreement(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========创建应用策略==========")
+ resp, err := client.CreateApplicationAgreement("614bf4af279893d5ab645e58", "cccqq", nil, nil)
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_ListApplicationAgreement(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========遍历应用策略==========")
+ resp, err := client.ListApplicationAgreement("614bf4af279893d5ab645e58")
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_ModifyApplicationAgreement(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========修改应用策略==========")
+ resp, err := client.ModifyApplicationAgreement("614bf4af279893d5ab645e58", "249", "cccqq2", nil, nil)
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_DeleteApplicationAgreement(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========删除应用策略==========")
+ resp, err := client.DeleteApplicationAgreement("614bf4af279893d5ab645e58", "249")
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_SortApplicationAgreement(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========删除应用策略==========")
+ resp, err := client.SortApplicationAgreement("614bf4af279893d5ab645e58", []string{"238"})
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_ApplicationTenants(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========获取应用关联租户==========")
+ resp, err := client.ApplicationTenants("61b8366efa768b57d65b6394")
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
diff --git a/lib/management/audit_log_management_client.go b/lib/management/audit_log_management_client.go
new file mode 100644
index 0000000..8204325
--- /dev/null
+++ b/lib/management/audit_log_management_client.go
@@ -0,0 +1,83 @@
+package management
+
+import (
+ "errors"
+ "fmt"
+ "github.com/Authing/authing-go-sdk/lib/model"
+ jsoniter "github.com/json-iterator/go"
+ "net/http"
+)
+
+// ListAuditLogs
+// 审计日志列表查询
+func (c *Client) ListAuditLogs(req *model.ListAuditLogsRequest) (*struct {
+ List []interface{} `json:"list"`
+ TotalCount int64 `json:"totalCount"`
+}, error) {
+
+ if req.UserIds != nil {
+
+ var formatUserIds = make([]string, 0)
+ for _, d := range *req.UserIds {
+ formatUserId := "arn:cn:authing:user:" + d
+ formatUserIds = append(formatUserIds, formatUserId)
+ }
+ req.UserIds = &formatUserIds
+ }
+ vars := make(map[string]interface{})
+ url := fmt.Sprintf("%s/api/v2/analysis/audit", c.Host)
+ b, err := c.SendHttpRestRequest(url, http.MethodGet, vars)
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data struct {
+ List []interface{} `json:"list"`
+ TotalCount int64 `json:"totalCount"`
+ } `json:"data"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return &resp.Data, nil
+}
+
+// ListUserAction
+// 查看用户操作日志
+func (c *Client) ListUserAction(req *model.ListUserActionRequest) (*struct {
+ List []interface{} `json:"list"`
+ TotalCount int64 `json:"totalCount"`
+}, error) {
+
+ if req.UserIds != nil {
+
+ var formatUserIds = make([]string, 0)
+ for _, d := range *req.UserIds {
+ formatUserId := "arn:cn:authing:user:" + d
+ formatUserIds = append(formatUserIds, formatUserId)
+ }
+ req.UserIds = &formatUserIds
+ }
+ vars := make(map[string]interface{})
+ url := fmt.Sprintf("%s/api/v2/analysis/user-action", c.Host)
+ b, err := c.SendHttpRestRequest(url, http.MethodGet, vars)
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data struct {
+ List []interface{} `json:"list"`
+ TotalCount int64 `json:"totalCount"`
+ } `json:"data"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return &resp.Data, nil
+}
diff --git a/lib/management/audit_log_management_client_test.go b/lib/management/audit_log_management_client_test.go
new file mode 100644
index 0000000..f69192a
--- /dev/null
+++ b/lib/management/audit_log_management_client_test.go
@@ -0,0 +1,44 @@
+package management
+
+import (
+ "fmt"
+ "github.com/Authing/authing-go-sdk/lib/model"
+ "log"
+ "testing"
+)
+
+func TestClient_ListAuditLogs(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========审计日志列表==========")
+ var userIds = []string{"xx", "xxq"}
+ page := 1
+ limit := 10
+ req := &model.ListAuditLogsRequest{
+ Page: &page,
+ Limit: &limit,
+ UserIds: &userIds,
+ }
+ resp, err := client.ListAuditLogs(req)
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_ListUserActionLogs(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========用户操作日志列表==========")
+ var userIds = []string{"xx", "xxq"}
+ page := 1
+ limit := 10
+ req := &model.ListUserActionRequest{
+ Page: &page,
+ Limit: &limit,
+ UserIds: &userIds,
+ }
+ resp, err := client.ListUserAction(req)
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
diff --git a/lib/management/groups_management_client.go b/lib/management/groups_management_client.go
new file mode 100644
index 0000000..27ff55a
--- /dev/null
+++ b/lib/management/groups_management_client.go
@@ -0,0 +1,258 @@
+package management
+
+import (
+ "errors"
+ "github.com/Authing/authing-go-sdk/lib/constant"
+ "github.com/Authing/authing-go-sdk/lib/model"
+ jsoniter "github.com/json-iterator/go"
+ "net/http"
+)
+
+// CreateGroups
+// 创建分组
+func (c *Client) CreateGroups(req *model.CreateGroupsRequest) (*model.GroupModel, error) {
+ data, _ := jsoniter.Marshal(req)
+ variables := make(map[string]interface{})
+ jsoniter.Unmarshal(data, &variables)
+
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.CreateGroupsDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ CreateGroup model.GroupModel `json:"createGroup"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.CreateGroup, nil
+}
+
+// UpdateGroups
+// 修改分组
+func (c *Client) UpdateGroups(req *model.UpdateGroupsRequest) (*model.GroupModel, error) {
+ data, _ := jsoniter.Marshal(req)
+ variables := make(map[string]interface{})
+ jsoniter.Unmarshal(data, &variables)
+
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.UpdateGroupsDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ UpdateGroup model.GroupModel `json:"updateGroup"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.UpdateGroup, nil
+}
+
+// DetailGroups
+// 获取分组详情
+func (c *Client) DetailGroups(code string) (*model.GroupModel, error) {
+
+ variables := map[string]interface{}{"code": code}
+
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.DetailGroupsDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ Group model.GroupModel `json:"group"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.Group, nil
+}
+
+// DeleteGroups
+// 删除分组
+func (c *Client) DeleteGroups(code string) (*model.CommonMessageAndCode, error) {
+ r, e := c.BatchDeleteGroups([]string{code})
+ return r, e
+}
+
+// ListGroups
+// 获取分组列表
+func (c *Client) ListGroups(page, limit int) (*struct {
+ TotalCount int64 `json:"totalCount"`
+ List []model.GroupModel `json:"list"`
+}, error) {
+
+ variables := map[string]interface{}{"page": page, "limit": limit}
+
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.ListGroupsDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ Groups struct {
+ TotalCount int64 `json:"totalCount"`
+ List []model.GroupModel `json:"list"`
+ } `json:"groups"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.Groups, nil
+}
+
+// BatchDeleteGroups
+// 批量删除分组
+func (c *Client) BatchDeleteGroups(codes []string) (*model.CommonMessageAndCode, error) {
+ variables := map[string]interface{}{"codeList": codes}
+
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.DeleteGroupsDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ DeleteGroups model.CommonMessageAndCode `json:"deleteGroups"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.DeleteGroups, nil
+}
+
+// ListGroupsUser
+// 获取分组用户列表
+func (c *Client) ListGroupsUser(code string, page, limit int, withCustomData bool) (*struct {
+ TotalCount int `json:"totalCount"`
+ List []model.User `json:"list"`
+}, error) {
+ variables := map[string]interface{}{
+ "code": code,
+ "page": page,
+ "limit": limit,
+ }
+ query := constant.ListGroupUserDocument
+ if withCustomData {
+ query = constant.ListGroupUserWithCustomDocument
+ }
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, query, variables)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ Group model.GetGroupUserResponse `json:"group"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.Group.Users, nil
+}
+
+// AddUserToGroups
+// 添加用户
+func (c *Client) AddUserToGroups(code string, userIds []string) (*model.CommonMessageAndCode, error) {
+ variables := map[string]interface{}{
+ "code": code,
+ "userIds": userIds,
+ }
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.AddUserToGroupDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ AddUserToGroup model.CommonMessageAndCode `json:"addUserToGroup"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.AddUserToGroup, nil
+}
+
+//RemoveGroupUsers
+//移除用户
+func (c *Client) RemoveGroupUsers(code string, userIds []string) (*model.CommonMessageAndCode, error) {
+
+ variables := map[string]interface{}{
+ "code": code,
+ "userIds": userIds,
+ }
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.RemoveUserInGroupDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ RemoveUserFromGroup model.CommonMessageAndCode `json:"removeUserFromGroup"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.RemoveUserFromGroup, nil
+}
+
+//ListGroupsAuthorizedResources
+//获取分组被授权的所有资源
+func (c *Client) ListGroupsAuthorizedResources(req *model.ListGroupsAuthorizedResourcesRequest) (*struct {
+ TotalCount int64 `json:"totalCount"`
+ List []model.AuthorizedResource `json:"list"`
+}, error) {
+ data, _ := jsoniter.Marshal(req)
+ variables := make(map[string]interface{})
+ jsoniter.Unmarshal(data, &variables)
+
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.ListGroupAuthorizedResourcesDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ Group struct {
+ AuthorizedResources struct {
+ TotalCount int64 `json:"totalCount"`
+ List []model.AuthorizedResource `json:"list"`
+ } `json:"authorizedResources"`
+ } `json:"group"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.Group.AuthorizedResources, nil
+}
diff --git a/lib/management/groups_management_client_test.go b/lib/management/groups_management_client_test.go
new file mode 100644
index 0000000..2da66ba
--- /dev/null
+++ b/lib/management/groups_management_client_test.go
@@ -0,0 +1,99 @@
+package management
+
+import (
+ "fmt"
+ "github.com/Authing/authing-go-sdk/lib/model"
+ "log"
+ "testing"
+)
+
+func TestClient_CreateGroups(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========创建分组==========")
+ req := &model.CreateGroupsRequest{
+ Code: "goSDK",
+ Name: "goSDK",
+ }
+ resp, err := client.CreateGroups(req)
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_UpdateGroups(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========更新分组==========")
+ newCode := "newGoSdk"
+ req := &model.UpdateGroupsRequest{
+ Code: "goSDK",
+ NewCode: &newCode,
+ }
+ resp, err := client.UpdateGroups(req)
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_DetailGroups(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========分组详情==========")
+
+ resp, err := client.DetailGroups("newGoSdk")
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_DeleteGroups(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========删除分组==========")
+
+ resp, err := client.DeleteGroups("newGoSdk")
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_ListGroups(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========分组列表==========")
+
+ resp, err := client.ListGroups(1, 10)
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_ListGroupsUser(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========分组下的用户列表==========")
+
+ resp, err := client.ListGroupsUser("jjwjl", 1, 10, false)
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_ListGroupsAuthorizedResources(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========获取分组授权资源==========")
+ cc := model.EnumResourceTypeAPI
+ nm := "default"
+ req := &model.ListGroupsAuthorizedResourcesRequest{
+ Code: "kcerb",
+ //Code: "kmvnk",
+ ResourceType: &cc,
+ Namespace: &nm,
+ }
+ resp, err := client.ListGroupsAuthorizedResources(req)
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
diff --git a/lib/management/management_client.go b/lib/management/management_client.go
new file mode 100644
index 0000000..813beca
--- /dev/null
+++ b/lib/management/management_client.go
@@ -0,0 +1,469 @@
+package management
+
+import (
+ "bytes"
+ "context"
+ "encoding/json"
+ "errors"
+ "fmt"
+ "github.com/Authing/authing-go-sdk/lib/constant"
+ "github.com/Authing/authing-go-sdk/lib/model"
+ "github.com/Authing/authing-go-sdk/lib/util/cacheutil"
+ "github.com/bitly/go-simplejson"
+ jsoniter "github.com/json-iterator/go"
+ "github.com/valyala/fasthttp"
+ "golang.org/x/oauth2"
+ "io/ioutil"
+ "net/http"
+ "strings"
+ "sync"
+ "time"
+)
+
+// Client is a client for interacting with the GraphQL API of `Authing`
+type Client struct {
+ HttpClient *http.Client
+ userPoolId string
+ secret string
+ Host string
+
+ // Log is called with various debug information.
+ // To log to standard out, use:
+ // client.Log = func(s string) { log.Println(s) }
+ Log func(s string)
+}
+
+func NewClient(userPoolId string, secret string, host ...string) *Client {
+ var clientHost string
+ if len(host) == 0 {
+ clientHost = constant.CoreAuthingDefaultUrl
+ } else {
+ clientHost = host[0]
+ }
+ c := &Client{
+ userPoolId: userPoolId,
+ secret: secret,
+ Host: clientHost,
+ }
+ if c.HttpClient == nil {
+ c.HttpClient = &http.Client{}
+ accessToken, err := GetAccessToken(c)
+ if err != nil {
+ return nil
+ }
+ src := oauth2.StaticTokenSource(
+ &oauth2.Token{AccessToken: accessToken},
+ )
+ c.HttpClient = oauth2.NewClient(context.Background(), src)
+ }
+ return c
+}
+
+func NewClientWithError(userPoolId string, secret string, host ...string) (*Client, error) {
+ if userPoolId == "" {
+ return nil, errors.New("请填写 userPoolId 参数")
+ }
+ if secret == "" {
+ return nil, errors.New("请填写 secret 参数")
+ }
+ var clientHost string
+ if len(host) == 0 {
+ clientHost = constant.CoreAuthingDefaultUrl
+ } else {
+ clientHost = host[0]
+ }
+ c := &Client{
+ userPoolId: userPoolId,
+ secret: secret,
+ Host: clientHost,
+ }
+ if c.HttpClient == nil {
+ c.HttpClient = &http.Client{}
+ accessToken, err := GetAccessToken(c)
+ if err != nil {
+ return nil, err
+ }
+ src := oauth2.StaticTokenSource(
+ &oauth2.Token{AccessToken: accessToken},
+ )
+ c.HttpClient = oauth2.NewClient(context.Background(), src)
+ }
+ return c, nil
+}
+
+// NewHttpClient creates a new Authing user endpoint GraphQL API client
+func NewHttpClient(userPoolId string, appSecret string, isDev bool) *Client {
+ c := &Client{
+ userPoolId: userPoolId,
+ }
+
+ /*if c.Client == nil {
+ var endpointURL string
+ if isDev {
+ endpointURL = constant.CoreEndPointDevUrl + "/graphql/v2"
+ } else {
+ endpointURL = constant.CoreEndPointProdUrl + "/graphql/v2"
+ }
+ accessToken, err := GetAccessToken(userPoolId, appSecret)
+ if err != nil {
+ log.Println(err)
+ //return nil
+ }
+ src := oauth2.StaticTokenSource(
+ &oauth2.Token{AccessToken: accessToken},
+ )
+ c.HttpClient = oauth2.NewClient(context.Background(), src)
+
+ c.Client = graphql.NewClient(endpointURL, c.HttpClient)
+ }*/
+
+ return c
+}
+
+// NewOauthClient creates a new Authing oauth endpoint GraphQL API client
+func NewOauthClient(userPoolId string, appSecret string, isDev bool) *Client {
+ c := &Client{
+ userPoolId: userPoolId,
+ }
+
+ /*if c.Client == nil {
+ var endpointURL string
+ if isDev {
+ endpointURL = constant.CoreEndPointDevUrl
+ } else {
+ endpointURL = constant.CoreEndPointProdUrl
+ }
+ accessToken, err := GetAccessToken(userPoolId, appSecret)
+ if err != nil {
+ log.Println(err)
+ return nil
+ }
+
+ src := oauth2.StaticTokenSource(
+ &oauth2.Token{AccessToken: accessToken},
+ )
+
+ httpClient := oauth2.NewClient(context.Background(), src)
+
+ if isDev {
+ endpointURL = constant.CoreEndPointDevUrl
+ } else {
+ endpointURL = constant.CoreEndPointProdUrl
+ }
+
+ c.Client = graphql.NewClient(endpointURL, httpClient)
+ }*/
+
+ return c
+}
+
+func (c *Client) SendHttpRequest(url string, method string, query string, variables map[string]interface{}) ([]byte, error) {
+ var req *http.Request
+ if method == constant.HttpMethodGet {
+ req, _ = http.NewRequest(http.MethodGet, url, nil)
+ if variables != nil && len(variables) > 0 {
+ q := req.URL.Query()
+ for key, value := range variables {
+ q.Add(key, fmt.Sprintf("%v", value))
+ }
+ req.URL.RawQuery = q.Encode()
+ }
+
+ } else {
+ in := struct {
+ Query string `json:"query"`
+ Variables map[string]interface{} `json:"variables,omitempty"`
+ }{
+ Query: query,
+ Variables: variables,
+ }
+ var buf bytes.Buffer
+ var err error
+ if query == constant.StringEmpty {
+ err = json.NewEncoder(&buf).Encode(variables)
+ } else {
+ err = json.NewEncoder(&buf).Encode(in)
+ }
+ if err != nil {
+ return nil, err
+ }
+ req, err = http.NewRequest(method, url, &buf)
+ req.Header.Add("Content-Type", "application/json")
+ }
+
+ //增加header选项
+ if !strings.HasPrefix(query, "query accessToken") {
+ token, _ := GetAccessToken(c)
+ req.Header.Add("Authorization", "Bearer "+token)
+ }
+ req.Header.Add("x-authing-userpool-id", ""+c.userPoolId)
+ req.Header.Add("x-authing-request-from", constant.SdkType)
+ req.Header.Add("x-authing-sdk-version", constant.SdkVersion)
+ req.Header.Add("x-authing-app-id", ""+constant.AppId)
+ res, err := c.HttpClient.Do(req)
+ if err != nil {
+ return nil, err
+ }
+ defer res.Body.Close()
+ body, err := ioutil.ReadAll(res.Body)
+ if err != nil {
+ return nil, err
+ }
+ return body, nil
+}
+
+func (c *Client) SendHttpRestRequest(url string, method string, variables map[string]interface{}) ([]byte, error) {
+ var req *http.Request
+ if method == constant.HttpMethodGet {
+ req, _ = http.NewRequest(http.MethodGet, url, nil)
+ if variables != nil && len(variables) > 0 {
+ q := req.URL.Query()
+ for key, value := range variables {
+ q.Add(key, fmt.Sprintf("%v", value))
+ }
+ req.URL.RawQuery = q.Encode()
+ }
+
+ } else {
+
+ var buf bytes.Buffer
+ var err error
+ if variables != nil {
+ err = json.NewEncoder(&buf).Encode(variables)
+
+ }
+ if err != nil {
+ return nil, err
+ }
+ req, err = http.NewRequest(method, url, &buf)
+ req.Header.Add("Content-Type", "application/json")
+ }
+
+ token, _ := GetAccessToken(c)
+ req.Header.Add("Authorization", "Bearer "+token)
+
+ req.Header.Add("x-authing-userpool-id", ""+c.userPoolId)
+ req.Header.Add("x-authing-request-from", constant.SdkType)
+ req.Header.Add("x-authing-sdk-version", constant.SdkVersion)
+ req.Header.Add("x-authing-app-id", ""+constant.AppId)
+ res, err := c.HttpClient.Do(req)
+ if err != nil {
+ return nil, err
+ }
+ defer res.Body.Close()
+ body, err := ioutil.ReadAll(res.Body)
+ return body, nil
+}
+
+func (c *Client) httpGet(url string, client *http.Client) (string, error) {
+ reqest, err := http.NewRequest(constant.HttpMethodGet, c.Host+url, nil)
+ if err != nil {
+ return "", err
+ }
+
+ //增加header选项
+ token, _ := GetAccessToken(c)
+ reqest.Header.Add("Authorization", "Bearer "+token)
+ reqest.Header.Add("x-authing-userpool-id", ""+c.userPoolId)
+ reqest.Header.Add("x-authing-request-from", constant.SdkType)
+ reqest.Header.Add("x-authing-sdk-version", constant.SdkVersion)
+ reqest.Header.Add("x-authing-app-id", ""+constant.AppId)
+
+ resp, err := client.Do(reqest)
+ if err != nil {
+ return "", err
+ }
+ body, err := ioutil.ReadAll(resp.Body)
+ if err != nil {
+ return "", err
+ }
+ result := string(body)
+ return result, nil
+}
+
+func (c *Client) SendHttpRequestV2(url string, method string, query string, variables map[string]interface{}) ([]byte, error) {
+ in := struct {
+ Query string `json:"query"`
+ Variables map[string]interface{} `json:"variables,omitempty"`
+ }{
+ Query: query,
+ Variables: variables,
+ }
+
+ var buf bytes.Buffer
+ err := json.NewEncoder(&buf).Encode(in)
+ if err != nil {
+ return nil, err
+ }
+ req := fasthttp.AcquireRequest()
+
+ req.SetRequestURI(url)
+ token, _ := GetAccessToken(c)
+ req.Header.Add("Authorization", "Bearer "+token)
+ req.Header.Add("x-authing-userpool-id", ""+c.userPoolId)
+ req.Header.Add("x-authing-request-from", constant.SdkType)
+ req.Header.Add("x-authing-sdk-version", constant.SdkVersion)
+ req.Header.Add("x-authing-app-id", ""+constant.AppId)
+ req.Header.SetMethod(method)
+ req.SetBody(buf.Bytes())
+
+ resp := fasthttp.AcquireResponse()
+ client := &fasthttp.Client{}
+ client.Do(req, resp)
+ body := resp.Body()
+ return body, err
+}
+
+func QueryAccessToken(client *Client) (*model.AccessTokenRes, error) {
+ type Data struct {
+ AccessToken model.AccessTokenRes `json:"accessToken"`
+ }
+ type Result struct {
+ Data Data `json:"data"`
+ }
+
+ variables := map[string]interface{}{
+ "userPoolId": client.userPoolId,
+ "secret": client.secret,
+ }
+
+ b, err := client.SendHttpRequest(client.Host+constant.CoreAuthingGraphqlPath, constant.HttpMethodPost, constant.AccessTokenDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ err = checkError(b)
+ if err != nil {
+ return nil, err
+ }
+ var r Result
+ if b != nil {
+ json.Unmarshal(b, &r)
+ }
+ return &r.Data.AccessToken, nil
+}
+
+func checkError(b []byte) error {
+ json, err := simplejson.NewJson(b)
+ if err != nil {
+ return err
+ }
+ repErrors, exist := json.CheckGet("errors")
+ if !exist {
+ return nil
+ }
+ result, err := repErrors.Array()
+ if err != nil {
+ return err
+ }
+ if result != nil && len(result) > 0 {
+ reason, err := json.Get("errors").GetIndex(0).Get("message").Get("message").String()
+ if err != nil {
+ return err
+ }
+ return errors.New(reason)
+ }
+ return nil
+}
+
+func GetAccessToken(client *Client) (string, error) {
+ // 从缓存获取token
+ cacheToken, b := cacheutil.GetCache(constant.TokenCacheKeyPrefix + client.userPoolId)
+ if b && cacheToken != nil {
+ return cacheToken.(string), nil
+ }
+ // 从服务获取token,加锁
+ var mutex sync.Mutex
+ mutex.Lock()
+ defer mutex.Unlock()
+ cacheToken, b = cacheutil.GetCache(constant.TokenCacheKeyPrefix + client.userPoolId)
+ if b && cacheToken != nil {
+ return cacheToken.(string), nil
+ }
+ token, err := QueryAccessToken(client)
+ if err != nil {
+ return "", err
+ }
+ var expire = (*(token.Exp) - time.Now().Unix() - 259200) * int64(time.Second)
+ cacheutil.SetCache(constant.TokenCacheKeyPrefix+client.userPoolId, *token.AccessToken, time.Duration(expire))
+ return *token.AccessToken, nil
+}
+
+func CreateRequestParam(param struct{}) map[string]interface{} {
+ data, _ := json.Marshal(¶m)
+ variables := make(map[string]interface{})
+ json.Unmarshal(data, &variables)
+ return variables
+}
+
+// SendEmail
+// 发送邮件
+func (c *Client) SendEmail(email string, scene model.EnumEmailScene) (*model.CommonMessageAndCode, error) {
+
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.SendMailDocument,
+ map[string]interface{}{"email": email, "scene": scene})
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ SendMail model.CommonMessageAndCode `json:"sendEmail"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.SendMail, nil
+}
+
+// CheckLoginStatusByToken
+// 检测登录状态
+func (c *Client) CheckLoginStatusByToken(token string) (*model.CheckLoginStatusResponse, error) {
+
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.CheckLoginStatusDocument,
+ map[string]interface{}{"token": token})
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ CheckLoginStatus model.CheckLoginStatusResponse `json:"checkLoginStatus"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.CheckLoginStatus, nil
+}
+
+// IsPasswordValid
+// 检测密码是否合法
+func (c *Client) IsPasswordValid(password string) (*struct {
+ Valid bool `json:"valid"`
+ Message string `json:"message"`
+}, error) {
+
+ url := fmt.Sprintf("%s/api/v2/password/check", c.Host)
+ b, err := c.SendHttpRestRequest(url, http.MethodPost, map[string]interface{}{"password": password})
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data struct {
+ Valid bool `json:"valid"`
+ Message string `json:"message"`
+ } `json:"data"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return &resp.Data, nil
+}
diff --git a/lib/management/namespace_management_client.go b/lib/management/namespace_management_client.go
new file mode 100644
index 0000000..8f15ff1
--- /dev/null
+++ b/lib/management/namespace_management_client.go
@@ -0,0 +1,105 @@
+package management
+
+import (
+ "encoding/json"
+ "errors"
+ "fmt"
+ "github.com/Authing/authing-go-sdk/lib/model"
+ jsoniter "github.com/json-iterator/go"
+ "net/http"
+)
+
+// CreateNamespace
+// 创建权限分组
+func (c *Client) CreateNamespace(request *model.EditNamespaceRequest) (*model.Namespace, error) {
+ data, _ := json.Marshal(&request)
+ variables := make(map[string]interface{})
+ json.Unmarshal(data, &variables)
+ url := fmt.Sprintf("%s/api/v2/resource-namespace/%s", c.Host, c.userPoolId)
+ b, err := c.SendHttpRestRequest(url, http.MethodPost, variables)
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data model.Namespace `json:"data"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return &resp.Data, nil
+}
+
+// UpdateNamespace
+// 修改权限分组
+func (c *Client) UpdateNamespace(id string, request *model.EditNamespaceRequest) (*model.Namespace, error) {
+
+ data, _ := json.Marshal(&request)
+ variables := make(map[string]interface{})
+ json.Unmarshal(data, &variables)
+
+ url := fmt.Sprintf("%s/api/v2/resource-namespace/%s/%s", c.Host, c.userPoolId, id)
+ b, err := c.SendHttpRestRequest(url, http.MethodPut, variables)
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data model.Namespace `json:"data"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return &resp.Data, nil
+}
+
+// DeleteNamespace
+// 删除权限分组
+func (c *Client) DeleteNamespace(id string) (*string, error) {
+
+ url := fmt.Sprintf("%s/api/v2/resource-namespace/%s/%s", c.Host, c.userPoolId, id)
+ b, err := c.SendHttpRestRequest(url, http.MethodDelete, nil)
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return &resp.Message, nil
+}
+
+// ListNamespace
+// 权限分组列表
+func (c *Client) ListNamespace(page, limit int) (*struct {
+ List []model.Namespace `json:"list"`
+ Total int64 `json:"total"`
+}, error) {
+
+ url := fmt.Sprintf("%s/api/v2/resource-namespace/%s?page=%v&limit=%v", c.Host, c.userPoolId, page, limit)
+ b, err := c.SendHttpRestRequest(url, http.MethodGet, nil)
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data struct {
+ List []model.Namespace `json:"list"`
+ Total int64 `json:"total"`
+ } `json:"data"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return &resp.Data, nil
+}
diff --git a/lib/management/namespace_management_client_test.go b/lib/management/namespace_management_client_test.go
new file mode 100644
index 0000000..c980f0d
--- /dev/null
+++ b/lib/management/namespace_management_client_test.go
@@ -0,0 +1,62 @@
+package management
+
+import (
+ "fmt"
+ "github.com/Authing/authing-go-sdk/lib/model"
+ "log"
+ "testing"
+)
+
+func TestClient_CreateNamespace(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========创建权限分组==========")
+ code := "qCode"
+ name := "qName"
+ req := &model.EditNamespaceRequest{
+ Code: &code,
+ Name: &name,
+ }
+ resp, err := client.CreateNamespace(req)
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_UpdateNamespace(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========修改权限分组==========")
+ code := "qCodeww"
+ name := "qNameww"
+ req := &model.EditNamespaceRequest{
+ Code: &code,
+ Name: &name,
+ }
+ resp, err := client.UpdateNamespace("54156", req)
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_ListNamespace(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========权限分组列表==========")
+
+ resp, err := client.ListNamespace(1, 10)
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_DeleteNamespace(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========权限分组列表==========")
+
+ resp, err := client.DeleteNamespace("54156")
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
diff --git a/lib/management/organization_management_client.go b/lib/management/organization_management_client.go
new file mode 100644
index 0000000..2b209e4
--- /dev/null
+++ b/lib/management/organization_management_client.go
@@ -0,0 +1,611 @@
+package management
+
+import (
+ "encoding/json"
+ "errors"
+ "fmt"
+ "github.com/Authing/authing-go-sdk/lib/constant"
+ "github.com/Authing/authing-go-sdk/lib/enum"
+ "github.com/Authing/authing-go-sdk/lib/model"
+ jsoniter "github.com/json-iterator/go"
+ "log"
+ "net/http"
+)
+
+// ExportAll
+// 导出所有组织机构
+func (c *Client) ExportAll() ([]model.OrgNode, error) {
+ var q []model.OrgNode
+ b, err := c.SendHttpRequest(c.Host+"/api/v2/orgs/export", constant.HttpMethodGet, "", nil)
+ if err != nil {
+ return q, err
+ }
+ var response model.ExportAllOrganizationResponse
+ err = jsoniter.Unmarshal(b, &response)
+ if err != nil {
+ log.Println(err)
+ }
+ return response.Data, nil
+}
+
+// ListMembers
+// 获取节点成员
+func (c *Client) ListMembers(req *model.ListMemberRequest) (*model.Node, error) {
+ if req.SortBy == "" {
+ req.SortBy = enum.SortByCreatedAtAsc
+ }
+ if req.Page == 0 {
+ req.Page = 1
+ }
+ if req.Limit == 0 {
+ req.Limit = 10
+ }
+ variables := map[string]interface{}{
+ "id": req.NodeId,
+ "limit": req.Limit,
+ "sortBy": req.SortBy,
+ "page": req.Page,
+ "includeChildrenNodes": req.IncludeChildrenNodes,
+ }
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, constant.HttpMethodPost, constant.NodeByIdWithMembersDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ var response model.NodeByIdResponse
+ jsoniter.Unmarshal(b, &response)
+ return &response.Data.NodeById, nil
+}
+
+// TODO
+func (c *Client) GetOrganizationList(request model.QueryListRequest) (model.PaginatedOrgs, error) {
+ var result model.PaginatedOrgs
+ data, _ := json.Marshal(&request)
+ variables := make(map[string]interface{})
+ json.Unmarshal(data, &variables)
+ b, err := c.SendHttpRequest(c.Host+"/api/v2/orgs/pagination", constant.HttpMethodGet, "", variables)
+ if err != nil {
+ return result, err
+ }
+ var response model.ListOrganizationResponse
+ jsoniter.Unmarshal(b, &response)
+ return response.Data, nil
+}
+
+// GetOrganizationById
+// 获取组织机构详情
+func (c *Client) GetOrganizationById(orgId string) (*model.Org, error) {
+ variables := map[string]interface{}{
+ "id": orgId,
+ }
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, constant.HttpMethodPost, constant.OrgDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ var response model.GetOrganizationByIdResponse
+ jsoniter.Unmarshal(b, &response)
+ return &response.Data.Org, nil
+}
+
+// GetOrganizationChildren
+// 获取子节点列表
+func (c *Client) GetOrganizationChildren(nodeId string, depth int) (*[]model.Node, error) {
+ var result *[]model.Node
+ variables := map[string]interface{}{
+ "nodeId": nodeId,
+ "depth": depth,
+ }
+ b, err := c.SendHttpRequest(c.Host+"/api/v2/orgs/children", constant.HttpMethodGet, "", variables)
+ if err != nil {
+ return result, err
+ }
+ var response model.GetOrganizationChildrenResponse
+ jsoniter.Unmarshal(b, &response)
+ return &response.Data, nil
+}
+
+// CreateOrg
+// 创建组织机构
+func (c *Client) CreateOrg(req *model.CreateOrgRequest) (*model.OrgResponse, error) {
+ data, _ := jsoniter.Marshal(req)
+ variables := make(map[string]interface{})
+ jsoniter.Unmarshal(data, &variables)
+
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.CreateOrgDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ CreateOrg model.OrgResponse `json:"createOrg"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.CreateOrg, nil
+}
+
+// DeleteOrgById
+// 删除组织机构
+func (c *Client) DeleteOrgById(id string) (*model.CommonMessageAndCode, error) {
+
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.DeleteOrgDocument, map[string]interface{}{
+ "id": id,
+ })
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ DeleteOrg model.CommonMessageAndCode `json:"deleteOrg"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.DeleteOrg, nil
+}
+
+// ListOrg
+// 获取用户池组织机构列表
+func (c *Client) ListOrg(page, limit int) (*model.PaginatedOrgs, error) {
+
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.ListOrgDocument, map[string]interface{}{
+ "page": page,
+ "limit": limit,
+ })
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ Orgs model.PaginatedOrgs `json:"orgs"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.Orgs, nil
+}
+
+// AddOrgNode
+// 在组织机构中添加一个节点
+func (c *Client) AddOrgNode(req *model.AddOrgNodeRequest) (*model.AddNodeOrg, error) {
+ data, _ := jsoniter.Marshal(req)
+ variables := make(map[string]interface{})
+ jsoniter.Unmarshal(data, &variables)
+
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.AddOrgNodeDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ AddNode model.AddNodeOrg `json:"addNode"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.AddNode, nil
+}
+
+// GetOrgNodeById
+// 获取某个节点详情
+func (c *Client) GetOrgNodeById(id string) (*model.OrgNodeChildStr, error) {
+
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.GetOrgNodeDocument, map[string]interface{}{
+ "id": id,
+ })
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ NodeById model.OrgNodeChildStr `json:"nodeById"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.NodeById, nil
+}
+
+// UpdateOrgNode
+// 修改节点
+func (c *Client) UpdateOrgNode(req *model.UpdateOrgNodeRequest) (*model.Node, error) {
+ data, _ := jsoniter.Marshal(req)
+ variables := make(map[string]interface{})
+ jsoniter.Unmarshal(data, &variables)
+
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.UpdateOrgNodeDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ UpdateNode model.Node `json:"updateNode"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.UpdateNode, nil
+}
+
+// DeleteOrgNode
+// 删除节点
+func (c *Client) DeleteOrgNode(orgId, nodeId string) (*model.CommonMessageAndCode, error) {
+
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.DeleteOrgNodeDocument, map[string]interface{}{
+ "orgId": orgId,
+ "nodeId": nodeId,
+ })
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ DeleteNode model.CommonMessageAndCode `json:"deleteNode"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.DeleteNode, nil
+}
+
+// IsRootNode
+// 判断是否为根节点
+func (c *Client) IsRootNode(orgId, nodeId string) (*bool, error) {
+
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.IsRootNodeDocument, map[string]interface{}{
+ "orgId": orgId,
+ "nodeId": nodeId,
+ })
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ IsRootNode bool `json:"isRootNode"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.IsRootNode, nil
+}
+
+// MoveOrgNode
+// 移动节点
+func (c *Client) MoveOrgNode(orgId, nodeId, targetParentId string) (*model.AddNodeOrg, error) {
+
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.MoveNodeDocument, map[string]interface{}{
+ "orgId": orgId,
+ "nodeId": nodeId,
+ "targetParentId": targetParentId,
+ })
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ MoveNode model.AddNodeOrg `json:"moveNode"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.MoveNode, nil
+}
+
+// GetRootNode
+// 获取根节点
+func (c *Client) GetRootNode(orgId string) (*model.OrgNodeChildStr, error) {
+
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.GetRootNodeDocument, map[string]interface{}{
+ "orgId": orgId,
+ })
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ RootNode model.OrgNodeChildStr `json:"rootNode"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.RootNode, nil
+}
+
+// ImportNodeByJSON
+// 通过 JSON 导入
+func (c *Client) ImportNodeByJSON(jsonStr string) (*string, error) {
+
+ url := fmt.Sprintf("%s/api/v2/orgs/import", c.Host)
+ b, err := c.SendHttpRestRequest(url, http.MethodPost, map[string]interface{}{
+ "filetype": "json",
+ "file": jsonStr,
+ })
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return &resp.Message, nil
+}
+
+// AddMembers
+// 节点添加成员
+func (c *Client) AddMembers(nodeId string, userIds []string) (*model.Node, error) {
+
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.AddMembersDocument, map[string]interface{}{
+ "nodeId": nodeId,
+ "userIds": userIds,
+ })
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ AddMember model.Node `json:"addMember"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.AddMember, nil
+}
+
+// MoveNodeMembers
+// 移动节点成员
+func (c *Client) MoveNodeMembers(nodeId, targetNodeId string, userIds []string) (*model.CommonMessageAndCode, error) {
+
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.MoveNodeMembersDocument, map[string]interface{}{
+ "userIds": userIds,
+ "targetNodeId": targetNodeId,
+ "sourceNodeId": nodeId,
+ })
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ MoveMembers model.CommonMessageAndCode `json:"moveMembers"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.MoveMembers, nil
+}
+
+// DeleteNodeMembers
+// 删除节点成员
+func (c *Client) DeleteNodeMembers(nodeId string, userIds []string) (*model.Node, error) {
+
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.RemoveNodeMembersDocument, map[string]interface{}{
+ "userIds": userIds,
+ "nodeId": nodeId,
+ })
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ RemoveMembers model.Node `json:"removeMember"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.RemoveMembers, nil
+}
+
+// SetMainDepartment
+// 设置用户主部门
+func (c *Client) SetMainDepartment(departmentId, userId string) (*model.CommonMessageAndCode, error) {
+
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.SetUserMainDepartmentDocument, map[string]interface{}{
+ "userId": userId,
+ "departmentId": departmentId,
+ })
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ SetMainDepartment model.CommonMessageAndCode `json:"setMainDepartment"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.SetMainDepartment, nil
+}
+
+// ExportByOrgId
+// 导出某个组织机构
+func (c *Client) ExportByOrgId(orgId string) (*model.OrgNode, error) {
+
+ url := fmt.Sprintf("%s/api/v2/orgs/export?org_id=%s", c.Host, orgId)
+ b, err := c.SendHttpRestRequest(url, http.MethodGet, nil)
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data model.OrgNode `json:"data"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return &resp.Data, nil
+}
+
+// ListAuthorizedResourcesByNodeId
+// 获取组织机构节点被授权的所有资源
+func (c *Client) ListAuthorizedResourcesByNodeId(req *model.ListAuthorizedResourcesByIdRequest) (*struct {
+ TotalCount int64 `json:"totalCount"`
+ List []model.AuthorizedResource `json:"list"`
+}, error) {
+ data, _ := json.Marshal(&req)
+ variables := make(map[string]interface{})
+ json.Unmarshal(data, &variables)
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.ListNodeByIdAuthorizedResourcesDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ NodeByCode struct {
+ AuthorizedResources struct {
+ TotalCount int64 `json:"totalCount"`
+ List []model.AuthorizedResource `json:"list"`
+ } `json:"authorizedResources"`
+ } `json:"nodeByCode"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.NodeByCode.AuthorizedResources, nil
+}
+
+// ListAuthorizedResourcesByNodeCode
+// 获取组织机构节点被授权的所有资源
+func (c *Client) ListAuthorizedResourcesByNodeCode(req *model.ListAuthorizedResourcesByNodeCodeRequest) (*struct {
+ TotalCount int64 `json:"totalCount"`
+ List []model.AuthorizedResource `json:"list"`
+}, error) {
+ data, _ := json.Marshal(&req)
+ variables := make(map[string]interface{})
+ json.Unmarshal(data, &variables)
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.ListNodeByIdAuthorizedResourcesDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ NodeById struct {
+ AuthorizedResources struct {
+ TotalCount int64 `json:"totalCount"`
+ List []model.AuthorizedResource `json:"list"`
+ } `json:"authorizedResources"`
+ } `json:"nodeById"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.NodeById.AuthorizedResources, nil
+}
+
+// SearchNodes
+// 搜索组织机构节点
+func (c *Client) SearchNodes(keywords string) (*[]model.OrgNodeChildStr, error) {
+
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost,
+ constant.SearchNodesDocument, map[string]interface{}{"keyword": keywords})
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ SearchNodes []model.OrgNodeChildStr `json:"searchNodes"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.SearchNodes, nil
+}
+
+//
+//// StartSync
+//// 组织机构同步
+//func (c *Client) StartSync(providerType constant.ProviderTypeEnum, connectionId *string) (*interface{}, error) {
+//
+// url:=fmt.Sprintf("%s/connections/enterprise/%s/start-sync",c.Host,providerType)
+// vars:=make(map[string]interface{})
+// if providerType == constant.AD {
+// url = fmt.Sprintf("%s/api/v2/ad/sync",c.Host)
+// vars["connectionId"]=connectionId
+// }
+// b, err := c.SendHttpRestRequest(url, http.MethodPost, vars)
+// if err != nil {
+// return nil, err
+// }
+// resp :=&struct {
+// Message string `json:"message"`
+// Code int64 `json:"code"`
+// Data interface{} `json:"data"`
+// }{}
+// jsoniter.Unmarshal(b, &resp)
+// if resp.Code != 200 {
+// return nil, errors.New(resp.Message)
+// }
+// return &resp.Data, nil
+//}
diff --git a/lib/management/organization_management_client_test.go b/lib/management/organization_management_client_test.go
new file mode 100644
index 0000000..002a2e5
--- /dev/null
+++ b/lib/management/organization_management_client_test.go
@@ -0,0 +1,232 @@
+package management
+
+import (
+ "fmt"
+ "github.com/Authing/authing-go-sdk/lib/enum"
+ "github.com/Authing/authing-go-sdk/lib/model"
+ "log"
+ "testing"
+)
+
+const (
+ // prod
+
+ //userPoolId = ""
+ //appSecret = ""
+ //userPoolId = ""
+ //appSecret = ""
+ userPoolId = ""
+ appSecret = ""
+)
+
+func TestClient_ExportAll(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========导出所有组织机构数据==========")
+ resp, err := client.ExportAll()
+ if err != nil {
+ log.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_All(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========导出所有组织机构数据==========")
+ resp, _ := client.ExportAll()
+ log.Printf("%+v\n", resp)
+ log.Println("==========获取节点成员==========")
+ var req = &model.ListMemberRequest{
+ NodeId: "60cd9d3a4b96cfff16e7e5f4",
+ Page: 1,
+ Limit: 10,
+ IncludeChildrenNodes: true,
+ }
+ resp1, _ := client.ListMembers(req)
+ log.Printf("%+v\n", resp1)
+}
+
+func TestClient_GetOrganizationList(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========获取用户池组织机构列表==========")
+ req := model.QueryListRequest{
+ Page: 1,
+ Limit: 10,
+ SortBy: enum.SortByCreatedAtAsc,
+ }
+ resp, _ := client.GetOrganizationList(req)
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_GetOrganizationById(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========获取组织机构详情==========")
+ resp, _ := client.GetOrganizationById("60cd9d3ab98280ce211bc834")
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_GetOrganizationChildren(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========获取第 n 层组织机构==========")
+ resp, _ := client.GetOrganizationChildren("60cd9d3a4b96cfff16e7e5f4", 1)
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_CreateOrg(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========创建组织机构==========")
+ req := &model.CreateOrgRequest{
+ Name: "GoSDKOrg2",
+ }
+ resp, _ := client.CreateOrg(req)
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_DeleteOrgById(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========删除组织机构==========")
+ resp, _ := client.DeleteOrgById("617224b00869fe94de9357de")
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_ListOrg(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========遍历组织机构==========")
+ resp, _ := client.ListOrg(1, 10)
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_GetOrgNodeById(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========根据ID获取节点==========")
+ resp, _ := client.GetOrgNodeById("61725b9f3ad07a44b85302b1")
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_UpdateOrgNode(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========修改节点==========")
+ updateName := "updateName"
+ req := &model.UpdateOrgNodeRequest{
+ Name: &updateName,
+ Id: "617230eba040848abb3689b7",
+ }
+ resp, _ := client.UpdateOrgNode(req)
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_DeleteOrgNode(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========删除节点==========")
+ resp, _ := client.DeleteOrgNode("617230eba040848abb3689b7", "6172315f5371116d5ad5ead9")
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_IsRootNode(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========判断是否根节点==========")
+ resp, _ := client.IsRootNode("6142c2c41c6e6c6cc3edfd88", "6142e08f64d5a8873598e9fb")
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_MoveOrgNode(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========移动节点==========")
+ resp, _ := client.MoveOrgNode("6142c2c41c6e6c6cc3edfd88", "6142e08f64d5a8873598e9fb", "6142e03436f09aa7e66c1935")
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_GetRootNode(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========获取根节点==========")
+ resp, _ := client.GetRootNode("6142c2c41c6e6c6cc3edfd88")
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_ImportNodeByJSON(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========通过JSON导入==========")
+ json := `
+ {
+ "name": "北京非凡科技有限公司",
+ "code": "feifan",
+ "children": []
+ }`
+ resp, _ := client.ImportNodeByJSON(json)
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_AddMembers(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========新增节点成员==========")
+ resp, _ := client.AddMembers("61722ece541df9301478b17d", []string{"6141876341abedef979c3740"})
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_MoveNodeMembers(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========移动节点成员==========")
+ resp, _ := client.MoveNodeMembers("61722ece541df9301478b17d", "617230eba040848abb3689b7", []string{"6141876341abedef979c3740"})
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_DeleteNodeMembers(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========删除节点成员==========")
+ resp, _ := client.DeleteNodeMembers("617230eba040848abb3689b7", []string{"6141876341abedef979c3740"})
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_SetMainDepartment(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========设置用户主部门==========")
+ resp, _ := client.SetMainDepartment("6142e0483f54818690c99600", "6141876341abedef979c3740")
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_ExportByOrgId(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========设置用户主部门==========")
+ resp, _ := client.ExportByOrgId("6142c2c41c6e6c6cc3edfd88")
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_ListAuthorizedResourcesByNodeId(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========获取机构授权资源==========")
+ req := &model.ListAuthorizedResourcesByIdRequest{Id: "61725b9f321fcc1ca9e36ddc"}
+ resp, _ := client.ListAuthorizedResourcesByNodeId(req)
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_SearchNodes(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========获取机构授权资源==========")
+
+ resp, _ := client.SearchNodes("qq")
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_AddOrgNode(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========权限分组列表==========")
+
+ req := &model.AddOrgNodeRequest{
+ Name: "qqqw",
+ ParentNodeId: "617230eba040848abb3689b7",
+ OrgId: "61722ececf7cd66d1ec27075",
+ }
+ resp, err := client.AddOrgNode(req)
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+//
+//func TestClient_StartSync(t *testing.T) {
+// client := NewClient(userPoolId, appSecret)
+// log.Println("==========获取机构授权资源==========")
+//
+// resp, _ := client.StartSync( constant.WechatWork,nil)
+// log.Printf("%+v\n", resp)
+//}
diff --git a/lib/management/policies_management_client.go b/lib/management/policies_management_client.go
new file mode 100644
index 0000000..69274c1
--- /dev/null
+++ b/lib/management/policies_management_client.go
@@ -0,0 +1,274 @@
+package management
+
+import (
+ "errors"
+ "github.com/Authing/authing-go-sdk/lib/constant"
+ "github.com/Authing/authing-go-sdk/lib/model"
+ jsoniter "github.com/json-iterator/go"
+ "net/http"
+)
+
+// CreatePolicy
+// 添加策略
+func (c *Client) CreatePolicy(req *model.PolicyRequest) (*model.CreatePolicyResponse, error) {
+ data, _ := jsoniter.Marshal(req)
+ vars := make(map[string]interface{})
+ jsoniter.Unmarshal(data, &vars)
+
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.CreatePolicyDocument, vars)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ CreatePolicy model.CreatePolicyResponse `json:"createPolicy"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.CreatePolicy, nil
+}
+
+// ListPolicy
+// 获取策略列表
+func (c *Client) ListPolicy(page, limit int) (*model.PaginatedPolicies, error) {
+
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.ListPolicyDocument,
+ map[string]interface{}{"page": page, "limit": limit})
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ Policies model.PaginatedPolicies `json:"policies"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.Policies, nil
+}
+
+// DetailPolicy
+// 获取策略详情
+func (c *Client) DetailPolicy(code string) (*model.Policy, error) {
+
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost,
+ constant.DetailPolicyDocument, map[string]interface{}{"code": code})
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ Policy model.Policy `json:"policy"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.Policy, nil
+}
+
+// UpdatePolicy
+// 修改策略
+func (c *Client) UpdatePolicy(req *model.PolicyRequest) (*model.UpdatePolicyResponse, error) {
+ data, _ := jsoniter.Marshal(req)
+ vars := make(map[string]interface{})
+ jsoniter.Unmarshal(data, &vars)
+
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.UpdatePolicyDocument, vars)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ UpdatePolicy model.UpdatePolicyResponse `json:"updatePolicy"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.UpdatePolicy, nil
+}
+
+// DeletePolicy
+// 删除策略
+func (c *Client) DeletePolicy(code string) (*model.CommonMessageAndCode, error) {
+
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost,
+ constant.DeletePolicyDocument, map[string]interface{}{"code": code})
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ DeletePolicy model.CommonMessageAndCode `json:"deletePolicy"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.DeletePolicy, nil
+}
+
+// BatchDeletePolicy
+// 删除策略
+func (c *Client) BatchDeletePolicy(codeList []string) (*model.CommonMessageAndCode, error) {
+
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost,
+ constant.BatchDeletePolicyDocument, map[string]interface{}{"codeList": codeList})
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ DeletePolicies model.CommonMessageAndCode `json:"deletePolicies"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.DeletePolicies, nil
+}
+
+// ListAssignments
+// 获取策略授权记录
+func (c *Client) ListAssignments(code string, page, limit int) (*model.PaginatedPolicyAssignments, error) {
+
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.PolicyAssignmentsDocument,
+ map[string]interface{}{"code": code, "page": page, "limit": limit})
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ PolicyAssignments model.PaginatedPolicyAssignments `json:"policyAssignments"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.PolicyAssignments, nil
+}
+
+// AddAssignments
+// 添加策略授权
+func (c *Client) AddAssignments(req *model.PolicyAssignmentsRequest) (*model.CommonMessageAndCode, error) {
+ data, _ := jsoniter.Marshal(req)
+ vars := make(map[string]interface{})
+ jsoniter.Unmarshal(data, &vars)
+
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.AddAssignmentsDocument, vars)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ AddPolicyAssignments model.CommonMessageAndCode `json:"addPolicyAssignments"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.AddPolicyAssignments, nil
+}
+
+// RemoveAssignments
+// 撤销策略授权
+func (c *Client) RemoveAssignments(req *model.PolicyAssignmentsRequest) (*model.CommonMessageAndCode, error) {
+ data, _ := jsoniter.Marshal(req)
+ vars := make(map[string]interface{})
+ jsoniter.Unmarshal(data, &vars)
+
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.RemoveAssignmentsDocument, vars)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ RemovePolicyAssignments model.CommonMessageAndCode `json:"removePolicyAssignments"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.RemovePolicyAssignments, nil
+}
+
+// EnableAssignments
+// 设置策略授权状态为开启
+func (c *Client) EnableAssignments(req *model.SwitchPolicyAssignmentsRequest) (*model.CommonMessageAndCode, error) {
+ data, _ := jsoniter.Marshal(req)
+ vars := make(map[string]interface{})
+ jsoniter.Unmarshal(data, &vars)
+
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.EnablePolicyAssignmentDocument, vars)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ EnablePolicyAssignment model.CommonMessageAndCode `json:"enablePolicyAssignment"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.EnablePolicyAssignment, nil
+}
+
+// DisableAssignments
+// 设置策略授权状态为关闭
+func (c *Client) DisableAssignments(req *model.SwitchPolicyAssignmentsRequest) (*model.CommonMessageAndCode, error) {
+ data, _ := jsoniter.Marshal(req)
+ vars := make(map[string]interface{})
+ jsoniter.Unmarshal(data, &vars)
+
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.DisablePolicyAssignmentDocument, vars)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ DisablePolicyAssignment model.CommonMessageAndCode `json:"disbalePolicyAssignment"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.DisablePolicyAssignment, nil
+}
diff --git a/lib/management/policies_management_client_test.go b/lib/management/policies_management_client_test.go
new file mode 100644
index 0000000..8183f93
--- /dev/null
+++ b/lib/management/policies_management_client_test.go
@@ -0,0 +1,152 @@
+package management
+
+import (
+ "fmt"
+ "github.com/Authing/authing-go-sdk/lib/model"
+ "log"
+ "testing"
+)
+
+func TestClient_CreatePolicy(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========创建策略==========")
+ ef := model.EnumPolicyEffectAllow
+ stateMents := &model.PolicyStatement{
+ Resource: "book:222c",
+ Effect: &ef,
+ Actions: []string{"'booksc:read'"},
+ }
+ req := &model.PolicyRequest{
+ Code: "qqx",
+ Statements: []model.PolicyStatement{*stateMents},
+ }
+ resp, err := client.CreatePolicy(req)
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_ListPolicy(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========创建策略==========")
+
+ resp, err := client.ListPolicy(1, 10)
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_DetailPolicy(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========策略详情==========")
+
+ resp, err := client.DetailPolicy("qqx")
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", *resp.Statements[0].Effect)
+}
+
+func TestClient_UpdatePolicy(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========修改策略==========")
+ ef := model.EnumPolicyEffectAllow
+ stateMents := &model.PolicyStatement{
+ Resource: "book:222cw",
+ Effect: &ef,
+ Actions: []string{"'booksc:read'"},
+ }
+ req := &model.PolicyRequest{
+ Code: "qqx",
+ Statements: []model.PolicyStatement{*stateMents},
+ }
+ resp, err := client.UpdatePolicy(req)
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_DeletePolicy(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========删除策略==========")
+
+ resp, err := client.DeletePolicy("qqx")
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_ListAssignments(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========删除策略==========")
+
+ resp, err := client.ListAssignments("tliewdutrn", 1, 10)
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_AddAssignments(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========授权策略==========")
+ req := &model.PolicyAssignmentsRequest{
+ Policies: []string{"tliewdutrn"},
+ TargetType: model.EnumPolicyAssignmentTargetTypeUser,
+ TargetIdentifiers: []string{"616e905ebc18f0f106973a29"},
+ }
+ resp, err := client.AddAssignments(req)
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_RemoveAssignments(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========撤销策略==========")
+ req := &model.PolicyAssignmentsRequest{
+ Policies: []string{"tliewdutrn"},
+ TargetType: model.EnumPolicyAssignmentTargetTypeUser,
+ TargetIdentifiers: []string{"616e905ebc18f0f106973a29"},
+ }
+ resp, err := client.RemoveAssignments(req)
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_EnableAssignments(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========撤销策略==========")
+ req := &model.SwitchPolicyAssignmentsRequest{
+ Policy: "tliewdutrn",
+ TargetType: model.EnumPolicyAssignmentTargetTypeUser,
+ TargetIdentifier: "616e905ebc18f0f106973a29",
+ }
+ resp, err := client.EnableAssignments(req)
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_DisableAssignments(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========撤销策略==========")
+ req := &model.SwitchPolicyAssignmentsRequest{
+ Policy: "tliewdutrn",
+ TargetType: model.EnumPolicyAssignmentTargetTypeUser,
+ TargetIdentifier: "616e905ebc18f0f106973a29",
+ }
+ resp, err := client.DisableAssignments(req)
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
diff --git a/lib/management/principal_authentication_management_client.go b/lib/management/principal_authentication_management_client.go
new file mode 100644
index 0000000..8b9d729
--- /dev/null
+++ b/lib/management/principal_authentication_management_client.go
@@ -0,0 +1,61 @@
+package management
+
+import (
+ "errors"
+ "fmt"
+ "github.com/Authing/authing-go-sdk/lib/model"
+ jsoniter "github.com/json-iterator/go"
+ "net/http"
+)
+
+// PrincipalAuthDetail
+// 获取主体认证详情
+func (c *Client) PrincipalAuthDetail(userId string) (*struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data interface{} `json:"data"`
+}, error) {
+
+ url := fmt.Sprintf("%s/api/v2/users/%s/management/principal_authentication", c.Host, userId)
+ b, err := c.SendHttpRestRequest(url, http.MethodGet, nil)
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data interface{} `json:"data"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return resp, nil
+}
+
+// PrincipalAuthenticate
+// 进行主体认证
+func (c *Client) PrincipalAuthenticate(userId string, req *model.PrincipalAuthenticateRequest) (*struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data interface{} `json:"data"`
+}, error) {
+ data, _ := jsoniter.Marshal(req)
+ vars := make(map[string]interface{})
+ jsoniter.Unmarshal(data, &vars)
+ url := fmt.Sprintf("%s/api/v2/users/%s/management/principal_authentication", c.Host, userId)
+ b, err := c.SendHttpRestRequest(url, http.MethodPost, vars)
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data interface{} `json:"data"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return resp, nil
+}
diff --git a/lib/management/principal_authentication_management_client_test.go b/lib/management/principal_authentication_management_client_test.go
new file mode 100644
index 0000000..db00c34
--- /dev/null
+++ b/lib/management/principal_authentication_management_client_test.go
@@ -0,0 +1,34 @@
+package management
+
+import (
+ "fmt"
+ "github.com/Authing/authing-go-sdk/lib/constant"
+ "github.com/Authing/authing-go-sdk/lib/model"
+ "log"
+ "testing"
+)
+
+func TestClient_PrincipalAuthDetail(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========主体认证详情==========")
+
+ resp, err := client.PrincipalAuthDetail("6139c4d24e78a4d706b7545b")
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+func TestClient_PrincipalAuthenticate(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========主体认证详情==========")
+ req := &model.PrincipalAuthenticateRequest{
+ Name: "xx",
+ Type: constant.P,
+ IdCard: "123123",
+ }
+ resp, err := client.PrincipalAuthenticate("6139c4d24e78a4d706b7545b", req)
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
diff --git a/lib/management/role_management_client.go b/lib/management/role_management_client.go
new file mode 100644
index 0000000..15a1ae4
--- /dev/null
+++ b/lib/management/role_management_client.go
@@ -0,0 +1,497 @@
+package management
+
+import (
+ "encoding/json"
+ "errors"
+ "github.com/Authing/authing-go-sdk/lib/constant"
+ "github.com/Authing/authing-go-sdk/lib/model"
+ jsoniter "github.com/json-iterator/go"
+ "net/http"
+)
+
+// GetRoleList
+// 获取角色列表
+func (c *Client) GetRoleList(request model.GetRoleListRequest) (*model.PaginatedRoles, error) {
+ data, _ := json.Marshal(&request)
+ variables := make(map[string]interface{})
+ json.Unmarshal(data, &variables)
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, constant.HttpMethodPost, constant.RolesDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ var response model.GetRoleListResponse
+ jsoniter.Unmarshal(b, &response)
+ return &response.Data.Roles, nil
+}
+
+// GetRoleUserList
+// 获取角色用户列表
+func (c *Client) GetRoleUserList(request model.GetRoleUserListRequest) (*struct {
+ TotalCount int64 `json:"totalCount"`
+ List []model.User `json:"list"`
+}, error) {
+ data, _ := json.Marshal(&request)
+ variables := make(map[string]interface{})
+ json.Unmarshal(data, &variables)
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, constant.HttpMethodPost, constant.RoleWithUsersDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ Role struct {
+ Users struct {
+ TotalCount int64 `json:"totalCount"`
+ List []model.User `json:"list"`
+ } `json:"users"`
+ } `json:"role"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ return &response.Data.Role.Users, nil
+}
+
+// CreateRole 创建角色
+func (c *Client) CreateRole(request model.CreateRoleRequest) (*model.Role, error) {
+ data, _ := json.Marshal(&request)
+ variables := make(map[string]interface{})
+ json.Unmarshal(data, &variables)
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.CreateRoleDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ //var response model.CreateRoleResponse
+ var response = &struct {
+ Data struct {
+ CreateRole model.Role `json:"createRole"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+
+ if response.Errors != nil {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.CreateRole, nil
+}
+
+// DeleteRole
+// 删除角色
+func (c *Client) DeleteRole(request model.DeleteRoleRequest) (*model.CommonMessageAndCode, error) {
+ data, _ := json.Marshal(&request)
+ variables := make(map[string]interface{})
+ json.Unmarshal(data, &variables)
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.DeleteRoleDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct{ DeleteRole model.CommonMessageAndCode } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+
+ if response.Errors != nil {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.DeleteRole, nil
+}
+
+// BatchDeleteRole
+// 批量删除角色
+func (c *Client) BatchDeleteRole(request model.BatchDeleteRoleRequest) (*model.CommonMessageAndCode, error) {
+ data, _ := json.Marshal(&request)
+ variables := make(map[string]interface{})
+ json.Unmarshal(data, &variables)
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.BatchDeleteRoleDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct{ DeleteRoles model.CommonMessageAndCode } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.DeleteRoles, nil
+}
+
+// RoleDetail
+// 角色详情
+func (c *Client) RoleDetail(request model.RoleDetailRequest) (*model.Role, error) {
+ data, _ := json.Marshal(&request)
+ variables := make(map[string]interface{})
+ json.Unmarshal(data, &variables)
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.RoleDetailDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ Role model.Role `json:"role"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.Role, nil
+}
+
+// UpdateRole
+// 更新角色
+func (c *Client) UpdateRole(request model.UpdateRoleRequest) (*model.Role, error) {
+ data, _ := json.Marshal(&request)
+ variables := make(map[string]interface{})
+ json.Unmarshal(data, &variables)
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.UpdateRoleDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct{ UpdateRole model.Role } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.UpdateRole, nil
+}
+
+// AssignRole
+// 角色 添加用户
+func (c *Client) AssignRole(request model.AssignAndRevokeRoleRequest) (*model.CommonMessageAndCode, error) {
+ data, _ := json.Marshal(&request)
+ variables := make(map[string]interface{})
+ json.Unmarshal(data, &variables)
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.AssignRoleDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct{ AssignRole model.CommonMessageAndCode } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.AssignRole, nil
+}
+
+// RevokeRole
+// 角色 移除用户
+func (c *Client) RevokeRole(request model.AssignAndRevokeRoleRequest) (*model.CommonMessageAndCode, error) {
+ data, _ := json.Marshal(&request)
+ variables := make(map[string]interface{})
+ json.Unmarshal(data, &variables)
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.RevokeRoleDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct{ RevokeRole model.CommonMessageAndCode } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.RevokeRole, nil
+}
+
+// ListRolePolicies
+// 获取角色策略列表
+func (c *Client) ListRolePolicies(request model.ListPoliciesRequest) (*model.ListPoliciesResponse, error) {
+
+ if request.Page == 0 {
+ request.Page = 1
+ }
+ if request.Limit == 0 {
+ request.Limit = 10
+ }
+
+ data, _ := json.Marshal(&request)
+ variables := make(map[string]interface{})
+ json.Unmarshal(data, &variables)
+
+ variables["targetType"] = constant.ROLE
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.ListPoliciesDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ PolicyAssignments model.ListPoliciesResponse `json:"policyAssignments"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.PolicyAssignments, nil
+}
+
+// AddRolePolicies
+// 给角色授权策略
+func (c *Client) AddRolePolicies(code string, policiesCode []string) (*model.CommonMessageAndCode, error) {
+
+ variables := make(map[string]interface{})
+
+ variables["policies"] = policiesCode
+ variables["targetType"] = constant.ROLE
+ variables["targetIdentifiers"] = []string{code}
+
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.AddPoliciesDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ AddPolicyAssignments model.CommonMessageAndCode `json:"addPolicyAssignments"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.AddPolicyAssignments, nil
+}
+
+// RemoveRolePolicies
+// 角色移除策略
+func (c *Client) RemoveRolePolicies(code string, policiesCode []string) (*model.CommonMessageAndCode, error) {
+
+ variables := make(map[string]interface{})
+
+ variables["policies"] = policiesCode
+ variables["targetType"] = constant.ROLE
+ variables["targetIdentifiers"] = []string{code}
+
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.RemovePoliciesDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ AddPolicyAssignments model.CommonMessageAndCode `json:"removePolicyAssignments"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.AddPolicyAssignments, nil
+}
+
+// ListRoleAuthorizedResources
+// 获取角色被授权的所有资源
+func (c *Client) ListRoleAuthorizedResources(code, namespace string, resourceType model.EnumResourceType) (*model.AuthorizedResources, error) {
+
+ variables := make(map[string]interface{})
+
+ variables["code"] = code
+ variables["resourceType"] = resourceType
+ variables["namespace"] = namespace
+
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.ListRoleAuthorizedResourcesDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ Role struct {
+ AuthorizedResources model.AuthorizedResources `json:"authorizedResources"`
+ } `json:"role"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.Role.AuthorizedResources, nil
+}
+
+// GetRoleUdfValue
+// 获取某个角色扩展字段列表
+func (c *Client) GetRoleUdfValue(id string) (*[]model.UserDefinedData, error) {
+
+ variables := make(map[string]interface{})
+
+ variables["targetType"] = constant.ROLE
+ variables["targetId"] = id
+
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.GetRoleUdfValueDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ Udv []model.UserDefinedData `json:"udv"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.Udv, nil
+}
+
+// GetRoleSpecificUdfValue
+// 获取某个角色某个扩展字段
+func (c *Client) GetRoleSpecificUdfValue(id string) (*[]model.UserDefinedData, error) {
+ return c.GetRoleUdfValue(id)
+}
+
+// BatchGetRoleUdfValue
+// 获取多个角色扩展字段列表
+func (c *Client) BatchGetRoleUdfValue(ids []string) (map[string][]model.UserDefinedData, error) {
+
+ variables := make(map[string]interface{})
+
+ variables["targetType"] = constant.ROLE
+ variables["targetIds"] = ids
+
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.BatchGetRoleUdfValueDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ UdfValueBatch []model.BatchRoleUdv `json:"udfValueBatch"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ resultMap := make(map[string][]model.UserDefinedData)
+ for _, v := range response.Data.UdfValueBatch {
+ resultMap[v.TargetId] = v.Data
+ }
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return resultMap, nil
+}
+
+// SetRoleUdfValue
+// 设置某个角色扩展字段列表
+func (c *Client) SetRoleUdfValue(id string, udv *model.KeyValuePair) (*[]model.UserDefinedData, error) {
+
+ variables := make(map[string]interface{})
+
+ v, _ := json.Marshal(udv.Value)
+ udv.Value = string(v)
+ variables["targetType"] = constant.ROLE
+ variables["targetId"] = id
+ variables["udvList"] = []model.KeyValuePair{*udv}
+
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.SetRoleUdfValueDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ SetUdvBatch []model.UserDefinedData `json:"setUdvBatch"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.SetUdvBatch, nil
+}
+
+// BatchSetRoleUdfValue
+// 设置多个角色扩展字段列表
+func (c *Client) BatchSetRoleUdfValue(request *[]model.SetUdfValueBatchInput) (*model.CommonMessageAndCode, error) {
+
+ variables := make(map[string]interface{})
+ input := make([]model.SetUdfValueBatchInput, 0)
+ for _, req := range *request {
+ v, _ := json.Marshal(&req.Value)
+ req.Value = string(v)
+ input = append(input, req)
+ }
+
+ variables["targetType"] = constant.ROLE
+ variables["input"] = input
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.BatchSetUdfValueDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ SetUdfValueBatch model.CommonMessageAndCode `json:"setUdfValueBatch"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.SetUdfValueBatch, nil
+}
+
+// RemoveRoleUdfValue
+// 删除用户的扩展字段
+func (c *Client) RemoveRoleUdfValue(id, key string) (*[]model.UserDefinedData, error) {
+
+ variables := make(map[string]interface{})
+ variables["targetType"] = constant.ROLE
+ variables["targetId"] = id
+ variables["key"] = key
+
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.RemoveUdfValueDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ RemoveUdv []model.UserDefinedData `json:"removeUdv"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.RemoveUdv, nil
+}
diff --git a/lib/management/role_management_client_test.go b/lib/management/role_management_client_test.go
new file mode 100644
index 0000000..55efa33
--- /dev/null
+++ b/lib/management/role_management_client_test.go
@@ -0,0 +1,252 @@
+package management
+
+import (
+ "fmt"
+ "github.com/Authing/authing-go-sdk/lib/enum"
+ "github.com/Authing/authing-go-sdk/lib/model"
+ "log"
+ "testing"
+)
+
+func TestClient_GetRoleList(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========获取角色列表==========")
+ req := model.GetRoleListRequest{
+ Page: 1,
+ Limit: 10,
+ SortBy: enum.SortByCreatedAtAsc,
+ }
+ resp, _ := client.GetRoleList(req)
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_GetRoleUserList(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========获取角色列表==========")
+ defaultNamespace := "default"
+ req := model.GetRoleUserListRequest{
+ Page: 1,
+ Limit: 10,
+ Code: "develop",
+ Namespace: &defaultNamespace,
+ }
+ resp, _ := client.GetRoleUserList(req)
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_CreateRole(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========创建角色==========")
+ req := model.CreateRoleRequest{
+ Code: "develop123456",
+ }
+ resp, err := client.CreateRole(req)
+
+ log.Printf("%+v\n %+v\n", resp, err)
+}
+
+func TestClient_DeleteRole(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========删除角色==========")
+ req := model.DeleteRoleRequest{
+ Code: "develop123456",
+ }
+ resp, err := client.DeleteRole(req)
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_DeleteRoles(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========批量删除角色==========")
+
+ req := model.BatchDeleteRoleRequest{
+ CodeList: []string{"develop123456", "develop1234562"},
+ }
+ resp, err := client.BatchDeleteRole(req)
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_UpdateRole(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========更新角色==========")
+
+ req := model.CreateRoleRequest{
+ Code: "ttCode",
+ }
+ resp, err := client.CreateRole(req)
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+
+ updateRequest := model.UpdateRoleRequest{
+ Code: "ttCode",
+ }
+ resp, err = client.UpdateRole(updateRequest)
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_RoleDetail(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========角色详情==========")
+
+ req := model.RoleDetailRequest{
+ Code: "NewCode",
+ }
+ resp, err := client.RoleDetail(req)
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_AssignRole(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========分配角色==========")
+
+ req := model.AssignAndRevokeRoleRequest{
+ RoleCodes: []string{"NewCode"},
+ UserIds: []string{"615551a3dcdd486139a917b1"},
+ }
+ resp, err := client.AssignRole(req)
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_RevokeRole(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========撤回角色==========")
+
+ req := model.AssignAndRevokeRoleRequest{
+ RoleCodes: []string{"NewCode"},
+ UserIds: []string{"615551a3dcdd486139a917b1"},
+ }
+ resp, err := client.RevokeRole(req)
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_ListRolePolicies(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========查询角色策略==========")
+
+ req := model.ListPoliciesRequest{
+ Code: "NewCode",
+ //Code: "rndyxyjuan",
+ }
+ resp, err := client.ListRolePolicies(req)
+ //resp, err := client.ListRolePolicies("rndyxyjuan", 1, 10)
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_AddRolePolicies(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========查询角色策略==========")
+ resp, err := client.AddRolePolicies("develop1234", []string{"ehsncbahxr"})
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_RemoveRolePolicies(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========查询角色策略==========")
+ resp, err := client.RemoveRolePolicies("develop1234", []string{"ehsncbahxr"})
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_ListRoleAuthorizedResources(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========查询角色被授权资源==========")
+ resp, err := client.ListRoleAuthorizedResources("NewCode", "default", model.EnumResourceTypeAPI)
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_GetRoleUdfValue(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========查询角色自定义字段==========")
+ resp, err := client.GetRoleSpecificUdfValue("61692d23d17aec55f4cfcfa6")
+ if err != nil {
+ fmt.Println(err)
+ }
+ fmt.Println((*resp)[0].Key)
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_BatchGetRoleUdfValue(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========批量查询角色自定义字段==========")
+ resp, err := client.BatchGetRoleUdfValue([]string{"61692d23d17aec55f4cfcfa6", "61386f82e3a0b1c8a5bd7491"})
+ if err != nil {
+ fmt.Println(err)
+ }
+ d := resp["61692d23d17aec55f4cfcfa6"]
+ fmt.Println(d[0].Key)
+ log.Printf("%+v\n", resp)
+
+}
+
+func TestClient_SetRoleUdfValue(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========设置角色自定义字段==========")
+ kv := &model.KeyValuePair{
+ Key: "school",
+ Value: "西财",
+ }
+ resp, err := client.SetRoleUdfValue("624298162086c052b6dc8e5f", kv)
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_BatchSetRoleUdfValue(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========批量设置角色自定义字段==========")
+ f := &model.SetUdfValueBatchInput{
+ Key: "lhucskosfr",
+ Value: "123",
+ TargetId: "616d112b7e387494d1ed0676",
+ }
+ tc := &model.SetUdfValueBatchInput{
+ Key: "lhucskosfr",
+ Value: "1235",
+ TargetId: "61692d23d17aec55f4cfcfa6",
+ }
+ param := []model.SetUdfValueBatchInput{*f, *tc}
+ resp, err := client.BatchSetRoleUdfValue(¶m)
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_RemoveRoleUdfValue(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========删除角色自定义字段==========")
+ resp, err := client.RemoveRoleUdfValue("61692d23d17aec55f4cfcfa6", "lhucskosfr")
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
diff --git a/lib/management/tenant_management_client.go b/lib/management/tenant_management_client.go
new file mode 100644
index 0000000..1462dc3
--- /dev/null
+++ b/lib/management/tenant_management_client.go
@@ -0,0 +1,486 @@
+package management
+
+import (
+ "errors"
+ "fmt"
+
+ // "github.com/Authing/authing-go-sdk/lib/constant"
+ "net/http"
+
+ "github.com/Authing/authing-go-sdk/lib/model"
+ jsoniter "github.com/json-iterator/go"
+)
+
+// GetTenantList
+// 获取用户池下租户列表
+func (c *Client) GetTenantList(request *model.CommonPageRequest) (*model.GetTenantListResponse, error) {
+
+ url := fmt.Sprintf("%s/api/v2/tenants?page=%v&limit=%v", c.Host, request.Page, request.Limit)
+ b, err := c.SendHttpRestRequest(url, http.MethodGet, nil)
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data model.GetTenantListResponse `json:"data"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return &resp.Data, nil
+}
+
+// GetTenantDetails
+// 获取租户详情
+func (c *Client) GetTenantDetails(tenantId string) (*model.TenantDetails, error) {
+
+ url := fmt.Sprintf("%s/api/v2/tenant/%s", c.Host, tenantId)
+ b, err := c.SendHttpRestRequest(url, http.MethodGet, nil)
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data model.TenantDetails `json:"data"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return &resp.Data, nil
+}
+
+// CreateTenant
+// 创建租户
+func (c *Client) CreateTenant(request *model.CreateTenantRequest) (*model.TenantDetails, error) {
+ data, _ := jsoniter.Marshal(request)
+ variables := make(map[string]interface{})
+ jsoniter.Unmarshal(data, &variables)
+
+ url := fmt.Sprintf("%s/api/v2/tenant", c.Host)
+ b, err := c.SendHttpRestRequest(url, http.MethodPost, variables)
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data model.TenantDetails `json:"data"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return &resp.Data, nil
+}
+
+// UpdateTenant
+// 修改租户
+func (c *Client) UpdateTenant(tenantId string, request *model.CreateTenantRequest) (bool, error) {
+
+ data, _ := jsoniter.Marshal(request)
+ variables := make(map[string]interface{})
+ jsoniter.Unmarshal(data, &variables)
+
+ url := fmt.Sprintf("%s/api/v2/tenant/%s", c.Host, tenantId)
+ b, err := c.SendHttpRestRequest(url, http.MethodPost, variables)
+ if err != nil {
+ return false, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data bool `json:"data"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return false, errors.New(resp.Message)
+ }
+ return true, nil
+}
+
+// RemoveTenant
+// 删除租户
+func (c *Client) RemoveTenant(tenantId string) (*string, error) {
+
+ url := fmt.Sprintf("%s/api/v2/tenant/%s", c.Host, tenantId)
+ b, err := c.SendHttpRestRequest(url, http.MethodDelete, nil)
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return &resp.Message, nil
+}
+
+// ConfigTenant
+// 配置租户品牌化
+func (c *Client) ConfigTenant(tenantId string, request *model.ConfigTenantRequest) (bool, error) {
+
+ data, _ := jsoniter.Marshal(request)
+ variables := make(map[string]interface{})
+ jsoniter.Unmarshal(data, &variables)
+
+ url := fmt.Sprintf("%s/api/v2/tenant/%s", c.Host, tenantId)
+ b, err := c.SendHttpRestRequest(url, http.MethodPost, variables)
+ if err != nil {
+ return false, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data bool `json:"data"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return resp.Data, errors.New(resp.Message)
+ }
+ return resp.Data, nil
+}
+
+// GetTenantMembers
+// 获取租户成员列表
+func (c *Client) GetTenantMembers(tenantId string, request *model.CommonPageRequest) (*model.TenantMembersResponse, error) {
+
+ url := fmt.Sprintf("%s/api/v2/tenant/%s/users?page=%v&limit=%v", c.Host, tenantId, request.Page, request.Limit)
+ b, err := c.SendHttpRestRequest(url, http.MethodGet, nil)
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data model.TenantMembersResponse `json:"data"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return &resp.Data, nil
+}
+
+// AddTenantMembers
+// 添加租户成员
+func (c *Client) AddTenantMembers(tenantId string, userIds []string) (*model.AddTenantMembersResponse, error) {
+
+ url := fmt.Sprintf("%s/api/v2/tenant/%s/user", c.Host, tenantId)
+ b, err := c.SendHttpRestRequest(url, http.MethodPost, map[string]interface{}{
+ "userIds": userIds,
+ })
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data model.AddTenantMembersResponse `json:"data"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return &resp.Data, nil
+}
+
+// RemoveTenantMembers
+// 删除租户成员
+func (c *Client) RemoveTenantMembers(tenantId string, userId string) (*string, error) {
+
+ url := fmt.Sprintf("%s/api/v2/tenant/%s/user?userId=%s", c.Host, tenantId, userId)
+ b, err := c.SendHttpRestRequest(url, http.MethodDelete, nil)
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return &resp.Message, nil
+}
+
+// ListExtIdp
+// 获取身份源列表
+func (c *Client) ListExtIdp(tenantId string) (*[]model.ListExtIdpResponse, error) {
+
+ url := fmt.Sprintf("%s/api/v2/extIdp?tenantId=%s", c.Host, tenantId)
+ b, err := c.SendHttpRestRequest(url, http.MethodGet, nil)
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data []model.ListExtIdpResponse `json:"data"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return &resp.Data, nil
+}
+
+// ExtIdpDetail
+// 获取身份源详细信息
+func (c *Client) ExtIdpDetail(extIdpId string) (*model.ExtIdpDetailResponse, error) {
+
+ url := fmt.Sprintf("%s/api/v2/extIdp/%s", c.Host, extIdpId)
+ b, err := c.SendHttpRestRequest(url, http.MethodGet, nil)
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data model.ExtIdpDetailResponse `json:"data"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return &resp.Data, nil
+}
+
+// CreateExtIdp
+// 创建身份源
+func (c *Client) CreateExtIdp(request *model.CreateExtIdpRequest) (*model.ExtIdpDetailResponse, error) {
+
+ data, _ := jsoniter.Marshal(request)
+ variables := make(map[string]interface{})
+ jsoniter.Unmarshal(data, &variables)
+
+ url := fmt.Sprintf("%s/api/v2/extIdp", c.Host)
+ b, err := c.SendHttpRestRequest(url, http.MethodPost, variables)
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data model.ExtIdpDetailResponse `json:"data"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return &resp.Data, nil
+}
+
+// UpdateExtIdp
+// 更新身份源
+func (c *Client) UpdateExtIdp(extIdpId string, request *model.UpdateExtIdpRequest) (*string, error) {
+
+ data, _ := jsoniter.Marshal(request)
+ variables := make(map[string]interface{})
+ jsoniter.Unmarshal(data, &variables)
+
+ url := fmt.Sprintf("%s/api/v2/extIdp/%v", c.Host, extIdpId)
+ b, err := c.SendHttpRestRequest(url, http.MethodPut, variables)
+
+ if err != nil {
+ return nil, err
+ }
+
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ }{}
+
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return &resp.Message, nil
+}
+
+// DeleteExtIdp
+// 删除身份源
+func (c *Client) DeleteExtIdp(extIdpId string) (*string, error) {
+
+ url := fmt.Sprintf("%s/api/v2/extIdp/%v", c.Host, extIdpId)
+ b, err := c.SendHttpRestRequest(url, http.MethodDelete, nil)
+
+ if err != nil {
+ return nil, err
+ }
+
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ }{}
+
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return &resp.Message, nil
+}
+
+// CreateExtIdpConnection
+// 创建身份源连接
+func (c *Client) CreateExtIdpConnection(request *model.CreateExtIdpConnectionRequest) (*model.ExtIdpConnectionDetails, error) {
+
+ data, _ := jsoniter.Marshal(request)
+ variables := make(map[string]interface{})
+ jsoniter.Unmarshal(data, &variables)
+
+ url := fmt.Sprintf("%s/api/v2/extIdpConn", c.Host)
+ b, err := c.SendHttpRestRequest(url, http.MethodPost, variables)
+
+ if err != nil {
+ return nil, err
+ }
+
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data model.ExtIdpConnectionDetails `json:"data"`
+ }{}
+
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return &resp.Data, nil
+}
+
+// UpdateExtIdpConnection
+// 更新身份源连接
+func (c *Client) UpdateExtIdpConnection(extIdpConnectionId string, request *model.UpdateExtIdpConnectionRequest) (*string, error) {
+
+ data, _ := jsoniter.Marshal(request)
+ variables := make(map[string]interface{})
+ jsoniter.Unmarshal(data, &variables)
+
+ url := fmt.Sprintf("%s/api/v2/extIdpConn/%v", c.Host, extIdpConnectionId)
+ b, err := c.SendHttpRestRequest(url, http.MethodPut, variables)
+
+ if err != nil {
+ return nil, err
+ }
+
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ }{}
+
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return &resp.Message, nil
+}
+
+// DeleteExtIdpConnection
+// 删除身份源连接
+func (c *Client) DeleteExtIdpConnection(extIdpConnectionId string) (*string, error) {
+
+ url := fmt.Sprintf("%s/api/v2/extIdpConn/%v", c.Host, extIdpConnectionId)
+ b, err := c.SendHttpRestRequest(url, http.MethodDelete, nil)
+
+ if err != nil {
+ return nil, err
+ }
+
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ }{}
+
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return &resp.Message, nil
+}
+
+// CheckExtIdpConnectionIdentifierUnique
+// 检查连接唯一标识是否冲突
+func (c *Client) CheckExtIdpConnectionIdentifierUnique(identifier string) (bool, error) {
+
+ url := fmt.Sprintf("%s/api/v2/check/extIdpConn/identifier", c.Host)
+ b, err := c.SendHttpRestRequest(url, http.MethodPost, map[string]interface{}{
+ "identifier": identifier,
+ })
+
+ if err != nil {
+ return true, err
+ }
+
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ }{}
+
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return true, errors.New(resp.Message)
+ }
+ return false, nil
+}
+
+// ChangeExtIdpConnectionState
+// 开关身份源连接
+func (c *Client) ChangeExtIdpConnectionState(extIdpConnectionId string, request *model.ChangeExtIdpConnectionStateRequest) (bool, error) {
+
+ data, _ := jsoniter.Marshal(request)
+ variables := make(map[string]interface{})
+ jsoniter.Unmarshal(data, &variables)
+
+ url := fmt.Sprintf("%s/api/v2/extIdpConn/%v/state", c.Host, extIdpConnectionId)
+ b, err := c.SendHttpRestRequest(url, http.MethodPut, variables)
+
+ if err != nil {
+ return false, err
+ }
+
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ }{}
+
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return false, errors.New(resp.Message)
+ }
+ return true, nil
+}
+
+// BatchChangeExtIdpConnectionState
+// 批量开关身份源连接
+func (c *Client) BatchChangeExtIdpConnectionState(extIdpId string, request *model.ChangeExtIdpConnectionStateRequest) (bool, error) {
+
+ data, _ := jsoniter.Marshal(request)
+ variables := make(map[string]interface{})
+ jsoniter.Unmarshal(data, &variables)
+
+ url := fmt.Sprintf("%s/api/v2/extIdp/%v/connState", c.Host, extIdpId)
+ b, err := c.SendHttpRestRequest(url, http.MethodPut, variables)
+
+ if err != nil {
+ return false, err
+ }
+
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ }{}
+
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return false, errors.New(resp.Message)
+ }
+ return true, nil
+}
diff --git a/lib/management/tenant_management_client_test.go b/lib/management/tenant_management_client_test.go
new file mode 100644
index 0000000..d44daac
--- /dev/null
+++ b/lib/management/tenant_management_client_test.go
@@ -0,0 +1,278 @@
+package management
+
+import (
+ "fmt"
+ "log"
+ "testing"
+
+ "github.com/Authing/authing-go-sdk/lib/model"
+)
+
+func TestClient_GetTenantList(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========获取用户池下租户列表==========")
+ resp, err := client.GetTenantList(&model.CommonPageRequest{
+ Page: 1,
+ Limit: 10,
+ })
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_GetTenantDetails(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========根据租户 ID 查询租户==========")
+ resp, err := client.GetTenantDetails("61b83950c110f5a2955221df")
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_CreateTenant(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========创建租户==========")
+ resp, err := client.CreateTenant(&model.CreateTenantRequest{
+ Name: "测试lnoi",
+ AppIds: "61503af19ddff2aa185b665a",
+ })
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_UpdateTenant(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========修改租户==========")
+ resp, err := client.UpdateTenant("61b95412098eb8dd16d5a7f4", &model.CreateTenantRequest{
+ Name: "测试 go 修改eve",
+ })
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_DeleteTenant(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========删除租户==========")
+ resp, err := client.RemoveTenant("61b95412098eb8dd16d5a7f4")
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_ConfigTenant(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========配置租户品牌化==========")
+ resp, err := client.ConfigTenant("61b83950c110f5a2955221df", &model.ConfigTenantRequest{
+ CSS: ".btnId {\n text-color: #ffff}",
+ SsoPageCustomizationSettings: &model.TenantSsoPageCustomizationSettings{
+ AutoRegisterThenLogin: false,
+ },
+ })
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_GetTenantMembers(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========获取租户成员列表==========")
+ resp, err := client.GetTenantMembers("61b83950c110f5a2955221df", &model.CommonPageRequest{
+ Page: 1,
+ Limit: 10,
+ })
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_AddTenantMembers(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========添加租户成员==========")
+ resp, err := client.AddTenantMembers("61b83950c110f5a2955221df", []string{"61b85b9da80ac34ac3a9451d", "61b85b945468e9865acae737"})
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_RemoveTenantMembers(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========删除租户成员==========")
+ resp, err := client.RemoveTenantMembers("61b83950c110f5a2955221df", "61b85b9da80ac34ac3a9451d")
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_ListExtIdp(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========获取身份源列表==========")
+ resp, err := client.ListExtIdp("61b83950c110f5a2955221df")
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_ExtIdpDetail(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========获取身份源==========")
+
+ resp, err := client.ExtIdpDetail("61b868aea25030db174529f1")
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_CreateExtIdp(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========创建身份源==========")
+ fields := map[string]string{
+ "displayName": "测试创建",
+ "baseURL": "https://gitlab.com/wfr",
+ "clientID": "everwew",
+ "clientSecret": "everwew",
+ }
+
+ resp, err := client.CreateExtIdp(&model.CreateExtIdpRequest{
+ Name: "GitLab",
+ Type: "gitlab",
+ TenantUd: "61b83950c110f5a2955221df",
+ Connections: []model.ExtIdpConnection{{
+ Identifier: "nboenboei",
+ Type: "gitlab",
+ DisplayName: "测试创建envoengoie",
+ Fields: fields,
+ }},
+ })
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_UpdateExtIdp(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========更新身份源==========")
+ resp, err := client.UpdateExtIdp("61b958a18a3f153bf3674e5b", &model.UpdateExtIdpRequest{
+ Name: "cscwecw",
+ })
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_DeleteExtIdp(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========删除身份源==========")
+ resp, err := client.DeleteExtIdp("61b958a18a3f153bf3674e5b")
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_CreateExtIdpConnection(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========创建身份源==========")
+ fields := map[string]string{
+ "displayName": "测试创建连接",
+ "baseURL": "https://gitlab.com/123456",
+ "clientID": "123456",
+ "clientSecret": "123456",
+ }
+
+ resp, err := client.CreateExtIdpConnection(&model.CreateExtIdpConnectionRequest{
+ ExtIdpId: "61b955fd8f70040602f8ebe4",
+ Identifier: "prmoroorobrnro",
+ Type: "gitlab",
+ DisplayName: "测试创建envoengoioi",
+ Fields: fields,
+ })
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_UpdateExtIdpConnection(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("=========更新身份源连接==========")
+ fields := map[string]string{
+ "displayName": "测试连接修改2",
+ "baseURL": "https://gitlab.com/123456",
+ "clientID": "123456",
+ "clientSecret": "123456",
+ }
+
+ resp, err := client.UpdateExtIdpConnection("61b9602bac8e32162db6d9d5", &model.UpdateExtIdpConnectionRequest{
+ DisplayName: "测试连接修改2",
+ Fields: fields,
+ })
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_DeleteExtIdpConnection(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========删除身份源连接==========")
+
+ resp, err := client.DeleteExtIdpConnection("61b9602bac8e32162db6d9d5")
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_CheckExtIdpConnectionIdentifierUnique(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========检查连接唯一标识是否冲突==========")
+
+ resp, err := client.CheckExtIdpConnectionIdentifierUnique("emoo")
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_ChangeExtIdpConnectionState(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========开关身份源连接==========")
+
+ resp, err := client.ChangeExtIdpConnectionState("61b868ae560f5e2ef2bd9e91", &model.ChangeExtIdpConnectionStateRequest{
+ Enabled: true,
+ TenantID: "61b83950c110f5a2955221df",
+ })
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_BatchChangeExtIdpConnectionState(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========批量开关身份源连接==========")
+
+ resp, err := client.BatchChangeExtIdpConnectionState("61b98798fab83706ed7f853f", &model.ChangeExtIdpConnectionStateRequest{
+ Enabled: false,
+ TenantID: "61b83950c110f5a2955221df",
+ })
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
diff --git a/lib/management/udf_management_client.go b/lib/management/udf_management_client.go
new file mode 100644
index 0000000..a52095b
--- /dev/null
+++ b/lib/management/udf_management_client.go
@@ -0,0 +1,132 @@
+package management
+
+import (
+ "errors"
+ "github.com/Authing/authing-go-sdk/lib/constant"
+ "github.com/Authing/authing-go-sdk/lib/model"
+ jsoniter "github.com/json-iterator/go"
+ "net/http"
+)
+
+// ListUdf
+// 获取自定义字段定义
+func (c *Client) ListUdf(targetType model.EnumUDFTargetType) (*[]model.UserDefinedField, error) {
+
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.ListUdfDocument,
+ map[string]interface{}{"targetType": targetType})
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ Udf []model.UserDefinedField `json:"udf"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.Udf, nil
+}
+
+// SetUdf
+// 设置自定义字段元数据
+func (c *Client) SetUdf(req *model.SetUdfInput) (*model.UserDefinedField, error) {
+ data, _ := jsoniter.Marshal(req)
+ vars := make(map[string]interface{})
+ jsoniter.Unmarshal(data, &vars)
+
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.SetUdfDocument, vars)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ SetUdf model.UserDefinedField `json:"setUdf"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.SetUdf, nil
+}
+
+// RemoveUdf
+// 删除自定义字段
+func (c *Client) RemoveUdf(targetType model.EnumUDFTargetType, key string) (*model.CommonMessageAndCode, error) {
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.RemoveUdfDocument, map[string]interface{}{
+ "targetType": targetType,
+ "key": key,
+ })
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ RemoveUdf model.CommonMessageAndCode `json:"removeUdf"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.RemoveUdf, nil
+}
+
+// ListUdfValue
+// 获取某一实体的自定义字段数据列表
+func (c *Client) ListUdfValue(targetType model.EnumUDFTargetType, targetId string) (*[]model.UserDefinedData, error) {
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.UdvDocument, map[string]interface{}{
+ "targetType": targetType,
+ "targetId": targetId,
+ })
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ Udv []model.UserDefinedData `json:"udv"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.Udv, nil
+}
+
+// SetUdvBatch
+// 批量添加自定义数据
+func (c *Client) SetUdvBatch(id string, targetType model.EnumUDFTargetType, udv *[]model.KeyValuePair) (*[]model.UserDefinedData, error) {
+ variables := make(map[string]interface{})
+
+ variables["targetType"] = targetType
+ variables["targetId"] = id
+ variables["udvList"] = udv
+
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.SetRoleUdfValueDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ SetUdvBatch []model.UserDefinedData `json:"setUdvBatch"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.SetUdvBatch, nil
+}
diff --git a/lib/management/udf_management_client_test.go b/lib/management/udf_management_client_test.go
new file mode 100644
index 0000000..b695fd7
--- /dev/null
+++ b/lib/management/udf_management_client_test.go
@@ -0,0 +1,69 @@
+package management
+
+import (
+ "fmt"
+ "github.com/Authing/authing-go-sdk/lib/model"
+ "log"
+ "testing"
+)
+
+func TestClient_ListUdf(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========自定义字段列表==========")
+ resp, err := client.ListUdf(model.EnumUDFTargetTypeUSER)
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_SetUdf(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========自定义字段列表==========")
+ req := &model.SetUdfInput{
+ TargetType: model.EnumUDFTargetTypeUSER,
+ DataType: model.EnumUDFDataTypeSTRING,
+ Key: "goSDK",
+ Label: "goSDK",
+ }
+ resp, err := client.SetUdf(req)
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_RemoveUdf(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========自定义字段列表==========")
+
+ resp, err := client.RemoveUdf(model.EnumUDFTargetTypeUSER, "goSDK")
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_ListUdfValue(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========某对象自定义字段列表==========")
+
+ resp, err := client.ListUdfValue(model.EnumUDFTargetTypeUSER, "616d41b7410a33da0cb70e65")
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_SetUdvBatch(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========某对象自定义字段列表==========")
+
+ resp, err := client.SetUdvBatch("616d41b7410a33da0cb70e65", model.EnumUDFTargetTypeUSER, &[]model.KeyValuePair{
+ {Key: "goSDK", Value: "goSDK"},
+ })
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
diff --git a/lib/management/user_management_client.go b/lib/management/user_management_client.go
new file mode 100644
index 0000000..aeb4455
--- /dev/null
+++ b/lib/management/user_management_client.go
@@ -0,0 +1,1007 @@
+package management
+
+import (
+ "encoding/json"
+ "errors"
+ "fmt"
+ "net/http"
+ "strconv"
+
+ "github.com/Authing/authing-go-sdk/lib/constant"
+ "github.com/Authing/authing-go-sdk/lib/model"
+ "github.com/Authing/authing-go-sdk/lib/util"
+ "github.com/bitly/go-simplejson"
+ jsoniter "github.com/json-iterator/go"
+)
+
+// Detail
+// 获取用户详情
+func (c *Client) Detail(userId string) (*model.User, error) {
+ b, err := c.SendHttpRequest(c.Host+"/api/v2/users/"+userId, constant.HttpMethodGet, "", nil)
+ if err != nil {
+ return nil, err
+ }
+ var userDetail model.UserDetailResponse
+ jsoniter.Unmarshal(b, &userDetail)
+ return &userDetail.Data, nil
+}
+
+// Detail
+// 获取用户详情
+func (c *Client) GetUserInfo(request model.QueryUserInfoRequest) (*model.User, error) {
+ url := c.Host + "/api/v2/users/" + request.UserId + "?with_custom_data=" + strconv.FormatBool(request.WithCustomData)
+ b, err := c.SendHttpRequest(url, constant.HttpMethodGet, "", nil)
+ if err != nil {
+ return nil, err
+ }
+ var userDetail model.UserDetailResponse
+ jsoniter.Unmarshal(b, &userDetail)
+ return &userDetail.Data, nil
+}
+
+// GetUserList
+// 获取用户列表
+func (c *Client) GetUserList(request model.QueryListRequest) (*model.PaginatedUsers, error) {
+ data, _ := json.Marshal(&request)
+ variables := make(map[string]interface{})
+ json.Unmarshal(data, &variables)
+ query := constant.UsersDocument
+ if request.WithCustomData != nil && *request.WithCustomData == true {
+ query = constant.UsersWithCustomDocument
+ }
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, constant.HttpMethodPost, query, variables)
+ if err != nil {
+ return nil, err
+ }
+ result := model.ListUserResponse{}
+ err = json.Unmarshal(b, &result)
+ if err != nil {
+ return nil, err
+ }
+ return &result.Data.Users, nil
+}
+
+// GetUserDepartments
+// 获取用户所在部门
+func (c *Client) GetUserDepartments(request model.GetUserDepartmentsRequest) (*model.PaginatedDepartments, error) {
+ data, _ := json.Marshal(&request)
+ variables := make(map[string]interface{})
+ json.Unmarshal(data, &variables)
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, constant.HttpMethodPost, constant.GetUserDepartmentsDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ result := model.GetUserDepartmentsResponse{}
+ err = json.Unmarshal(b, &result)
+ if err != nil {
+ return nil, err
+ }
+ return result.Data.User.Departments, nil
+}
+
+// CheckUserExists
+// 检查用户是否存在
+func (c *Client) CheckUserExists(request model.CheckUserExistsRequest) (bool, error) {
+ data, _ := json.Marshal(&request)
+ variables := make(map[string]interface{})
+ //json.Unmarshal(data, &variables)
+ jsoniter.Unmarshal(data, &variables)
+ b, err := c.SendHttpRequest(c.Host+"/api/v2/users/is-user-exists", constant.HttpMethodGet, constant.StringEmpty, variables)
+ result := model.CheckUserExistsResponse{}
+ err = json.Unmarshal(b, &result)
+ if err != nil {
+ return false, err
+ }
+ return result.Data, err
+}
+
+// CreateUser
+// 创建用户
+func (c *Client) CreateUser(request model.CreateUserRequest) (*model.User, error) {
+ if request.UserInfo.Password != nil {
+ pwd := util.RsaEncrypt(*request.UserInfo.Password)
+ request.UserInfo.Password = &pwd
+ }
+ data, _ := json.Marshal(&request)
+ variables := make(map[string]interface{})
+ json.Unmarshal(data, &variables)
+
+ query := constant.CreateUserDocument
+ if request.CustomData != nil {
+ query = constant.CreateUserWithCustomDataDocument
+ customData, _ := json.Marshal(&request.CustomData)
+ variables["params"] = customData
+ }
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, query, variables)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ CreateUser model.User `json:"createUser"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.CreateUser, nil
+}
+
+//UpdateUser
+//修改用户资料
+func (c *Client) UpdateUser(id string, updateInfo model.UpdateUserInput) (*model.User, error) {
+ if updateInfo.Password != nil {
+ pwd := util.RsaEncrypt(*updateInfo.Password)
+ updateInfo.Password = &pwd
+ }
+ variables := make(map[string]interface{})
+ variables["id"] = id
+ variables["input"] = updateInfo
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.UpdateUserDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ UpdateUser model.User `json:"updateUser"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.UpdateUser, nil
+}
+
+//DeleteUser
+//删除用户
+func (c *Client) DeleteUser(id string) (*model.CommonMessageAndCode, error) {
+
+ variables := make(map[string]interface{})
+ variables["id"] = id
+
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.DeleteUserDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ DeleteUser model.CommonMessageAndCode `json:"deleteUser"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.DeleteUser, nil
+}
+
+//BatchDeleteUser
+//批量删除用户
+func (c *Client) BatchDeleteUser(ids []string) (*model.CommonMessageAndCode, error) {
+ variables := make(map[string]interface{})
+ variables["ids"] = ids
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.BatchDeleteUserDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ DeleteUsers model.CommonMessageAndCode `json:"deleteUsers"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.DeleteUsers, nil
+}
+
+//BatchGetUser
+//通过 ID、username、email、phone、email、externalId 批量获取用户详情
+func (c *Client) BatchGetUser(ids []string, queryField string, withCustomData bool) (*[]model.User, error) {
+
+ variables := make(map[string]interface{})
+ variables["ids"] = ids
+ variables["type"] = queryField
+ query := constant.BatchGetUserDocument
+ if withCustomData {
+ query = constant.BatchGetUserWithCustomDocument
+ }
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, query, variables)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ BatchGetUsers []model.User `json:"userBatch"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.BatchGetUsers, nil
+}
+
+//ListArchivedUsers
+//获取已归档用户列表
+func (c *Client) ListArchivedUsers(request model.CommonPageRequest) (*model.CommonPageUsersResponse, error) {
+ data, _ := json.Marshal(&request)
+ variables := make(map[string]interface{})
+ json.Unmarshal(data, &variables)
+
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.ListArchivedUsersDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ ArchivedUsers model.CommonPageUsersResponse `json:"archivedUsers"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.ArchivedUsers, nil
+}
+
+//FindUser
+//查找用户
+func (c *Client) FindUser(request *model.FindUserRequest) (*model.User, error) {
+
+ data, _ := json.Marshal(&request)
+ variables := make(map[string]interface{})
+ json.Unmarshal(data, &variables)
+
+ query := constant.FindUserDocument
+ if request.WithCustomData {
+ query = constant.FindUserWithCustomDocument
+ }
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, query, variables)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ FindUser model.User `json:"findUser"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.FindUser, nil
+}
+
+//SearchUser
+//搜索用户
+func (c *Client) SearchUser(request *model.SearchUserRequest) (*model.CommonPageUsersResponse, error) {
+ if request.Page == 0 {
+ request.Page = 1
+ }
+ if request.Limit == 0 {
+ request.Limit = 10
+ }
+
+ data, _ := json.Marshal(&request)
+ variables := make(map[string]interface{})
+ json.Unmarshal(data, &variables)
+
+ query := constant.SearchUserDocument
+ if request.WithCustomData {
+ query = constant.SearchUserWithCustomDocument
+ }
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, query, variables)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ SearchUser model.CommonPageUsersResponse `json:"searchUser"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.SearchUser, nil
+}
+
+//RefreshUserToken
+//刷新用户 token
+func (c *Client) RefreshUserToken(userId string) (*model.RefreshToken, error) {
+ variables := make(map[string]interface{})
+ variables["id"] = userId
+
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.RefreshUserTokenDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ RefreshToken model.RefreshToken `json:"refreshToken"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.RefreshToken, nil
+}
+
+//GetUserGroups
+//获取用户分组列表
+func (c *Client) GetUserGroups(userId string) (*struct {
+ TotalCount int `json:"totalCount"`
+ List []model.GroupModel `json:"list"`
+}, error) {
+ variables := make(map[string]interface{})
+ variables["id"] = userId
+
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.GetUserGroupsDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ User model.GetUserGroupsResponse `json:"user"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.User.Groups, nil
+}
+
+//AddUserToGroup
+//将用户加入分组
+func (c *Client) AddUserToGroup(userId, groupCode string) (*model.CommonMessageAndCode, error) {
+
+ variables := make(map[string]interface{})
+ variables["userIds"] = []string{userId}
+ variables["code"] = groupCode
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.AddUserToGroupDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ AddUserToGroup model.CommonMessageAndCode `json:"addUserToGroup"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.AddUserToGroup, nil
+}
+
+//RemoveUserInGroup
+//将用户退出分组
+func (c *Client) RemoveUserInGroup(userId, groupCode string) (*model.CommonMessageAndCode, error) {
+
+ variables := make(map[string]interface{})
+ variables["userIds"] = []string{userId}
+ variables["code"] = groupCode
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.RemoveUserInGroupDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ RemoveUserFromGroup model.CommonMessageAndCode `json:"removeUserFromGroup"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.RemoveUserFromGroup, nil
+}
+
+//GetUserRoles
+//获取用户角色列表
+func (c *Client) GetUserRoles(request model.GetUserRolesRequest) (*struct {
+ TotalCount int `json:"totalCount"`
+ List []model.RoleModel `json:"list"`
+}, error) {
+
+ data, _ := json.Marshal(&request)
+ variables := make(map[string]interface{})
+ json.Unmarshal(data, &variables)
+
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.GetUserRolesDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ User model.GetUserRolesResponse `json:"user"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.User.Roles, nil
+}
+
+//AddUserToRoles
+//将用户加入角色
+func (c *Client) AddUserToRoles(request model.UserRoleOptRequest) (*model.CommonMessageAndCode, error) {
+ data, _ := jsoniter.Marshal(request)
+ variables := make(map[string]interface{})
+ jsoniter.Unmarshal(data, &variables)
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.AddUserToRoleDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ AssignRole model.CommonMessageAndCode `json:"assignRole"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.AssignRole, nil
+}
+
+//RemoveUserInRoles
+//将用户从角色中移除
+func (c *Client) RemoveUserInRoles(request model.UserRoleOptRequest) (*model.CommonMessageAndCode, error) {
+ data, _ := jsoniter.Marshal(request)
+ variables := make(map[string]interface{})
+ jsoniter.Unmarshal(data, &variables)
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.RemoveUserInRoleDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ RevokeRole model.CommonMessageAndCode `json:"revokeRole"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.RevokeRole, nil
+}
+
+//ListUserOrg
+//获取用户所在组织机构
+func (c *Client) ListUserOrg(userId string) (*[][]model.OrgModel, error) {
+
+ url := fmt.Sprintf("%v/api/v2/users/%v/orgs", c.Host, userId)
+ b, err := c.SendHttpRequest(url, http.MethodGet, "", nil)
+ if err != nil {
+ return nil, err
+ }
+
+ var response [][]model.OrgModel
+ var resultMap map[string]interface{}
+ e := jsoniter.Unmarshal(b, &resultMap)
+
+ if e != nil || resultMap["code"].(float64) != 200 {
+ return nil, errors.New("ListUserOrg Error")
+ }
+ data, _ := jsoniter.Marshal(resultMap["data"])
+ jsoniter.Unmarshal(data, &response)
+ return &response, nil
+}
+
+//GetUserUdfValue
+//获取某个用户的所有自定义数据
+func (c *Client) GetUserUdfValue(userId string) (*[]model.UserDefinedData, error) {
+ variables := make(map[string]interface{})
+
+ variables["targetType"] = constant.USER
+ variables["targetId"] = userId
+
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.GetRoleUdfValueDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ Udv []model.UserDefinedData `json:"udv"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.Udv, nil
+}
+
+// ListUserAuthorizedResources
+// 获取用户被授权的所有资源
+func (c *Client) ListUserAuthorizedResources(request model.ListUserAuthResourceRequest) (*model.AuthorizedResources, error) {
+
+ data, _ := jsoniter.Marshal(request)
+ variables := make(map[string]interface{})
+ jsoniter.Unmarshal(data, &variables)
+
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.ListUserAuthorizedResourcesDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ User struct {
+ AuthorizedResources model.AuthorizedResources `json:"authorizedResources"`
+ } `json:"user"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.User.AuthorizedResources, nil
+}
+
+// BatchGetUserUdfValue
+// 批量获取多个用户的自定义数据
+func (c *Client) BatchGetUserUdfValue(ids []string) (map[string][]model.UserDefinedData, error) {
+
+ variables := make(map[string]interface{})
+
+ variables["targetType"] = constant.USER
+ variables["targetIds"] = ids
+
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.BatchGetRoleUdfValueDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ UdfValueBatch []model.BatchRoleUdv `json:"udfValueBatch"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ resultMap := make(map[string][]model.UserDefinedData)
+ for _, v := range response.Data.UdfValueBatch {
+ resultMap[v.TargetId] = v.Data
+ }
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return resultMap, nil
+}
+
+// SetUserUdfValue
+// 设置某个用户的自定义数据
+func (c *Client) SetUserUdfValue(id string, udv *model.KeyValuePair) (*[]model.UserDefinedData, error) {
+
+ variables := make(map[string]interface{})
+
+ variables["targetType"] = constant.USER
+ variables["targetId"] = id
+ variables["key"] = udv.Key
+ v, _ := json.Marshal(udv.Value)
+ variables["value"] = string(v)
+
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.SetUdvDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ SetUdvBatch []model.UserDefinedData `json:"setUdv"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.SetUdvBatch, nil
+}
+
+// BatchSetUserUdfValue
+// 批量设置自定义数据
+func (c *Client) BatchSetUserUdfValue(request *[]model.SetUdfValueBatchInput) (*model.CommonMessageAndCode, error) {
+ variables := make(map[string]interface{})
+ input := make([]model.SetUdfValueBatchInput, 0)
+ for _, req := range *request {
+ v, _ := json.Marshal(&req.Value)
+ req.Value = string(v)
+ input = append(input, req)
+ }
+ variables["targetType"] = constant.USER
+ variables["input"] = input
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.BatchSetUdfValueDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ SetUdfValueBatch model.CommonMessageAndCode `json:"setUdfValueBatch"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.SetUdfValueBatch, nil
+}
+
+// RemoveUserUdfValue
+// 清除用户的自定义数据
+func (c *Client) RemoveUserUdfValue(id, key string) (*[]model.UserDefinedData, error) {
+
+ variables := make(map[string]interface{})
+ variables["targetType"] = constant.USER
+ variables["targetId"] = id
+ variables["key"] = key
+
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.RemoveUdfValueDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ RemoveUdv []model.UserDefinedData `json:"removeUdv"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.RemoveUdv, nil
+}
+
+// ListUserPolicies
+// 获取策略列表
+func (c *Client) ListUserPolicies(request model.ListPoliciesOnIdRequest) (*model.ListPoliciesResponse, error) {
+
+ if request.Page == 0 {
+ request.Page = 1
+ }
+ if request.Limit == 0 {
+ request.Limit = 10
+ }
+
+ data, _ := json.Marshal(&request)
+ variables := make(map[string]interface{})
+ json.Unmarshal(data, &variables)
+
+ variables["targetType"] = constant.USER
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.ListPoliciesDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ PolicyAssignments model.ListPoliciesResponse `json:"policyAssignments"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.PolicyAssignments, nil
+}
+
+// AddUserPolicies
+// 批量添加策略
+func (c *Client) AddUserPolicies(userId string, policiesCode []string) (*model.CommonMessageAndCode, error) {
+
+ variables := make(map[string]interface{})
+
+ variables["policies"] = policiesCode
+ variables["targetType"] = constant.USER
+ variables["targetIdentifiers"] = []string{userId}
+
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.AddPoliciesDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ AddPolicyAssignments model.CommonMessageAndCode `json:"addPolicyAssignments"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.AddPolicyAssignments, nil
+}
+
+// RemoveUserPolicies
+// 批量移除策略
+func (c *Client) RemoveUserPolicies(userId string, policiesCode []string) (*model.CommonMessageAndCode, error) {
+
+ variables := make(map[string]interface{})
+
+ variables["policies"] = policiesCode
+ variables["targetType"] = constant.USER
+ variables["targetIdentifiers"] = []string{userId}
+
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.RemovePoliciesDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ AddPolicyAssignments model.CommonMessageAndCode `json:"removePolicyAssignments"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.AddPolicyAssignments, nil
+}
+
+// UserHasRole
+// 判断用户是否有某个角色
+func (c *Client) UserHasRole(userId, roleCode, namespace string) (bool, error) {
+ req := model.GetUserRolesRequest{
+ Id: userId,
+ Namespace: namespace,
+ }
+ hasRole := false
+ list, err := c.GetUserRoles(req)
+ if err != nil {
+ return false, err
+ }
+ if list.TotalCount == 0 {
+ return false, nil
+ }
+ for _, v := range list.List {
+ if v.Code == roleCode {
+ hasRole = true
+ break
+ }
+ }
+ return hasRole, nil
+}
+
+//KickUser
+//强制一批用户下线
+func (c *Client) KickUser(userIds []string) (*model.CommonMessageAndCode, error) {
+
+ url := fmt.Sprintf("%v/api/v2/users/kick", c.Host)
+ json := make(map[string]interface{})
+ json["userIds"] = userIds
+ b, err := c.SendHttpRequest(url, http.MethodPost, "", json)
+ if err != nil {
+ return nil, err
+ }
+ var response model.CommonMessageAndCode
+ jsoniter.Unmarshal(b, &response)
+ return &response, nil
+}
+
+func (c *Client) ListAuthorizedResources(request model.ListAuthorizedResourcesByIdRequest) (*model.User, error) {
+ data, _ := json.Marshal(&request)
+ variables := make(map[string]interface{})
+ json.Unmarshal(data, &variables)
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, constant.HttpMethodPost, constant.ListUserAuthorizedResourcesDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ result := model.User{}
+ resultJson, err := simplejson.NewJson(b)
+ byteUser, err := resultJson.Get("data").Get("user").MarshalJSON()
+ err = json.Unmarshal(byteUser, &result)
+ if err != nil {
+ return nil, err
+ }
+ return &result, nil
+}
+
+func (c *Client) GetUserRoleList(request model.GetUserRoleListRequest) (*model.PaginatedRoles, error) {
+ variables := make(map[string]interface{}, 0)
+ if request.Namespace != nil {
+ variables["namespace"] = *request.Namespace
+ }
+ b, err := c.SendHttpRequest(c.Host+"/api/v2/users/"+request.UserId+"/roles", constant.HttpMethodGet, constant.StringEmpty, variables)
+ result := model.PaginatedRoles{}
+ resultJson, err := simplejson.NewJson(b)
+ byteUser, err := resultJson.Get("data").MarshalJSON()
+ err = json.Unmarshal(byteUser, &result)
+ if err != nil {
+ return nil, err
+ }
+ return &result, err
+}
+
+func (c *Client) GetUserGroupList(userId string) (*model.PaginatedGroups, error) {
+ variables := make(map[string]interface{}, 0)
+ variables["id"] = userId
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, constant.HttpMethodPost, constant.GetUserGroupsDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ result := model.PaginatedGroups{}
+ resultJson, err := simplejson.NewJson(b)
+ byteUser, err := resultJson.Get("data").Get("user").Get("groups").MarshalJSON()
+ err = json.Unmarshal(byteUser, &result)
+ if err != nil {
+ return nil, err
+ }
+ return &result, nil
+}
+
+//CheckLoginStatus
+//检查用户登录状态
+func (c *Client) CheckLoginStatus(userId string, appId, deviceId *string) (*model.CommonMessageAndCode, error) {
+ variables := make(map[string]interface{}, 0)
+ if appId != nil {
+ variables["appId"] = appId
+ }
+ if deviceId != nil {
+ variables["deviceId"] = deviceId
+ }
+ variables["userId"] = userId
+
+ url := fmt.Sprintf("%v/api/v2/users/login-status", c.Host)
+ b, err := c.SendHttpRequest(url, constant.HttpMethodGet, constant.StringEmpty, variables)
+ result := model.CommonMessageAndCode{}
+
+ err = json.Unmarshal(b, &result)
+ if err != nil {
+ return nil, err
+ }
+ return &result, err
+}
+
+//LogOut
+//用户退出
+func (c *Client) LogOut(userId string, appId *string) (*model.CommonMessageAndCode, error) {
+ variables := make(map[string]interface{}, 0)
+ if appId != nil {
+ variables["appId"] = appId
+ }
+
+ variables["userId"] = userId
+
+ url := fmt.Sprintf("%v/logout", c.Host)
+ b, err := c.SendHttpRequest(url, http.MethodGet, constant.StringEmpty, variables)
+ result := model.CommonMessageAndCode{}
+
+ err = json.Unmarshal(b, &result)
+ if err != nil {
+ return nil, err
+ }
+ return &result, err
+}
+
+// SendFirstLoginVerifyEmail
+// 发送首次登录验证邮件
+func (c *Client) SendFirstLoginVerifyEmail(userId, appId string) (*model.CommonMessageAndCode, error) {
+
+ variables := make(map[string]interface{})
+ variables["appId"] = appId
+ variables["userId"] = userId
+
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.SendFirstLoginVerifyEmailDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ SendFirstLoginVerifyEmail model.CommonMessageAndCode `json:"sendFirstLoginVerifyEmail"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.SendFirstLoginVerifyEmail, nil
+}
+
+// GetUserTenants
+// 获取用户所在租户
+func (c *Client) GetUserTenants(userId string) (*model.GetUserTenantsResponse, error) {
+
+ url := fmt.Sprintf("%s/api/v2/users/%v/tenants", c.Host, userId)
+ b, err := c.SendHttpRestRequest(url, http.MethodGet, nil)
+ if err != nil {
+ return nil, err
+ }
+
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data model.GetUserTenantsResponse `json:"data"`
+ }{}
+
+ jsoniter.Unmarshal(b, &resp)
+
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return &resp.Data, nil
+}
+
+//SuspendUser
+//停用账号
+func (c *Client) SuspendUser(userId string) (*model.CommonMessageAndCode, error) {
+ url := fmt.Sprintf("%v/api/v2/users/%v/suspend", c.Host, userId)
+ json := make(map[string]interface{})
+ b, err := c.SendHttpRequest(url, http.MethodPost, "", json)
+ if err != nil {
+ return nil, err
+ }
+ var response model.CommonMessageAndCode
+ jsoniter.Unmarshal(b, &response)
+ return &response, nil
+}
+
+//ActivateUser
+//解除停用账号
+func (c *Client) ActivateUser(userId string) (*model.CommonMessageAndCode, error) {
+ url := fmt.Sprintf("%v/api/v2/users/%v/activate", c.Host, userId)
+ json := make(map[string]interface{})
+ b, err := c.SendHttpRequest(url, http.MethodPost, "", json)
+ if err != nil {
+ return nil, err
+ }
+ var response model.CommonMessageAndCode
+ jsoniter.Unmarshal(b, &response)
+ return &response, nil
+}
+
+//ResignUser
+//离职用户
+func (c *Client) ResignUser(userId string) (*model.CommonMessageAndCode, error) {
+ url := fmt.Sprintf("%v/api/v2/users/%v/resign", c.Host, userId)
+ json := make(map[string]interface{})
+ b, err := c.SendHttpRequest(url, http.MethodPost, "", json)
+ if err != nil {
+ return nil, err
+ }
+ var response model.CommonMessageAndCode
+ jsoniter.Unmarshal(b, &response)
+ return &response, nil
+}
diff --git a/lib/management/user_management_client_test.go b/lib/management/user_management_client_test.go
new file mode 100644
index 0000000..9bd5045
--- /dev/null
+++ b/lib/management/user_management_client_test.go
@@ -0,0 +1,529 @@
+package management
+
+import (
+ "log"
+ "reflect"
+ "testing"
+
+ "github.com/Authing/authing-go-sdk/lib/model"
+)
+
+func TestClient_Detail(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========通过 ID 获取用户信息==========")
+ resp2, _ := client.Detail("618154277c11794e8cf63bc3")
+ log.Printf("%+v\n", resp2)
+}
+
+func TestClient_GetUserInfo(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========通过 ID 获取用户信息==========")
+ req := model.QueryUserInfoRequest{
+ UserId: "618154277c11794e8cf63bc3",
+ WithCustomData: false,
+ }
+ resp2, _ := client.GetUserInfo(req)
+ log.Printf("%+v\n", resp2)
+}
+
+func TestClient_GetUserList(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========导出所有组织机构数据==========")
+ //custom := true
+ req := model.QueryListRequest{
+ Page: 1,
+ Limit: 10,
+ }
+ resp, _ := client.GetUserList(req)
+ log.Printf("%+v\n", resp)
+ log.Println(*resp)
+}
+
+func TestClient_GetUserDepartments(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========获取用户部门列表==========")
+ req := model.GetUserDepartmentsRequest{
+ Id: "60e400c1701ea5b98dae628d",
+ OrgId: nil,
+ }
+ resp, _ := client.GetUserDepartments(req)
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_CheckUserExists(t *testing.T) {
+ // client := NewClient("62263a89959fb81af270caf0", "3c9eb5950608582d6ff17fd88e5c32dc", "http://localhost:3000")
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========检查用户是否存在==========")
+ email := "994004397@qq.com"
+ //phone := ""
+ req := model.CheckUserExistsRequest{
+ Email: &email,
+ }
+ resp, _ := client.CheckUserExists(req)
+ log.Println(resp)
+}
+
+func TestClient_CreateUser(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========创建用户==========")
+ //email := "t041gyqw0b@gmail.com"
+ phone := "15761403457222"
+ username := "xx"
+ pwd := "123456789"
+ var userInfo = &model.CreateUserInput{
+ Username: &username,
+ Phone: &phone,
+ Password: &pwd,
+ }
+ req := model.CreateUserRequest{
+ UserInfo: *userInfo,
+ }
+ resp, err := client.CreateUser(req)
+ log.Println(resp)
+ log.Println(err)
+}
+
+func TestClient_CreateUserWithCustom(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========创建用户包含自定义数据==========")
+ //email := "t041gyqw0b@gmail.com"
+ phone := "15761403457222122"
+ username := "xxqq12"
+ pwd := "123456789"
+ var userInfo = &model.CreateUserInput{
+ Username: &username,
+ Phone: &phone,
+ Password: &pwd,
+ }
+ req := model.CreateUserRequest{
+ UserInfo: *userInfo,
+ CustomData: []model.KeyValuePair{
+ model.KeyValuePair{
+ Key: "objhvfwdbi",
+ Value: "qq",
+ },
+ },
+ }
+ resp, err := client.CreateUser(req)
+ log.Println(resp)
+ log.Println(err)
+}
+
+func TestClient_UpdateUser(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========更新用户==========")
+ //email := "t041gyqw0b@gmail.com"
+ phone := "15761403457222122"
+ username := "xxqq123"
+ //pwd:="123456789"
+ var userInfo = &model.UpdateUserInput{
+ Username: &username,
+ Phone: &phone,
+ //Password: &pwd,
+ }
+
+ resp, err := client.UpdateUser("616d4333b809f9f4768db847", *userInfo)
+ log.Println(resp)
+ log.Println(err)
+}
+
+func TestClient_DeleteUser(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========删除用户==========")
+
+ resp, err := client.DeleteUser("616d57e96dfa54908eda326f")
+ log.Println(resp)
+ log.Println(err)
+}
+
+func TestClient_BatchDeleteUser(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========批量删除用户==========")
+
+ resp, err := client.BatchDeleteUser([]string{"616d430d58dbf82d1364453e"})
+ log.Println(resp)
+ log.Println(err)
+}
+
+func TestClient_BatchGetUser(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========批量获取用户==========")
+
+ resp, err := client.BatchGetUser([]string{"xxq", "xx"}, "username", true)
+ log.Println(resp)
+ log.Println(err)
+}
+
+func TestClient_ListArchivedUsers(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========获取归档用户==========")
+
+ resp, err := client.ListArchivedUsers(model.CommonPageRequest{
+ Page: 1,
+ Limit: 10,
+ })
+ log.Println(resp)
+ log.Println(err)
+}
+
+func TestClient_FindUser(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========查找用户==========")
+ userName := "xxqq"
+ resp, err := client.FindUser(&model.FindUserRequest{
+ Username: &userName,
+ })
+ log.Println(resp)
+ log.Println(err)
+}
+
+func TestClient_SearchUser(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========查找用户==========")
+
+ resp, err := client.SearchUser(&model.SearchUserRequest{
+ Query: "xxqq",
+ })
+ log.Println(resp)
+ log.Println(err)
+}
+
+func TestClient_UpdateUser2(t *testing.T) {
+ username := "111"
+ phone := "222"
+ var userInfo = &model.UpdateUserInput{
+ Username: &username,
+ Phone: &phone,
+ //Password: &pwd,
+ }
+ u := "U"
+ var defVal *string
+ defVal = &u
+ target := reflect.ValueOf(*userInfo)
+ rUsername := target.FieldByName("Username")
+ rIsVal := target.FieldByName("Gender")
+
+ defaultVal := reflect.ValueOf(&defVal)
+ log.Println(defaultVal.CanAddr())
+ rIsVal.Set(defaultVal)
+
+ log.Println(rUsername, rIsVal)
+ log.Println(*userInfo.Gender)
+}
+
+func TestClient_RefreshUserToken(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========刷新用户Token==========")
+
+ resp, err := client.RefreshUserToken("616d41b7410a33da0cb70e65")
+ log.Println(*resp)
+ log.Println(err)
+}
+
+func TestClient_GetUserGroups(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========获取用户所属组==========")
+
+ resp, err := client.GetUserGroups("616d41b7410a33da0cb70e65")
+ log.Println(resp)
+
+ for k, v := range resp.List {
+ log.Println(k)
+ log.Println(v)
+ }
+ log.Println(err)
+}
+
+func TestClient_AddUserToGroup(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========添加用户到组==========")
+
+ resp, err := client.AddUserToGroup("616d41b7410a33da0cb70e65", "pngrn")
+ log.Println(resp)
+ log.Println(err)
+}
+
+func TestClient_RemoveUserInGroup(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========移除组内用户==========")
+
+ resp, err := client.RemoveUserInGroup("616d41b7410a33da0cb70e65", "pngrn")
+ log.Println(resp)
+ log.Println(err)
+}
+
+func TestClient_AddUserToRoles(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========用户分配角色==========")
+ request := &model.UserRoleOptRequest{
+ UserIds: []string{"616d41b7410a33da0cb70e65"},
+ RoleCodes: []string{"wwqhd"},
+ }
+ resp, err := client.AddUserToRoles(*request)
+ log.Println(resp)
+ log.Println(err)
+}
+
+func TestClient_GetUserRoles(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========查询用户角色==========")
+ request := &model.GetUserRolesRequest{
+ Id: "616d41b7410a33da0cb70e65",
+ }
+ resp, err := client.GetUserRoles(*request)
+ log.Println(resp)
+ log.Println(err)
+}
+
+func TestClient_RemoveUserInRoles(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========撤回用户角色==========")
+ request := &model.UserRoleOptRequest{
+ UserIds: []string{"616d41b7410a33da0cb70e65"},
+ RoleCodes: []string{"wwqhd"},
+ }
+ resp, err := client.RemoveUserInRoles(*request)
+ log.Println(resp)
+ log.Println(err)
+}
+
+func TestClient_ListUserOrg(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========查询用户机构==========")
+ resp, err := client.ListUserOrg("616d41b7410a33da0cb70e65")
+ log.Println(resp)
+ log.Println(err)
+}
+
+func TestClient_GetUserUdfValue(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========查询用户自定义字段==========")
+ resp, err := client.GetUserUdfValue("616d41b7410a33da0cb70e65")
+ log.Println(resp)
+ log.Println(err)
+}
+
+func TestClient_ListUserAuthorizedResources(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========查询用户授权资源==========")
+
+ req := &model.ListUserAuthResourceRequest{
+ Id: "616d41b7410a33da0cb70e65",
+ Namespace: "default",
+ ResourceType: model.EnumResourceTypeAPI,
+ }
+ resp, err := client.ListUserAuthorizedResources(*req)
+ log.Println(resp)
+ log.Println(err)
+}
+
+func TestClient_BatchGetUserUdfValue(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========批量查询用户自定义字段==========")
+ resp, err := client.BatchGetUserUdfValue([]string{"621dcbede60e7b7eda97d82a"})
+ log.Println(resp)
+ log.Println(err)
+}
+
+func TestClient_SetUserUdfValue(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========批量查询用户自定义字段==========")
+ udv := model.KeyValuePair{
+ Key: "runCount",
+ Value: 23,
+ }
+ resp, err := client.SetUserUdfValue("621dcbede60e7b7eda97d82a", &udv)
+ log.Println(resp)
+ log.Println(err)
+}
+
+func TestClient_BatchSetUserUdfValue(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========批量设置用户自定义字段==========")
+ udvs := make([]model.SetUdfValueBatchInput, 0)
+ udv := model.SetUdfValueBatchInput{
+ TargetId: "621dcbede60e7b7eda97d82a",
+ Key: "school",
+ Value: "西财",
+ }
+ udv1 := model.SetUdfValueBatchInput{
+ TargetId: "621dcbede60e7b7eda97d82a",
+ Key: "student",
+ Value: true,
+ }
+ udvs = append(udvs, udv)
+ udvs = append(udvs, udv1)
+ resp, err := client.BatchSetUserUdfValue(&udvs)
+ log.Println(resp)
+ log.Println(err)
+}
+
+func TestClient_AddUserPolicies(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========授权用户策略==========")
+
+ resp, err := client.AddUserPolicies("616d41b7410a33da0cb70e65", []string{"ehsncbahxr"})
+ log.Println(resp)
+ log.Println(err)
+}
+
+func TestClient_ListUserPolicies(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========遍历用户策略==========")
+ req := model.ListPoliciesOnIdRequest{
+ Id: "616d41b7410a33da0cb70e65",
+ }
+ resp, err := client.ListUserPolicies(req)
+ log.Println(resp)
+ log.Println(err)
+}
+
+func TestClient_RemoveUserPolicies(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========撤销用户策略==========")
+
+ resp, err := client.RemoveUserPolicies("616d41b7410a33da0cb70e65", []string{"ehsncbahxr"})
+ log.Println(resp)
+ log.Println(err)
+}
+
+func TestClient_UserHasRole(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========判断用户是否具有角色==========")
+
+ resp, err := client.UserHasRole("616d41b7410a33da0cb70e65", "NewCode", "default")
+ log.Println(resp)
+ log.Println(err)
+}
+
+func TestClient_KickUser(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========强制用户下线==========")
+
+ resp, err := client.KickUser([]string{"5a597f35085a2000144a10ed"})
+ log.Println(resp)
+ log.Println(err)
+}
+
+func TestClient_ListAuthorizedResources(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========获取用户被授权的所有资源列表==========")
+
+ req := model.ListAuthorizedResourcesByIdRequest{
+ Id: "611b2ff477d701441c25e29e",
+ Namespace: "6123528118b7794b2420b311",
+ ResourceType: nil,
+ }
+ resp, _ := client.ListAuthorizedResources(req)
+ log.Printf("%+v\n", resp.AuthorizedResources)
+}
+
+func TestClient_GetUserRoleList(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========获取用户角色列表==========")
+ namespace := "default"
+ req := model.GetUserRoleListRequest{
+ UserId: "611a149db64310ca4764ab15",
+ Namespace: &namespace,
+ }
+ resp, _ := client.GetUserRoleList(req)
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_GetUserGroupList(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========获取用户分组列表==========")
+ resp, _ := client.GetUserGroupList("611a149db64310ca4764ab15")
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_CheckLoginStatus(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========检查用户登录状态==========")
+
+ resp, err := client.CheckLoginStatus("5a597f35085a2000144a10ed", nil, nil)
+ log.Println(resp)
+ log.Println(err)
+}
+
+func TestClient_LogOut(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========用户退出==========")
+
+ resp, err := client.LogOut("5a597f35085a2000144a10ed", nil)
+ log.Println(resp)
+ log.Println(err)
+}
+
+func TestClient_SendFirstLoginVerifyEmail(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========发送用户首次登录邮件==========")
+
+ resp, err := client.SendFirstLoginVerifyEmail("616d4333b809f9f4768db847", "6168f95e81d5e20f9cb72f22")
+ log.Println(resp)
+ log.Println(err)
+}
+
+func TestClient_CheckLoginStatus2(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========检验登录状态根据Token==========")
+ tx, e := GetAccessToken(client)
+ log.Println(tx, e)
+ resp, err := client.CheckLoginStatusByToken(tx)
+ log.Println(resp)
+ log.Println(err)
+}
+
+func TestClient_IsPasswordValid(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========检验登录状态根据Token==========")
+ tx, e := GetAccessToken(client)
+ log.Println(tx, e)
+ resp, err := client.IsPasswordValid("tx")
+ log.Println(resp)
+ log.Println(err)
+}
+
+func TestClient_GetUserTenants(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========获取用户所在租户==========")
+
+ resp, err := client.GetUserTenants("61b85b945468e9865acae737")
+ if err != nil {
+ log.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_SuspendUser(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========停用账号==========")
+
+ resp, err := client.SuspendUser("623946dd3615b3a2ee65832d")
+ if err != nil {
+ log.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_ActivateUser(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========停用账号==========")
+
+ resp, err := client.ActivateUser("623946dd3615b3a2ee65832d")
+ if err != nil {
+ log.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_ResignUser(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========停用账号==========")
+
+ resp, err := client.ResignUser("623946dd3615b3a2ee65832d")
+ if err != nil {
+ log.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
diff --git a/lib/management/user_pool_management_client.go b/lib/management/user_pool_management_client.go
new file mode 100644
index 0000000..e368dbf
--- /dev/null
+++ b/lib/management/user_pool_management_client.go
@@ -0,0 +1,117 @@
+package management
+
+import (
+ "errors"
+ "fmt"
+ "github.com/Authing/authing-go-sdk/lib/constant"
+ "github.com/Authing/authing-go-sdk/lib/model"
+ jsoniter "github.com/json-iterator/go"
+ "net/http"
+)
+
+// UserPoolDetail
+// 查询用户池配置
+func (c *Client) UserPoolDetail() (*model.UserPool, error) {
+
+ url := fmt.Sprintf("%s/api/v2/userpools/detail", c.Host)
+ b, err := c.SendHttpRestRequest(url, http.MethodGet, nil)
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data model.UserPool `json:"data"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return &resp.Data, nil
+}
+
+// UpdateUserPool
+// 更新用户池配置
+func (c *Client) UpdateUserPool(request model.UpdateUserpoolInput) (*model.UserPool, error) {
+ variables := make(map[string]interface{})
+ variables["input"] = request
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.UpdateUserPoolDocument, variables)
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ UpdateUserPool model.UserPool `json:"updateUserpool"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+
+ jsoniter.Unmarshal(b, &response)
+
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.UpdateUserPool, nil
+
+}
+
+// ListUserPoolEnv
+// 获取环境变量列表
+func (c *Client) ListUserPoolEnv() (*[]model.UserPoolEnv, error) {
+
+ url := fmt.Sprintf("%s/api/v2/env", c.Host)
+ b, err := c.SendHttpRestRequest(url, http.MethodGet, nil)
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data []model.UserPoolEnv `json:"data"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return &resp.Data, nil
+}
+
+// RemoveUserPoolEnv
+// 移除环境变量列表
+func (c *Client) RemoveUserPoolEnv(key string) (*model.CommonMessageAndCode, error) {
+
+ url := fmt.Sprintf("%s/api/v2/env/%s", c.Host, key)
+ b, err := c.SendHttpRestRequest(url, http.MethodDelete, nil)
+ if err != nil {
+ return nil, err
+ }
+ var resp model.CommonMessageAndCode
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return &resp, nil
+}
+
+// AddUserPoolEnv
+// 新增环境变量列表
+func (c *Client) AddUserPoolEnv(key, value string) (*model.UserPoolEnv, error) {
+
+ url := fmt.Sprintf("%s/api/v2/env", c.Host)
+ b, err := c.SendHttpRestRequest(url, http.MethodPost, map[string]interface{}{
+ "key": key, "value": value,
+ })
+ if err != nil {
+ return nil, err
+ }
+ resp := &struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data model.UserPoolEnv `json:"data"`
+ }{}
+ jsoniter.Unmarshal(b, &resp)
+ if resp.Code != 200 {
+ return nil, errors.New(resp.Message)
+ }
+ return &resp.Data, nil
+}
diff --git a/lib/management/user_pool_management_client_test.go b/lib/management/user_pool_management_client_test.go
new file mode 100644
index 0000000..6c8c3a9
--- /dev/null
+++ b/lib/management/user_pool_management_client_test.go
@@ -0,0 +1,49 @@
+package management
+
+import (
+ "fmt"
+ "github.com/Authing/authing-go-sdk/lib/model"
+ "log"
+ "testing"
+)
+
+func TestClient_UserPoolDetail(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========用户池详情==========")
+ resp, err := client.UserPoolDetail()
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_UpdateUserPool(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========修改用户池==========")
+ userPoolName := "otherSdk9989995"
+ req := &model.UpdateUserpoolInput{
+ Name: &userPoolName,
+ Domain: &userPoolName,
+ }
+ resp, err := client.UpdateUserPool(*req)
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_UserPoolEnv(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========用户池环境变量==========")
+
+ resp, err := client.ListUserPoolEnv()
+ if err != nil {
+ fmt.Println(err)
+ }
+ resp1, err1 := client.AddUserPoolEnv("qnm", "qnm")
+ fmt.Println(resp1, err1)
+ resp2, err2 := client.RemoveUserPoolEnv("qnm")
+ fmt.Println(resp2, err2)
+ resp, err = client.ListUserPoolEnv()
+ log.Printf("%+v\n", resp)
+}
diff --git a/lib/management/while_list_manangement_client.go b/lib/management/while_list_manangement_client.go
new file mode 100644
index 0000000..1fa591a
--- /dev/null
+++ b/lib/management/while_list_manangement_client.go
@@ -0,0 +1,142 @@
+package management
+
+import (
+ "errors"
+ "github.com/Authing/authing-go-sdk/lib/constant"
+ "github.com/Authing/authing-go-sdk/lib/model"
+ jsoniter "github.com/json-iterator/go"
+ "net/http"
+)
+
+//GetWhileList
+//获取白名单记录
+func (c *Client) GetWhileList(whileListType model.EnumWhitelistType) (*[]model.WhiteList, error) {
+
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.WhileListDocument, map[string]interface{}{
+ "type": whileListType,
+ })
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ WhileList []model.WhiteList `json:"whitelist"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.WhileList, nil
+}
+
+//AddWhileList
+//添加白名单记录
+func (c *Client) AddWhileList(whileListType model.EnumWhitelistType, ids []string) (*[]model.WhiteList, error) {
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.AddWhileListDocument, map[string]interface{}{
+ "type": whileListType,
+ "list": ids,
+ })
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ WhileList []model.WhiteList `json:"addWhitelist"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.WhileList, nil
+}
+
+//RemoveWhileList
+//移除白名单记录
+func (c *Client) RemoveWhileList(whileListType model.EnumWhitelistType, ids []string) (*[]model.WhiteList, error) {
+ b, err := c.SendHttpRequest(c.Host+constant.CoreAuthingGraphqlPath, http.MethodPost, constant.RemoveWhileListDocument, map[string]interface{}{
+ "type": whileListType,
+ "list": ids,
+ })
+ if err != nil {
+ return nil, err
+ }
+ var response = &struct {
+ Data struct {
+ WhileList []model.WhiteList `json:"removeWhitelist"`
+ } `json:"data"`
+ Errors []model.GqlCommonErrors `json:"errors"`
+ }{}
+ jsoniter.Unmarshal(b, &response)
+ if len(response.Errors) > 0 {
+ return nil, errors.New(response.Errors[0].Message.Message)
+ }
+ return &response.Data.WhileList, nil
+}
+
+//EnableWhileList
+//开启白名单
+func (c *Client) EnableWhileList(whileListType model.EnumWhitelistType) (*model.UserPool, error) {
+ var req model.UpdateUserpoolInput
+ enable := true
+ if whileListType == model.EnumWhitelistTypeUsername {
+ req = model.UpdateUserpoolInput{
+ Whitelist: &model.RegisterWhiteListConfigInput{
+ UsernameEnabled: &enable,
+ },
+ }
+ }
+
+ if whileListType == model.EnumWhitelistTypeEmail {
+ req = model.UpdateUserpoolInput{
+ Whitelist: &model.RegisterWhiteListConfigInput{
+ EmailEnabled: &enable,
+ },
+ }
+ }
+
+ if whileListType == model.EnumWhitelistTypePhone {
+ req = model.UpdateUserpoolInput{
+ Whitelist: &model.RegisterWhiteListConfigInput{
+ PhoneEnabled: &enable,
+ },
+ }
+ }
+ rep, err := c.UpdateUserPool(req)
+ return rep, err
+}
+
+//DisableWhileList
+//关闭白名单
+func (c *Client) DisableWhileList(whileListType model.EnumWhitelistType) (*model.UserPool, error) {
+ var req model.UpdateUserpoolInput
+ flag := false
+ if whileListType == model.EnumWhitelistTypeUsername {
+ req = model.UpdateUserpoolInput{
+ Whitelist: &model.RegisterWhiteListConfigInput{
+ UsernameEnabled: &flag,
+ },
+ }
+ }
+
+ if whileListType == model.EnumWhitelistTypeEmail {
+ req = model.UpdateUserpoolInput{
+ Whitelist: &model.RegisterWhiteListConfigInput{
+ EmailEnabled: &flag,
+ },
+ }
+ }
+
+ if whileListType == model.EnumWhitelistTypePhone {
+ req = model.UpdateUserpoolInput{
+ Whitelist: &model.RegisterWhiteListConfigInput{
+ PhoneEnabled: &flag,
+ },
+ }
+ }
+ rep, err := c.UpdateUserPool(req)
+ return rep, err
+}
diff --git a/lib/management/while_list_manangement_client_test.go b/lib/management/while_list_manangement_client_test.go
new file mode 100644
index 0000000..3fe86bc
--- /dev/null
+++ b/lib/management/while_list_manangement_client_test.go
@@ -0,0 +1,52 @@
+package management
+
+import (
+ "fmt"
+ "github.com/Authing/authing-go-sdk/lib/model"
+ "log"
+ "testing"
+)
+
+func TestClient_GetWhileList(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========获取白名单==========")
+
+ resp, err := client.GetWhileList(model.EnumWhitelistTypeUsername)
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_AddWhileList(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========获取白名单==========")
+
+ resp, err := client.AddWhileList(model.EnumWhitelistTypeUsername, []string{"qqxccx", "qweqwe"})
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_RemoveWhileList(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========移除白名单==========")
+
+ resp, err := client.RemoveWhileList(model.EnumWhitelistTypeUsername, []string{"qqxccx"})
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
+
+func TestClient_EnableWhileList(t *testing.T) {
+ client := NewClient(userPoolId, appSecret)
+ log.Println("==========移除白名单==========")
+
+ resp, err := client.EnableWhileList(model.EnumWhitelistTypeUsername)
+ if err != nil {
+ fmt.Println(err)
+ }
+ log.Printf("%+v\n", resp)
+}
diff --git a/lib/model/application_model.go b/lib/model/application_model.go
new file mode 100644
index 0000000..1f3103d
--- /dev/null
+++ b/lib/model/application_model.go
@@ -0,0 +1,226 @@
+package model
+
+import "time"
+
+type Application struct {
+ QrcodeScanning struct {
+ Redirect bool `json:"redirect"`
+ Interval int `json:"interval"`
+ } `json:"qrcodeScanning"`
+ Id string `json:"id"`
+ CreatedAt time.Time `json:"createdAt"`
+ UpdatedAt time.Time `json:"updatedAt"`
+ UserPoolId string `json:"userPoolId"`
+ Protocol string `json:"protocol"`
+ IsOfficial bool `json:"isOfficial"`
+ IsDeleted bool `json:"isDeleted"`
+ IsDefault bool `json:"isDefault"`
+ IsDemo bool `json:"isDemo"`
+ Name string `json:"name"`
+ Description string `json:"description"`
+ Secret string `json:"secret"`
+ Identifier string `json:"identifier"`
+ Jwks struct {
+ Keys []struct {
+ E string `json:"e"`
+ N string `json:"n"`
+ D string `json:"d"`
+ P string `json:"p"`
+ Q string `json:"q"`
+ Dp string `json:"dp"`
+ Dq string `json:"dq"`
+ Qi string `json:"qi"`
+ Kty string `json:"kty"`
+ Kid string `json:"kid"`
+ Alg string `json:"alg"`
+ Use string `json:"use"`
+ } `json:"keys"`
+ } `json:"jwks"`
+ SsoPageCustomizationSettings interface{} `json:"ssoPageCustomizationSettings"`
+ Logo string `json:"logo"`
+ RedirectUris []string `json:"redirectUris"`
+ LogoutRedirectUris []interface{} `json:"logoutRedirectUris"`
+ OidcProviderEnabled bool `json:"oidcProviderEnabled"`
+ OauthProviderEnabled bool `json:"oauthProviderEnabled"`
+ SamlProviderEnabled bool `json:"samlProviderEnabled"`
+ CasProviderEnabled bool `json:"casProviderEnabled"`
+ RegisterDisabled bool `json:"registerDisabled"`
+ LoginTabs []string `json:"loginTabs"`
+ PasswordTabConfig struct {
+ EnabledLoginMethods []string `json:"enabledLoginMethods"`
+ } `json:"passwordTabConfig"`
+ DefaultLoginTab string `json:"defaultLoginTab"`
+ RegisterTabs []string `json:"registerTabs"`
+ DefaultRegisterTab string `json:"defaultRegisterTab"`
+ LdapConnections interface{} `json:"ldapConnections"`
+ AdConnections []interface{} `json:"adConnections"`
+ DisabledSocialConnections interface{} `json:"disabledSocialConnections"`
+ DisabledOidcConnections []interface{} `json:"disabledOidcConnections"`
+ DisabledSamlConnections []interface{} `json:"disabledSamlConnections"`
+ DisabledOauth2Connections []interface{} `json:"disabledOauth2Connections"`
+ DisabledCasConnections []interface{} `json:"disabledCasConnections"`
+ DisabledAzureAdConnections []interface{} `json:"disabledAzureAdConnections"`
+ ExtendsFieldsEnabled bool `json:"extendsFieldsEnabled"`
+ ExtendsFields []interface{} `json:"extendsFields"`
+ Ext struct {
+ DontFinishNotYet bool `json:"_dontFinishNotYet"`
+ AppName string `json:"_appName"`
+ AliyunDomain string `json:"AliyunDomain"`
+ AliyunAccountId string `json:"AliyunAccountId"`
+ SamlConfig struct {
+ } `json:"samlConfig"`
+ } `json:"ext"`
+ Css interface{} `json:"css"`
+ OidcConfig struct {
+ GrantTypes []string `json:"grant_types"`
+ ResponseTypes []string `json:"response_types"`
+ IdTokenSignedResponseAlg string `json:"id_token_signed_response_alg"`
+ TokenEndpointAuthMethod string `json:"token_endpoint_auth_method"`
+ AuthorizationCodeExpire int `json:"authorization_code_expire"`
+ IdTokenExpire int `json:"id_token_expire"`
+ AccessTokenExpire int `json:"access_token_expire"`
+ RefreshTokenExpire int `json:"refresh_token_expire"`
+ CasExpire int `json:"cas_expire"`
+ SkipConsent bool `json:"skip_consent"`
+ RedirectUris []string `json:"redirect_uris"`
+ PostLogoutRedirectUris []interface{} `json:"post_logout_redirect_uris"`
+ ClientId string `json:"client_id"`
+ ClientSecret string `json:"client_secret"`
+ } `json:"oidcConfig"`
+ OidcJWEConfig interface{} `json:"oidcJWEConfig"`
+ SamlConfig struct {
+ Acs string `json:"acs"`
+ Audience string `json:"audience"`
+ Recipient string `json:"recipient"`
+ Destination string `json:"destination"`
+ Mappings interface{} `json:"mappings"`
+ DigestAlgorithm string `json:"digestAlgorithm"`
+ SignatureAlgorithm string `json:"signatureAlgorithm"`
+ AuthnContextClassRef string `json:"authnContextClassRef"`
+ LifetimeInSeconds int `json:"lifetimeInSeconds"`
+ SignResponse bool `json:"signResponse"`
+ NameIdentifierFormat string `json:"nameIdentifierFormat"`
+ SamlRequestSigningCert string `json:"samlRequestSigningCert"`
+ SamlResponseSigningCert string `json:"samlResponseSigningCert"`
+ SamlResponseSigningKey string `json:"samlResponseSigningKey"`
+ SamlResponseSigningCertFingerprint string `json:"samlResponseSigningCertFingerprint"`
+ EmailDomainSubstitution string `json:"emailDomainSubstitution"`
+ } `json:"samlConfig"`
+ OauthConfig interface{} `json:"oauthConfig"`
+ CasConfig interface{} `json:"casConfig"`
+ ShowAuthorizationPage bool `json:"showAuthorizationPage"`
+ EnableSubAccount bool `json:"enableSubAccount"`
+ EnableDeviceMutualExclusion bool `json:"enableDeviceMutualExclusion"`
+ LoginRequireEmailVerified bool `json:"loginRequireEmailVerified"`
+ AgreementEnabled bool `json:"agreementEnabled"`
+ IsIntegrate bool `json:"isIntegrate"`
+ SsoEnabled bool `json:"ssoEnabled"`
+ Template string `json:"template"`
+ SkipMfa bool `json:"skipMfa"`
+ CasExpireBaseBrowser bool `json:"casExpireBaseBrowser"`
+ PermissionStrategy struct {
+ Enabled bool `json:"enabled"`
+ DefaultStrategy string `json:"defaultStrategy"`
+ AllowPolicyId interface{} `json:"allowPolicyId"`
+ DenyPolicyId interface{} `json:"denyPolicyId"`
+ } `json:"permissionStrategy"`
+}
+
+type ApplicationActiveUsers struct {
+ ThirdPartyIdentity struct {
+ Provider string `json:"provider"`
+ RefreshToken string `json:"refreshToken"`
+ AccessToken string `json:"accessToken"`
+ Scope string `json:"scope"`
+ ExpiresIn string `json:"expiresIn"`
+ UpdatedAt string `json:"updatedAt"`
+ } `json:"thirdPartyIdentity"`
+ Id string `json:"id"`
+ CreatedAt time.Time `json:"createdAt"`
+ UpdatedAt time.Time `json:"updatedAt"`
+ UserPoolId string `json:"userPoolId"`
+ IsRoot bool `json:"isRoot"`
+ Status string `json:"status"`
+ Oauth string `json:"oauth"`
+ Email string `json:"email"`
+ Phone string `json:"phone"`
+ Username string `json:"username"`
+ Unionid string `json:"unionid"`
+ Openid string `json:"openid"`
+ Nickname string `json:"nickname"`
+ Company string `json:"company"`
+ Photo string `json:"photo"`
+ Browser string `json:"browser"`
+ Device string `json:"device"`
+ Password string `json:"password"`
+ Salt string `json:"salt"`
+ LoginsCount int `json:"loginsCount"`
+ LastIp string `json:"lastIp"`
+ Name string `json:"name"`
+ GivenName string `json:"givenName"`
+ FamilyName string `json:"familyName"`
+ MiddleName string `json:"middleName"`
+ Profile string `json:"profile"`
+ PreferredUsername string `json:"preferredUsername"`
+ Website string `json:"website"`
+ Gender string `json:"gender"`
+ Birthdate string `json:"birthdate"`
+ Zoneinfo string `json:"zoneinfo"`
+ Locale string `json:"locale"`
+ Address string `json:"address"`
+ Formatted string `json:"formatted"`
+ StreetAddress string `json:"streetAddress"`
+ Locality string `json:"locality"`
+ Region string `json:"region"`
+ PostalCode string `json:"postalCode"`
+ City string `json:"city"`
+ Province string `json:"province"`
+ Country string `json:"country"`
+ RegisterSource []string `json:"registerSource"`
+ SecretInfo interface{} `json:"secretInfo"`
+ EmailVerified bool `json:"emailVerified"`
+ PhoneVerified bool `json:"phoneVerified"`
+ LastLogin time.Time `json:"lastLogin"`
+ Blocked bool `json:"blocked"`
+ IsDeleted bool `json:"isDeleted"`
+ SendSmsCount int `json:"sendSmsCount"`
+ SendSmsLimitCount int `json:"sendSmsLimitCount"`
+ DataVersion string `json:"dataVersion"`
+ EncryptedPassword string `json:"encryptedPassword"`
+ SignedUp time.Time `json:"signedUp"`
+ ExternalId string `json:"externalId"`
+ MainDepartmentId string `json:"mainDepartmentId"`
+ MainDepartmentCode string `json:"mainDepartmentCode"`
+ LastMfaTime string `json:"lastMfaTime"`
+ PasswordSecurityLevel int `json:"passwordSecurityLevel"`
+ ResetPasswordOnFirstLogin bool `json:"resetPasswordOnFirstLogin"`
+ SyncExtInfo interface{} `json:"syncExtInfo"`
+ PhoneCountryCode string `json:"phoneCountryCode"`
+ Source interface{} `json:"source"`
+ LastIP string `json:"lastIP"`
+ Token string `json:"token"`
+ TokenExpiredAt time.Time `json:"tokenExpiredAt"`
+}
+
+type ApplicationAgreement struct {
+ UserPoolId string `json:"userPoolId"`
+ AppId string `json:"appId"`
+ Title string `json:"title"`
+ Lang string `json:"lang"`
+ Required bool `json:"required"`
+ Order int `json:"order"`
+ Id int `json:"id"`
+}
+
+type ApplicationTenantDetails struct {
+ ID string `json:"id"`
+ Name string `json:"name"`
+ Logo string `json:"logo"`
+ Domain string `json:"domain"`
+ Description string `json:"description"`
+ CreatedAt time.Time `json:"createdAt"`
+ UpdatedAt time.Time `json:"updatedAt"`
+ Protocol string `json:"protocol"`
+ IsIntegrate bool `json:"isIntegrate"`
+ Tenants []Tenant `json:"tenants"`
+}
diff --git a/lib/model/group_model.go b/lib/model/group_model.go
new file mode 100644
index 0000000..e633d1a
--- /dev/null
+++ b/lib/model/group_model.go
@@ -0,0 +1,31 @@
+package model
+
+import "time"
+
+type GroupModel struct {
+ Code string `json:"code"`
+ Name string `json:"name"`
+ Description string `json:"description"`
+ CreatedAt time.Time `json:"createdAt"`
+ UpdatedAt time.Time `json:"updatedAt"`
+}
+
+type CreateGroupsRequest struct {
+ Code string `json:"code"`
+ Name string `json:"name"`
+ Description *string `json:"description,omitempty"`
+}
+
+type UpdateGroupsRequest struct {
+ Code string `json:"code"`
+ NewCode *string `json:"newCode,omitempty"`
+ Name *string `json:"name,omitempty"`
+ Description *string `json:"description,omitempty"`
+}
+
+type GetGroupUserResponse struct {
+ Users struct {
+ TotalCount int `json:"totalCount"`
+ List []User `json:"list"`
+ } `json:"users"`
+}
diff --git a/lib/model/mfa_model.go b/lib/model/mfa_model.go
new file mode 100644
index 0000000..13947fe
--- /dev/null
+++ b/lib/model/mfa_model.go
@@ -0,0 +1,39 @@
+package model
+
+import (
+ "github.com/Authing/authing-go-sdk/lib/constant"
+ "time"
+)
+
+type MfaInput struct {
+ MfaToken *string
+ MfaType *string `json:"type"`
+ MfaSource *constant.MfaSource `json:"source"`
+}
+
+type GetMfaAuthenticatorsResponse struct {
+ Id string `json:"id"`
+ CreatedAt time.Time `json:"createdAt"`
+ UpdatedAt time.Time `json:"updatedAt"`
+ UserId string `json:"userId"`
+ Enable bool `json:"enable"`
+ Secret string `json:"secret"`
+ AuthenticatorType string `json:"authenticatorType"`
+ RecoveryCode string `json:"recoveryCode"`
+ Source string `json:"source"`
+}
+
+type AssociateMfaAuthenticatorResponse struct {
+ AuthenticatorType string `json:"authenticator_type"`
+ Secret string `json:"secret"`
+ QrcodeUri string `json:"qrcode_uri"`
+ QrcodeDataUrl string `json:"qrcode_data_url"`
+ RecoveryCode string `json:"recovery_code"`
+}
+
+type ConfirmAssociateMfaAuthenticatorRequest struct {
+ Totp string `json:"totp"`
+ AuthenticatorType *string `json:"authenticatorType"`
+ MfaSource *constant.MfaSource `json:"source"`
+ MfaToken *string
+}
diff --git a/lib/model/models.go b/lib/model/models.go
new file mode 100644
index 0000000..401071a
--- /dev/null
+++ b/lib/model/models.go
@@ -0,0 +1,797 @@
+// Code generated by go generate; DO NOT EDIT.
+// This file was generated from GraphQL schema
+
+package model
+
+import "time"
+
+type EnumEmailTemplateType string
+
+const EnumEmailTemplateTypeResetPassword EnumEmailTemplateType = "RESET_PASSWORD"
+const EnumEmailTemplateTypePasswordResetedNotification EnumEmailTemplateType = "PASSWORD_RESETED_NOTIFICATION"
+const EnumEmailTemplateTypeChangePassword EnumEmailTemplateType = "CHANGE_PASSWORD"
+const EnumEmailTemplateTypeWelcome EnumEmailTemplateType = "WELCOME"
+const EnumEmailTemplateTypeVerifyEmail EnumEmailTemplateType = "VERIFY_EMAIL"
+const EnumEmailTemplateTypeChangeEmail EnumEmailTemplateType = "CHANGE_EMAIL"
+
+type EnumResourceType string
+
+const EnumResourceTypeDATA EnumResourceType = "DATA"
+const EnumResourceTypeAPI EnumResourceType = "API"
+const EnumResourceTypeMENU EnumResourceType = "MENU"
+const EnumResourceTypeUI EnumResourceType = "UI"
+const EnumResourceTypeBUTTON EnumResourceType = "BUTTON"
+
+type EnumSortByEnum string
+
+const EnumSortByEnumCREATEDAT_DESC EnumSortByEnum = "CREATEDAT_DESC"
+const EnumSortByEnumCREATEDAT_ASC EnumSortByEnum = "CREATEDAT_ASC"
+const EnumSortByEnumUPDATEDAT_DESC EnumSortByEnum = "UPDATEDAT_DESC"
+const EnumSortByEnumUPDATEDAT_ASC EnumSortByEnum = "UPDATEDAT_ASC"
+
+type EnumUserStatus string
+
+const EnumUserStatusSuspended EnumUserStatus = "Suspended"
+const EnumUserStatusResigned EnumUserStatus = "Resigned"
+const EnumUserStatusActivated EnumUserStatus = "Activated"
+const EnumUserStatusArchived EnumUserStatus = "Archived"
+
+type Enum__TypeKind string
+
+const TypeKindScalar Enum__TypeKind = "SCALAR"
+const TypeKindObject Enum__TypeKind = "OBJECT"
+const TypeKindInterface Enum__TypeKind = "INTERFACE"
+const TypeKindUnion Enum__TypeKind = "UNION"
+const TypeKindEnum Enum__TypeKind = "ENUM"
+const TypeKindInputObject Enum__TypeKind = "INPUT_OBJECT"
+const TypeKindList Enum__TypeKind = "LIST"
+const TypeKindNonNull Enum__TypeKind = "NON_NULL"
+
+type EnumEmailScene string
+
+const EnumEmailSceneResetPassword EnumEmailScene = "RESET_PASSWORD"
+const EnumEmailSceneVerifyEmail EnumEmailScene = "VERIFY_EMAIL"
+const EnumEmailSceneChangeEmail EnumEmailScene = "CHANGE_EMAIL"
+const EnumEmailSceneMfaVerify EnumEmailScene = "MFA_VERIFY"
+
+type EnumOperator string
+
+const EnumOperatorAnd EnumOperator = "AND"
+const EnumOperatorOr EnumOperator = "OR"
+
+type EnumPolicyAssignmentTargetType string
+
+const EnumPolicyAssignmentTargetTypeUser EnumPolicyAssignmentTargetType = "USER"
+const EnumPolicyAssignmentTargetTypeRole EnumPolicyAssignmentTargetType = "ROLE"
+const EnumPolicyAssignmentTargetTypeGroup EnumPolicyAssignmentTargetType = "GROUP"
+const EnumPolicyAssignmentTargetTypeOrg EnumPolicyAssignmentTargetType = "ORG"
+const EnumPolicyAssignmentTargetTypeAkSk EnumPolicyAssignmentTargetType = "AK_SK"
+
+type EnumPolicyEffect string
+
+const EnumPolicyEffectAllow EnumPolicyEffect = "ALLOW"
+const EnumPolicyEffectDeny EnumPolicyEffect = "DENY"
+
+type EnumUDFDataType string
+
+const EnumUDFDataTypeSTRING EnumUDFDataType = "STRING"
+const EnumUDFDataTypeNUMBER EnumUDFDataType = "NUMBER"
+const EnumUDFDataTypeDATETIME EnumUDFDataType = "DATETIME"
+const EnumUDFDataTypeBOOLEAN EnumUDFDataType = "BOOLEAN"
+const EnumUDFDataTypeOBJECT EnumUDFDataType = "OBJECT"
+
+type EnumUDFTargetType string
+
+const EnumUDFTargetTypeNODE EnumUDFTargetType = "NODE"
+const EnumUDFTargetTypeORG EnumUDFTargetType = "ORG"
+const EnumUDFTargetTypeUSER EnumUDFTargetType = "USER"
+const EnumUDFTargetTypeUSERPOOL EnumUDFTargetType = "USERPOOL"
+const EnumUDFTargetTypeROLE EnumUDFTargetType = "ROLE"
+const EnumUDFTargetTypePERMISSION EnumUDFTargetType = "PERMISSION"
+const EnumUDFTargetTypeAPPLICATION EnumUDFTargetType = "APPLICATION"
+
+type EnumWhitelistType string
+
+const EnumWhitelistTypeUsername EnumWhitelistType = "USERNAME"
+const EnumWhitelistTypeEmail EnumWhitelistType = "EMAIL"
+const EnumWhitelistTypePhone EnumWhitelistType = "PHONE"
+
+type Enum__DirectiveLocation string
+
+const DirectiveLocationQuery Enum__DirectiveLocation = "QUERY"
+const DirectiveLocationMutation Enum__DirectiveLocation = "MUTATION"
+const DirectiveLocationSubscription Enum__DirectiveLocation = "SUBSCRIPTION"
+const DirectiveLocationField Enum__DirectiveLocation = "FIELD"
+const DirectiveLocationFragmentDefinition Enum__DirectiveLocation = "FRAGMENT_DEFINITION"
+const DirectiveLocationFragmentSpread Enum__DirectiveLocation = "FRAGMENT_SPREAD"
+const DirectiveLocationInlineFragment Enum__DirectiveLocation = "INLINE_FRAGMENT"
+const DirectiveLocationVariableDefinition Enum__DirectiveLocation = "VARIABLE_DEFINITION"
+const DirectiveLocationSchema Enum__DirectiveLocation = "SCHEMA"
+const DirectiveLocationScalar Enum__DirectiveLocation = "SCALAR"
+const DirectiveLocationObject Enum__DirectiveLocation = "OBJECT"
+const DirectiveLocationFieldDefinition Enum__DirectiveLocation = "FIELD_DEFINITION"
+const DirectiveLocationArgumentDefinition Enum__DirectiveLocation = "ARGUMENT_DEFINITION"
+const DirectiveLocationInterface Enum__DirectiveLocation = "INTERFACE"
+const DirectiveLocationUnion Enum__DirectiveLocation = "UNION"
+const DirectiveLocationEnum Enum__DirectiveLocation = "ENUM"
+const DirectiveLocationEnumValue Enum__DirectiveLocation = "ENUM_VALUE"
+const DirectiveLocationInputObject Enum__DirectiveLocation = "INPUT_OBJECT"
+const DirectiveLocationInputFieldDefinition Enum__DirectiveLocation = "INPUT_FIELD_DEFINITION"
+
+type __Schema struct {
+ Types []__Type `json:"types"`
+ QueryType __Type `json:"queryType"`
+ MutationType *__Type `json:"mutationType"`
+ SubscriptionType *__Type `json:"subscriptionType"`
+ Directives []__Directive `json:"directives"`
+}
+
+type __Type struct {
+ Kind Enum__TypeKind `json:"kind"`
+ Name *string `json:"name"`
+ Description *string `json:"description"`
+ Fields []__Field `json:"fields"`
+ Interfaces []__Type `json:"interfaces"`
+ PossibleTypes []__Type `json:"possibleTypes"`
+ EnumValues []__EnumValue `json:"enumValues"`
+ InputFields []__InputValue `json:"inputFields"`
+ OfType *__Type `json:"ofType"`
+}
+
+type __Field struct {
+ Name string `json:"name"`
+ Description *string `json:"description"`
+ Args []__InputValue `json:"args"`
+ Type __Type `json:"type"`
+ IsDeprecated bool `json:"isDeprecated"`
+ DeprecationReason *string `json:"deprecationReason"`
+}
+
+type __InputValue struct {
+ Name string `json:"name"`
+ Description *string `json:"description"`
+ Type __Type `json:"type"`
+ DefaultValue *string `json:"defaultValue"`
+}
+
+type __EnumValue struct {
+ Name string `json:"name"`
+ Description *string `json:"description"`
+ IsDeprecated bool `json:"isDeprecated"`
+ DeprecationReason *string `json:"deprecationReason"`
+}
+
+type __Directive struct {
+ Name string `json:"name"`
+ Description *string `json:"description"`
+ Locations []Enum__DirectiveLocation `json:"locations"`
+ Args []__InputValue `json:"args"`
+ IsRepeatable bool `json:"isRepeatable"`
+}
+
+type AccessTokenRes struct {
+ AccessToken *string `json:"accessToken"`
+ Exp *int64 `json:"exp"`
+ Iat *int64 `json:"iat"`
+}
+
+type App2WxappLoginStrategy struct {
+ TicketExpriresAfter *int64 `json:"ticketExpriresAfter"`
+ TicketExchangeUserInfoNeedSecret *bool `json:"ticketExchangeUserInfoNeedSecret"`
+}
+
+type App2WxappLoginStrategyInput struct {
+ TicketExpriresAfter *int64 `json:"ticketExpriresAfter,omitempty"`
+ TicketExchangeUserInfoNeedSecret *bool `json:"ticketExchangeUserInfoNeedSecret,omitempty"`
+}
+
+type AuthorizedResource struct {
+ Code string `json:"code"`
+ Type *EnumResourceType `json:"type"`
+ Actions []string `json:"actions"`
+}
+
+type AuthorizedTargetsActionsInput struct {
+ Op EnumOperator `json:"op"`
+ List []*string `json:"list"`
+}
+
+type AuthorizeResourceOpt struct {
+ TargetType EnumPolicyAssignmentTargetType `json:"targetType"`
+ TargetIdentifier string `json:"targetIdentifier"`
+ Actions []string `json:"actions"`
+}
+
+type BatchOperationResult struct {
+ SucceedCount int64 `json:"succeedCount"`
+ FailedCount int64 `json:"failedCount"`
+ Message *string `json:"message"`
+ Errors []string `json:"errors"`
+}
+
+type ChangeEmailStrategy struct {
+ VerifyOldEmail *bool `json:"verifyOldEmail,omitempty"`
+}
+
+type ChangeEmailStrategyInput struct {
+ VerifyOldEmail *bool `json:"verifyOldEmail,omitempty"`
+}
+
+type ChangePhoneStrategy struct {
+ VerifyOldPhone *bool `json:"verifyOldPhone,omitempty"`
+}
+
+type ChangePhoneStrategyInput struct {
+ VerifyOldPhone *bool `json:"verifyOldPhone,omitempty"`
+}
+
+type CheckPasswordStrengthResult struct {
+ Valid bool `json:"valid"`
+ Message *string `json:"message"`
+}
+
+type CommonMessage struct {
+ Message *string `json:"message"`
+ Code *int64 `json:"code"`
+}
+
+type ConfigEmailTemplateInput struct {
+ Type EnumEmailTemplateType `json:"type"`
+ Name string `json:"name"`
+ Subject string `json:"subject"`
+ Sender string `json:"sender"`
+ Content string `json:"content"`
+ RedirectTo *string `json:"redirectTo"`
+ HasURL *bool `json:"hasURL"`
+ ExpiresIn *int64 `json:"expiresIn"`
+}
+
+type CreateFunctionInput struct {
+ Name string `json:"name"`
+ SourceCode string `json:"sourceCode"`
+ Description *string `json:"description"`
+ Url *string `json:"url"`
+}
+
+type CreateSocialConnectionInput struct {
+ Provider string `json:"provider"`
+ Name string `json:"name"`
+ Logo string `json:"logo"`
+ Description *string `json:"description"`
+ Fields []SocialConnectionFieldInput `json:"fields"`
+}
+
+type CreateSocialConnectionInstanceFieldInput struct {
+ Key string `json:"key"`
+ Value string `json:"value"`
+}
+
+type CreateSocialConnectionInstanceInput struct {
+ Provider string `json:"provider"`
+ Fields []*CreateSocialConnectionInstanceFieldInput `json:"fields"`
+}
+
+type CustomSMSProvider struct {
+ Enabled *bool `json:"enabled"`
+ Provider *string `json:"provider"`
+ Config *string `json:"config"`
+}
+
+type CustomSMSProviderInput struct {
+ Enabled *bool `json:"enabled,omitempty"`
+ Provider *string `json:"provider,omitempty"`
+ Config *string `json:"config,omitempty"`
+}
+
+type EmailTemplate struct {
+ Type EnumEmailTemplateType `json:"type"`
+ Name string `json:"name"`
+ Subject string `json:"subject"`
+ Sender string `json:"sender"`
+ Content string `json:"content"`
+ RedirectTo *string `json:"redirectTo"`
+ HasURL *bool `json:"hasURL"`
+ ExpiresIn *int64 `json:"expiresIn"`
+ Enabled *bool `json:"enabled"`
+ IsSystem *bool `json:"isSystem"`
+}
+
+type FrequentRegisterCheckConfig struct {
+ TimeInterval *int64 `json:"timeInterval"`
+ Limit *int64 `json:"limit"`
+ Enabled *bool `json:"enabled"`
+}
+
+type FrequentRegisterCheckConfigInput struct {
+ TimeInterval *int64 `json:"timeInterval,omitempty"`
+ Limit *int64 `json:"limit,omitempty"`
+ Enabled *bool `json:"enabled,omitempty"`
+}
+
+type Function struct {
+ Id string `json:"id"`
+ Name string `json:"name"`
+ SourceCode string `json:"sourceCode"`
+ Description *string `json:"description"`
+ Url *string `json:"url"`
+}
+
+type Group struct {
+ Code string `json:"code"`
+ Name string `json:"name"`
+ Description *string `json:"description"`
+ CreatedAt *string `json:"createdAt"`
+ UpdatedAt *string `json:"updatedAt"`
+ Users PaginatedUsers `json:"users"`
+ AuthorizedResources *PaginatedAuthorizedResources `json:"authorizedResources"`
+}
+
+type Identity struct {
+ Id *string `json:"id"`
+ Openid *string `json:"openid"`
+ UserIdInIdp *string `json:"userIdInIdp"`
+ UserId *string `json:"userId"`
+ ExtIdpId *string `json:"extIdpId"`
+ IsSocial *bool `json:"isSocial"`
+ Provider *string `json:"provider"`
+ UserPoolId *string `json:"userPoolId"`
+ RefreshToken *string `json:"refreshToken"`
+ AccessToken *string `json:"accessToken"`
+ Type *string `json:"type"`
+ UserInfoInIdp *interface{} `json:"userInfoInIdp"`
+}
+
+type JWTTokenStatus struct {
+ Code *int64 `json:"code"`
+ Message *string `json:"message"`
+ Status *bool `json:"status"`
+ Exp *int64 `json:"exp"`
+ Iat *int64 `json:"iat"`
+ Data *JWTTokenStatusDetail `json:"data"`
+}
+
+type JWTTokenStatusDetail struct {
+ Id *string `json:"id"`
+ UserPoolId *string `json:"userPoolId"`
+ Arn *string `json:"arn"`
+}
+
+type KeyValuePair struct {
+ Key string `json:"key"`
+ Value interface{} `json:"value"`
+}
+
+type LoginByEmailInput struct {
+ Email string `json:"email"`
+ Password string `json:"password"`
+ CaptchaCode *string `json:"captchaCode"`
+ AutoRegister *bool `json:"autoRegister"`
+ ClientIp *string `json:"clientIp"`
+ Params *string `json:"params"`
+ Context *string `json:"context"`
+}
+
+type LoginByPhoneCodeInput struct {
+ Phone string `json:"phone"`
+ Code string `json:"code"`
+ AutoRegister *bool `json:"autoRegister"`
+ ClientIp *string `json:"clientIp"`
+ Params *string `json:"params"`
+ Context *string `json:"context"`
+}
+
+type LoginByPhonePasswordInput struct {
+ Phone string `json:"phone"`
+ Password string `json:"password"`
+ CaptchaCode *string `json:"captchaCode"`
+ AutoRegister *bool `json:"autoRegister"`
+ ClientIp *string `json:"clientIp"`
+ Params *string `json:"params"`
+ Context *string `json:"context"`
+}
+
+type LoginByUsernameInput struct {
+ Username string `json:"username"`
+ Password string `json:"password"`
+ CaptchaCode *string `json:"captchaCode"`
+ AutoRegister *bool `json:"autoRegister"`
+ ClientIp *string `json:"clientIp"`
+ Params *string `json:"params"`
+ Context *string `json:"context"`
+}
+
+type LoginFailCheckConfig struct {
+ TimeInterval *int64 `json:"timeInterval"`
+ Limit *int64 `json:"limit"`
+ Enabled *bool `json:"enabled"`
+}
+
+type LoginFailCheckConfigInput struct {
+ TimeInterval *int64 `json:"timeInterval,omitempty"`
+ Limit *int64 `json:"limit,omitempty"`
+ Enabled *bool `json:"enabled,omitempty"`
+}
+
+type LoginPasswordFailCheckConfig struct {
+ TimeInterval *int64 `json:"timeInterval,omitempty"`
+ Limit *int64 `json:"limit,omitempty"`
+ Enabled *bool `json:"enabled,omitempty"`
+}
+
+type LoginPasswordFailCheckConfigInput struct {
+ TimeInterval *int64 `json:"timeInterval,omitempty"`
+ Limit *int64 `json:"limit,omitempty"`
+ Enabled *bool `json:"enabled,omitempty"`
+}
+
+type Mfa struct {
+ Id string `json:"id"`
+ UserId string `json:"userId"`
+ UserPoolId string `json:"userPoolId"`
+ Enable bool `json:"enable"`
+ Secret *string `json:"secret"`
+}
+
+type PaginatedAuthorizedResources struct {
+ TotalCount int64 `json:"totalCount"`
+ List []AuthorizedResource `json:"list"`
+}
+
+type PaginatedAuthorizedTargets struct {
+ List []*ResourcePermissionAssignment `json:"list"`
+ TotalCount *int64 `json:"totalCount"`
+}
+
+type PaginatedDepartments struct {
+ List []UserDepartment `json:"list"`
+ TotalCount int64 `json:"totalCount"`
+}
+
+type PaginatedFunctions struct {
+ List []Function `json:"list"`
+ TotalCount int64 `json:"totalCount"`
+}
+
+type PaginatedGroups struct {
+ TotalCount int64 `json:"totalCount"`
+ List []Group `json:"list"`
+}
+
+type PaginatedRoles struct {
+ TotalCount int64 `json:"totalCount"`
+ List []Role `json:"list"`
+}
+
+type PaginatedUserpool struct {
+ TotalCount int64 `json:"totalCount"`
+ List []UserPool `json:"list"`
+}
+
+type PaginatedUsers struct {
+ TotalCount int64 `json:"totalCount"`
+ List []User `json:"list"`
+}
+
+type QrcodeLoginStrategy struct {
+ QrcodeExpiresAfter *int64 `json:"qrcodeExpiresAfter"`
+ ReturnFullUserInfo *bool `json:"returnFullUserInfo"`
+ AllowExchangeUserInfoFromBrowser *bool `json:"allowExchangeUserInfoFromBrowser"`
+ TicketExpiresAfter *int64 `json:"ticketExpiresAfter"`
+}
+
+type QrcodeLoginStrategyInput struct {
+ QrcodeExpiresAfter *int64 `json:"qrcodeExpiresAfter,omitempty"`
+ ReturnFullUserInfo *bool `json:"returnFullUserInfo,omitempty"`
+ AllowExchangeUserInfoFromBrowser *bool `json:"allowExchangeUserInfoFromBrowser,omitempty"`
+ TicketExpiresAfter *int64 `json:"ticketExpiresAfter,omitempty"`
+}
+
+type RefreshAccessTokenRes struct {
+ AccessToken *string `json:"accessToken"`
+ Exp *int64 `json:"exp"`
+ Iat *int64 `json:"iat"`
+}
+
+type RefreshToken struct {
+ Token *string `json:"token"`
+ Iat *int64 `json:"iat"`
+ Exp *int64 `json:"exp"`
+}
+
+type RegisterByEmailInput struct {
+ Email string `json:"email"`
+ Password string `json:"password"`
+ Profile *RegisterProfile `json:"profile,omitempty"`
+ ForceLogin *bool `json:"forceLogin,omitempty"`
+ GenerateToken *bool `json:"generateToken,omitempty"`
+ ClientIp *string `json:"clientIp,omitempty"`
+ Params *string `json:"params,omitempty"`
+ Context *string `json:"context,omitempty"`
+}
+
+type RegisterByPhoneCodeInput struct {
+ Phone string `json:"phone"`
+ Code string `json:"code"`
+ Password *string `json:"password"`
+ Profile *RegisterProfile `json:"profile"`
+ ForceLogin *bool `json:"forceLogin"`
+ GenerateToken *bool `json:"generateToken"`
+ ClientIp *string `json:"clientIp"`
+ Params *string `json:"params"`
+ Context *string `json:"context"`
+}
+
+type RegisterByUsernameInput struct {
+ Username string `json:"username"`
+ Password string `json:"password"`
+ Profile *RegisterProfile `json:"profile"`
+ ForceLogin *bool `json:"forceLogin"`
+ GenerateToken *bool `json:"generateToken"`
+ ClientIp *string `json:"clientIp"`
+ Params *string `json:"params"`
+ Context *string `json:"context"`
+}
+
+type RegisterProfile struct {
+ Ip *string `json:"ip"`
+ Oauth *string `json:"oauth"`
+ Username *string `json:"username"`
+ Nickname *string `json:"nickname"`
+ Company *string `json:"company"`
+ Photo *string `json:"photo"`
+ Device *string `json:"device"`
+ Browser *string `json:"browser"`
+ Name *string `json:"name"`
+ GivenName *string `json:"givenName"`
+ FamilyName *string `json:"familyName"`
+ MiddleName *string `json:"middleName"`
+ Profile *string `json:"profile"`
+ PreferredUsername *string `json:"preferredUsername"`
+ Website *string `json:"website"`
+ Gender *string `json:"gender"`
+ Birthdate *string `json:"birthdate"`
+ Zoneinfo *string `json:"zoneinfo"`
+ Locale *string `json:"locale"`
+ Address *string `json:"address"`
+ Formatted *string `json:"formatted"`
+ StreetAddress *string `json:"streetAddress"`
+ Locality *string `json:"locality"`
+ Region *string `json:"region"`
+ PostalCode *string `json:"postalCode"`
+ Country *string `json:"country"`
+ Udf []UserDdfInput `json:"udf"`
+}
+
+type RegisterWhiteListConfig struct {
+ PhoneEnabled *bool `json:"phoneEnabled"`
+ EmailEnabled *bool `json:"emailEnabled"`
+ UsernameEnabled *bool `json:"usernameEnabled"`
+}
+
+type RegisterWhiteListConfigInput struct {
+ PhoneEnabled *bool `json:"phoneEnabled,omitempty"`
+ EmailEnabled *bool `json:"emailEnabled,omitempty"`
+ UsernameEnabled *bool `json:"usernameEnabled,omitempty"`
+}
+
+type ResourcePermissionAssignment struct {
+ TargetType *EnumPolicyAssignmentTargetType `json:"targetType"`
+ TargetIdentifier *string `json:"targetIdentifier"`
+ Actions []string `json:"actions"`
+}
+
+type SearchUserDepartmentOpt struct {
+ DepartmentId *string `json:"departmentId"`
+ IncludeChildrenDepartments *bool `json:"includeChildrenDepartments"`
+}
+
+type SearchUserGroupOpt struct {
+ Code *string `json:"code"`
+}
+
+type SearchUserRoleOpt struct {
+ Namespace *string `json:"namespace"`
+ Code string `json:"code"`
+}
+
+type SetUdfValueBatchInput struct {
+ TargetId string `json:"targetId"`
+ Key string `json:"key"`
+ Value interface{} `json:"value"`
+}
+
+type SocialConnection struct {
+ Provider string `json:"provider"`
+ Name string `json:"name"`
+ Logo string `json:"logo"`
+ Description *string `json:"description"`
+ Fields []SocialConnectionField `json:"fields"`
+}
+
+type SocialConnectionField struct {
+ Key *string `json:"key"`
+ Label *string `json:"label"`
+ Type *string `json:"type"`
+ Placeholder *string `json:"placeholder"`
+ Children []*SocialConnectionField `json:"children"`
+}
+
+type SocialConnectionFieldInput struct {
+ Key *string `json:"key"`
+ Label *string `json:"label"`
+ Type *string `json:"type"`
+ Placeholder *string `json:"placeholder"`
+ Children []*SocialConnectionFieldInput `json:"children"`
+}
+
+type SocialConnectionInstance struct {
+ Provider string `json:"provider"`
+ Enabled bool `json:"enabled"`
+ Fields []*SocialConnectionInstanceField `json:"fields"`
+}
+
+type SocialConnectionInstanceField struct {
+ Key string `json:"key"`
+ Value string `json:"value"`
+}
+
+type UpdateFunctionInput struct {
+ Id string `json:"id"`
+ Name *string `json:"name"`
+ SourceCode *string `json:"sourceCode"`
+ Description *string `json:"description"`
+ Url *string `json:"url"`
+}
+
+type UpdateUserpoolInput struct {
+ Name *string `json:"name,omitempty"`
+ Logo *string `json:"logo,omitempty"`
+ Domain *string `json:"domain,omitempty"`
+ Description *string `json:"description,omitempty"`
+ UserpoolTypes []string `json:"userpoolTypes,omitempty"`
+ EmailVerifiedDefault *bool `json:"emailVerifiedDefault,omitempty"`
+ SendWelcomeEmail *bool `json:"sendWelcomeEmail,omitempty"`
+ RegisterDisabled *bool `json:"registerDisabled,omitempty"`
+ AppSsoEnabled *bool `json:"appSsoEnabled,omitempty"`
+ AllowedOrigins *string `json:"allowedOrigins,omitempty"`
+ TokenExpiresAfter *int64 `json:"tokenExpiresAfter,omitempty"`
+ FrequentRegisterCheck *FrequentRegisterCheckConfigInput `json:"frequentRegisterCheck,omitempty"`
+ LoginFailCheck *LoginFailCheckConfigInput `json:"loginFailCheck,omitempty"`
+ LoginFailStrategy *string `json:"loginFailStrategy,omitempty"`
+ LoginPasswordFailCheck *LoginPasswordFailCheckConfigInput `json:"loginPasswordFailCheck,omitempty"`
+ ChangePhoneStrategy *ChangePhoneStrategyInput `json:"changePhoneStrategy,omitempty"`
+ ChangeEmailStrategy *ChangeEmailStrategyInput `json:"changeEmailStrategy,omitempty"`
+ QrcodeLoginStrategy *QrcodeLoginStrategyInput `json:"qrcodeLoginStrategy,omitempty"`
+ App2WxappLoginStrategy *App2WxappLoginStrategyInput `json:"app2WxappLoginStrategy,omitempty"`
+ Whitelist *RegisterWhiteListConfigInput `json:"whitelist,omitempty"`
+ CustomSMSProvider *CustomSMSProviderInput `json:"customSMSProvider,omitempty"`
+ LoginRequireEmailVerified *bool `json:"loginRequireEmailVerified,omitempty"`
+ VerifyCodeLength *int64 `json:"verifyCodeLength,omitempty"`
+}
+
+type UserDepartment struct {
+ Department Node `json:"department"`
+ IsMainDepartment bool `json:"isMainDepartment"`
+ JoinedAt *string `json:"joinedAt"`
+}
+
+type UserPool struct {
+ Id string `json:"id"`
+ Name string `json:"name"`
+ Domain string `json:"domain"`
+ Description *string `json:"description"`
+ Secret string `json:"secret"`
+ JwtSecret string `json:"jwtSecret"`
+ OwnerId *string `json:"ownerId"`
+ UserpoolTypes []UserPoolType `json:"userpoolTypes"`
+ Logo string `json:"logo"`
+ CreatedAt *string `json:"createdAt"`
+ UpdatedAt *string `json:"updatedAt"`
+ EmailVerifiedDefault bool `json:"emailVerifiedDefault"`
+ SendWelcomeEmail bool `json:"sendWelcomeEmail"`
+ RegisterDisabled bool `json:"registerDisabled"`
+ AppSsoEnabled bool `json:"appSsoEnabled"`
+ ShowWxQRCodeWhenRegisterDisabled *bool `json:"showWxQRCodeWhenRegisterDisabled"`
+ AllowedOrigins *string `json:"allowedOrigins"`
+ TokenExpiresAfter *int64 `json:"tokenExpiresAfter"`
+ IsDeleted *bool `json:"isDeleted"`
+ FrequentRegisterCheck *FrequentRegisterCheckConfig `json:"frequentRegisterCheck"`
+ LoginFailCheck *LoginFailCheckConfig `json:"loginFailCheck"`
+ LoginPasswordFailCheck *LoginPasswordFailCheckConfig `json:"loginPasswordFailCheck"`
+ LoginFailStrategy *string `json:"loginFailStrategy"`
+ ChangePhoneStrategy *ChangePhoneStrategy `json:"changePhoneStrategy"`
+ ChangeEmailStrategy *ChangeEmailStrategy `json:"changeEmailStrategy"`
+ QrcodeLoginStrategy *QrcodeLoginStrategy `json:"qrcodeLoginStrategy"`
+ App2WxappLoginStrategy *App2WxappLoginStrategy `json:"app2WxappLoginStrategy"`
+ Whitelist *RegisterWhiteListConfig `json:"whitelist"`
+ CustomSMSProvider *CustomSMSProvider `json:"customSMSProvider"`
+ PackageType *int64 `json:"packageType"`
+ UseCustomUserStore *bool `json:"useCustomUserStore"`
+ LoginRequireEmailVerified *bool `json:"loginRequireEmailVerified"`
+ VerifyCodeLength *int64 `json:"verifyCodeLength"`
+}
+
+type UserPoolType struct {
+ Code *string `json:"code"`
+ Name *string `json:"name"`
+ Description *string `json:"description"`
+ Image *string `json:"image"`
+ Sdks []*string `json:"sdks"`
+}
+
+type WhiteList struct {
+ CreatedAt *string `json:"createdAt"`
+ UpdatedAt *string `json:"updatedAt"`
+ Value string `json:"value"`
+}
+
+type GqlCommonErrors struct {
+ Message CommonMessageAndCode `json:"message"`
+ Locations []struct {
+ Line int `json:"line"`
+ Column int `json:"column"`
+ } `json:"locations"`
+ Extensions struct {
+ Code string `json:"code"`
+ Extension struct {
+ Name string `json:"name"`
+ } `json:"extension"`
+ }
+}
+
+type CommonMessageAndCode struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+}
+
+type UserPoolEnv struct {
+ UserPoolId string `json:"userPoolId"`
+ Key string `json:"key"`
+ CreatedAt time.Time `json:"createdAt"`
+ UpdatedAt time.Time `json:"updatedAt"`
+ Id string `json:"id"`
+}
+
+type UserOrgs []struct {
+ Type string `json:"type"`
+ Id string `json:"id"`
+ CreatedAt time.Time `json:"createdAt"`
+ UpdatedAt time.Time `json:"updatedAt"`
+ UserPoolId string `json:"userPoolId"`
+ RootNodeId string `json:"rootNodeId,omitempty"`
+ Logo string `json:"logo"`
+ OrgId string `json:"orgId,omitempty"`
+ Name string `json:"name,omitempty"`
+ NameI18N string `json:"nameI18n"`
+ Description *string `json:"description,omitempty"`
+ DescriptionI18N string `json:"descriptionI18n"`
+ Order string `json:"order"`
+ Code *string `json:"code,omitempty"`
+ LeaderUserId string `json:"leaderUserId"`
+ Source []interface{} `json:"source,omitempty"`
+ DataVersion interface{} `json:"dataVersion"`
+ SourceData interface{} `json:"sourceData"`
+}
+
+type GetSecurityLevelResponse struct {
+ Score int `json:"score"`
+ Email bool `json:"email"`
+ Phone bool `json:"phone"`
+ Password bool `json:"password"`
+ PasswordSecurityLevel int `json:"passwordSecurityLevel"`
+ Mfa bool `json:"mfa"`
+}
+
+type LoginBySubAccountRequest struct {
+ Account string `json:"account"`
+ Password string `json:"password"`
+ CaptchaCode string `json:"captchaCode,omitempty"`
+ ClientIp string `json:"clientIp,omitempty"`
+}
+
+type IsUserExistsRequest struct {
+ Username *string `json:"username,omitempty"`
+ Email *string `json:"email,omitempty"`
+ Phone *string `json:"phone,omitempty"`
+ ExternalId *string `json:"externalId,omitempty"`
+}
diff --git a/lib/model/namespace_model.go b/lib/model/namespace_model.go
new file mode 100644
index 0000000..e76e7b4
--- /dev/null
+++ b/lib/model/namespace_model.go
@@ -0,0 +1,25 @@
+package model
+
+type Namespace struct {
+ UserPoolId string `json:"userPoolId"`
+ Name string `json:"name"`
+ Code string `json:"code"`
+ Description string `json:"description"`
+ Status int `json:"status"`
+ ApplicationId string `json:"applicationId"`
+ IsIntegrateApp bool `json:"isIntegrateApp"`
+ IsDefaultApp bool `json:"isDefaultApp"`
+ Id int `json:"id"`
+}
+
+type EditNamespaceRequest struct {
+ Code *string `json:"code,omitempty"`
+ Name *string `json:"name,omitempty"`
+ Description *string `json:"description,omitempty"`
+}
+
+type ListGroupsAuthorizedResourcesRequest struct {
+ Code string `json:"code"`
+ Namespace *string `json:"namespace,omitempty"`
+ ResourceType *EnumResourceType `json:"resourceType,omitempty"`
+}
diff --git a/lib/model/org_model.go b/lib/model/org_model.go
new file mode 100644
index 0000000..6b08b7e
--- /dev/null
+++ b/lib/model/org_model.go
@@ -0,0 +1,109 @@
+package model
+
+type CreateOrgRequest struct {
+ Name string `json:"name"`
+ Code *string `json:"code,omitempty"`
+ Description *string `json:"description,omitempty"`
+}
+
+type OrgNode struct {
+ Id string `json:"id"`
+ OrgId *string `json:"orgId"`
+ CreatedAt *string `json:"createdAt"`
+ UpdatedAt *string `json:"updatedAt"`
+ UserPoolId *string `json:"userPoolId"`
+ Name string `json:"name"`
+ Description *string `json:"description"`
+ DescriptionI18n *string `json:"descriptionI18n"`
+ Order *int64 `json:"order"`
+ Code *string `json:"code"`
+ Members *[]User `json:"members,omitempty"`
+ Children *[]OrgNode `json:"children,omitempty"`
+}
+
+type OrgResponse struct {
+ Id string `json:"id"`
+ RootNode *OrgNode `json:"rootNode,omitempty"`
+ Nodes *[]OrgNode `json:"nodes,omitempty"`
+}
+
+type PaginatedOrgs struct {
+ TotalCount int64 `json:"totalCount"`
+ List []Org `json:"list"`
+}
+
+type Node struct {
+ Id string `json:"id"`
+ OrgId *string `json:"orgId"`
+ Name string `json:"name"`
+ NameI18n *string `json:"nameI18n"`
+ Description *string `json:"description"`
+ DescriptionI18n *string `json:"descriptionI18n"`
+ Order *int64 `json:"order"`
+ Code *string `json:"code"`
+ Root *bool `json:"root"`
+ Depth *int64 `json:"depth"`
+ Path []string `json:"path"`
+ CodePath []*string `json:"codePath"`
+ NamePath []string `json:"namePath"`
+ CreatedAt *string `json:"createdAt"`
+ UpdatedAt *string `json:"updatedAt"`
+ Children []string `json:"children"`
+ Users PaginatedUsers `json:"users"`
+ AuthorizedResources *PaginatedAuthorizedResources `json:"authorizedResources"`
+}
+
+type Org struct {
+ Id string `json:"id"`
+ RootNode Node `json:"rootNode"`
+ Nodes []Node `json:"nodes"`
+}
+
+type AddNodeOrg struct {
+ Id string `json:"id"`
+ RootNode OrgNodeChildStr `json:"rootNode"`
+ Nodes []OrgNodeChildStr `json:"nodes"`
+}
+type AddOrgNodeRequest struct {
+ OrgId string `json:"orgId"`
+ ParentNodeId string `json:"parentNodeId"`
+ Name string `json:"name"`
+ Code *string `json:"code,omitempty"`
+ Description *string `json:"description,omitempty"`
+ Order *int `json:"order,omitempty"`
+ NameI18N *string `json:"nameI18n,omitempty"`
+ DescriptionI18N *string `json:"descriptionI18n,omitempty"`
+}
+
+type OrgNodeChildStr struct {
+ Id string `json:"id"`
+ OrgId *string `json:"orgId"`
+ Name string `json:"name"`
+ NameI18n *string `json:"nameI18n"`
+ Description *string `json:"description"`
+ DescriptionI18n *string `json:"descriptionI18n"`
+ Order *int64 `json:"order"`
+ Code *string `json:"code"`
+ Root *bool `json:"root"`
+ Depth *int64 `json:"depth"`
+ Path []string `json:"path"`
+ CodePath []*string `json:"codePath"`
+ NamePath []string `json:"namePath"`
+ CreatedAt *string `json:"createdAt"`
+ UpdatedAt *string `json:"updatedAt"`
+ Children []string `json:"children"`
+}
+
+type UpdateOrgNodeRequest struct {
+ Id string `json:"id"`
+ Name *string `json:"name,omitempty"`
+ Code *string `json:"code,omitempty"`
+ Description *string `json:"description,omitempty"`
+}
+
+type ListAuthorizedResourcesByNodeCodeRequest struct {
+ Id string `json:"id"`
+ Code string `json:"code"`
+ Namespace *string `json:"namespace,omitempty"`
+ ResourceType *string `json:"resourceType,omitempty"`
+}
diff --git a/lib/model/policy_model.go b/lib/model/policy_model.go
new file mode 100644
index 0000000..8407513
--- /dev/null
+++ b/lib/model/policy_model.go
@@ -0,0 +1,99 @@
+package model
+
+import (
+ "time"
+)
+
+type PolicyRequest struct {
+ Code string `json:"code"`
+ Description *string `json:"description,omitempty"`
+ Statements []PolicyStatement `json:"statements,omitempty"`
+}
+
+type CreatePolicyResponse struct {
+ Namespace string `json:"namespace"`
+ Code string `json:"code"`
+ IsDefault bool `json:"isDefault"`
+ Description string `json:"description"`
+ Statements []PolicyStatement `json:"statements"`
+ CreatedAt time.Time `json:"createdAt"`
+ UpdatedAt time.Time `json:"updatedAt"`
+ AssignmentsCount int `json:"assignmentsCount"`
+}
+
+type UpdatePolicyResponse struct {
+ Namespace string `json:"namespace"`
+ Code string `json:"code"`
+ IsDefault bool `json:"isDefault"`
+ Description string `json:"description"`
+ Statements []PolicyStatement `json:"statements"`
+ CreatedAt time.Time `json:"createdAt"`
+ UpdatedAt time.Time `json:"updatedAt"`
+}
+
+type PaginatedPolicies struct {
+ TotalCount int64 `json:"totalCount"`
+ List []Policy `json:"list"`
+}
+
+type PaginatedPolicyAssignments struct {
+ TotalCount int64 `json:"totalCount"`
+ List []PolicyAssignment `json:"list"`
+}
+
+type Policy struct {
+ Namespace string `json:"namespace"`
+ Code string `json:"code"`
+ IsDefault bool `json:"isDefault"`
+ Description *string `json:"description"`
+ Statements []PolicyStatement `json:"statements"`
+ CreatedAt *string `json:"createdAt"`
+ UpdatedAt *string `json:"updatedAt"`
+ AssignmentsCount int64 `json:"assignmentsCount"`
+ Assignments []PolicyAssignment `json:"assignments"`
+}
+
+type PolicyAssignment struct {
+ Code string `json:"code"`
+ TargetType EnumPolicyAssignmentTargetType `json:"targetType"`
+ TargetIdentifier string `json:"targetIdentifier"`
+}
+
+type PolicyStatement struct {
+ Resource string `json:"resource"`
+ Actions []string `json:"actions"`
+ Effect *EnumPolicyEffect `json:"effect"`
+ Condition []PolicyStatementCondition `json:"condition,omitempty"`
+}
+
+type PolicyStatementCondition struct {
+ Param string `json:"param"`
+ Operator string `json:"operator"`
+ //Value Object `json:"value"`
+}
+
+type PolicyStatementConditionInput struct {
+ Param string `json:"param"`
+ Operator string `json:"operator"`
+ //Value Object `json:"value"`
+}
+
+type PolicyStatementInput struct {
+ Resource string `json:"resource"`
+ Actions []string `json:"actions"`
+ Effect *EnumPolicyEffect `json:"effect"`
+ Condition []PolicyStatementConditionInput `json:"condition"`
+}
+
+type PolicyAssignmentsRequest struct {
+ Policies []string `json:"policies"`
+ TargetType EnumPolicyAssignmentTargetType `json:"targetType"`
+ TargetIdentifiers []string `json:"targetIdentifiers"`
+}
+
+type SwitchPolicyAssignmentsRequest struct {
+ Policy string `json:"policy"`
+ TargetType EnumPolicyAssignmentTargetType `json:"targetType"`
+ TargetIdentifier string `json:"targetIdentifier"`
+ Namespace *string `json:"namespace,omitempty"`
+}
diff --git a/lib/model/role_model.go b/lib/model/role_model.go
new file mode 100644
index 0000000..c6ca38f
--- /dev/null
+++ b/lib/model/role_model.go
@@ -0,0 +1,111 @@
+package model
+
+import "github.com/Authing/authing-go-sdk/lib/enum"
+
+type Role struct {
+ Id string `json:"id"`
+ Namespace string `json:"namespace"`
+ Code string `json:"code"`
+ Arn string `json:"arn"`
+ Description *string `json:"description,omitempty"`
+ IsSystem *bool `json:"isSystem,omitempty"`
+ CreatedAt *string `json:"createdAt,omitempty"`
+ UpdatedAt *string `json:"updatedAt,omitempty"`
+ Users PaginatedUsers `json:"users"`
+ AuthorizedResources *PaginatedAuthorizedResources `json:"authorizedResources,omitempty"`
+ Parent *Role `json:"parent,omitempty"`
+}
+
+type RoleModel struct {
+ Id string `json:"id"`
+ Namespace string `json:"namespace"`
+ Code string `json:"code"`
+ Arn string `json:"arn"`
+ Description *string `json:"description,omitempty"`
+ CreatedAt *string `json:"createdAt,omitempty"`
+ UpdatedAt *string `json:"updatedAt,omitempty"`
+ Parent *struct {
+ Id string `json:"id,omitempty"`
+ Namespace string `json:"namespace,omitempty"`
+ Code string `json:"code,omitempty"`
+ Arn string `json:"arn,omitempty"`
+ Description *string `json:"description,omitempty"`
+ CreatedAt *string `json:"createdAt,omitempty"`
+ UpdatedAt *string `json:"updatedAt,omitempty"`
+ } `json:"parent,omitempty"`
+}
+
+type GetRoleListRequest struct {
+ Page int `json:"page"`
+ Limit int `json:"limit"`
+ SortBy enum.SortByEnum `json:"sortBy"`
+ Namespace string `json:"namespace"`
+}
+
+type Roles struct {
+ Roles PaginatedRoles `json:"roles"`
+}
+type GetRoleListResponse struct {
+ Data Roles `json:"data"`
+}
+
+type GetRoleUserListRequest struct {
+ Page int `json:"page"`
+ Limit int `json:"limit"`
+ Code string `json:"code"`
+ Namespace *string `json:"namespace,omitempty"`
+}
+
+type CreateRoleRequest struct {
+ Code string `json:"code"`
+ Namespace *string `json:"namespace,omitempty"`
+ Description *string `json:"description,omitempty"`
+ ParentCode *string `json:"parent,omitempty"`
+}
+
+type DeleteRoleRequest struct {
+ Code string `json:"code"`
+ Namespace *string `json:"namespace,omitempty"`
+}
+
+type DeleteRole struct {
+ DeleteRole Role `json:"createRole"`
+}
+
+type BatchDeleteRoleRequest struct {
+ CodeList []string `json:"codeList"`
+ Namespace *string `json:"namespace,omitempty"`
+}
+
+type UpdateRoleRequest struct {
+ Code string `json:"code"`
+ NewCode *string `json:"newCode,omitempty"`
+ Namespace *string `json:"namespace,omitempty"`
+ Description *string `json:"description,omitempty"`
+ ParentCode *string `json:"parent,omitempty"`
+}
+
+type RoleDetailRequest struct {
+ Code string `json:"code"`
+ Namespace *string `json:"namespace,omitempty"`
+}
+
+type AssignAndRevokeRoleRequest struct {
+ RoleCodes []string `json:"roleCodes"`
+ Namespace *string `json:"namespace,omitempty"`
+ UserIds []string `json:"userIds"`
+}
+
+type AuthorizedResources struct {
+ TotalCount int `json:"totalCount"`
+ List []struct {
+ Code string `json:"code"`
+ Type string `json:"type"`
+ Actions []string `json:"actions"`
+ } `json:"list"`
+}
+
+type BatchRoleUdv struct {
+ TargetId string `json:"targetId"`
+ Data []UserDefinedData `json:"data"`
+}
diff --git a/lib/model/tenant_model.go b/lib/model/tenant_model.go
new file mode 100644
index 0000000..70df9e9
--- /dev/null
+++ b/lib/model/tenant_model.go
@@ -0,0 +1,239 @@
+package model
+
+import "time"
+
+type Tenant struct {
+ ID string `json:"id"`
+ CreatedAt time.Time `json:"createdAt"`
+ UpdatedAt time.Time `json:"updatedAt"`
+ UserPoolID string `json:"userPoolId"`
+ Name string `json:"name"`
+ Logo string `json:"logo"`
+ Description interface{} `json:"description"`
+ CSS interface{} `json:"css"`
+ SsoPageCustomizationSettings interface{} `json:"ssoPageCustomizationSettings"`
+ DefaultLoginTab string `json:"defaultLoginTab"`
+ DefaultRegisterTab string `json:"defaultRegisterTab"`
+ PasswordTabConfig struct {
+ EnabledLoginMethods []string `json:"enabledLoginMethods"`
+ } `json:"passwordTabConfig"`
+ LoginTabs []string `json:"loginTabs"`
+ RegisterTabs []string `json:"registerTabs"`
+ ExtendsFields interface{} `json:"extendsFields"`
+}
+
+type GetTenantListResponse struct {
+ TotalCount int64 `json:"totalCount"`
+ List []Tenant `json:"list"`
+}
+
+type TenantDetails struct {
+ Tenant
+ Apps []struct {
+ QrcodeScanning struct {
+ Redirect bool `json:"redirect"`
+ Interval int `json:"interval"`
+ } `json:"qrcodeScanning"`
+ ID string `json:"id"`
+ CreatedAt time.Time `json:"createdAt"`
+ UpdatedAt time.Time `json:"updatedAt"`
+ UserPoolID string `json:"userPoolId"`
+ Protocol string `json:"protocol"`
+ IsOfficial bool `json:"isOfficial"`
+ IsDeleted bool `json:"isDeleted"`
+ IsDefault bool `json:"isDefault"`
+ IsDemo bool `json:"isDemo"`
+ Name string `json:"name"`
+ Description interface{} `json:"description"`
+ Secret string `json:"secret"`
+ Identifier string `json:"identifier"`
+ Jwks struct {
+ Keys []struct {
+ E string `json:"e"`
+ N string `json:"n"`
+ D string `json:"d"`
+ P string `json:"p"`
+ Q string `json:"q"`
+ Dp string `json:"dp"`
+ Dq string `json:"dq"`
+ Qi string `json:"qi"`
+ Kty string `json:"kty"`
+ Kid string `json:"kid"`
+ Alg string `json:"alg"`
+ Use string `json:"use"`
+ } `json:"keys"`
+ } `json:"jwks"`
+ SsoPageCustomizationSettings interface{} `json:"ssoPageCustomizationSettings"`
+ Logo string `json:"logo"`
+ RedirectUris []string `json:"redirectUris"`
+ LogoutRedirectUris []interface{} `json:"logoutRedirectUris"`
+ InitLoginURL interface{} `json:"initLoginUrl"`
+ OidcProviderEnabled bool `json:"oidcProviderEnabled"`
+ OauthProviderEnabled bool `json:"oauthProviderEnabled"`
+ SamlProviderEnabled bool `json:"samlProviderEnabled"`
+ CasProviderEnabled bool `json:"casProviderEnabled"`
+ RegisterDisabled bool `json:"registerDisabled"`
+ LoginTabs []string `json:"loginTabs"`
+ PasswordTabConfig struct {
+ EnabledLoginMethods []string `json:"enabledLoginMethods"`
+ } `json:"passwordTabConfig"`
+ DefaultLoginTab string `json:"defaultLoginTab"`
+ RegisterTabs []string `json:"registerTabs"`
+ DefaultRegisterTab string `json:"defaultRegisterTab"`
+ ExtendsFieldsEnabled bool `json:"extendsFieldsEnabled"`
+ ExtendsFields []interface{} `json:"extendsFields"`
+ ComplateFiledsPlace []interface{} `json:"complateFiledsPlace"`
+ SkipComplateFileds bool `json:"skipComplateFileds"`
+ Ext interface{} `json:"ext"`
+ CSS interface{} `json:"css"`
+ OidcConfig struct {
+ GrantTypes []string `json:"grant_types"`
+ ResponseTypes []string `json:"response_types"`
+ IDTokenSignedResponseAlg string `json:"id_token_signed_response_alg"`
+ TokenEndpointAuthMethod string `json:"token_endpoint_auth_method"`
+ AuthorizationCodeExpire int `json:"authorization_code_expire"`
+ IDTokenExpire int `json:"id_token_expire"`
+ AccessTokenExpire int `json:"access_token_expire"`
+ RefreshTokenExpire int `json:"refresh_token_expire"`
+ CasExpire int `json:"cas_expire"`
+ SkipConsent bool `json:"skip_consent"`
+ RedirectUris []string `json:"redirect_uris"`
+ PostLogoutRedirectUris []interface{} `json:"post_logout_redirect_uris"`
+ ClientID string `json:"client_id"`
+ ClientSecret string `json:"client_secret"`
+ } `json:"oidcConfig"`
+ OidcJWEConfig interface{} `json:"oidcJWEConfig"`
+ SamlConfig interface{} `json:"samlConfig"`
+ OauthConfig struct {
+ ID string `json:"id"`
+ ClientSecret string `json:"client_secret"`
+ RedirectUris []string `json:"redirect_uris"`
+ Grants []string `json:"grants"`
+ AccessTokenLifetime int `json:"access_token_lifetime"`
+ RefreshTokenLifetime int `json:"refresh_token_lifetime"`
+ IntrospectionEndpointAuthMethod string `json:"introspection_endpoint_auth_method"`
+ RevocationEndpointAuthMethod string `json:"revocation_endpoint_auth_method"`
+ } `json:"oauthConfig"`
+ CasConfig interface{} `json:"casConfig"`
+ ShowAuthorizationPage bool `json:"showAuthorizationPage"`
+ EnableSubAccount bool `json:"enableSubAccount"`
+ EnableDeviceMutualExclusion bool `json:"enableDeviceMutualExclusion"`
+ LoginRequireEmailVerified bool `json:"loginRequireEmailVerified"`
+ AgreementEnabled bool `json:"agreementEnabled"`
+ IsIntegrate bool `json:"isIntegrate"`
+ SsoEnabled bool `json:"ssoEnabled"`
+ Template interface{} `json:"template"`
+ SkipMfa bool `json:"skipMfa"`
+ CasExpireBaseBrowser bool `json:"casExpireBaseBrowser"`
+ AppType string `json:"appType"`
+ PermissionStrategy struct {
+ Enabled bool `json:"enabled"`
+ DefaultStrategy string `json:"defaultStrategy"`
+ AllowPolicyID interface{} `json:"allowPolicyId"`
+ DenyPolicyID interface{} `json:"denyPolicyId"`
+ } `json:"permissionStrategy"`
+ } `json:"apps"`
+}
+
+type CreateTenantRequest struct {
+ Name string `json:"name"`
+ AppIds string `json:"appIds"`
+ Logo string `json:"logo,omitempty"`
+ Description string `json:"description,omitempty"`
+}
+
+type TenantSsoPageCustomizationSettings struct {
+ AutoRegisterThenLogin bool `json:"autoRegisterThenLogin,omitempty"`
+ HideForgetPassword bool `json:"hideForgetPassword,omitempty"`
+ HideIdp bool `json:"hideIdp,omitempty"`
+ HideSocialLogin bool `json:"hideSocialLogin,omitempty"`
+}
+
+type ConfigTenantRequest struct {
+ CSS string `json:"css,omitempty"`
+ SsoPageCustomizationSettings *TenantSsoPageCustomizationSettings `json:"ssoPageCustomizationSettings,omitempty"`
+}
+
+type TenantMembersResponse struct {
+ ListTotal int64 `json:"listTotal"`
+ List []struct {
+ ID string `json:"id"`
+ TenantID string `json:"tenantId"`
+ User *User `json:"user"`
+ } `json:"list"`
+}
+
+type AddTenantMembersResponse struct {
+ Tenant
+ Users *[]User `json:"users"`
+}
+
+type ListExtIdpResponse struct {
+ ID string `json:"id"`
+ Name string `json:"name"`
+ Type string `json:"type"`
+ TenantID string `json:"tenantId"`
+ Connections []struct {
+ ID string `json:"id"`
+ Type string `json:"type"`
+ Identifier string `json:"identifier"`
+ DisplayName string `json:"displayName"`
+ Logo string `json:"logo"`
+ Enabled bool `json:"enabled"`
+ } `json:"connections"`
+}
+
+type ExtIdpDetailResponse struct {
+ ID string `json:"id"`
+ Name string `json:"name"`
+ Type string `json:"type"`
+ Connections []ExtIdpConnectionDetails `json:"connections"`
+}
+
+type ExtIdpConnection struct {
+ Type string `json:"type"`
+ Identifier string `json:"identifier"`
+ DisplayName string `json:"displayName"`
+ Fields interface{} `json:"fields"`
+ Logo string `json:"logo,omitempty"`
+ UserMatchFields []string `json:"userMatchFields,omitempty"`
+}
+
+type ExtIdpConnectionDetails struct {
+ ID string `json:"id"`
+ ExtIdpConnection
+}
+
+type CreateExtIdpRequest struct {
+ Name string `json:"name"`
+ Type string `json:"type"`
+ TenantUd string `json:"tenantUd"`
+ Connections []ExtIdpConnection `json:"connections"`
+}
+
+type UpdateExtIdpRequest struct {
+ Name string `json:"name"`
+}
+
+type CreateExtIdpConnectionRequest struct {
+ ExtIdpId string `json:"extIdpId"`
+ Type string `json:"type"`
+ Identifier string `json:"identifier"`
+ DisplayName string `json:"displayName"`
+ Fields interface{} `json:"fields"`
+ Logo string `json:"logo,omitempty"`
+ UserMatchFields []string `json:"userMatchFields,omitempty"`
+}
+
+type UpdateExtIdpConnectionRequest struct {
+ DisplayName string `json:"displayName"`
+ Fields interface{} `json:"fields"`
+ Logo string `json:"logo,omitempty"`
+ UserMatchFields []string `json:"userMatchFields,omitempty"`
+}
+
+type ChangeExtIdpConnectionStateRequest struct {
+ AppID string `json:"appId,omitempty"`
+ TenantID string `json:"tenantId,omitempty"`
+ Enabled bool `json:"enabled"`
+}
diff --git a/lib/model/user_model.go b/lib/model/user_model.go
new file mode 100644
index 0000000..a6e6fc9
--- /dev/null
+++ b/lib/model/user_model.go
@@ -0,0 +1,279 @@
+package model
+
+import (
+ "time"
+)
+
+type CreateUserInput struct {
+ Username *string `json:"username,omitempty"`
+ Email *string `json:"email,omitempty"`
+ EmailVerified *bool `json:"emailVerified,omitempty"`
+ Phone *string `json:"phone,omitempty"`
+ PhoneVerified *bool `json:"phoneVerified,omitempty"`
+ Unionid *string `json:"unionid,omitempty"`
+ Openid *string `json:"openid,omitempty"`
+ Nickname *string `json:"nickname,omitempty"`
+ Photo *string `json:"photo,omitempty"`
+ Password *string `json:"password,omitempty"`
+ RegisterSource []string `json:"registerSource,omitempty"`
+ Browser *string `json:"browser,omitempty"`
+ Oauth *string `json:"oauth,omitempty"`
+ LoginsCount *int64 `json:"loginsCount,omitempty"`
+ LastLogin *string `json:"lastLogin,omitempty"`
+ Company *string `json:"company,omitempty"`
+ LastIP *string `json:"lastIP,omitempty"`
+ SignedUp *string `json:"signedUp,omitempty"`
+ Blocked *bool `json:"blocked,omitempty"`
+ IsDeleted *bool `json:"isDeleted,omitempty"`
+ Device *string `json:"device,omitempty"`
+ Name *string `json:"name,omitempty"`
+ GivenName *string `json:"givenName,omitempty"`
+ FamilyName *string `json:"familyName,omitempty"`
+ MiddleName *string `json:"middleName,omitempty"`
+ Profile *string `json:"profile,omitempty"`
+ PreferredUsername *string `json:"preferredUsername,omitempty"`
+ Website *string `json:"website,omitempty"`
+ Gender *string `json:"gender,omitempty"`
+ Birthdate *string `json:"birthdate,omitempty"`
+ Zoneinfo *string `json:"zoneinfo,omitempty"`
+ Locale *string `json:"locale,omitempty"`
+ Address *string `json:"address,omitempty"`
+ Formatted *string `json:"formatted,omitempty"`
+ StreetAddress *string `json:"streetAddress,omitempty"`
+ Locality *string `json:"locality,omitempty"`
+ Region *string `json:"region,omitempty"`
+ PostalCode *string `json:"postalCode,omitempty"`
+ Country *string `json:"country,omitempty"`
+ ExternalId *string `json:"externalId,omitempty"`
+}
+
+type UpdateUserInput struct {
+ Email *string `json:"email,omitempty"`
+ Unionid *string `json:"unionid,omitempty"`
+ Openid *string `json:"openid,omitempty"`
+ EmailVerified *bool `json:"emailVerified,omitempty"`
+ Phone *string `json:"phone,omitempty"`
+ PhoneVerified *bool `json:"phoneVerified,omitempty"`
+ Username *string `json:"username,omitempty"`
+ Nickname *string `json:"nickname,omitempty"`
+ Password *string `json:"password,omitempty"`
+ Photo *string `json:"photo,omitempty"`
+ Company *string `json:"company,omitempty"`
+ Browser *string `json:"browser,omitempty"`
+ Device *string `json:"device,omitempty"`
+ Oauth *string `json:"oauth,omitempty"`
+ TokenExpiredAt *string `json:"tokenExpiredAt,omitempty"`
+ LoginsCount *int64 `json:"loginsCount,omitempty"`
+ LastLogin *string `json:"lastLogin,omitempty"`
+ LastIP *string `json:"lastIP,omitempty"`
+ Blocked *bool `json:"blocked,omitempty"`
+ Name *string `json:"name,omitempty"`
+ GivenName *string `json:"givenName,omitempty"`
+ FamilyName *string `json:"familyName,omitempty"`
+ MiddleName *string `json:"middleName,omitempty"`
+ Profile *string `json:"profile,omitempty"`
+ PreferredUsername *string `json:"preferredUsername"`
+ Website *string `json:"website,omitempty"`
+ Gender *string `json:"gender,omitempty"`
+ Birthdate *string `json:"birthdate,omitempty"`
+ Zoneinfo *string `json:"zoneinfo,omitempty"`
+ Locale *string `json:"locale,omitempty"`
+ Address *string `json:"address,omitempty"`
+ Formatted *string `json:"formatted,omitempty"`
+ StreetAddress *string `json:"streetAddress,omitempty"`
+ Locality *string `json:"locality,omitempty"`
+ Region *string `json:"region,omitempty"`
+ PostalCode *string `json:"postalCode,omitempty"`
+ City *string `json:"city,omitempty"`
+ Province *string `json:"province,omitempty"`
+ Country *string `json:"country,omitempty"`
+ ExternalId *string `json:"externalId,omitempty"`
+}
+
+type User struct {
+ Id string `json:"id"`
+ Arn string `json:"arn"`
+ Status *EnumUserStatus `json:"status"`
+ UserPoolId string `json:"userPoolId"`
+ Username *string `json:"username"`
+ Email *string `json:"email"`
+ EmailVerified *bool `json:"emailVerified"`
+ Phone *string `json:"phone"`
+ PhoneVerified *bool `json:"phoneVerified"`
+ Unionid *string `json:"unionid"`
+ Openid *string `json:"openid"`
+ Identities []*Identity `json:"identities"`
+ Nickname *string `json:"nickname"`
+ RegisterSource []string `json:"registerSource"`
+ Photo *string `json:"photo"`
+ Password *string `json:"password"`
+ Oauth *string `json:"oauth"`
+ Token *string `json:"token"`
+ TokenExpiredAt *string `json:"tokenExpiredAt"`
+ LoginsCount *int64 `json:"loginsCount"`
+ LastLogin *string `json:"lastLogin"`
+ LastIP *string `json:"lastIP"`
+ SignedUp *string `json:"signedUp"`
+ Blocked *bool `json:"blocked"`
+ IsDeleted *bool `json:"isDeleted"`
+ Device *string `json:"device"`
+ Browser *string `json:"browser"`
+ Company *string `json:"company"`
+ Name *string `json:"name"`
+ GivenName *string `json:"givenName"`
+ FamilyName *string `json:"familyName"`
+ MiddleName *string `json:"middleName"`
+ Profile *string `json:"profile"`
+ PreferredUsername *string `json:"preferredUsername"`
+ Website *string `json:"website"`
+ Gender *string `json:"gender"`
+ Birthdate *string `json:"birthdate"`
+ Zoneinfo *string `json:"zoneinfo"`
+ Locale *string `json:"locale"`
+ Address *string `json:"address"`
+ Formatted *string `json:"formatted"`
+ StreetAddress *string `json:"streetAddress"`
+ Locality *string `json:"locality"`
+ Region *string `json:"region"`
+ PostalCode *string `json:"postalCode"`
+ City *string `json:"city"`
+ Province *string `json:"province"`
+ Country *string `json:"country"`
+ CreatedAt *string `json:"createdAt"`
+ UpdatedAt *string `json:"updatedAt"`
+ Roles *PaginatedRoles `json:"roles"`
+ Groups *PaginatedGroups `json:"groups"`
+ Departments *PaginatedDepartments `json:"departments"`
+ AuthorizedResources *PaginatedAuthorizedResources `json:"authorizedResources"`
+ ExternalId *string `json:"externalId"`
+ CustomData []*UserCustomData `json:"customData"`
+}
+
+type UserCustomData struct {
+ Key string `json:"key,omitempty"`
+ Value *string `json:"value,omitempty"`
+ Label *string `json:"label,omitempty"`
+ DataType EnumUDFDataType `json:"dataType,omitempty"`
+}
+
+type UserDdfInput struct {
+ Key string `json:"key,omitempty"`
+ Value string `json:"value,omitempty"`
+}
+
+type UserDefinedData struct {
+ Key string `json:"key,omitempty"`
+ DataType EnumUDFDataType `json:"dataType,omitempty"`
+ Value string `json:"value,omitempty"`
+ Label *string `json:"label,omitempty"`
+}
+
+type UserDefinedDataInput struct {
+ Key string `json:"key,omitempty"`
+ Value *string `json:"value,omitempty"`
+}
+
+type UserDefinedDataMap struct {
+ TargetId string `json:"targetId"`
+ Data []UserDefinedData `json:"data"`
+}
+
+type UserDefinedField struct {
+ TargetType EnumUDFTargetType `json:"targetType"`
+ DataType EnumUDFDataType `json:"dataType"`
+ Key string `json:"key"`
+ Label *string `json:"label"`
+ Options *string `json:"options"`
+}
+
+type CreateUserRequest struct {
+ UserInfo CreateUserInput `json:"userInfo,omitempty"`
+ KeepPassword bool `json:"keepPassword,omitempty"`
+ CustomData []KeyValuePair `json:"params,omitempty"`
+}
+
+type CommonPageUsersResponse struct {
+ TotalCount int `json:"totalCount"`
+ List []User `json:"list"`
+}
+
+type FindUserRequest struct {
+ Email *string `json:"email,omitempty"`
+ Username *string `json:"username,omitempty"`
+ Phone *string `json:"phone,omitempty"`
+ ExternalId *string `json:"externalId,omitempty"`
+ WithCustomData bool `json:"withCustomData,omitempty"`
+}
+
+type SearchUserRequest struct {
+ Query string `json:"query"`
+ Page int `json:"page"`
+ Limit int `json:"limit"`
+ DepartmentOpts *[]string `json:"departmentOpts,omitempty"`
+ GroupOpts *[]string `json:"groupOpts,omitempty"`
+ RoleOpts *[]string `json:"roleOpts,omitempty"`
+ WithCustomData bool
+}
+
+type GetUserGroupsResponse struct {
+ Groups struct {
+ TotalCount int `json:"totalCount"`
+ List []GroupModel `json:"list"`
+ } `json:"groups"`
+}
+
+type GetUserRolesRequest struct {
+ Id string `json:"id"`
+ Namespace string `json:"namespace,omitempty"`
+}
+
+type GetUserRolesResponse struct {
+ Roles struct {
+ TotalCount int `json:"totalCount"`
+ List []RoleModel `json:"list"`
+ } `json:"roles"`
+}
+
+type UserRoleOptRequest struct {
+ UserIds []string `json:"userIds"`
+ RoleCodes []string `json:"roleCodes"`
+ Namespace *string `json:"namespace"`
+}
+
+type OrgModel struct {
+ RootNodeId string `json:"rootNodeId"`
+ Logo string `json:"logo"`
+ Type string `json:"type"`
+ Id string `json:"id"`
+ CreatedAt time.Time `json:"createdAt"`
+ UpdatedAt time.Time `json:"updatedAt"`
+ UserPoolId string `json:"userPoolId"`
+ OrgId string `json:"orgId"`
+ Name string `json:"name"`
+ NameI18N string `json:"nameI18n"`
+ Description string `json:"description"`
+ DescriptionI18N string `json:"descriptionI18n"`
+ Order string `json:"order"`
+ Code string `json:"code"`
+ LeaderUserId string `json:"leaderUserId"`
+ Source []string `json:"source"`
+ DataVersion string `json:"dataVersion"`
+ SourceData string `json:"sourceData"`
+}
+
+type ListUserOrgResponse struct {
+ Code string `json:"code"`
+ Message string `json:"message"`
+ Data [][]OrgModel `json:"data"`
+}
+
+type ListUserAuthResourceRequest struct {
+ Id string `json:"id"`
+ Namespace string `json:"namespace"`
+ ResourceType EnumResourceType `json:"resourceType"`
+}
+
+type GetUserTenantsResponse struct {
+ User
+ Tenants []Tenant `json:"tenants"`
+}
diff --git a/lib/model/vo_model.go b/lib/model/vo_model.go
new file mode 100644
index 0000000..5a54f32
--- /dev/null
+++ b/lib/model/vo_model.go
@@ -0,0 +1,413 @@
+package model
+
+import (
+ "github.com/Authing/authing-go-sdk/lib/constant"
+ "github.com/Authing/authing-go-sdk/lib/enum"
+ "time"
+)
+
+type ListMemberRequest struct {
+ NodeId string `json:"nodeId"`
+ Page int `json:"page"`
+ Limit int `json:"limit"`
+ SortBy enum.SortByEnum `json:"sortBy"`
+ IncludeChildrenNodes bool `json:"includeChildrenNodes"`
+}
+
+type UserDetailData struct {
+ ThirdPartyIdentity User `json:"thirdPartyIdentity"`
+}
+
+type UserDetailResponse struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data User `json:"data"`
+}
+
+type ExportAllOrganizationResponse struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data []OrgNode `json:"data"`
+}
+
+type NodeByIdDetail struct {
+ NodeById Node `json:"nodeById"`
+}
+
+type NodeByIdResponse struct {
+ Data NodeByIdDetail `json:"data"`
+}
+
+type QueryListRequest struct {
+ Page int `json:"page"`
+ Limit int `json:"limit"`
+ SortBy enum.SortByEnum `json:"sortBy"`
+ WithCustomData *bool
+}
+
+type QueryUserInfoRequest struct {
+ UserId string `json:"user_id"`
+ WithCustomData bool
+}
+
+type Users struct {
+ Users PaginatedUsers `json:"users"`
+}
+type ListUserResponse struct {
+ Data Users `json:"data"`
+}
+
+/*type OrganizationChildren struct {
+ Id string `json:"id"`
+ CreatedAt *string `json:"createdAt"`
+ UpdateAt *string `json:"updateAt"`
+ UserPoolId *string `json:"userPoolId"`
+ OrgId *string `json:"orgId"`
+ Name string `json:"name"`
+ Description *string `json:"description"`
+ Order *int64 `json:"order"`
+ Code *string `json:"code"`
+}*/
+
+type ListOrganizationResponse struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data PaginatedOrgs `json:"data"`
+}
+
+type GetOrganizationChildrenResponse struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data []Node `json:"data"`
+}
+
+type GetOrganizationByIdData struct {
+ Org Org `json:"org"`
+}
+
+type GetOrganizationByIdResponse struct {
+ Data GetOrganizationByIdData `json:"data"`
+}
+
+type ValidateTokenRequest struct {
+ AccessToken string `json:"accessToken"`
+ IdToken string `json:"idToken"`
+}
+
+type ClientCredentialInput struct {
+ AccessKey string `json:"access_key"`
+ SecretKey string `json:"secret_key"`
+}
+
+type GetAccessTokenByClientCredentialsRequest struct {
+ Scope string `json:"scope"`
+ ClientCredentialInput *ClientCredentialInput `json:"client_credential_input"`
+}
+
+type OidcParams struct {
+ AppId string
+ RedirectUri string
+ ResponseType string
+ ResponseMode string
+ State string
+ Nonce string
+ Scope string
+ CodeChallengeMethod string
+ CodeChallenge string
+}
+
+type GetUserDepartmentsRequest struct {
+ Id string `json:"id"`
+ OrgId *string `json:"orgId"`
+}
+
+type CheckUserExistsRequest struct {
+ Email *string `json:"email,omitempty"`
+ Phone *string `json:"phone,omitempty"`
+ Username *string `json:"username,omitempty"`
+ ExternalId *string `json:"externalId,omitempty"`
+}
+
+type CheckUserExistsResponse struct {
+ Message string `json:"message"`
+ Code int64 `json:"code"`
+ Data bool `json:"data"`
+}
+
+type UserDepartments struct {
+ Departments *PaginatedDepartments `json:"departments"`
+}
+
+type UserDepartmentsData struct {
+ User UserDepartments `json:"user"`
+}
+type GetUserDepartmentsResponse struct {
+ Data UserDepartmentsData `json:"data"`
+}
+
+type CommonPageRequest struct {
+ Page int `json:"page"`
+ Limit int `json:"limit"`
+}
+
+type ListPoliciesResponse struct {
+ TotalCount int `json:"totalCount"`
+ List []struct {
+ Code string `json:"code"`
+ TargetType string `json:"targetType"`
+ TargetIdentifier string `json:"targetIdentifier"`
+ } `json:"list"`
+}
+
+type ListPoliciesRequest struct {
+ Code string `json:"targetIdentifier"`
+ Page int `json:"page"`
+ Limit int `json:"limit"`
+}
+
+type ListPoliciesOnIdRequest struct {
+ Id string `json:"targetIdentifier"`
+ Page int `json:"page"`
+ Limit int `json:"limit"`
+}
+
+type ListAuthorizedResourcesByIdRequest struct {
+ Id string `json:"id"`
+ Namespace string `json:"namespace,omitempty"`
+ ResourceType *string `json:"resourceType"`
+}
+
+type ListUserAuthorizedResourcesResponse struct {
+ User User `json:"user"`
+}
+
+type IsAllowedRequest struct {
+ Resource string `json:"resource"`
+ Action string `json:"action"`
+ UserId string `json:"userId"`
+ Namespace *string `json:"namespace"`
+}
+
+type AllowRequest struct {
+ Resource string `json:"resource"`
+ Action string `json:"action"`
+ UserId string `json:"userId"`
+ Namespace string `json:"namespace"`
+}
+
+type AuthorizeResourceRequest struct {
+ Namespace string `json:"namespace"`
+ Resource string `json:"resource"`
+ ResourceType EnumResourceType `json:"resourceType"`
+ Opts []AuthorizeResourceOpt `json:"opts"`
+}
+
+type RevokeResourceRequest struct {
+ Namespace string `json:"namespace"`
+ Resource string `json:"resource"`
+ ResourceType EnumResourceType `json:"resourceType"`
+ Opts []AuthorizeResourceOpt `json:"opts"`
+}
+
+type GetUserRoleListRequest struct {
+ UserId string `json:"userId"`
+ Namespace *string `json:"namespace"`
+}
+
+type CheckResourcePermissionBatchRequest struct {
+ UserId string `json:"userId"`
+ Namespace string `json:"namespace"`
+ Resources []string `json:"resources"`
+}
+
+type GetAuthorizedResourcesOfResourceKindRequest struct {
+ UserId string `json:"userId"`
+ Namespace string `json:"namespace"`
+ Resource string `json:"resource"`
+}
+
+type ListAuthorizedResourcesRequest struct {
+ TargetIdentifier string `json:"targetIdentifier"`
+ Namespace string `json:"namespace"`
+ TargetType constant.ResourceTargetTypeEnum `json:"targetType"`
+ ResourceType *EnumResourceType `json:"resourceType"`
+}
+
+type ProgrammaticAccessAccount struct {
+ AppId string `json:"appId"`
+ Secret string `json:"secret"`
+ TokenLifetime int `json:"tokenLifetime"`
+ CreatedAt time.Time `json:"createdAt"`
+ UpdatedAt time.Time `json:"updatedAt"`
+ Id string `json:"id"`
+ Remarks string `json:"remarks"`
+ UserId string `json:"userId"`
+ Enabled bool `json:"enabled"`
+}
+
+type ListResourceRequest struct {
+ Namespace string `json:"namespace"`
+ ResourceType EnumResourceType `json:"resourceType,omitempty"`
+ Page int `json:"page"`
+ Limit int `json:"limit"`
+}
+type ActionsModel struct {
+ Name string `json:"name"`
+ Description string `json:"description"`
+}
+type Resource struct {
+ Id string `json:"id"`
+ CreatedAt time.Time `json:"createdAt"`
+ UpdatedAt time.Time `json:"updatedAt"`
+ UserPoolId string `json:"userPoolId"`
+ Code string `json:"code"`
+ Actions []ActionsModel `json:"actions"`
+ Type string `json:"type"`
+ Description string `json:"description"`
+ NamespaceId int `json:"namespaceId"`
+ ApiIdentifier *string `json:"apiIdentifier"`
+ Namespace string `json:"namespace,omitempty"`
+}
+type ResourceResponse struct {
+ Id string `json:"id"`
+ CreatedAt time.Time `json:"createdAt"`
+ UpdatedAt time.Time `json:"updatedAt"`
+ UserPoolId string `json:"userPoolId"`
+ Code string `json:"code"`
+ Actions []ActionsModel `json:"actions"`
+ Type string `json:"type"`
+ Description string `json:"description"`
+ NamespaceId int `json:"namespaceId"`
+ ApiIdentifier *string `json:"apiIdentifier"`
+}
+
+type ListNamespaceResourceResponse struct {
+ List []Resource `json:"list"`
+ TotalCount int `json:"totalCount"`
+}
+
+type CreateResourceRequest struct {
+ Code string `json:"code"`
+ Actions []ActionsModel `json:"actions,omitempty"`
+ Type string `json:"type,omitempty"`
+ Description *string `json:"description,omitempty"`
+ ApiIdentifier *string `json:"apiIdentifier,omitempty"`
+ Namespace string `json:"namespace,omitempty"`
+}
+
+type UpdateResourceRequest struct {
+ Actions []ActionsModel `json:"actions,omitempty"`
+ Type string `json:"type,omitempty"`
+ Description *string `json:"description,omitempty"`
+ ApiIdentifier *string `json:"apiIdentifier,omitempty"`
+ Namespace string `json:"namespace,omitempty"`
+}
+
+type ApplicationAccessPolicies struct {
+ AssignedAt time.Time `json:"assignedAt"`
+ InheritByChildren interface{} `json:"inheritByChildren"`
+ Enabled bool `json:"enabled"`
+ PolicyId string `json:"policyId"`
+ Code string `json:"code"`
+ Policy struct {
+ Id string `json:"id"`
+ CreatedAt time.Time `json:"createdAt"`
+ UpdatedAt time.Time `json:"updatedAt"`
+ UserPoolId string `json:"userPoolId"`
+ IsDefault bool `json:"isDefault"`
+ IsAuto bool `json:"isAuto"`
+ Hidden bool `json:"hidden"`
+ Code string `json:"code"`
+ Description string `json:"description"`
+ Statements []struct {
+ Resource string `json:"resource"`
+ Actions []string `json:"actions"`
+ Effect string `json:"effect"`
+ Condition []interface{} `json:"condition"`
+ ResourceType EnumResourceType `json:"resourceType"`
+ } `json:"statements"`
+ NamespaceId int `json:"namespaceId"`
+ } `json:"policy"`
+ TargetNamespace string `json:"targetNamespace"`
+ TargetType string `json:"targetType"`
+ TargetIdentifier string `json:"targetIdentifier"`
+ Target struct {
+ Id string `json:"id"`
+ CreatedAt time.Time `json:"createdAt"`
+ UpdatedAt time.Time `json:"updatedAt"`
+ UserPoolId string `json:"userPoolId"`
+ Code string `json:"code"`
+ Description string `json:"description"`
+ ParentCode string `json:"parentCode"`
+ IsSystem bool `json:"isSystem"`
+ NamespaceId int `json:"namespaceId"`
+ } `json:"target"`
+ Namespace string `json:"namespace"`
+}
+
+type GetApplicationAccessPoliciesResponse struct {
+ List []ApplicationAccessPolicies `json:"list"`
+ TotalCount int `json:"totalCount"`
+}
+
+type ApplicationAccessPoliciesRequest struct {
+ TargetIdentifiers []string `json:"targetIdentifiers,omitempty"`
+ TargetType constant.ResourceTargetTypeEnum `json:"targetType,omitempty"`
+ Namespace string `json:"namespace,omitempty"`
+ InheritByChildren bool `json:"inheritByChildren,omitempty"`
+}
+
+type GetAuthorizedTargetsRequest struct {
+ TargetType constant.ResourceTargetTypeEnum `json:"targetType"`
+ Namespace string `json:"namespace"`
+ Resource string `json:"resource"`
+ ResourceType EnumResourceType `json:"resourceType"`
+ Actions *struct {
+ Op constant.GetAuthorizedTargetsOpt `json:"op,omitempty"`
+ List []string `json:"list,omitempty"`
+ } `json:"actions,omitempty"`
+}
+
+type ListAuditLogsRequest struct {
+ ClientIp *string `json:"clientip,omitempty"`
+ OperationNames *[]string `json:"operation_name,omitempty"`
+ UserIds *[]string `json:"operator_arn,omitempty"`
+ AppIds *[]string `json:"app_id,omitempty"`
+ Page *int `json:"page,omitempty"`
+ Limit *int `json:"limit,omitempty"`
+}
+
+type ListUserActionRequest struct {
+ ClientIp *string `json:"clientip,omitempty"`
+ OperationNames *[]string `json:"operation_name,omitempty"`
+ UserIds *[]string `json:"operator_arn,omitempty"`
+ Page *int `json:"page,omitempty"`
+ Limit *int `json:"limit,omitempty"`
+}
+
+type CheckLoginStatusResponse struct {
+ Code int `json:"code"`
+ Message string `json:"message"`
+ Status bool `json:"status"`
+ Exp int `json:"exp"`
+ Iat int `json:"iat"`
+ Data struct {
+ Id string `json:"id"`
+ UserPoolId string `json:"userPoolId"`
+ Arn string `json:"arn"`
+ } `json:"data"`
+}
+
+type SetUdfInput struct {
+ TargetType EnumUDFTargetType `json:"targetType"`
+ Key string `json:"key"`
+ DataType EnumUDFDataType `json:"dataType"`
+ Label string `json:"label"`
+}
+
+type PrincipalAuthenticateRequest struct {
+ Type constant.PrincipalAuthenticateType `json:"type"`
+ Name string `json:"name"`
+ IdCard string `json:"idCard"`
+ Ext string `json:"ext"`
+}
diff --git a/lib/util/cacheutil/cache_token.go b/lib/util/cacheutil/cache_token.go
new file mode 100644
index 0000000..2a64547
--- /dev/null
+++ b/lib/util/cacheutil/cache_token.go
@@ -0,0 +1,2 @@
+package cacheutil
+
diff --git a/util/cache/cache_util.go b/lib/util/cacheutil/cache_util.go
similarity index 89%
rename from util/cache/cache_util.go
rename to lib/util/cacheutil/cache_util.go
index 8b4dace..94601de 100644
--- a/util/cache/cache_util.go
+++ b/lib/util/cacheutil/cache_util.go
@@ -1,4 +1,4 @@
-package cache
+package cacheutil
import (
"github.com/patrickmn/go-cache"
@@ -31,6 +31,6 @@ func AddCache(k string, x interface{}, d time.Duration) {
}
// IncrementIntCache 对已存在的key 值自增n
-func IncrementIntCache(k string, n int) (num int, err error) {
+func IncrementIntCache(k string, n int) (num int, err error){
return cacheAdapter.IncrementInt(k, n)
-}
+}
\ No newline at end of file
diff --git a/lib/util/http_utils.go b/lib/util/http_utils.go
new file mode 100644
index 0000000..c7d8682
--- /dev/null
+++ b/lib/util/http_utils.go
@@ -0,0 +1 @@
+package util
diff --git a/lib/util/rsa_utils.go b/lib/util/rsa_utils.go
new file mode 100644
index 0000000..c201d87
--- /dev/null
+++ b/lib/util/rsa_utils.go
@@ -0,0 +1,29 @@
+package util
+
+import (
+ "crypto/rand"
+ "crypto/rsa"
+ "crypto/x509"
+ "encoding/base64"
+ "github.com/Authing/authing-go-sdk/lib/constant"
+)
+
+func RsaEncrypt(plainText string) string {
+ //pem解码
+ //block, _ := pem.Decode([]byte(constant.PublicKey))
+ block, _ := base64.StdEncoding.DecodeString(constant.PublicKey)
+ //x509解码
+ publicKeyInterface, err := x509.ParsePKIXPublicKey(block)
+ if err != nil {
+ panic(err)
+ }
+ //类型断言
+ publicKey := publicKeyInterface.(*rsa.PublicKey)
+ //对明文进行加密
+ cipherText, err := rsa.EncryptPKCS1v15(rand.Reader, publicKey, []byte(plainText))
+ if err != nil {
+ panic(err)
+ }
+ //返回密文
+ return base64.StdEncoding.EncodeToString(cipherText)
+}
diff --git a/lib/util/string_utils.go b/lib/util/string_utils.go
new file mode 100644
index 0000000..3fbec7e
--- /dev/null
+++ b/lib/util/string_utils.go
@@ -0,0 +1,28 @@
+package util
+
+import (
+ "github.com/Authing/authing-go-sdk/lib/constant"
+ "math/rand"
+)
+
+var letters = []rune("abcdefhijkmnprstwxyz2345678")
+
+func RandomString(length int) string {
+ b := make([]rune, length)
+ for i := range b {
+ b[i] = letters[rand.Intn(len(letters))]
+ }
+ return string(b)
+}
+
+func GetValidValue(value ...string) string {
+ if value == nil || len(value) == 0 {
+ return constant.StringEmpty
+ }
+ for _, val := range value {
+ if val != "" {
+ return val
+ }
+ }
+ return constant.StringEmpty
+}
diff --git a/lib/util/url_utils.go b/lib/util/url_utils.go
new file mode 100644
index 0000000..0fe8f48
--- /dev/null
+++ b/lib/util/url_utils.go
@@ -0,0 +1,20 @@
+package util
+
+import (
+ "github.com/Authing/authing-go-sdk/lib/constant"
+ "net/url"
+)
+
+func GetQueryString(queryMap map[string]string) string {
+ if queryMap == nil || len(queryMap) == 0 {
+ return constant.StringEmpty
+ }
+ queryValue := url.Values{}
+ for key, value := range queryMap {
+ if value == "" {
+ continue
+ }
+ queryValue.Add(key, value)
+ }
+ return queryValue.Encode()
+}
diff --git a/util/cache/json_util.go b/util/cache/json_util.go
deleted file mode 100644
index ca77033..0000000
--- a/util/cache/json_util.go
+++ /dev/null
@@ -1,5 +0,0 @@
-package cache
-
-func CreateErrorResponse(response interface{}) {
-
-}
\ No newline at end of file